In MATLAB, a high-performance language for technical computing, you can efficiently perform mathematical tasks, data analysis, and algorithm development using concise syntax.
Here's a simple example of calculating the sum of an array:
% Define an array
A = [1, 2, 3, 4, 5];
% Calculate the sum of the array
total = sum(A);
Getting Started in MATLAB
Setting Up MATLAB
Installation Process
To begin your journey in MATLAB, you need to install it on your computer. You can download it from the official MathWorks website. Follow these steps to ensure a smooth installation:
- Create a MathWorks account if you don’t have one.
- Select the appropriate MATLAB package based on your requirements.
- Download the installer and run it on your computer.
- Follow the on-screen instructions to complete the installation process.
Creating Your First Script
Once MATLAB is installed, you can create your first script. Open the MATLAB application, and navigate to the Editor by selecting New Script from the Home tab. You can now write your first command.
disp('Hello, MATLAB!')
This command will display "Hello, MATLAB!" in the Command Window when executed. You can save the file with a `.m` extension (e.g., `hello.m`) to keep your work for later use.
Basic MATLAB Commands
Entering Commands in the Command Window
The Command Window is your main workspace in MATLAB. Here, you can enter and execute commands directly. For instance, performing a simple arithmetic operation is straightforward:
result = 5 + 10
When you run this code, MATLAB will display `result = 15`, showing how seamlessly it handles computations.
Working with Variables
In MATLAB, declaring variables is simple. You can assign values as follows:
a = 5;
b = 10;
c = a + b;
In this example, `c` will hold the value `15`, demonstrating how easy it is to manipulate variables in MATLAB.
Understanding the Workspace
Exploring the Workspace
The Workspace is an interactive display of all variables currently in memory. You can view, manage, and modify these variables without hassle. Use the command `whos` in the Command Window to display all the variables, along with their types and sizes.
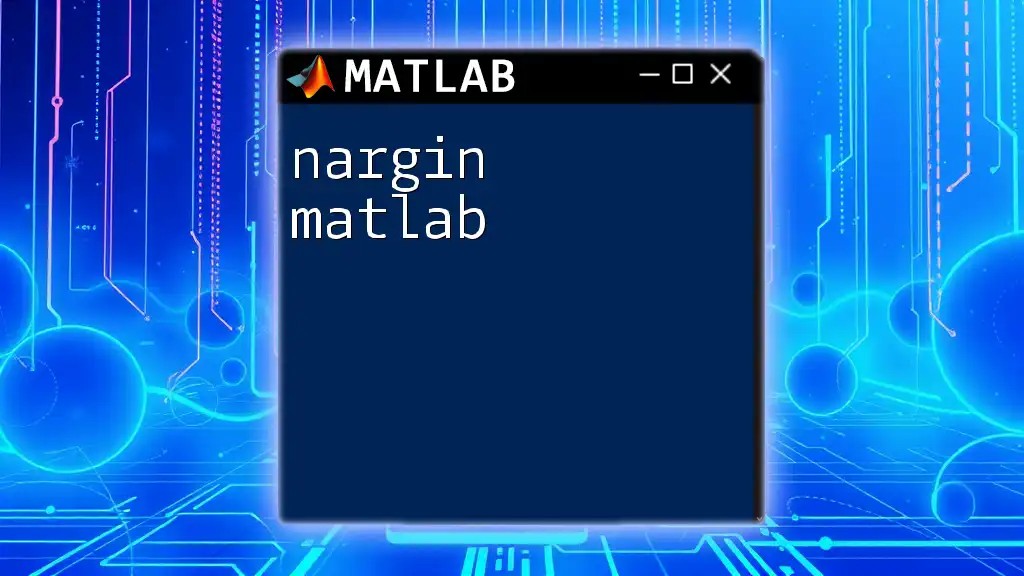
Advanced MATLAB Commands
Array and Matrix Operations
Creating Arrays and Matrices
One of the core features of MATLAB is its robust support for arrays and matrices. You can create a row vector or a column vector using square brackets:
rowVector = [1, 2, 3, 4];
columnVector = [1; 2; 3; 4];
These simple notations allow you to construct complex data structures effortlessly.
Basic Operations on Arrays
In MATLAB, performing operations on arrays can be done cleanly and efficiently. You can perform element-wise operations or matrix operations using the symbols `.*` for multiplication and `./` for division.
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A * B; % Matrix multiplication
D = A .* B; % Element-wise multiplication
In this case, `C` will contain the results of matrix multiplication, while `D` will hold the element-wise products.
Control Flow Statements
Conditional Statements
Conditional statements such as `if`, `elseif`, and `else` allow you to execute code conditionally. For example:
x = 10;
if x > 5
disp('x is greater than 5');
elseif x == 5
disp('x is equal to 5');
else
disp('x is less than 5');
end
This will display "x is greater than 5," showing how control structures work in MATLAB.
Loops in MATLAB
Loops are essential for repetitive tasks. The `for` loop and `while` loop are commonly used in MATLAB. Here’s an example of a `for` loop:
for i = 1:5
disp(['Iteration: ', num2str(i)]);
end
This code will display the iteration number from 1 to 5, demonstrating MATLAB’s ability to iterate over a range effortlessly.
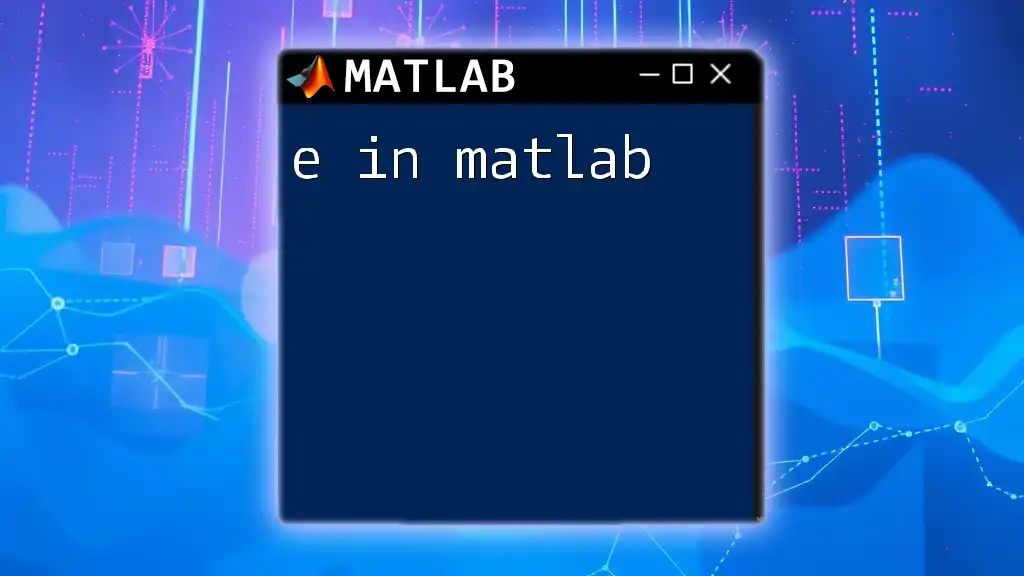
Essential Functions in MATLAB
Built-in Functions
Mathematical Functions
MATLAB offers a plethora of built-in mathematical functions. Functions like `sum`, `mean`, and `std` can be used to perform calculations on arrays with ease:
data = [1, 2, 3, 4, 5];
total = sum(data); % Total = 15
average = mean(data); % Average = 3
These functions make data analysis straightforward and efficient.
Custom Functions
Creating custom functions expands MATLAB’s capabilities to suit specific needs. Here’s how you can define a simple function to calculate the factorial of a number:
function result = factorial(n)
if n == 0
result = 1;
else
result = n * factorial(n - 1);
end
end
You can call this function by using `factorial(5)`, which will return `120`.
Working with Data
Reading and Writing Data
MATLAB provides easy methods for managing data files. You can import data using commands like `readtable` for tabular data:
dataTable = readtable('data.csv');
For exporting data, you can use `writetable`:
writetable(dataTable, 'output.csv');
Visualizing Data
Creating Plots
Visualizing data in MATLAB is intuitive. You can create 2D and 3D plots with simple commands. For a basic line plot of a mathematical function:
x = 0:0.1:10; % Creates a range of values from 0 to 10
y = sin(x); % Calculate the sine of each value
plot(x, y); % Plot the values
This command will generate a clean sine wave plot, demonstrating MATLAB's powerful graphic capabilities.
Customizing Plots
Once you have your plots, you might want to enhance their aesthetic quality and readability. You can add titles, labels, and legends as follows:
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
legend('sin(x)');
These additions make your visualizations informative and professional.
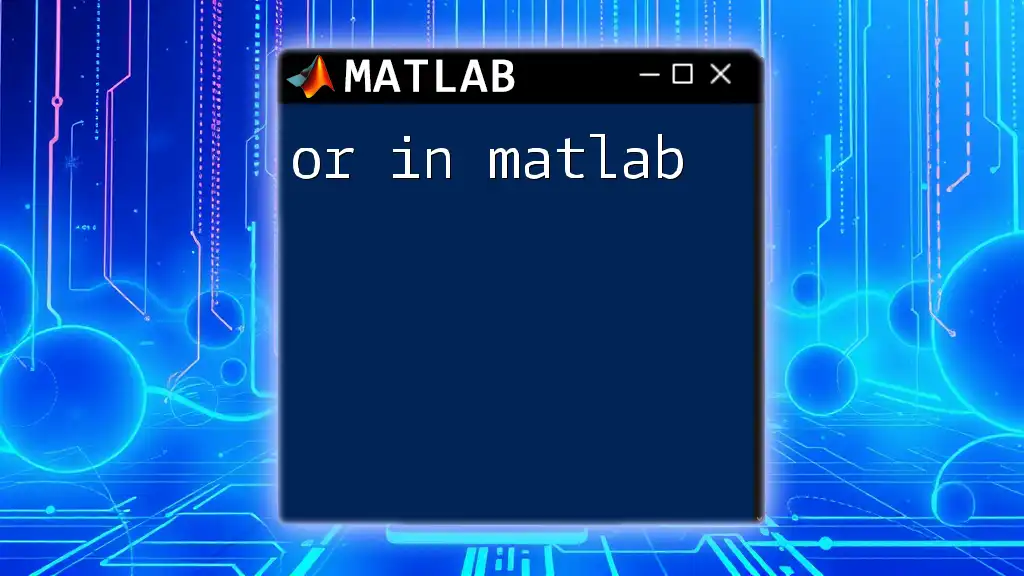
Debugging and Error Handling
Common Errors in MATLAB
Understanding Error Messages
Encountering errors is part of coding. In MATLAB, error messages can guide you toward resolving issues, from syntax errors to runtime errors. For example, an undefined function error suggests you are calling a function that hasn't been defined or loaded.
Using the Debugger
The MATLAB debugging tool allows you to identify and solve problems efficiently. You can set breakpoints in your scripts to step through code line by line:
- In the Editor, click in the margin to set a breakpoint.
- Run your script; MATLAB will pause at the breakpoint, allowing you to inspect variable values.
This functionality is invaluable for tracking down issues quickly.
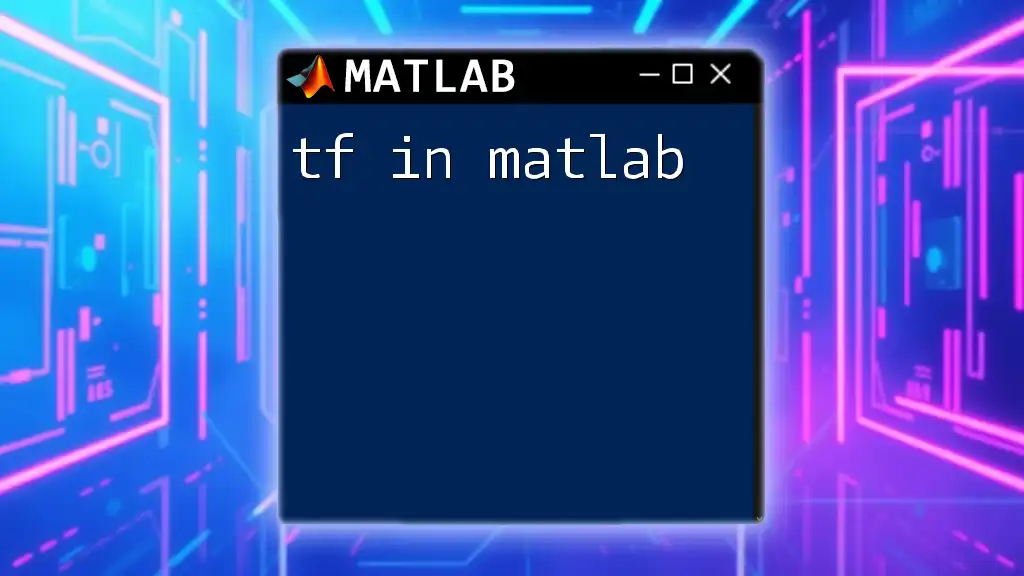
Best Practices in MATLAB
Code Optimization
Writing Efficient Code
Efficiency is key when working in MATLAB. Avoid using loops for tasks that can be vectorized. For instance:
% Using a loop
for i = 1:length(data)
result(i) = data(i)^2;
end
% Vectorized approach
result = data.^2;
The vectorized approach not only simplifies your code but also enhances performance dramatically.
Code Readability
Commenting and Structuring Code
Writing clear and well-commented code is essential for maintaining and understanding your scripts later. Use comments to explain the purpose of complex sections of your code:
% This function computes the square of input values
function result = computeSquare(data)
result = data.^2;
end
By keeping your code organized and documented, you make it easier for yourself and others to work with.
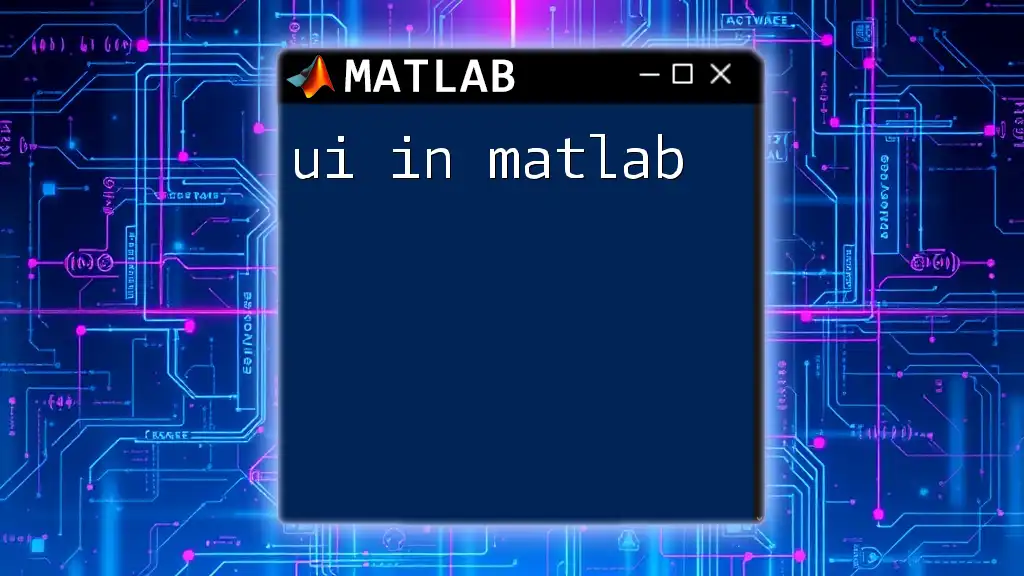
Conclusion
In conclusion, understanding how to use MATLAB efficiently opens up a world of possibilities in data analysis, engineering simulations, and mathematical modeling. By mastering the commands and features presented in this guide, you empower yourself to harness MATLAB’s full potential, whether you're a novice or looking to refine your skills.
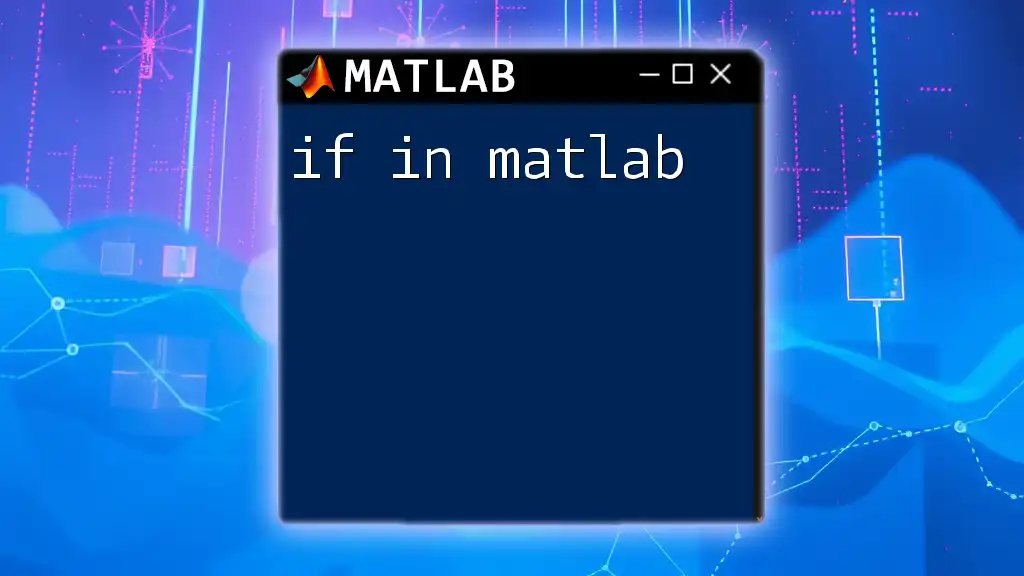
Call to Action
We encourage you to explore the topics discussed here further. Dive into the documentation, practice coding, and don't hesitate to reach out with questions or comments. Join our workshops to deepen your understanding and become proficient in MATLAB!