The `elseif` statement in MATLAB allows you to create multiple conditional branches within an `if` statement, enabling the execution of different code blocks based on varying conditions.
x = 10;
if x < 5
disp('x is less than 5');
elseif x < 15
disp('x is between 5 and 15');
else
disp('x is 15 or greater');
end
Understanding `elseif`
What is `elseif`?
The `elseif` statement in MATLAB allows programmers to test multiple conditions in a structured way within conditional logic. It serves as an extension of the simple `if` statement, enabling complex decision-making flows. The basic purpose is to provide alternative paths of execution depending on the evaluation of conditions. This becomes particularly valuable when there are more than two possible conditions to check.
Syntax of `elseif`
The syntax for using `elseif` in MATLAB follows a clear, structured format:
if condition1
% Code to execute if condition1 is true
elseif condition2
% Code to execute if condition2 is true
else
% Code to execute if all conditions are false
end
In this structure:
- The `if` statement starts the conditional check.
- The `elseif` keyword allows for multiple conditions to be checked sequentially.
- The `else` keyword is optional and provides a default action if none of the previous conditions are met.
- The entire block concludes with the `end` statement.
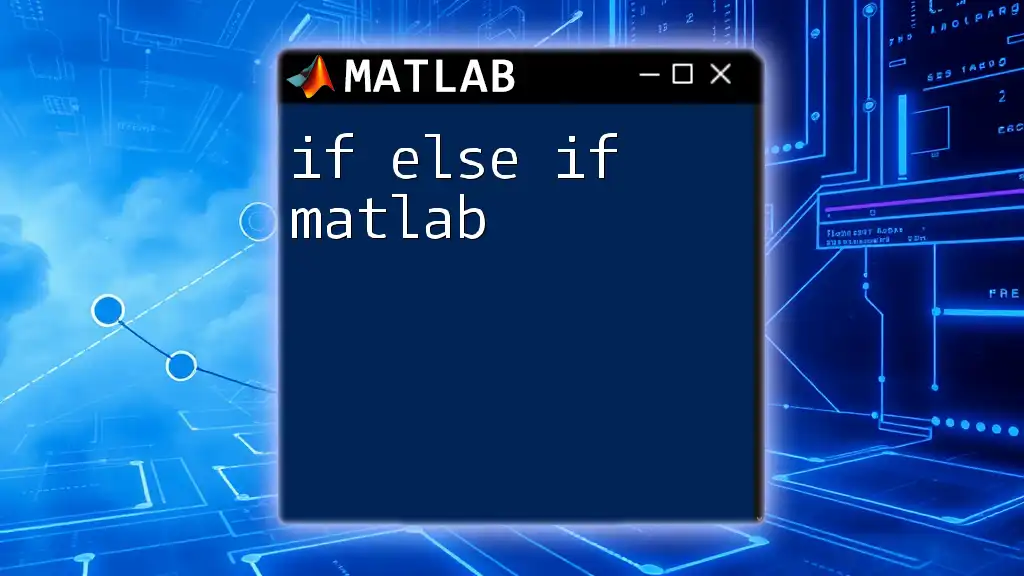
How to Use `elseif` Statements
When to Use `elseif`
Using `elseif` is essential when your program's logic requires evaluating several potential conditions. Rather than nesting `if` statements, which can lead to cumbersome and difficult-to-read code, `elseif` provides a clear alternative. For instance, consider a grading system, where scores can fall within various ranges, or a temperature classification where values dictate the appropriate category such as freezing, cold, or hot.
Example of `elseif` in Action
Here’s a straightforward example illustrating how to utilize `elseif` in a practical application, such as calculating a grade based on a score:
score = 85; % Example score input
if score >= 90
grade = 'A';
elseif score >= 80
grade = 'B';
elseif score >= 70
grade = 'C';
elseif score >= 60
grade = 'D';
else
grade = 'F';
end
disp(['Your grade is: ', grade]);
In this example, the code checks:
- If the score is 90 or above, it assigns an 'A'.
- If not, it checks for a score of 80 or above for a 'B', and so on.
- If none of the conditions are satisfied, the 'else' statement defaults to assigning an 'F'.
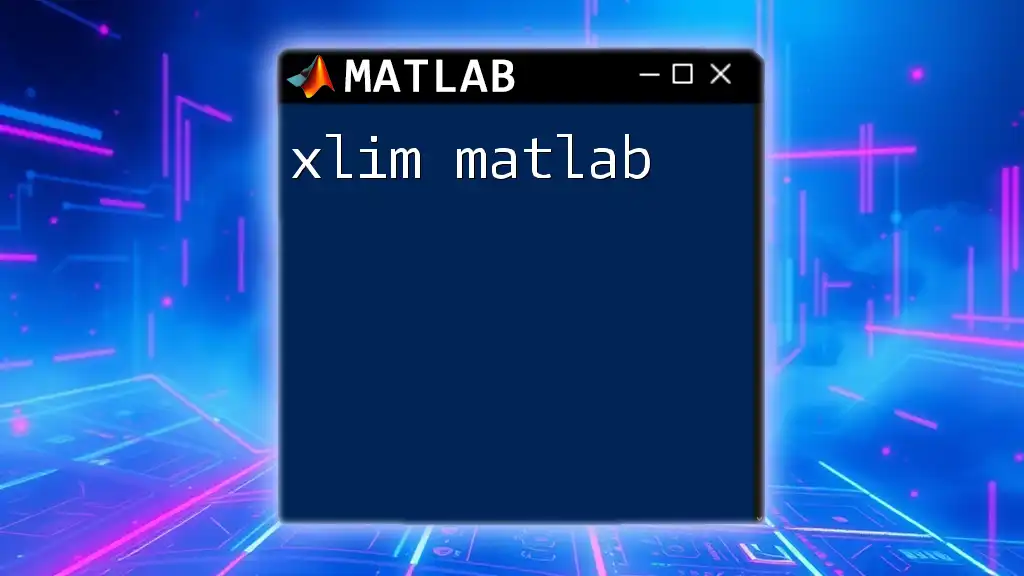
Nesting `elseif` Statements
What is Nesting?
Nesting `elseif` statements involves placing an `if` or `elseif` statement inside another `if` or `elseif` block. This approach allows for even more complex decision structures, maintaining clarity in situations where multiple layers of conditions need to be evaluated.
Example of Nested `elseif`
Consider using nesting in temperature classifications:
temperature = 30; % Example temperature input
if temperature < 0
weather = 'Freezing';
elseif temperature >= 0 && temperature < 20
weather = 'Cold';
elseif temperature >= 20 && temperature < 30
weather = 'Warm';
else
weather = 'Hot';
end
disp(['The weather is: ', weather]);
This code logic helps in categorizing the temperature into specific text conditions:
- The first `if` checks for freezing temperatures.
- The following `elseif` checks for 'Cold' conditions and so forth.
- The final `else` assigns conditions for all temperatures above 30 degrees.
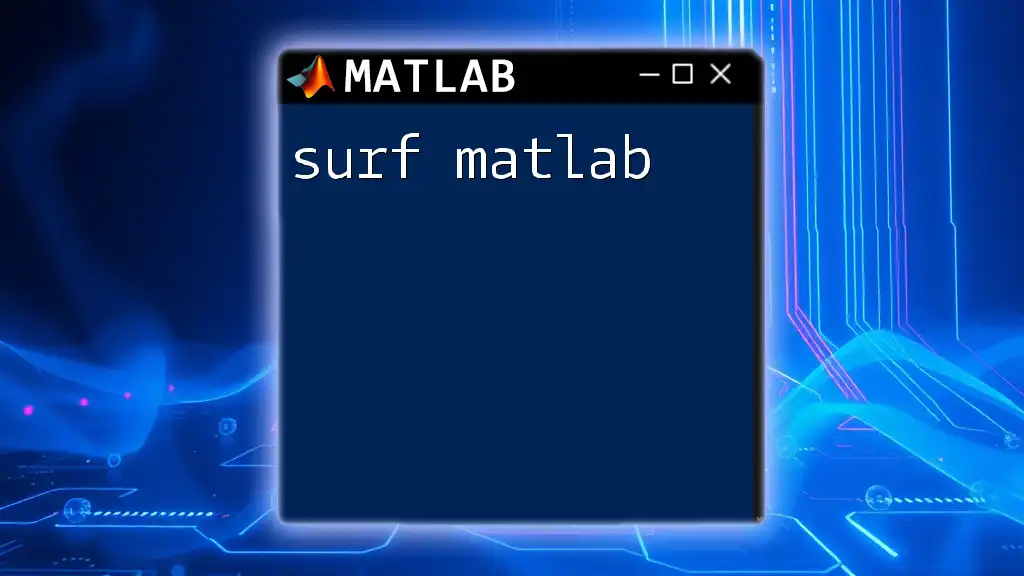
Common Errors and How to Troubleshoot
Syntax Errors
When using `elseif`, it's crucial to adhere to the correct syntax. Common mistakes include:
- Forgetting to include the `end` statement, which will result in a syntax error.
- Failing to properly indent or structure code can lead to misunderstandings regarding which conditions are associated with which blocks of code.
Logical Errors
Logical errors occur when code runs without syntax errors but does not produce the intended results. Debugging tools in MATLAB, such as breakpoints and the MATLAB workspace, can help track down where the logic may be incorrect. Carefully checking each condition and ensuring they are mutually exclusive can help prevent these errors.
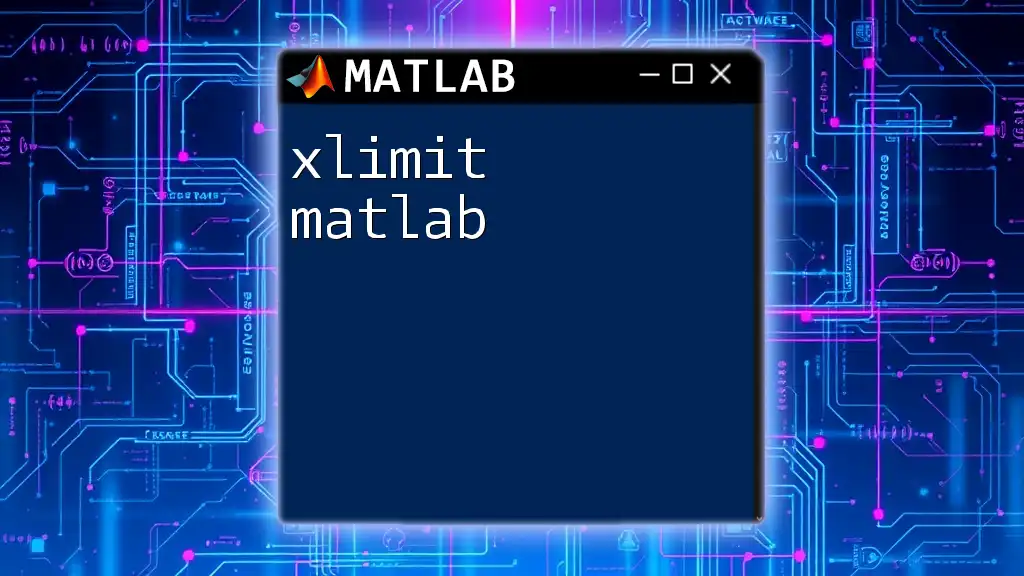
Practical Applications of `elseif` in MATLAB
Real-World Example: Categorizing Data
One practical application is categorizing age groups based on a person's age. Here’s an example that demonstrates this concept:
age = 25; % Example age input
if age < 13
category = 'Child';
elseif age < 20
category = 'Teenager';
elseif age < 65
category = 'Adult';
else
category = 'Senior';
end
disp(['The person is categorized as: ', category]);
In this example, the `elseif` structure allows for clear classification:
- It assigns 'Child' for ages less than 13, 'Teenager' for ages under 20, and likewise for adults and seniors.
- This straightforward categorization highlights how `elseif` can simplify data processing flows.
Use Cases in MATLAB Functions
Incorporating `elseif` within functions enhances code organization and reusability. For example, you can create a function that categorically assesses numerical values:
function result = categorizeValue(value)
if value < 0
result = 'Negative';
elseif value == 0
result = 'Zero';
else
result = 'Positive';
end
end
Here, the function `categorizeValue` checks the input value and returns a string representation of its category. This modular design allows for easier testing and implementation across different parts of your MATLAB code.
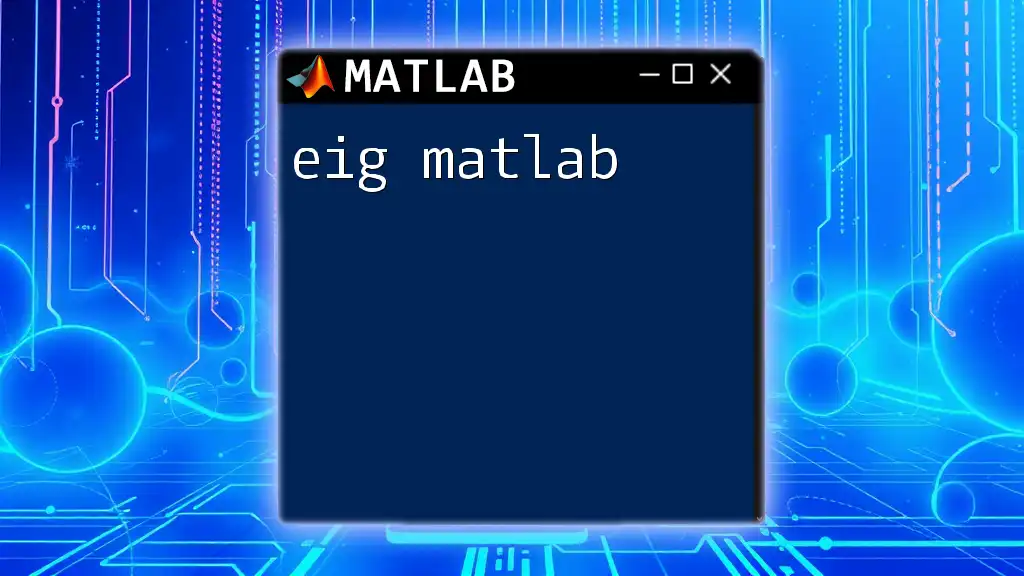
Conclusion
In summary, mastering the use of `elseif` statements in MATLAB vastly enhances your programming capabilities. It allows for clearer and more organized code when dealing with multiple conditions. Understanding how to effectively implement `elseif` can lead to better decision-making structures within your code. With practice, you'll find that this command is an essential tool in your MATLAB toolbox.
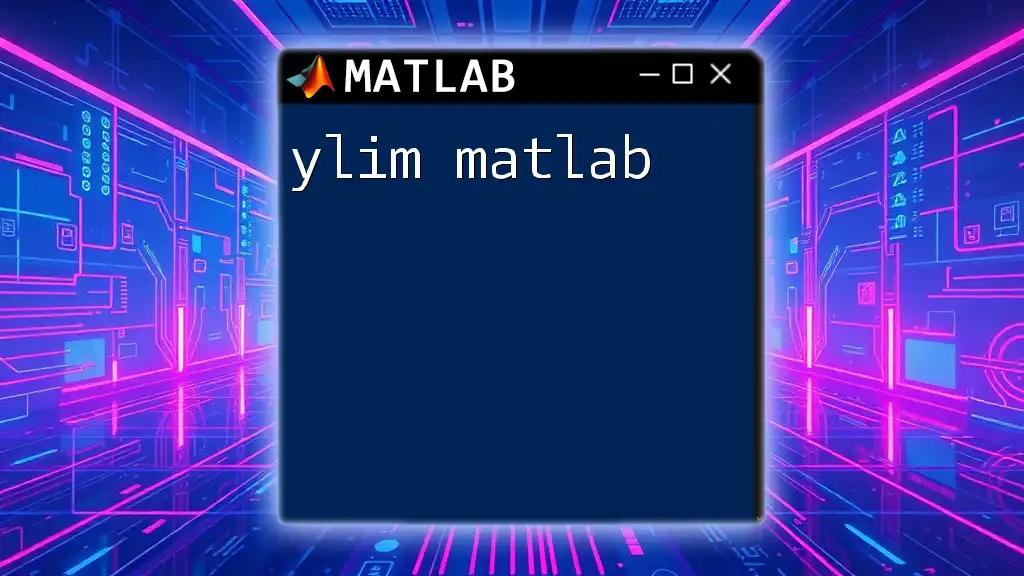
Additional Resources
To further enhance your understanding, consider exploring additional materials, including official MATLAB documentation, online tutorials, and coding challenges that incorporate `elseif` logic. Practicing regularly will not only solidify your knowledge but also improve your coding skills in MATLAB.