The `interp` function in MATLAB is used for interpolating data points to estimate values at intermediate points based on existing data.
Here’s a simple code snippet demonstrating linear interpolation using `interp1`:
% Define data points
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
% New data points for interpolation
xi = 1:0.1:5;
% Perform linear interpolation
yi = interp1(x, y, xi);
% Plotting the results
plot(x, y, 'o', xi, yi, '-');
title('Linear Interpolation Example');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Interpolated Line');
Understanding the Basics of the `interp1` Function
Interpolation is a fundamental concept in data analysis, used to estimate unknown values from known data points. The `interp` family of functions provides powerful tools for interpolation in MATLAB, and among them, `interp1` is specifically designed for one-dimensional data.
The syntax for the `interp1` function is as follows:
yi = interp1(x, y, xi)
In this syntax:
- `x` is the vector of known x-coordinates.
- `y` is the vector of corresponding y-coordinates.
- `xi` represents the new x-coordinates at which we want to interpolate the values.
- `yi` is the output that gives the interpolated y-values corresponding to the new x-coordinates.
Example: Simple 1D Interpolation
To illustrate the use of `interp1`, consider a simple example:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
xi = 2.5;
yi = interp1(x, y, xi);
disp(yi); % Output: 4.0
In this example, the function estimates the value of `y` at `x = 2.5` based on the provided data. The output `4.0` indicates that at `x = 2.5`, the interpolated value of `y` is 4.
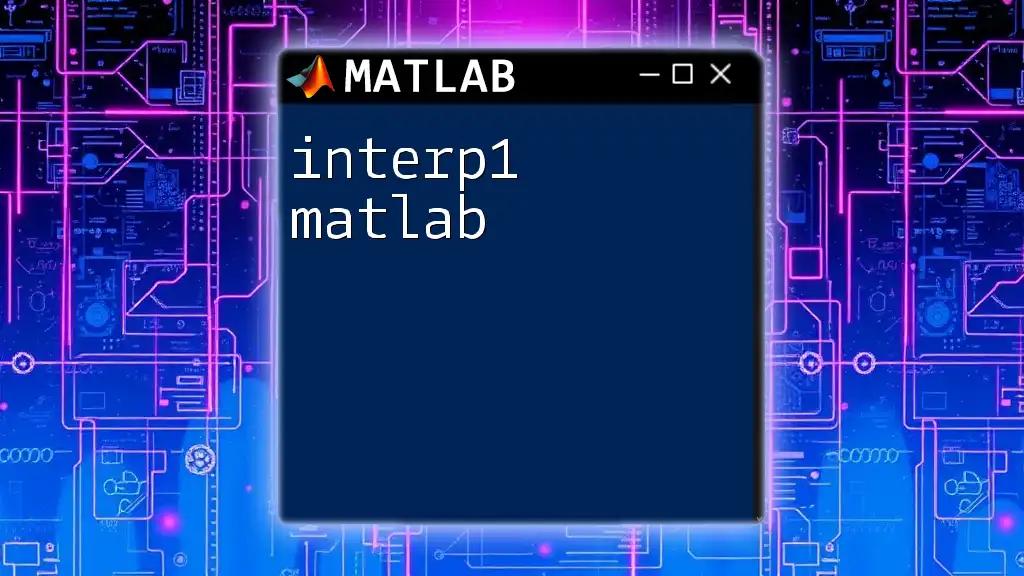
Types of Interpolation Methods
MATLAB offers several interpolation methods, each with unique advantages.
Linear Interpolation
Linear interpolation connects data points with straight lines. This method is straightforward and computationally efficient.
To execute linear interpolation, you can specify the method in the `interp1` function:
yi = interp1(x, y, xi, 'linear');
This command will give you the same output as before but explicitly uses linear interpolation.
Spline Interpolation
Spline interpolation uses piecewise polynomial functions, resulting in a smoother curve than linear interpolation. It’s particularly useful when a smooth approximation of the data is required.
Here’s how to perform spline interpolation:
yi = interp1(x, y, xi, 'spline');
This code snippet provides a smooth surface fit through the data points, enhancing visual representation and analytical accuracy.
Nearest Neighbor Interpolation
Nearest neighbor interpolation assigns the value of the nearest data point to the interpolated value. This approach is simple but can lead to discontinuities in the output.
You can implement this method as follows:
yi = interp1(x, y, xi, 'nearest');
Pchip Interpolation
Pchip, or shape-preserving piecewise cubic Hermite interpolating polynomial, maintains the monotonicity of the data. It’s especially effective when you need to avoid overshooting the original data points.
To use the Pchip method, write:
yi = interp1(x, y, xi, 'pchip');
This method ensures that the interpolated curve closely follows the shape of the provided data.
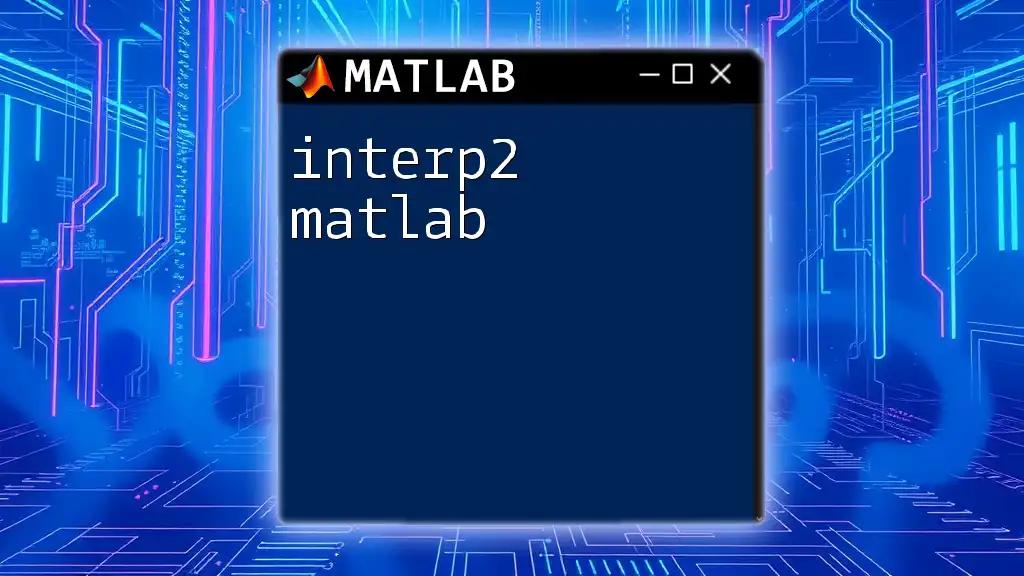
Multi-Dimensional Interpolation with `interp2`
Introduction to `interp2`
When dealing with two-dimensional data, the `interp2` function serves as the appropriate tool. The syntax resembles that of `interp1` but is designed to handle matrices:
zi = interp2(X, Y, Z, xi, yi)
In this case:
- `X` and `Y` define the grid of known points, while `Z` holds the corresponding z-values.
- `xi` and `yi` specify the new coordinates for which you want to interpolate the value.
Example: 2D Interpolation of a Surface
Let’s consider a practical example of interpolating values on a surface defined by mesh grids:
[X, Y] = meshgrid(1:5, 1:5);
Z = sin(X) + cos(Y);
xi = 2.5; yi = 3.5;
zi = interp2(X, Y, Z, xi, yi);
In this code, the `sin` and `cos` functions create a surface, and `interp2` estimates the value at the point `(2.5, 3.5)`. The resulting `zi` provides the interpolated z-value.
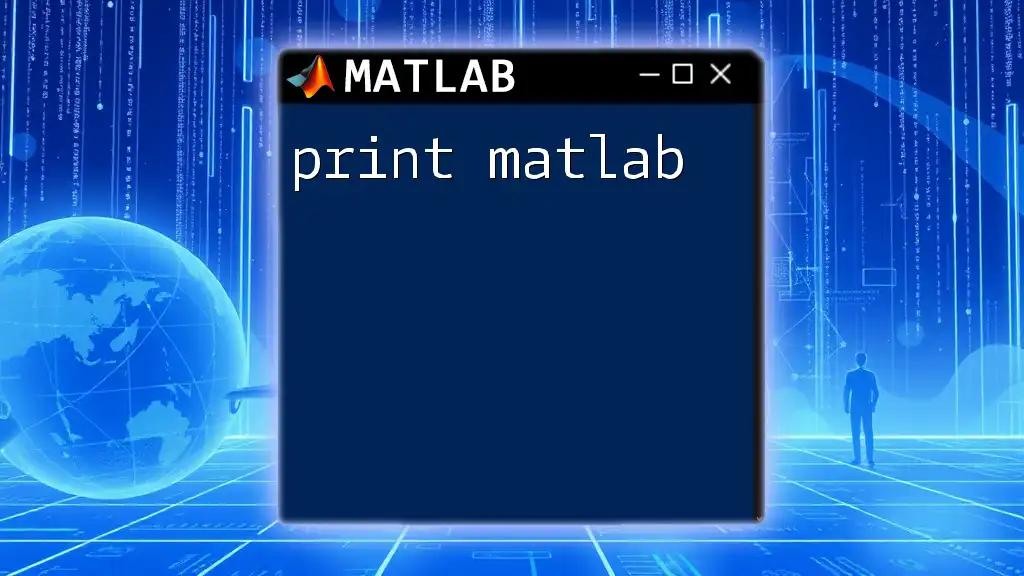
Advanced Use Cases of the `interp` Functions
Vectorization Techniques
MATLAB is designed to handle operations on vectors and matrices efficiently. When employing interpolation functions, leveraging vectorization can enhance performance significantly.
For instance:
xi = linspace(1, 5, 10);
yi = interp1(x, y, xi);
This code generates ten interpolated values between the range defined by vector `x`, demonstrating an efficient way to handle multiple interpolations simultaneously.
Handling Out of Bounds Data
When the new x-coordinates (`xi`) fall outside the range of existing data, MATLAB can still provide interpolated values through extrapolation. The syntax allows for this with the 'extrap' option:
yi = interp1(x, y, 0, 'linear', 'extrap');
In this example, we extrapolate the value of `y` at `x = 0`. While useful, it is essential to note that extrapolation may introduce errors, especially if the data does not behave linearly outside the known range.
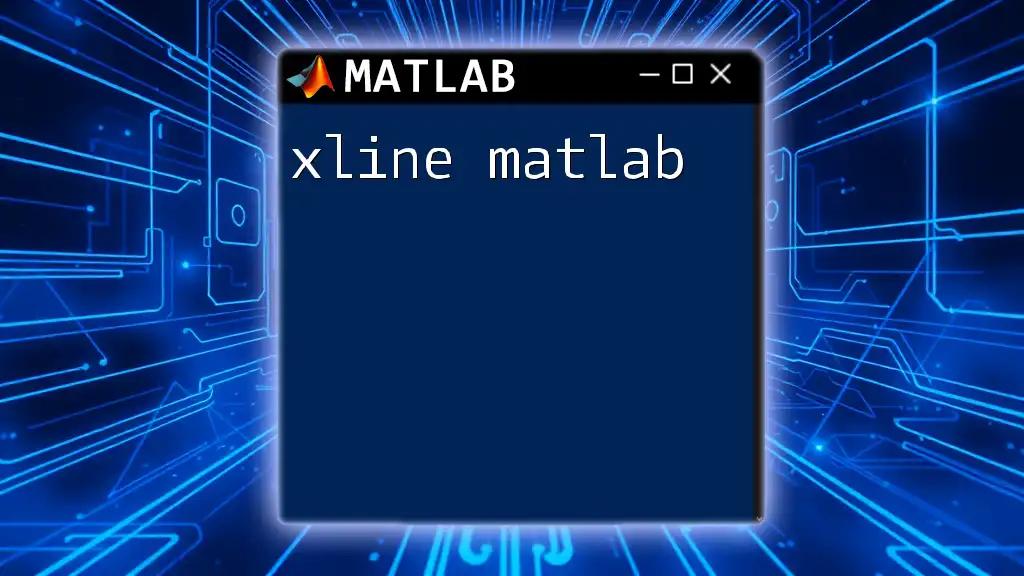
Troubleshooting Common Errors and Issues
Error Messages Explanation: It's common to encounter dimension mismatch errors when using `interp`. This typically happens if the lengths of vectors `x` and `y` are not the same, or if the new x-coordinates `xi` do not align properly with `x`. Always ensure your vectors are compatible.
Performance Considerations: When dealing with extensive datasets, consider using methods that reduce computational load. For instance, avoid unnecessary calculations by using logical indexing or vectorization techniques. This practice not only enhances speed but also ensures better performance.
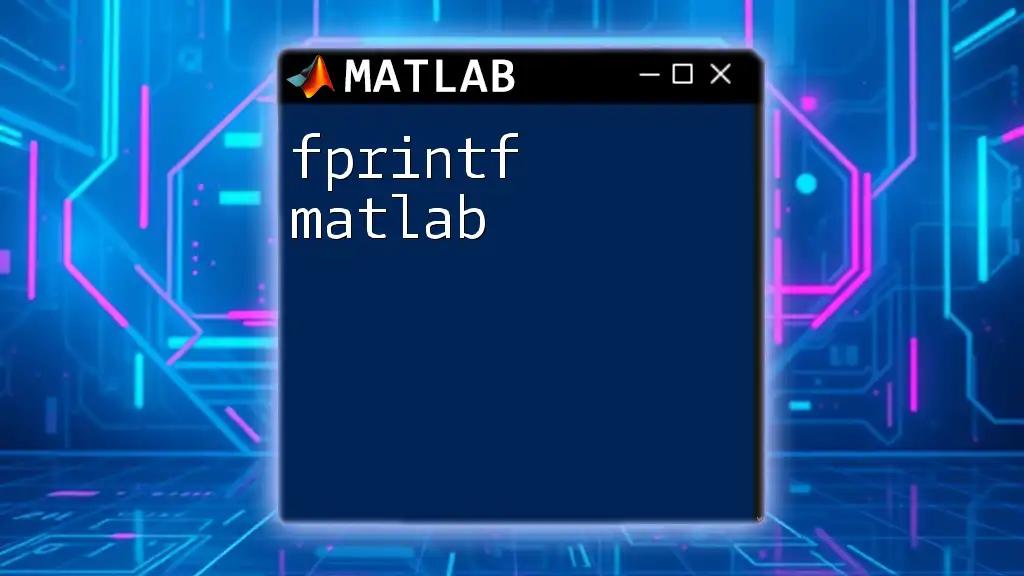
Conclusion
In summary, the `interp` functions in MATLAB provide powerful tools for interpolating one-dimensional and multi-dimensional data. By mastering functions like `interp1` and `interp2`, along with various interpolation methods, you can significantly enhance your data analysis capabilities. Practicing with diverse datasets will solidify your understanding and prepare you for real-world applications.
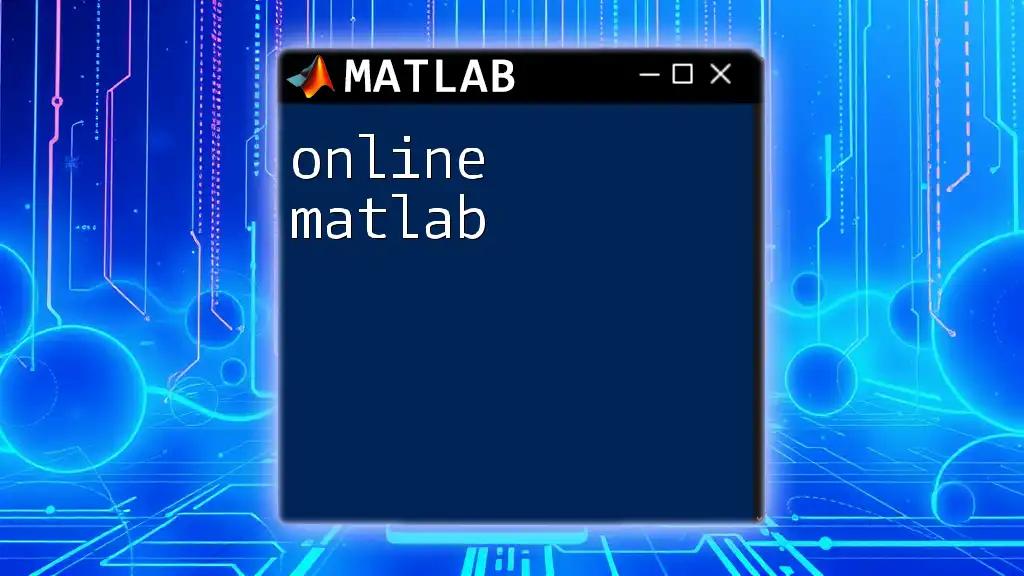
Additional Resources
MATLAB Documentation Links: For more detailed study and advanced functionalities, consult MATLAB's official documentation on interpolation functions.
Suggested Exercises: To further your learning, try interpolating various data sets using different methods, visualizing the results and comparing the effectiveness of each interpolation technique. This hands-on approach will help reinforce the concepts discussed in this guide.