In MATLAB, the `if` statement executes a block of code conditionally based on whether a specified logical expression evaluates to true.
x = 10;
if x > 5
disp('x is greater than 5');
end
Understanding the Syntax of the "if" Statement
Basic Structure of the "if" Statement
The if in MATLAB statement has a straightforward structure that is essential for control flow in your code. At its core, the syntax consists of:
if condition
% Code to execute if condition is true
end
This structure begins with the keyword `if`, followed by a condition that evaluates to either true or false. If the condition is true, the code block within the `if` statement executes; if not, MATLAB simply skips to the next line after the `end` keyword.
Adding the "else" Clause
To manage scenarios where the condition is false, you can introduce an `else` clause. This allows you to define an alternative code block that executes when the initial condition fails.
if condition
% Code if condition is true
else
% Code if condition is false
end
This expanded structure supports clearer decision-making in code, leading to better programming practices.
Using "elseif" for Multiple Conditions
When you need to evaluate multiple conditions, `elseif` is your best friend. You can use this construct to create a chain of conditions, each of which is evaluated in sequence.
if condition1
% Code for condition1
elseif condition2
% Code for condition2
else
% Code if none of the conditions are true
end
This allows for more complex decision trees to be handled efficiently, minimizing redundancy in your code.
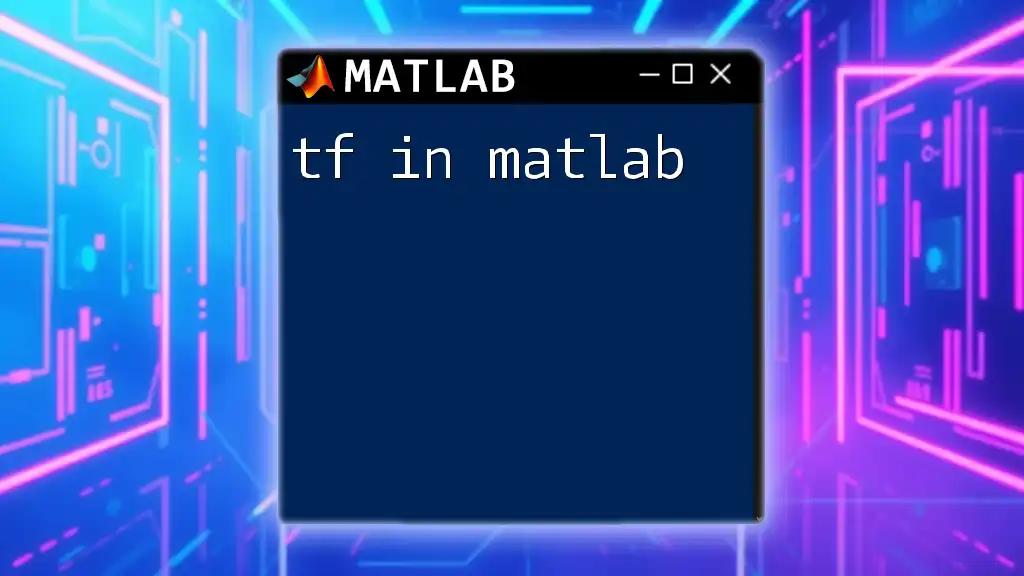
Examples of the "if" Statement in Action
Example 1: Simple Number Comparison
A practical implementation of the if in MATLAB statement can be illustrated through a number comparison task. Imagine you want to find out which of two numbers is larger:
a = 10;
b = 20;
if a > b
disp('a is greater than b');
else
disp('b is greater than a');
end
In this example, if `a` is greater than `b`, the output will indicate that. If not, it will state that `b` is larger. Exploring such problems lays the foundation for understanding conditional logic.
Example 2: Grading System
To further demonstrate the power of conditions, let’s create a grading system based on numeric scores.
score = 85;
if score >= 90
grade = 'A';
elseif score >= 80
grade = 'B';
elseif score >= 70
grade = 'C';
else
grade = 'F';
end
In this scenario, the program evaluates the `score` variable and assigns a letter grade accordingly. This example highlights not only how to chain conditions but also how important logical evaluation is for practical applications.

Nested "if" Statements
Understanding Nested Conditions
Nested "if" statements allow you to check for conditions within other conditions. This can be invaluable when you have multiple layers of decision-making.
a = 5;
if a > 0
disp('Positive number');
if mod(a, 2) == 0
disp('Even number');
else
disp('Odd number');
end
end
In this code, first, the program checks if `a` is positive. If it is, it then checks if it is even or odd. This structure empowers you to handle more specific evaluations based on earlier conditions.
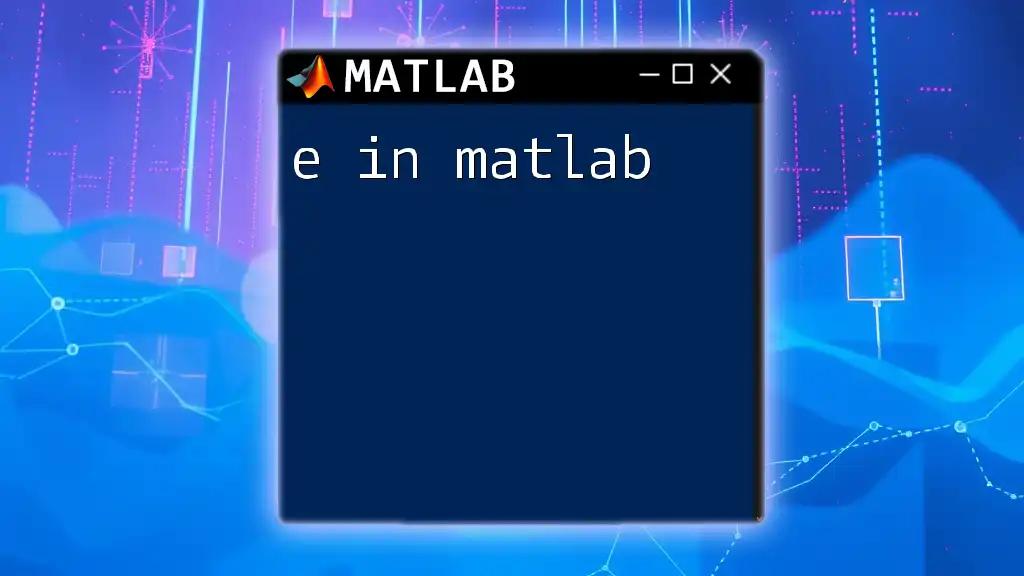
Best Practices for Using Conditional Statements
Keep It Simple and Readable
When implementing if in MATLAB, it is crucial to maintain clarity and simplicity. Overly complicated structures can lead to confusion and bugs. Aim for a clean layout that reflects the logical path your code follows.
Use Clear Conditions
Writing clear and descriptive conditions improves the readability of your code. Instead of using cryptic variables or complex expressions, focus on clarity, which will benefit you and anyone who reads your code in the future.
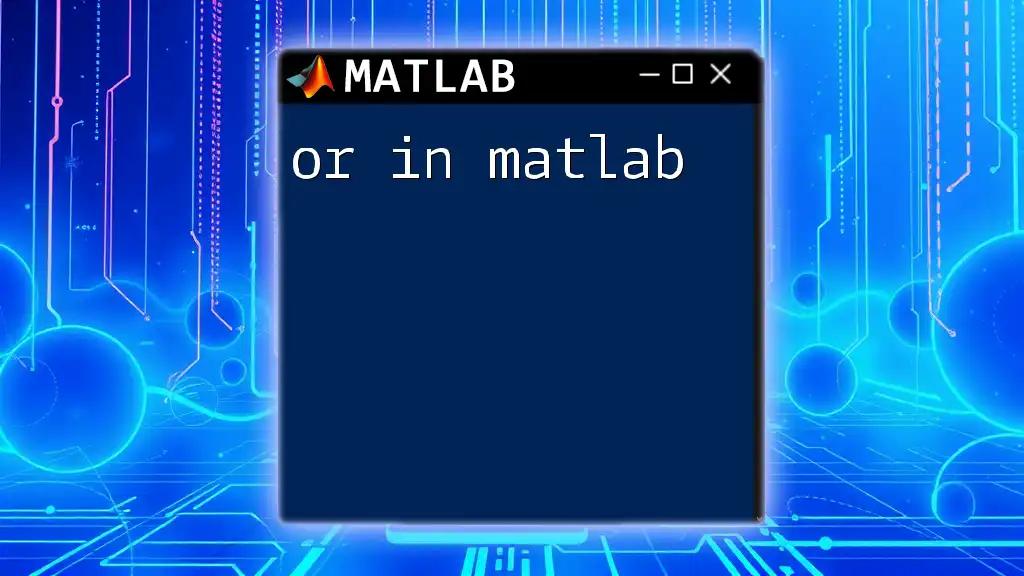
Common Mistakes and How to Avoid Them
Forgetting the "end" Keyword
A frequent mistake among newcomers is neglecting to close `if` statements properly with the `end` keyword. Always ensure that each `if` statement is paired with its corresponding `end` to avoid syntax errors.
Overcomplicating Conditions
Overly complex conditional expressions can lead to logical errors. Strive for clarity by breaking down conditions into simpler, easy-to-understand statements. If a condition feels convoluted, consider restructuring it.
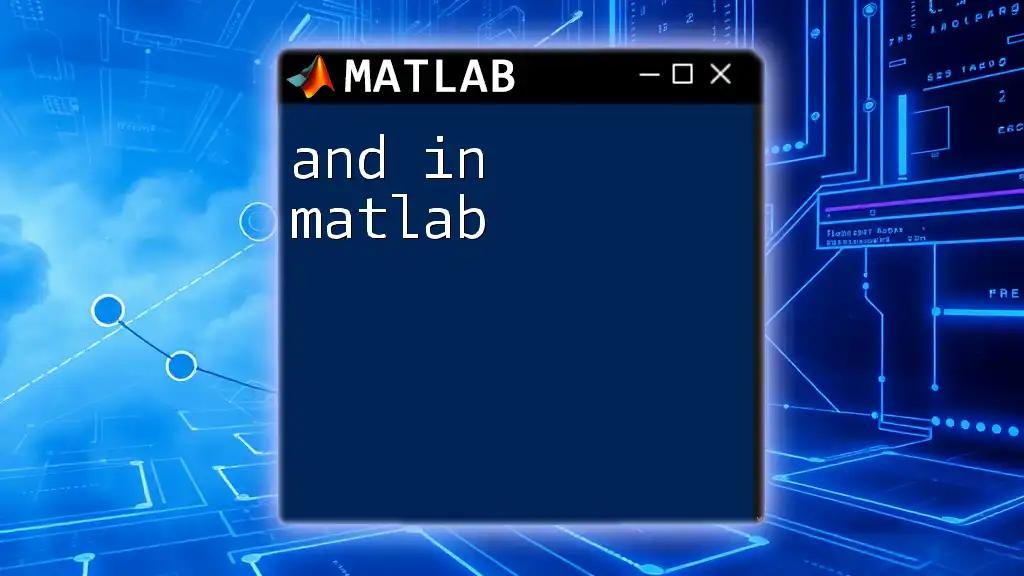
Conclusion
In summary, the if in MATLAB statement is a fundamental building block of control flow in programming. From simple comparisons to complex grading systems and nested evaluations, mastering this tool is essential for any MATLAB user.
As you practice writing and reworking these statements, remember to prioritize readability and logic. The more comfortable you become, the easier it will be to write robust and efficient code.
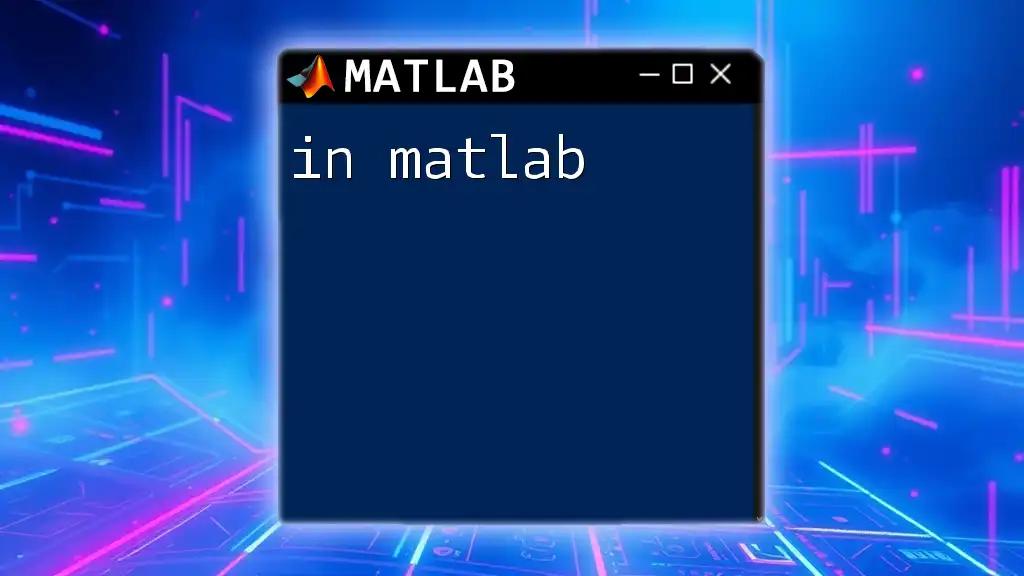
Additional Resources
To further enhance your understanding, consider exploring additional tutorials, MATLAB’s official documentation, and targeted programming books. Each of these resources can provide deeper insights and broader knowledge about conditional statements and their applications in MATLAB.