The `lsim` function in MATLAB simulates the time response of a linear system to arbitrary inputs, using the system's state-space representation or transfer function.
Here’s a code snippet demonstrating its usage:
% Define a transfer function G(s) = 1 / (s^2 + 2s + 1)
num = [1]; % Numerator coefficients
den = [1 2 1]; % Denominator coefficients
sys = tf(num, den); % Create transfer function
% Define time vector and input signal
t = 0:0.01:10; % Time from 0 to 10 seconds
u = sin(t); % Input signal (sine wave)
% Simulate the system's response
lsim(sys, u, t);
xlabel('Time (s)');
ylabel('Response');
title('System Response to Input Signal');
Understanding the `lsim` Function in MATLAB
What is `lsim`?
The `lsim` function in MATLAB is a powerful tool used for simulating the time response of a linear time-invariant (LTI) system to arbitrary inputs. Unlike other functions like `step` or `impulse`, which are used for specific types of input signals (step and impulse functions, respectively), `lsim` provides the flexibility to explore how a system responds to any input signal. This ability to simulate various scenarios makes `lsim` invaluable in fields such as control systems design, signal processing, and system dynamics analysis.
Key Terminology
Before diving into `lsim`, it’s essential to understand some foundational terms:
-
State-space representation: This is a mathematical representation of a system in terms of first-order differential equations. It consists of matrices that define the input, output, and state equations of the system.
-
Transfer function: A transfer function represents the relationship between the input and output of a system in the frequency domain. It is defined as the ratio of the Laplace transform of the output to the Laplace transform of the input.
-
Input and output signals: Understanding what types of input signals can be applied and how the system output is generated is crucial for utilizing `lsim` effectively.
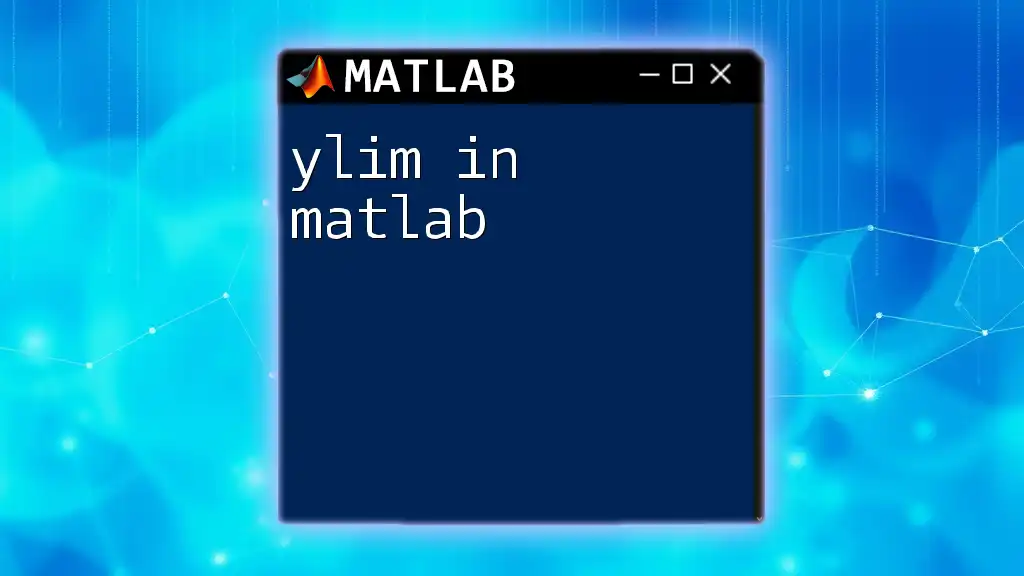
Syntax of `lsim`
General Syntax
The general syntax of `lsim` is as follows:
lsim(sys, U, T)
Where:
- sys is the state-space or transfer function model.
- U is the input signal vector.
- T is the time vector over which the response is calculated.
Parameter Descriptions
When using `lsim`, it is important to provide the correct types of inputs:
-
sys: This can be defined using either a state-space representation or a transfer function. Understanding how to represent your system correctly will determine how effectively you can simulate its response.
-
U: The input signal can take various forms, including constant inputs, step inputs, sinusoids, or any user-defined function. It should be structured as a column vector that correlates with the time vector `T`.
-
T: This time vector defines the duration over which you wish to observe the system's response and must have the same length as the input signal `U`.
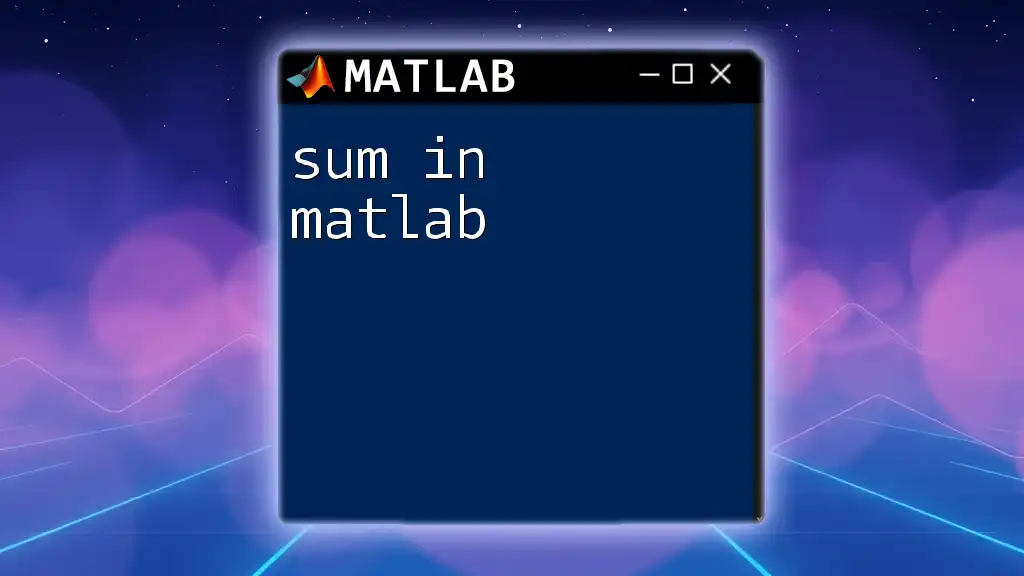
Examples of Using `lsim`
Example 1: Simple First-Order System
To illustrate the use of `lsim`, let’s consider a simple first-order linear system.
System Definition
We can define a first-order system with the following transfer function parameters:
num = [1];
den = [1, 1];
sys = tf(num, den);
In this code, `num` defines the numerator of our transfer function, while `den` defines the denominator.
Using `lsim`
To simulate the response of this first-order system, we can use unit step input over a time vector defined as:
t = 0:0.1:10; % time vector
u = ones(size(t)); % input signal
lsim(sys, u, t);
In this example, `u` is a vector of ones, representing a step input. The output of `lsim` will show how the system responds over 10 seconds to this constant input.
Example 2: Second-Order System with Damping
Let’s now examine a second-order system to add complexity to our understanding.
System Definition
To define a second-order damped system, we can use the following code:
num = [1];
den = [1, 3, 2];
sys = tf(num, den);
Here, the system is characterized by a damping ratio that influences its response.
Using `lsim`
Next, we will simulate the system's response to a sinusoidal input:
t = 0:0.01:10; % time vector
u = sin(t); % sinusoidal input
lsim(sys, u, t);
This code simulates how the system behaves when subject to a sinusoidal forcing function. The output plot will illustrate the natural frequencies and damping effects inherent to this second-order system.
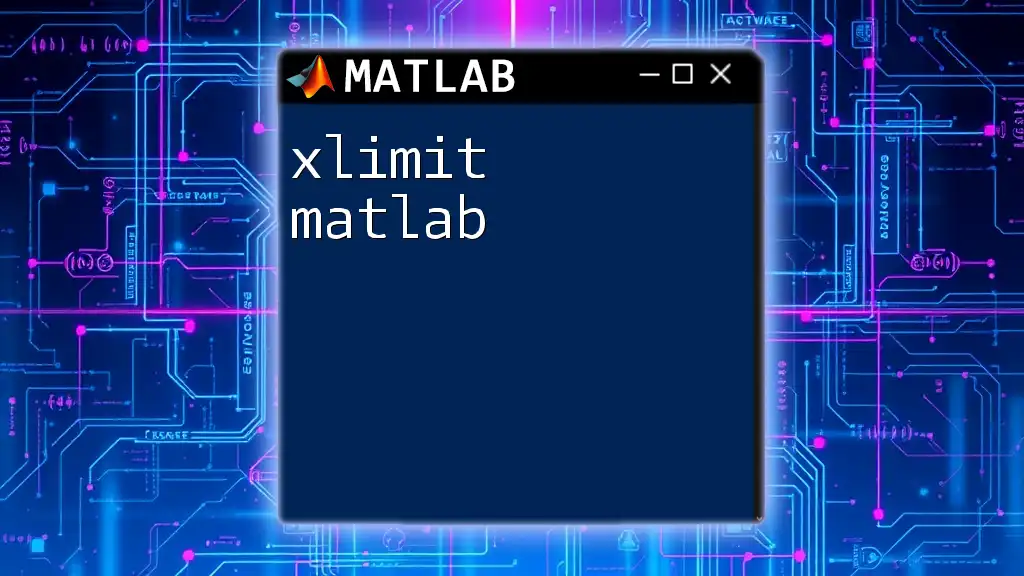
Analyzing the Output of `lsim`
Understanding the Graphical Output
After running `lsim`, MATLAB generates a plot displaying the system response over the selected time vector. The shape of the output is intimately tied to the form of the input signal.
For instance, with a step input, you generally expect a gradual approach to a steady state, whereas sinusoidal inputs will produce oscillatory behavior dependent on the system’s damping characteristics. By carefully analyzing these outputs, one can gain insights into the dynamic behavior of the system under various conditions.
Common Issues and Troubleshooting
Users may encounter certain common issues when using `lsim`:
-
Time vector mismatches: Ensure that the length of the time vector `T` matches the length of input signal `U`. A mismatch will lead to errors.
-
Improper scaling or non-physical responses: If the output responses seem unrealistic (such as unbounded growth), double-check your system definition and input specifications. Ensuring the system is defined for stable dynamics is crucial.
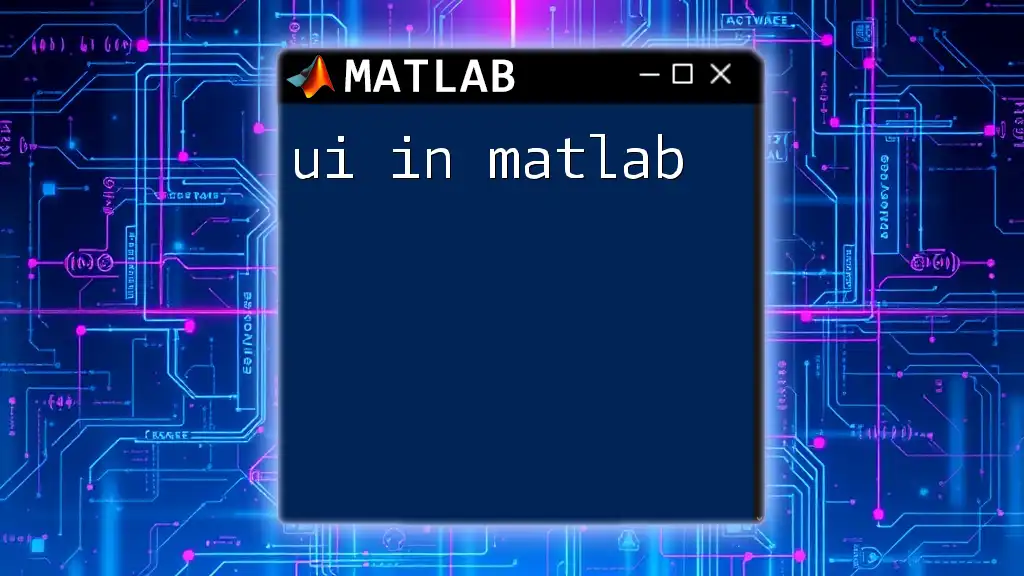
Advanced `lsim` Features
Plotting Options
MATLAB’s plotting capabilities can enhance the visualization of the `lsim` output. You can customize your plots with commands like `title`, `xlabel`, and `ylabel`. For example:
lsim(sys, u, t);
title('System Response to Sinusoidal Input');
xlabel('Time (seconds)');
ylabel('Output Response');
These additional plot settings allow for a clearer understanding and presentation of results.
Utilizing `lsim` with Multiple Inputs
Another powerful feature of `lsim` is its ability to handle multiple input signals. If you have multiple input vectors, they can be arranged as follows:
U = [u1; u2]; % stack two input signals
lsim(sys, U, T);
This allows you to simulate how a system behaves with various concurrent inputs, facilitating a better understanding of combined signal effects.
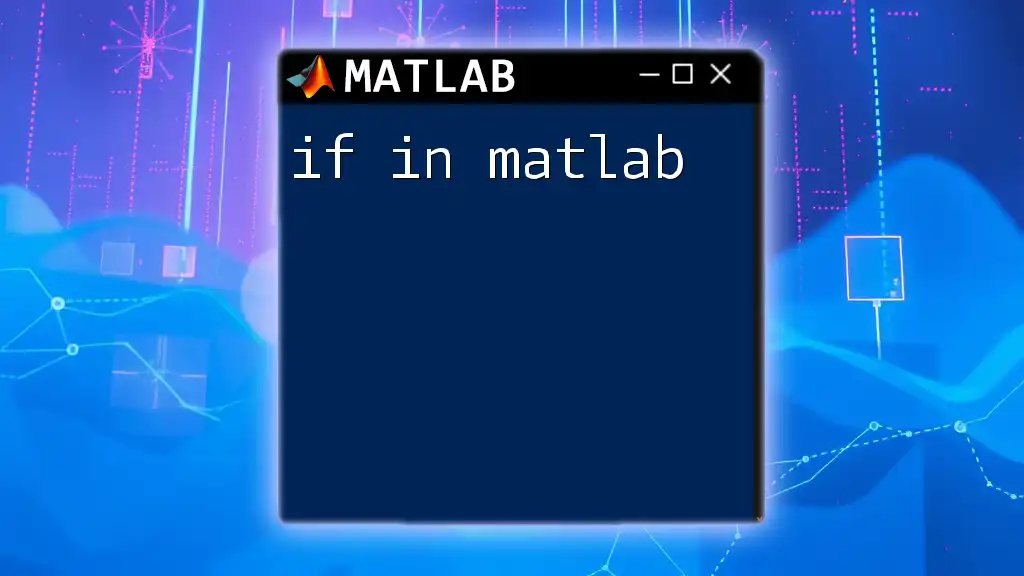
Conclusion
The `lsim` function in MATLAB is a versatile and essential tool for simulating the response of linear time-invariant systems to arbitrary inputs. By understanding its syntax and functionalities, users can effectively analyze system behaviors across a range of scenarios. Practice with different systems and inputs will further enhance your proficiency with `lsim`, enabling you to tackle real-world applications in control systems and beyond.
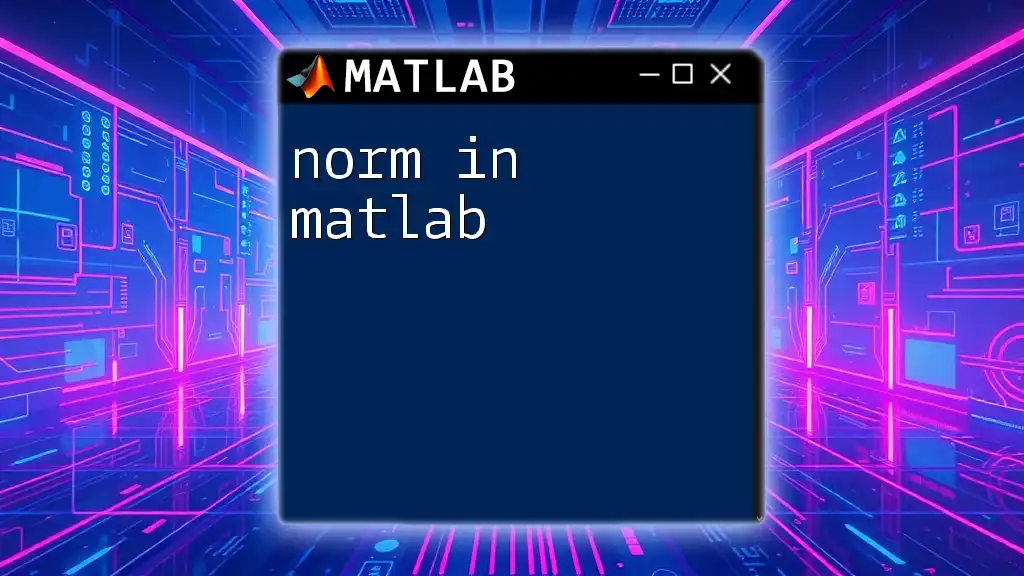
Additional Resources
Recommended MATLAB Documentation
For further exploration and detailed examples, check the official MATLAB documentation on `lsim`. This invaluable resource offers extensive insights and code examples that can deepen your understanding.
Community and Forums
Engage with the MATLAB community through platforms like MATLAB Central, where you can ask questions, share insights, and learn from the experiences of others in the field.