`fplot` in MATLAB is a function that allows you to plot a function easily by specifying its function handle and the range for the independent variable.
Here's a quick example using `fplot`:
fplot(@(x) sin(x), [-pi, pi]); % Plots the sine function from -π to π
What is fplot?
The fplot function in MATLAB is a powerful tool specifically designed for plotting functions. Unlike traditional plotting functions, fplot automatically selects appropriate points, providing a quick and effective way to visualize mathematical functions. It serves as an essential element in any MATLAB user's toolkit, especially for those interested in visualizing continuous functions without manually specifying each point.
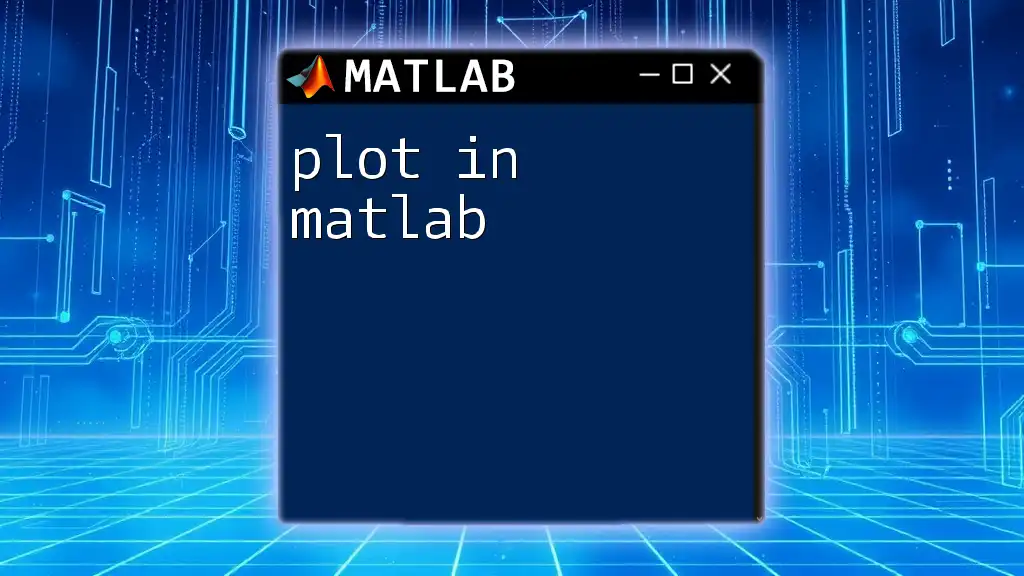
When to Use fplot
There are several situations where fplot shines:
- Quick Function Visualization: When you need to rapidly plot a function without worrying about the underlying points or intervals.
- Continuous Functions: Ideal for functions that are continuous over a specified range, ensuring smooth graphs without gaps.
- Complex Functions: Great for handling complex mathematical expressions, as fplot can process them efficiently.
While other plotting functions are useful, fplot provides a streamlined approach, particularly for mathematical functions. It outperforms functions like `plot` or `ezplot` when it comes to ease of use and flexibility.
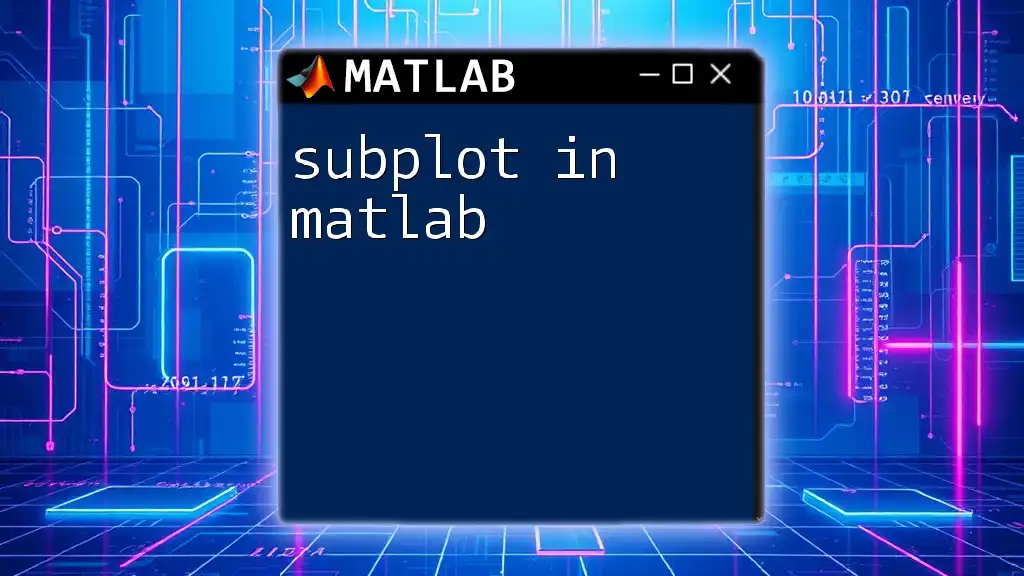
Basic Syntax of fplot
To use fplot, you should be familiar with its basic syntax:
fplot(fun, limits)
Parameters Explained
-
fun: This represents the function to be plotted. You can specify a function in several ways:
- Function Handles: The most common method, allowing you to reference any existing function directly.
- Anonymous Functions: A unique MATLAB feature, letting you define a function on-the-fly.
-
limits: This is a two-element vector defining the x-range for the plot, such as `[xmin, xmax]`. Setting appropriate limits is crucial for displaying the function accurately.
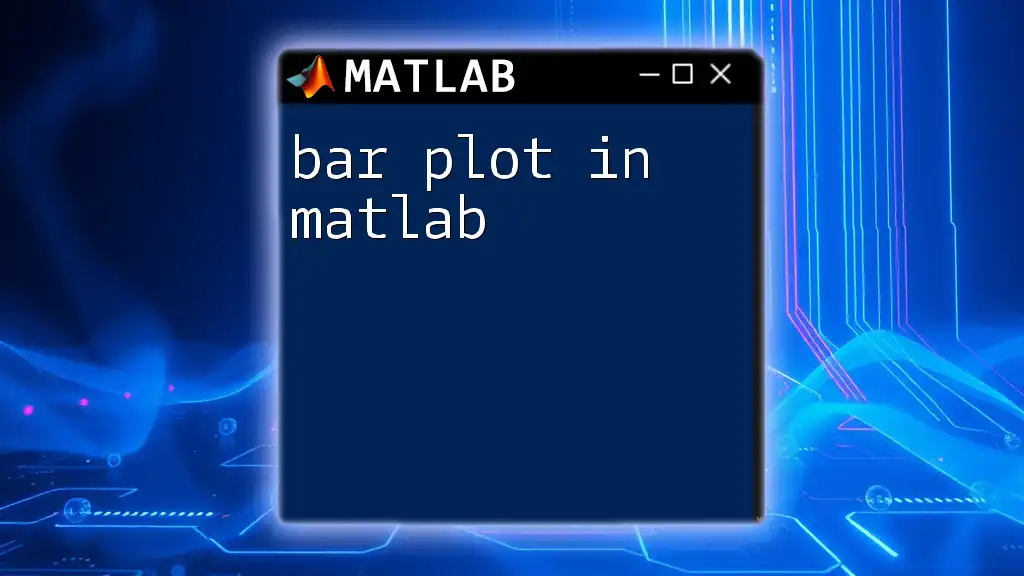
Plotting Basic Functions with fplot
Let's explore how to plot some basic functions using fplot.
Simple Examples
For instance, if you want to plot a linear function such as \( y = x \):
fplot(@(x) x, [-10, 10]);
In this example, we define the function using an anonymous function and specify the range from -10 to 10. The graph will depict a straight line passing through the origin, showcasing the linear relationship.
Another example is plotting a quadratic function like \( y = x^2 \):
fplot(@(x) x.^2, [-10, 10]);
This code produces a parabolic shape that opens upwards, demonstrating the characteristics of quadratic functions.
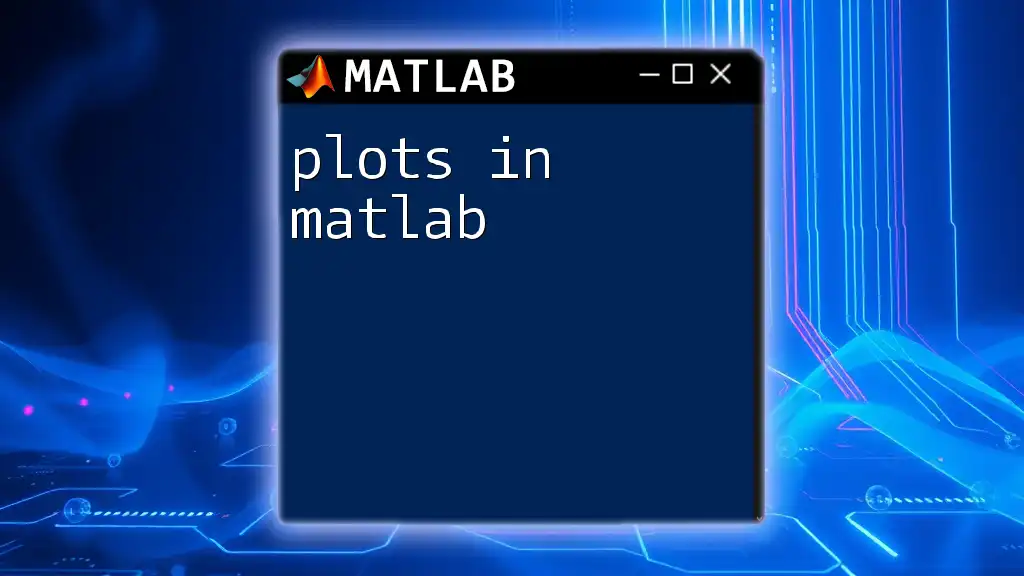
Customizing the fplot Output
To enhance your plots, MATLAB allows a range of customization options.
Line Properties
Color and Style
You can customize the color and style of your lines easily. For example, to plot a sine wave in red with a dashed line:
fplot(@(x) sin(x), [-2*pi, 2*pi], 'Color', 'r', 'LineStyle', '--');
This command not only produces the sine wave but also makes it visually appealing and easier to differentiate.
Line Width
Modifying the line width is straightforward. For instance, if you wish to plot the cosine function with a thicker line:
fplot(@(x) cos(x), [-2*pi, 2*pi], 'LineWidth', 2);
It’s critical not just for aesthetics but also for making your plots more readable.
Adding Titles and Labels
Enhancing your plot with titles and labels is essential for clarity. Here’s how to add a title and labels to your graph:
fplot(@(x) exp(x), [0, 5]);
title('Exponential Function');
xlabel('x');
ylabel('exp(x)');
This adds essential context to your graph, making it more informative for anyone viewing it.
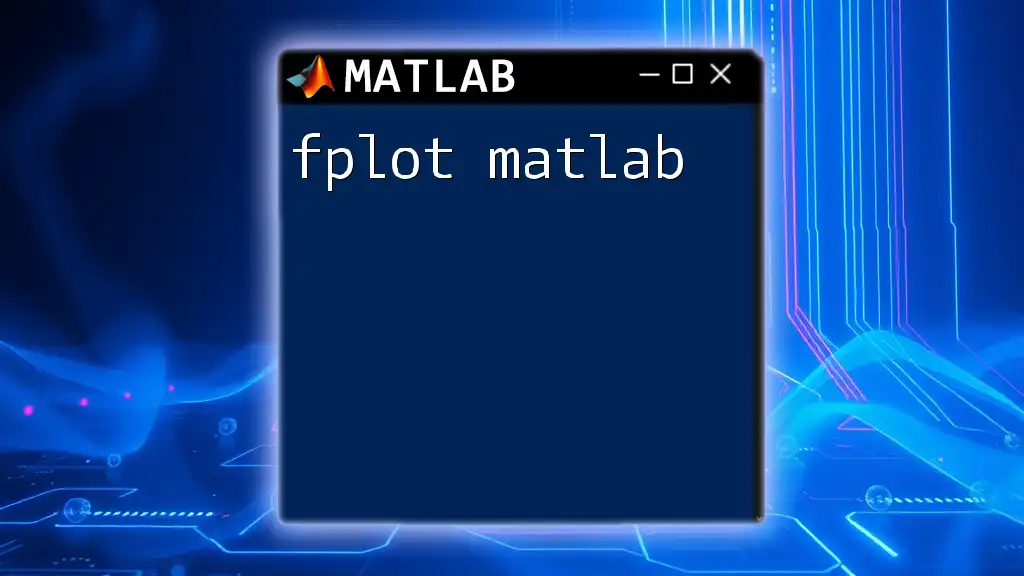
Combining Multiple Functions
Sometimes, you may want to plot multiple functions on the same axes.
Using Hold On to Overlay Graphs
The hold on command enables you to overlay different functions. Here’s an example:
fplot(@(x) sin(x), [-2*pi, 2*pi]);
hold on;
fplot(@(x) cos(x), [-2*pi, 2*pi]);
This results in both the sine and cosine functions being plotted on the same graph. It's vital for comparison and visualization of relations between different mathematical expressions.
Color-Coding Different Functions
To enhance clarity, you can color-code each function. By assigning different colors to the sine and cosine functions, you make them easily distinguishable to viewers.
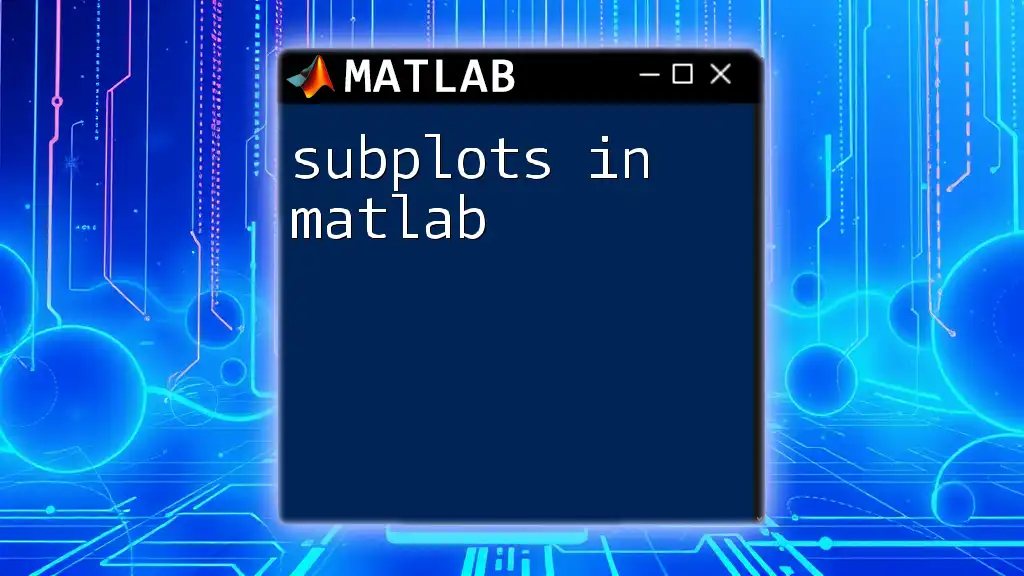
Advanced Features of fplot
MATLAB also provides advanced features for more control over your plots.
Specifying the Number of Points
Sometimes, the default sampling might not be adequate, especially for functions with rapid fluctuations. By using the NumPoints property, you can adjust this:
fplot(@(x) log(x), [0.1, 10], 'NumPoints', 100);
Increasing the number of points can result in a smoother and more accurate representation of the function.
Using Function Handles with Extra Parameters
If your function requires additional parameters, fplot handles this gracefully. For example:
fplot(@(x,a) a*x.^2, [0, 10], {'a', 1});
This flexibility allows you to create dynamic plots that can adjust based on varying parameters.
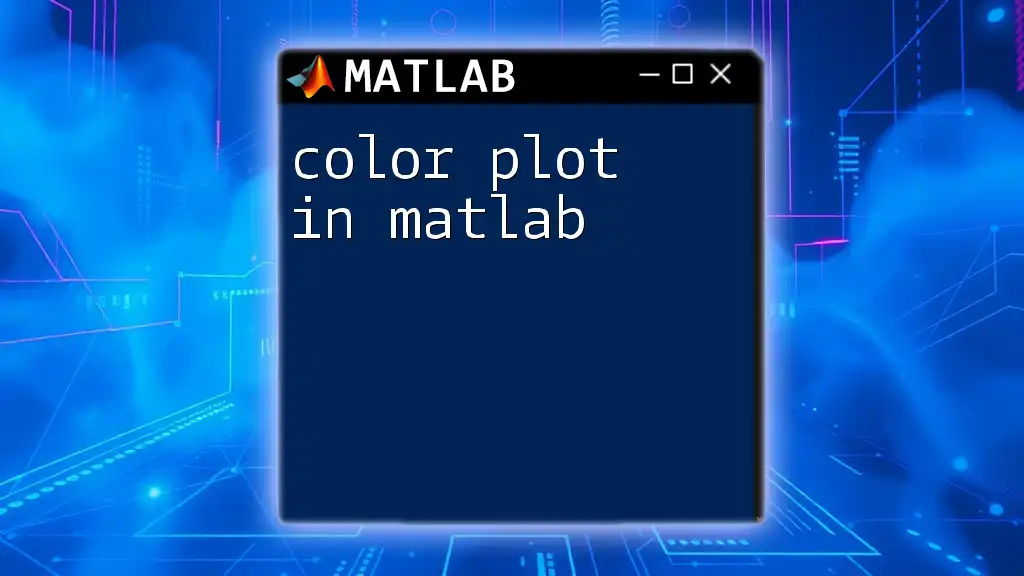
Error Handling and Troubleshooting
Even the best tools come with challenges. Here are some common errors users face when utilizing fplot:
Common Errors When Using fplot
- Invalid Function Handles: Ensure that your function handle correctly points to a valid function.
- Out-of-Bounds Limits: Specifying limits outside the function's domain can result in an empty graph.
Best Practices
To avoid issues, always double-check that function handles are defined properly, and ensure the limits are logical. Testing with simple functions initially can also help build confidence with the tool.
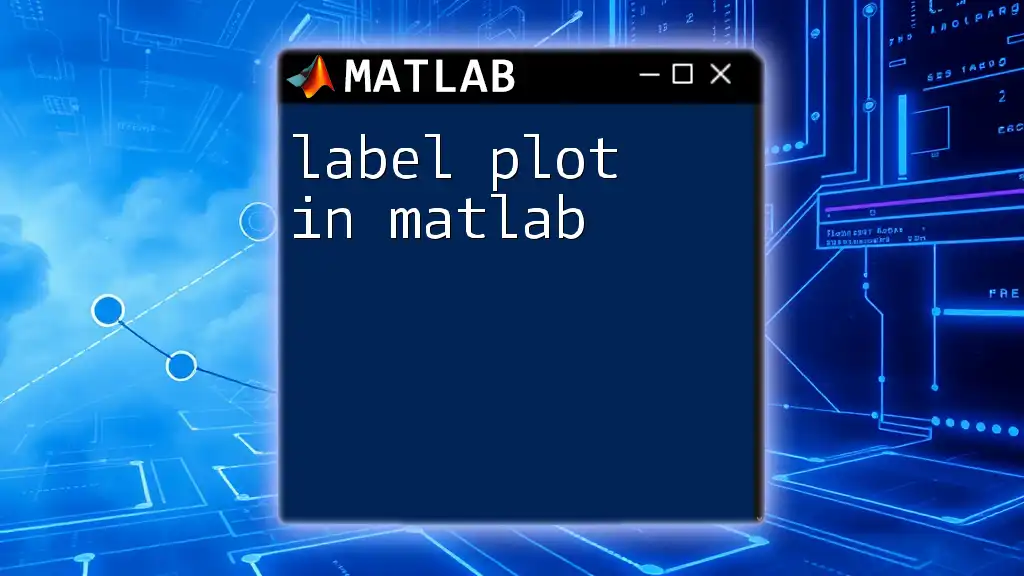
Conclusion
In recap, fplot in MATLAB is an invaluable function that simplifies the process of visualizing mathematical functions effortlessly. Its combination of ease of use, customization, and powerful features makes it a preferred choice for both novice and experienced users.
I encourage you to experiment with various functions, explore different customization options, and integrate fplot into your MATLAB projects. To deepen your understanding, consider exploring additional resources, documentation, and community forums available online.
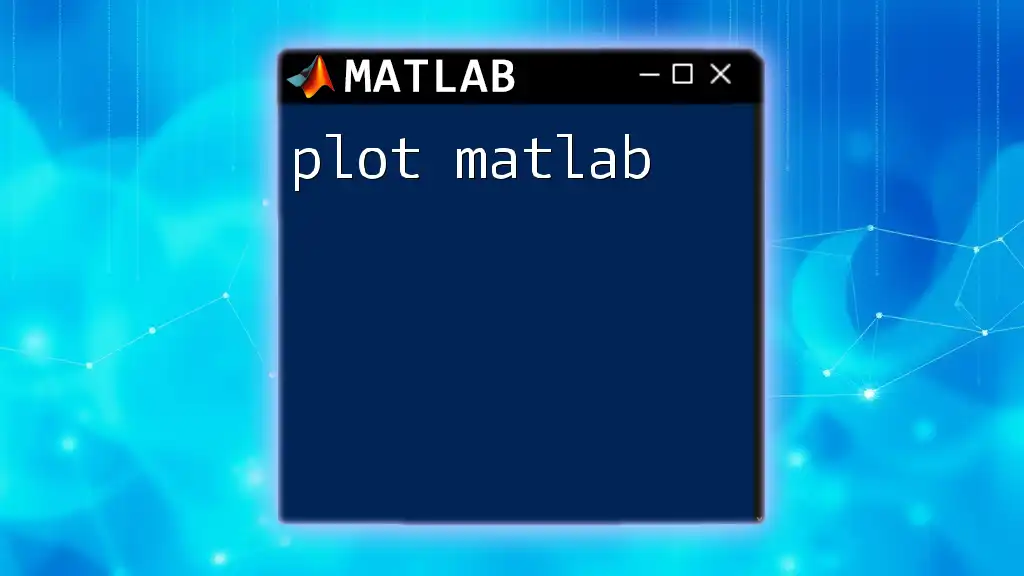
Call to Action
If you’re passionate about mastering MATLAB, join our upcoming workshop! We provide practical tips and hands-on exercises to help you become proficient in using MATLAB commands. Don't forget to subscribe to our newsletter for more insightful MATLAB tips and tricks straight to your inbox!