MATLAB matrix indexing allows you to access and manipulate specific elements or subsets of a matrix using row and column indices.
Here's a simple example of how to index a matrix:
% Create a 3x3 matrix
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Access the element in the second row and third column
element = A(2, 3);
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a rectangular array of numbers or symbols arranged in rows and columns, serving as a fundamental structure in linear algebra. In MATLAB, matrices are not just a type of variable; they are the core of data representation. MATLAB stands for "Matrix Laboratory," which indicates its inherent capability to handle matrix operations efficiently.
Creating Matrices
MATLAB provides various ways to create matrices. You can utilize predefined functions or define them manually.
Using Predefined Functions:
Common functions include:
- `zeros(n)` creates an n x n matrix filled with zeros.
- `ones(n)` generates an n x n matrix filled with ones.
- `eye(n)` produces an n x n identity matrix.
Manual Creation:
You can also create a matrix by specifying its elements directly using square brackets. For example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
In this example, A is a 3x3 matrix.
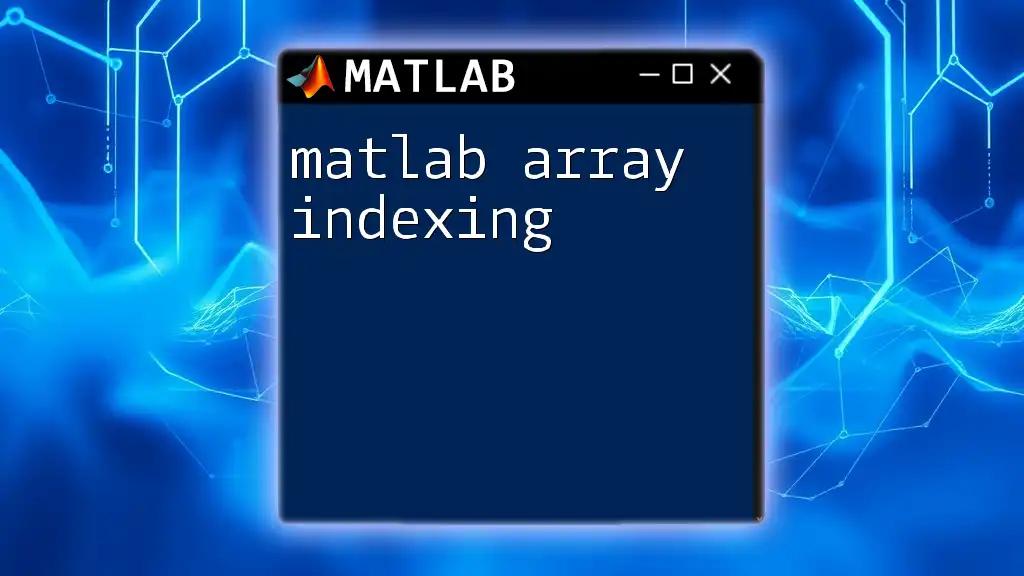
Basics of Indexing
What is Indexing?
Indexing is the means of accessing elements within a matrix. In MATLAB, indexing is not only a primary method for retrieving data but also essential for manipulating data efficiently.
Types of Indexing in MATLAB
MATLAB provides different methods for matrix indexing, making it versatile in handling data.
Logical Indexing
Logical indexing allows you to extract elements from a matrix based on specific conditions. This method is intuitive and powerful for filtering data.
For example, consider a matrix A. To retrieve elements greater than 5, you can use:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
logicalIndex = A > 5; % Returns a logical matrix
B = A(logicalIndex); % Extracts values greater than 5
Here, `logicalIndex` creates a logical array where each element is true if the corresponding element of A is greater than 5, allowing B to store those values.
Linear Indexing
In MATLAB, you can access elements in a multidimensional array using linear indexing, which simplifies access when dealing with matrices.
For instance, using the same matrix A, you can retrieve the 5th element directly:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
value = A(5); % Extracts the element in the 5th position (5)
The linear indexing treats the matrix as a single column vector. Thus, the 5th element corresponds to the value 5 in A.
Subscript Indexing
Subscript indexing allows you to access specific elements using row and column indices. This is particularly useful when you want to manipulate or examine specific sections of a matrix.
For instance, to access an entire row or column:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
row2 = A(2, :); % Retrieves the entire second row
column3 = A(:, 3); % Retrieves the entire third column
In this example, `row2` contains `[4, 5, 6]`, and `column3` contains `[3; 6; 9]`.
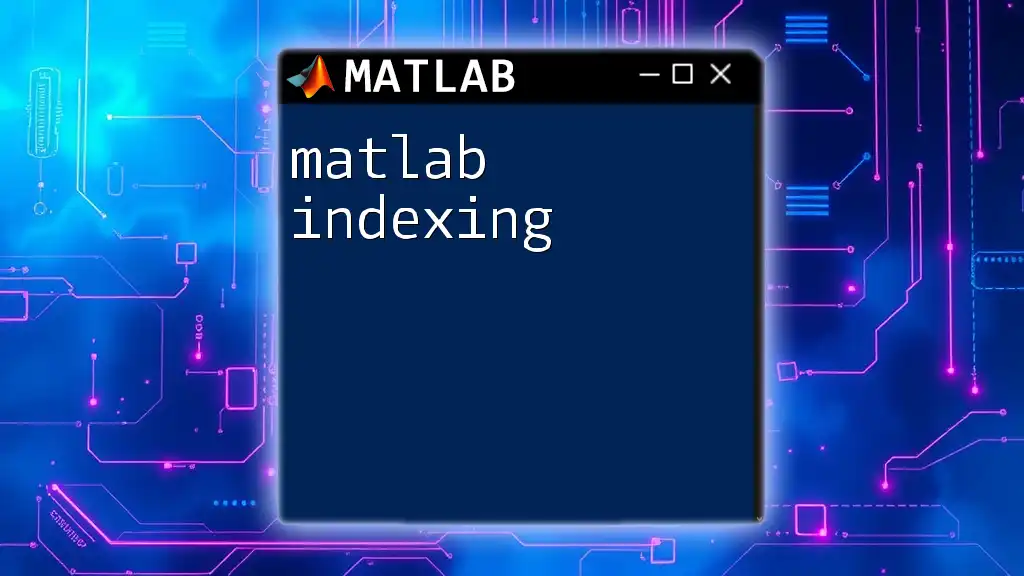
Advanced Matrix Indexing Techniques
Slicing Matrices
Slicing allows you to extract a portion of a matrix, providing flexibility when working with data.
Consider the matrix A; you can slice it to obtain a submatrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
subMatrix = A(1:2, 2:3); % Retrieves a submatrix
The `subMatrix` will contain:
[2, 3;
5, 6]
This ability to slice can be incredibly useful for operations on smaller datasets or when performing analyses on subsets of data.
Modifying Matrix Elements
Indexing also allows you to modify specific elements within a matrix effectively.
For example, to change the first element of matrix A to 10:
A(1, 1) = 10; % Changes the first element to 10
After this operation, matrix A will become:
[10, 2, 3;
4, 5, 6;
7, 8, 9]
Using the `end` Keyword
The `end` keyword is a powerful feature in MATLAB that helps in dynamic indexing, especially when you're unsure about the dimensions of your matrix.
To modify the last element of matrix A, you can use:
A(end, end) = 20; % Modifies the last element of the matrix
This approach is beneficial when working with matrices of varying sizes, ensuring your code remains adaptable.
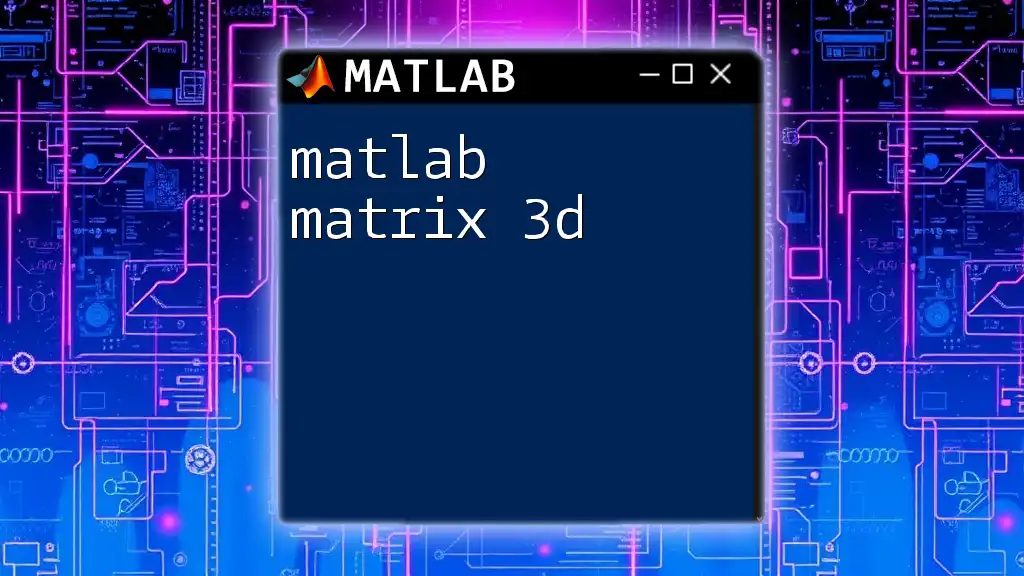
Practical Applications of Matrix Indexing
Data Manipulation
Efficient indexing allows for quick adjustments and checks when manipulating data. For instance, you can replace all negative values in a data set with zero succinctly:
data = [-1, 2, -3; 4, -5, 6; 7, -8, 9];
data(data < 0) = 0; % Replaces all negative values with 0
Extracting Data from Larger Datasets
When dealing with larger datasets, indexing becomes indispensable. By utilizing matrix indexing, you can isolate valuable subsets quickly.
For example, suppose you have a 100x100 matrix of random numbers:
largeMatrix = rand(100, 100); % Create a 100x100 random matrix
extractedData = largeMatrix(1:10, 1:10); % Extracts a 10x10 submatrix
This technique allows you to focus on a relevant portion of the dataset without needing to manipulate the entire matrix.
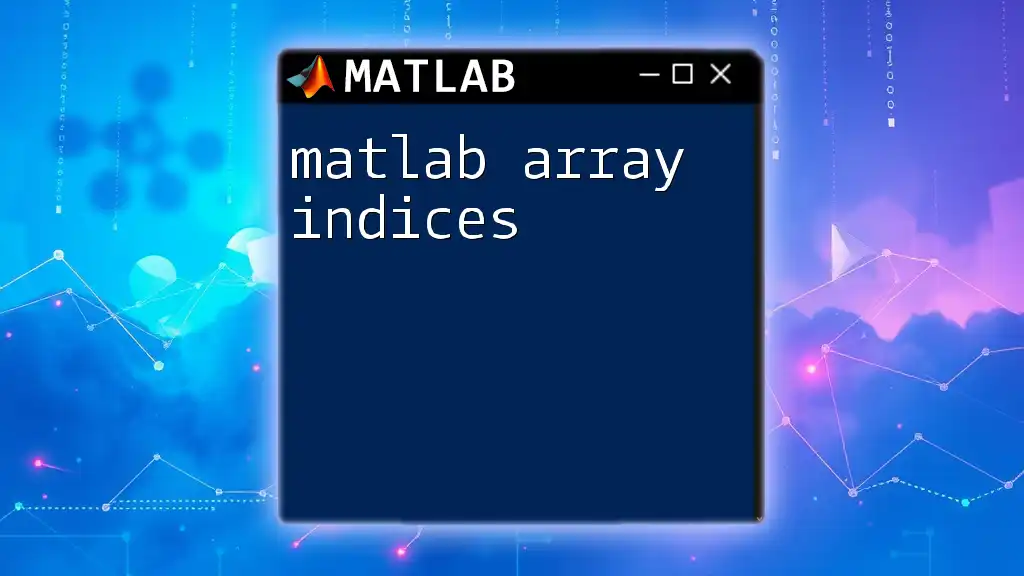
Common Indexing Errors and Troubleshooting
Out-of-Bounds Errors
Out-of-bounds errors often occur when attempting to access an index that exceeds the matrix dimensions. To avoid these errors, always verify the size of your matrix using:
size(A)
Mismatched Dimensions
Mismatched dimensions often lead to errors during matrix operations. To troubleshoot, ensure that the dimensions align with any operation you wish to perform. Use the `size()` function to check the dimensions of matrices before executing operations.
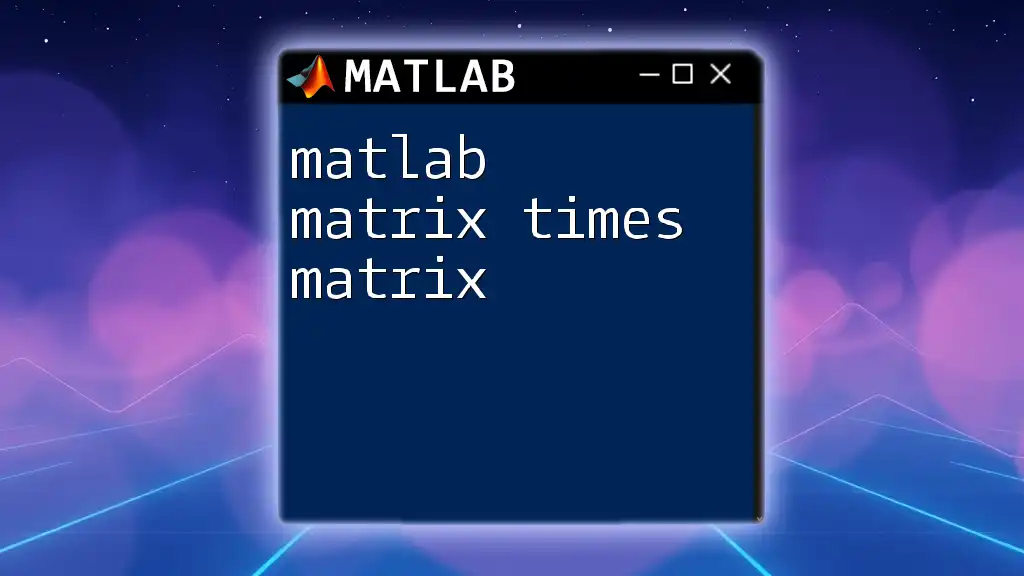
Best Practices for Efficient Indexing
Performance Considerations
Indexing can impact performance, especially with large matrices. Techniques such as preallocating matrices and using logical indexing can enhance efficiency. It’s important to minimize the number of times MATLAB reallocates memory, as this can slow down execution.
Readability and Maintainability
Writing clear and maintainable indexing code is critical. Use meaningful variable names and ensure your indexing logic is straightforward, making it easier for others (or yourself in the future) to understand the code. Include comments where necessary to explain complex lines of indexing.
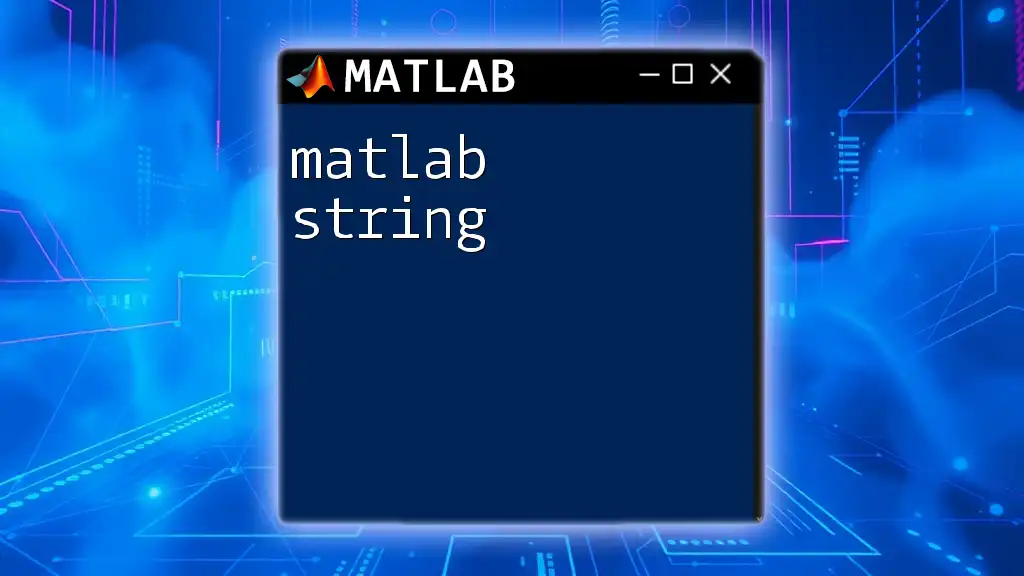
Conclusion
Through this guide, you have gained a comprehensive understanding of MATLAB matrix indexing. From basic techniques to advanced strategies, these concepts equip you with the necessary tools to manipulate and analyze data effectively. Engage with the practical applications, experiment with the examples provided, and enhance your proficiency in utilizing MATLAB.