The inverse tangent function in MATLAB, referred to as `atan`, computes the angle in radians whose tangent is a given value.
Here's a code snippet demonstrating its usage:
% Calculate the inverse tangent of a value
value = 1; % Example value
angle_radians = atan(value); % Compute the inverse tangent
disp(angle_radians); % Display the result
What is Inverse Tangent?
Definition
The inverse tangent function, often represented as \(\tan^{-1}\) or atan, is a fundamental mathematical function used to determine the angle whose tangent is a given number. In simpler terms, when you input a value into the inverse tangent function, you receive an angle in return. This angle is crucial in various fields including engineering, physics, and computer graphics.
Properties of Inverse Tangent
The inverse tangent function possesses several key characteristics:
- Range: The output of the \(\text{atan}\) function is restricted to the interval \(-\frac{\pi}{2} < y < \frac{\pi}{2}\) (or approximately -1.57 to 1.57 radians).
- Domain: The function accepts any real number as input.
- Monotonicity: The function is monotonically increasing, meaning as the input increases, the output also increases.
- Asymptotic Behavior: The outputs approach \(-\frac{\pi}{2}\) as the input approaches negative infinity, and approach \(\frac{\pi}{2}\) as the input approaches positive infinity.
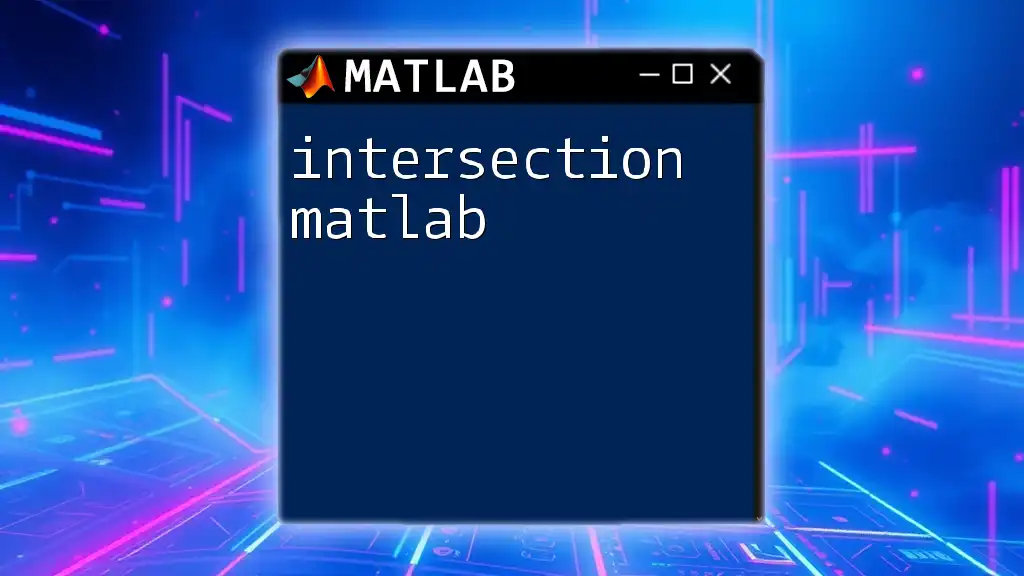
Using the `atan` Function in Matlab
Basic Syntax of `atan`
In Matlab, the atan function is used to compute the inverse tangent. The syntax is straightforward:
y = atan(x)
Here, `y` is the output (the angle in radians), and `x` is the input (the tangent value).
Example: Simple Usage
Consider the simplest case of calculating the angle whose tangent is 1:
result = atan(1);
disp(result);
When you execute this code, the output will be \(0.7854\), which is equivalent to \(\frac{\pi}{4}\) radians or \(45\) degrees. This illustrates how atan provides angles in radians, a point that is fundamental for effective use of this function.
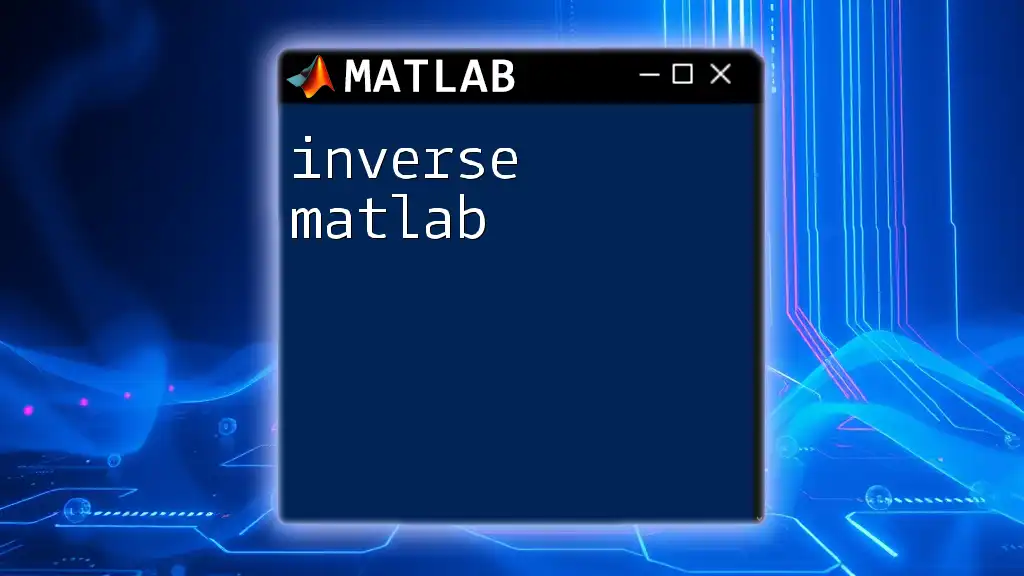
Understanding the Output
Radian vs Degree
The output produced by the atan function is in radians, which can sometimes confuse new users. To convert radians into degrees, Matlab provides a convenient function called rad2deg. Here is how you can convert the output:
degrees = rad2deg(result);
disp(degrees);
Running this snippet will yield \(45\) degrees, reinforcing your understanding of the conversion process.
Practical Use Cases
Understanding the inverse tangent function has several practical implications. For example, in engineering, it can be used to calculate angles in mechanisms and electrical circuits. In computer graphics, it can assist in determining angles for rendering images or 3D objects accurately.
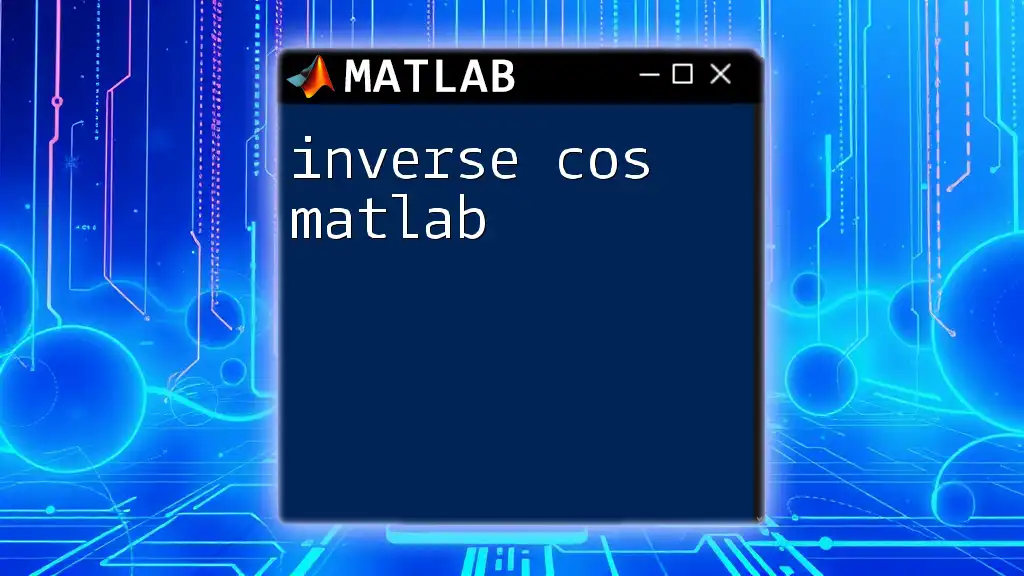
Related Functions in Matlab
`atan2` Function
In addition to the atan function, Matlab provides another related function called atan2. The syntax for this function is:
y = atan2(y, x)
This function takes two inputs: `y` and `x`, and returns the angle whose tangent is the quotient of the two arguments. One significant advantage of atan2 over atan is that it considers the signs of both arguments to determine the correct quadrant for the angle.
Comparison of Outputs
Consider the following example where both functions are utilized:
y = 2;
x = 2;
angle_atan = atan(y/x);
angle_atan2 = atan2(y, x);
disp(angle_atan);
disp(angle_atan2);
While both will provide a similar angle in this case, atan2 offers a more robust solution when dealing with inputs located in different quadrants, preventing potential errors in angle calculation.
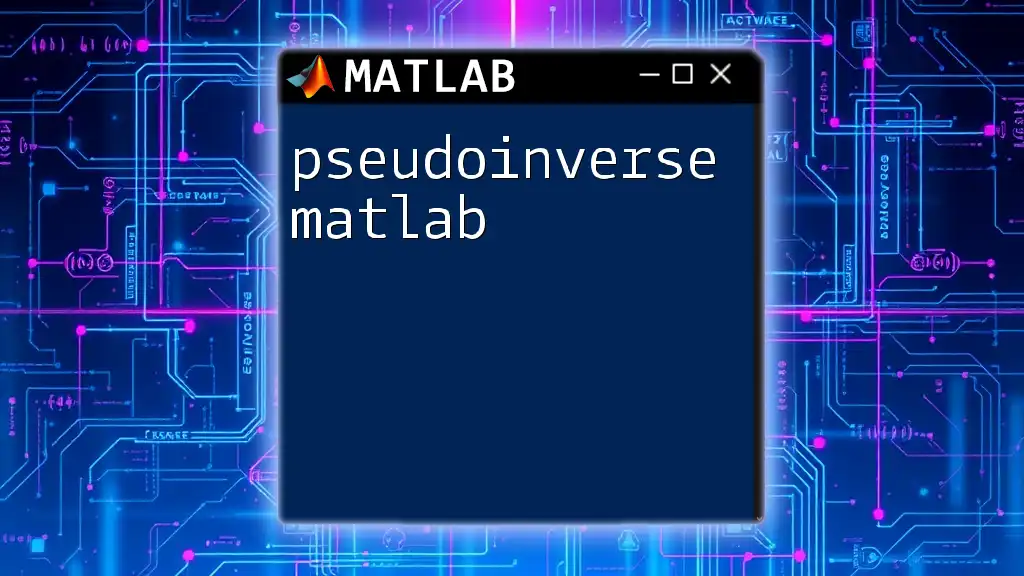
Visualization of the Inverse Tangent Function
Plotting `atan` in Matlab
Visual representation offers a deeper insight into how the inverse tangent function behaves. By plotting atan in Matlab, you can create a visual understanding of the function:
x = -10:0.1:10; % Define the range for x
y = atan(x); % Calculate inverse tangent for each x value
plot(x, y);
title('Plot of the Inverse Tangent Function');
xlabel('x');
ylabel('atan(x)');
grid on;
Executing the above code creates a graph illustrating the input-output relationship of the inverse tangent function.
Interpreting the Plot
The plot showcases the smooth curve of the inverse tangent function, sharply illustrating its asymptotic behavior as it approaches \(-\frac{\pi}{2}\) and \(\frac{\pi}{2}\). Key points on the graph further clarify how the function behaves at different ranges of input, providing valuable insights for applications.
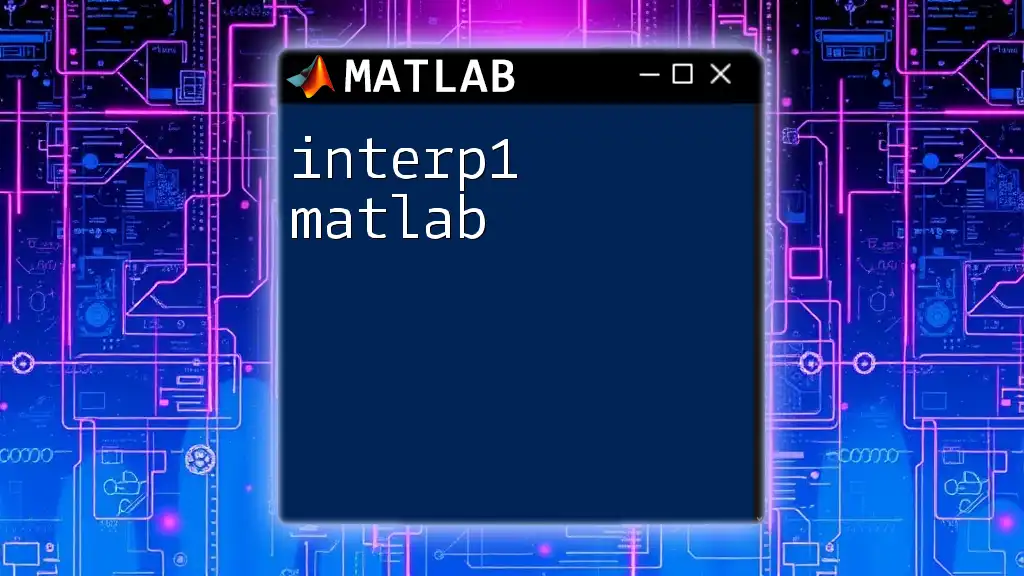
Common Errors and Troubleshooting
Mistakes When Using `atan`
Users often run into pitfalls when using the atan function, such as forgetting to convert radians to degrees or misinterpreting the behavior with negative inputs. Developing a familiarity with these aspects can enhance your usage of the function significantly.
Handling Complex Inputs
An interesting feature of the atan function is its capability to process complex numbers. Here’s how you can use it:
complexInput = 1 + 1i;
result = atan(complexInput);
disp(result);
This example calculates the inverse tangent of a complex number, illustrating the flexibility of the atan function. Understanding the behavior of complex inputs is essential for users dealing with advanced mathematical concepts.
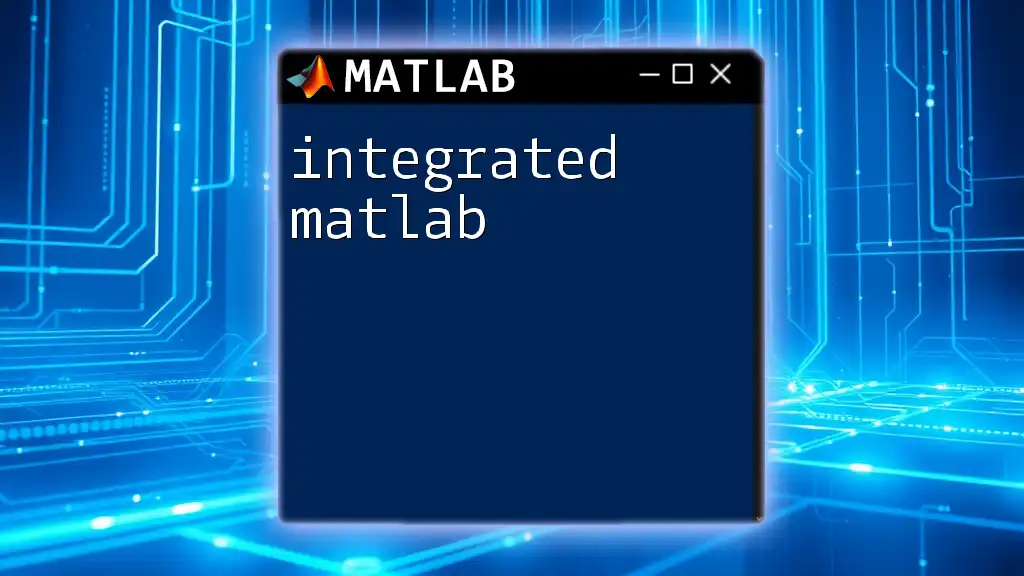
Conclusion
Mastering `inverse tan matlab` and its various applications is a valuable skill for students, engineers, and developers alike. By familiarizing yourself with the syntax, properties, and usage scenarios of inverse tangent and related functions, you can enhance your problem-solving capabilities and improve the precision of your calculations.
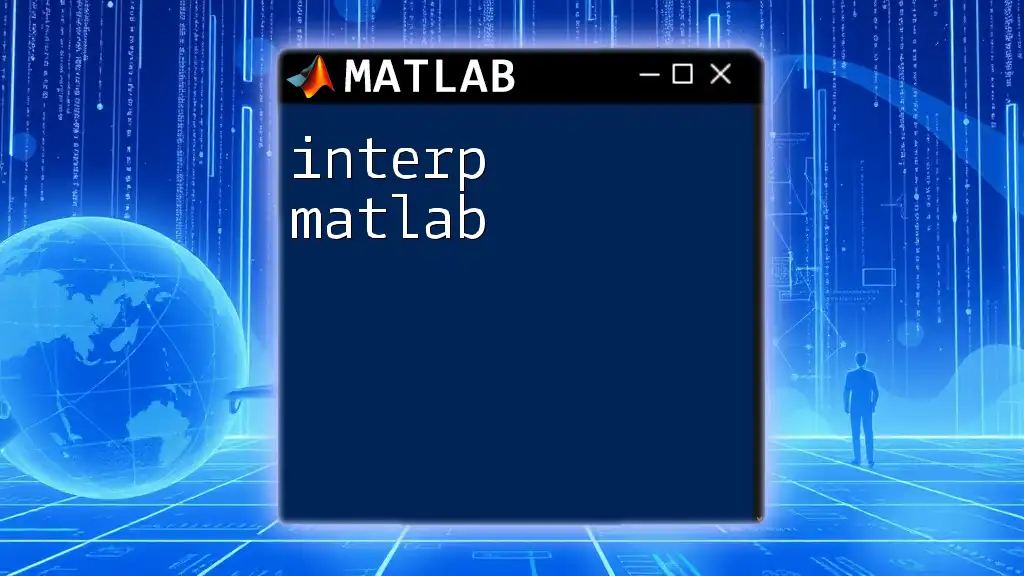
Additional Resources
For those wanting to deepen their understanding of this topic, additional reading and materials can be found in the official Matlab documentation specifically focused on the atan and atan2 functions. These resources provide detailed explanations and more complex examples that will elevate your Matlab skills.
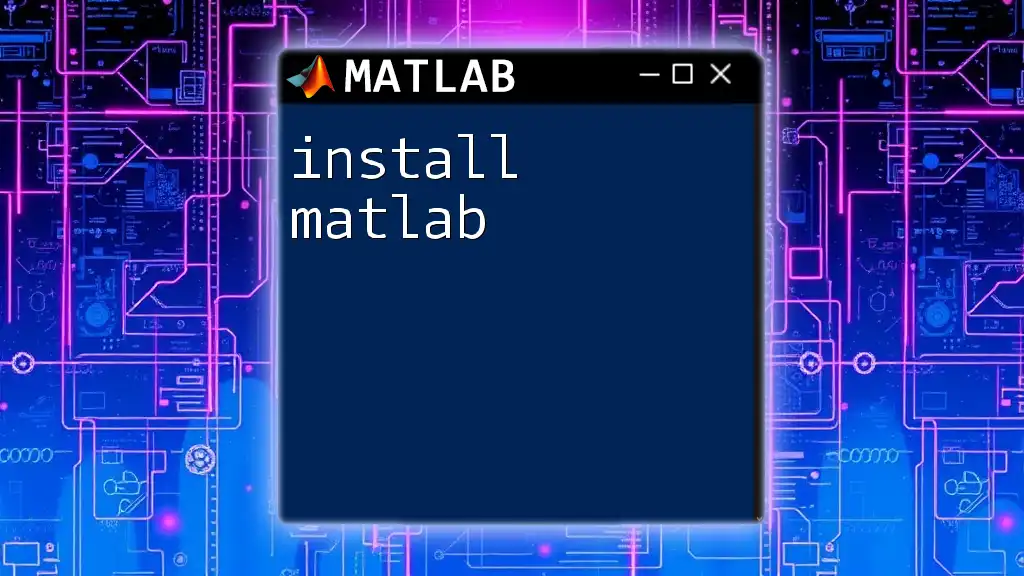
Call to Action
For more concise and practical Matlab lessons, consider joining our learning platform. Subscribe for updates or follow us on social media to receive quick tips and tricks that can enhance your coding efficiency!