The `xlim` function in MATLAB is used to set or get the limits of the x-axis in a plot, allowing users to specify the range of values displayed along this axis.
xlim([0 10]); % Sets the x-axis limits to range from 0 to 10
What is `xlim`?
`xlim` is a fundamental MATLAB command used to control the limits of the x-axis in graphical representations. This command plays a crucial role in enhancing the clarity and quality of data visualizations, allowing users to focus on specific sections of their data. Effectively setting axis limits can identify trends, anomalies, or important features within a dataset.
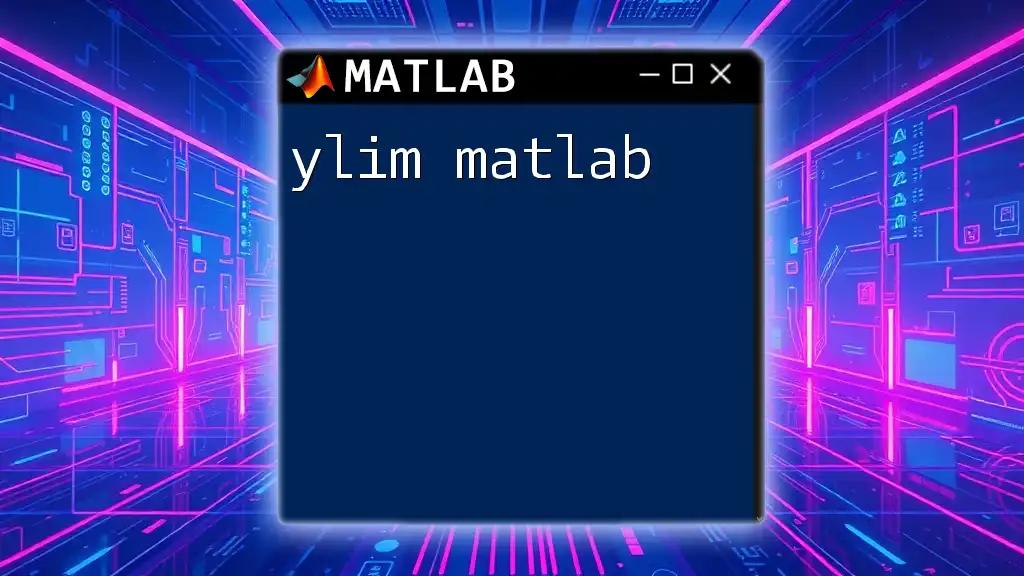
Why Use `xlim`?
Utilizing `xlim` improves the display of plots by allowing you to define the visible range of the x-axis. This capability not only helps in presenting data more clearly but also facilitates better data interpretation and decision-making. By focusing on relevant portions of data, users can gain insights that might otherwise be overlooked.
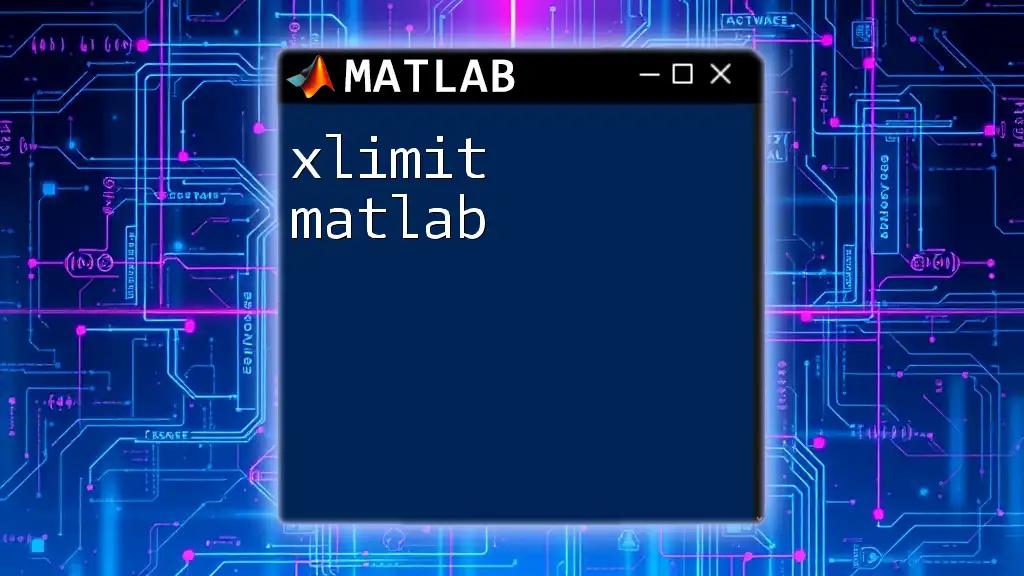
Understanding the Basics of `xlim`
Default Behavior of `xlim`
By default, MATLAB automatically determines the limits of the x-axis based on the data you provide. For instance, if you plot a simple graph using a dataset ranging from 1 to 10, MATLAB will automatically set the x-axis limits to this range. However, in some cases, the automatic limits may not present the data as intended or may obscure critical details.
Syntax Overview
The basic syntax for the `xlim` function is as follows:
xlim([xmin xmax])
This command sets the limits for the x-axis from `xmin` to `xmax`. Beyond this primary use, `xlim` also accepts the following syntactic variations:
- Setting to Auto: `xlim('auto')` allows MATLAB to compute the limits based on the data you input.
- Manual Control: `xlim('manual')` fixes the current limits so that future plotting commands do not disturb them.
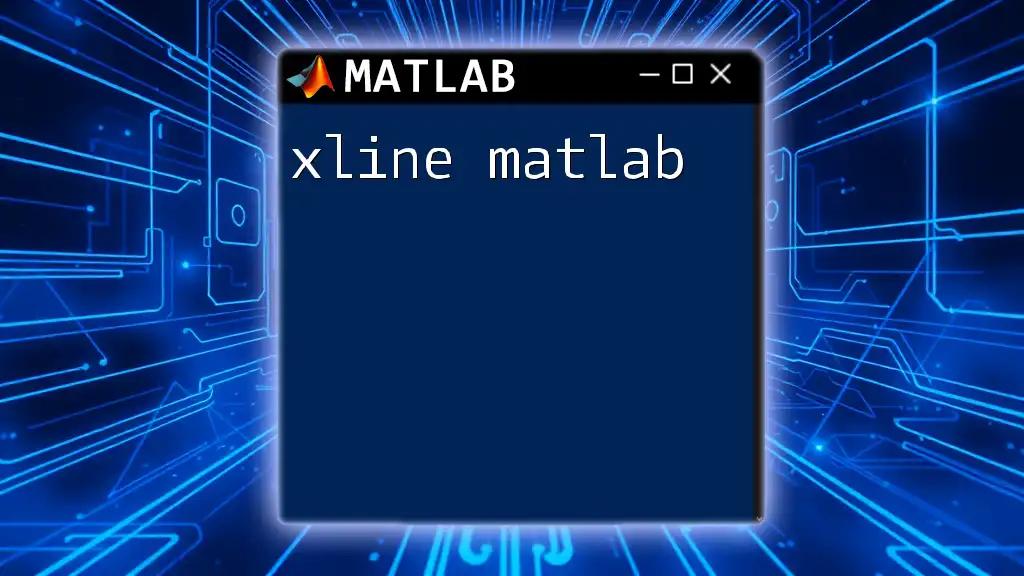
Setting X-Axis Limits
Specifying Limits with `xlim`
To set fixed limits for the x-axis, you can directly specify the desired range using the `xlim` function after generating your plot. For example:
x = 1:10;
y = rand(1, 10);
plot(x, y);
xlim([2 8]); % Setting fixed x-axis limits
In this snippet, the x-axis is limited to the range from 2 to 8, allowing viewers to focus on a specific section of the data and enhancing visibility for detailed analysis.
Dynamic X-Axis Limits
To make your plots more interactive or user-responsive, you can dynamically adjust x-axis limits based on user input. This can be particularly useful in graphical user interfaces (GUIs). An example usage could be utilizing an input function to determine the user-defined limits:
xmin = input('Enter minimum x-axis limit: ');
xmax = input('Enter maximum x-axis limit: ');
xlim([xmin xmax]); % Setting x-axis limits based on user input
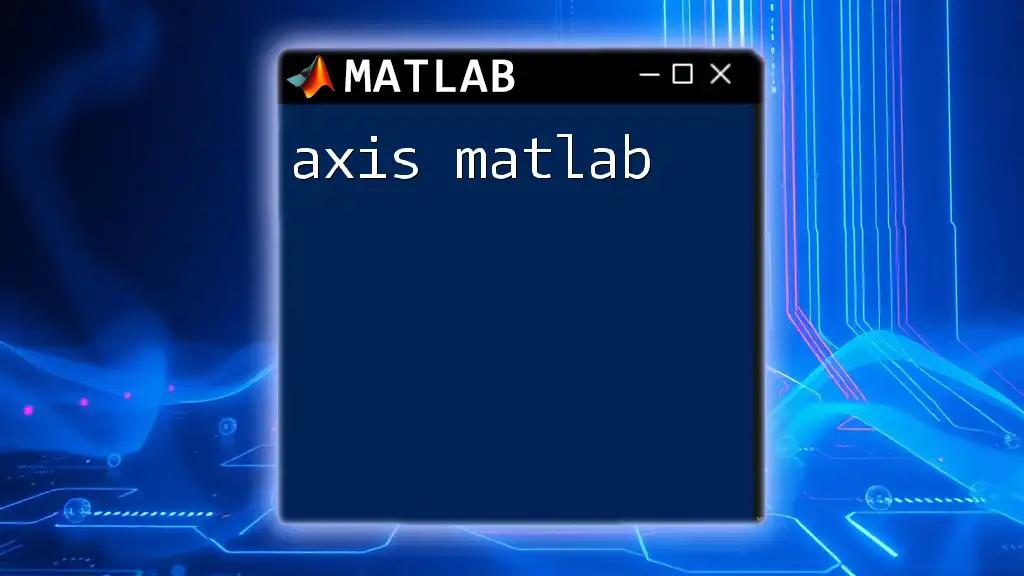
Practical Applications of `xlim`
Zooming into Data
Employing `xlim` allows you to zoom into specific areas of your dataset for a more detailed examination. For example, if you want to analyze a subset of a sine wave, you could write:
x = linspace(0, 10, 100);
y = sin(x);
plot(x, y);
xlim([4 6]); % Zoom into x-axis limits for a closer look at that section
By zooming in, you gain valuable insights that might be hidden when viewing the entire dataset.
Adjusting Limits During Data Processing
With progressive data analysis, maintaining control over visualization is essential. Here’s a way to dynamically update the x-axis limits within a loop:
for n = 1:10
plot(x, sin(n*x));
xlim([0 10]); % Keeps the x-axis limits fixed for clarity during iterations
pause(1); % Pause to observe each iteration
end
This loop displays multiple sine wave functions while keeping the x-axis limits constant for easier comparison.
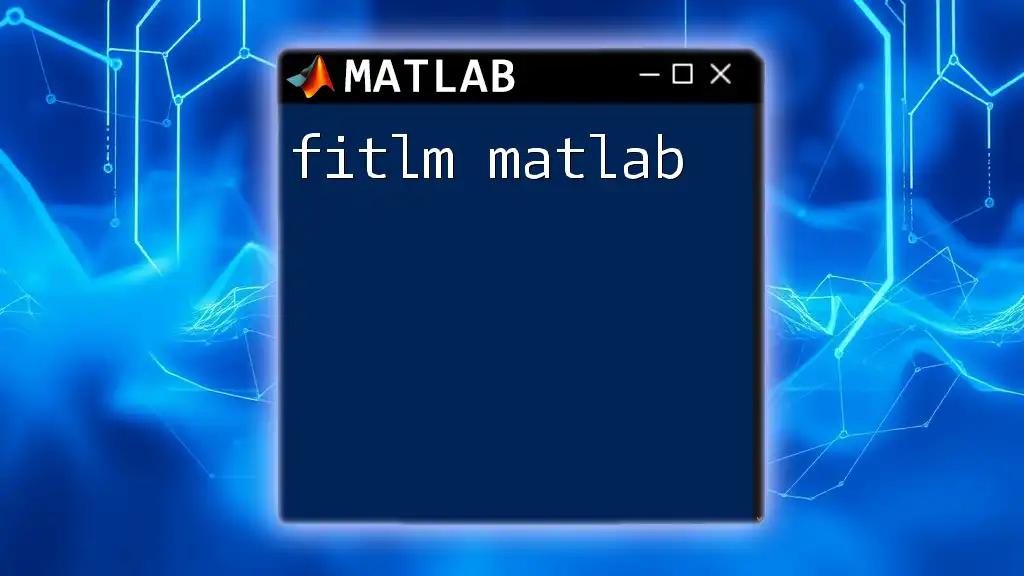
Advanced Features of `xlim`
Setting `xlim` to Auto
When you want MATLAB to automatically adjust the x-axis limits based on the data, use the command:
xlim('auto'); % Instruct MATLAB to recalculate limits based on the latest data
This command is particularly useful when the data changes dynamically, such as in simulations or interactive plots.
Manual Control of Limits
Sometimes, you may prefer to freeze the current limits to prevent MATLAB from changing them during subsequent plotting. You can achieve this with:
xlim('manual'); % Fixes the current limits to avoid automatic adjustments
This feature ensures that your chosen limits remain in effect until you decide to modify them again.
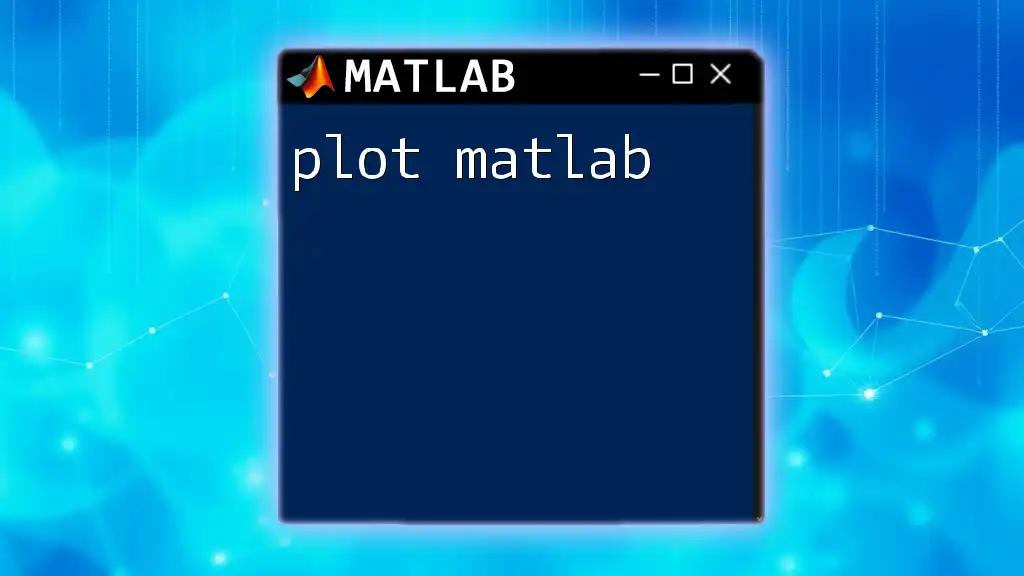
Combining `xlim` with Other Axis Commands
Using with `ylim`
For complete control over your graphs, it’s common to combine `xlim` with `ylim`. Here’s how you can achieve this:
ylim([-1 1]); % Setting y-axis limits for the plot
xlim([0 10]); % Applying x-axis limits simultaneously
By clearly defining both axes, you ensure a focused view that highlights the key aspects of your data.
Using `xlim` with Multiple Subplots
When working with multiple subplots, it’s important to maintain consistency. The following example sets the same x-axis limits across different subplots for uniformity:
subplot(2,1,1);
plot(x, sin(x));
xlim([0 10]); % Consistent limits for clarity
subplot(2,1,2);
plot(x, cos(x));
xlim([0 10]); % Matching limits across subplots
This practice ensures that all plots share a common perspective, enhancing comparability.
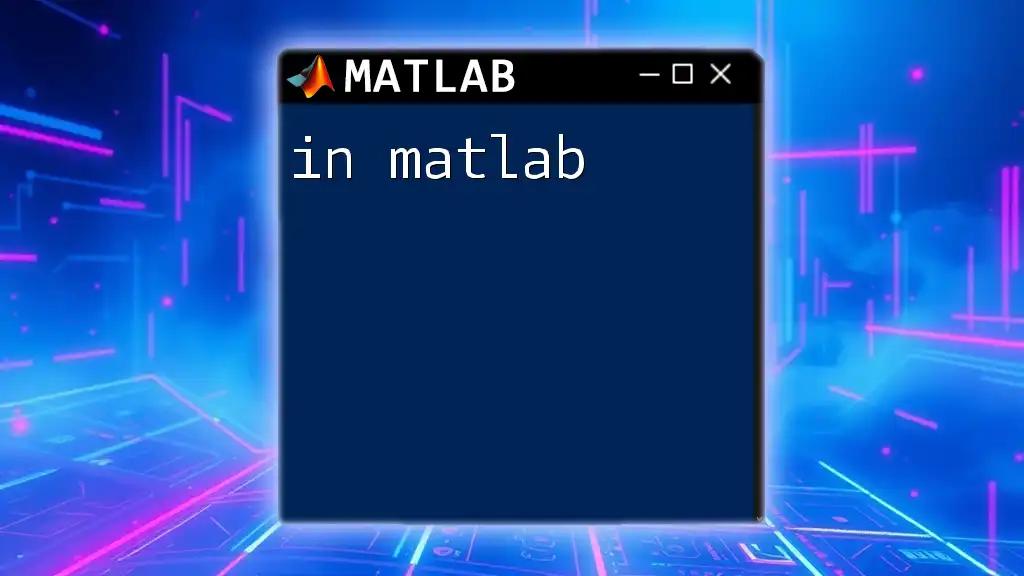
Common Mistakes and Troubleshooting
Overlapping Commands
One common issue users face is conflict between multiple commands affecting axis limits. If you have set `xlim` in one section of your code but modify x-axis limits elsewhere, MATLAB may override your previously defined limits. Always check the sequencing of your commands to avoid this.
Axis Limits Not Taking Effect
If you notice that your defined limits don’t appear to alter the plot, it might stem from other functions that adjust axis limits or from not applying `xlim` after the plot command, which should be checked carefully when debugging.
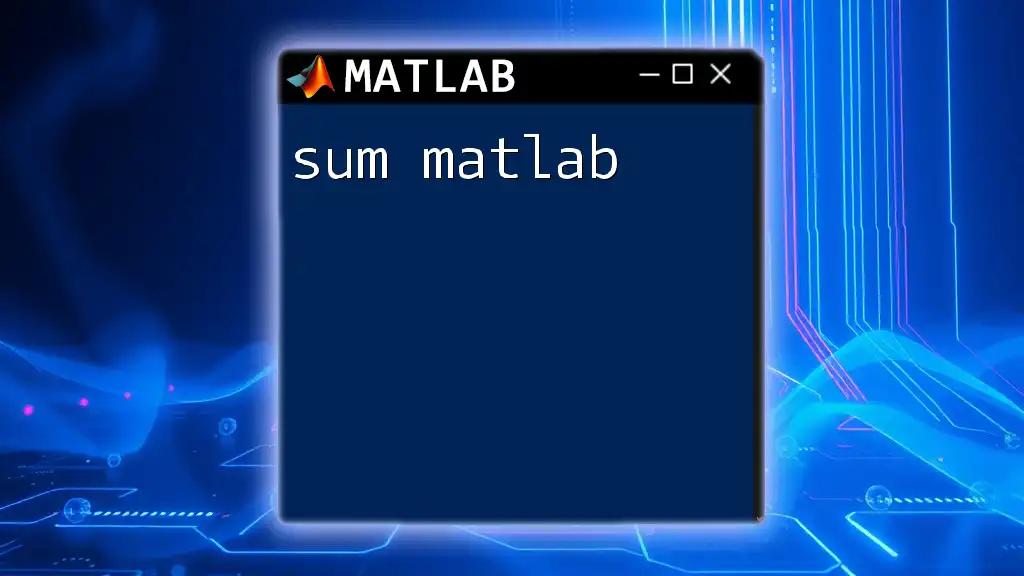
Conclusion
In summary, the `xlim` command in MATLAB is an essential tool for anyone looking to enhance the clarity and effectiveness of their data visualizations. By mastering the use of `xlim`, you can ensure that your plots not only convey the necessary information but do so in a visually appealing and easily interpretable manner.
Encourage experimentation with `xlim` within different contexts, whether it’s for detailed analysis or enhancing presentation quality. The better you can control the x-axis limits, the more impactful your visualizations will become.
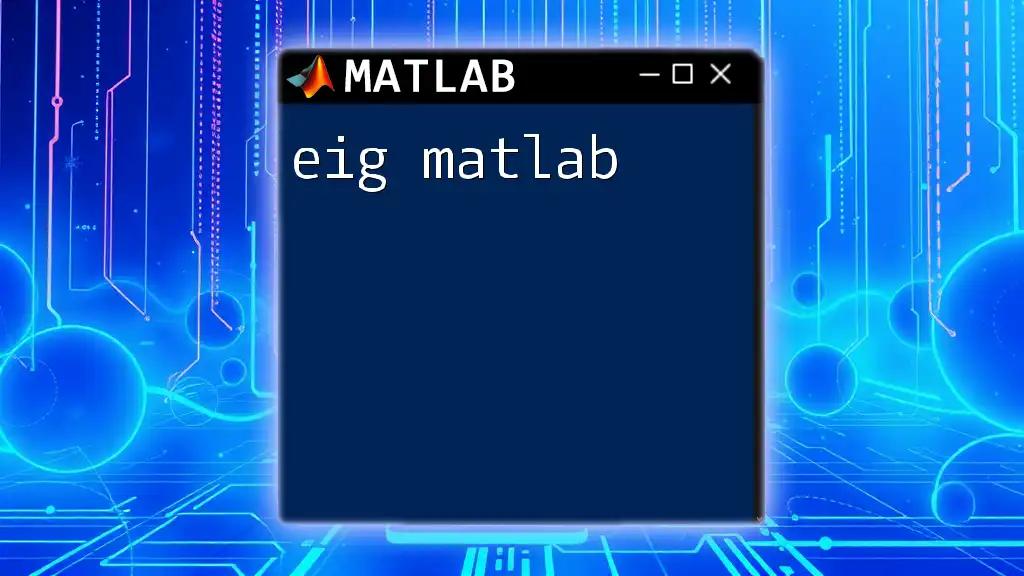
Additional Resources
For further study and exploration, consult the official MATLAB documentation which provides extensive coverage of `xlim` and related plotting tools. Additionally, consider engaging in community forums where users share insights and solutions related to specific challenges in MATLAB command usage. These interactions can deepen your understanding and enhance your skills.