The `log10` function in MATLAB computes the base-10 logarithm of an array of values, returning the logarithm of each element in the input array.
x = [10, 100, 1000]; % Input array
y = log10(x); % Calculate base-10 logarithm
disp(y); % Display results
Understanding the log10 Function
What is log10?
The `log10` function in MATLAB computes the base-10 logarithm of a given input. This mathematical operation is fundamental, particularly in fields like engineering, signal processing, and data analysis. It helps measure the relative magnitude of numbers on a logarithmic scale, which is crucial for handling a wide range of values, particularly large data sets.
Why Use log10 in MATLAB?
MATLAB’s built-in functions, including `log10`, are optimized for efficiency and speed, offering precise computations with minimal user effort. This function is widely applicable, from converting power measurements into decibels in signal processing to normalizing data for statistical analysis. Using built-in functions allows users to avoid coding complex mathematical operations from scratch, saving time and reducing potential errors.
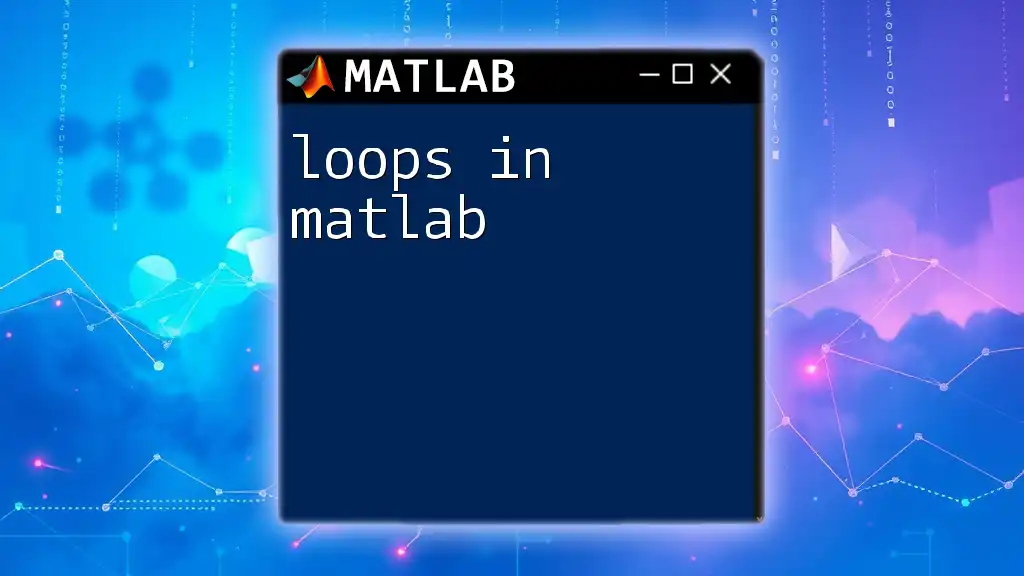
Getting Started with log10 in MATLAB
Syntax of log10
The basic syntax to use `log10` in MATLAB is straightforward. You simply pass your input value(s) into the function:
y = log10(x);
Where:
- x: This can be a scalar, vector, or matrix representing the input value(s).
- y: The output value(s) after applying the base-10 logarithm.
Input Types for log10
One of the strengths of MATLAB is its ability to handle different types of inputs seamlessly:
Scalar Input
When using `log10` with scalar values, the output is a single logarithmic value:
log10(100); % Returns 2
Vector Input
You can also apply `log10` to vectors. The function will return a vector of logarithmic values corresponding to each element:
log10([10, 100, 1000]); % Returns [1, 2, 3]
Matrix Input
Similarly, the function can handle matrices. Each element will be processed to yield a matrix of logarithmic values:
log10([1, 10; 100, 1000]); % Returns [0, 1; 2, 3]
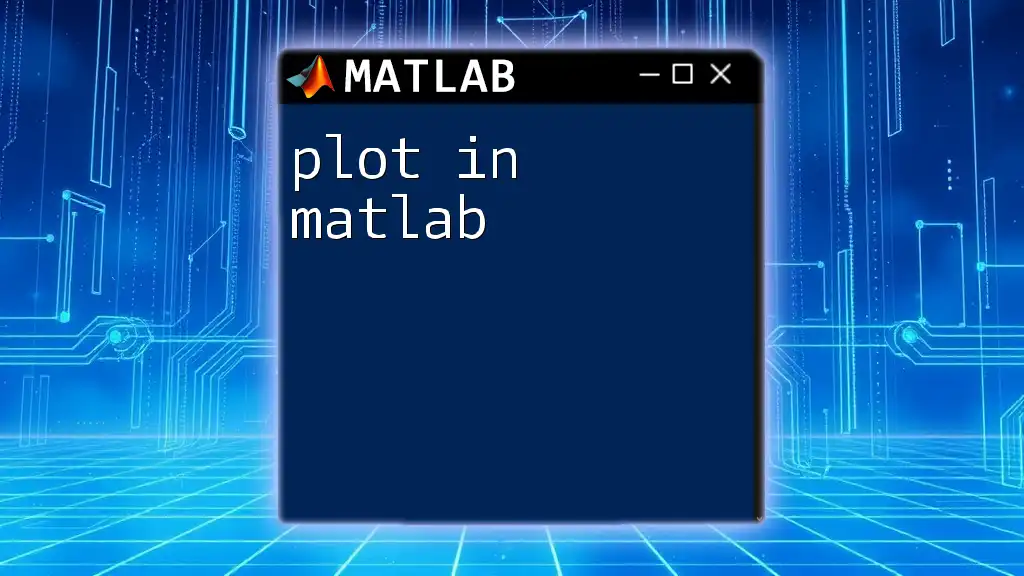
Practical Applications of log10 in MATLAB
Data Normalization
Logarithmic transformations using `log10` play a significant role in normalizing data, especially when dealing with right-skewed distributions. For instance:
data = [10, 100, 1000];
normalized_data = log10(data);
% Display: normalized_data will be [1, 2, 3]
By transforming the data, it becomes easier to visualize patterns and relationships that might not be apparent in the original values.
Signal Processing
In the realm of signal processing, `log10` is instrumental for converting power levels to decibels (dB), a logarithmic scale that represents sound intensity. The formula to convert power to decibels is:
power = 100; % Watts
db = 10 * log10(power); % Converts power to decibels
Using `log10` in this context allows for a more manageable representation of large variations in power levels.
Understanding Growth Patterns
In data analysis, logarithmic scales can help visualize growth patterns, such as population growth or financial forecasts. For instance, if you’re observing exponential growth, you can use `log10` to depict the data:
x = 1:10;
y = 10.^x; % Exponential growth
plot(x, log10(y)); % Logarithmic scale
title('Logarithmic Representation of Exponential Growth');
xlabel('X-axis');
ylabel('log10(Y)');
This approach highlights trends and patterns that can be obscured in a standard linear representation.
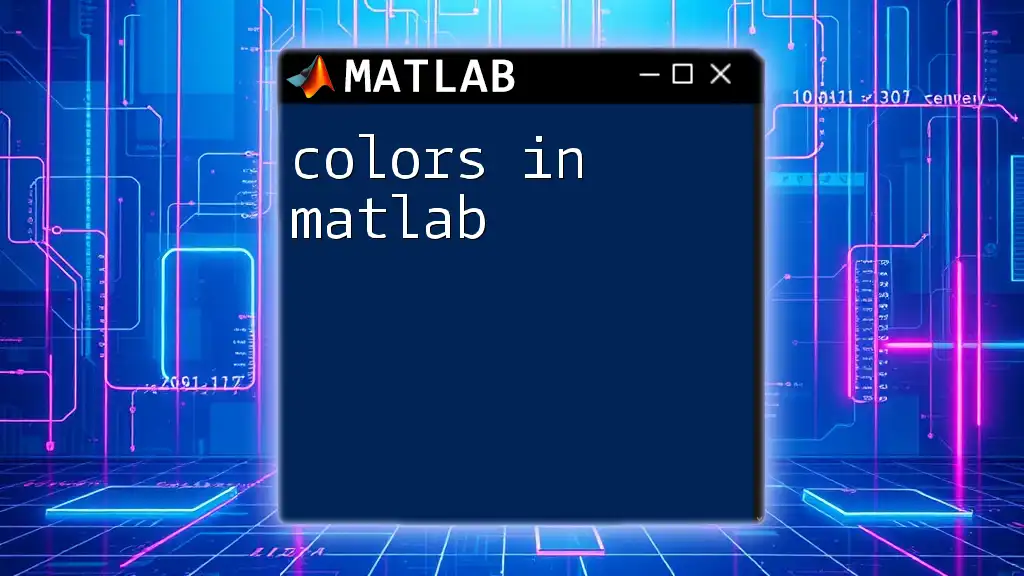
Error Handling with log10
Dealing with Negative Values
One crucial aspect of using logarithmic functions is handling invalid inputs. The logarithm of any negative number is undefined, which can lead to computational errors. To manage this, you can implement error handling:
value = -10;
if value <= 0
error('Input must be positive for log10.');
else
result = log10(value);
end
This code ensures that the input is valid before attempting to compute the logarithm, thus enhancing the robustness of your MATLAB scripts.
Avoiding Complex Results
It's common to face situations where the logarithm of zero is also undefined. Implementing checks helps avoid unintended results:
value = 0; % Logarithm of zero is undefined
if value <= 0
warning('Input must be greater than zero. Result will be NaN.');
result = NaN;
else
result = log10(value);
end
This example illustrates best practices for ensuring input validity, minimizing errors during calculations.
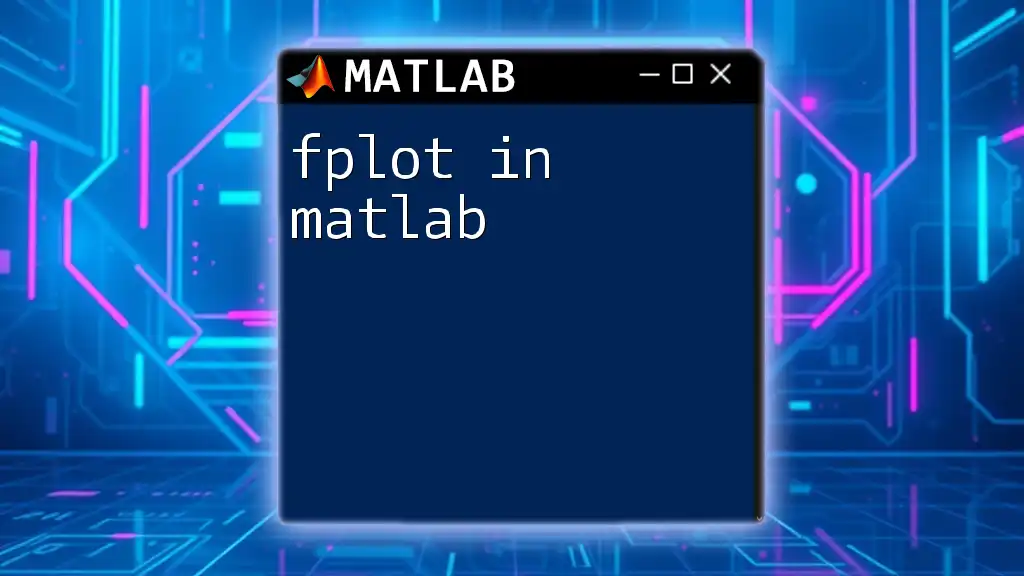
Visualizing Outputs
Using MATLAB Graphics to Illustrate log10 Function
MATLAB is equipped with robust graphics capabilities, which you can use to visualize the outputs of `log10`. For instance, plotting the `log10` function can provide insights into its behavior across a range of values:
x = linspace(0.1, 100, 100); % Avoid zero to prevent domain error
y = log10(x);
plot(x, y);
title('Plot of log10(x)');
xlabel('x');
ylabel('log10(x)');
grid on;
This code generates a clear graph that illustrates how `log10` behaves over a specified domain, enhancing understanding and interpretation of the logarithmic function.
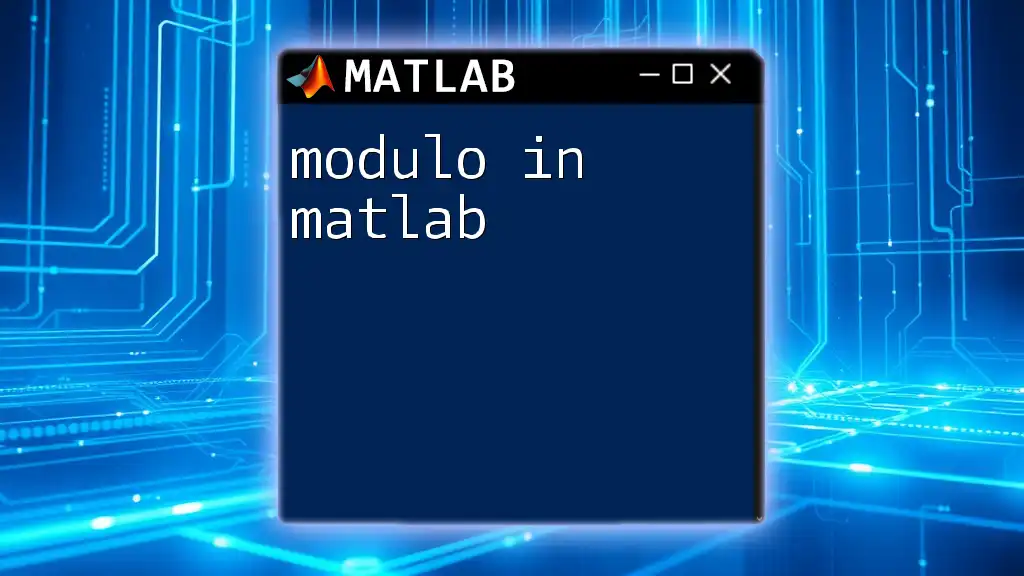
Conclusion
Key Takeaways
The `log10 in MATLAB` function is an essential mathematical tool, facilitating various applications, from data normalization to signal processing. Its versatility enables efficient handling of large data sets and enhances the clarity of growth patterns. Furthermore, implementing error handling practices ensures robustness and reliability in computations. As you explore its applications, you can unlock new insights and better manage the complexities of mathematical modeling and data analysis.
Further Resources
For users looking to dive deeper into the topic, the official MATLAB documentation on mathematical functions provides comprehensive coverage and examples. Additionally, engaging with recommended books and online courses will further enhance your understanding of MATLAB, allowing you to master its powerful functions like `log10`.