In MATLAB, the modulo operation is performed using the `mod` function, which returns the remainder after division of one number by another.
result = mod(10, 3); % This will set result to 1, since 10 divided by 3 leaves a remainder of 1
What is the Modulo Operation?
Definition of Modulo
The modulo operation represents the remainder of a division between two integers. Mathematically, it can be expressed as `a mod b`, where `a` is the dividend and `b` is the divisor. The result of this operation is the remainder when `a` is divided by `b`. For example, the expression `7 mod 3` would yield `1`, since 3 goes into 7 two times, leaving a remainder of 1.
Real-world Applications of Modulo
The modulo operation has a variety of practical applications in programming. Here are just a few:
- Even and Odd Number Checks: By using `mod`, you can easily determine if a number is even or odd. If `num mod 2` equals `0`, the number is even.
- Cyclic Operations: In scenarios where you need to loop or cycle through values (like animations or circular buffers), the modulo operation can help you wrap around to the beginning when exceeding the limits.
- Date and Time Calculations: When computing hours or minutes (e.g., handling time formats), modulo helps reset values back to zero after reaching a defined limit (e.g., 24 hours in a day).
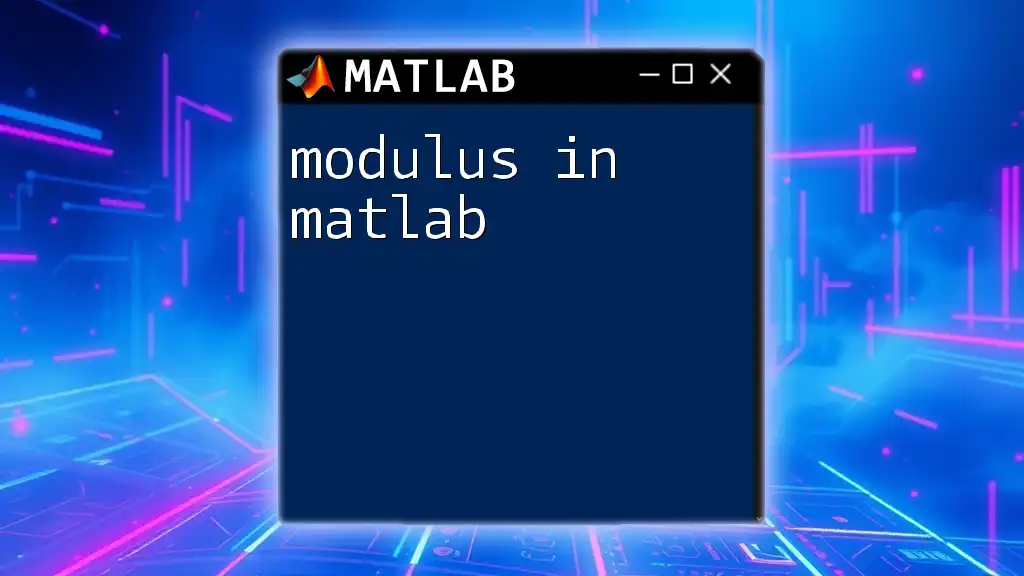
MATLAB and the Modulo Operation
Understanding Modulo in MATLAB
In MATLAB, the modulus is computed using the `mod` function. It’s important to note that MATLAB provides two functions for obtaining the remainder: `mod` and `rem`. The `mod` function always returns a non-negative remainder, while `rem` can yield negative results when the dividend is negative.
Basic Syntax of the Modulo Function
To use the modulo function in MATLAB, you would apply the following syntax:
r = mod(a, b);
Where:
- `a` represents the dividend,
- `b` signifies the divisor, and
- `r` is the resultant remainder.
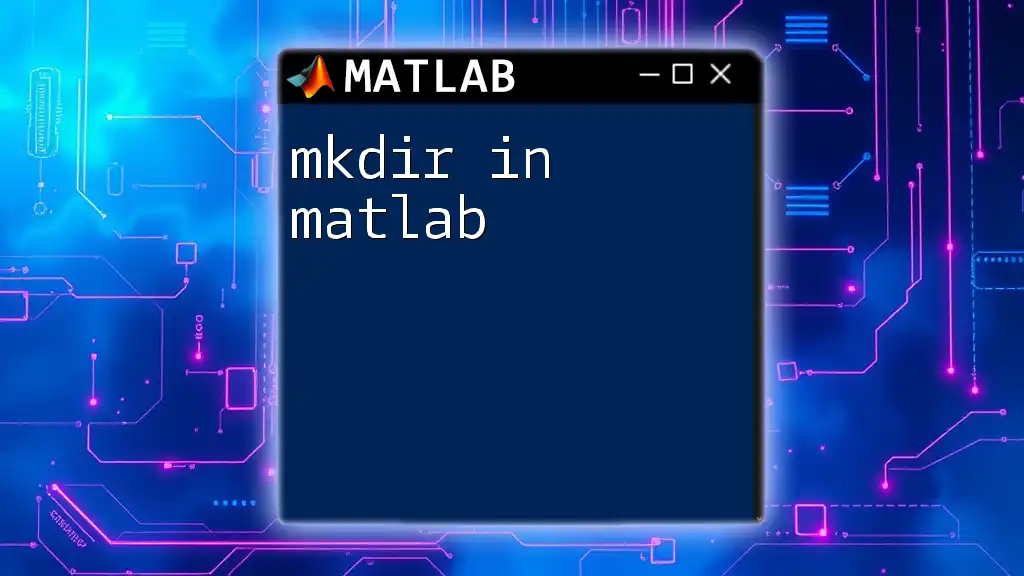
Practical Use Cases of Modulo in MATLAB
Example 1: Basic Modulo Calculation
Let's begin with a straightforward example:
a = 10;
b = 3;
result = mod(a, b);
disp(result); % Expected output: 1
In this case, `10 mod 3` yields a remainder of `1` because `3` fits into `10` three times, leaving a remainder of `1`. Understanding this fundamental example sets the stage for more complex applications.
Example 2: Checking Even or Odd Numbers
The modulo operation makes it simple to check if a number is even or odd:
number = 15;
if mod(number, 2) == 0
disp('Even');
else
disp('Odd');
end
When executing this code, it checks `15 mod 2`, which equals `1`, leading to the output of `Odd`. This technique can be applied across various scenarios where such checks are necessary.
Example 3: Circular Array Access
Circular arrays are a frequent requirement in programming. Here’s how modulo simplifies access:
array = [1, 2, 3, 4, 5];
index = 8; % Accessing the 8th element in a circular manner
circularIndex = mod(index-1, length(array)) + 1;
disp(array(circularIndex)); % Expected output: 3
In this example, the value of `index` is out of bounds for the `array` length of `5`. By applying modulo to wrap around, we can successfully access the `3rd` element.
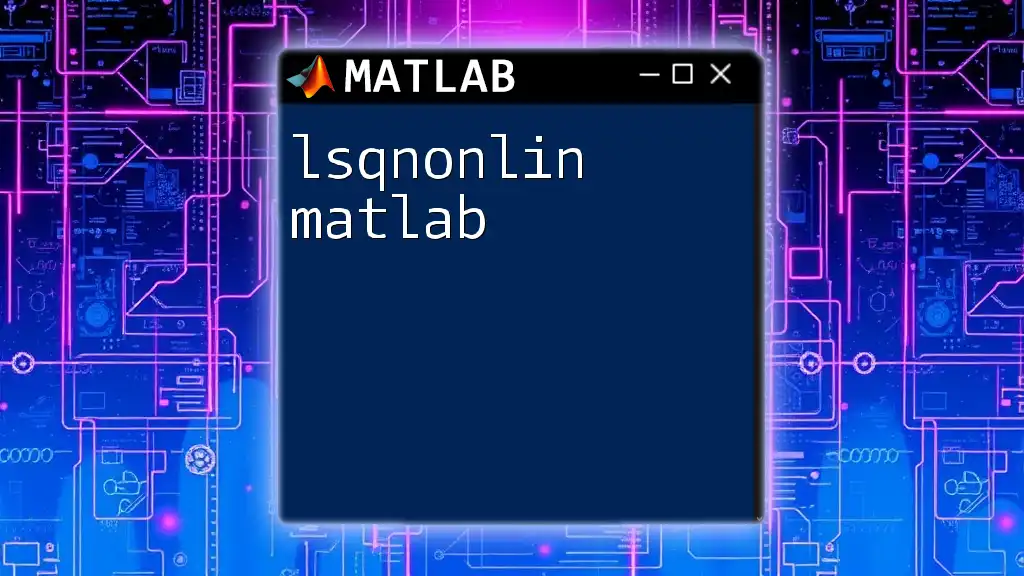
Using Modulo with Arrays
Vectorized Modulo Operations
MATLAB excels at handling operations on entire arrays simultaneously. Here’s how you can apply the modulo operation across a vector:
A = [10, 20, 30, 40];
B = 6;
result = mod(A, B);
disp(result); % Expected output: [4, 2, 0, 4]
This snippet calculates the remainder for each element in `A` when divided by `6`. The output `[4, 2, 0, 4]` illustrates the efficiency of vectorized computations.
Modulo with Complex Numbers
MATLAB also supports modulo calculations with complex numbers:
C = [3 + 4i; 5 + 12i];
D = 5;
result = mod(C, D);
disp(result);
The result will exhibit how MATLAB seamlessly handles complex numbers in the context of modulo operations, emphasizing its versatility.
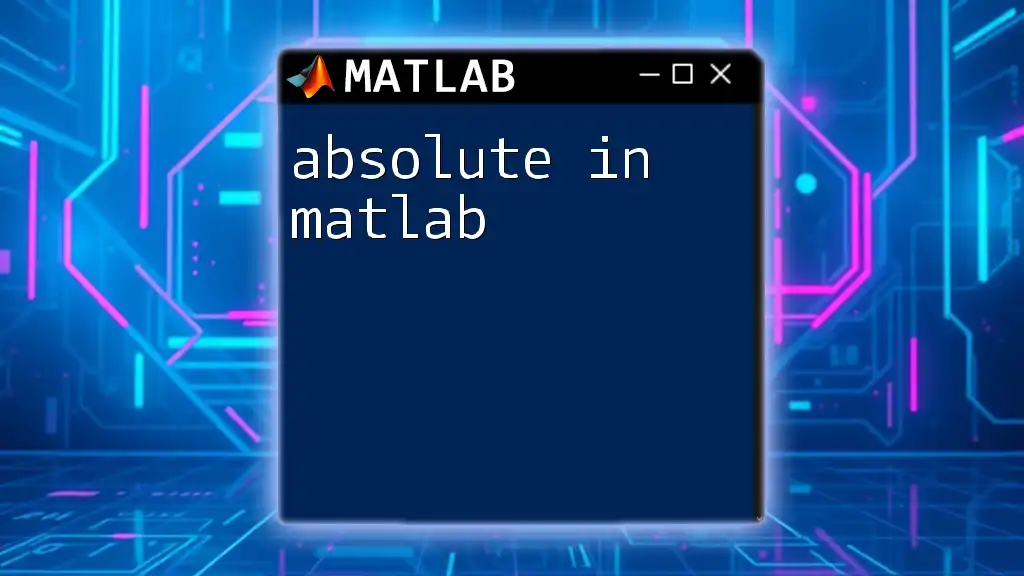
Advanced Topics
Comparing `mod` and `rem`
One important aspect of working with modulo in MATLAB is understanding the differences between `mod` and `rem`. The `mod` function always returns a non-negative result, while `rem` can yield a negative result if the dividend is negative. Understanding this distinction is crucial for predictable outputs.
Here's a direct comparison:
num1 = -5;
num2 = 3;
modResult = mod(num1, num2);
remResult = rem(num1, num2);
disp([modResult, remResult]); % Expected output: [1, -2]
In this example, `mod(-5, 3)` results in `1`, while `rem(-5, 3)` yields `-2`. Choosing the correct function based on your needs is essential.
MATLAB's Handling of Other Types
String and Cell Arrays
It's important to recognize that the `mod` operation does not apply to string or cell arrays. Instead, you can convert these types to numerical values first, or manipulate them using appropriate string functions if needed.
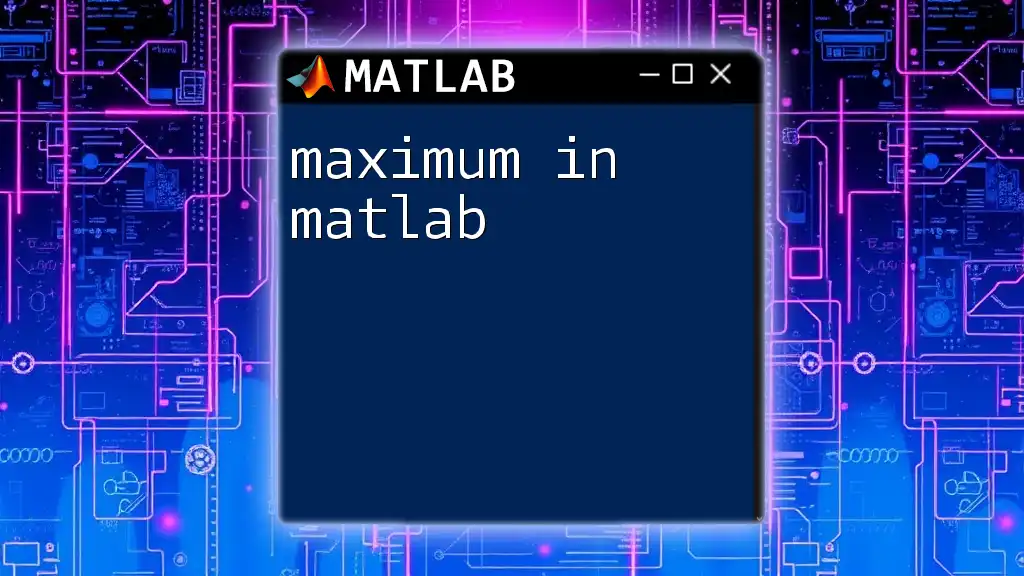
Common Mistakes and Troubleshooting
Not Considering Data Types
One common mistake programmers make is neglecting the data type of the numbers involved in the operation. Ensure that your variables are of numeric types before applying the `mod` function. Converting types might be necessary to avoid unexpected errors.
Misunderstanding Zero Division
Another critical point to consider is dividing by zero. Attempting to use `mod` with a divisor of `0` will result in an error. To prevent this, you can implement error handling as follows:
try
result = mod(10, 0);
catch ME
disp('Error: Division by zero is not allowed.');
end
This code provides a safe way to check for errors during the calculation and avoids runtime exceptions.
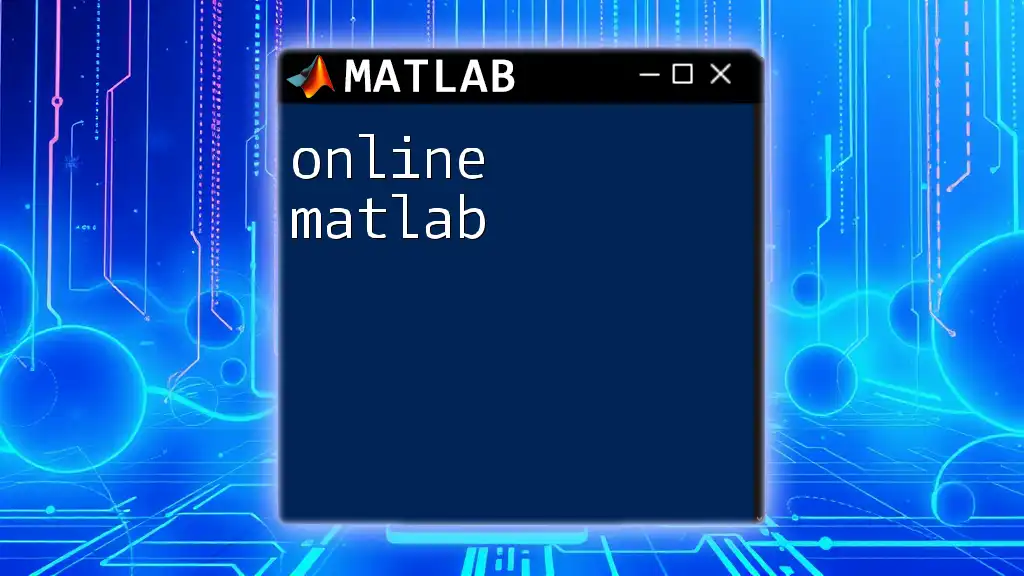
Summary
The modulo in MATLAB is a powerful tool that allows programmers to perform various calculations efficiently. Understanding its nuances, including how to use `mod` versus `rem`, the implications of data types, and practical examples helps solidify your grasp of this operation. Whether it's checking if numbers are even or odd or accessing elements in circular arrays, mastering the modulo operation expands your capabilities in programming with MATLAB.
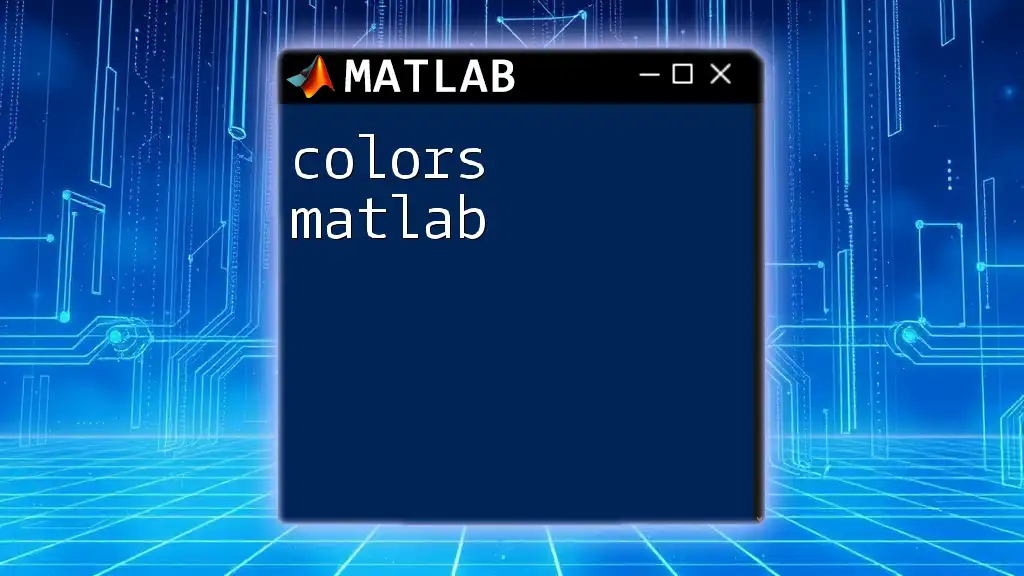
Additional Resources
For further exploration, consider referring to the official MATLAB documentation for comprehensive insights and examples. You may also find value in suggested tutorials and books designed to deepen your understanding of MATLAB commands. Engaging with community forums and Q&A platforms can also provide practical answers and enhance your learning experience.
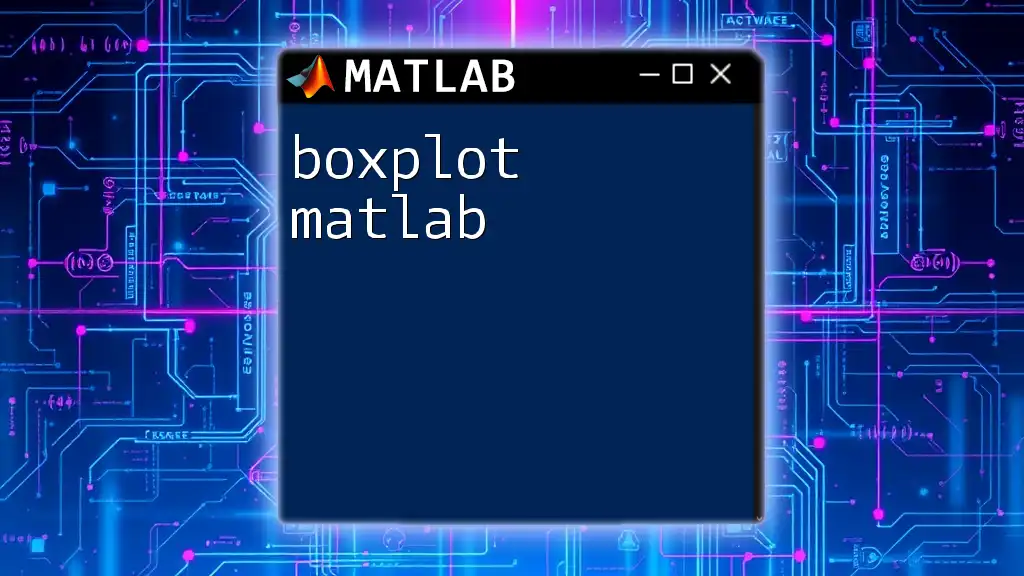
Call to Action
If you’re eager to learn more about using MATLAB efficiently and want to dive deeper into various commands and functions, consider joining our course. Feel free to reach out with any inquiries or feedback regarding this article!