Interpolation in MATLAB is a technique used to estimate unknown values that fall between known data points, and can be easily implemented using functions like `interp1`, `interp2`, or `interp3` for one-dimensional, two-dimensional, and three-dimensional data, respectively.
Here's a simple example using `interp1`:
% Sample data points
x = [1, 2, 3, 4, 5];
y = [2, 4, 6, 8, 10];
% Interpolation
xq = 2.5; % Query point for interpolation
yq = interp1(x, y, xq); % Interpolated value at xq
disp(yq); % Output: 5
Understanding Interpolation
What is Interpolation?
Interpolation is a mathematical technique used to estimate unknown values that fall within the range of known data points. By leveraging the relationships between these known values, interpolation enables us to create a continuous function that approximates the behavior of the data. In practical applications, interpolation is often used in engineering, data analysis, and computer graphics to fill missing data, smooth curves, or generate new data points for modeling.
Types of Interpolation
Linear Interpolation
Linear interpolation is the simplest form of interpolation, where a straight line is drawn between two known points. This method is quick and efficient, making it ideal for datasets where the relationship between the points is approximately linear. The formula for linear interpolation is given by:
\[ y = y_0 + \frac{(x - x_0)(y_1 - y_0)}{(x_1 - x_0)} \]
In this case, \( (x_0, y_0) \) and \( (x_1, y_1) \) are the known points, and \( x \) is the point at which you want to estimate \( y \).
Polynomial Interpolation
Polynomial interpolation uses a polynomial function to estimate values based on known data points. This method can achieve higher accuracy compared to linear interpolation but may introduce complexity as the degree of the polynomial increases. One important consideration when using polynomial interpolation is avoiding Runge's phenomenon, where high-degree polynomials behave unpredictably outside their interpolation range.
Spline Interpolation
Spline interpolation employs piecewise polynomials to connect the known data points smoothly. The most commonly used splines are cubic splines, which ensure both function value and first derivatives are continuous at the data points. Spline interpolation is especially useful when working with datasets that exhibit complex behaviors or when one needs to avoid the oscillations that can result from high-degree polynomial fits.
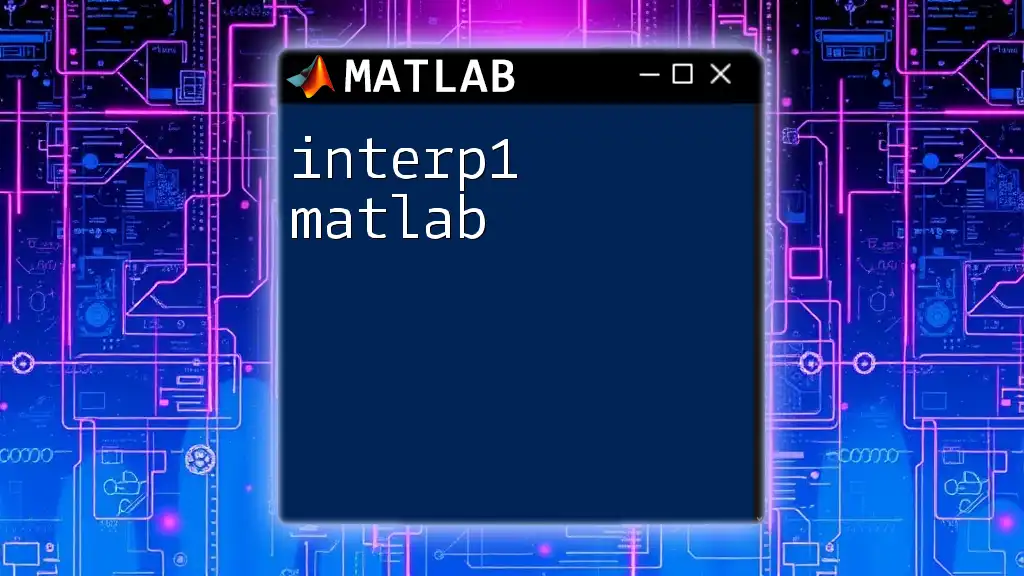
MATLAB Interpolation Functions
Built-in Interpolation Functions
interp1: 1-D Interpolation
MATLAB provides a convenient function called `interp1` for one-dimensional interpolation. The basic syntax is:
vq = interp1(x, v, xq, method)
Here, `x` contains the known data points, `v` is the corresponding values, `xq` holds the points where you want to interpolate, and `method` specifies the type of interpolation (e.g., 'linear', 'spline').
Example Usage: Suppose you have the following datasets:
x = [1, 2, 3, 4, 5];
y = [2, 4, 6, 8, 10];
new_x = 2.5;
interpolated_value = interp1(x, y, new_x, 'linear');
This code will return the interpolated value for \( x = 2.5 \).
interp2: 2-D Interpolation
For two-dimensional data, the `interp2` function can be used. It allows you to perform interpolation on grid data. This is particularly useful in applications like image processing or surface fitting.
interp3: 3-D Interpolation
`interp3` extends the concept of interpolation to three dimensions, useful for analyzing volumetric data, such as medical imaging or 3D simulations.
griddatan: N-D Interpolation
The `griddatan` function is suited for multidimensional scattered data points, making it a powerful tool in various applications where data does not conform to a regular grid.
Choosing the Right Interpolation Method
When deciding on the most appropriate interpolation function, consider the following:
- Data Characteristics: Assess the nature of your data (linear, nonlinear, smooth) to choose between linear, polynomial, or spline methods.
- Accuracy vs. Complexity: More complex models like high-degree polynomials may fit the data better but increase the risk of overfitting.
- Computational Efficiency: Simpler methods are often faster. Depending on your dataset's size and the required accuracy, choose accordingly.
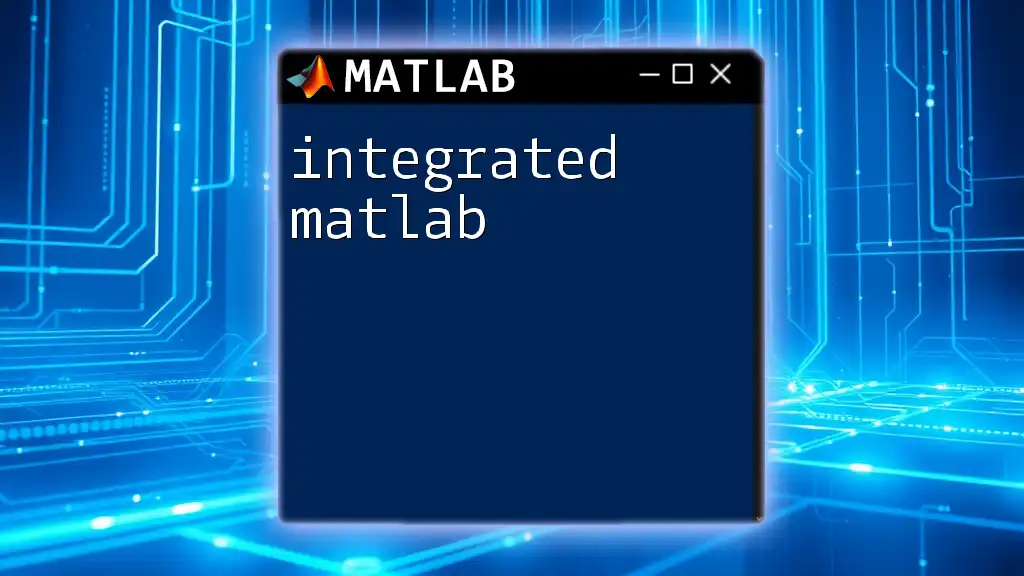
How to Implement Interpolation in MATLAB
Step-by-Step Guide to Using interp1
To implement `interp1`, follow these steps:
- Import Data: Start by importing your data vectors \( x \) and \( y \) into MATLAB.
- Execution: Use the `interp1` function by specifying your query points.
- Output: Capture the interpolated values in a new variable.
Example with a Real Dataset: Imagine you have temperature readings at different hours:
hours = [0, 1, 2, 3, 4, 5];
temperatures = [30, 32, 35, 34, 33, 32];
query_hours = 1.5;
interpolated_temperature = interp1(hours, temperatures, query_hours, 'linear');
Example Code for Multiple Interpolation Methods
To compare linear, spline, and nearest neighbor interpolations using the same dataset, you can use the following MATLAB code:
x = [1, 2, 3, 4, 5];
y = [2, 4, 6, 8, 10];
xq = linspace(1, 5, 100); % Creating a finer grid
% Linear Interpolation
yq_linear = interp1(x, y, xq, 'linear');
% Spline Interpolation
yq_spline = interp1(x, y, xq, 'spline');
% Nearest Neighbor Interpolation
yq_nearest = interp1(x, y, xq, 'nearest');
Visualizing Interpolation Results
Visualizing the results enhances your understanding of how well your interpolation method performs. Use MATLAB’s plotting functions to display the interpolated results alongside the original data points.
figure;
plot(x, y, 'o', xq, yq_linear, '-r', ...
xq, yq_spline, '-g', ...
xq, yq_nearest, '-b');
legend('Data Points', 'Linear', 'Spline', 'Nearest');
title('Interpolation Methods Comparison');
grid on;
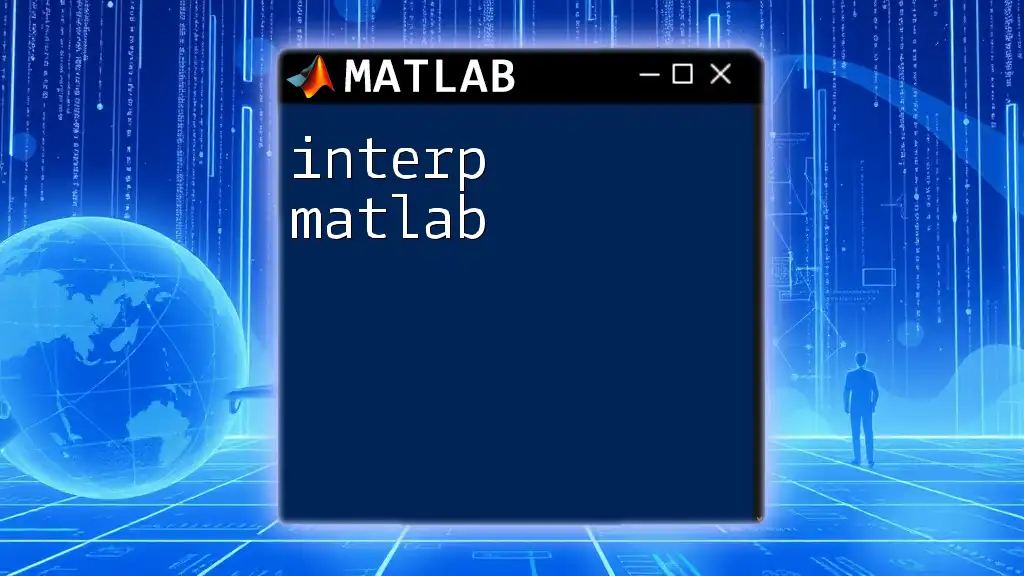
Advanced Interpolation Techniques
Multi-dimensional Interpolation
In cases where you have two-dimensional data, such as temperature vs. pressure, you can utilize the `interp2` function to perform interpolation efficiently. The use of surface plots can also reveal the behavior of your interpolated surface.
Handling Data Gaps
When encountering missing data, interpolation can help fill these gaps. MATLAB features functions like `fillmissing`, allowing you to specify interpolation methods to estimate these lost values.
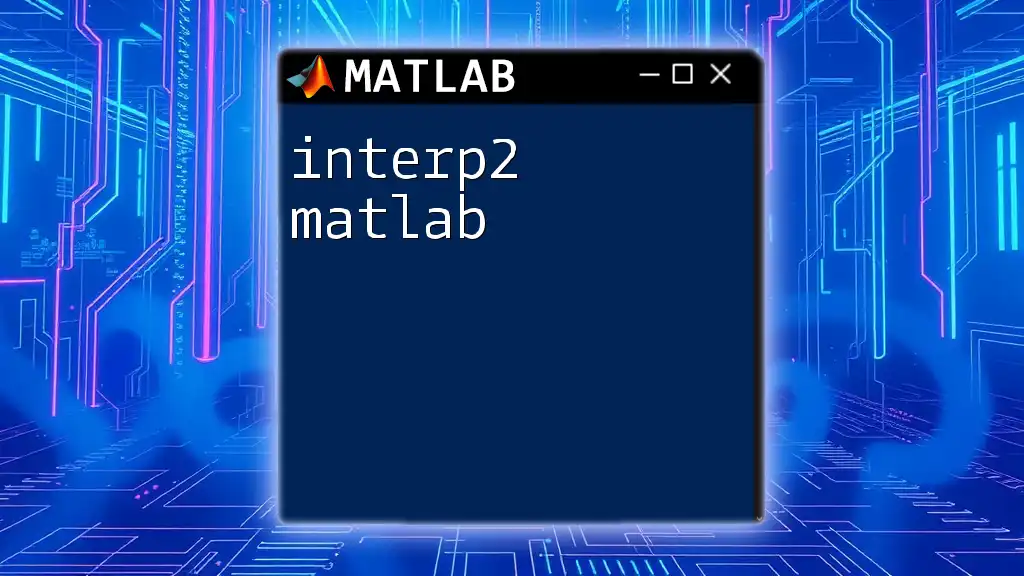
Best Practices in Interpolation
Avoiding Common Pitfalls
Be cautious of overfitting when using high-degree polynomials for interpolation. These can result in oscillations and inaccuracies outside the bounds of the known data. Always evaluate the method against real-world scenarios and understand its limitations.
Performance Optimization
For large datasets, computational performance can be crucial. Using vectorized operations rather than loops can significantly speed up calculations. Additionally, ensure that your data is pre-processed efficiently to avoid redundancy in computation.
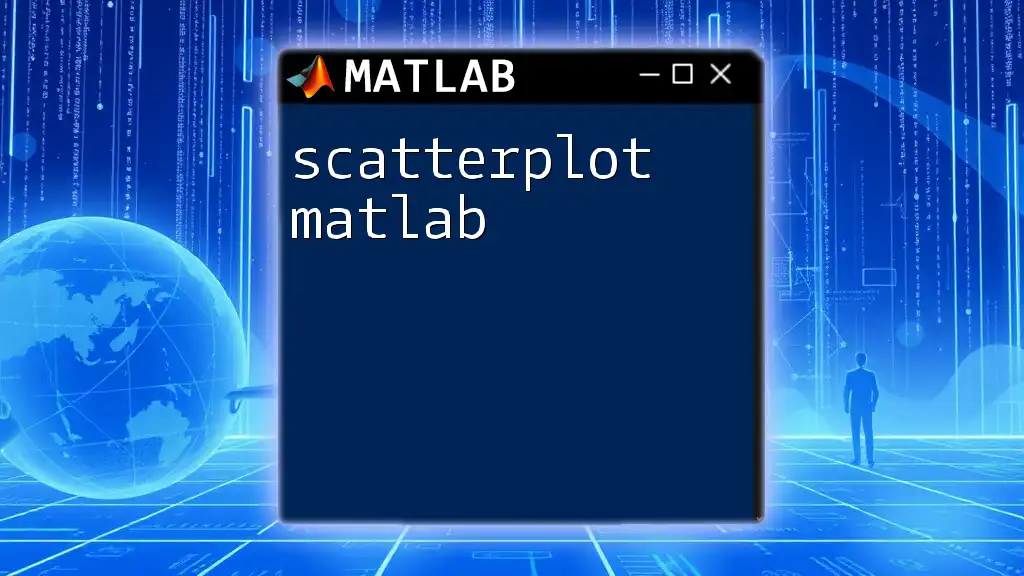
Conclusion
Interpolation is a powerful tool in MATLAB that can significantly enhance your data analysis capabilities. By mastering various interpolation methods, you can not only approximate unknown values effectively but also ensure data integrity in your analysis. Through practice and exploration, you will become proficient in utilizing MATLAB for all your interpolation needs.
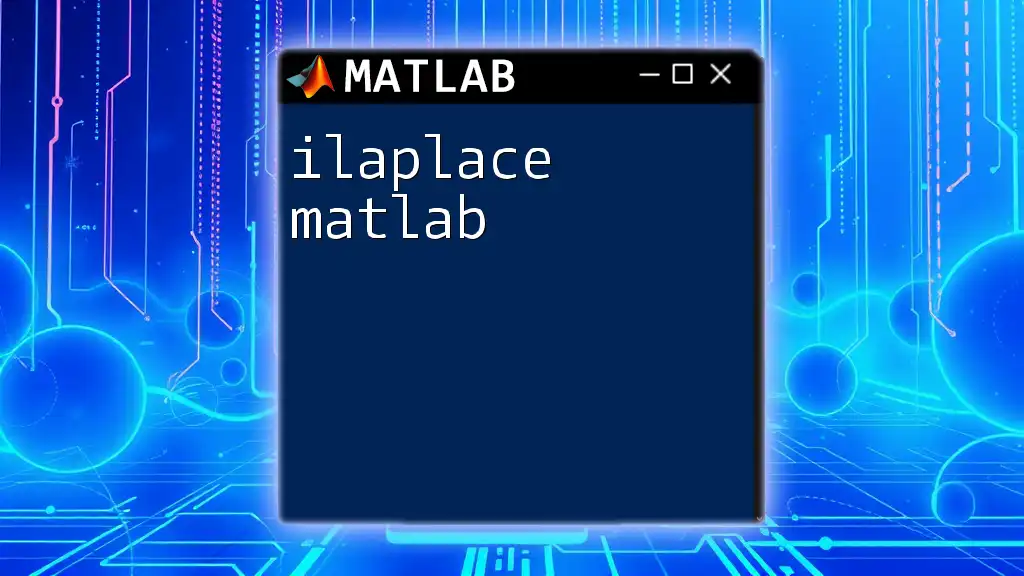
Additional Resources
To deepen your understanding of interpolation methods and their applications in MATLAB, consider exploring the official MATLAB documentation and additional recommended readings available online.