Subplots in MATLAB allow you to display multiple plots in a single figure window by dividing the figure into a grid layout.
% Create a 2x2 grid of subplots
subplot(2, 2, 1); % First subplot
plot(x, y1);
title('Plot 1');
subplot(2, 2, 2); % Second subplot
plot(x, y2);
title('Plot 2');
subplot(2, 2, 3); % Third subplot
plot(x, y3);
title('Plot 3');
subplot(2, 2, 4); % Fourth subplot
plot(x, y4);
title('Plot 4');
Understanding Subplots in MATLAB
Subplots in MATLAB are a powerful feature that allows users to create multiple plots in a single figure. This is particularly useful for comparing data or illustrating different aspects of a dataset side by side. Subplots enhance data visualization efficiency by saving space and providing a coherent view of related information.
Benefits of Using Subplots
The use of subplots comes with several advantages:
- Effective Data Visualization: Displaying multiple plots allows users to quickly spot trends and anomalies in different datasets.
- Space Efficiency in Figures: Instead of creating multiple figures for each plot, subplots allow them to be consolidated, making better use of visual space.
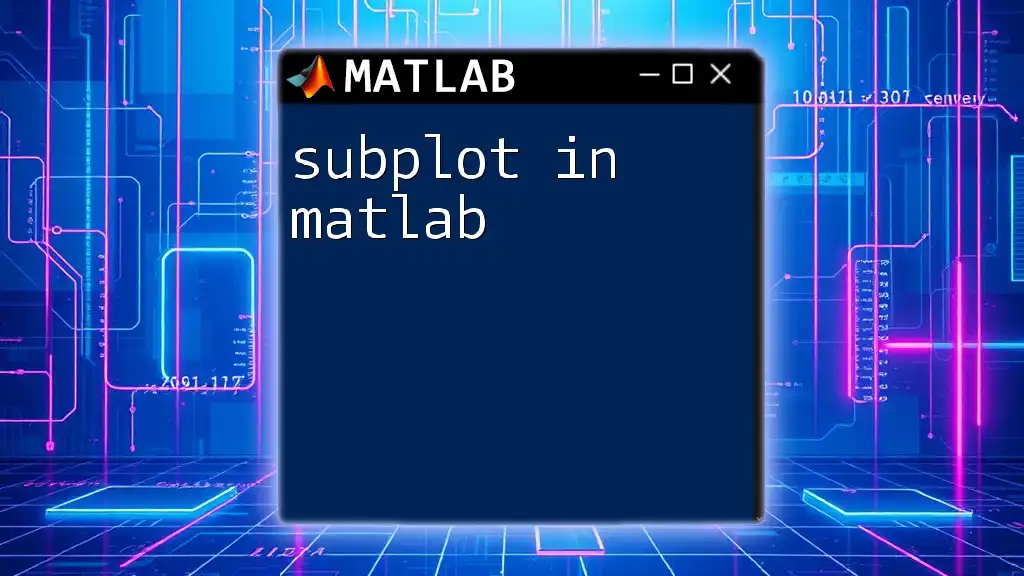
Creating Subplots
To create a subplot, you utilize the `subplot` command, which allows you to define the arrangement of your plots within a grid layout.
Basic Structure of the subplot Command
The basic syntax for creating a subplot is as follows:
subplot(m, n, p)
Here:
- m is the number of rows.
- n is the number of columns.
- p is the position of the subplot.
Example Code:
figure;
subplot(2, 2, 1); % 2 rows, 2 columns, first subplot
plot(rand(10, 1));
title('Random Data 1');
In this example, a figure is created that contains a grid of 4 subplots, and the first one displays random data.
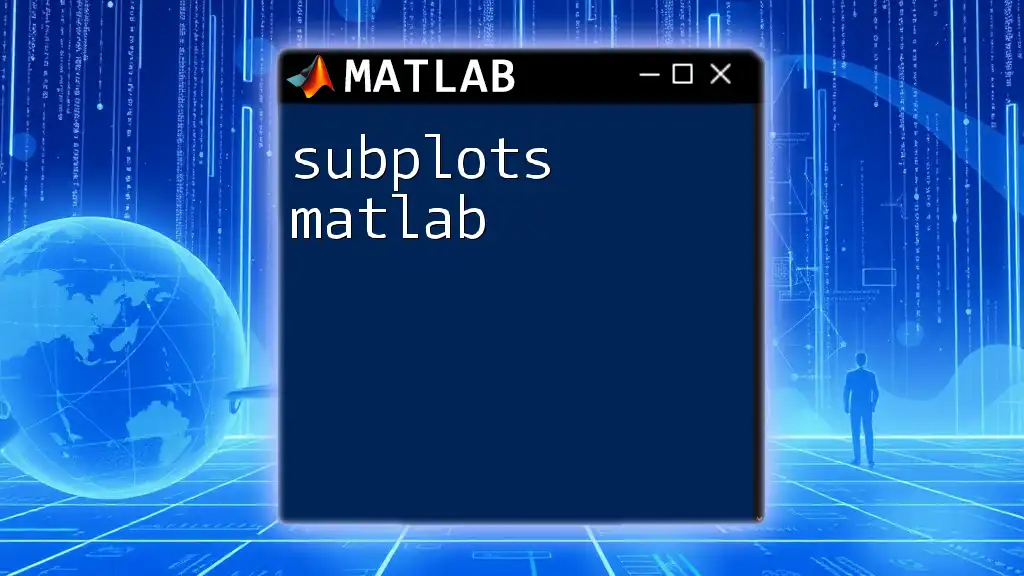
Customizing Subplots
Customization is key to making your subplots visually appealing and informative.
Adjusting Subplot Size and Spacing
You have the flexibility to arrange your subplots in various configurations. Using `hold on` and `hold off` commands allows for multiple datasets to be displayed on a single subplot without overwriting previous graphs.
Example Code:
subplot(2, 2, 2);
hold on;
plot(sin(1:0.1:10));
plot(cos(1:0.1:10));
hold off;
title('Sine and Cosine Functions');
Adding Titles and Labels to Subplots
Adding titles, along with x-axis and y-axis labels, enhances clarity and allows viewers to grasp the data presented quickly. It's essential to maintain consistency in your labeling throughout your subplots.
Example Code:
subplot(2, 2, 3);
plot(rand(10, 1));
title('Random Data 2');
xlabel('X-axis Label');
ylabel('Y-axis Label');
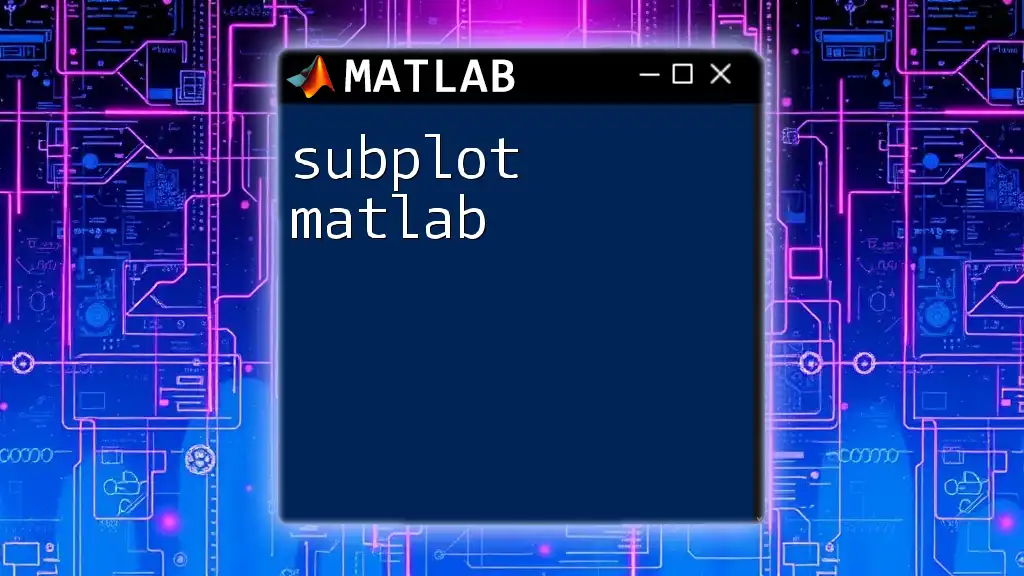
Enhancing Aesthetics of Subplots
Creating visually engaging plots is crucial for effective communication of data insights.
Color and Marker Customization
In MATLAB, it's easy to modify line colors and styles to differentiate between datasets. Utilizing different colors makes it visually clear what data pertains to which plot.
Example Code:
subplot(2, 2, 4);
plot(rand(10,1), 'r--'); % Red dashed line
title('Another Random Data');
Adding Legends to Subplots
Incorporating legends helps clarify which data series corresponds to which visual representations, especially when multiple datasets are displayed together.
Example Code:
subplot(2, 2, 1);
hold on;
h1 = plot(rand(10,1), 'b-');
h2 = plot(rand(10,1), 'g-');
hold off;
legend([h1 h2], 'Data Series 1', 'Data Series 2');
title('Multiple Data Series');
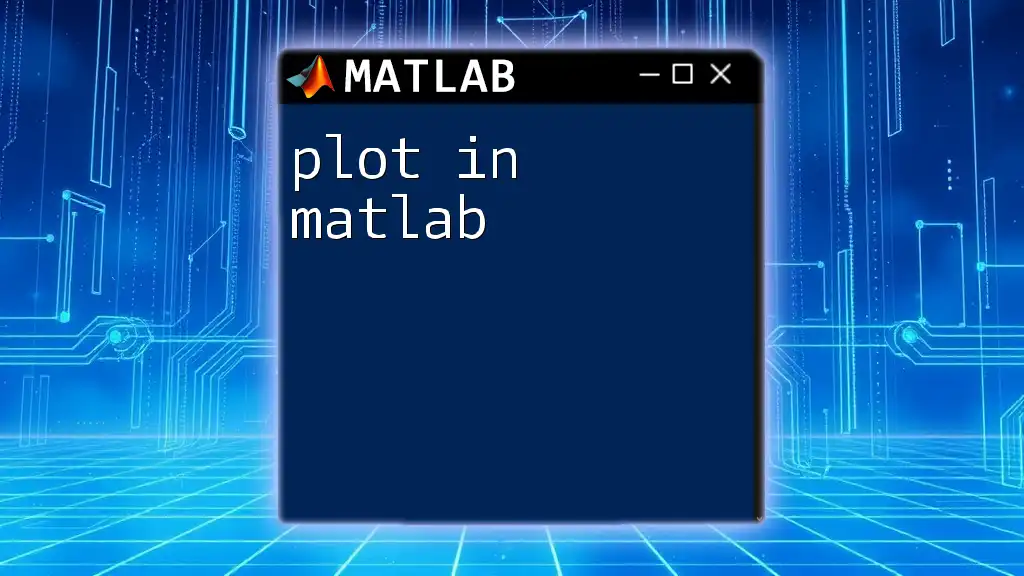
Advanced Techniques with Subplots
Creating a Grid of Subplots
For larger datasets or more comparisons, you might want to create a grid of subplots, such as a 3x3 layout.
Example Code:
figure;
for i = 1:9
subplot(3, 3, i);
plot(rand(10,1));
title(['Plot ', num2str(i)]);
end
Using Subplots with Different Axes
Sometimes, it is useful to have subplots that utilize independent axis limits to provide more informative views of the individual datasets.
Example Code:
subplot(2, 1, 1);
plot(rand(10,1));
ylim([0, 1]); % Specify Y-axis limits
subplot(2, 1, 2);
plot(rand(10,1) * 10);
ylim([0, 10]); % Different Y-axis limits
title('Independent Y-axis Limits');
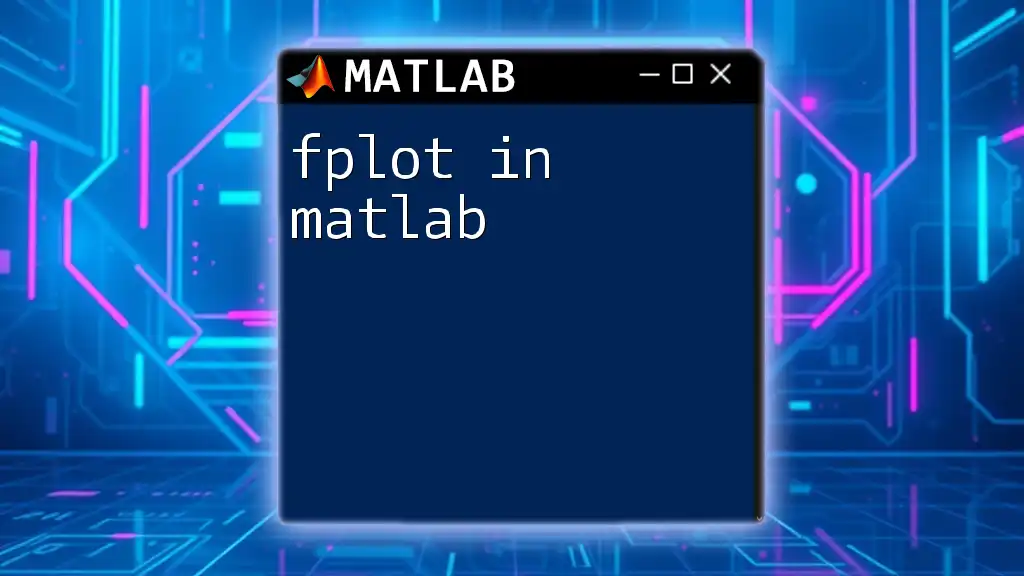
Utilities and Best Practices
Using sgtitle for Overall Titles (MATLAB R2018b and later)
When creating multiple subplots, it can be helpful to add an overall title for the entire figure using the `sgtitle` command, which simplifies the process of titling complex figures.
Example Code:
sgtitle('Overall Title for Subplots');
Common Errors and Troubleshooting Tips
When working with subplots, users may encounter errors such as overlapping plots or incorrect syntax. Common issues include specifying the wrong subplot position or failing to retain the formatting through the `hold on` command. Troubleshooting often involves careful checking of syntax and ensuring that the intended modifications are clearly defined.
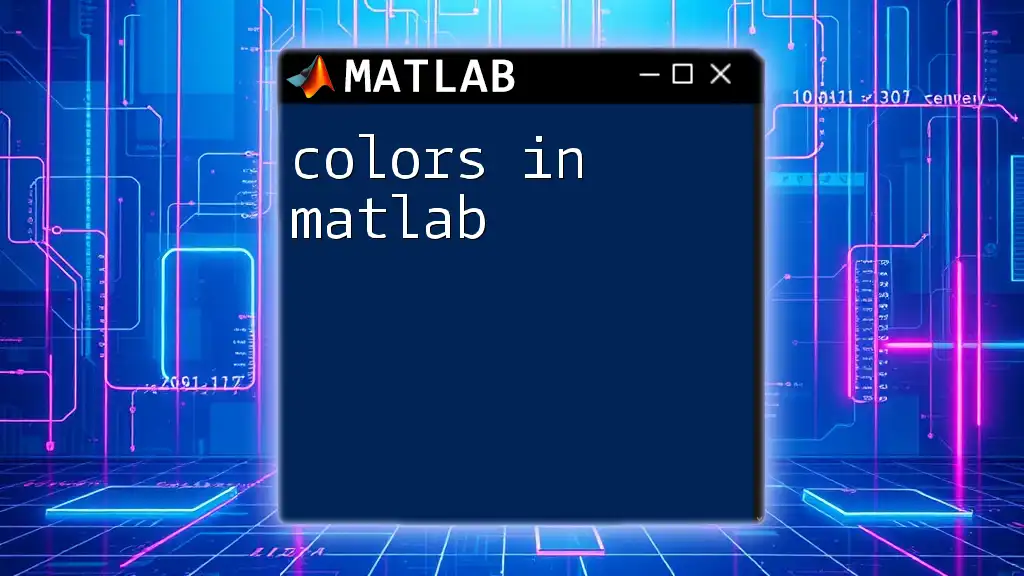
Practical Examples of Subplots
Example 1: Visualizing Multiple Data Sets
Consider a scenario where you need to compare multiple data trends. Subplots effectively present this data side by side.
Example Code:
figure;
subplot(3, 1, 1);
plot(rand(10,1)); % First data set
title('Data Set 1');
subplot(3, 1, 2);
plot(rand(10,1) * 10); % Second data set
title('Data Set 2');
subplot(3, 1, 3);
plot(rand(10,1) * 100); % Third data set
title('Data Set 3');
sgtitle('Comparison of Three Random Data Sets');
Example 2: Visualizing Functions
Subplots can also be used to visualize mathematical functions, offering clarity and insight into their behaviors.
Example Code:
x = 0:0.1:10;
figure;
subplot(2, 1, 1);
plot(x, sin(x));
title('Sine Function');
subplot(2, 1, 2);
plot(x, cos(x));
title('Cosine Function');
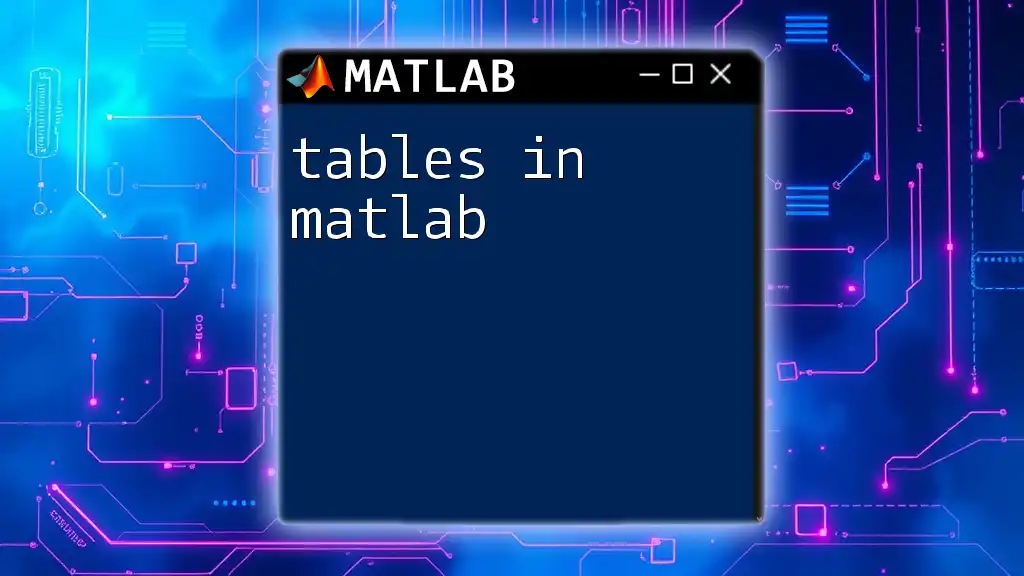
Conclusion
In summary, mastering subplots in MATLAB not only enhances your data visualization capabilities but can also significantly improve your analytical efficiency. Understanding the creation, customization, and aesthetic enhancement of subplots can lead to clearer, more informative figures. As you continue to explore MATLAB, experiment with different types of plots and graphs, making use of the subplots feature to its fullest potential.
Next Steps for Learning MATLAB
To further develop your skills, consider accessing additional resources or joining a community focused on MATLAB education. Engaging with others and practicing more complex coding scenarios will deepen your understanding and expertise.