The `sscanf` function in MATLAB is used to read formatted data from a string and convert it into numerical values based on specified format identifiers.
Here’s a code snippet demonstrating how to use `sscanf`:
dataString = '23, 45, 67';
dataArray = sscanf(dataString, '%d, %d, %d');
In this example, `sscanf` extracts three integer values from the string and stores them in the `dataArray`.
Understanding the Basics of `sscanf`
What is `sscanf`?
`sscanf` is a powerful MATLAB function that facilitates the parsing of formatted data from strings. It enables users to convert string representations of numbers into MATLAB numeric formats. This function is particularly useful when working with data that is extracted from text files, user inputs, or formatted strings, making it easier to manipulate and analyze the data effectively.
In comparison to other functions like `scanf` and `textscan`, which also deal with formatted input, `sscanf` is specifically designed for string inputs, allowing users to convert multiple values in a single call.
Syntax of `sscanf`
The general syntax of the `sscanf` function is as follows:
A = sscanf(S, formatSpec)
Parameters Explanation:
- `S`: This is the input string or a character array that you wish to parse.
- `formatSpec`: This string specifies the format in which the input string `S` is structured, including how to interpret various data types (e.g., integers, floats, strings).
- `A`: The output variable that will contain the parsed data. It is usually an array of numbers, but it can also be a character array for strings.
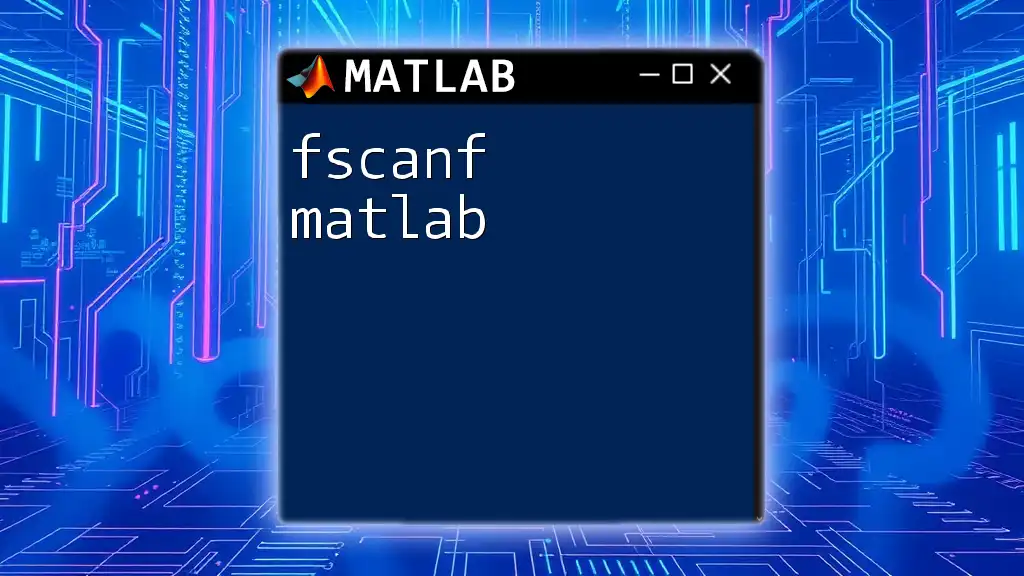
How `sscanf` Works
Parsing Input Strings
The function processes the input string based on the specified format. Understanding format specifiers is crucial, as these dictate how the input string will be interpreted. Common format specifiers include:
- `%d`: Read an integer
- `%f`: Read a floating-point number
- `%s`: Read a string
Each format specifier instructs `sscanf` on what kind of data to expect and how to store it in the output array.
Example 1: Basic Usage of `sscanf`
Consider the following code snippet:
data = '23 7.5 15';
result = sscanf(data, '%d %f %d');
disp(result);
In this example, the input string consists of three values: an integer, a float, and another integer. The format specification `%d %f %d` tells `sscanf` to expect an integer, followed by a float, followed by another integer. The output, stored in `result`, will be an array with the values `[23; 7.5; 15]`.
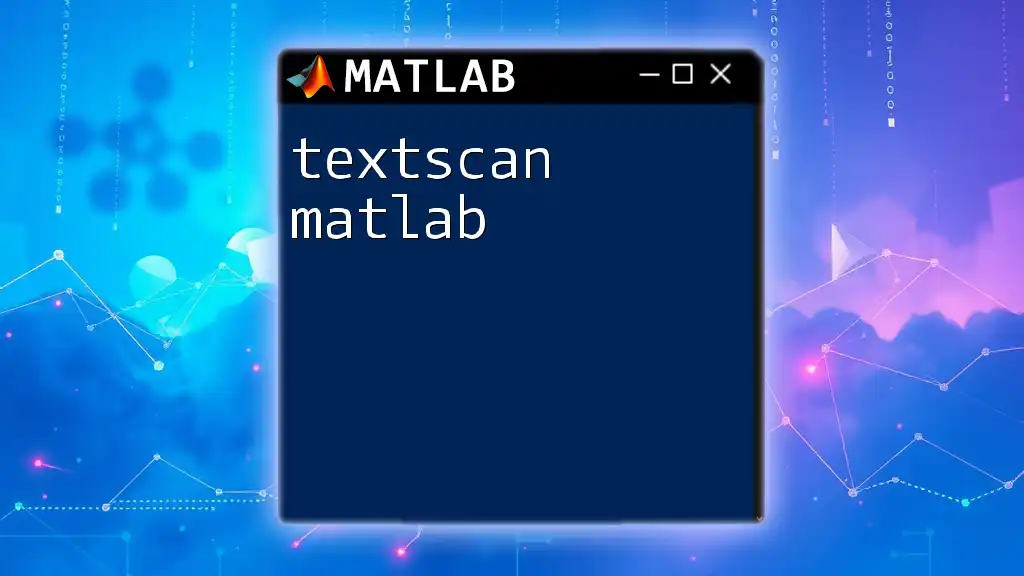
Advanced Usage of `sscanf`
Handling Multiple Data Types
`sscanf` is versatile enough to handle multiple data types simultaneously.
Example 2: Mixed Data Types
data = 'John 25 175.5';
result = sscanf(data, '%s %d %f');
disp(result);
In the above snippet, the string contains a name (a string), an age (integer), and a height (float). The format specifier `%s %d %f` directs `sscanf` to interpret the respective types correctly. Note that when dealing with `sscanf`, `%s` will only capture the first word (i.e., the first sequence of characters before a space) unless additional formatting instructions are provided.
Ignoring Unwanted Characters
`sscanf` also enables users to effectively ignore unwanted characters while parsing.
Example 3: Filtering Input
data = 'Year 2023: Revenue 15000';
result = sscanf(data, 'Year %d: Revenue %d');
disp(result);
In this scenario, the input string contains both text and numerical values. The format specification instructs `sscanf` to extract the year and revenue numbers, effectively ignoring the surrounding text and punctuation. The output will be an array with the values `[2023; 15000]`.
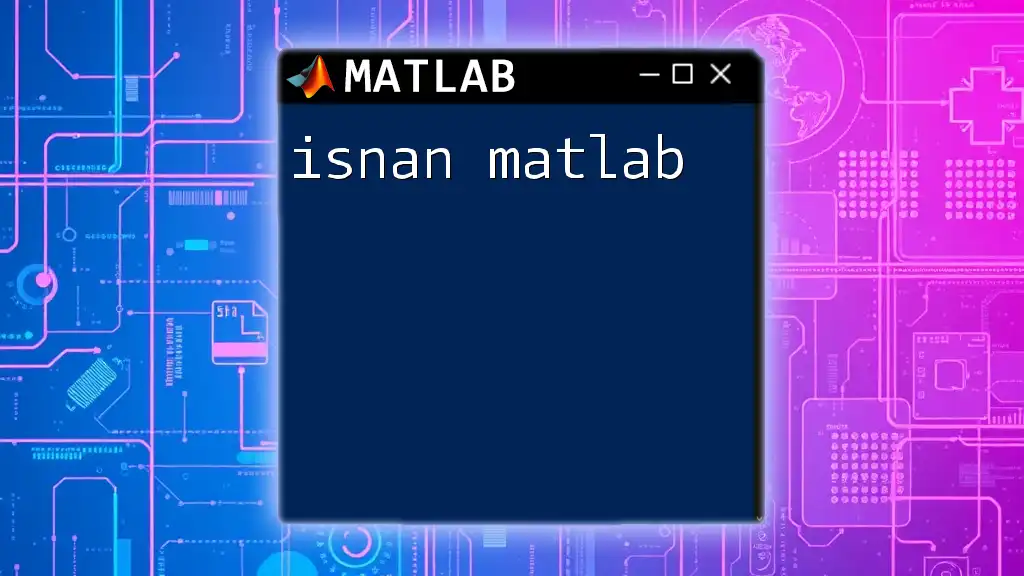
Error Handling and Troubleshooting
Common Errors with `sscanf`
When using `sscanf`, users may encounter various errors stemming from incorrect format specifications or improperly formatted strings. Some common mistakes include:
- Mismatched data types: Specifying a format that does not match the input string.
- Insufficient input: Providing an input string that lacks sufficient data for the specified format.
- Excess input: Adding additional data that `sscanf` cannot interpret with the provided format.
Debugging Tips
To effectively debug `sscanf` issues, users can use `disp` to check intermediate outputs, ensuring that the input string and format match expected structures. This step can save time in identifying sources of errors.
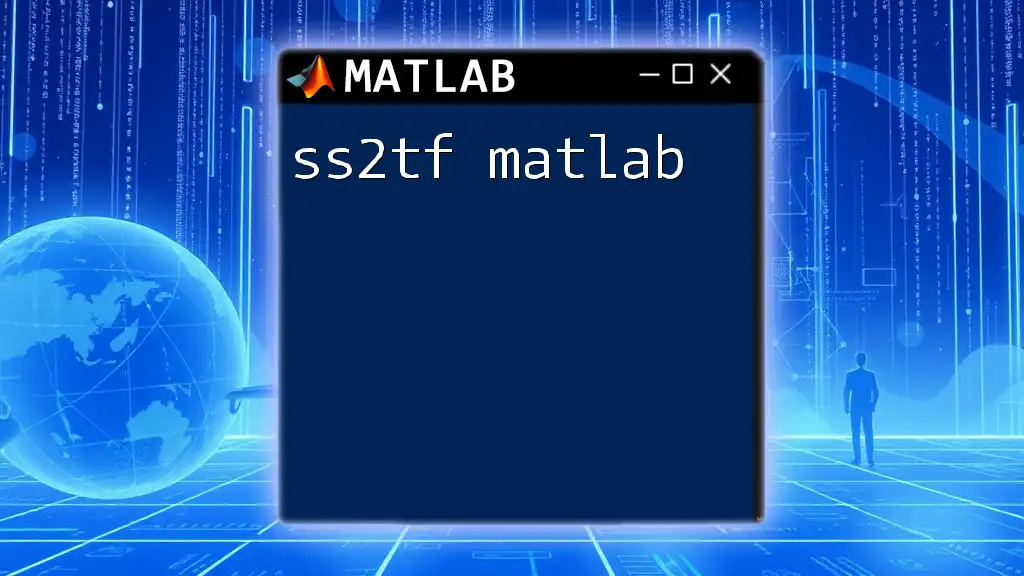
Practical Applications of `sscanf`
Data Extraction in Data Analysis
`sscanf` plays a significant role in data analysis tasks where formatted data extraction from strings is necessary. This function is frequently used in scenarios such as parsing log files, analyzing structured data from text sources, or converting user input from user interfaces. By using `sscanf`, analysts can seamlessly transition from raw string data to numeric arrays, making further statistical or graphical analysis possible.
Combining `sscanf` with Other Functions
Example 4: Using `sscanf` with `disp`
data = 'Value is: 42.0';
num = sscanf(data, 'Value is: %f');
disp(num);
By combining `sscanf` with other functions like `disp`, users can create efficient workflows. The input string is parsed to extract the floating-point number, which is then displayed, emphasizing how multiple operations can be streamlined for clarity and efficiency.
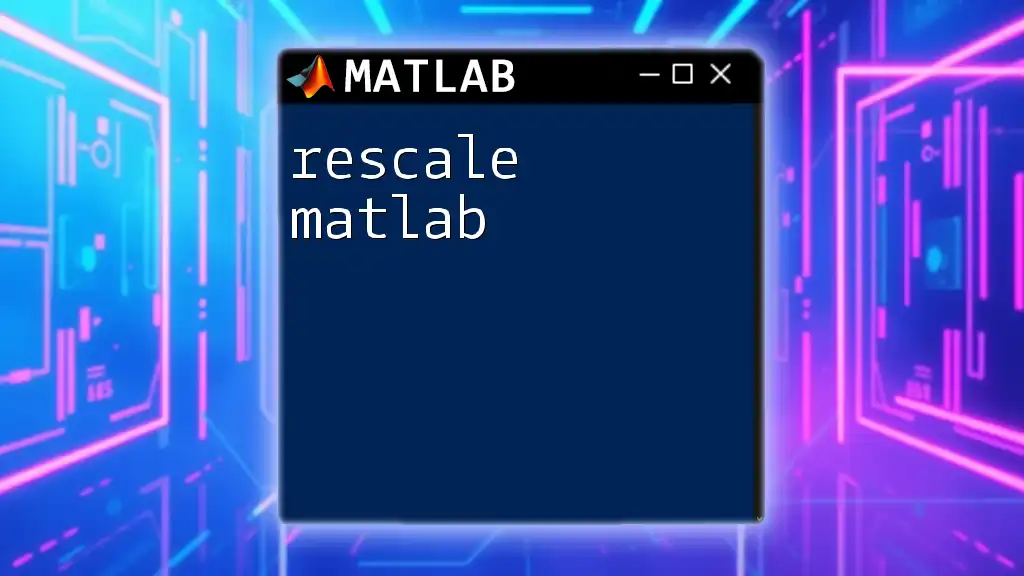
Best Practices When Using `sscanf`
Tips for Writing Clear Format Specifications
To enhance code clarity, it’s crucial to be as specific as possible with format specifications. Comprehensive comments that describe the data layout can make future code maintenance easier and can help others understand the data structure at a glance.
Performance Considerations
Being mindful of performance is vital, especially when parsing large datasets. Understanding when to utilize `sscanf` versus other MATLAB functions for reading data files (e.g., `textscan`) can reduce processing times and resource allocations.
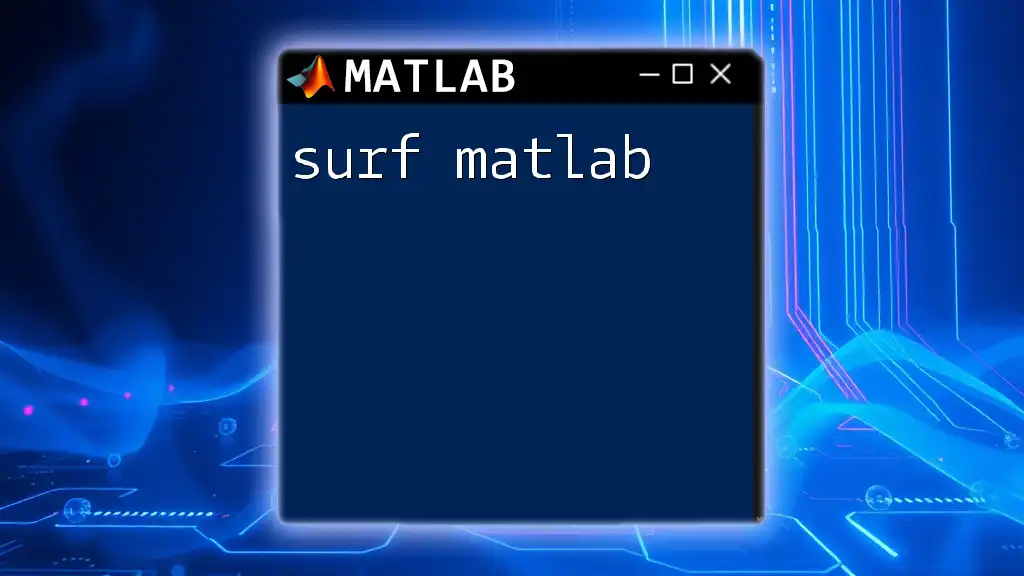
Conclusion
In conclusion, mastering the use of `sscanf` in MATLAB is instrumental for anyone involved in data parsing and analysis. Its ability to convert string data into numeric formats increases the efficiency of data manipulation tasks. Readers are encouraged to practice with various examples, experiment with different format specifications, and not hesitate to reach out with questions or to share experiences.
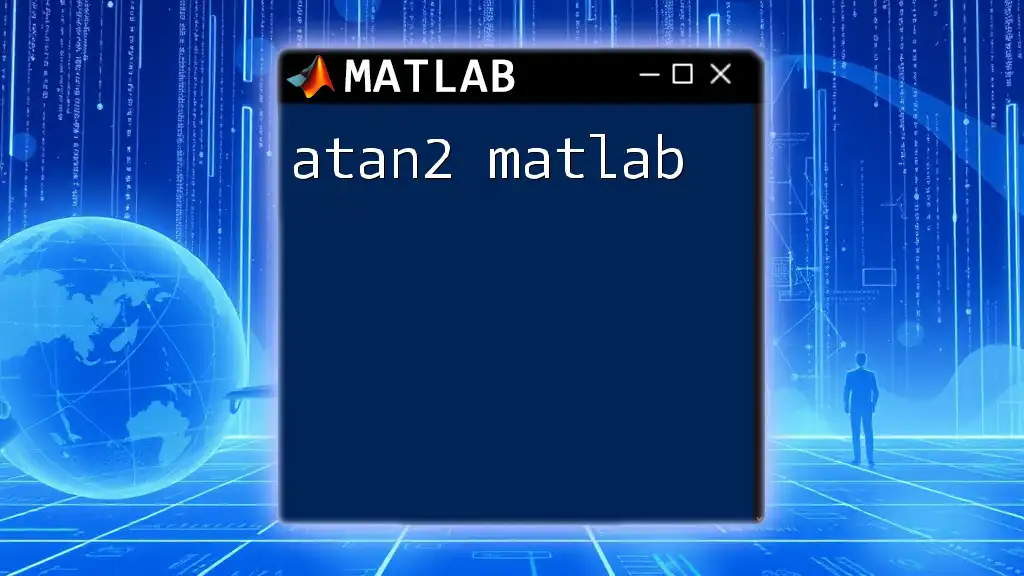
Further Resources
For additional learning, readers can refer to MATLAB’s official documentation on `sscanf`, engage with more tutorials on format specifiers, and explore online courses that focus on MATLAB programming and data analysis techniques.