The `disp` function in MATLAB is used to display text or variables without printing the variable name, making it useful for outputting simple messages or results.
disp('Hello, MATLAB World!');
Key Features of `disp`
Overview of Functionality
`disp` is a powerful built-in command in MATLAB that is primarily used for displaying text and other data types. One of its remarkable qualities is its ability to present output in a user-friendly manner without requiring additional formatting layers. The command is particularly favored for its simplicity, allowing developers to communicate the state of variables, show results, or provide feedback during program execution seamlessly.
Syntax and Basic Usage
The syntax for using `disp` is straightforward:
disp(X)
Here, `X` can be any variable—whether a string, numeric array, or other data types. This simplicity makes `disp` one of the first functions that beginners encounter when learning to program in MATLAB.
Comparison to Other Display Methods
When comparing `disp` to functions like `fprintf`, there are notable differences in flexibility and complexity. While `fprintf` allows for formatted output and more detailed control over the display (such as specifying the number of decimal places for floating-point numbers), `disp` remains a quick and efficient alternative when formatting is not a concern.
Feature | `disp` | `fprintf` |
---|---|---|
Simplicity | High | Moderate |
Formatted Output | No | Yes |
Data Types | Strings and Arrays | Strings, Arrays, and Formats |
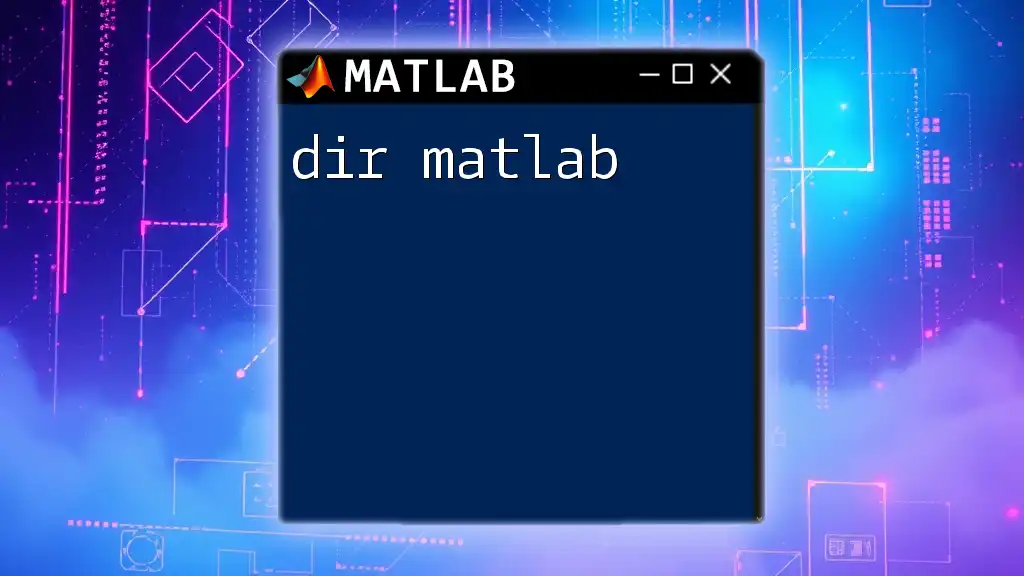
Practical Examples of Using `disp`
Example 1: Displaying a Simple Text
Let's start with a basic example. Here, we will display a simple text string:
disp('Hello, World!')
In this example, when executed, the output will display the message "Hello, World!" in the command window. This clean output illustrates the command's straightforward nature.
Example 2: Displaying Numeric Variables
Next, we can explore how `disp` can be used to showcase numeric arrays. Here’s how you can display a numeric array:
x = [1, 2, 3, 4, 5];
disp(x)
Executing this code will output the values of the array `x`, showcasing `disp`'s ability to handle various data types effortlessly.
Example 3: Displaying Multiple Variable Types
Using `disp`, you can also display a mix of strings and numeric values. This is especially useful for presenting user-friendly messages:
name = 'John';
age = 30;
disp(['Name: ', name, ', Age: ', num2str(age)]);
In this case, by combining `disp` with `num2str` (which converts numbers to strings), the output will neatly show "Name: John, Age: 30". This technique enhances the clarity and readability of outputs.
Example 4: Displaying Matrices
`disp` can be beneficial when dealing with more complex data structures, like matrices. To illustrate:
A = [1, 2; 3, 4; 5, 6];
disp('Matrix A:');
disp(A);
The output will first display "Matrix A:" followed by the contents of the matrix `A`. This showcases how `disp` can handle two-step outputs for more organized results.
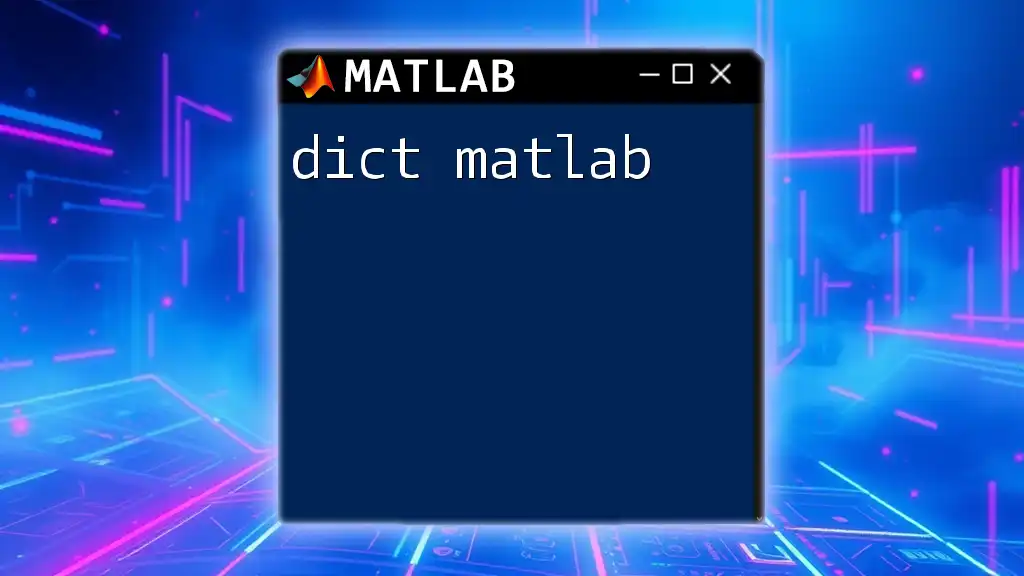
Advanced Usage of `disp`
Combining `disp` with Other Functions
`disp` can be highly effective when used in conjunction with conditional statements. This can be particularly useful for providing feedback based on the state of variables:
if age >= 18
disp('You are an adult.');
else
disp('You are a minor.');
end
In this example, the output will vary based on the value of `age`, demonstrating how `disp` can interact effectively with logic to communicate important information.
Displaying Complex Data Types
While `disp` excels with strings and arrays, handling complex data types like cell arrays and structures may require additional strategies. When trying to display these types, one must account for the inherent differences in how data is stored and represented in MATLAB. For example, if you have a cell array, you might convert it to a string for better readability before using `disp`.
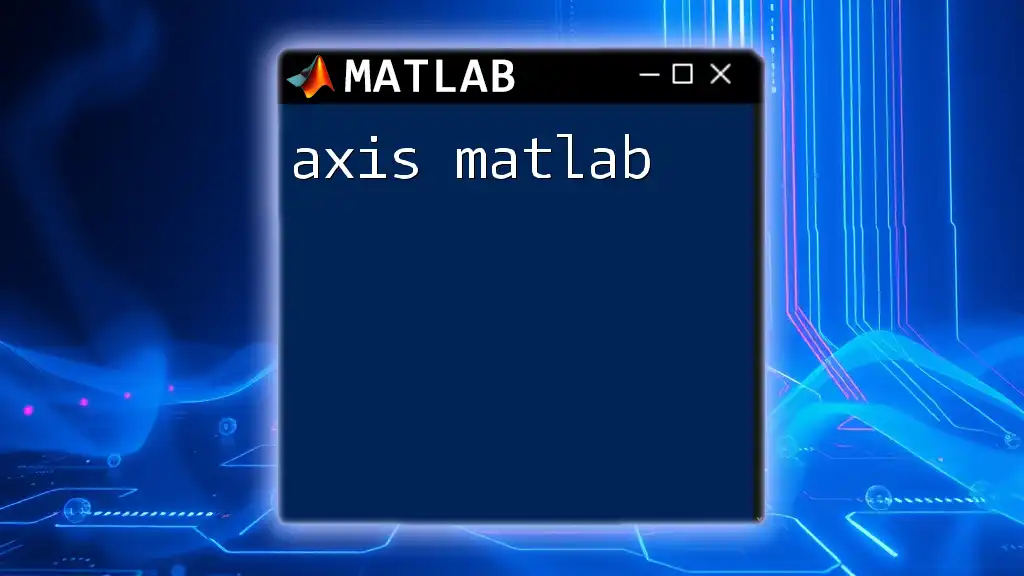
Tips and Best Practices for Using `disp`
Clarity in Output
To avoid confusion in outputs, especially when multiple variables or data types are involved, maintain organization in your displayed messages. A good practice is to concatenate strings meaningfully for clarity.
Performance Considerations
While `disp` is efficient, opting for it exclusively can lead to limitations in cases where you require formatted output. In structured output scenarios, prioritize `fprintf` or other specialized functions to achieve the desired presentation. Understanding when to use `disp` versus more complex display methods can significantly enhance your coding efficiency and the effectiveness of output communication.
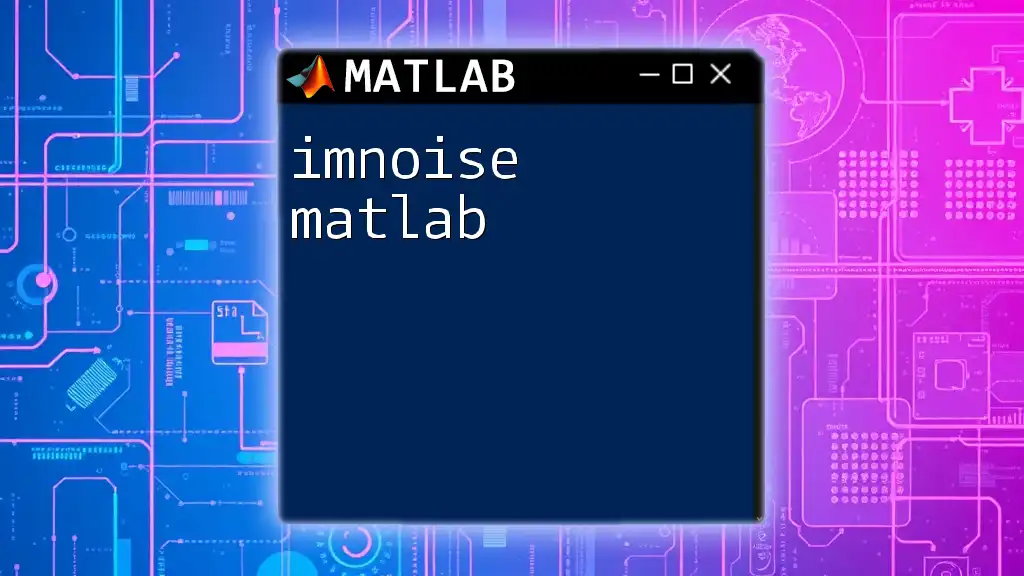
Troubleshooting Common Issues
Common Mistakes with `disp`
One common pitfall is attempting to display unsupported data types directly. For instance, attempting to display a function handle or a symbolic expression with `disp` may lead to unexpected outputs. Always ensure the data passed to `disp` is appropriate.
Handling Empty Variables
It's important to recognize how `disp` behaves when dealing with empty variables. For example:
emptyVar = [];
disp(emptyVar)
The output will be an empty line, which might confuse users who are unfamiliar with this behavior. Being aware of these nuances can save time and avoid misunderstandings in your programming sessions.
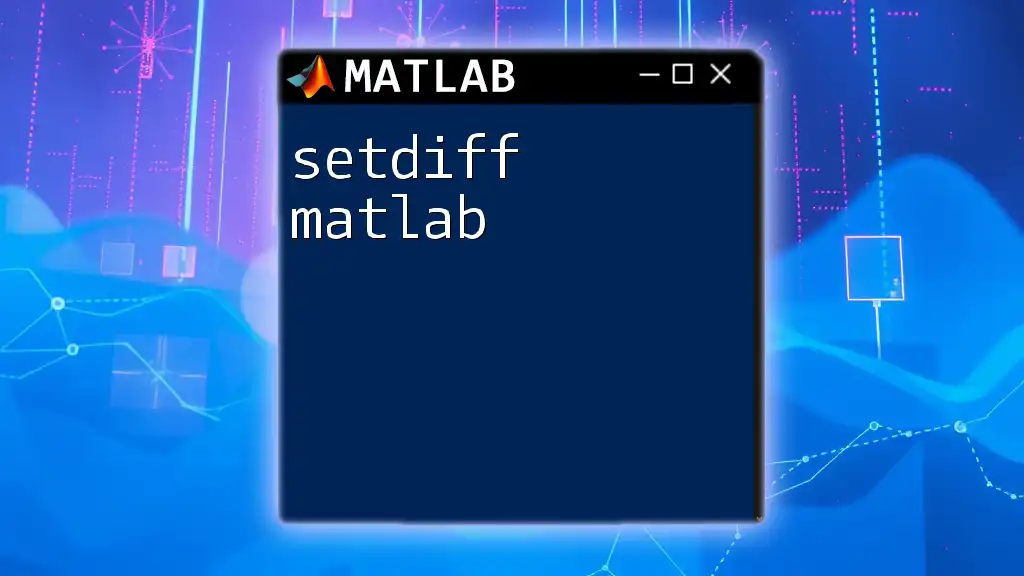
Conclusion
In conclusion, the `disp` command in MATLAB provides a straightforward method to display information, whether it's strings, numbers, or complex structures. By mastering its functionalities and understanding its limitations, you can enhance your MATLAB programming experience. As you venture further into using MATLAB, remember to practice employing `disp`, and start integrating it extensively to communicate effectively through your code.
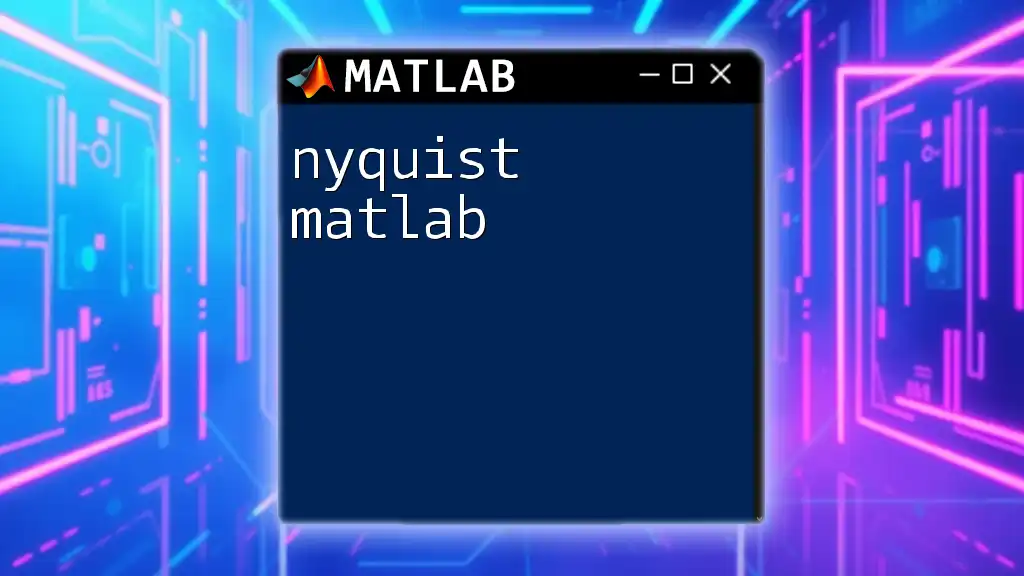
Additional Resources
For further exploration, consider visiting the official MATLAB documentation and online forums, where you can find a wealth of information and community-driven support. Engaging with additional resources will deepen your understanding and widen your skill set as you advance in MATLAB programming.