The `isnan` function in MATLAB is used to identify NaN (Not a Number) values in an array, returning a logical array of the same size where each element indicates whether the corresponding element in the original array is NaN.
% Example usage of isnan function
A = [1, NaN, 3; 4, 5, NaN];
result = isnan(A);
Understanding NaN in MATLAB
What is NaN?
In MATLAB, NaN stands for "Not a Number." It is a standard placeholder used to represent undefined or unrepresentable numerical results. You might encounter NaN in various scenarios, such as:
- Performing invalid mathematical operations (e.g., `0/0`).
- When reading in data that contains missing or corrupted values.
- When using functions that result in NaN as part of their calculations, like logarithms of negative numbers.
This ability to represent undefined values is crucial in ensuring the integrity of mathematical computations, particularly when analyzing real-world data.
Why Handling NaN is Important
Handling NaN values is vital in data analysis, as they can significantly impact the results of your calculations. Ignoring NaNs leads to:
- Inaccurate results: Mean, median, and other statistical computations can be skewed by the presence of NaN.
- Errors in logic: Functions may return errors or unexpected results when NaN values are present.
- Misleading visualizations: Graphs may misrepresent data if NaN values are not appropriately managed.
By addressing NaN values, you ensure cleaner datasets and more reliable outcomes.
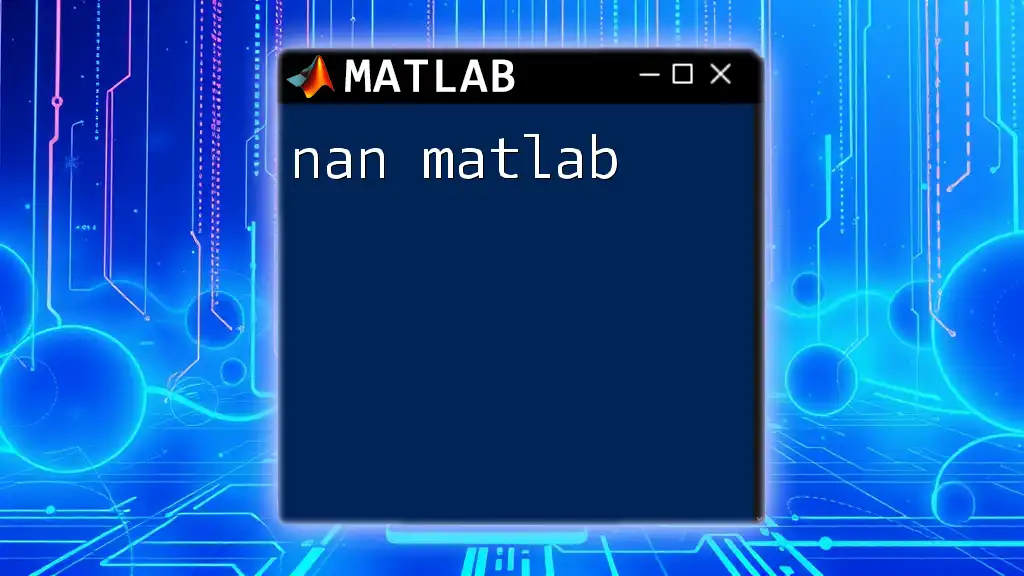
The `isnan` Function in MATLAB
Overview of the `isnan` Command
The `isnan` function in MATLAB helps identify which elements in an array are NaN. The basic syntax is as follows:
tf = isnan(A)
Here, `A` is your input array (which could be a scalar, vector, or matrix), and `tf` is the logical output array where each element is `true` (1) if the corresponding element in `A` is NaN, and `false` (0) otherwise.
How `isnan` Works
The `isnan` function offers straightforward functionality but it’s essential to understand how it operates:
- Input: You can pass any numeric array (scalars, vectors, matrices).
- Output: The output will be a logical array of the same size as the input, marking each NaN occurrence.
Examples of Using `isnan`
Basic Example
Consider the following simple example where we check an array for NaN values:
A = [1, 2, NaN, 4];
tf = isnan(A);
disp(tf);
The output will be:
0 0 1 0
This output indicates that only the third element is NaN, represented by `1`.
Working with Matrices
When applied to a matrix, `isnan` functions similarly. Let’s examine:
B = [1, NaN; 3, 4; NaN, 6];
tf_matrix = isnan(B);
disp(tf_matrix);
The output will display a matrix indicating NaNs:
0 1
0 0
1 0
Each `1` marks the position of a NaN in the original matrix.
Handling Multi-Dimensional Arrays
`isnan` also works with multi-dimensional arrays seamlessly, as shown in:
C = rand(3,2,2); % Random 3D array
C(2,1,1) = NaN; % Introducing NaN
tf_multidim = isnan(C);
disp(tf_multidim);
The logical matrix returned will show `1`s at the indices where NaN values exist, regardless of the dimensionality of the array.
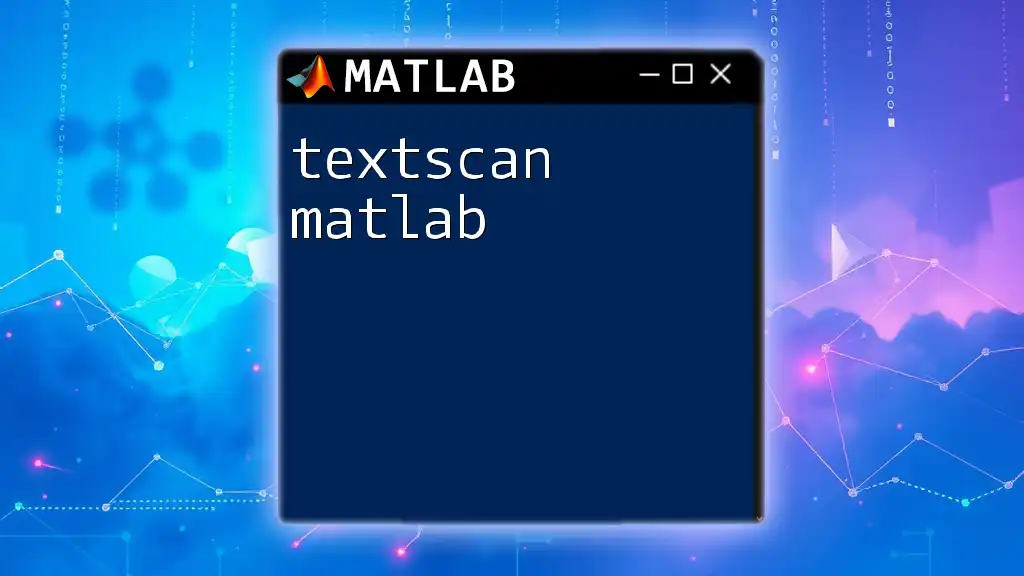
Combining `isnan` with Other Functions
Filtering Out NaN Values
You can effectively use `isnan` for filtering out NaN values and keeping valid data points intact. Here’s a practical example:
data = [1, 2, NaN, 4, NaN, 5];
filtered_data = data(~isnan(data));
disp(filtered_data);
The output will yield:
1 2 4 5
This result illustrates how to extract only the non-NaN values, leaving you with a clean dataset for further analysis.
Using `isnan` with Statistical Functions
When performing statistical calculations, NaN values will typically skew results if not handled. Here's how you can mitigate this using the `nan` variants of functions. For example:
mean_value = mean(data, 'omitnan');
disp(mean_value);
This command calculates the mean, ignoring any NaN values. The output will deliver an average based solely on valid numbers.
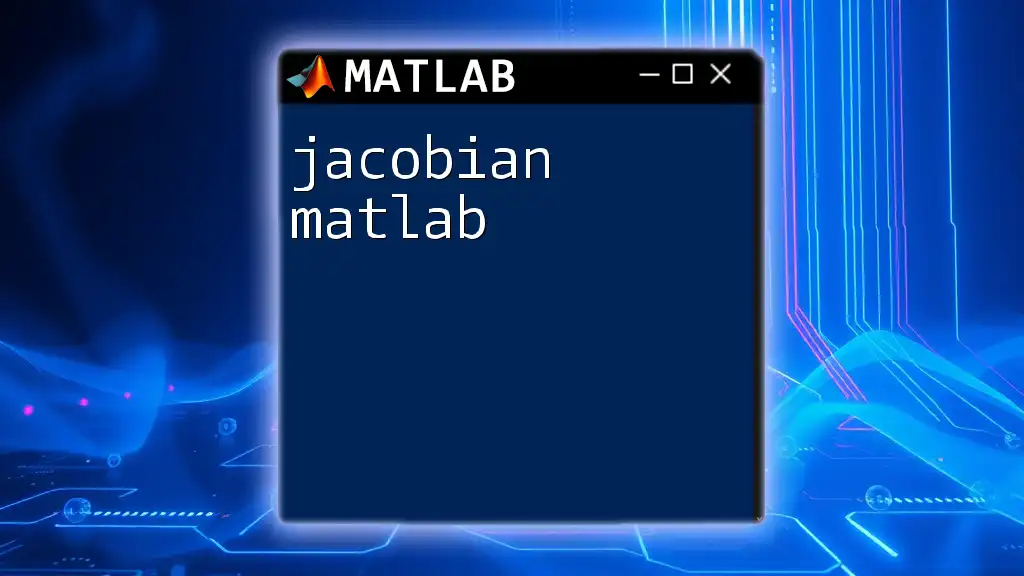
Performance Considerations
Speed and Efficiency of `isnan`
In large datasets, performance becomes a vital concern. The `isnan` function efficiently processes extensive arrays, allowing you to quickly identify NaNs without significant computational overhead. Implementing it wisely in your data preprocessing steps can optimize your workflows dramatically.
Avoiding Common Pitfalls
It’s crucial to avoid common mistakes when using `isnan`. Here are a few tips:
- Incorrect Data Types: Ensure the input to `isnan` is numeric. Non-numeric types (like cell arrays) will produce errors.
- Not Checking for Other Missing Values: While `isnan` handles NaN values, remember that datasets may also contain other forms of missing data (e.g., `Inf`, empty strings).
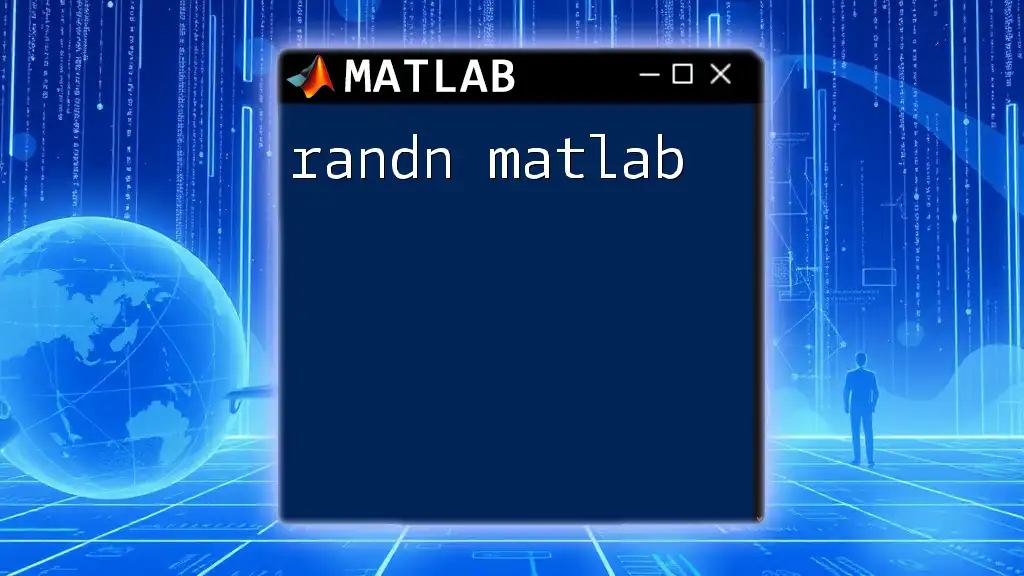
Practical Applications of `isnan`
Data Preprocessing in Machine Learning
Properly managing NaN values is imperative before using datasets in machine learning models. Handling missing values directly affects model accuracy. By identifying and optionally removing these values with `isnan`, you help ensure that your models train on high-quality, representative data.
Signal Processing and Analysis
In fields like signal processing, the presence of NaN can denote gaps in data collection or transmission errors. Applying the `isnan` function can help you quickly isolate these issues for corrective measures, ensuring the reliability of signal analysis.
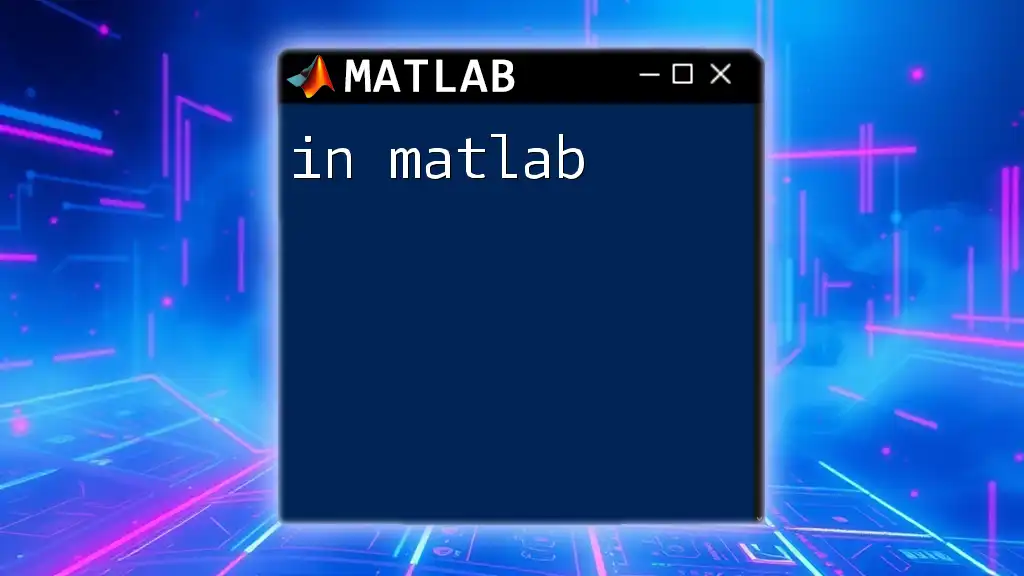
Conclusion
The `isnan` function in MATLAB is an essential tool for managing NaN values in your datasets. Mastering its use not only allows you to clean your data but also to improve the accuracy and integrity of your computations. By incorporating `isnan` into your analytical practices, you set a strong foundation for robust data analysis.
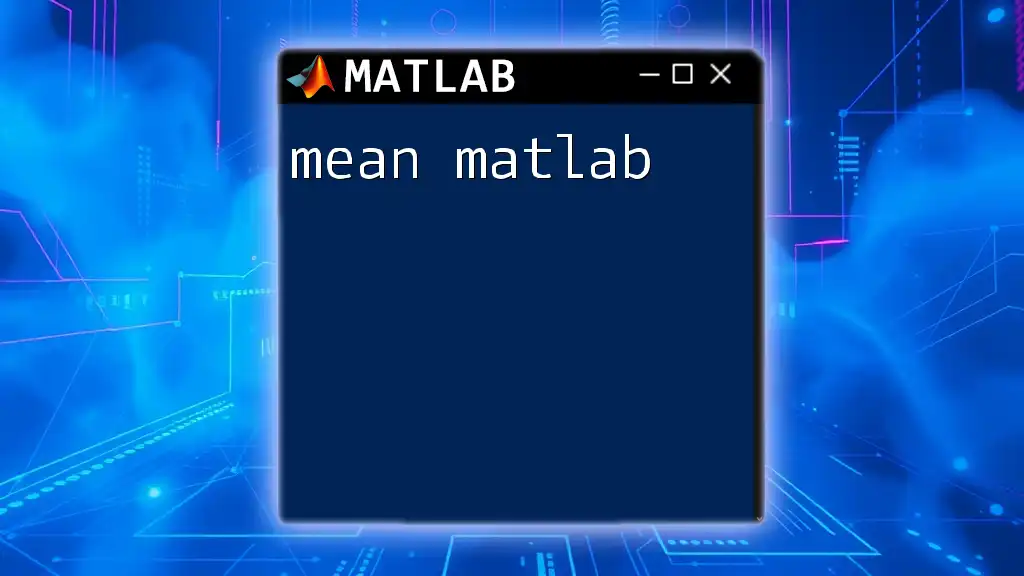
Additional Resources
Further Reading
For a deeper understanding, refer to the official MATLAB documentation on `isnan`, which provides comprehensive insights and additional examples.
Code Repositories
Explore GitHub repositories that include sample MATLAB scripts and functions specifically addressing NaN handling for practical applications in data analysis.