A MATLAB cheat sheet is a quick reference guide that consolidates essential commands and syntax to help users efficiently utilize MATLAB for their programming and analytical tasks.
Here's a simple example of loading a matrix and calculating its mean:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a matrix
mean_A = mean(A(:)); % Calculate the mean of all elements in A
What is a MATLAB Cheat Sheet?
A MATLAB cheat sheet serves as a quick reference guide, summarizing essential commands, functions, and workflows. It is particularly beneficial for users at all levels—from beginners to experienced professionals—who wish to enhance their efficiency and effectiveness when using MATLAB. By having a cheat sheet, users can avoid the endless search for commands, allowing them to focus more on problem-solving and analysis.
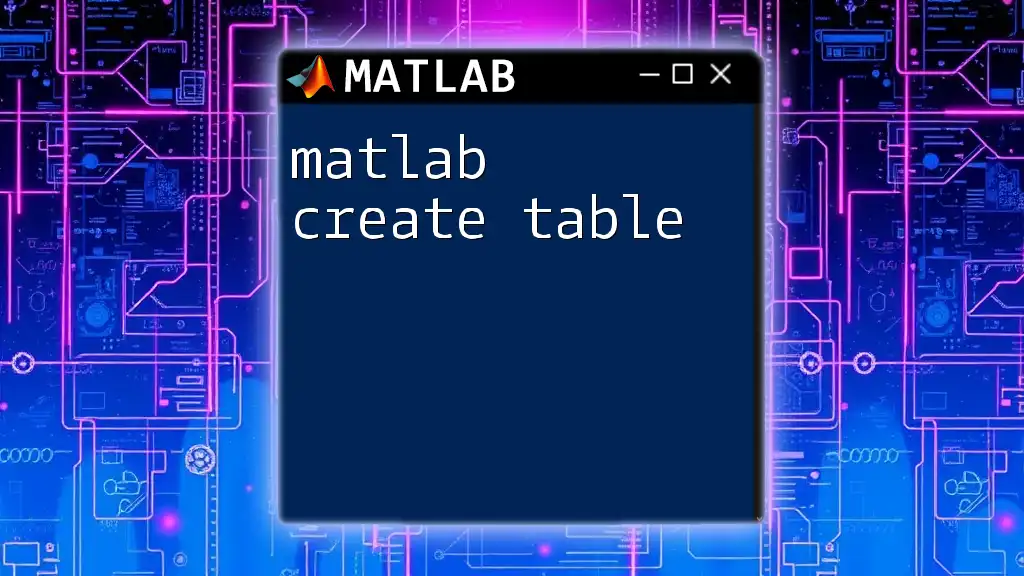
Basic MATLAB Commands
Starting and Exiting MATLAB
To kick off your MATLAB journey, you need to launch the application. The method may differ based on your operating system. For Windows, click on the MATLAB icon; for macOS, use Finder or Launchpad. To exit MATLAB, simply type the command below in the command window:
exit
This command gracefully closes the MATLAB session.
Working with Variables
Variables are central to any programming environment. In MATLAB, creating variables is straightforward. For example:
a = 10;
Here `a` is assigned the value of 10. It's essential to note variable naming conventions: variable names must start with a letter and can include letters, numbers, and underscores.
To display a variable’s value in the command window, you can use:
disp(a);
If you want to clear variables from workspace memory, employ either of the following commands:
clear a; % Removes variable 'a'
Or, to clear all variables, use:
clear all; % Caution: this removes all variables!
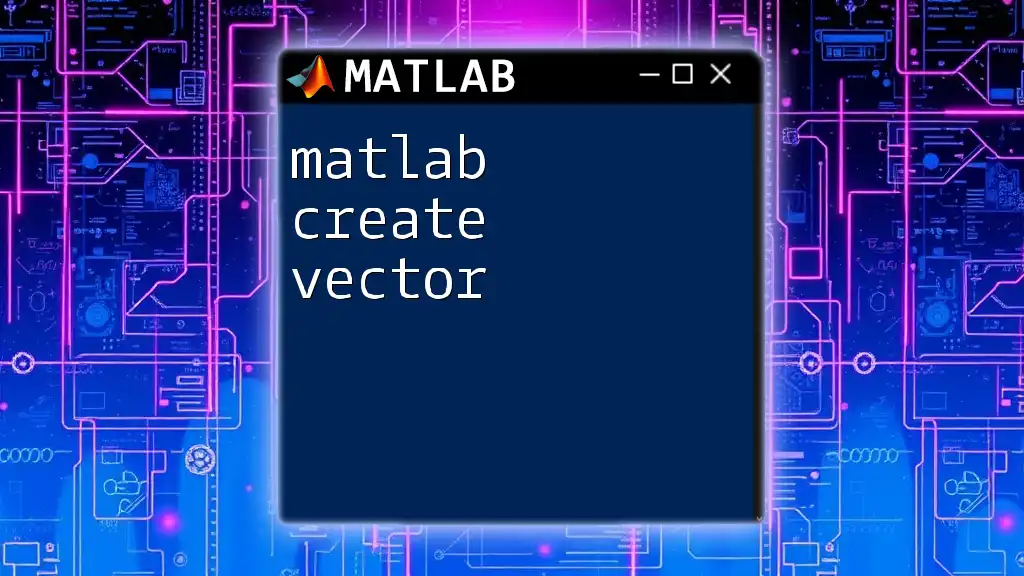
Data Types in MATLAB
Common Data Types
MATLAB supports various data types, crucial for different mathematical operations. The most common are:
- Numeric: Used for integers, floating-point numbers.
- Character: Stores text strings.
- Logical: Represents true/false values.
Examples of Data Types
For instance, to define numeric data:
b = 5.67; % A floating-point number
To create a character array:
str = 'Hello, MATLAB!'; % A string of characters
To assign a logical value:
isTrue = true; % Logical true value
Understanding these data types is vital for effective programming in MATLAB.
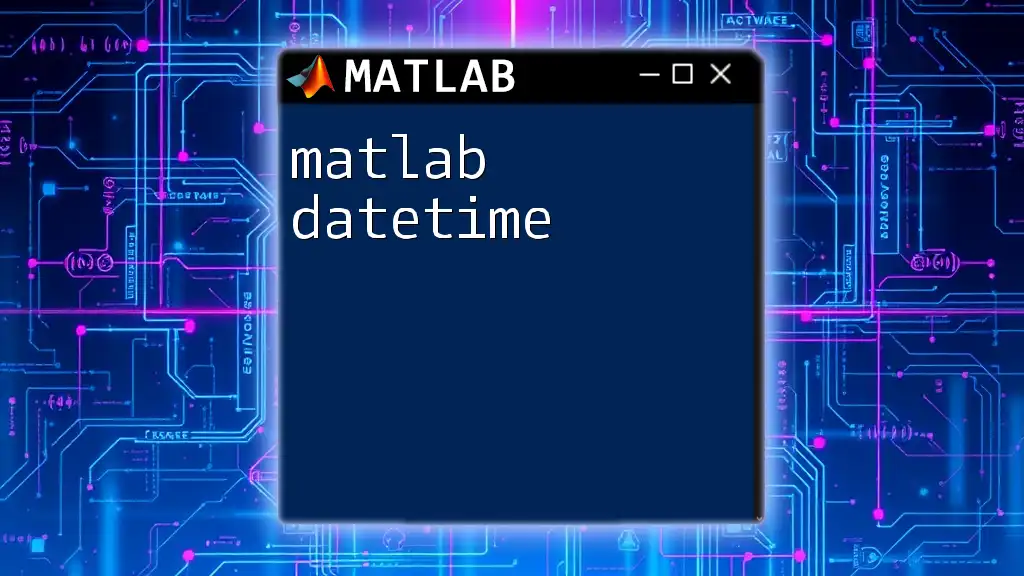
Essential MATLAB Functions
Math Functions
MATLAB offers a rich set of built-in math functions. Here are some basic yet powerful ones:
-
Sum of elements: Aggregate all elements within an array.
total = sum([1, 2, 3]); % Result: 6
-
Mean of an array: Calculate the average of elements.
average = mean(data); % Computes the mean of 'data'
-
Standard Deviation: Quantify the amount of variation or dispersion.
deviation = std(data); % Finds standard deviation of 'data'
Matrix Manipulation Functions
Creating and manipulating matrices is at the core of MATLAB:
Creating a matrix:
A = [1, 2; 3, 4]; % A 2x2 matrix
Accessing elements:
You can easily retrieve values from a matrix:
element = A(1,2); % Accesses the element in the first row and second column
Matrix Operations can be performed directly:
C = A + A; % Matrix addition
D = A * A; % Matrix multiplication
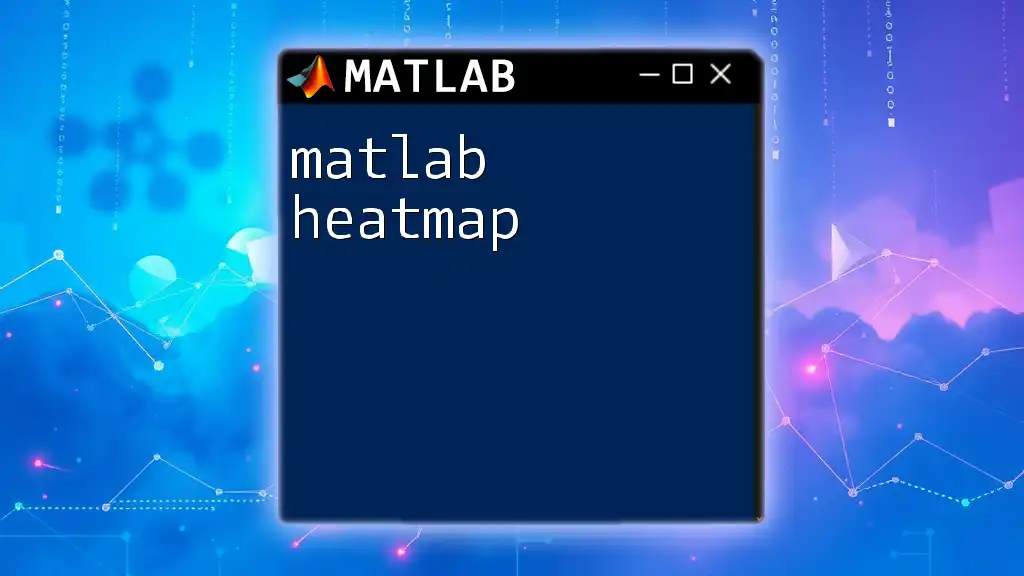
Plotting in MATLAB
Introduction to Plotting
Visualization is key in data analysis, and MATLAB provides extensive plotting functionalities to make this easy.
Basic Plotting Commands
To create a simple line plot, use the following example:
x = 0:0.1:10;
y = sin(x);
plot(x, y); % Plots a sine wave
Additional Plot Types you might find helpful include:
-
Scatter plots for showing relationships:
scatter(x, y); % Displays points defined by ‘x’ and ‘y’
-
Bar graphs for categorical data:
bar(data); % Creates a bar graph of the provided data
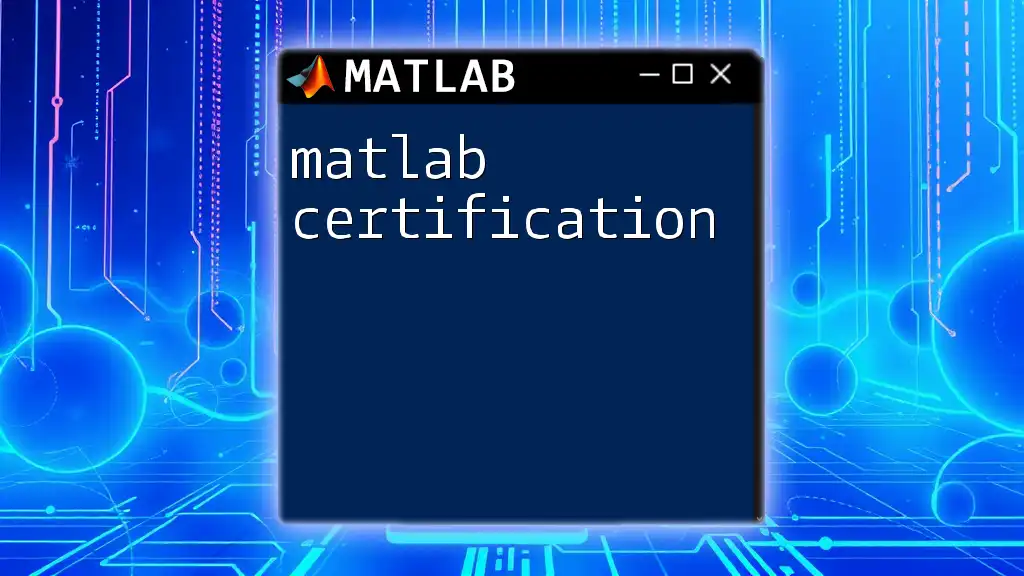
Control Flow in MATLAB
Conditional Statements
Control flow constructs allow for decision-making within your code.
If Statements
The syntax for if statements in MATLAB enables iterative evaluations:
if a > b
disp('a is greater than b');
end
Switch Statements
You may also utilize a switch statement which provides an alternative to lengthy if-else chains.
switch value
case 1
disp('Value is 1');
case 2
disp('Value is 2');
otherwise
disp('Value is not 1 or 2');
end
Loops
Looping constructs help automate repetitive tasks.
For Loop
The standard for loop structure in MATLAB looks as follows:
for i = 1:5
disp(i); % Displays numbers from 1 through 5
end
While Loop
To execute code as long as a condition holds true, consider the while loop:
i = 1;
while i <= 5
disp(i);
i = i + 1; % Increment
end
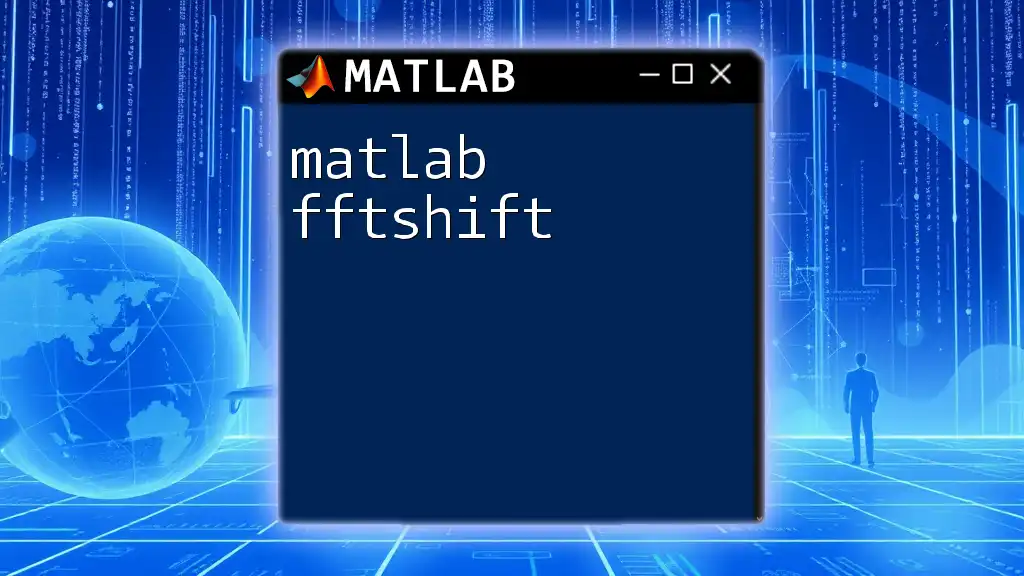
Functions in MATLAB
Creating Functions
Defining functions allows for reuse of code and modular programming. A simple function to calculate the area of a circle is:
function area = calcArea(radius)
area = pi * radius^2; % Calculates area using the radius provided
end
Built-in Functions
MATLAB comes with a myriad of built-in functions that simplify your coding, including `size`, `length`, and more. Familiarizing yourself with these can significantly streamline your workflow.
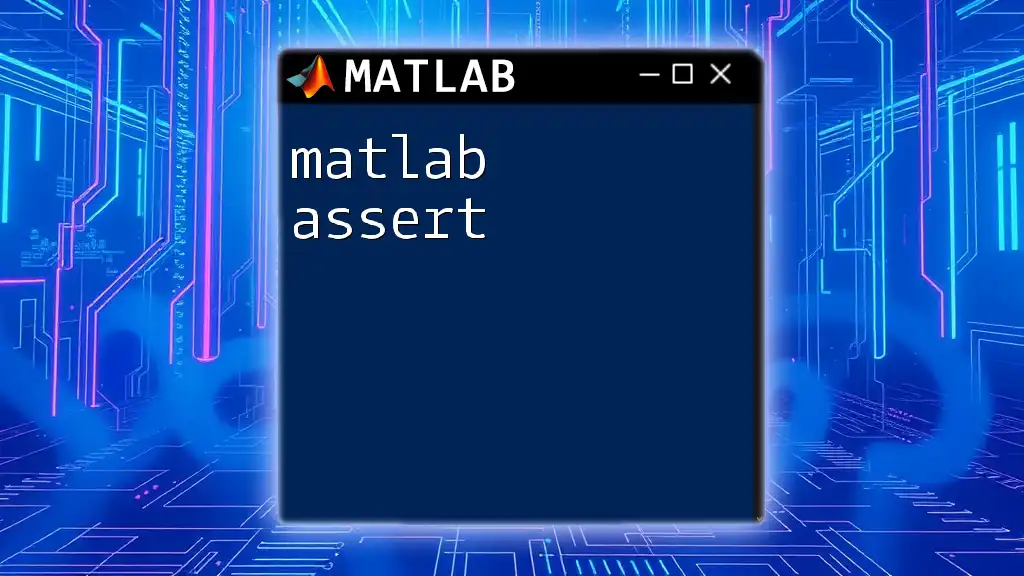
MATLAB Toolboxes
Popular Toolboxes Explained
MATLAB also provides toolboxes—collections of special-purpose functions that extend MATLAB’s capabilities.
- Image Processing Toolbox: Offers tools for image enhancement and manipulation.
- Statistics and Machine Learning Toolbox: Contains functions for statistical analysis and machine learning tasks.
Each toolbox is equipped with functions tailored to specific applications, as noted in the descriptions.
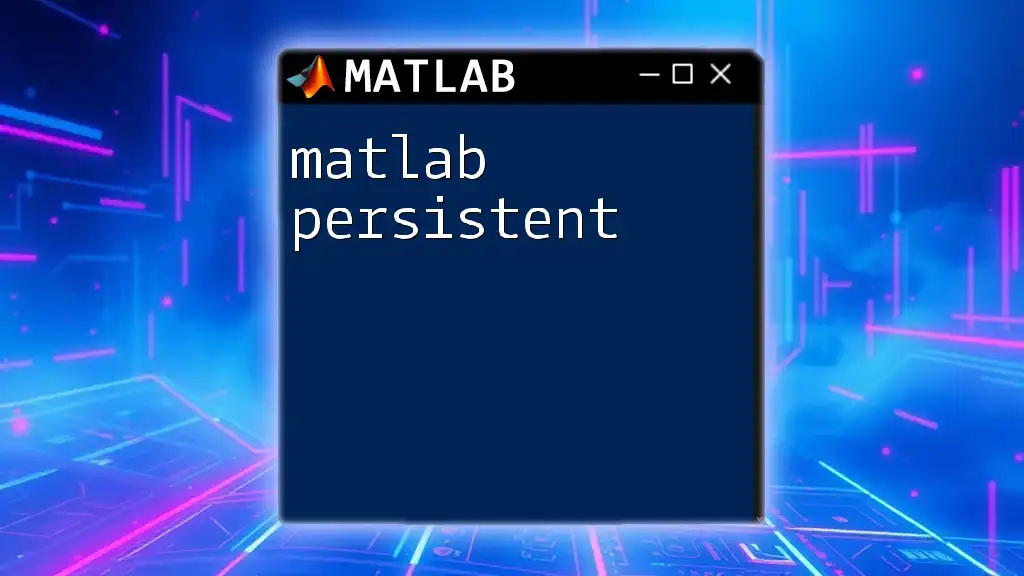
Tips for Using a MATLAB Cheat Sheet
To maximize the benefits of your MATLAB cheat sheet, consider the following tips:
- Use it for quick references: Keep it handy to minimize interruptions during coding.
- Organize commands: Categorize commands based on functionality for easy access.
- Personalize your notes: Add comments or examples that are relevant to your unique work style.
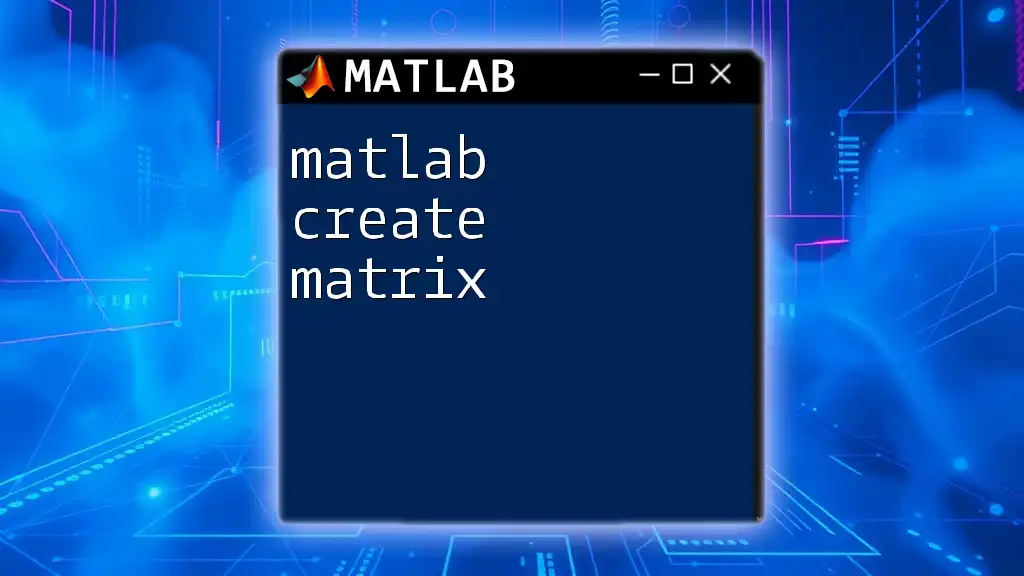
Conclusion
Incorporating a MATLAB cheat sheet into your learning and workflow can significantly heighten your efficiency and ease of use. By familiarizing yourself with essential commands and practices highlighted in this guide, you can augment your MATLAB skills effectively. Always remember, practice makes perfect, so explore further and experiment with the vast functionalities MATLAB offers.
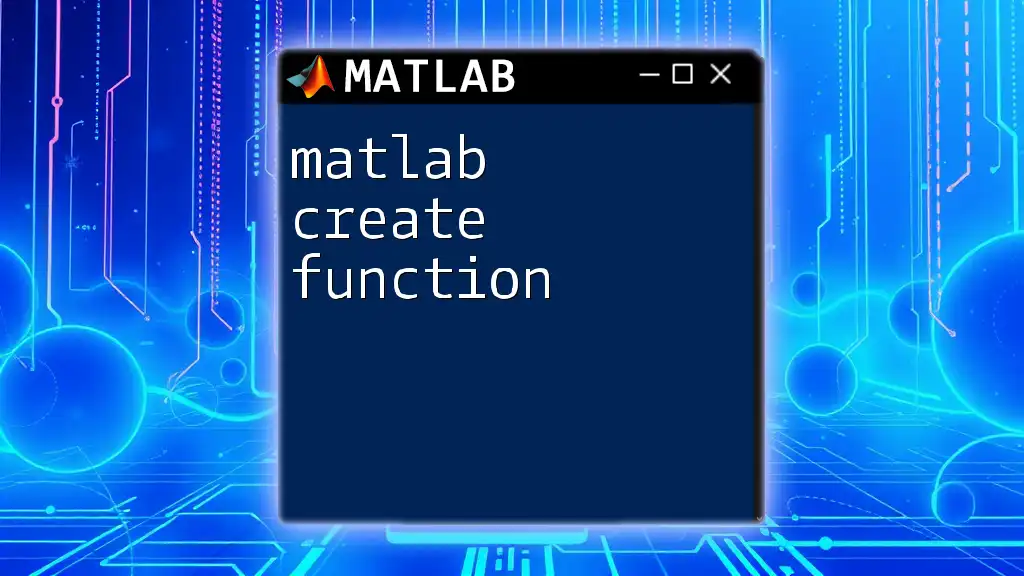
Additional Resources
For more in-depth learning resources, consider exploring recommended books, authoritative websites, and vibrant online forums. Networking with communities dedicated to MATLAB can introduce you to a wealth of knowledge and support as you advance in your journey.