To delete a file in MATLAB, you can use the `delete` command followed by the filename as a string.
delete('filename.txt')
Understanding the Importance of File Management
The Need for Efficient File Handling
Efficient file management is crucial in programming, particularly when working with MATLAB. Keeping your workspace organized not only enhances productivity but also reduces the chance of errors. File deletion plays a significant role in file management, especially in preventing memory overflow and maintaining a clean directory structure. As projects evolve, old or temporary files can accumulate, hindering performance and making it harder to locate relevant data.
Use Cases for Deleting Files
There are numerous scenarios where file deletion becomes necessary. For instance, temporary files generated during a computation can take up unnecessary space, ultimately affecting performance. Similarly, project updates may require you to delete outdated files to ensure that only the most relevant data is available for use. Implementing efficient file deletion practices in your programming routine can significantly improve your workflow.
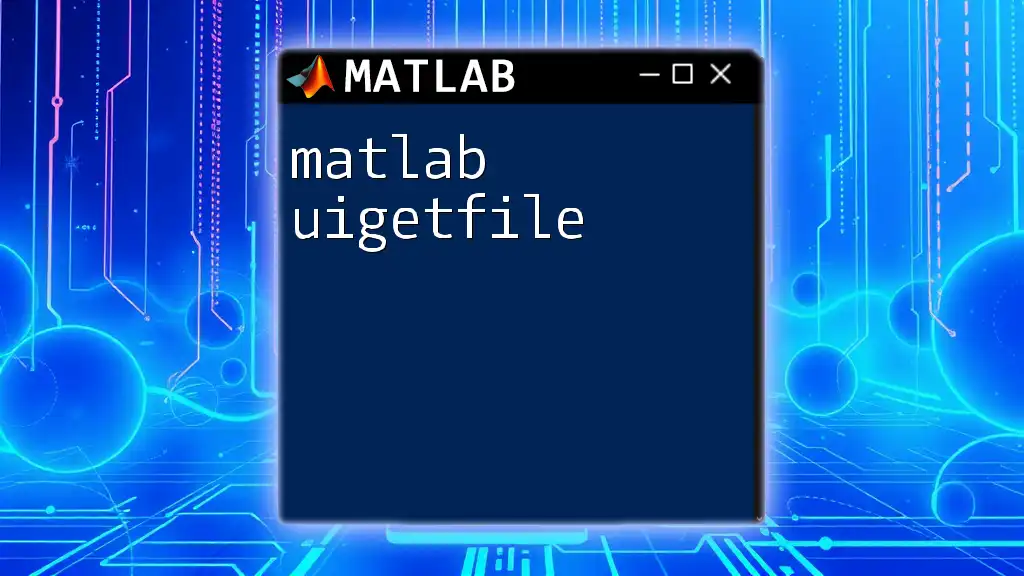
Getting Started with `delete` Command
What is the `delete` Function?
The `delete` function is a core command in MATLAB, enabling users to remove files from their directories. The basic syntax for the command is as follows:
delete(filename)
This command allows you to specify the file you want to delete by providing its name. Understanding how to use this command effectively is crucial for maintaining an organized workspace.
Basic Usage
To delete a single file with the `delete` command, you simply call it with the filename as a string argument. For instance, to remove a file named `example.txt`, you would use the following command:
delete('example.txt');
When this command is executed, the specified file will be permanently deleted from your MATLAB workspace. It’s essential to ensure that you have the correct filename and path, as deleted files cannot be recovered through MATLAB once the command is executed.
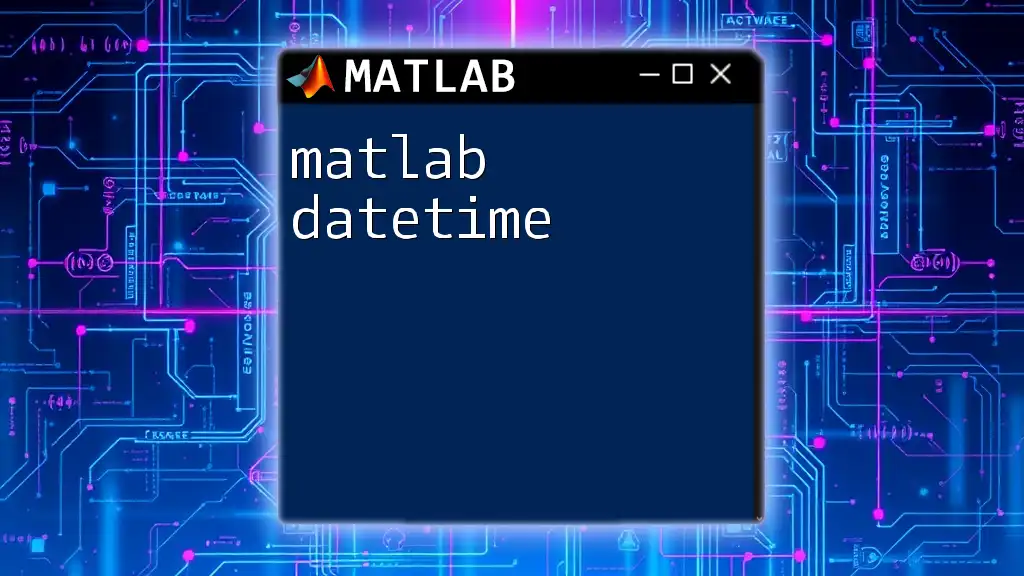
Advanced File Deletion Techniques
Deleting Multiple Files
The `delete` command also supports the use of wildcards, which can be incredibly useful when needing to delete multiple files at once. For example, if you wish to delete all files with the `.txt` extension in a directory, you could use:
delete('*.txt');
This command instructs MATLAB to search for and delete every file that ends with `.txt`. However, using wildcards can be powerful but potentially dangerous; it’s important to double-check your directory and selection criteria to avoid unintentional data loss.
Deleting Files Conditionally
To enhance safety, you can implement conditional statements that check whether a file exists before attempting to delete it. This provides a simple safeguard against errors. For example:
if exist('example.txt', 'file')
delete('example.txt');
end
In this example, the function `exist` checks if `example.txt` is present in the directory. This method ensures that your script won’t try to delete a non-existent file, which would otherwise lead to an error message.
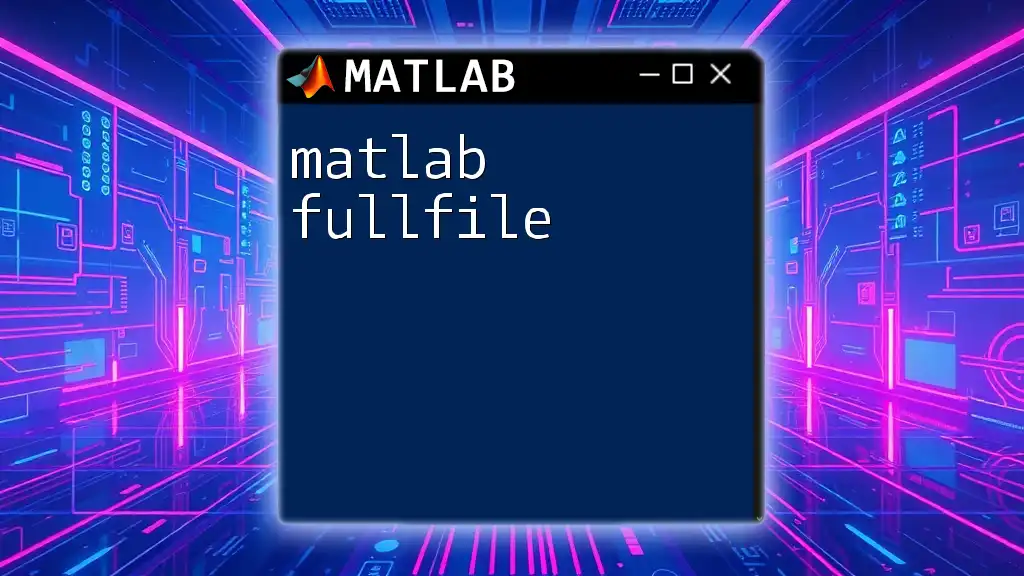
Handling Errors During Deletion
Common Errors
While using the `delete` command, it’s crucial to be aware of potential errors that may arise. Common issues include "File not found" errors or permission-related problems when trying to delete protected files. Understanding these errors can save valuable time and frustration.
Using Try-Catch for Robustness
Implementing error handling is essential for creating robust MATLAB scripts. By using the `try-catch` construct, you can gracefully manage errors without crashing your program. For instance:
try
delete('nonexistentfile.txt');
catch ME
disp('Error:');
disp(ME.message);
end
In this example, if MATLAB attempts to delete a file that doesn’t exist, it will catch the error and display a friendly message instead of stopping the script abruptly. This approach allows you to maintain control over your workflow while ensuring that your code is resilient.
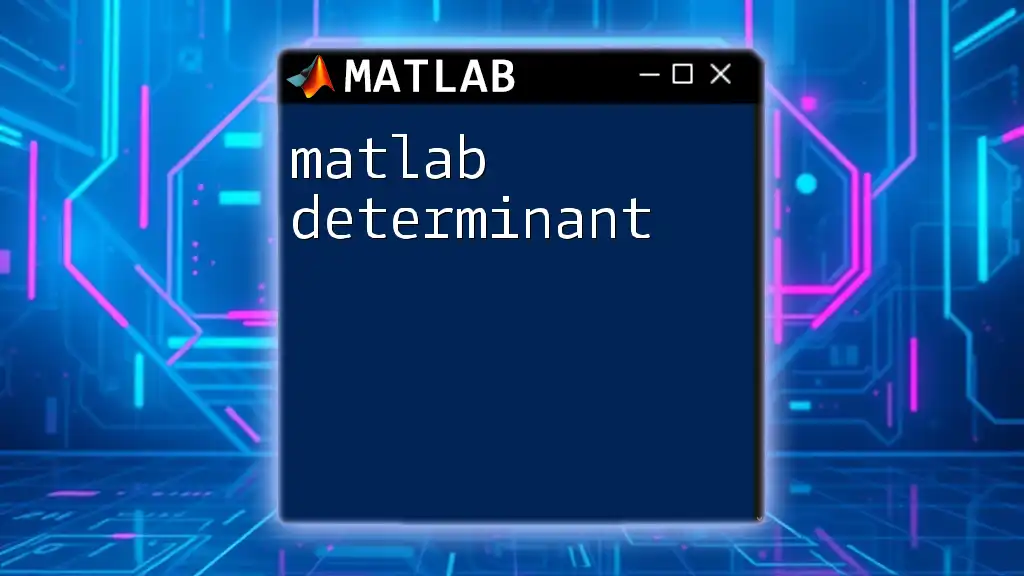
Best Practices for File Deletion
Avoid Accidental Deletion
Preventing unintentional deletions is vital to preserving your important data. To minimize risks, consider implementing confirmation steps before file deletions, such as prompting the user for confirmation or maintaining a backup of important files. Taking these precautions ensures that you don’t lose significant work due to simple mistakes.
Logging Deleted Files
Consider logging deleted files to track changes and prevent unintentional loss of essential data. You can create a log after performing deletions for future reference:
fileID = fopen('deletion_log.txt', 'a');
fprintf(fileID, 'Deleted: %s at %s\n', 'example.txt', datestr(now));
fclose(fileID);
In this code snippet, a log is created or appended to a file named `deletion_log.txt`, documenting which files were deleted and when. This logging strategy makes it easier to keep track of your changes and improves accountability in file management.
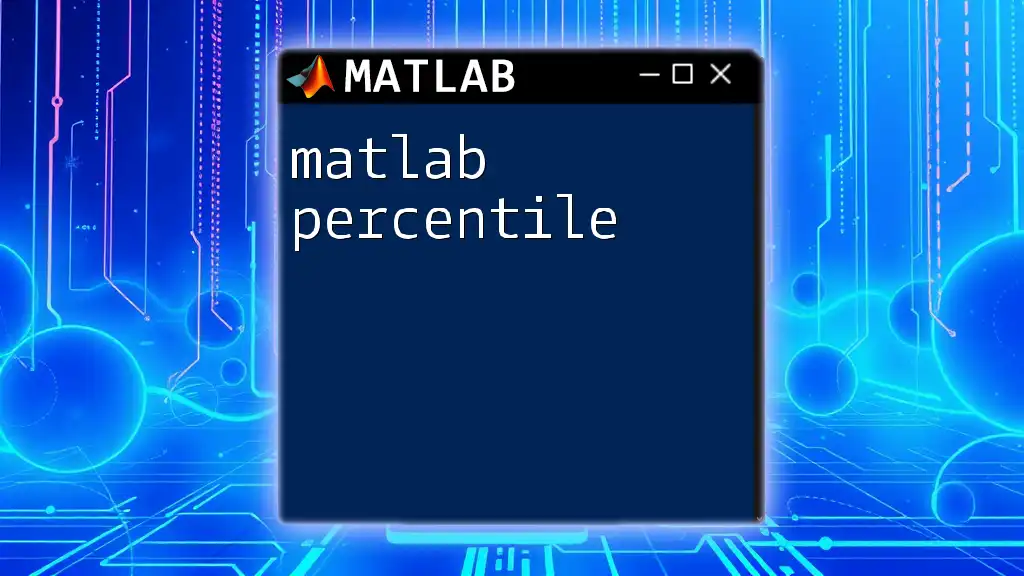
Conclusion
In this guide, we have explored the `delete` command in MATLAB, focusing on its usage, error handling, and best practices for file management. By understanding how to effectively use the `matlab delete file` command, you can maintain a well-organized workspace, safeguard important data, and enhance your programming efficiency. Implementing these strategies will not only streamline your workflow but will also pave the way for more sophisticated data management techniques in your projects.
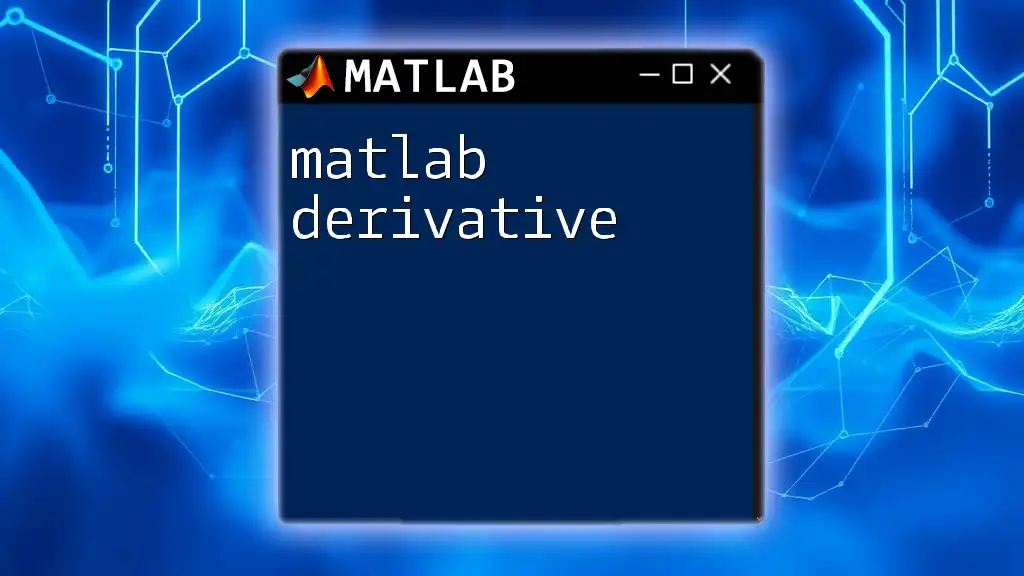
Further Resources
For additional learning, consider exploring more in-depth MATLAB documentation on file management and command references. Engaging with recommended tutorials and guides can bolster your skills in MATLAB, empowering you to become even more proficient in your programming endeavors.