The `interp1` function in MATLAB is used for 1-D interpolation of data points, allowing you to estimate values at intermediate points between known data points.
Here's a simple code snippet using `interp1`:
% Known data points
x = [1, 2, 3, 4, 5];
y = [10, 20, 30, 25, 15];
% Interpolating at new points
xi = 1:0.5:5; % New points
yi = interp1(x, y, xi, 'linear'); % Linear interpolation
% Displaying the results
disp([xi; yi]);
Understanding the Basics of Interpolation
Interpolation is the process of estimating unknown values that fall within the range of known data points. The interp1 function in MATLAB is a powerful tool for conducting one-dimensional interpolation. This method serves as a bridge to fill in gaps in datasets, making it invaluable in data analysis, signal processing, and various scientific applications.
In terms of application, interp1 can be used in numerous fields, including engineering, finance, and environmental science. It allows users to work with incomplete datasets and still derive meaningful conclusions from their analysis.
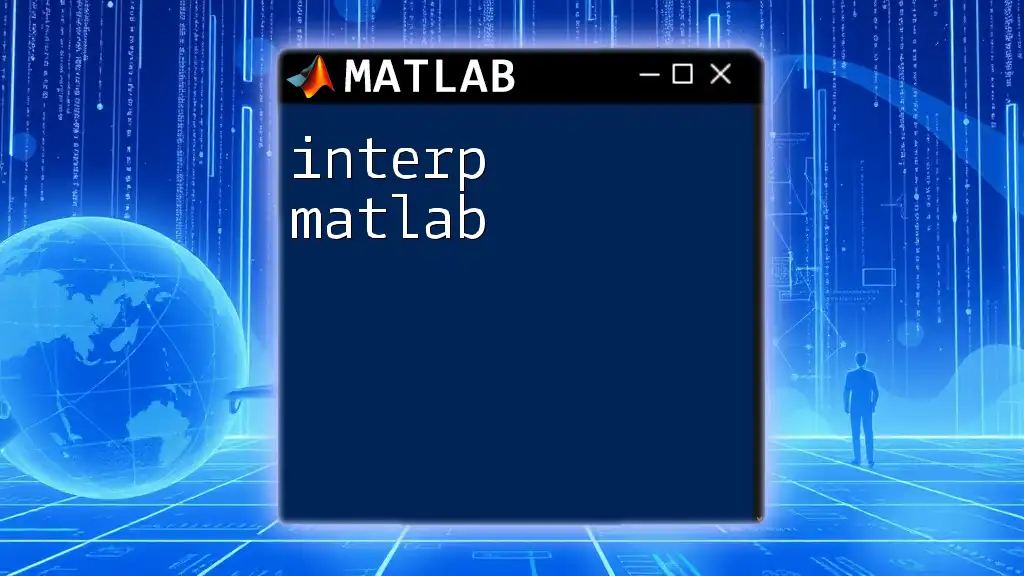
Syntax of interp1
The basic syntax for using the interp1 function in MATLAB is as follows:
Yq = interp1(X, Y, Xq)
Input Parameters Explained
- X: This vector contains the x-coordinates of the known data points. The values in X must be sorted in ascending order.
- Y: This vector holds the corresponding y-coordinates for the x-coordinates specified in X. Each element in Y maps to the respective element in X.
- Xq: The vector of query points where you wish to estimate y-values. This can contain values that fall within or outside the range of X.
Additionally, interp1 allows optional parameters to customize the interpolation method:
- Method: You can specify different interpolation techniques, such as 'linear', 'nearest', 'spline', or 'cubic'.
- Extrapolation: Use the 'extrap' option if you want to extend the interpolation to points outside the known data range.
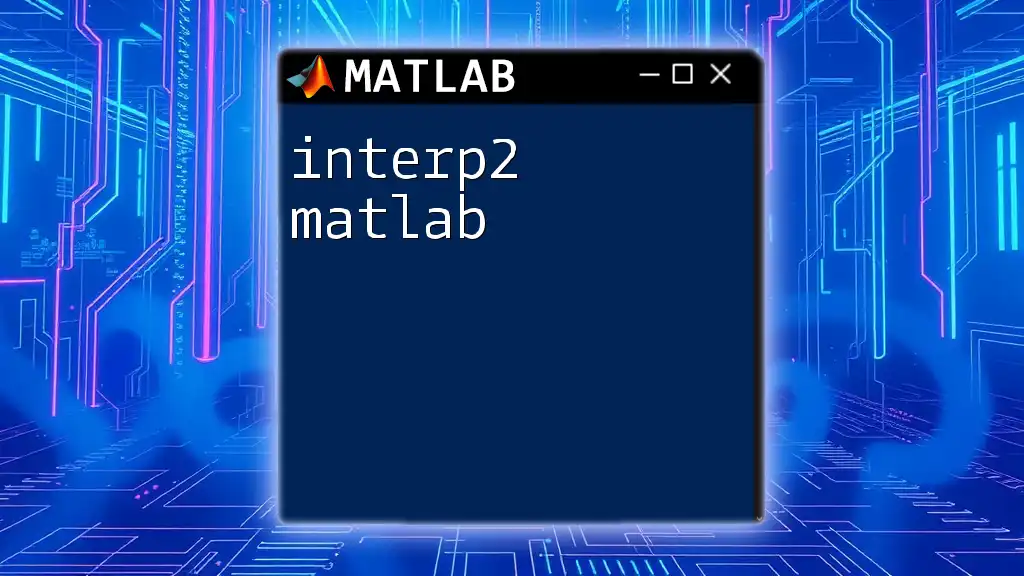
Different Interpolation Methods in interp1
Linear Interpolation
Linear interpolation connects data points with straight lines, providing a simple yet effective estimation method. This method is widely used for its ease of implementation and speed.
For example:
x = [1 2 3 4 5];
y = [10 20 30 40 50];
xq = [1.5 2.5 3.5];
Yq = interp1(x, y, xq, 'linear');
In this code, the function interpolates y-values at x-coordinates of 1.5, 2.5, and 3.5.
Nearest-neighbor Interpolation
Nearest-neighbor interpolation assigns the value of the nearest neighbor without averaging nearby points. This can be particularly useful in categorical data scenarios, where averaging may not make sense.
x = [1 2 3 4 5];
y = [10 20 30 40 50];
xq = [1.7 2.7 3.7];
Yq = interp1(x, y, xq, 'nearest');
Cubic Interpolation
Cubic interpolation often yields smoother curves than linear interpolation by fitting cubic polynomials between each pair of points.
Example:
x = [1 2 3 4 5];
y = [10 20 30 40 50];
xq = [1.5 2.5 3.5];
Yq = interp1(x, y, xq, 'pchip');
Here, 'pchip' is a specific cubic interpolation method that preserves shape and local extrema.
Spline Interpolation
Spline interpolation adds even more smoothness by using piecewise polynomials. It creates a continuous function that is often used in graphics and data visualization.
x = [1 2 3 4 5];
y = [10 20 30 40 50];
xq = [1.5 2.5 3.5];
Yq = interp1(x, y, xq, 'spline');
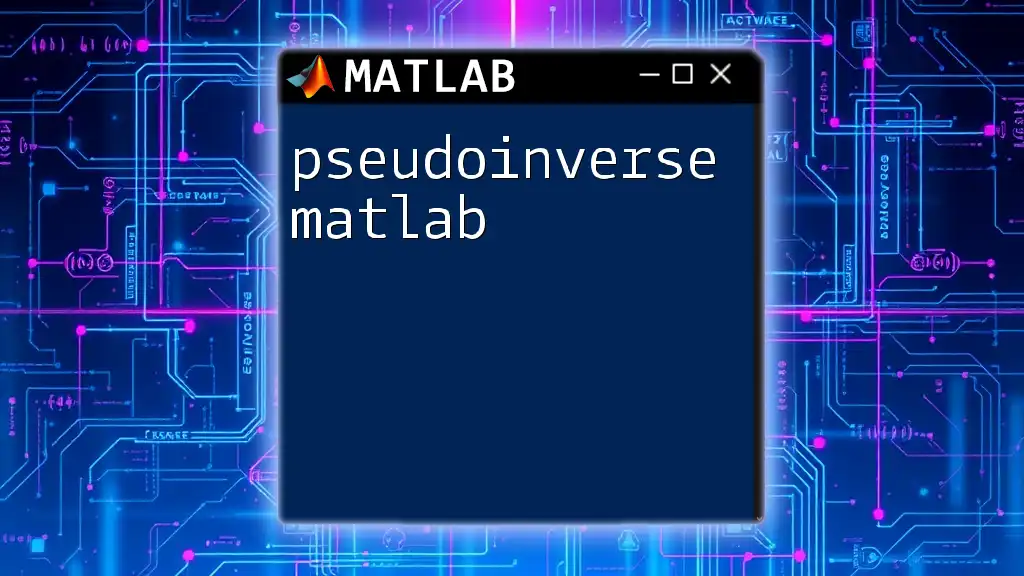
Interpolation with Extrapolation
Understanding Extrapolation
Extrapolation extends the estimation beyond the known data points. This can be risky and lead to inaccuracies, especially if the trends of known data do not hold outside the observed range.
Using interp1 to Extrapolate
To enable extrapolation, you can specify the 'extrap' option:
x = [1 2 3 4 5];
y = [10 20 30 40 50];
xq = [0.5 5.5];
Yq = interp1(x, y, xq, 'linear', 'extrap');
In this case, interp1 calculates y-values for x-coordinates of 0.5 and 5.5 that are outside the specified x-range.
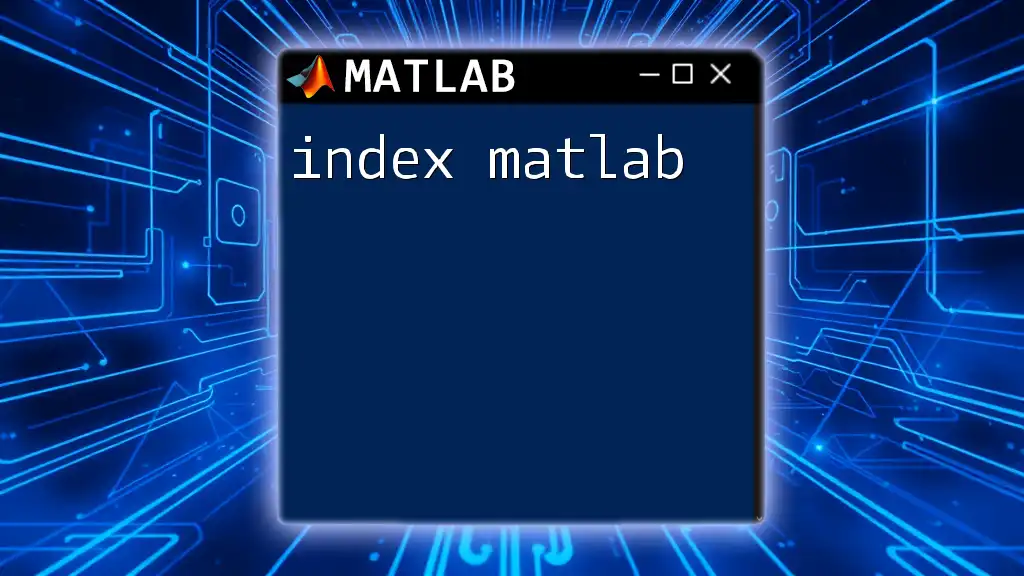
Handling Missing Data
Encountering NaN (Not a Number) values in datasets is common, and interp1 can handle such scenarios effectively.
Dealing with NaNs in Input Data
If your dataset has NaN values in Y, interp1 automatically ignores those corresponding x-values. If you're working with time-series data, clean your data before interpolation:
x = [1 2 3 NaN 5];
y = [10 NaN 30 40 50];
x = x(~isnan(y)); % Remove NaN entries
y = y(~isnan(y));
xq = [1.5 2.5 3.5];
Yq = interp1(x, y, xq, 'linear');
This code snippet illustrates how to clean your dataset by removing NaNs before proceeding with interpolation.
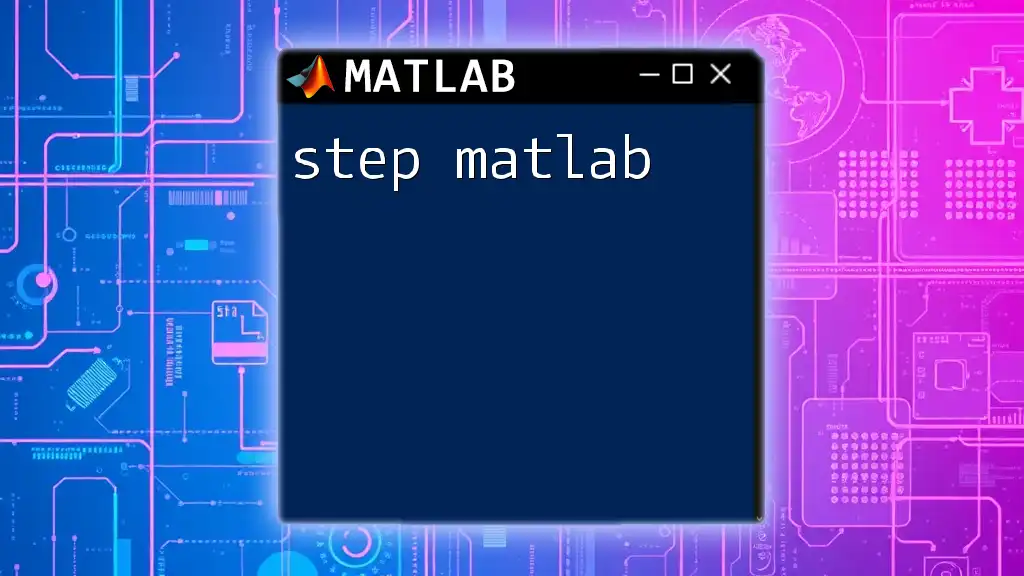
Practical Applications of interp1
Real-World Examples
- Engineering: In structural engineering, interpolate stress-strain data to predict material behavior under various conditions.
- Finance: Use interp1 for estimating asset prices between recorded data points.
- Environmental Science: Interpolate temperature or pollutant measurements across geographic locations for better analysis.
Case Study
Imagine a scenario where you're analyzing climate data. With temperature measurements taken at discrete intervals, use interp1 to estimate temperatures for undisclosed intervals to model trends accurately.
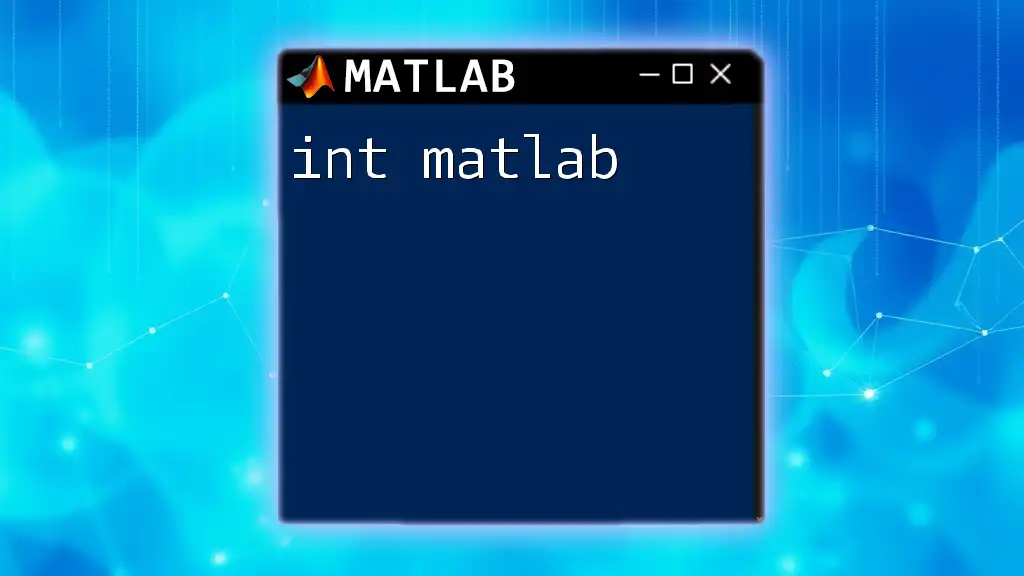
Optimizing interp1 Performance
Speeding Up Interpolation
For large datasets, computation speed can become an issue. Here are some best practices to maximize performance when using interp1:
- Reduce the size of your dataset by sampling or aggregation if possible.
- Consider using alternative functions like `griddatan` or `scatteredInterpolant` for multidimensional datasets, as these may offer better performance.
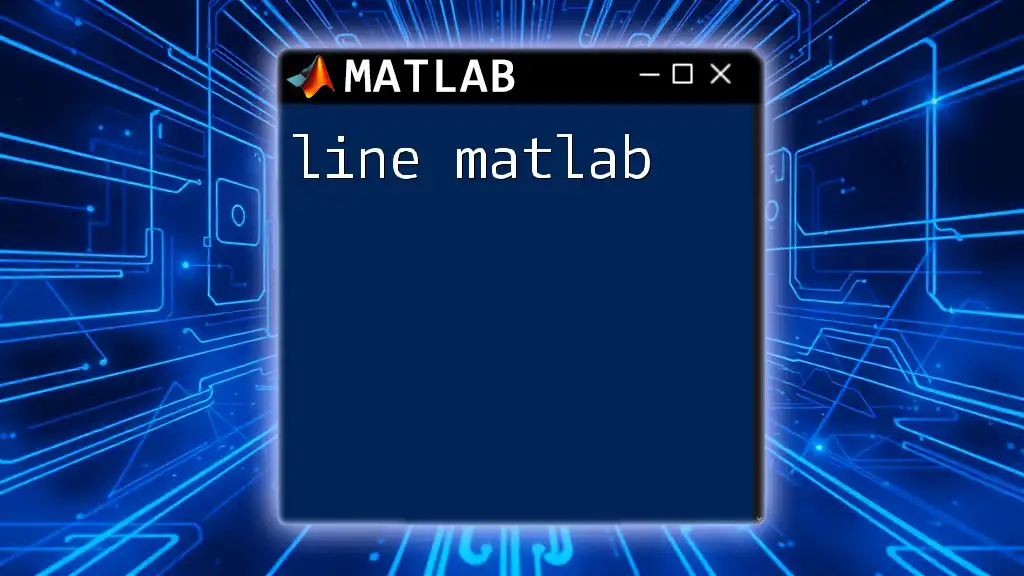
Common Pitfalls and Troubleshooting
Common Errors and Solutions in interp1
While using interp1, you may encounter several common pitfalls, such as:
- Out-of-bounds Queries: Make sure your query points fall within the range of x. Always check your data before interpolation.
- Sorting Errors: Ensure that your x vector is sorted; otherwise, interp1 will not work as expected. Use `sort` to arrange your data if necessary:
[x_sorted, idx] = sort(x); y_sorted = y(idx);
By understanding these errors and actively troubleshooting them, you'll become proficient in using interp1 effectively.
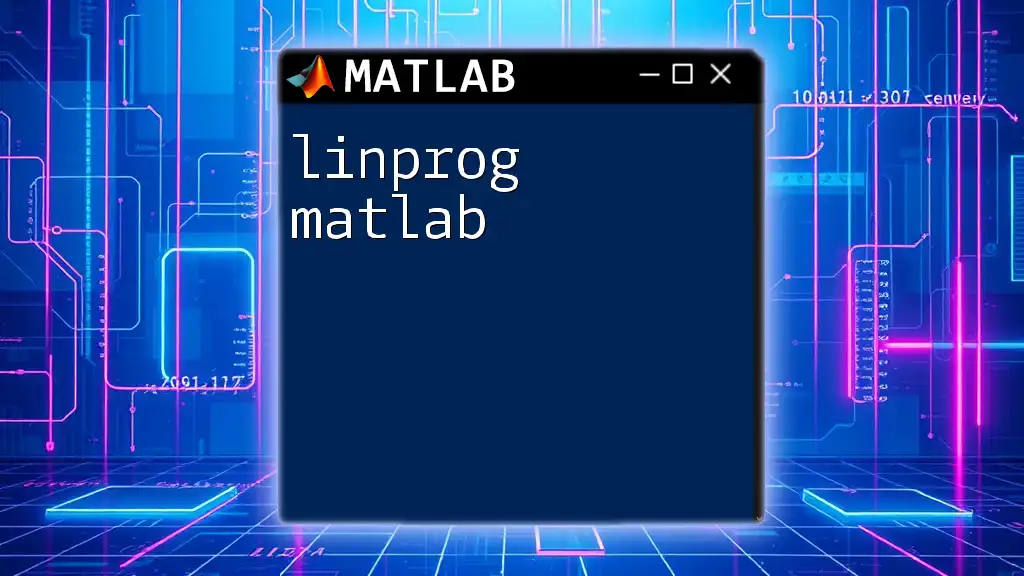
Conclusion
In this article, we explored the intricacies of interp1 MATLAB, a fundamental tool for one-dimensional interpolation. Covering syntax, various interpolation methods, extrapolation techniques, practical applications, and troubleshooting strategies allowed us to dive deep into its capabilities.
As you practice and experiment with interp1 in your projects, you will uncover the vast potential of MATLAB in producing reliable data analyses. Don’t hesitate to explore further resources to enhance your knowledge and skills!