The `gca` function in MATLAB returns the handle to the current axes in the current figure, allowing you to modify properties or retrieve information about the axes.
ax = gca; % Get the current axes handle
set(ax, 'XColor', 'red', 'YColor', 'blue'); % Change axes color properties
What is `gca`?
The `gca` command in MATLAB stands for "Get Current Axes." Its primary purpose is to retrieve the current axes object in the active figure. In MATLAB, figures display plots, and each figure can contain multiple axes. Thus, accessing the current axes is essential for manipulating properties related to that specific axes without needing to create new ones.
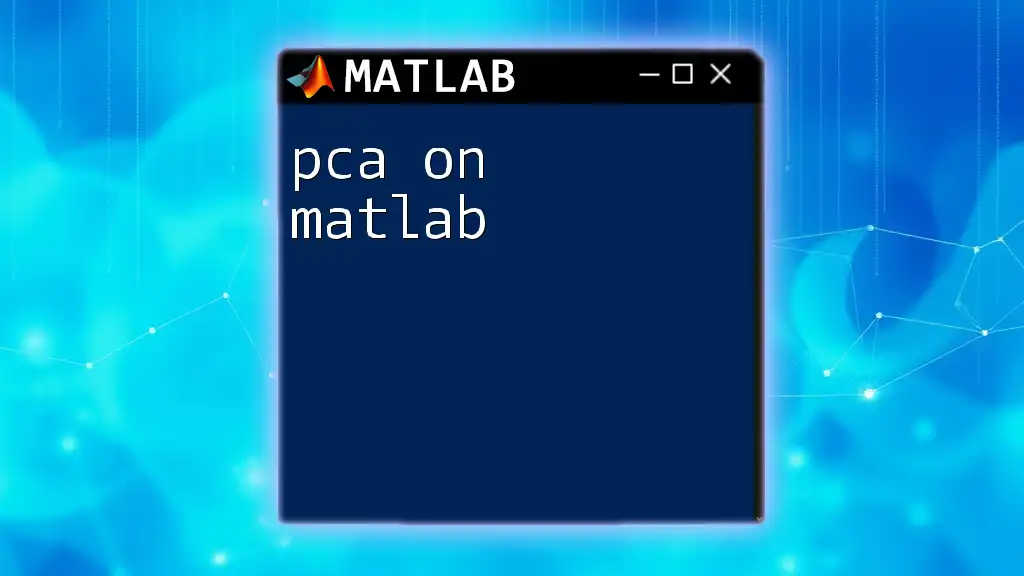
Importance of Current Axes in Plotting
Accessing the current axes matters because it allows users to customize and control the properties of the plot they are currently working on. This is particularly useful in cases where multiple plots are generated within a single figure, enabling fine-tuned adjustments without confusion.
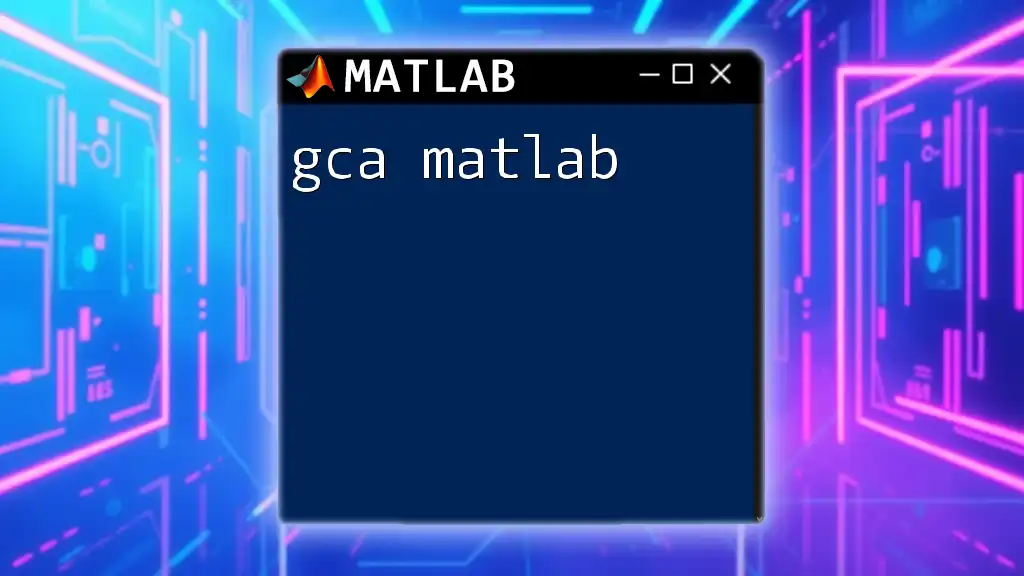
Understanding Axes in MATLAB
What are Axes?
In MATLAB, axes are the regions of a figure that display data. Each axes can contain various graphical elements, such as lines, markers, and images. MATLAB supports multiple types of axes, including 2D, 3D, polar, and geographic axes, allowing for a wide range of visualizations.
Creating Axes with `axes` Command
You can manually create axes in MATLAB using the `axes` command. This provides a flexible way to set precise properties, including position, size, and appearance. Here’s a basic example demonstrating how to create a new axes object.
figure;
ax = axes; % Create new axes object
In this example, a new figure is generated, and an axes object is created within that figure, ready for plotting.
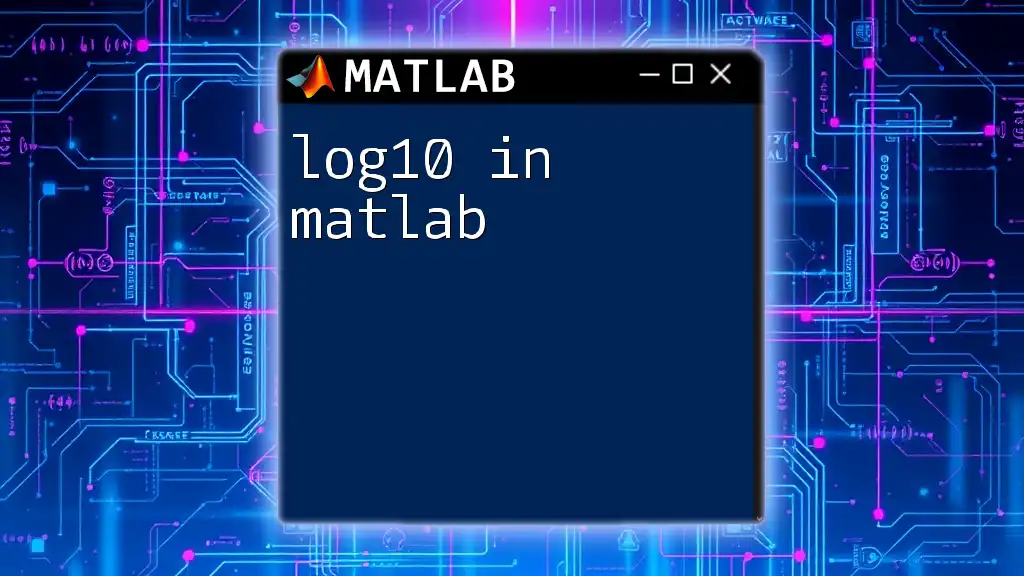
Using `gca`
Basic Syntax of `gca`
The syntax for `gca` is straightforward:
ax = gca; % Get the current axes
This command assigns the current axes object to the variable `ax`, enabling the user to access and manipulate its properties.
Accessing Properties of Current Axes
Once you've obtained the current axes, you can dive into its various properties. For example, you can fetch the limits of the X-axis and Y-axis using the commands:
currentAxes = gca;
xLimits = currentAxes.XLim; % Retrieve X-axis limits
yLimits = currentAxes.YLim; % Retrieve Y-axis limits
This capability is essential for tasks such as customizing the view window of your plots or dynamically adjusting the axes based on the data being displayed.
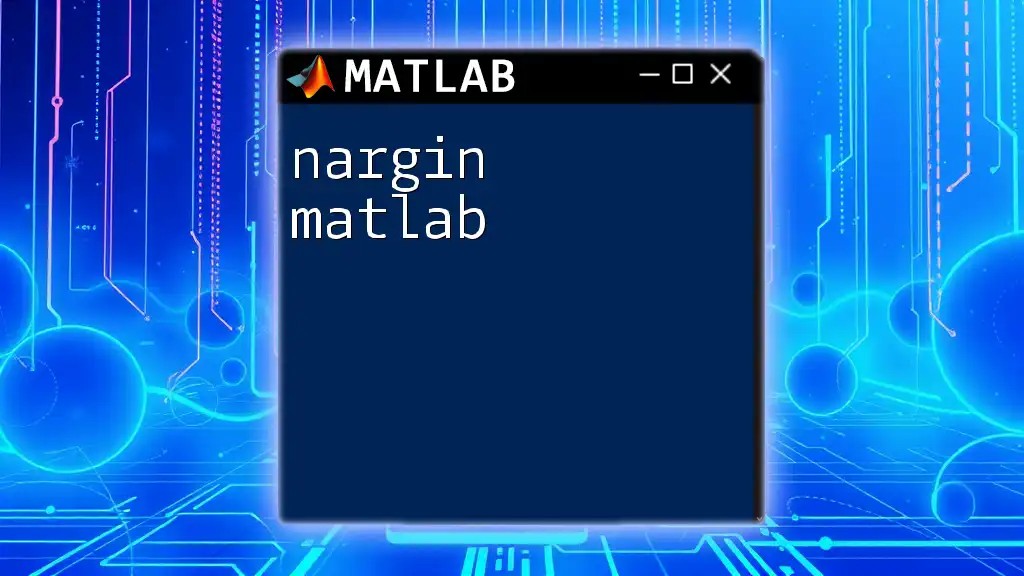
Practical Applications of `gca`
Modifying Axes Properties
By utilizing `gca`, adjusting various axes properties becomes straightforward. For example, you might want to set titles and labels for your plot. Here’s how to accomplish this:
plot(rand(10,1)); % Generate a simple plot
currentAxes = gca;
currentAxes.Title.String = 'Random Data'; % Set plot title
currentAxes.XLabel.String = 'Index'; % Set X-axis label
currentAxes.YLabel.String = 'Value'; % Set Y-axis label
This snippet not only plots random data but also enhances your plot with a descriptive title and axes labels, making it more informative.
Using `gca` in Custom Plotting Functions
When creating custom plotting functions, integrating `gca` allows for flexibility and efficiency. Here’s a sample function that creates a plot and ensures the axes limits are set appropriately:
function customPlot(data)
plot(data); % Plot the provided data
ax = gca; % Get current axes
ax.XLim = [0, length(data)]; % Adjust X-axis limits
end
This function will be beneficial as it not only visualizes the data but also configures the axes automatically according to the data length.
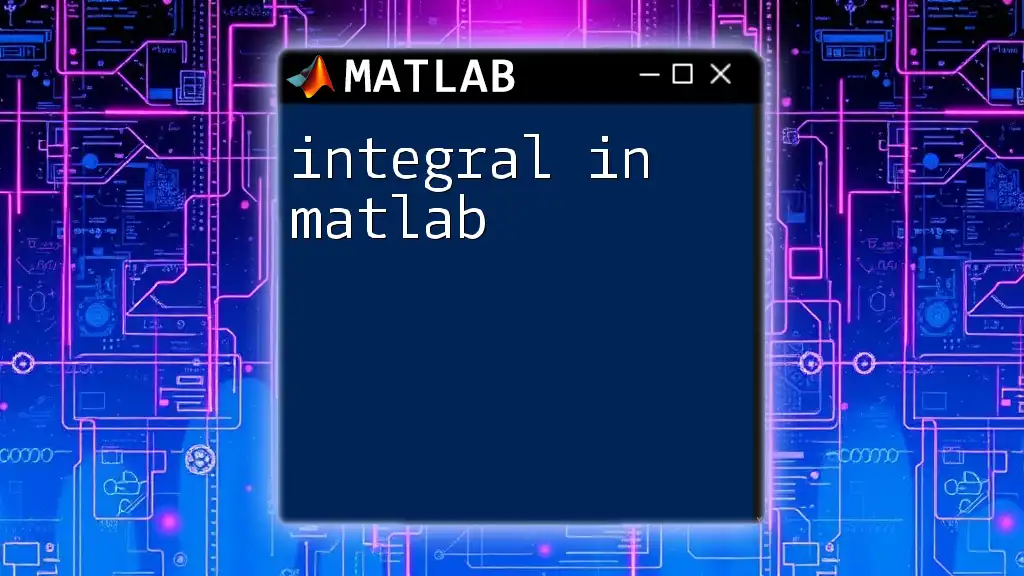
Advanced Features of `gca`
Working with Multiple Axes
In scenarios where a figure contains multiple axes, understanding how `gca` behaves becomes critical. When you create subplots, the most recent axes is returned by `gca`. Here’s how that works:
subplot(2,1,1); % Create first subplot
plot(sin(1:0.1:10));
subplot(2,1,2); % Create second subplot
plot(cos(1:0.1:10));
currentAxes = gca; % Will return axes of the last subplot
This snippet creates two subplots and retrieves the current axes tied to the second subplot for further manipulation or inspection.
Using `gca` with Grid and Box Properties
Another common use case is leveraging `gca` to modify the grid or box properties of axes. For example, you may want to add grid lines and control the appearance of the box surrounding the plot:
grid on; % Enable grid lines
currentAxes = gca;
currentAxes.Box = 'on'; % Enable box surrounding the axes
By activating the grid and enabling the surrounding box, the plot becomes clearer and more professionally presented.
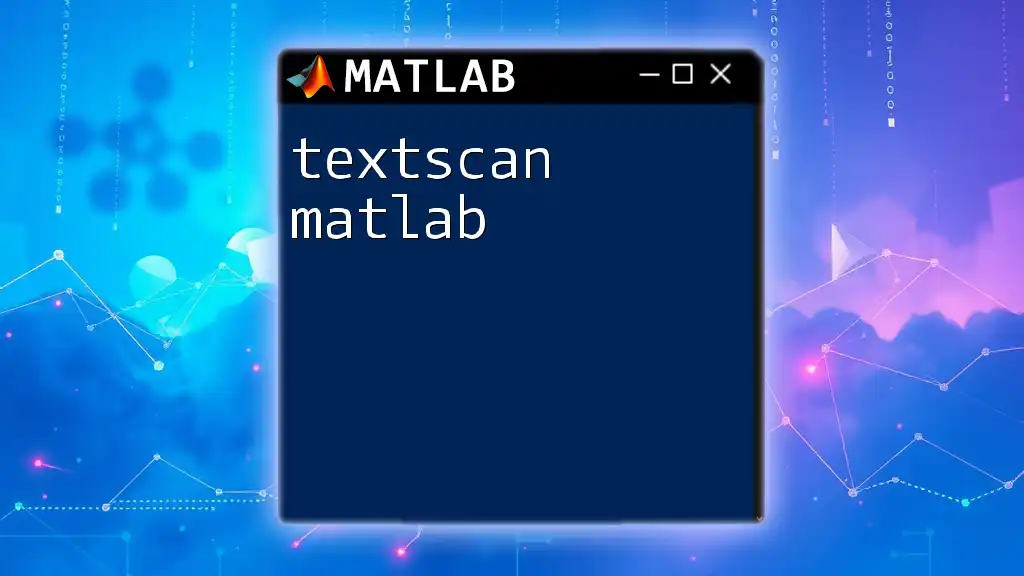
Troubleshooting Common Issues with `gca`
Errors Related to Current Axes
Sometimes, `gca` may not return the axes object you expect, especially if figures or axes are being created and deleted dynamically. To avoid such issues, ensure the figure you are working on is properly activated, or directly specify axes by creating and storing references.
Best Practices for Using `gca`
To utilize `gca` effectively, adhere to these best practices:
- Always verify that the desired figure is active before calling `gca`.
- When working with multiple axes, store references to specific axes objects whenever necessary instead of relying solely on `gca`.
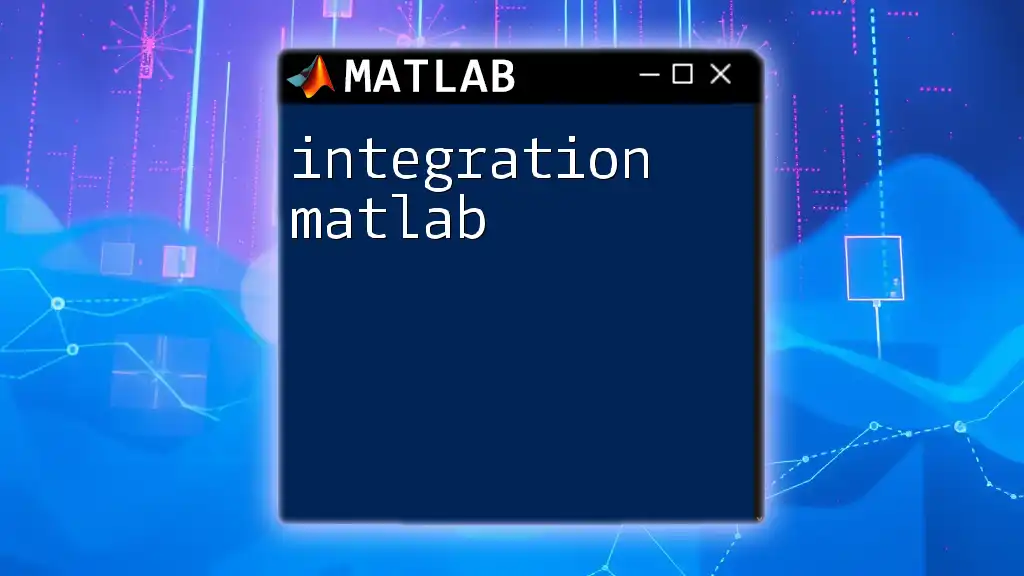
Conclusion
The `gca` in MATLAB command plays a pivotal role in plotting and customizing figure properties. By understanding its functionality, you can access and manipulate axes effortlessly, allowing for a rich, interactive plotting experience. Moreover, experimenting with `gca` can lead to improved data visualizations, elevating the quality of your work.
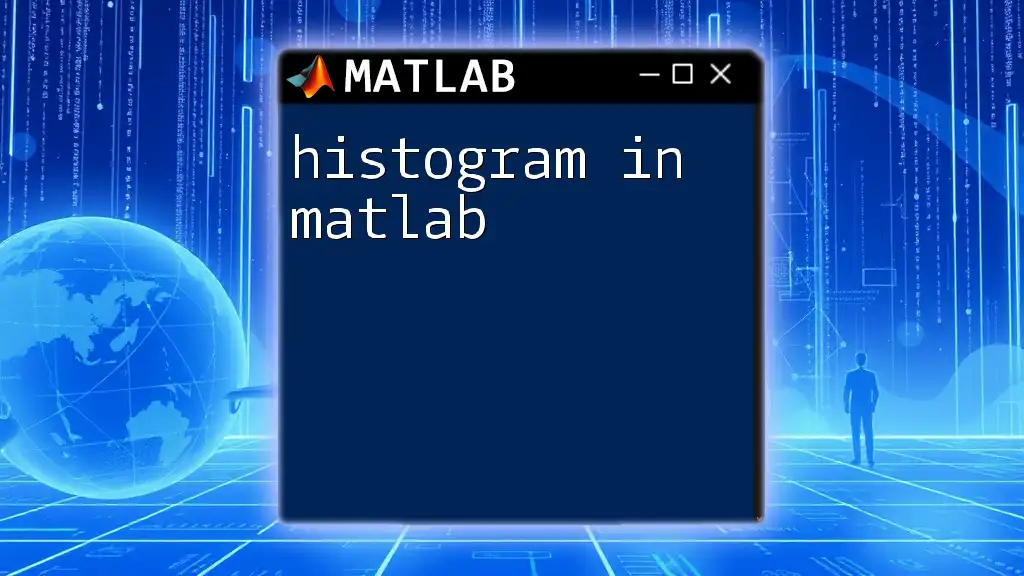
Call to Action
Join our MATLAB learning community today to enhance your skills with commands like `gca` and much more!
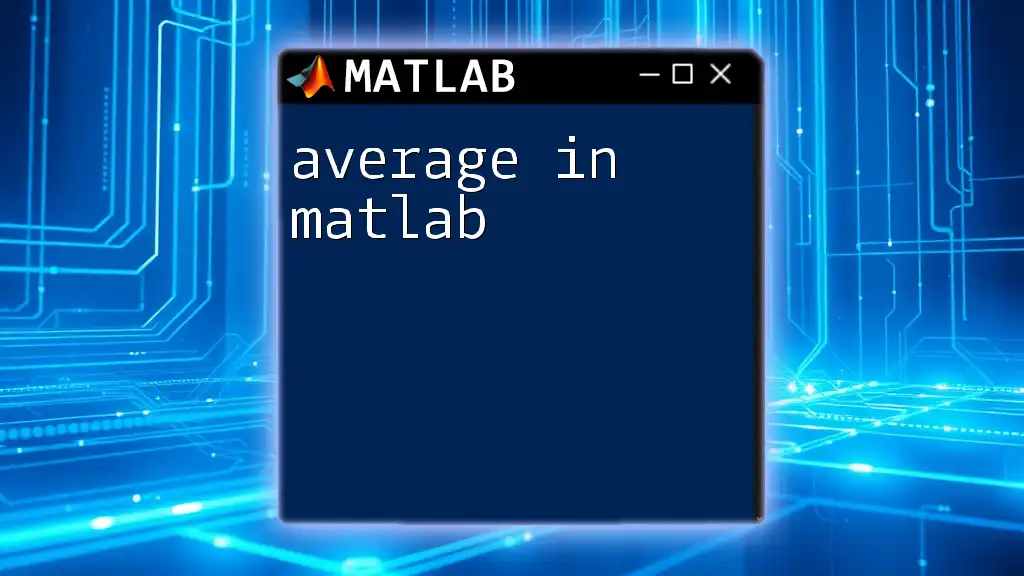
Further Resources
For those looking to deepen their understanding of MATLAB programming and plotting, check out our suggested reading materials and tutorials. Additionally, explore various example code repositories available on platforms like GitHub and MATLAB Central for practical implementations and inspiration.