UI in MATLAB refers to the various graphical user interface components that allow users to create interactive applications, enhancing user experience through visual elements and controls.
Here’s a simple example of creating a basic user interface with a button:
f = figure('Position', [100, 100, 300, 200]);
uicontrol('Style', 'pushbutton', 'String', 'Click Me', 'Position', [100, 80, 100, 40], 'Callback', @(src, event) disp('Button Clicked!'));
Understanding MATLAB's UI Components
What are UI Components?
UI components are the building blocks of user interfaces that facilitate interaction between users and applications. In MATLAB, these components serve as visual elements through which users can input data, initiate actions, and receive feedback. A well-designed UI enhances user experience and makes applications more intuitive.
Common UI Components in MATLAB
Buttons: Buttons in MATLAB are essential for triggering actions such as calculations or data submissions.
uicontrol('Style', 'pushbutton', 'String', 'Calculate', ...
'Position', [20 20 100 30], ...
'Callback', @calculateCallback);
In this example, we create a button labeled "Calculate". The `Callback` property specifies a function (`calculateCallback`) that will be called when the user interacts with the button.
Panels: Panels help organize UI components by grouping related elements together. They provide a way to create a structured layout.
uipanel('Title', 'Input Parameters', ...
'Position', [0.1 0.1 0.8 0.8]);
This code creates a panel titled "Input Parameters", which can house various UI controls.
Menus: Menus allow users to make selections from a list of options. These can be dropdown menus or context menus.
uimenu('Label', 'File', ...
'Callback', @fileMenuCallback);
With this code, a menu labeled "File" is created, which will call the function `fileMenuCallback` when selected.
Figures: The figure is the primary container for all UI components in MATLAB. It serves as a canvas on which you build your UI.
hFig = figure('Name', 'Simple UI Example', ...
'Position', [100, 100, 500, 400]);
Here, a simple figure is created named "Simple UI Example" with specified dimensions.
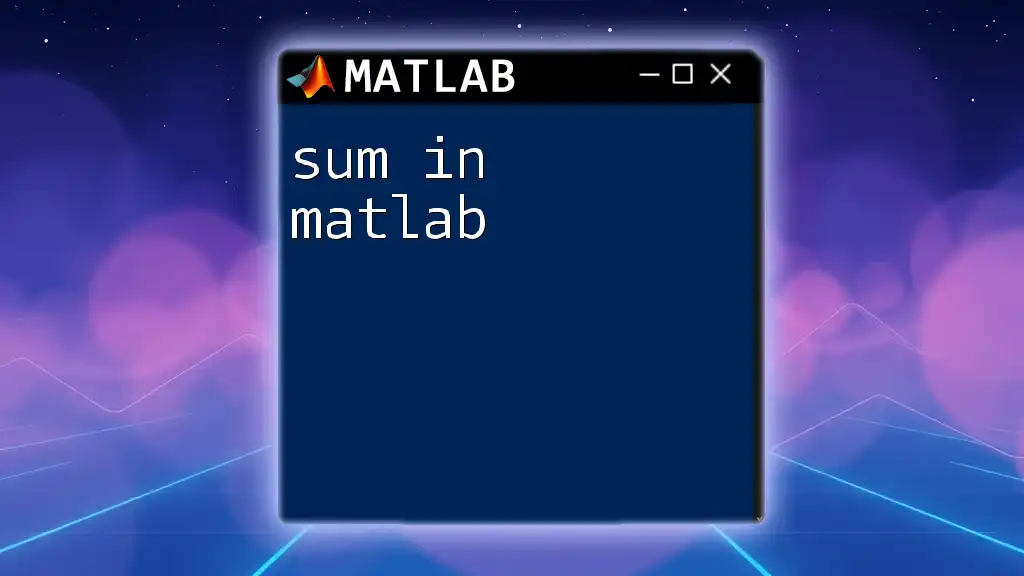
Building a Simple UI in MATLAB
Setting Up the UI Environment
To build a UI, first, you create a main figure that will hold all other components. Understanding the `Position` and `Size` properties is crucial to ensuring the layout is user-friendly. For instance, the `Position` property is specified in the format `[left bottom width height]`, where all dimensions are in pixels.
Integrating UI Components
Building a simple calculator UI can exemplify the integration of UI components well. Follow these steps to get started:
- Create the main figure to house the calculator.
- Add buttons for digits and operations like addition, subtraction, etc.
- Insert a display text area to show the ongoing calculations.
An example code snippet for creating this calculator might look as follows:
hFig = figure('Name', 'Simple Calculator', 'Position', [100, 100, 300, 400]);
% Display Area
uicontrol('Style', 'text', 'String', '0', ...
'Position', [20 350 260 30], ...
'Tag', 'DisplayArea');
% Number Buttons
uicontrol('Style', 'pushbutton', 'String', '1', ...
'Position', [20 300 60 60], 'Callback', @buttonCallback);
% Add more buttons as needed...
% Operation Buttons
uicontrol('Style', 'pushbutton', 'String', '+', ...
'Position', [200 300 60 60], 'Callback', @operationCallback);
The `DisplayArea` shows calculations, while the number and operation buttons handle user input.
Callback Functions
Callback functions are central to making an interactive UI. These functions are invoked upon specific user actions, enabling dynamic responses. Here's an example function that updates the display when a button is clicked:
function buttonCallback(src, ~)
display = findobj('Tag', 'DisplayArea');
newValue = get(display, 'String');
set(display, 'String', [newValue, src.String]);
end
This function retrieves the current display text and appends the value of the button that was clicked.
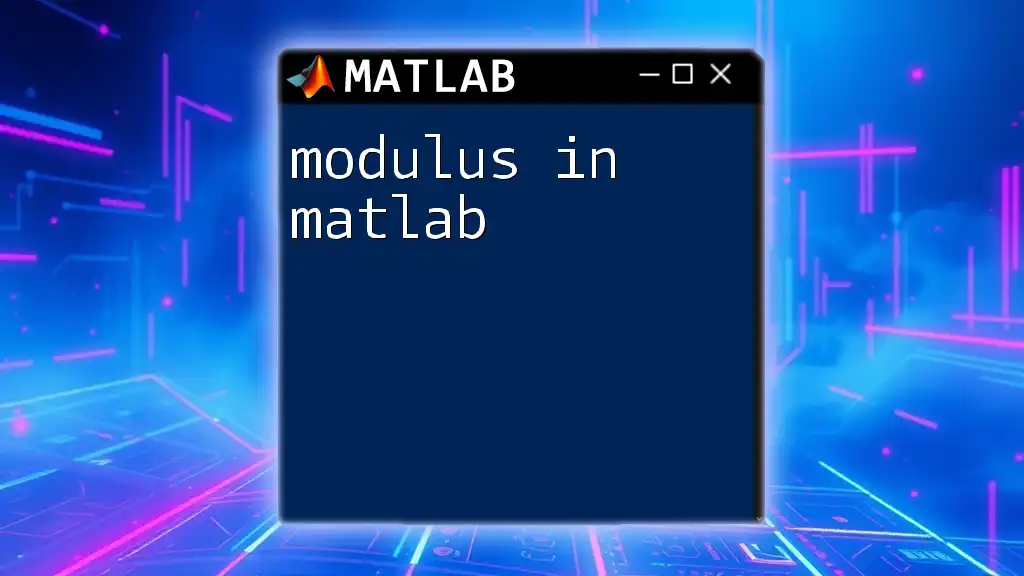
Advanced UI Techniques
Customizing UI Appearance
Customizing the appearance of UI components can significantly impact user experience. Change properties like color, size, and font to create a visually appealing interface.
set(hFig, 'Color', [0.8, 0.8, 0.8]); % Set figure background color
set(display, 'FontSize', 16, 'BackgroundColor', [1, 1, 1]); % Customize display
Advanced Layout Management
Utilizing `uigridlayout` simplifies managing complex layouts. This function automatically adjusts the placement of UI components in a grid format, giving you more flexibility. For example:
grid = uigridlayout(hFig, 3, 2);
uicontrol(grid, 'Style', 'pushbutton', 'Text', '1');
uicontrol(grid, 'Style', 'pushbutton', 'Text', '2');
With `uigridlayout`, your components will arrange themselves neatly across the specified rows and columns.
Handling User Inputs
MATLAB also provides functions to gather user inputs through dialogs. For example:
userInput = inputdlg('Enter a number:', 'Input', [1 35]);
This code snippet creates an input dialog prompting the user to enter a number.
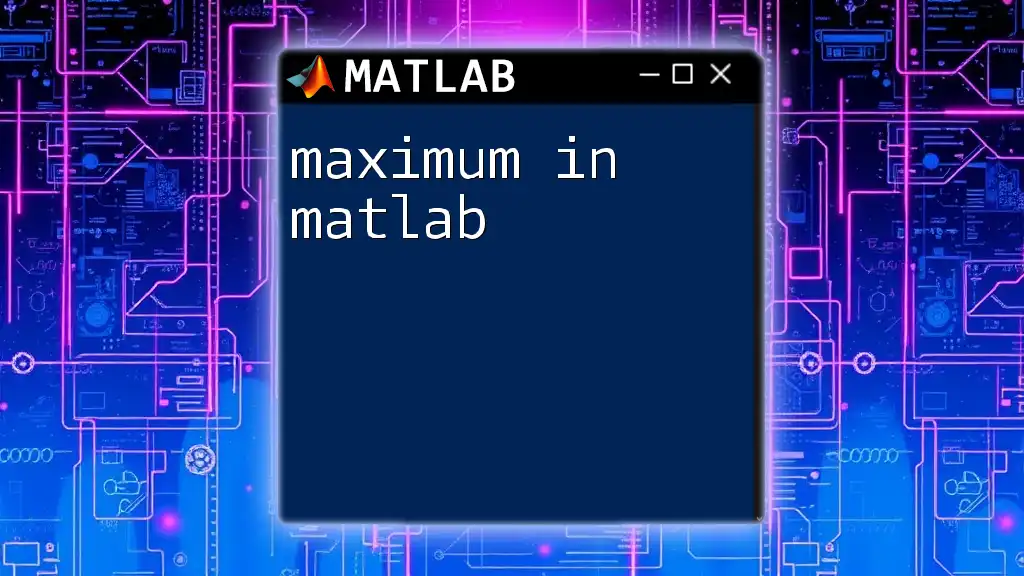
Best Practices for Designing UIs in MATLAB
User-Centered Design Principles
To ensure your UI is user-friendly, apply user-centered design principles. Understand your users’ needs and preferences, and design with those in mind. Prominently place navigation elements and provide clear labels to create an intuitive experience.
Optimizing Performance
When creating sophisticated UIs, optimize performance to minimize lag. Efficient coding practices, such as vectorization and minimizing the use of loops, contribute to a more responsive interface. Use listeners and updating strategies to efficiently manage UI updates without dragging down performance.
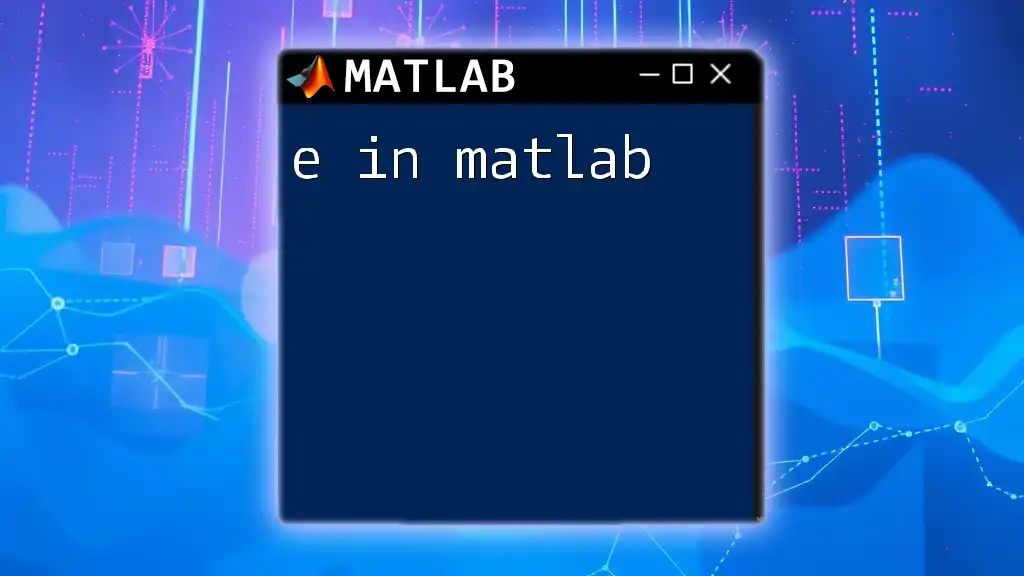
Troubleshooting Common UI Issues
Common Problems
Some of the common issues that may arise when building UIs include components not displaying correctly or callbacks not executing. Often, these issues can be resolved by ensuring that all properties are set correctly and that functions are properly defined and accessible in the current context.
Debugging Techniques
MATLAB provides robust debugging tools to assist in identifying and resolving problems. Utilize breakpoints within callback functions to step through code execution and gain insights into variable states and flow. It is often beneficial to print out values or states to the console to track down the source of errors.
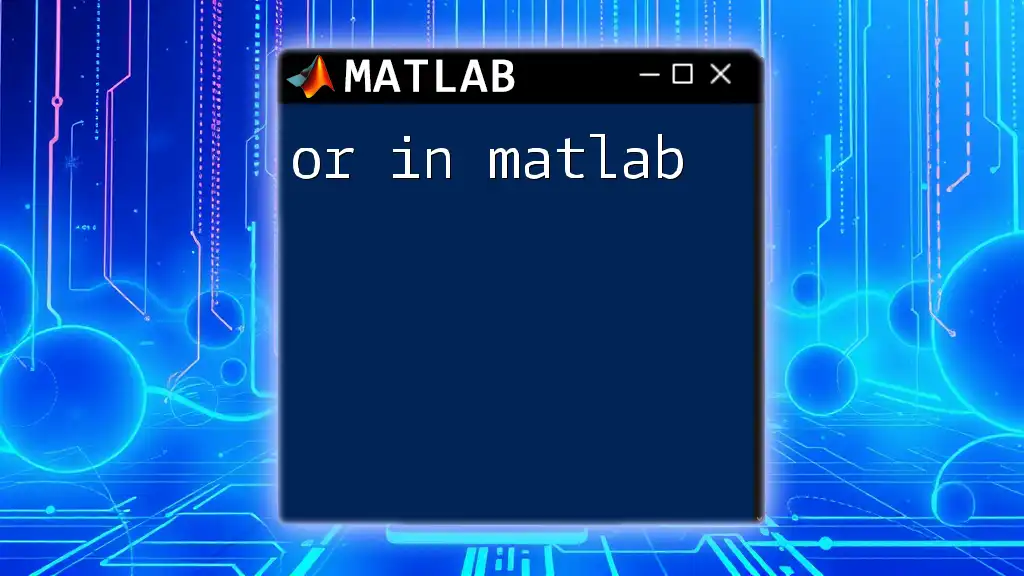
Conclusion
In this guide, we explored the fundamentals of UI in MATLAB, covering essential components, building a simple calculator, and more advanced techniques for creating responsive and attractive interfaces. As you delve deeper into UI design, remember that practice is key to mastering these concepts. Continued exploration and experimentation will enhance your capability to develop functional and user-friendly applications in MATLAB.
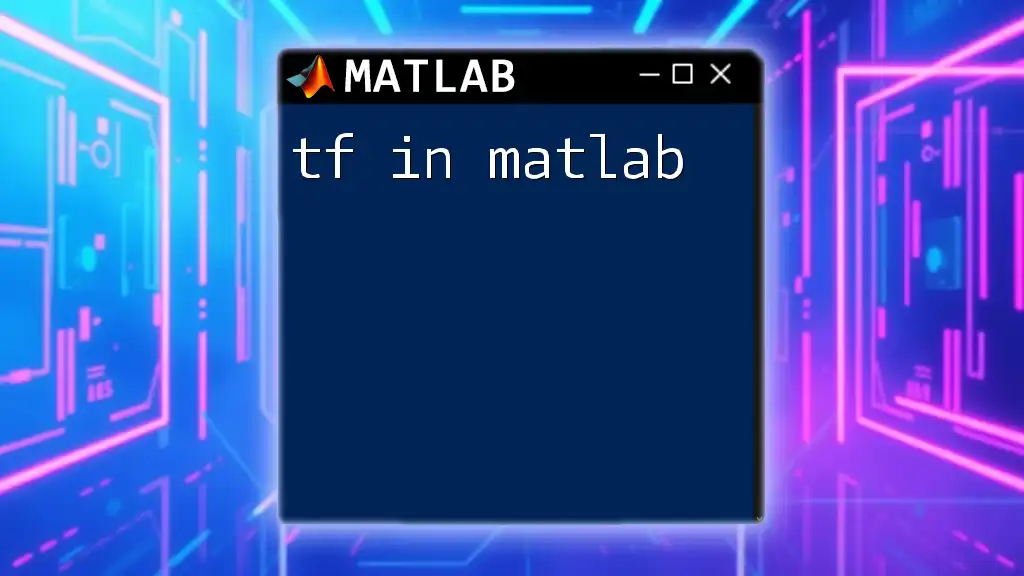
Additional Resources
For further learning, refer to MATLAB's official documentation, which offers extensive resources and examples. Community forums and online tutorials can also provide valuable insights and support in your journey to create effective UIs in MATLAB. Happy coding!