The `fscanf` function in MATLAB reads formatted data from a text file or string, allowing users to specify the format of the data they want to extract.
Here's a code snippet demonstrating the use of `fscanf` to read integers from a file:
% Open a file for reading
fileID = fopen('data.txt', 'r');
% Read formatted data from the file (in this case, integers)
data = fscanf(fileID, '%d');
% Close the file
fclose(fileID);
What is fscanf?
`fscanf` is a powerful function in MATLAB used primarily for reading formatted data from text files. It allows users to specify the format in which the data is stored, providing flexibility and control when dealing with different types of file inputs. Understanding how to use `fscanf matlab` effectively is crucial for anyone who needs to extract numerical or textual data from structured files.
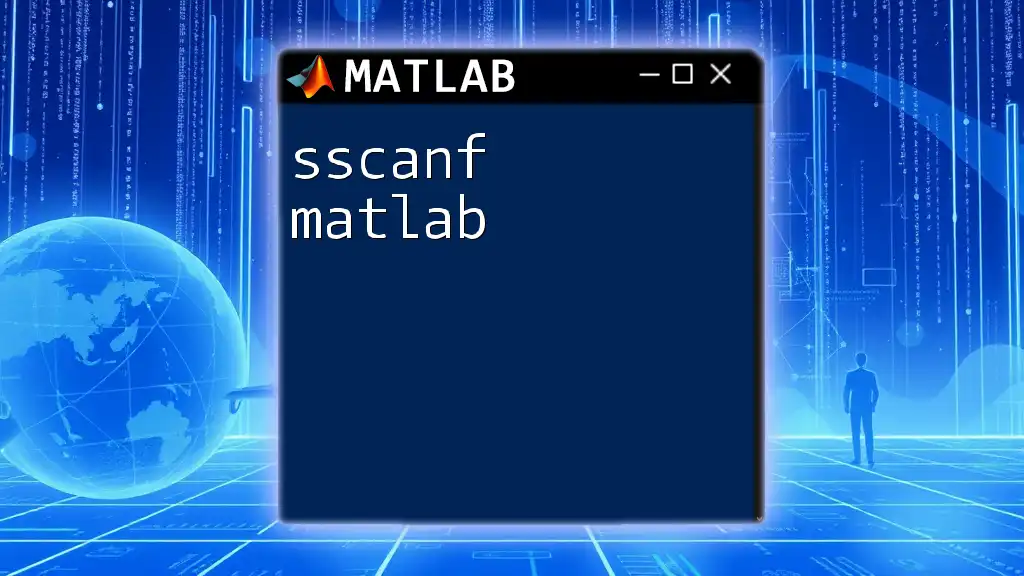
Overview of fscanf Functionality
The power of `fscanf` lies in its ability to read various data types, including integers, floating-point numbers, and characters, from files. This makes it particularly useful in scientific computing and data analysis, where data is often stored in text format.
When comparing `fscanf` to other file reading functions like `load` and `textscan`, it's important to note that `fscanf` is specifically designed for structured or formatted data. This can make it more suitable in instances where precise control over data types and structure is required.
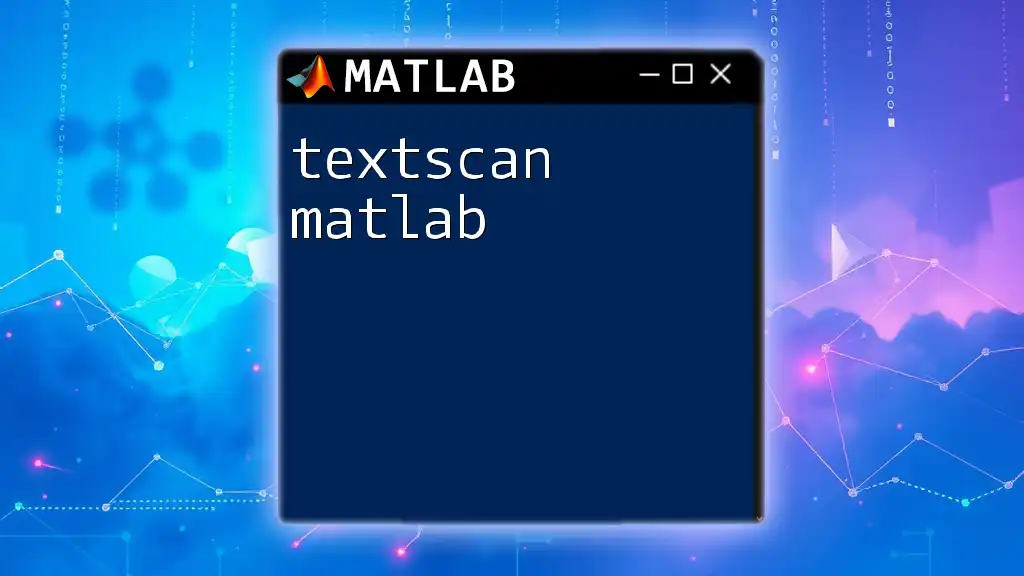
Understanding the Syntax of fscanf
Basic Syntax Explained
The basic syntax of `fscanf` can be broken down as follows:
A = fscanf(fileID, formatSpec, sizeA)
Parameters of fscanf
-
fileID: This is an identifier for the file opened using `fopen`. It is critical to ensure that the fileID corresponds to an open file; otherwise, the function will not work as intended.
-
formatSpec: The format specification string that defines the format of the input data. For example:
- `%d` for integers
- `%f` for floating-point numbers
- `%s` for strings.
A robust understanding of these format specifiers is vital, as they dictate how `fscanf` interprets the data in the file.
-
sizeA: This parameter indicates the size of the output array `A`. By default, if this parameter is not supplied, `fscanf` will read data until the end of file (EOF). Specifying `sizeA` can transform the output into a specific dimension, which is especially helpful when dealing with matrices.
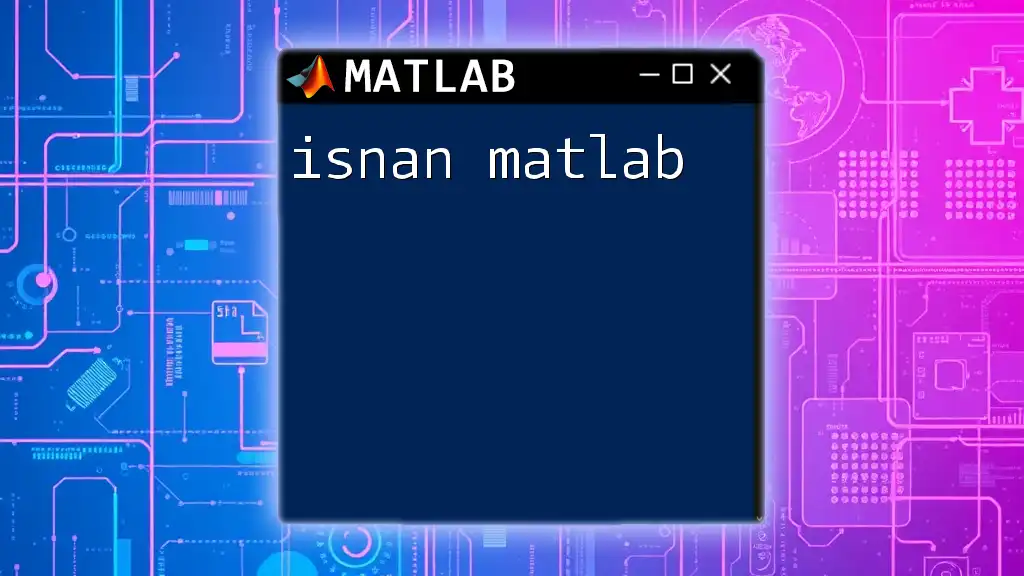
How to Use fscanf: Step-by-Step Tutorial
Step 1: Opening a File
Before any data can be read, you must first open the file using the `fopen` function. Here’s a simple example:
fileID = fopen('data.txt', 'r');
In this example, `'data.txt'` refers to the file containing the data, and `'r'` indicates that the file is opened in read mode. It's essential to handle the file permissions appropriately to avoid runtime errors.
Step 2: Reading Data with fscanf
Once the file is open, you can read the data using `fscanf`. Below is a simple example to demonstrate how one might read a list of floating-point numbers from a text file:
data = fscanf(fileID, '%f');
This command reads all floating-point numbers from the file assigned to `fileID` and stores them in the variable `data`. Understanding how this works requires familiarity with the file’s content and organization.
Step 3: Closing the File
It is essential to close the file after you finish reading data to free up system resources. This can be achieved with the following command:
fclose(fileID);
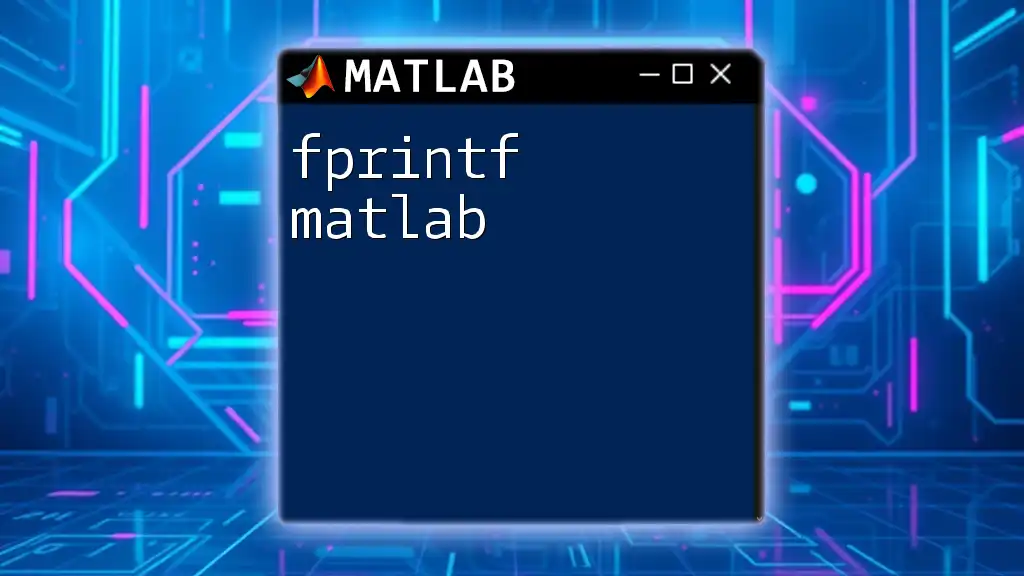
Practical Examples of fscanf in Action
Example 1: Reading Integer Data from a File
Let’s say you have a text file containing the following integers:
1
2
3
4
5
You can read these integers using:
fileID = fopen('integers.txt', 'r');
integers = fscanf(fileID, '%d');
fclose(fileID);
After executing this code, `integers` will contain an array with the values `[1; 2; 3; 4; 5]`. This demonstrates how `fscanf` can effectively parse integer data from a simple text format.
Example 2: Reading Floating-point Numbers
Suppose you want to read a set of decimal numbers from another file, say `floats.txt` containing:
3.14
2.718
1.618
You could use the following code snippet:
fileID = fopen('floats.txt', 'r');
floats = fscanf(fileID, '%f');
fclose(fileID);
Here, `floats` will hold a column vector with the values `[3.14; 2.718; 1.618]`, showcasing the ability to extract floating-point numbers seamlessly.
Example 3: Reading Multiple Data Types
Reading mixed-type data requires a more comprehensive approach. For example, if you have a file containing names and scores:
Alice 85
Bob 90
Charlie 75
You would need to customize your format string to accommodate both strings and numbers. Here’s a rough example:
fileID = fopen('scores.txt', 'r');
formatSpec = '%s %d'; % String followed by an integer
data = textscan(fileID, formatSpec);
fclose(fileID);
In this example, `textscan` is more appropriate for mixed data, but it showcases how you can specify types. Each column of data would be stored in a cell array, allowing for different dimensions depending on the columns.
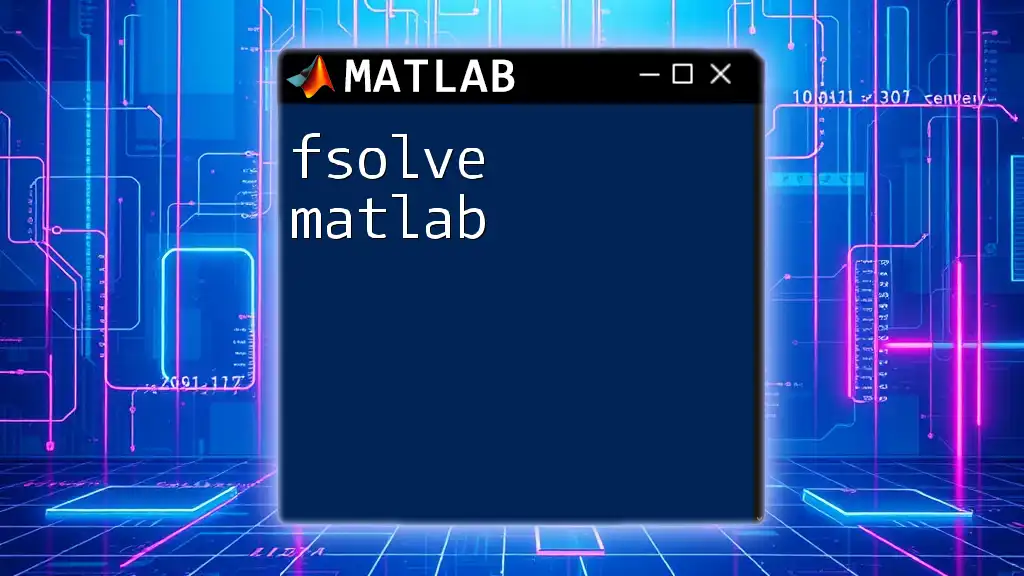
Advanced Usage of fscanf
Reading Data into Specific Array Shapes
`fscanf` can read data directly into a specific shape. For example, if you want to read numbers into a 3-row matrix regardless of how many numbers are present, you could do:
data = fscanf(fileID, '%f', [3, Inf]);
This command will read data into a matrix with three rows and as many columns as necessary.
Error Handling with fscanf
It’s crucial to manage errors properly when working with file I/O. Common issues can arise if the file does not exist, or if the format specifier does not match the data format in the file. Always check if `fileID` is valid:
if fileID == -1
error('File not found or could not be opened.');
end
This simple check ensures that the subsequent commands don’t attempt to operate on an invalid file ID, which would lead to unnecessary errors.
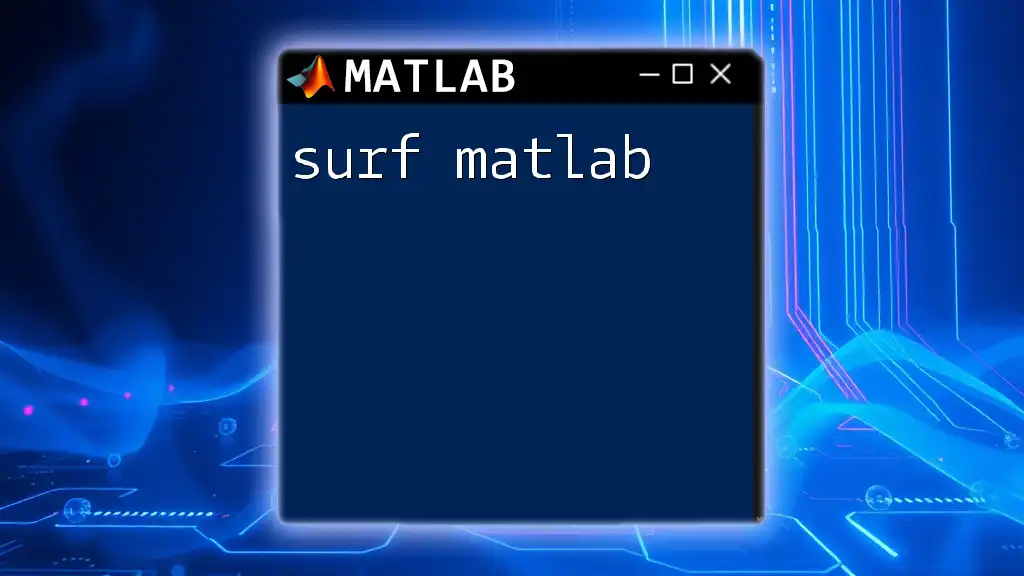
Best Practices for Using fscanf
Input Validation Techniques
Always validate that the data read aligns with your expectations concerning types and structures. This critical step can prevent subtle bugs in your analyses.
Performance Considerations
While `fscanf` is efficient for reading structured data, consider profiling its performance if you are handling extremely large datasets. In some cases, experimenting with alternatives like `textscan` may yield better performance.
Alternative Functions to Consider
In instances where data format varies significantly or is unstructured, consider using `textscan` or `readtable`. These functions offer more flexibility and are equipped to handle different types and structures effectively.
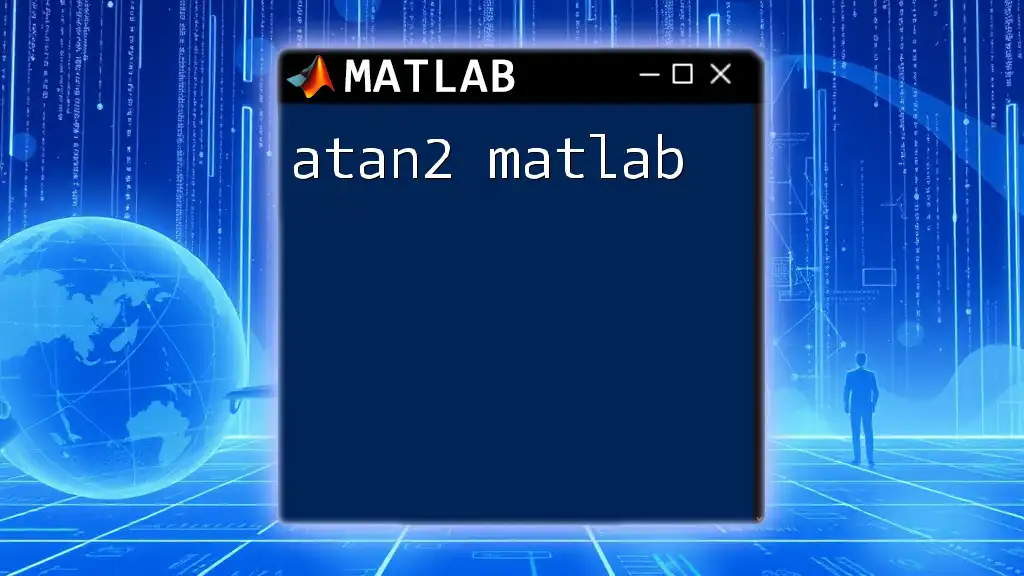
Conclusion
In summary, mastering `fscanf matlab` empowers you to manage file input effectively. Understanding its syntax and capabilities, coupled with practical examples, lays a solid foundation for applying this function in your projects. Practice using `fscanf` to enhance your MATLAB skills and streamline your data analysis tasks.
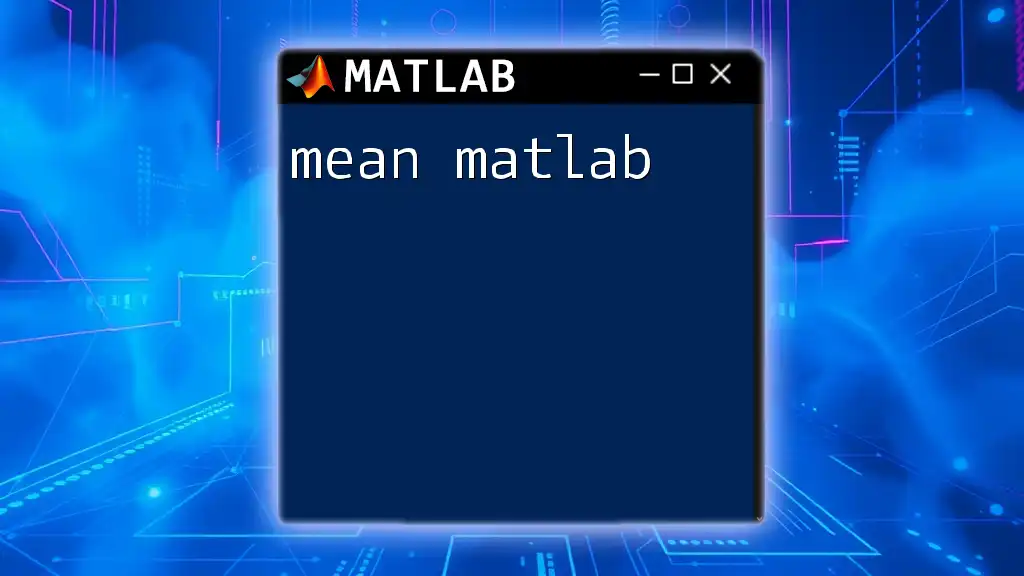
Additional Resources
For further exploration, refer to the official MATLAB documentation and consider joining community forums where you can share insights and learn from others in the field. As you advance, seeking out recommended tutorials and books can also significantly deepen your understanding of MATLAB programming.