The norm in MATLAB is a function that computes the length or magnitude of a vector or matrix, which is useful for various applications in numerical analysis and linear algebra.
% Example: Calculate the norm of a vector
v = [3, 4];
result = norm(v); % This will return 5, which is the Euclidean norm (L2 norm)
Understanding Norms
What is a Norm?
In mathematical terms, a norm is a function that assigns a non-negative length or size to vectors in a vector space. Norms provide a way to measure distances between points, quantify vector lengths, and evaluate function outputs. They are central in various applications, including optimization, machine learning, and numerical analysis.
Types of Norms
Different types of norms offer different interpretations of "length," depending on the context and the mathematical properties we want to emphasize. Here are three common norms in MATLAB:
-
L1 Norm (Manhattan Norm)
The L1 norm sums up the absolute values of individual components in a vector. It provides a measure of the total distance traveled if you were moving along the axes of an n-dimensional space. Mathematically, it is defined as: $$ ||x||_1 = \sum |x_i| $$ -
L2 Norm (Euclidean Norm)
The L2 norm computes the "straight-line" distance from the origin to a point in Euclidean space. It is defined as: $$ ||x||_2 = \sqrt{\sum x_i^2} $$ This is the most commonly used norm in many applications because it retains the geometric properties of distances. -
Infinity Norm (Max Norm)
The Infinity norm measures the maximum absolute value among the vector components. It emphasizes the largest single contribution to the norm. This is mathematically expressed as: $$ ||x||_\infty = \max |x_i| $$
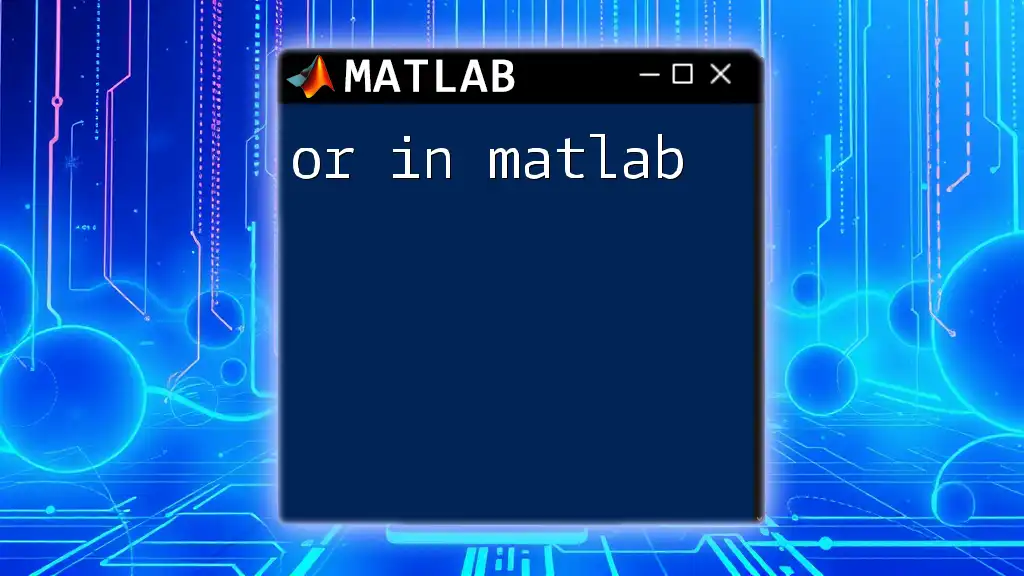
Norm Commands in MATLAB
Using the `norm` Function
The primary MATLAB function for calculating norms is the `norm` function, which allows for flexibility in choosing different types of norms. The basic syntax is given by:
norm(A, p)
Where `A` is the input vector or matrix and `p` specifies the type of norm.
Examples of Using `norm` Function
- L2 Norm:
x = [3, -4, 5];
l2_norm = norm(x);
disp(l2_norm); % Output: 7.0711
In this example, MATLAB computes the L2 norm, yielding \(7.0711\). This result represents the Euclidean distance from the origin point to the coordinates defined by vector \(x\).
- L1 Norm:
x = [3, -4, 5];
l1_norm = norm(x, 1);
disp(l1_norm); % Output: 12
The L1 norm sums the absolute values of each element in the vector, resulting in \(12\). This norm is especially valuable in applications such as sparse representation.
- Infinity Norm:
x = [3, -4, 5];
inf_norm = norm(x, Inf);
disp(inf_norm); % Output: 5
The output here is \(5\), which is the maximum absolute value in vector \(x\).
Finding Norms for Matrices
When working with matrices, the `norm` function adapts to compute the Frobenius norm, which is a measure of matrix size that generalizes the L2 norm for matrices:
A = [1, 2; 3, 4];
frobenius_norm = norm(A, 'fro');
disp(frobenius_norm); % Output: 5.4772
The Frobenius norm is computed as the square root of the sum of the absolute squares of the matrix elements, yielding \(5.4772\). This form of norm is widely used in matrix factorization and approximation algorithms.
Additional Norm Options
MATLAB allows users to define custom order norms by specifying a value for the `p` parameter. For instance, with `p=4`, the norm function will compute a quartic norm, providing further flexibility for specialized applications.
In addition, when working with complex numbers, the `norm` function seamlessly handles them by calculating the magnitude of the complex vector, ensuring that calculations remain straightforward and intuitive.
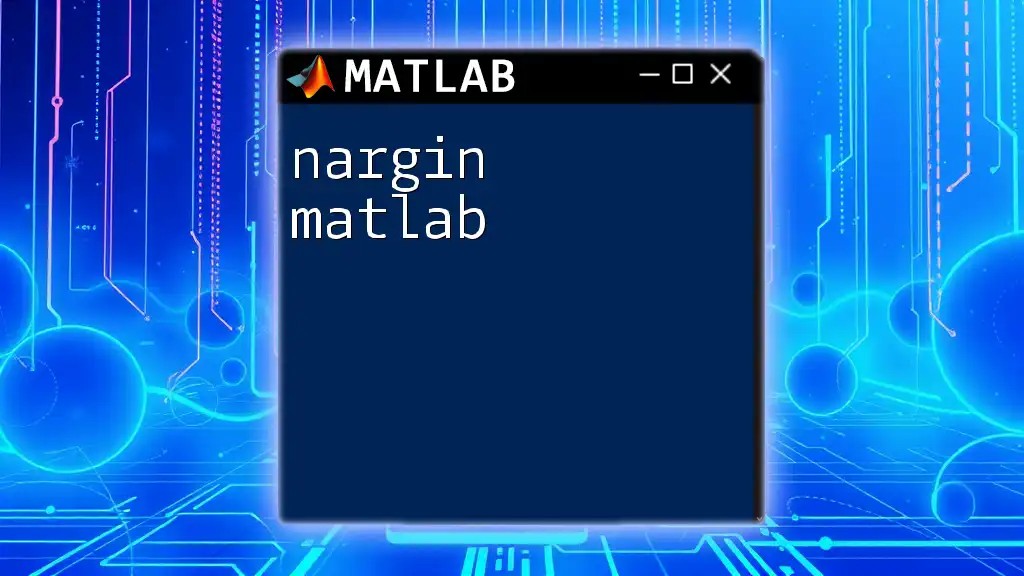
Applications of Norms in MATLAB
Use in Optimization Problems
In optimization, norms play a crucial role in defining cost functions that minimize distances. For example, the choice of L1 or L2 norms can significantly affect the performance of gradient descent algorithms. By minimizing the distance between predicted values and actual data, one can refine model parameters effectively.
Data Analysis
Norms are essential in techniques such as clustering—particularly in K-means algorithms, where they measure distances between data points to determine cluster centers. By effectively determining these distances, norms help in the formation of cohesive and well-defined clusters in high-dimensional data.
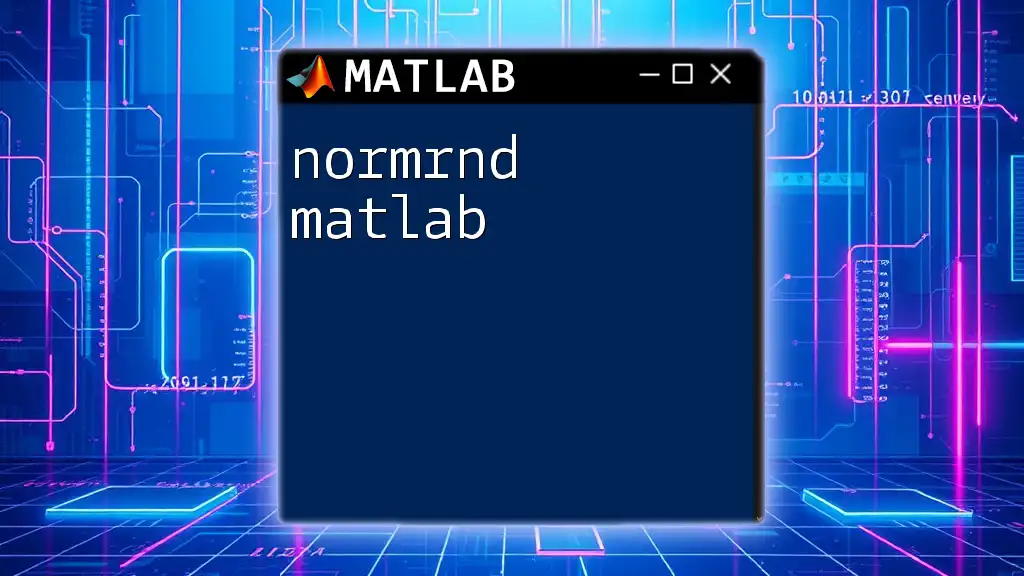
Best Practices
Choosing the Right Norm
Selecting the appropriate norm depends on the problem context. Here are some considerations:
- Use L1 norm when robustness to outliers is desired.
- The L2 norm is generally used when dealing with normally distributed data and is sensitive to outliers.
- The Infinity norm is useful in applications where the maximum deviation matters.
Performance Considerations
While the L2 norm is computationally efficient, other norms, such as L1 and Infinity, might introduce additional complexities. It’s important to understand the computational implications when selecting norms, especially in large-scale problems.
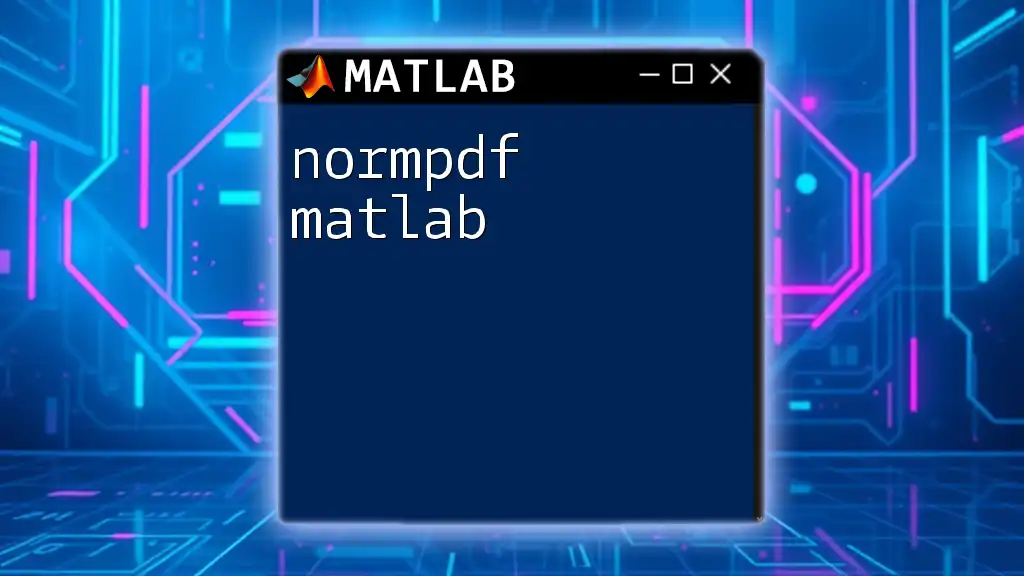
Conclusion
In summary, understanding norm in MATLAB is fundamental for anyone working with mathematical computations, data analysis, and optimization. The versatility of the `norm` function allows users to compute various norms effectively, making it a vital tool in many applications. By exploring and mastering this concept, you can enhance your analytical capabilities and deliver more refined solutions in your projects.
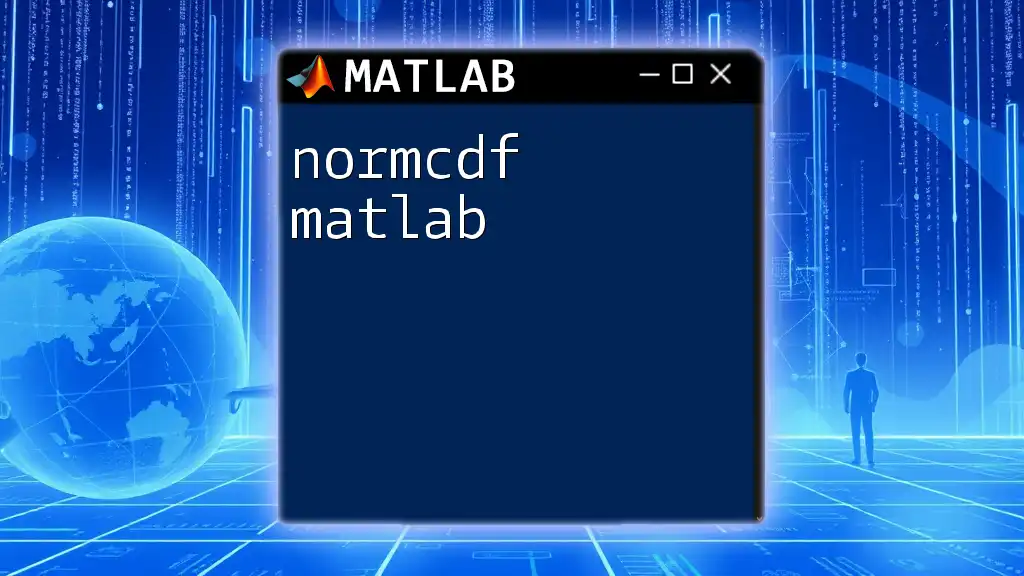
Additional Resources
To deepen your understanding of norms in MATLAB, consider browsing the official MATLAB documentation and exploring tutorials that focus on specific use cases involving norms and their applications in various fields.