In MATLAB, plotting can be easily accomplished using the `plot` function to visualize data in a 2D space. Here's a simple example:
x = 0:0.1:10; % Create a vector of x values from 0 to 10
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Generate a 2D plot of y versus x
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
title('Sine Wave Plot'); % Title of the plot
grid on; % Enable grid for better readability
Basics of Plotting in MATLAB
Understanding MATLAB's Graphical Environment
MATLAB provides a rich graphical environment for creating a wide variety of plots. At the core of this environment are figure windows, axes, and plots. The figure window is the area where graphical output appears, while axes serve as a coordinate system for plotting the data.
Each time you create a plot using MATLAB, a new figure window gets generated by default. Understanding this environment is essential for effectively visualizing your data and interpreting results.
Basic Plot Command
To create a basic 2D line plot, you'll primarily use the `plot` function. The syntax is straightforward:
plot(x,y)
Where `x` and `y` are vectors containing the data points to be plotted.
For example, consider the following code that plots a sine wave:
x = 0:0.1:10; % Generate x values from 0 to 10 in increments of 0.1
y = sin(x); % Calculate y values as the sine of x
plot(x, y); % Create the plot
title('Sine Wave'); % Add title
xlabel('X-axis'); % Label x-axis
ylabel('Y-axis'); % Label y-axis
In this snippet, `x` ranges from 0 to 10. The `sin` function generates corresponding `y` values. Each aspect—labeling the axes and adding a title—enhances the plot's clarity for viewers.
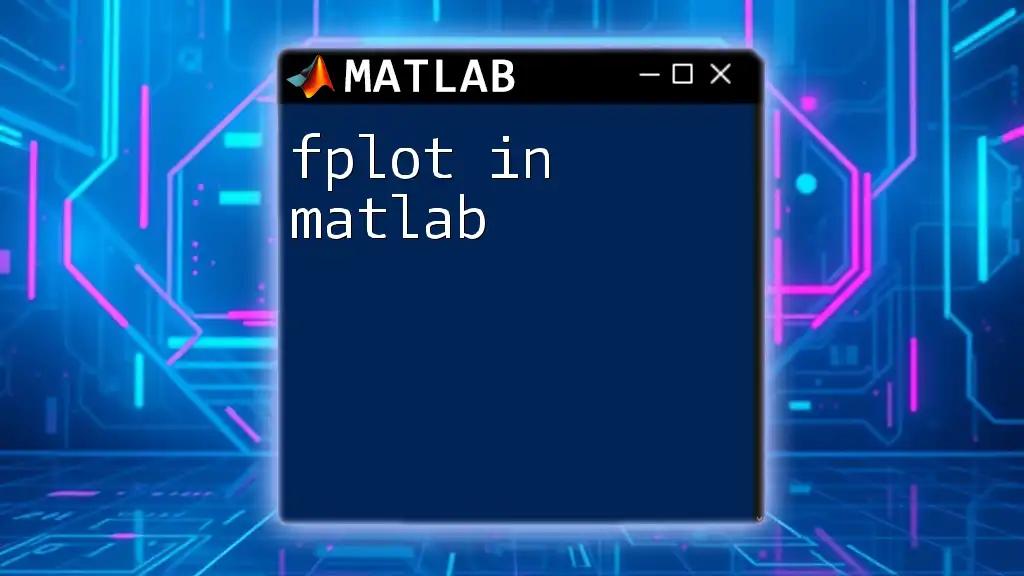
Different Types of Plots
Line Plots
Line plots are commonly used to display continuous data. They can visualize trends or relationships between two variables. You can also overlay multiple line plots in a single figure. For instance:
y2 = cos(x); % Compute cosine for the same x values
plot(x, y, 'r', x, y2, 'g'); % Plot sine in red and cosine in green
legend('Sine', 'Cosine'); % Add a legend for clarity
In this code, the sine function is plotted in red, and the cosine function in green. The `legend` function helps distinguish between the two plots.
Scatter Plots
Scatter plots are useful for displaying the relationship between two numeric variables, especially when exploring correlations. You can create a scatter plot using the following example:
x = rand(1, 100); % Generate 100 random x values
y = rand(1, 100); % Generate 100 random y values
scatter(x, y); % Create scatter plot
title('Random Scatter Plot'); % Add title
This code illustrates how to visualize random data, which is helpful in understanding distributions and potential relationships between variables.
Bar Graphs
Bar graphs depict categorical data and enable comparisons across different categories. You can create a simple bar graph like this:
categories = {'A', 'B', 'C'}; % Define categories
values = [3, 7, 5]; % Define corresponding values
bar(values); % Create the bar graph
set(gca, 'XTickLabel', categories); % Set category labels
title('Simple Bar Graph'); % Add title
This code generates a bar graph representing three categories with their respective values, making it easy to compare them visually.
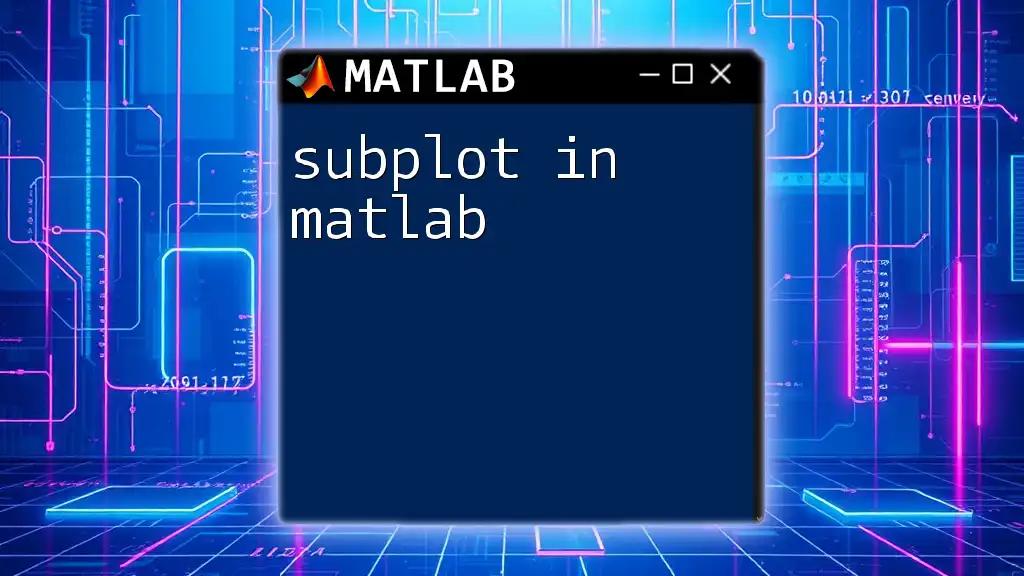
Plot Customization
Enhancing Plot Appearance
The aesthetic quality of your plots significantly affects interpretability. In MATLAB, you can customize colors, markers, and line styles to create visually appealing plots. For instance:
plot(x, y, 'o--', 'MarkerSize', 10, 'Color', 'b');
In this example, the plot uses circle markers with dashed lines, making it not just informative, but visually attractive.
Adding Annotations and Text
Annotations enhance understanding by providing context. MATLAB allows you to add titles, labels, and legends. For example:
text(5, sin(5), 'Point of Interest'); % Add annotation at a specific point
This code snippet places text at a defined location on the plot, which can highlight important features and findings.
Customizing Axes
Customizing the axes is essential for precise representation. You can set the limits of your axes like this:
xlim([0 10]); % Set x-axis limits
ylim([-1 1]); % Set y-axis limits
These commands ensure your plots focus on crucial data, avoiding unnecessary gaps or irrelevant sections of the axis.
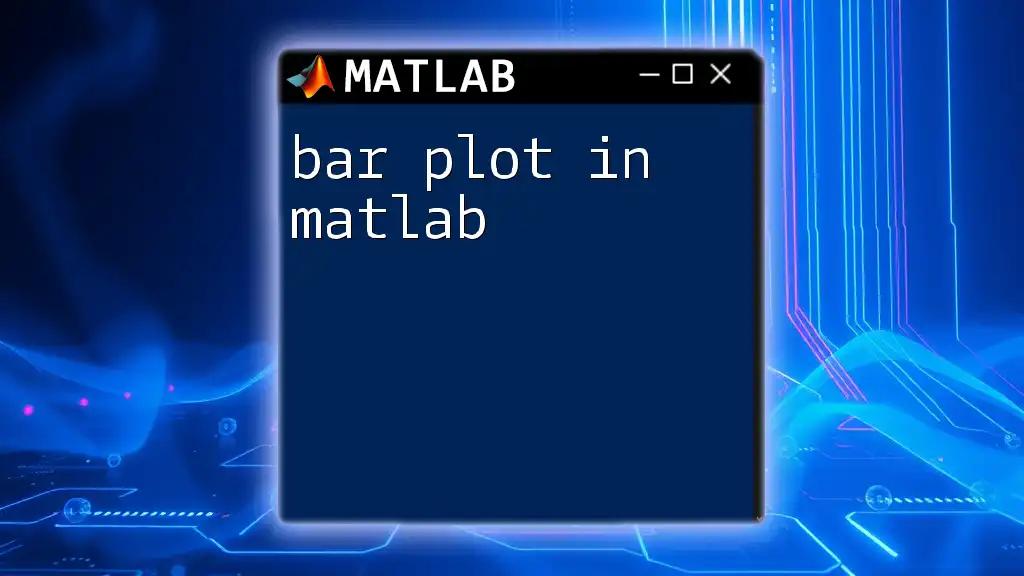
Advanced Plotting Techniques
Subplots
When you want to display multiple plots side-by-side, the `subplot` function comes in handy. It allows for organized comparison. Here’s how to create subplots:
subplot(2, 1, 1); % Create the first subplot
plot(x, y); % Plot sine function here
subplot(2, 1, 2); % Create the second subplot
plot(x, y2); % Plot cosine function here
This example divides the figure into two rows and one column, displaying two different plots neatly and efficiently.
3D Plotting
Creating more complex visualizations is possible with 3D plotting. The `plot3` function is invaluable. For example:
z = sin(x); % Generate z values based on x
plot3(x, y, z); % Create a 3D plot
title('3D Plot Example'); % Add title for the plot
This snippet generates a 3D line plot, enhancing the visual representation of relationships among three dimensions.

Tips for Efficient Plotting
Using MATLAB’s Built-in Functions
MATLAB provides a multitude of built-in functions that streamline plotting processes. Functions like `hold on`, `grid on`, and `axis equal` can greatly elevate your plots. For instance, using `hold on` allows further plotting of additional data on the same axes without clearing the existing plot.
Common Pitfalls in Plotting
While plotting in MATLAB, novices often make common mistakes, such as neglecting to label axes or creating overly complex plots. Remember, simplicity and clarity are key: the aim of plotting should be to enhance understanding, not complicate it.
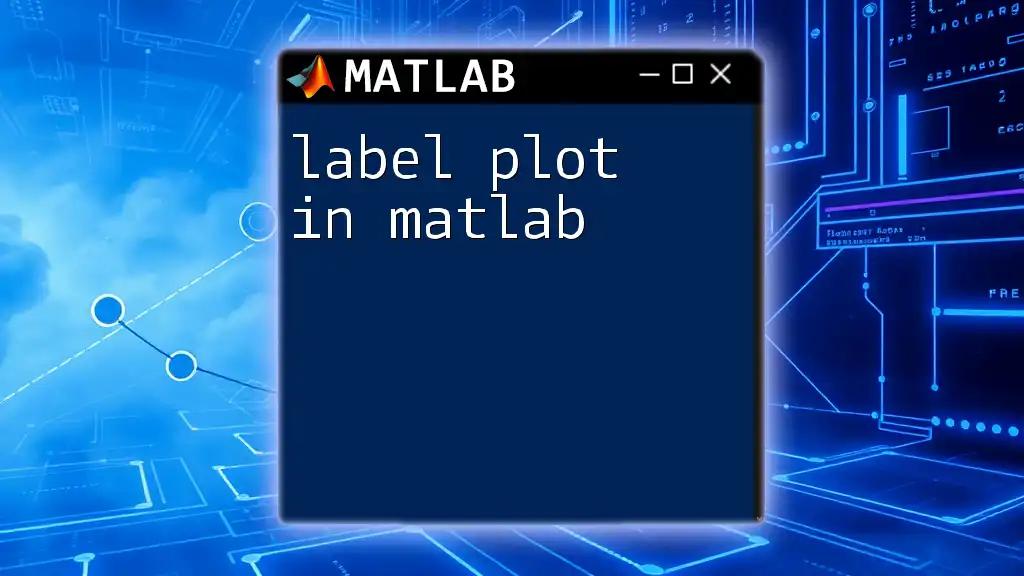
Conclusion
Creating effective visualizations in MATLAB is not only about executing commands but also about communicating insights through clear graphical representation. By mastering the various plotting functions and customization options, you can significantly enhance data analysis and presentation.
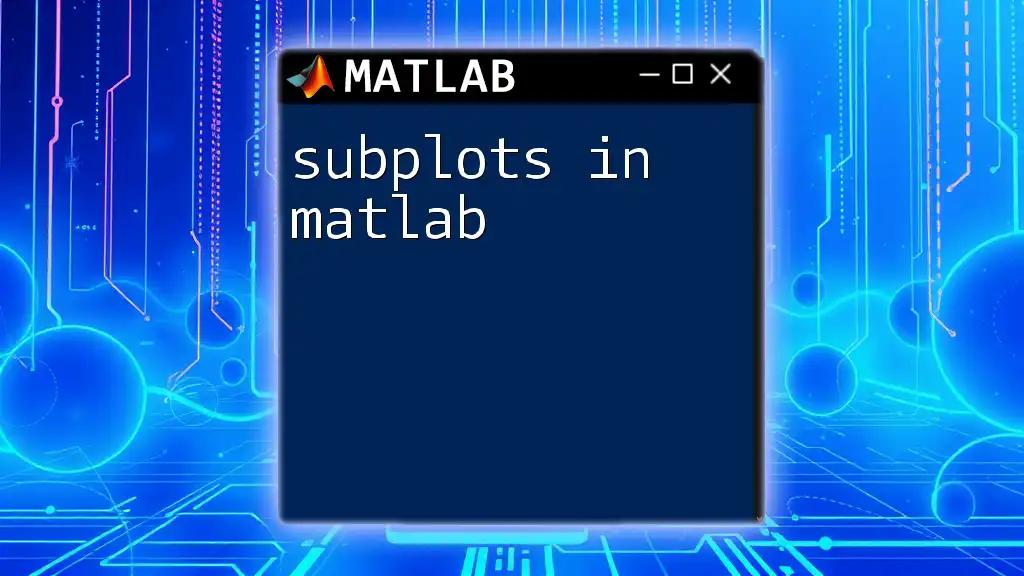
Further Resources
To deepen your understanding of MATLAB plotting, consider exploring books, online tutorials, and courses. Additionally, MATLAB's official documentation is a fantastic resource for comprehensive guidance and examples.
By exploring these avenues, you'll be well on your way to mastering how to plot in MATLAB and effectively communicate your data insights.