The `exist` function in MATLAB checks for the existence of a variable, file, or function and returns a corresponding numeric value indicating its presence.
Here’s a simple code snippet demonstrating its usage:
% Check if a variable 'myVar' exists in the workspace
if exist('myVar', 'var')
disp('myVar exists!');
else
disp('myVar does not exist.');
end
Understanding the Purpose of `exist`
The Role of `exist` in MATLAB
The `exist` function in MATLAB plays a fundamental role in programming logic by allowing users to check for the existence of variables, functions, files, and other entities within MATLAB's environment. This capability is crucial for effective error handling, validating assumptions about the data and functions in use, and ensuring that scripts and programs operate smoothly without encountering unexpected issues.
Why Knowing the Existence Matters
Knowing whether a variable or function exists before executing subsequent commands can prevent runtime errors and enhance the robustness of your code. For example, if you are trying to load a dataset or utilize a function that might not be available, using `exist` can provide a safeguard against crashes or exceptions during execution. Such proactive checks facilitate cleaner code and a more user-friendly experience when running scripts.
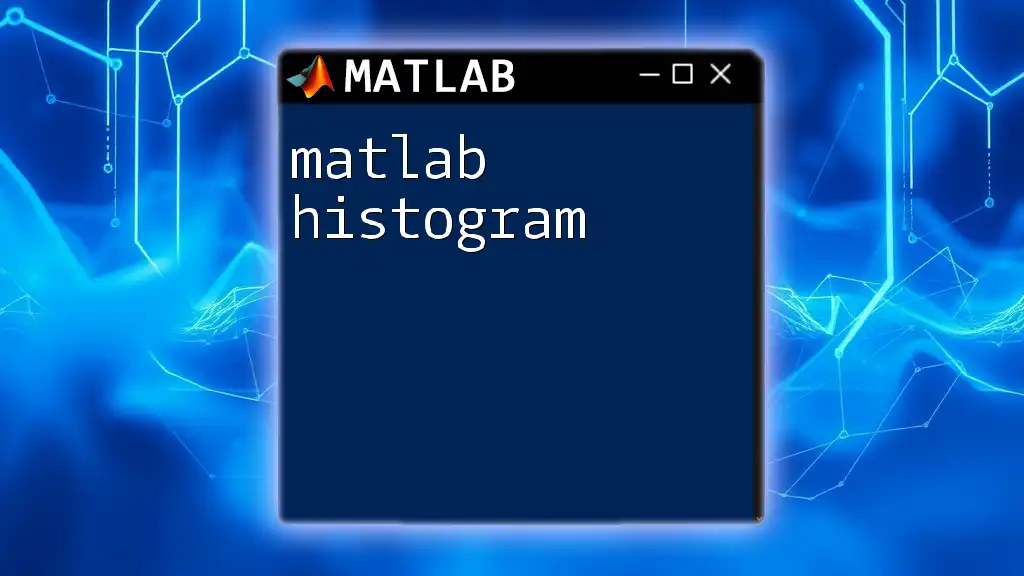
Syntax of the `exist` Function
Basic Syntax Overview
The syntax for the `exist` function is straightforward:
exists = exist(name, type)
This function takes two key arguments: `name`, which is the entity you want to check, and `type`, which specifies what kind of entity MATLAB should look for.
Parameter Descriptions
- name: A string representing the name of the variable, function, or file you wish to check for existence.
- type: A string that defines the type of entity to check. Common values include:
- `'var'` for variables in the workspace.
- `'func'` or `'file'` for functions.
Output Values
The `exist` function returns numerical output values that indicate the status of the requested entity. Here’s a summary of the return values:
- 0: Not found
- 1: Variable in the workspace
- 2: Function or M-file
- 3: P-file (protected file)
- 4: MEX file (compiled C/C++ programs)
- 5: Simulink model
- 6: Class name
Understanding these return values is critical as they dictate the flow of decisions in your code.
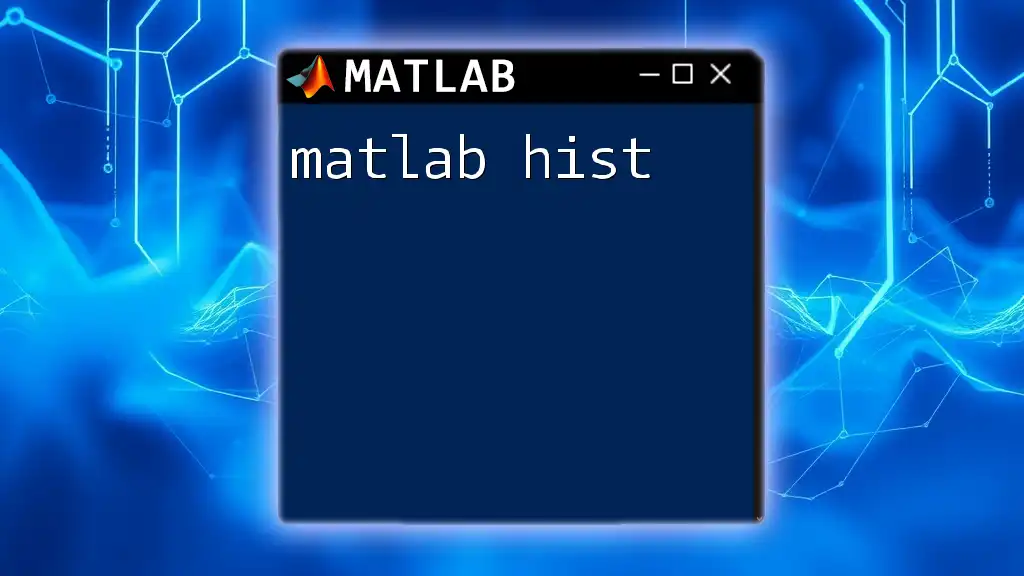
Examples of Using `exist`
Checking Variable Existence
One of the most common uses of `exist` is to check if a variable is defined in the workspace. For instance, consider the following code snippet:
if exist('myVariable', 'var')
disp('myVariable exists in the workspace.');
else
disp('myVariable does not exist.');
end
In this example, if `myVariable` is defined, the message confirming its existence prints; otherwise, a message indicating absence appears.
Checking Function Existence
Function checks can be equally essential, ensuring that you are calling a defined function before invoking it. Use `exist` as follows:
if exist('myFunction', 'file') == 2
disp('myFunction exists as a MATLAB function.');
else
disp('myFunction does not exist.');
end
In this scenario, checking whether `myFunction` exists prevents errors during execution, contributing to smoother user experiences.
Checking File Existence
When working with files, ensuring their presence before performing operations like reading data is vital. You can verify a file's existence using:
if exist('datafile.csv', 'file')
disp('The file exists and can be read.');
else
disp('The file does not exist.');
end
This check is especially important in data analysis workflows, as it avoids issues related to missing data files.
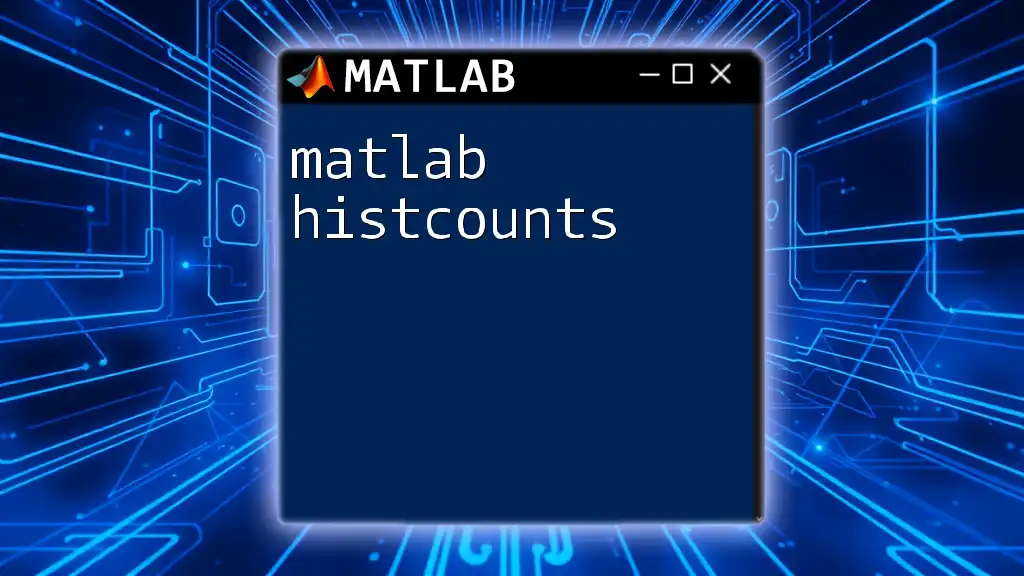
Common Use Cases for `exist`
Validating Inputs in Functions
Using `exist` to validate inputs received by functions can enhance robustness. For example, when creating a function that processes data files, it’s prudent to check that the file exists first. Consider this snippet:
function processFile(filename)
if exist(filename, 'file') ~= 2
error('The specified file does not exist: %s', filename);
end
% Proceed with processing
end
Such validation prevents actions on nonexistent files, leading to better error management.
Managing External Resource Dependencies
When your scripts rely on external files or functions, ensure that all dependencies are intact before execution. Using `exist` helps ensure that any resource requirements are met. For instance, checking for multiple dependencies before loading data ensures your script runs without issues:
requiredFiles = {'datafile.csv', 'config.mat'};
for i = 1:length(requiredFiles)
if exist(requiredFiles{i}, 'file') ~= 2
error('Missing required file: %s', requiredFiles{i});
end
end
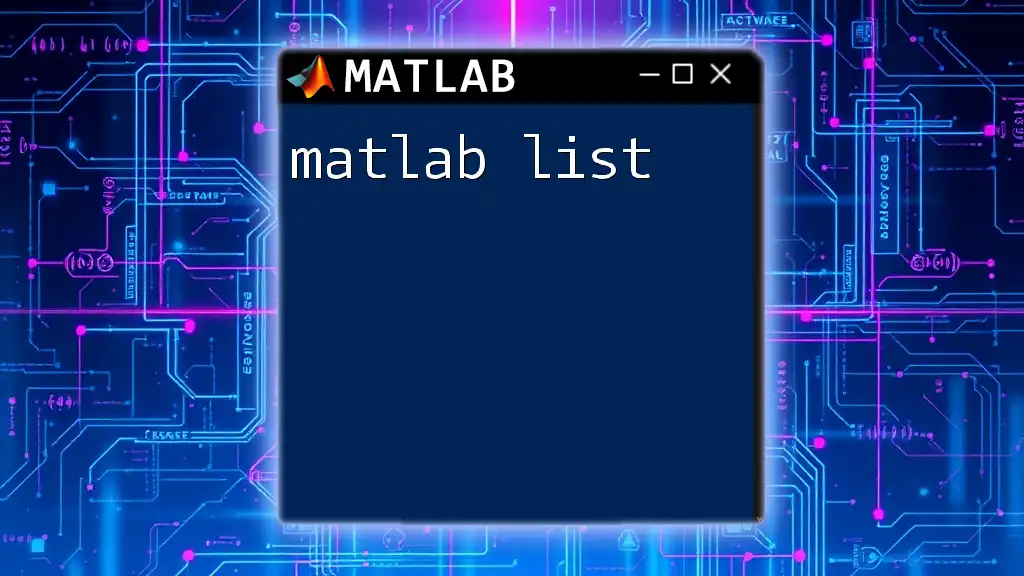
Error Handling with `exist`
Avoiding Fatal Errors
Incorporating `exist` in your code can help you handle potential errors gracefully. Consider this scenario where loading a variable might fail due to its absence:
try
data = load('data.mat');
catch
if exist('data.mat', 'file') == 0
error('data.mat file is missing.');
else
rethrow(lasterror);
end
end
By checking the file’s existence before attempting to load it, you can differentiate between various error scenarios and provide meaningful feedback to users.
Using `exist` in Conditional Logic
Employing `exist` in conditional statements allows for dynamic control flows based on current definitions. For example, you can load a default dataset if a specific variable doesn't exist:
if exist('myData', 'var')
data = myData;
else
data = load('defaultData.mat');
end
Here, the logic provides a fallback mechanism that enhances the reliability of the program.
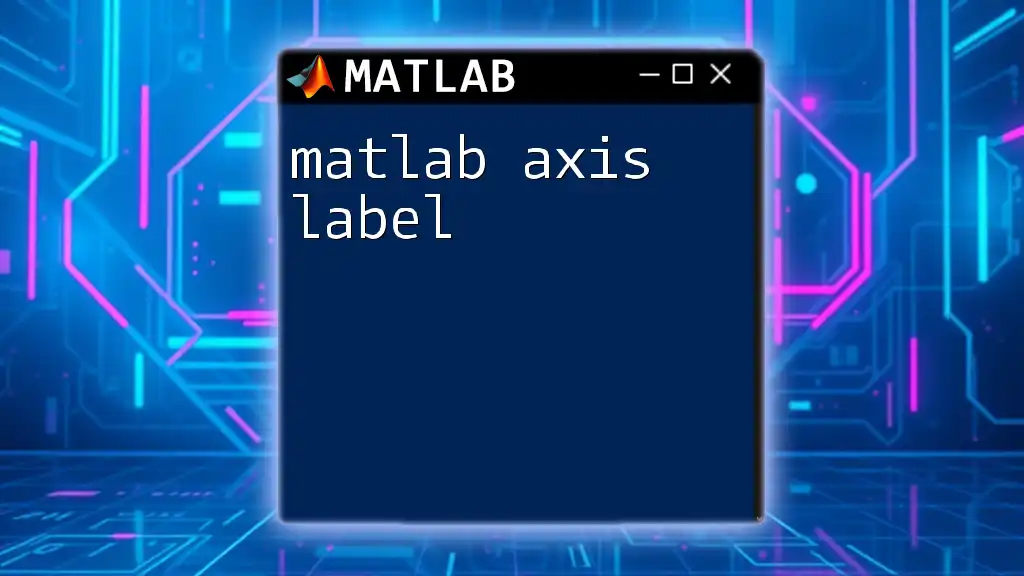
Performance Considerations
Short-Circuiting Checks
When utilizing `exist`, it's essential to be mindful of performance, especially in loops or large scripts. Efficiently checking for existence can lead to shorter execution times. Rather than checking multiple times within loops, consolidate your checks whenever possible.
Alternatives to `exist`
In some cases, you might find alternatives like `isfile()` or `isfunction()` more appropriate for your needs. These functions can be more readable and convey intent clearly. However, in circumstances requiring extensive entity checks, `exist` remains a powerful tool.
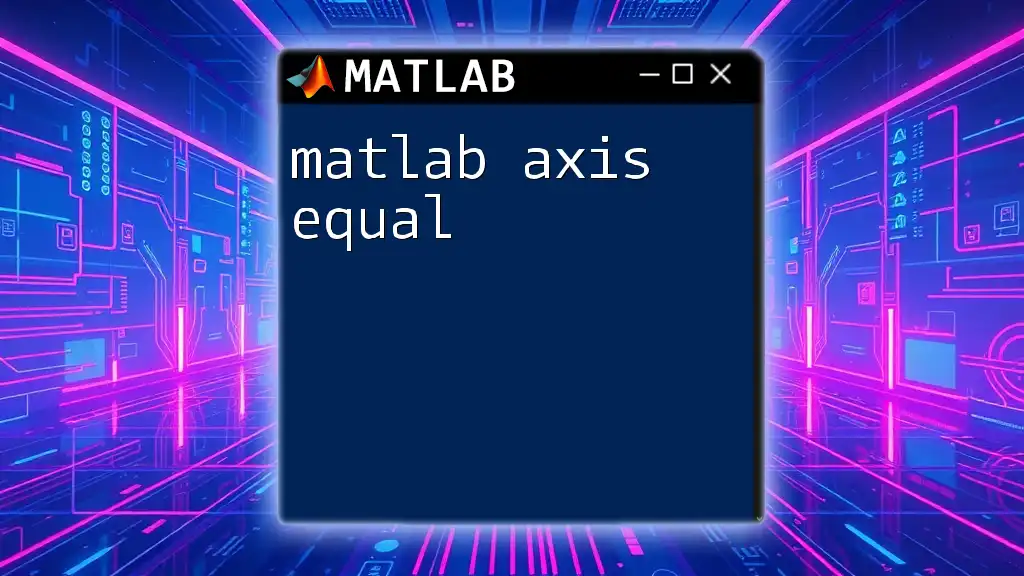
Practical Tips for Maximizing Use of `exist`
Based on Use Cases
When utilizing `exist`, consider your specific use cases carefully. If you often check for variables during data analyses, establish a pattern in your error handling to maintain consistency throughout your scripts.
Common Pitfalls
Common mistakes with `exist` include misunderstanding return values or misrepresenting the type parameter. Always keep the defined types in mind and refer to the documentation if there is any confusion. It's easy to assume a function or variable exists due to naming similarity, so always validate using `exist`.
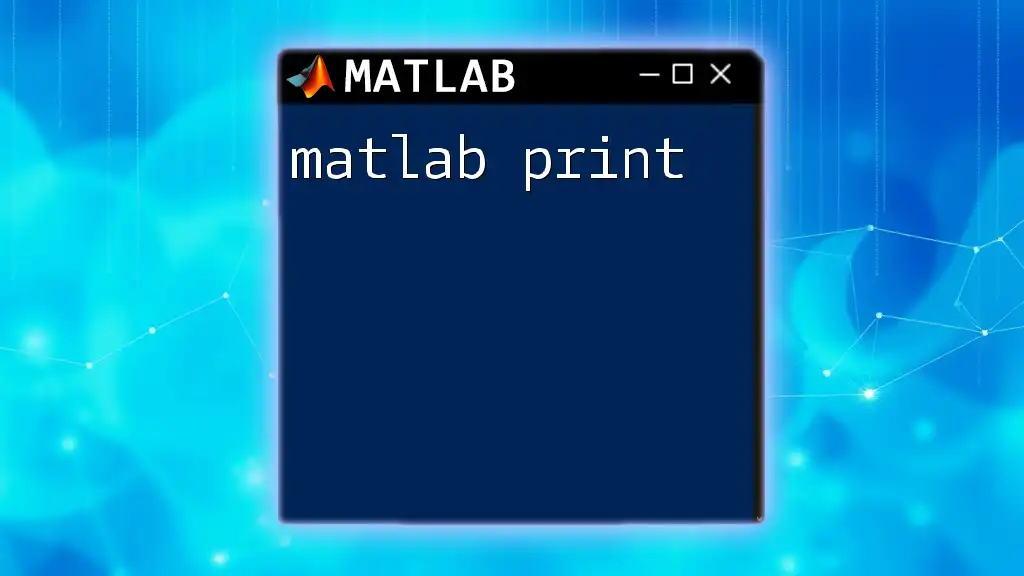
Conclusion
In summary, the MATLAB `exist` function is a powerful ally in ensuring that your scripts and functions operate smoothly without running into unexpected runtime errors. Utilizing `exist` to check for the existence of variables, files, and functions improves code reliability and enhances user experience. Embrace the strategies discussed and experiment with `exist` in your MATLAB projects to maximize your productivity and reduce the likelihood of errors.