In MATLAB, the `or` operator performs element-wise logical OR operations between two arrays or conditions, resulting in an output array where each element is true if at least one of the corresponding elements in the input arrays is true.
% Example of using the OR operator in MATLAB
A = [1, 0, 0; 0, 1, 1];
B = [0, 1, 0; 1, 0, 1];
C = A | B; % Element-wise logical OR
disp(C);
Understanding Logical Operators in MATLAB
In MATLAB, logical operators are crucial for constructing expressions that evaluate conditions, enabling programmers to perform decision-making based on data. Logical operators help in assessing whether certain criteria or conditions are met, providing the backbone for many functions, loops, and conditional statements in your scripts.
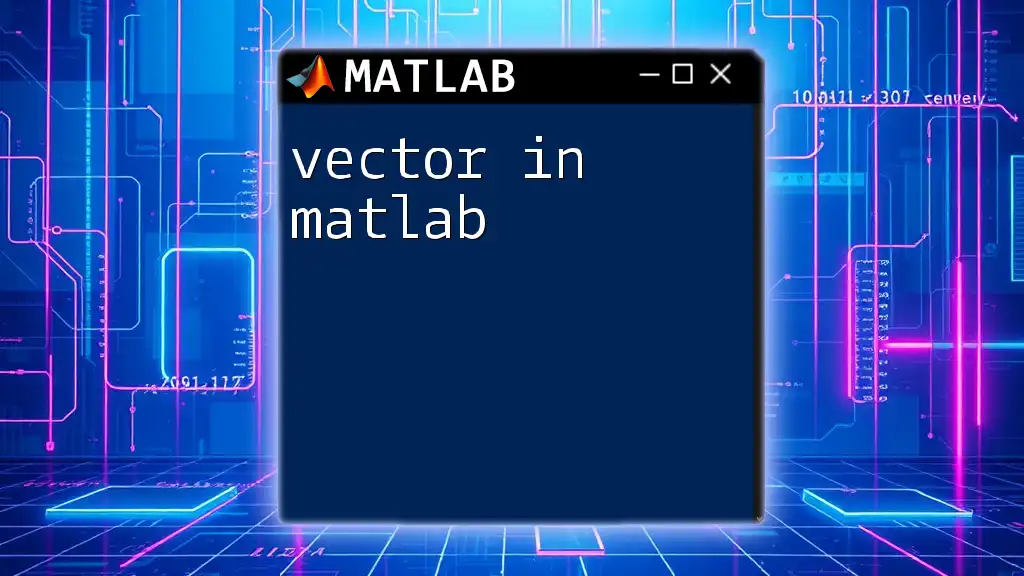
The 'or' Operator in MATLAB
The 'or' operator is used to evaluate two or more conditions and returns true if at least one of the conditions is true. This operator plays a vital role in logical comparisons, allowing you to create versatile and flexible conditional statements, commonly found in data validation and manipulations.
Syntax of the 'or' Operator
The syntax for the 'or' operator can vary based on its intended use — whether it's for scalar conditions or for working with arrays.
Here’s a basic syntax example:
result = a || b; % Returns true if either a or b is true
Types of 'or' Operators
Logical 'or' (||)
The logical 'or' operator is primarily used within conditional statements. It evaluates two conditions but stops evaluating as soon as it finds one that evaluates to true. This is known as short-circuiting.
For example, consider this code snippet:
if (x > 10 || y < 5)
disp('Condition met.');
end
In this example, if either `x` is greater than `10` or `y` is less than `5`, the message "Condition met." will be displayed. The advantage of using the logical 'or' is that it can help prevent unnecessary evaluations, especially if the second condition might be computationally expensive.
Element-wise 'or' (|)
The element-wise 'or' operator operates on arrays or matrices, comparing corresponding elements and returning true for each instance where at least one of the elements is true.
Here’s how it works:
A = [1, 0, 1];
B = [0, 1, 1];
result = A | B; % Element-wise comparison
In this case, the `result` will yield `[1, 1, 1]` because any corresponding pair of elements results in `true` for the first and third elements.
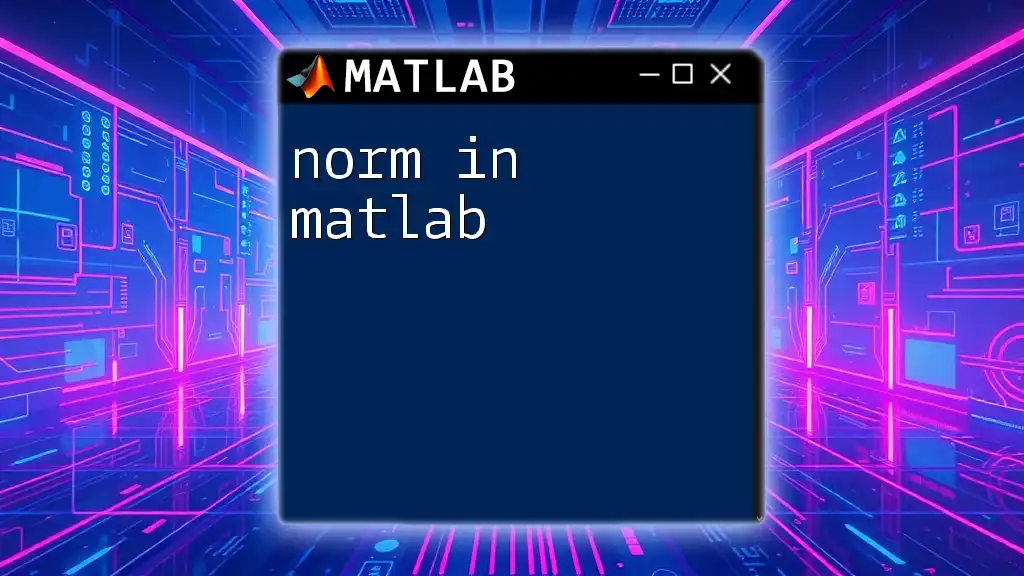
Comparison of Logical and Element-wise Operations
When using 'or' in MATLAB, it's important to know when to use each type of operator. Use `||` when dealing with scalar conditions, especially within control flow statements, and use `|` for operating on entire arrays.
Essentially:
- Use `||` for single, discrete logical evaluations.
- Use `|` when performing operations on matrices or arrays in bulk.
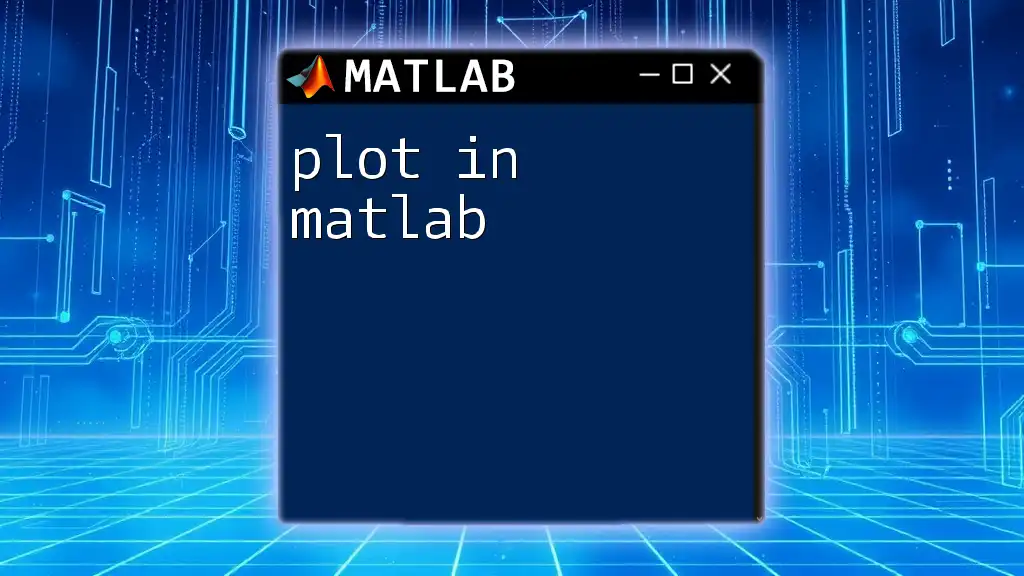
Practical Examples
Example 1: Filtering Data with 'or'
Logical operations allow you to filter datasets effectively. Using the 'or' operator can help extract elements from a vector that meet any of several conditions.
Here’s an example that filters out values:
data = [1, 3, 5, 7, 9];
filteredData = data(data < 3 | data > 7); % Returns [1, 9]
In this snippet, we want to capture elements that are less than `3` or greater than `7`. The resulting `filteredData` will include those specific values.
Example 2: Conditional Operations
You can also leverage the 'or' operator in loops and control structures to enhance your algorithm's logic. Consider this example:
for i = 1:10
if (mod(i,2) == 0 || i > 5)
disp(['Value: ', num2str(i)]);
end
end
In this loop, the code checks if the number is even (i.e., `mod(i,2) == 0`) or if it is greater than `5`. For numbers that meet either of these conditions, it prints the relevant value.
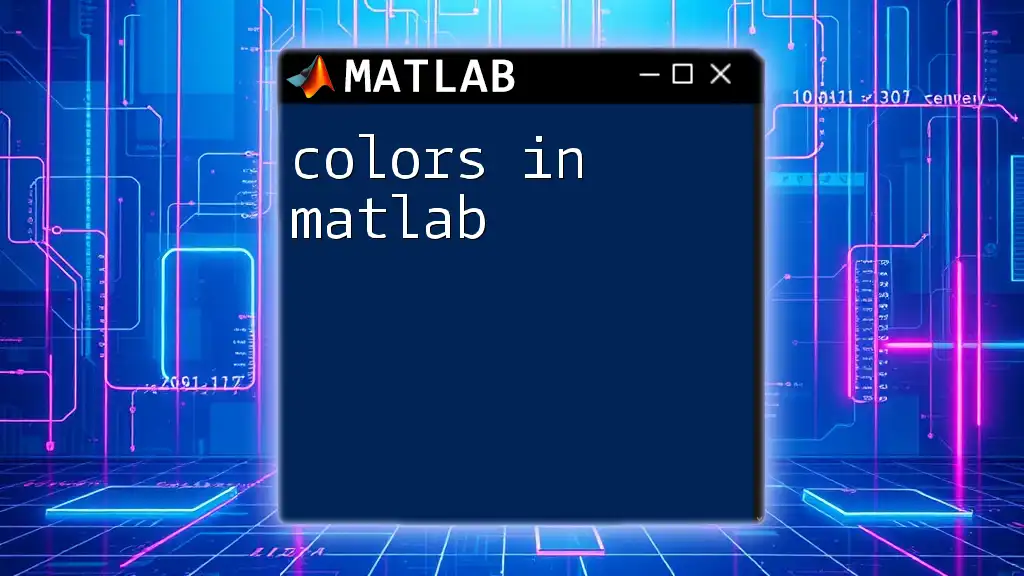
Common Mistakes to Avoid
Misunderstanding Logical 'or' Operator
One common mistake is misunderstanding how the logical 'or' operator handles evaluation. Due to its short-circuiting behavior, once one condition evaluates to true, the second is not evaluated. This can lead to unexpected results if the second condition includes function calls that do not execute.
Incorrect Use of Element-wise 'or'
When using the element-wise 'or' operator, ensure that the matrices or arrays being compared are of compatible dimensions. Mismatched dimensions can lead to runtime errors. Always verify that the sizes of both operands are the same before applying the element-wise operator.
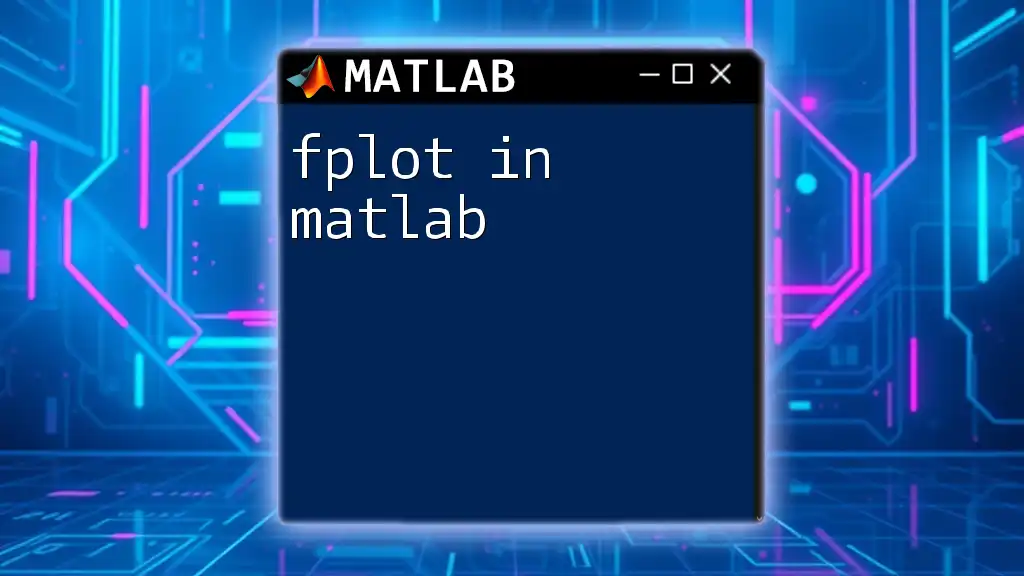
Best Practices for using the 'or' Operator
-
Readability in Code: Keep your code neat and readable. Clear logical expressions improve maintainability and collaboration. Employing meaningful variable names can also help in understanding the intent behind conditions.
-
Combining with Other Logical Operators: Often, you might combine 'or' with other logical operators like 'and' (&&), providing even more complex evaluations. Use parentheses to clearly dictate the order of operations.
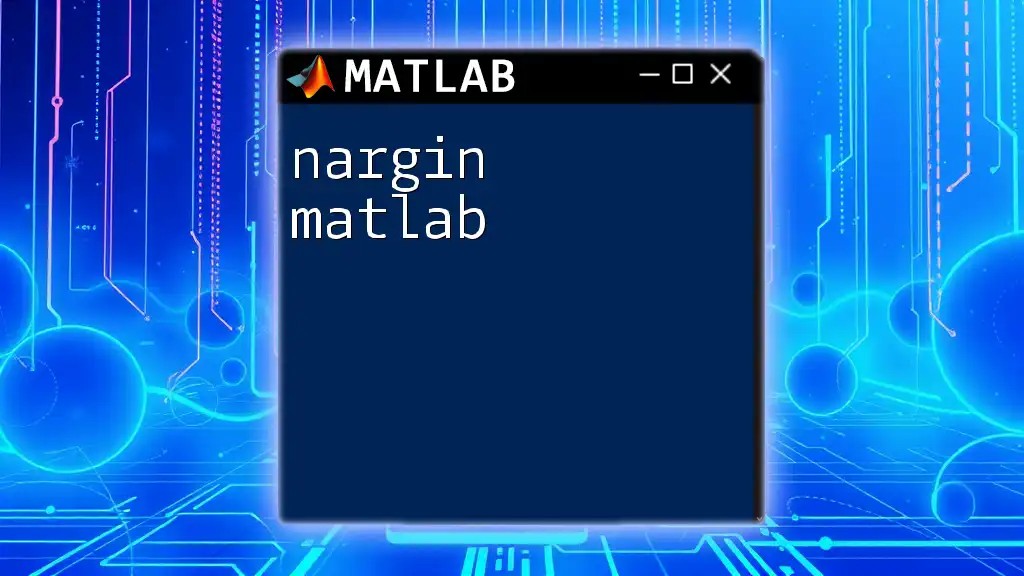
Conclusion
The 'or' operator in MATLAB is an essential tool for anyone looking to perform complex logical evaluations. Understanding how to effectively use both the logical `||` and element-wise `|` operators expands your coding capabilities, particularly when assessing conditions or manipulating data.
To truly master the 'or' operator, practice is key. Engage with the provided examples and try creating your own scenarios to solidify your comprehension of this powerful operator.
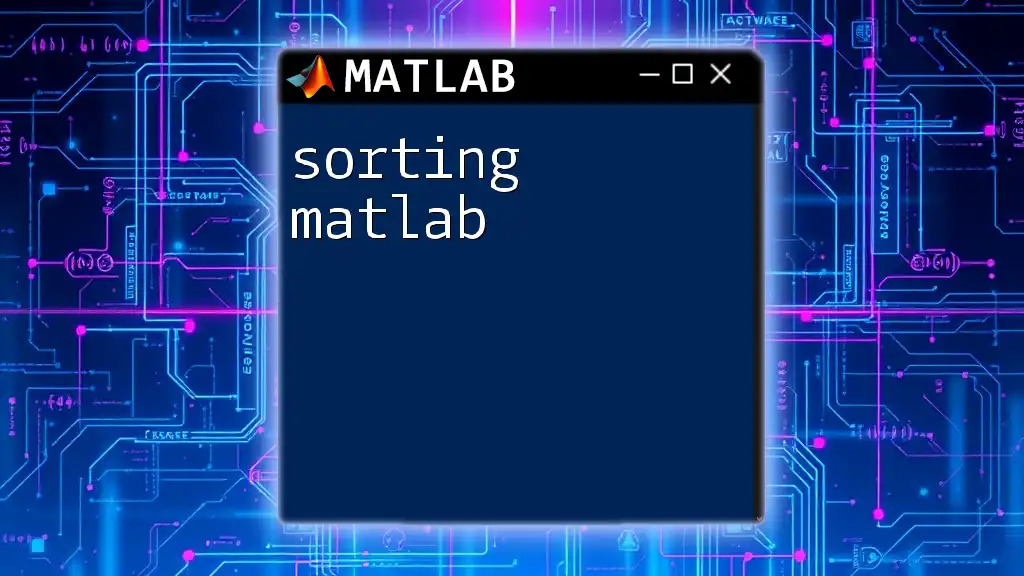
Additional Resources
For further reading, consult the official MATLAB documentation, which covers logical indexing and conditions comprehensively. Also, consider working through practice problems that entail filtering data and constructing multi-condition logic to enhance your skills. By continuously experimenting with 'or in MATLAB', you'll deepen your understanding and efficiency in MATLAB programming.