MATLAB code allows users to efficiently perform mathematical computations, visualizations, and data analysis through its high-level programming language.
Here's a simple example of a MATLAB script that calculates the sum of two numbers:
% Define two numbers
a = 5;
b = 10;
% Calculate their sum
sum = a + b;
% Display the result
disp(['The sum of ', num2str(a), ' and ', num2str(b), ' is: ', num2str(sum)]);
Understanding the MATLAB Environment
MATLAB offers a user-friendly interface that is designed to facilitate efficient programming and data analysis. Familiarizing yourself with the key components of the MATLAB interface is essential for effective code development.
The primary components you will encounter include the Command Window, where you execute commands and view outputs, the Workspace, which displays your current variables, and the Editor, used for writing and editing scripts. Each of these elements plays a crucial role in the development process.
Basic Commands and Syntax
Understanding the syntax of MATLAB is foundational to writing effective MATLAB code. Syntax errors can be a common pitfall for beginners, so recognizing the proper structure of commands is vital. For instance:
To display output in MATLAB, you can use the `disp` function:
disp('Hello, World!')
To create and assign variables, simply declare them like so:
x = 10;
y = 5;
Mastering these basic commands lays the groundwork for more advanced programming techniques.
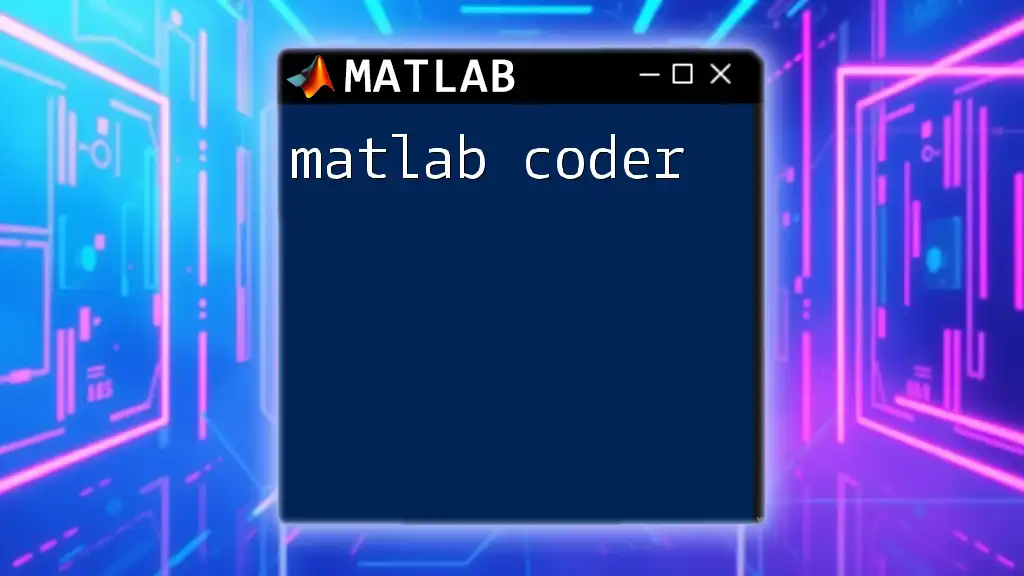
Writing and Executing MATLAB Code
Creating and Saving Scripts
Creating scripts in MATLAB allows you to execute a series of commands sequentially. To create a new script, open the Editor from the Home tab and simply start typing your code. Once you've crafted your script, save it in an appropriate directory using the `.m` file extension. To run your script, type its name in the Command Window without the extension.
Basic Programming Concepts in MATLAB
Variables and Data Types
MATLAB is a dynamically typed language, meaning you don't have to declare the type of the variable; MATLAB infers it. Common data types include numeric, character, and logical arrays.
Example:
a = 10; % Numeric variable
b = 'Hello'; % Character array
isTrue = true; % Logical variable
Understanding these data types is essential since they can impact how you manipulate data and perform calculations.
Control Structures
Control structures enable you to implement decision-making and repeat processes within your code.
If-Else Statements
An `if-else` statement allows you to execute different portions of code based on conditions:
if a > b
disp('A is greater');
else
disp('B is greater');
end
For Loops
`For` loops are useful for repetitive tasks, such as iterating over arrays:
for i = 1:5
disp(['Iteration: ', num2str(i)]);
end
While Loops
`While` loops continue executing as long as a condition remains true. They are beneficial for scenarios where the number of iterations is not fixed:
count = 0;
while count < 5
disp('Count is less than 5');
count = count + 1;
end
Each control structure has its purpose, and learning how to utilize them effectively expands your programming toolkit.
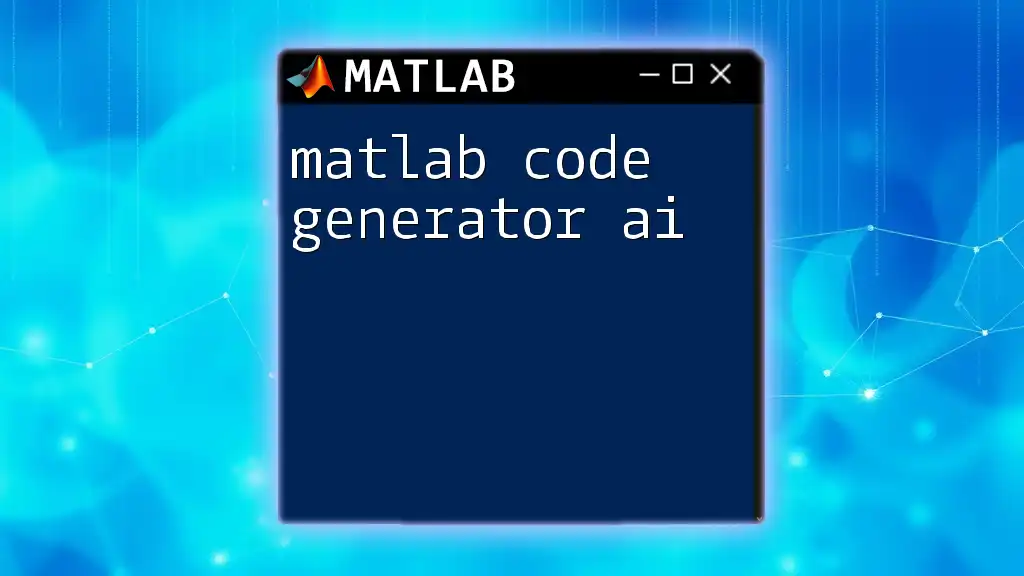
Essential MATLAB Commands
Array and Matrix Manipulation
One of MATLAB's strongest advantages is its powerful capabilities for handling arrays and matrices. MATLAB is short for Matrix Laboratory, and it shines in this domain. To create arrays and matrices, you can simply use square brackets:
A = [1, 2; 3, 4]; % A 2x2 matrix
B = [5, 6; 7, 8]; % Another 2x2 matrix
C = A + B; % Matrix addition
More advanced operations, such as matrix multiplication and element-wise operations, are also straightforward, thanks to MATLAB's intuitive syntax.
Plotting in MATLAB
Visualizing data is crucial in any analysis, and MATLAB makes this easy. Simple plotting can be executed with the `plot` function. For example, to plot a sine wave:
x = 0:0.1:2*pi; % Create an array from 0 to 2*pi
y = sin(x); % Calculate the sine of each element in x
plot(x, y); % Create the plot
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
This functionality allows users to create elegant visual representations of data, an essential skill for any MATLAB programmer.
Importing and Exporting Data
Working with external data is often necessary. MATLAB offers straightforward functions for reading and writing files. For instance, to read a CSV file:
data = readtable('data.csv'); % Import data from a CSV file
You can also export your results to a CSV file with:
writetable(data, 'output.csv'); % Write data to a new CSV file
These capabilities ensure that you can integrate MATLAB with other programs and databases seamlessly.
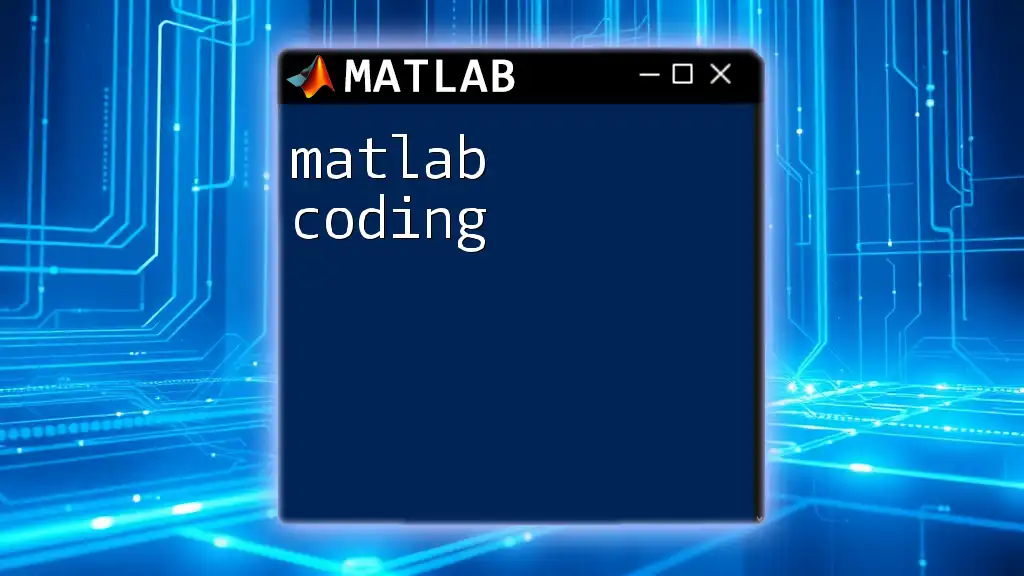
Debugging and Error Handling
As you develop your code, you'll likely encounter errors—both syntax and logical. Recognizing common errors, such as indexing errors or improper matrix dimensions, is the first step in debugging.
Debugging Techniques
MATLAB provides robust debugging tools. You can set breakpoints in your code by clicking on the left margin of the Editor. When you run the code, execution will pause at the breakpoint, allowing you to inspect variables and step through line by line.
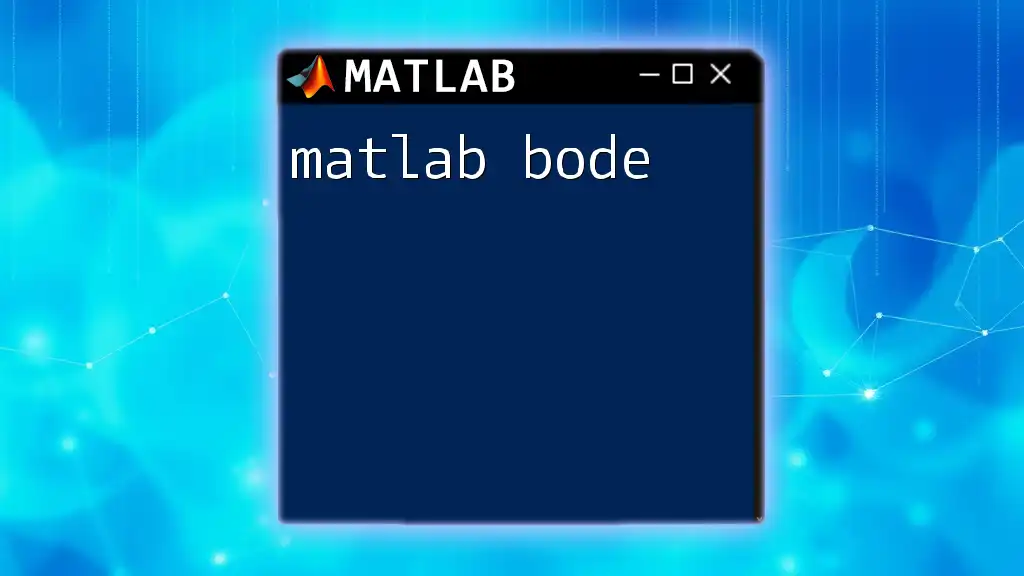
Advanced MATLAB Code Techniques
Functions and Scripts
One of the hallmarks of good programming is code reuse. Defining functions allows you to encapsulate logic, making it easy to call upon frequently used operations. Here’s a simple function that squares a number:
function output = square(num)
output = num^2;
end
You can then call this function from your script or command window simply by passing a number:
result = square(3); % result will be 9
Working with Toolboxes
MATLAB is also renowned for its extensive collection of toolboxes, each offering specialized functions for various applications. Whether you're diving into statistics, image processing, or neural networks, there’s likely a toolbox to meet your needs.
Optimizing MATLAB Code
Writing efficient code often requires understanding how MATLAB operates under the hood. Vectorization—instead of loops—is a common technique to increase code performance.
Example of vectorization:
A = 1:1000; % Creating a vector from 1 to 1000
B = A .* 2; % Element-wise multiplication
Using vectorized operations typically results in more concise and faster-running code, significantly enhancing performance.
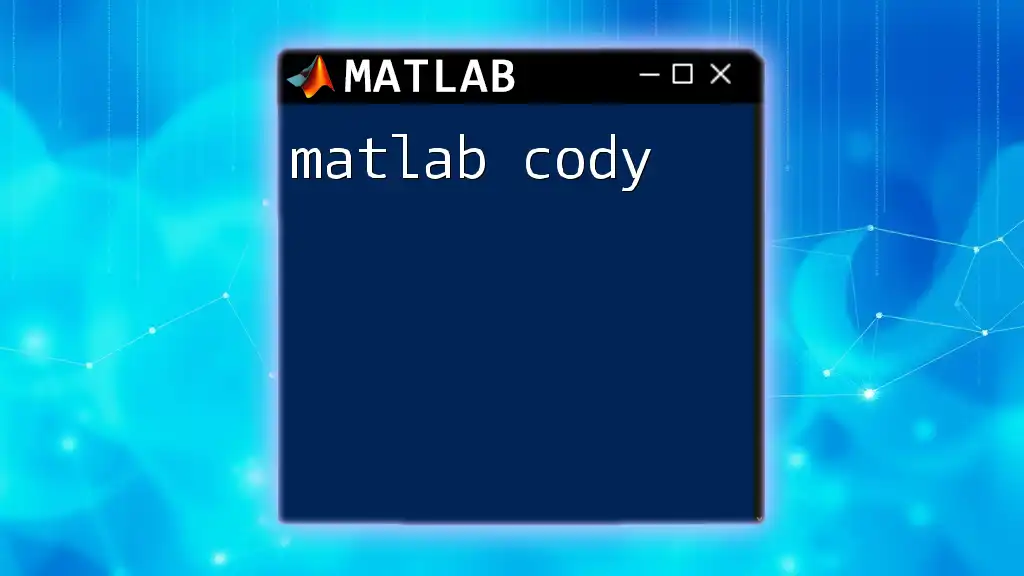
Conclusion
In this comprehensive guide to MATLAB code, we explored the fundamental concepts that every MATLAB user should know, from basic commands to advanced techniques. We covered the importance of the MATLAB environment, how to write and execute scripts, the power of data manipulation, effective debugging practices, and even optimizing your code.
By experimenting with the examples provided and exploring further, you'll sharpen your programming skills and harness the full potential of MATLAB for your work. Remember, practice is key to mastering MATLAB!
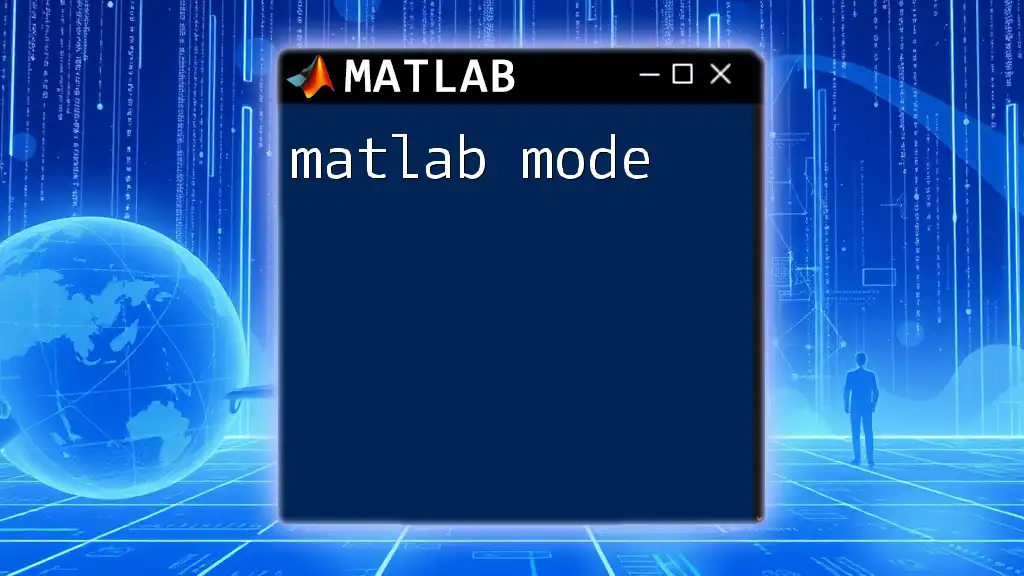
Frequently Asked Questions (FAQs)
As you navigate your journey of learning MATLAB, you may have questions. Here are some common inquiries with concise answers to guide you on your path:
-
What is MATLAB primarily used for?
- MATLAB is widely used for mathematical modeling, data analysis, algorithm development, and visualization.
-
How can I gain more experience with MATLAB?
- Consider enrolling in online courses, reading textbooks, and practicing with example projects to gain hands-on experience.
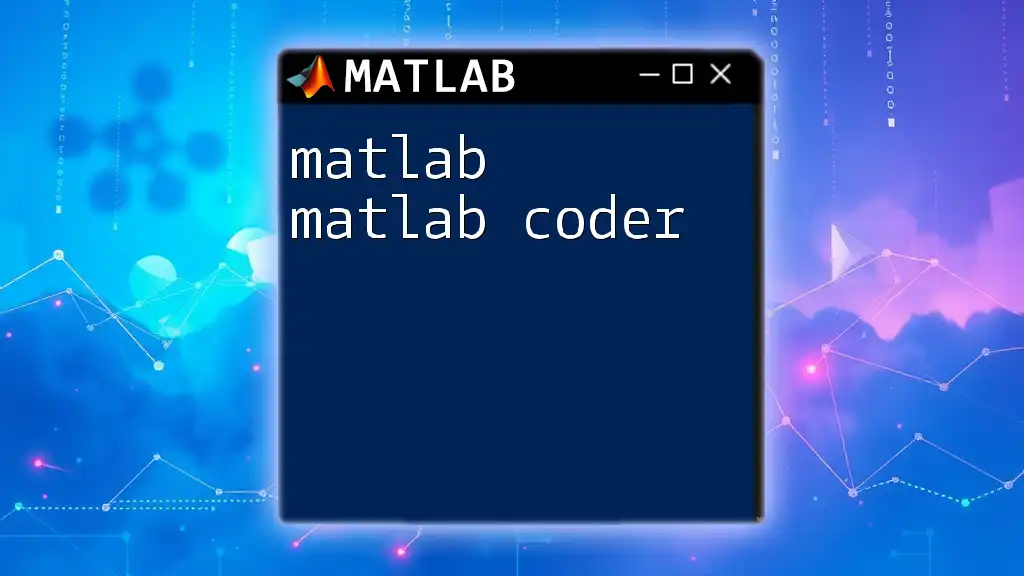
Call to Action
Are you eager to deepen your understanding of MATLAB code? Join our courses today for practical learning experiences and mentorship tailored to help you become proficient in MATLAB programming.