MATLAB Coder is a tool that allows you to convert MATLAB code into C/C++ code for high-performance computing and integration into other software applications.
Here’s a simple code snippet showcasing how to use MATLAB Coder to generate C code from a MATLAB function:
% Example MATLAB function for MATLAB Coder
function y = squareNumber(x)
y = x^2;
end
% To generate C code from this function, use:
% codegen squareNumber -o squareNumber_C -args {0}
What is MATLAB Coder?
MATLAB Coder is a powerful tool designed for efficiently converting MATLAB code into C and C++ code. This allows users to deploy their algorithms into devices and applications that require high-performance computing and real-time processing. One of the key advantages of MATLAB Coder is its ability to streamline the transition from a MATLAB environment to external applications, ensuring that engineers and developers can leverage the strengths of both MATLAB and C/C++ languages.
When to Use MATLAB Coder
MATLAB Coder shines in scenarios requiring real-time processing, such as embedded systems and high-performance applications. Examples include:
- Embedded Systems: Systems embedded in automobiles or other machinery where real-time reactions are crucial.
- Signal Processing: Algorithms that require fast computations, like digital communication systems.
- Control Systems: Systems designed to automate processes based on real-time feedback.
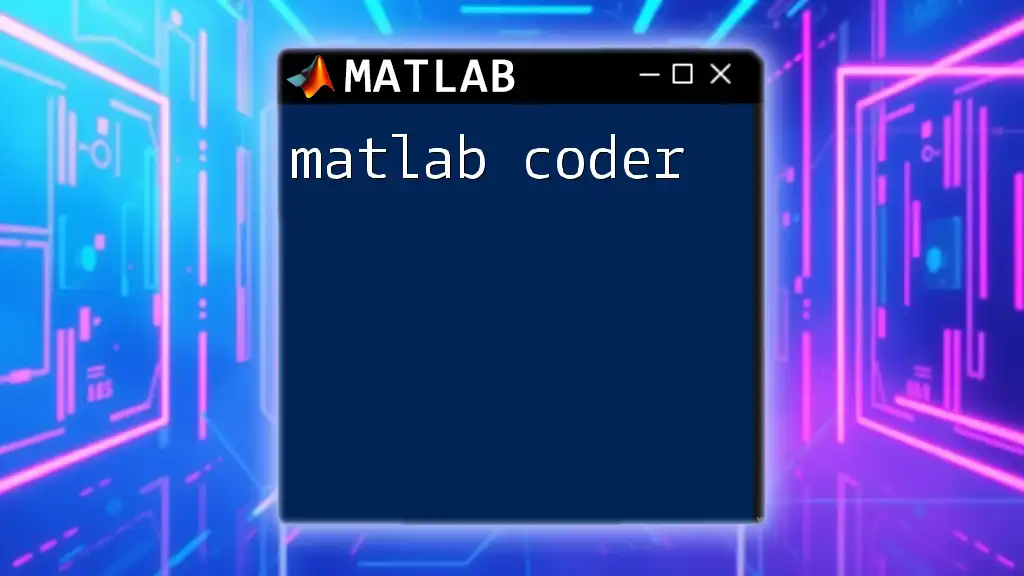
Getting Started with MATLAB Coder
Installation and Setup
To begin your journey with MATLAB Coder, ensure you have the appropriate version of MATLAB installed. Follow these steps for installation:
- Check Compatibility: Ensure both your MATLAB version and operating system support MATLAB Coder.
- Install from MATLAB Add-Ons: Within MATLAB, navigate to the Add-Ons menu and select "Get Add-Ons." Search for MATLAB Coder and follow the prompts to install it.
Basic Workflow
Once installed, the typical workflow to convert MATLAB code involves:
- Writing MATLAB code that adheres to the coding standards suitable for code generation.
- Configuring the code generation settings which can be done via the Code Generation pane.
- Generating the code and validating its execution.
This streamlined process encompasses all necessary configurations and optimizations for effective code generation.
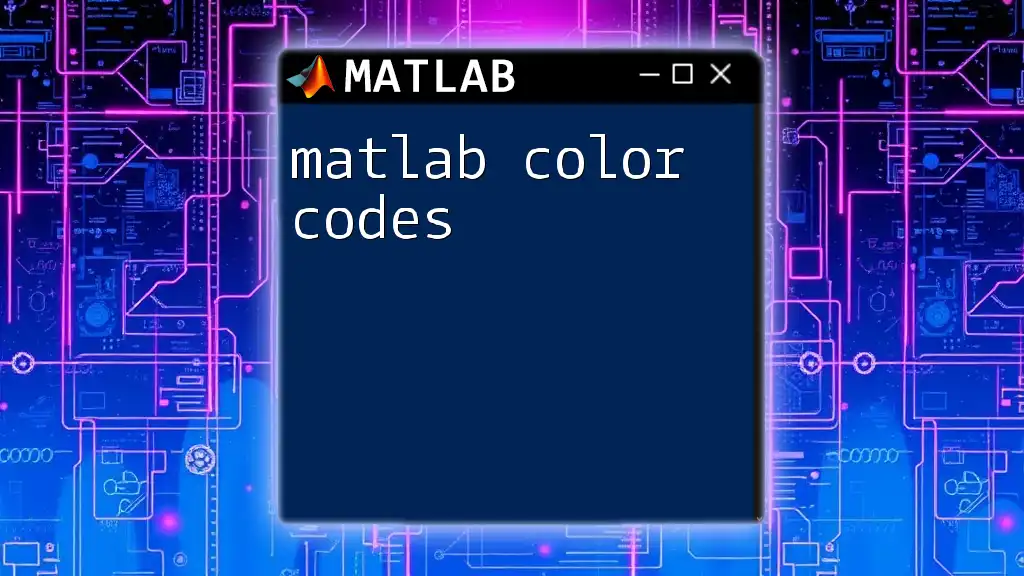
Key Features of MATLAB Coder
Automatic Code Generation
One of the standout features of MATLAB Coder is its ability to generate optimized C/C++ code automatically. When converting a simple piece of MATLAB code, such as:
function y = squareValue(x)
y = x^2;
end
the generated C code would be significantly more efficient, illustrating the compiler's optimization capabilities. Users often notice that the generated codes are optimized for performance, often resulting in faster execution times.
Customization of Generated Code
MATLAB Coder allows users to customize the settings for code generation. Key configuration options include:
- Target Environment: Options for the specific environment where the code will be executed (e.g., desktop application, embedded device).
- Output Type: Flexibility to generate static libraries, dynamic shared libraries, or even executables.
Example of Customization
To specify output type in the command line:
codegen squareValue -o squareValueLib -g
This command generates a shared library for the `squareValue` function.
Integration with Simulink
MATLAB Coder seamlessly integrates with Simulink, enabling users to convert Simulink models into C/C++ code various applications. This integration allows for rapid development of algorithms in a visual environment, followed by code generation for implementation.
Example
Given a Simulink model that computes the square of an input signal, the model can be compiled directly into C code using MATLAB Coder, ensuring that the fidelity of the model is maintained in the generated code.
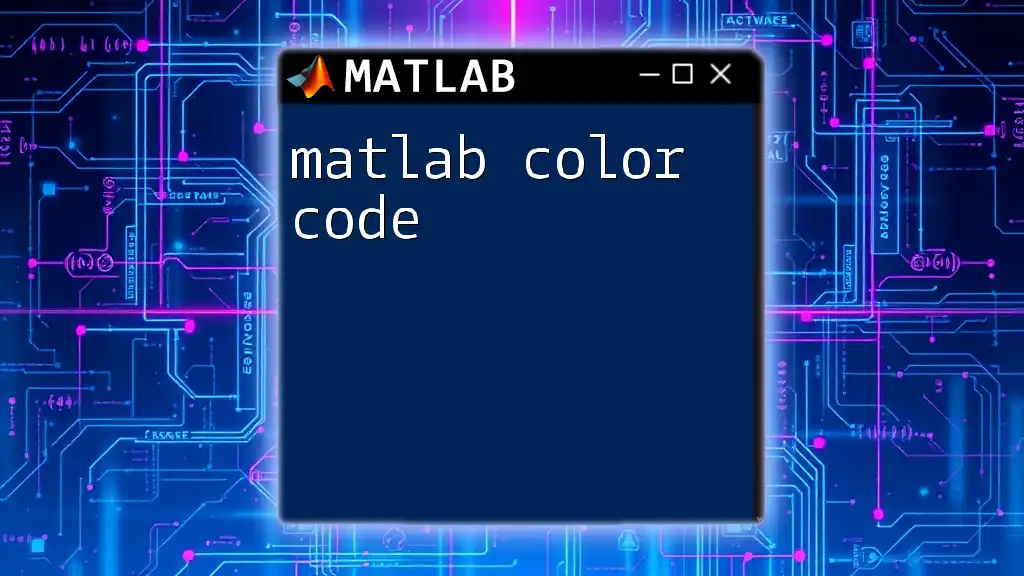
Writing Effective MATLAB Code for Coder
Coding Standards
To get the best results from MATLAB Coder, adhere to these coding practices:
- Use Functions: Break down complex algorithms into smaller, reusable functions.
- Avoid Dynamic Memory Allocation: MATLAB Coder works best with static allocations as dynamic memory could lead to unpredictable behavior in generated code.
- Minimize Global Variables: Instead, pass variables as arguments.
Code Optimization Techniques
Vectorization
Vectorization is an important concept in MATLAB that can dramatically improve performance. Instead of using loops, leverage MATLAB's matrix operations to perform calculations.
Example of Vectorization
Consider the following MATLAB code using a loop:
function y = squareArray(x)
for i = 1:length(x)
y(i) = x(i)^2;
end
end
This can be efficiently vectorized to:
function y = squareArray(x)
y = x.^2; % Vectorized operation
end
The vectorized version is not only shorter but significantly faster, especially for large arrays.
Using Built-in Functions
MATLAB is equipped with numerous highly optimized built-in functions. Utilizing these can lead to better performance and easier to maintain code. Functions like `fft`, `filter`, and `mean` should always be preferred when applicable.
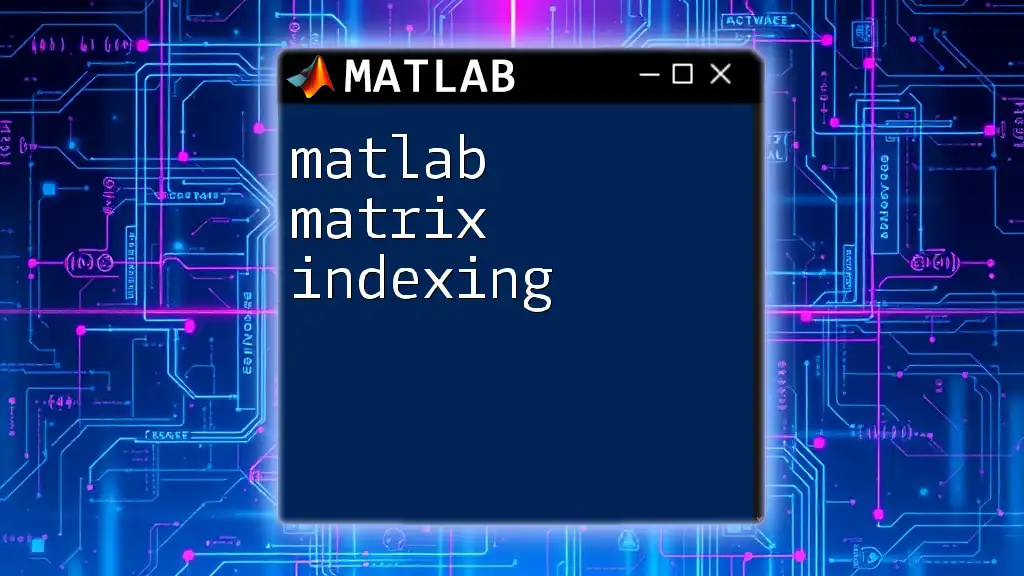
Advanced Features in MATLAB Coder
Generating Static and Dynamic Libraries
Generating libraries allows you to encapsulate your functions for ease of reuse across different projects.
To generate a static library, use:
codegen myFunction -static
This command compiles `myFunction` into a static library, which can be linked into other projects for deployment.
GPU Code Generation
MATLAB Coder can also generate code that utilizes GPU capabilities, enabling faster computation for suitable algorithms.
To specify GPU code generation:
codegen myFunction -gpu
This command converts `myFunction` to run on a GPU, which can greatly enhance performance for parallelizable tasks.
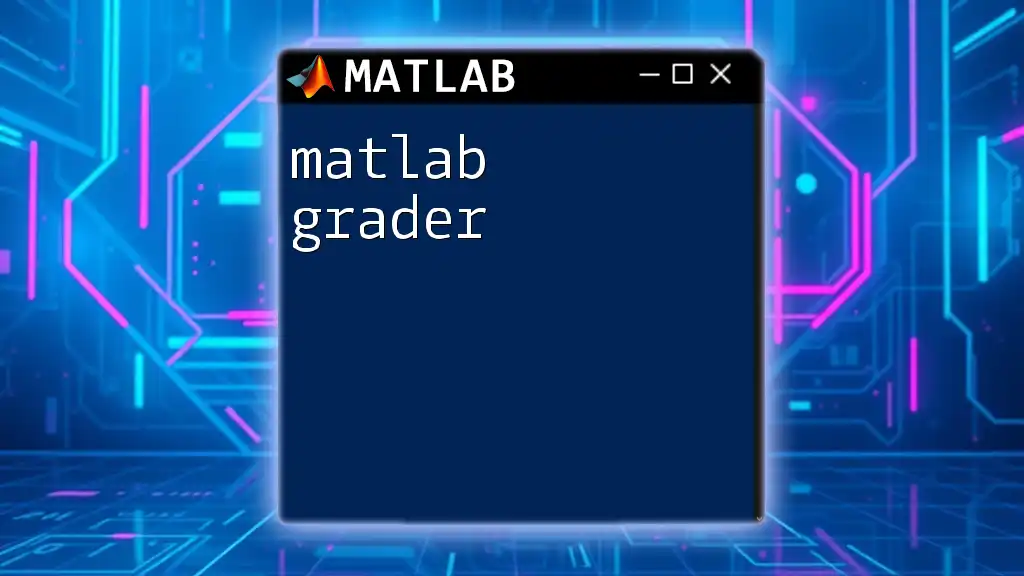
Debugging and Testing Generated Code
Debugging Techniques
When issues arise, debugging is essential. Common bugs in generated C/C++ code often stem from differences between MATLAB’s high-level operations and C’s stricter requirements. Use MATLAB’s debugging tools to step through both the MATLAB and generated C/C++ code to identify issues.
Testing Your Generated Code
Testing the generated code is vital to ensure functionality. Follow these best practices:
- Unit Testing: Create unit tests in MATLAB to validate the results of your generated code.
- Comparison Testing: Compare outputs from the MATLAB code with the generated code to ensure consistency.
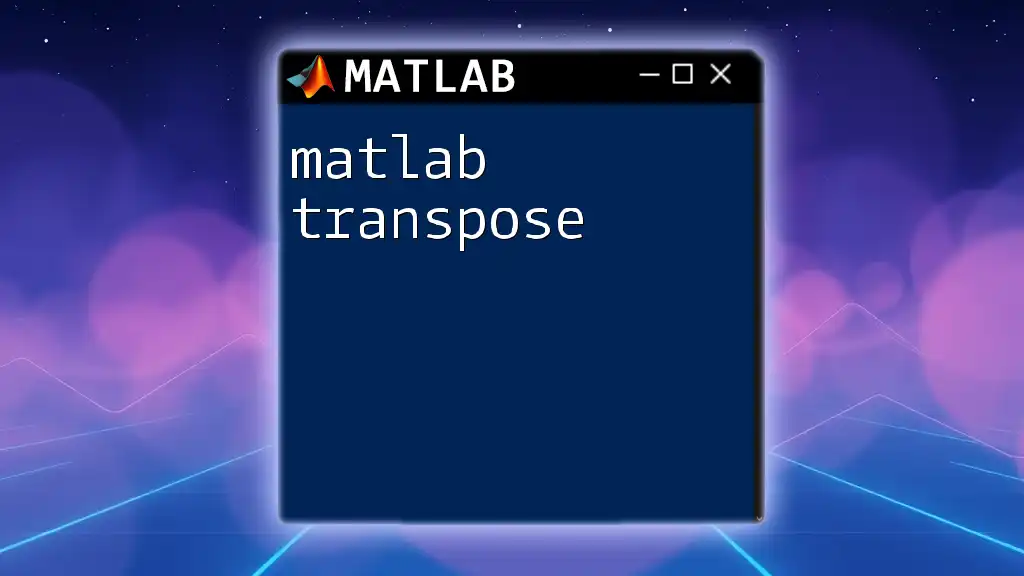
Real-World Applications of MATLAB Coder
Case Studies
Many industries have leveraged MATLAB Coder to improve their products:
- Healthcare: Medical imaging software utilizes MATLAB Coder to run algorithms for faster diagnostics.
- Automotive: Control systems developed for autonomous vehicles are often modeled in MATLAB and then converted for deployment in real-time settings.
Such real-world applications not only demonstrate the utility of MATLAB Coder but also highlight its importance in modern engineering workflows.
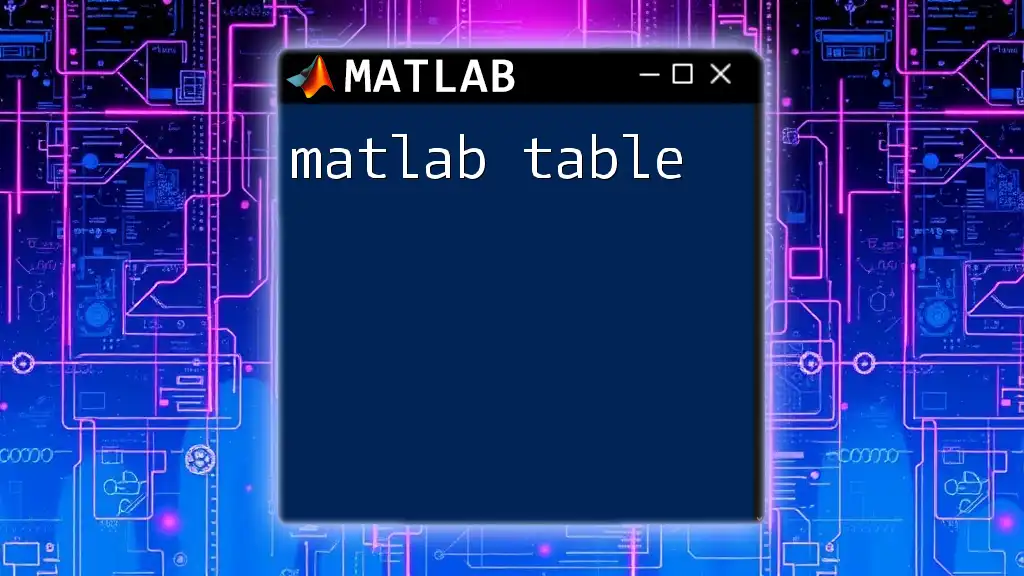
Conclusion
In conclusion, MATLAB Coder offers a powerful solution for transitioning MATLAB algorithms to C/C++ code. With its automatic code generation, flexibility in customization, and real-time application capabilities, it stands as an essential tool for engineers and developers looking to harness the power of their MATLAB code in various industries. By writing effective MATLAB code and understanding the intricacies of code generation, users can significantly enhance their productivity and code performance in real-world applications.
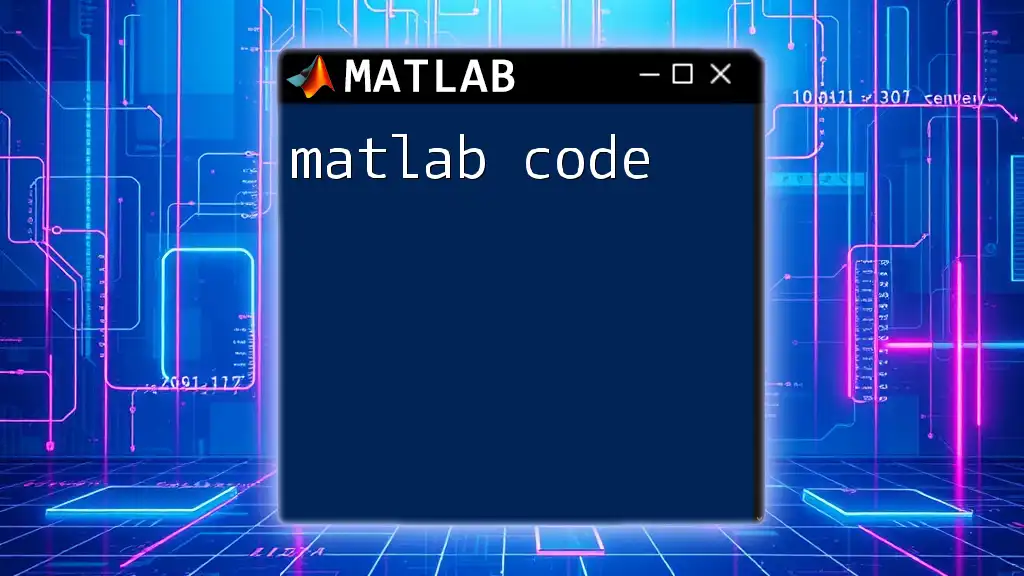
Further Resources
To further your knowledge and skills in MATLAB Coder, explore the following resources:
- Official MATLAB Documentation
- Recommended Online Courses for hands-on experience
- Community forums to join discussions and seek help from peers and experts
By diving deeper into these resources, you'll be well on your way to mastering MATLAB Coder and optimizing your workflows.