The `subplot` function in MATLAB allows you to create multiple plots within a single figure window by specifying the number of rows and columns of the subplot grid and the index of the current plot.
Here's a simple example:
subplot(2, 1, 1); % Creates a 2x1 grid and activates the first subplot
plot(1:10, rand(1, 10)); % Plots random data in the first subplot
subplot(2, 1, 2); % Activates the second subplot
plot(1:10, rand(1, 10), 'r'); % Plots random data in red in the second subplot
Understanding the Basic Syntax of the Subplot Command
The `subplot` function is a powerful tool in MATLAB that allows you to create multiple plots within a single figure window. This capability is incredibly useful for comparing datasets or showing relationships between variables. The command follows a straightforward syntax, defined as follows:
subplot(m, n, p)
In this syntax:
- `m` indicates the number of rows of subplots.
- `n` designates the number of columns of subplots.
- `p` specifies the position of the subplot you intend to create or modify, counted from left to right and top to bottom.
By cleverly arranging the plots within the same window, you can present complex data more effectively, allowing viewers to draw comparisons and insights more easily.
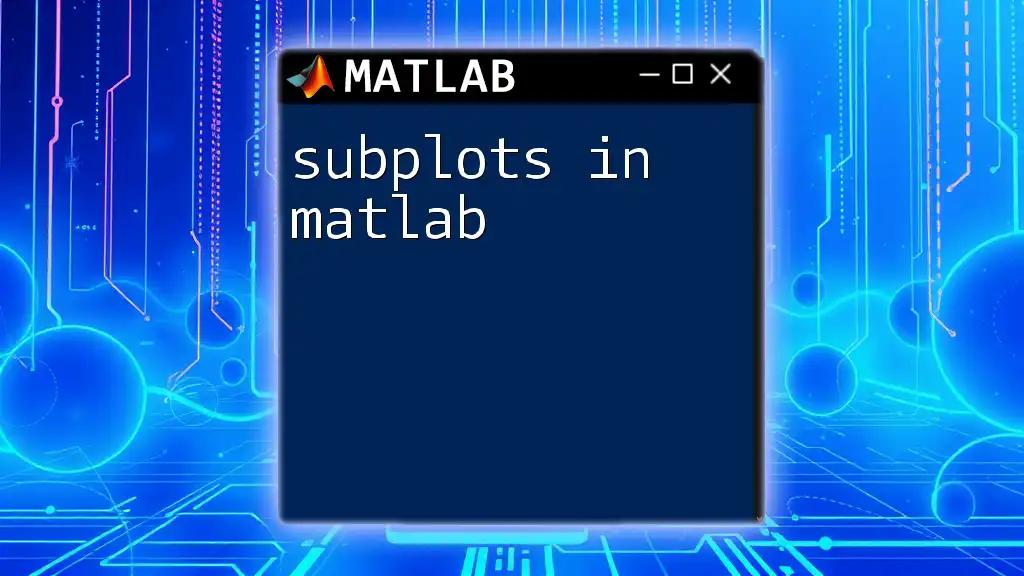
Creating Simple Subplots
Creating a simple subplot can be achieved with just a few lines of code. Consider the following example that demonstrates how to plot the sine and cosine functions:
x = 0:0.1:10; % Range of x values
y1 = sin(x); % Sine function
y2 = cos(x); % Cosine function
subplot(2, 1, 1); % 2 rows, 1 column, first plot
plot(x, y1); % Plot sine function
title('Sine Function'); % Title for the sine plot
subplot(2, 1, 2); % 2 rows, 1 column, second plot
plot(x, y2); % Plot cosine function
title('Cosine Function'); % Title for the cosine plot
In this example, we generate a range of x values and use them to calculate both the sine and cosine values. By setting up two subplots vertically, we can easily compare these two essential trigonometric functions. The layout helps viewers see the correlation and differences in behavior between the sine and cosine waves.
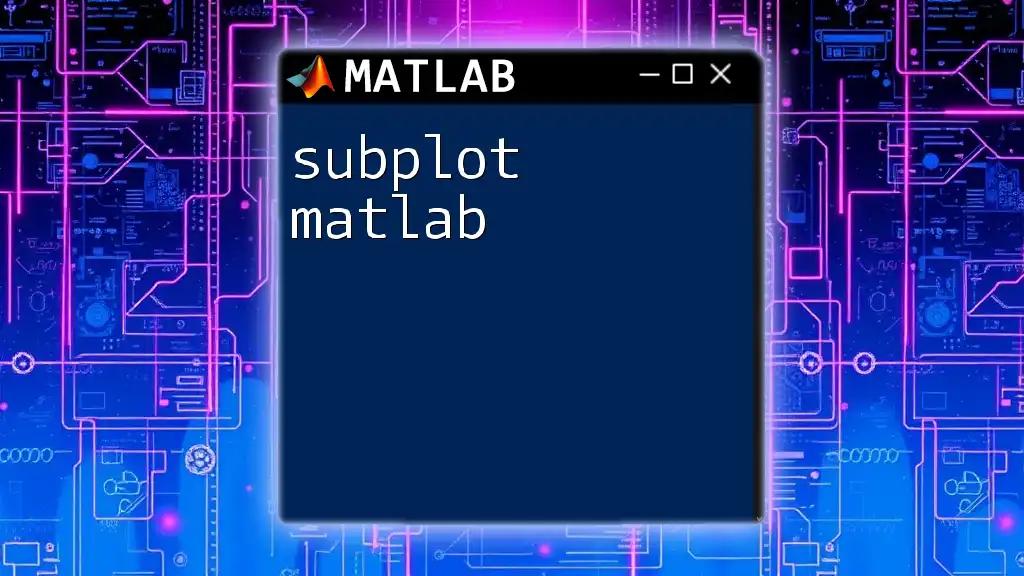
Customizing Subplot Characteristics
To make your subplots more informative and visually appealing, customizing various aspects is crucial. MATLAB allows you to adjust subplot spacing and add titles, labels, and legends seamlessly.
Adjusting Subplot Spacing
The default spacing between subplots may not always be ideal for visual clarity. You can enhance the visual representation by adjusting the `Position` property. If you want more space between subplots, you can use the following approach:
subplot('Position', [0.1, 0.55, 0.8, 0.4]); % Adjusting position
plot(x, y1);
title('Sine Function');
subplot('Position', [0.1, 0.1, 0.8, 0.4]); % Adjusting position
plot(x, y2);
title('Cosine Function');
Here, the `Position` property defines the subplot's location and size [left, bottom, width, height]. Adjusting these values can help make your plots integral parts of your overall presentation.
Adding Titles, Labels, and Legends
Enhancing readability in your subplots entails labeling your axes and adding informative titles. This practice helps guide the viewer's understanding of the data presented. Here’s how to add titles, labels, and legends effectively:
subplot(2, 1, 1);
plot(x, y1);
title('Sine Function');
xlabel('X-axis'); % Label for x-axis
ylabel('Y-axis'); % Label for y-axis
subplot(2, 1, 2);
plot(x, y2);
title('Cosine Function');
xlabel('X-axis'); % Label for x-axis
ylabel('Y-axis'); % Label for y-axis
By clearly defining what each axis represents through labels, and providing thorough titles, you ensure that your audience can quickly comprehend the information without needing further context. Legends can also be added if you are plotting multiple datasets on a single subplot. Use the `legend()` function to label different lines adequately.
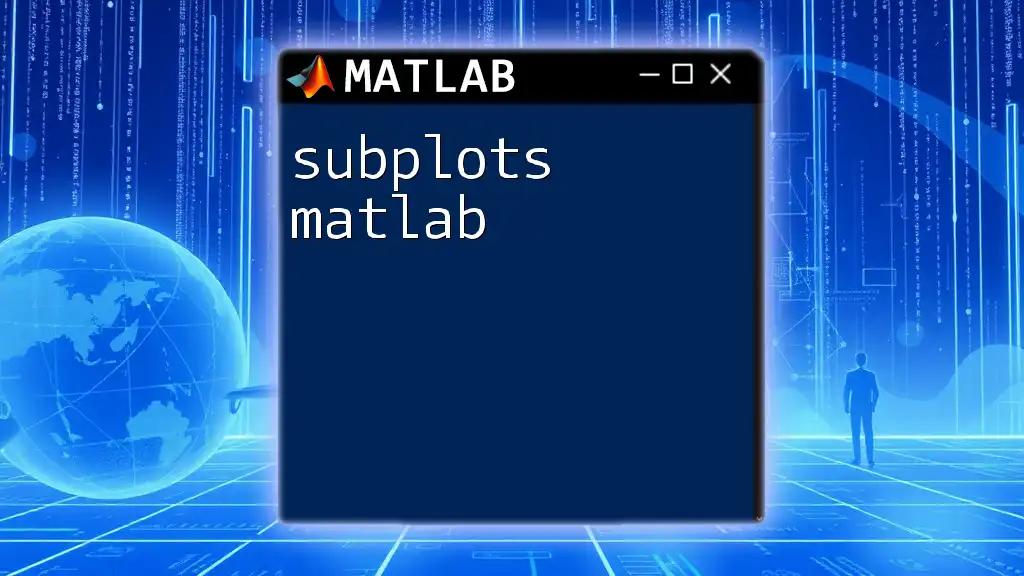
Advanced Subplot Techniques
For more complex presentations, you may find yourself needing advanced techniques to customize how your subplots are laid out. One such approach is utilizing `GridSpec` for a more flexible subplot structure.
Using GridSpec for Complex Layouts
`GridSpec` allows for the creation of subplots in a multipurpose grid format. This technique enables versatile arrangements of plots. The following example demonstrates how to implement a 3x2 grid layout:
gs = GridSpec(3, 2); % Create a grid of 3 rows and 2 columns
ax1 = subplot(gs(1, :)); % First row spans both columns
plot(x, y1);
ax2 = subplot(gs(2, 1)); % Second row, first column
plot(x, y2);
ax3 = subplot(gs(2, 2)); % Second row, second column
plot(x, y1 + y2);
ax4 = subplot(gs(3, :)); % Third row spans both columns
bar([1, 2, 3; 4, 5, 6]); % Example of a bar plot
In this instance, the first subplot occupies the top row entirely, while the second and third plots occupy the left and right halves of the middle row, respectively. The final subplot spans the bottom row, allowing for diverse data visualization in a dynamic format.
Combining Subplots with Other Functions
Subplots can also be enhanced when combined with other plotting functions such as `scatter` or `histogram`. This versatility provides a robust framework for presenting various data types:
subplot(2, 2, 1);
scatter(x, y1); % Scatter plot of sine values
title('Sine Scatter');
subplot(2, 2, 2);
histogram(y1); % Histogram of sine function values
title('Sine Histogram');
subplot(2, 2, 3);
scatter(x, y2); % Scatter plot of cosine values
title('Cosine Scatter');
subplot(2, 2, 4);
histogram(y2); % Histogram of cosine function values
title('Cosine Histogram');
This example illustrates a 2x2 grid of subplots, allowing for a comprehensive view encompassing scatter plots and histograms. Such combinations not only enrich the visual narrative but also provide deeper insights into data distributions and patterns.
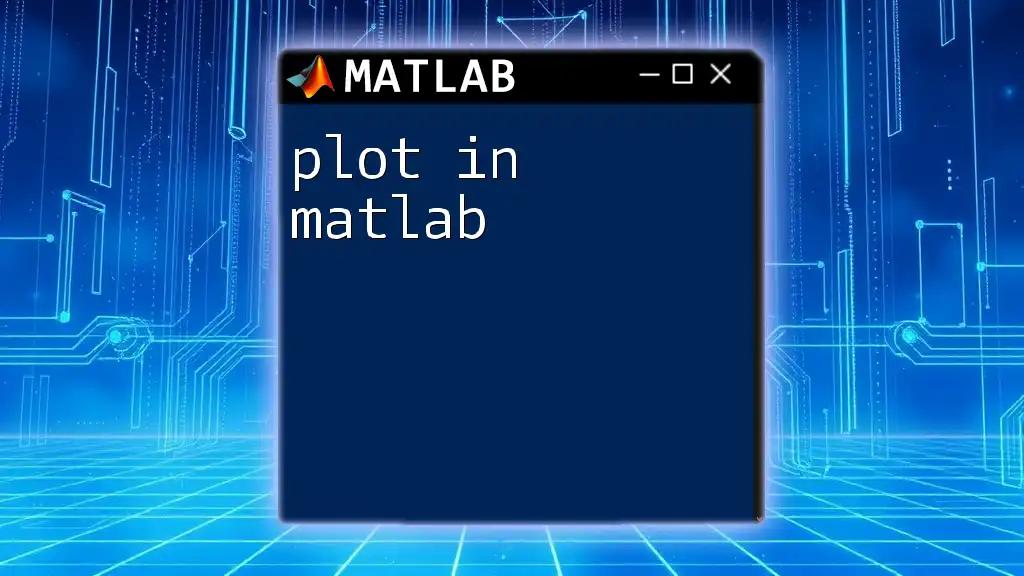
Tips for Effective Subplots
To maximize the effectiveness of your subplot presentations, consider some best practices:
-
Keep it Simple: While subplots allow for complex layouts, strive for simplicity. Too many plots can overwhelm your audience and dilute your message. Aim for clarity over complexity whenever possible.
-
Maintain Consistent Aesthetics: Use consistent colors, font sizes, and styles across your subplots. This uniformity helps ensure that your audience can focus on the data rather than being distracted by varying styles.
-
Scale and Orientation: Pay attention to the scaling of your axes. Unintended distortions can mislead viewers. Ensure your axes are properly scaled and presented in a consistent orientation.
Common Pitfalls to Avoid
During subplot creation, avoid these common errors:
-
Cluttered Visuals: Strive for balance. Too many datasets presented can lead to congestion and confusion. Use subplots strategically to present just the right amount of information.
-
Neglecting Axes: Always label your axes and provide legends where applicable. Neglecting this can lead to misinterpretations of the data.
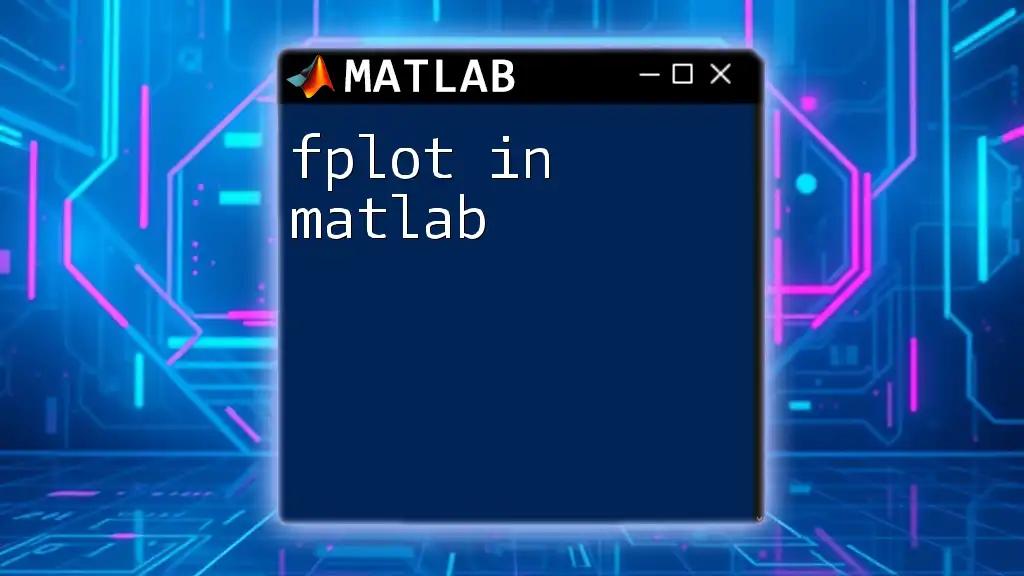
Conclusion
The `subplot` feature in MATLAB serves as an invaluable tool for data visualization, allowing for the effective comparison of multiple datasets within a single figure. By mastering the various aspects of subplot creation—from basic syntax to advanced techniques—you can significantly enhance your ability to communicate complex information clearly and concisely. As you grow more comfortable with this functionality, don't hesitate to experiment with various styles and layouts to find what best fits your data and audience. The world of subplots opens up a new dimension of presentation opportunities, so start harnessing its power today!
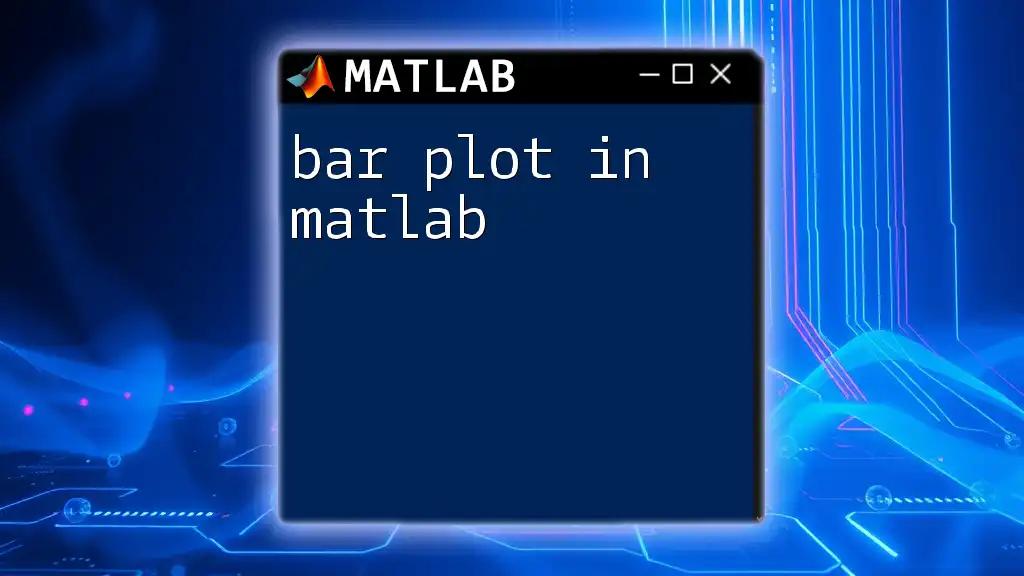
Sample Projects to Implement
To further commit your understanding of subplots, consider undertaking mini-projects where you can apply your newfound skills. For instance, visualize a complex dataset such as annual stock performance across multiple companies, or compare different ecological metrics among various species. The flexibility and power of subplots can help unveil insights that may otherwise go unnoticed, inviting your audience to explore and understand data like never before.