The `filtfilt` function in MATLAB applies a zero-phase digital filtering to a signal by performing filtering in both the forward and reverse directions, effectively removing phase distortion.
Here’s a simple example of how to use `filtfilt` to apply a low-pass Butterworth filter to a noisy signal:
% Define a sample signal
fs = 1000; % Sampling frequency
t = 0:1/fs:1; % Time vector
signal = sin(2*pi*50*t) + randn(size(t))*0.5; % Noisy signal
% Design a low-pass Butterworth filter
[b, a] = butter(4, 0.1); % 4th order, cutoff frequency 0.1 * Nyquist
% Apply the filter using filtfilt
filtered_signal = filtfilt(b, a, signal);
% Plot original and filtered signal
figure;
plot(t, signal, 'r', t, filtered_signal, 'b');
legend('Noisy Signal', 'Filtered Signal');
title('Signal Filtering using filtfilt');
xlabel('Time (s)');
ylabel('Amplitude');
Understanding `filtfilt` in MATLAB
Definition and Purpose
The `filtfilt` function in MATLAB is designed to perform zero-phase filtering. This allows a signal to be processed without introducing any phase distortion, making it particularly useful in scenarios where signal integrity is crucial. Zero-phase filtering means that the output signal is delayed by zero samples, aligning the filtered signal with the original data.
In contrast to conventional filtering methods, `filtfilt` applies the filter in both forward and backward directions. This involves two passes of the data through the filter, which cancels out the phase shift of the filtering process, preserving the waveform shape of the original signal. This is essential when working with data that requires accurate representation, such as in biomedical signals or high-fidelity audio applications.
Key Features of `filtfilt`
One of the standout features of `filtfilt` is its ability to eliminate phase distortion, which is a common problem when utilizing standard filtering techniques. In practical applications, such as digital signal processing, phase distortion may misalign data points over time, leading to significant errors in signal interpretation.
Another critical advantage is the ability to preserve the amplitude of the signal. When filtering, maintaining the original signal's amplitude can be crucial for effective analysis. `filtfilt` ensures that, unlike traditional methods, the output signal retains its original energy, making it suitable for detailed analyses.
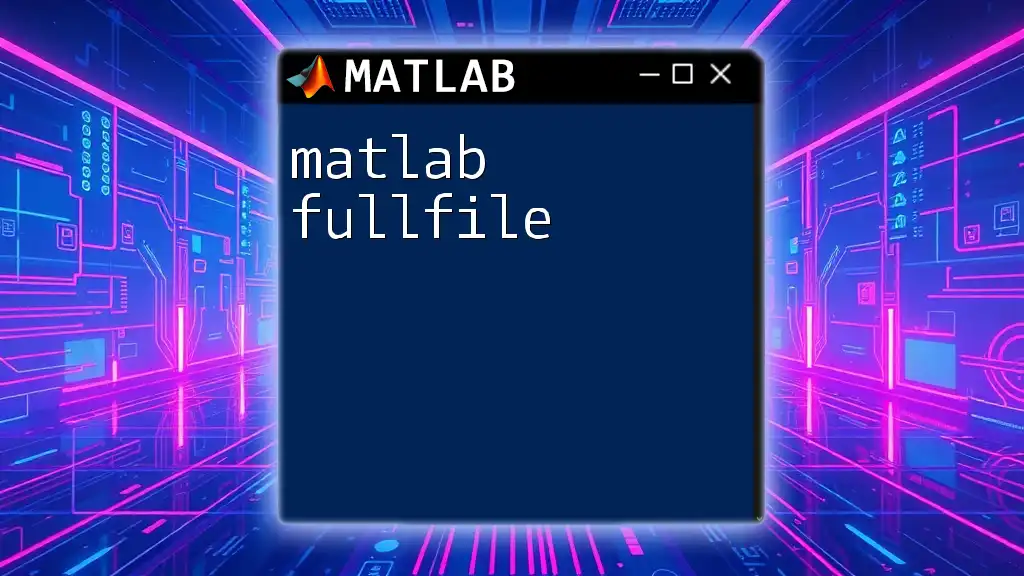
How `filtfilt` Works
The Mathematical Background
At its core, `filtfilt` executes a filtering algorithm that consists of two primary steps: forward filtering and backward filtering. The first stage applies the filter in the normal direction, processing the signal from start to finish. The second stage subsequently processes the result in reverse, moving from the end back to the start. This unique approach effectively cancels any phase shifts introduced during the first pass.
Filter coefficients play a pivotal role in the filtering process. These coefficients define how the filter behaves—determining its characteristics such as cut-off frequency and response type (e.g., low-pass, high-pass). Understanding and correctly configuring these coefficients is essential for effective filtering.
The `filtfilt` Function Syntax
The syntax for using the `filtfilt` function is straightforward:
y = filtfilt(b, a, x)
- `b`: This argument represents the numerator coefficients of the digital filter you wish to apply.
- `a`: This indicates the denominator coefficients of the filter, which, together with `b`, define the filter’s behavior.
- `x`: This is your input signal—the signal that you want to filter.
Examples of `filtfilt` Usage
Basic Example
To perform a basic filtering operation with `filtfilt`, you can begin by generating a simple signal. In this example, we create a noisy sine wave:
t = 0:0.001:1; % Create a time vector from 0 to 1 seconds with 1 kHz sample rate
x = sin(2*pi*5*t) + 0.5*randn(size(t)); % Create a signal with noise
Next, you need to design a low-pass filter to smooth the signal. A Butterworth filter is a common choice due to its smooth frequency response:
fs = 100; % Define the sampling frequency
fc = 10; % Define the cut-off frequency
[b, a] = butter(6, fc/(fs/2)); % Design a 6th-order Butterworth filter
Now you can apply the `filtfilt` function to filter the noisy signal:
y = filtfilt(b, a, x);
plot(t, x, 'b', t, y, 'r'); % Plot the original noisy and filtered signals
legend('Noisy Signal', 'Filtered Signal'); % Add a legend for clarity
title('filtfilt Example'); % Title for the plot
This example illustrates the efficacy of `filtfilt` in producing a cleaned-up version of your original signal.
Advanced Example with Multi-Dimensional Data
In more complex scenarios, you may encounter multi-dimensional data. The `filtfilt` function can handle these cases as well. Consider the following example using a mesh grid:
[X, t] = meshgrid(0:0.01:1, 0:0.1:10);
Y = sin(2*pi*X).*exp(-t); % Create a two-dimensional signal
To apply the filter along a specified dimension (in this case, the first dimension), you would use the following code:
filteredY = filtfilt(b, a, Y, [], 1); % Apply filtfilt along the 1st dimension
Ultimately, you can visualize the original and filtered data to observe the effects of the filtering process:
surf(X, t, Y);
title('Original Data'); % Title for the original data surface plot
figure;
surf(X, t, filteredY);
title('Filtered Data'); % Title for the filtered data surface plot
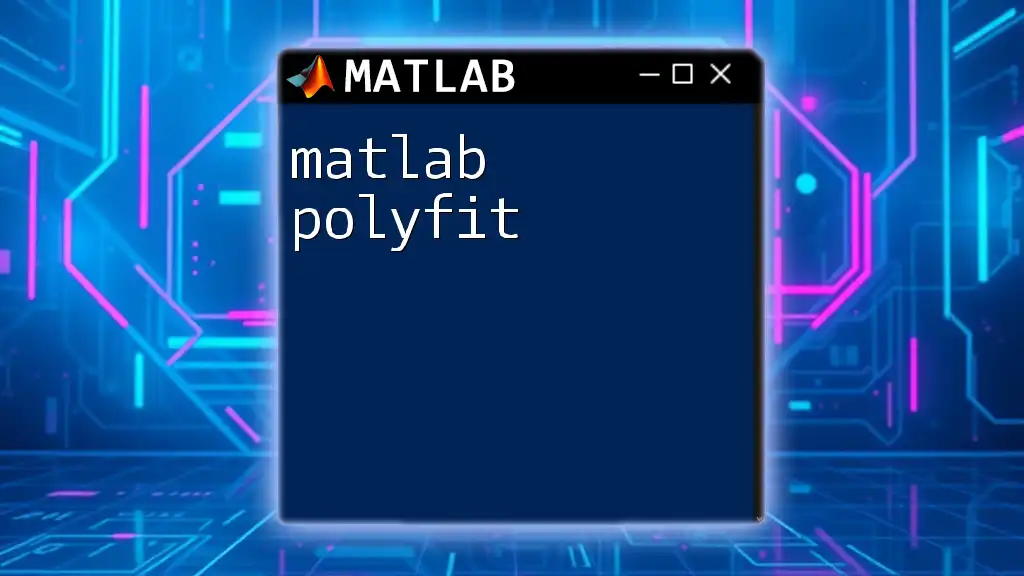
Common Applications of `filtfilt`
Signal Processing in Engineering
In engineering, `filtfilt` finds use in various signal processing applications, such as control systems where precision is vital. For instance, filtering sensor data can significantly reduce measurement noise, leading to better system performance.
Biomedical Applications
In biomedical fields, accurate data representation is critical. ECG and EEG signal processing often rely on `filtfilt` to maintain the integrity of waveforms. This zero-phase filtering ensures that the real-time analysis of these signals reflects true physiological states, which can be essential for diagnostics.
Financial Data Analysis
In finance, `filtfilt` can be instrumental for analyzing stock prices and trends. By filtering out short-term fluctuations, analysts can better identify underlying trends that can inform investment strategies.
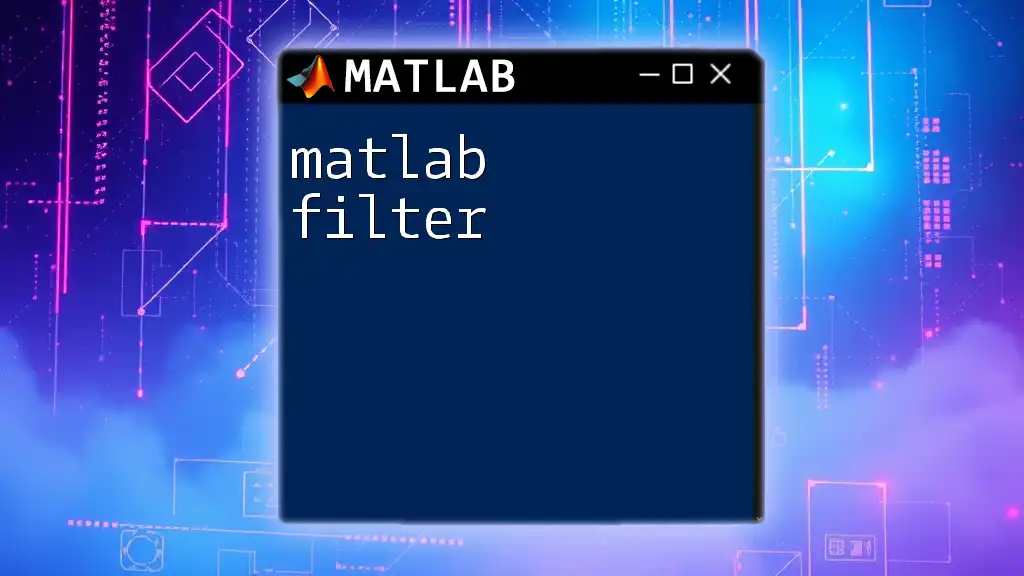
Tips for Using `filtfilt` Effectively
Choosing the Right Filter
Selecting an appropriate filter is essential for the success of your filtering task. Understanding the various filter types available in MATLAB, such as Butterworth, Chebyshev, and elliptic filters, will help you to choose the right one according to your specific needs and requirements. Moreover, determining the optimal filter order is crucial; a higher order may provide a sharper response but can also introduce ringing artifacts, which can distort the original signal.
Avoiding Common Pitfalls
When using `filtfilt`, it’s important to remain vigilant to common pitfalls. Overfitting can occur when using high-order filters, leading to an overly complex filter that can distort your results. Additionally, be cautious of edge artifacts that can appear at the beginning and end of the filtered signal, particularly in short signals.
Performance Considerations
For large datasets, the execution speed of filtering functions is essential. Ensure that your implementation is optimized for memory, especially if working with high-dimensional data. MATLAB's intrinsic vectorization capabilities can be particularly advantageous for enhancing performance in filter application.
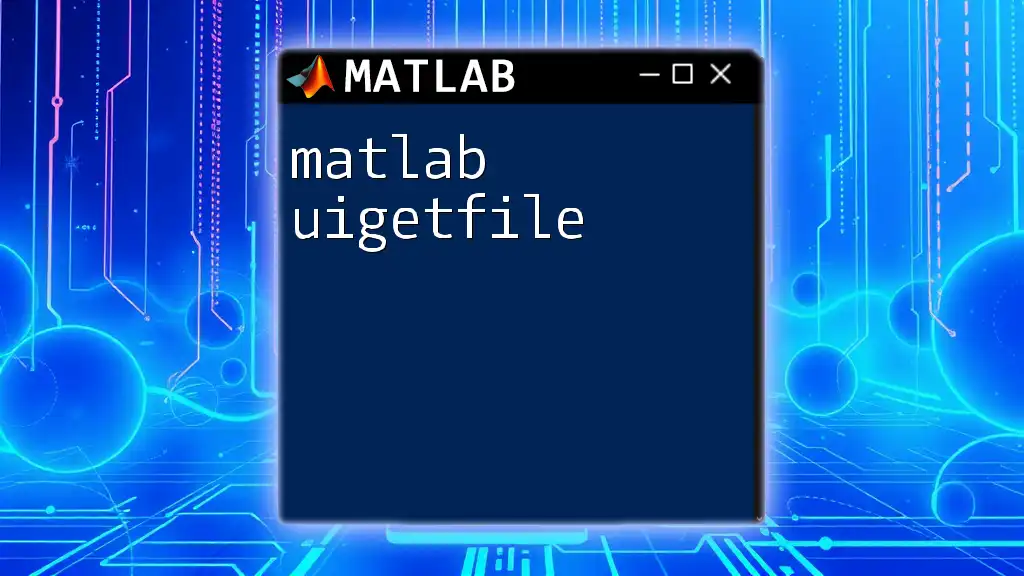
Conclusion
The `filtfilt` function in MATLAB is an indispensable tool for anyone involved in signal processing, data analysis, or related fields. By effectively eliminating phase distortion while preserving the integrity of the signal, it opens new avenues for accurate analysis across various applications. As you gain more experience with `filtfilt` and its companions in MATLAB's filtering suite, you will undoubtedly unlock more opportunities for insightful data representation and analysis.
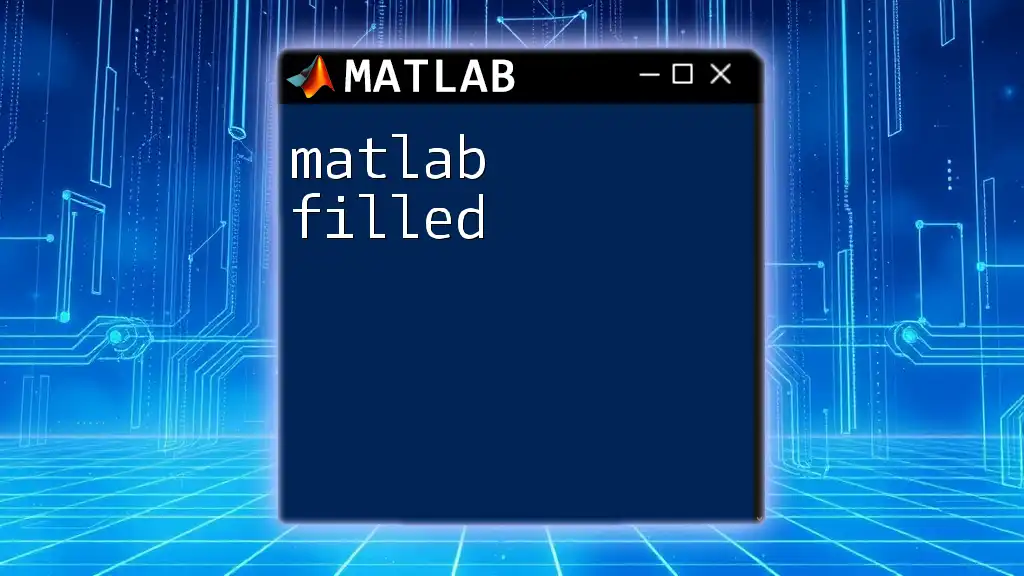
Additional Resources
To deepen your understanding of `filtfilt` and its applications, refer to the official MATLAB documentation, which provides a comprehensive overview of the function and related concepts.
Explore further reading and tutorials—including recommended books on signal processing and online courses—to enhance your expertise and knowledge in using MATLAB for complex data analysis.
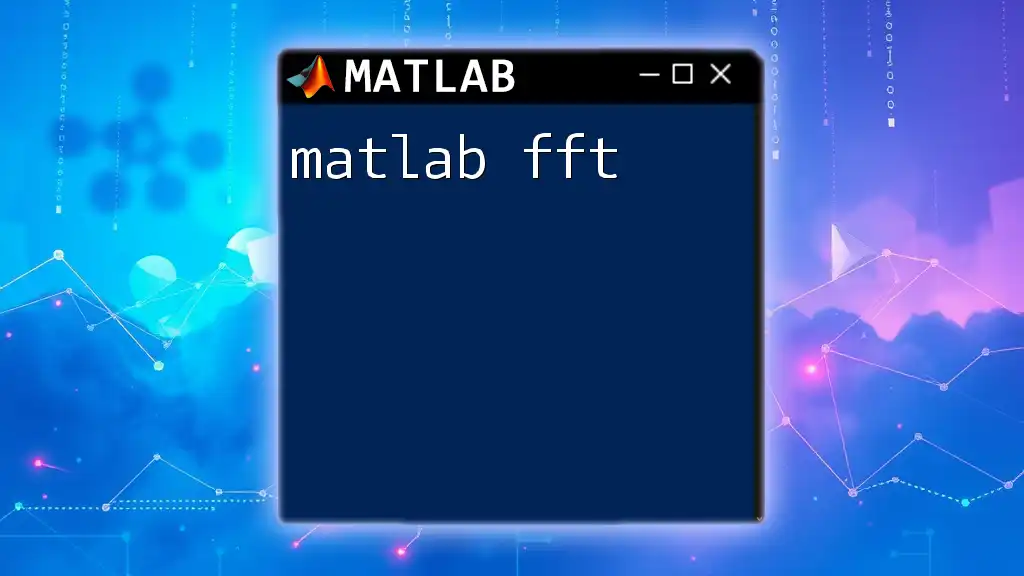
Call to Action
We invite you to share your experiences with `filtfilt` or any tips and tricks you may have uncovered. Join our MATLAB teaching community to learn more and engage with fellow enthusiasts who share a passion for efficient data processing methods!