In MATLAB, comments are used to annotate code for better readability and understanding, and they are indicated by the percentage sign `%` for single-line comments or by the `%%` delimiter for section breaks.
Here's an example of how to use comments in MATLAB:
% This is a single-line comment
x = 5; % Assigning the value 5 to the variable x
%% This is a new section
y = x^2; % Calculating the square of x
The Comprehensive Guide to MATLAB Comments
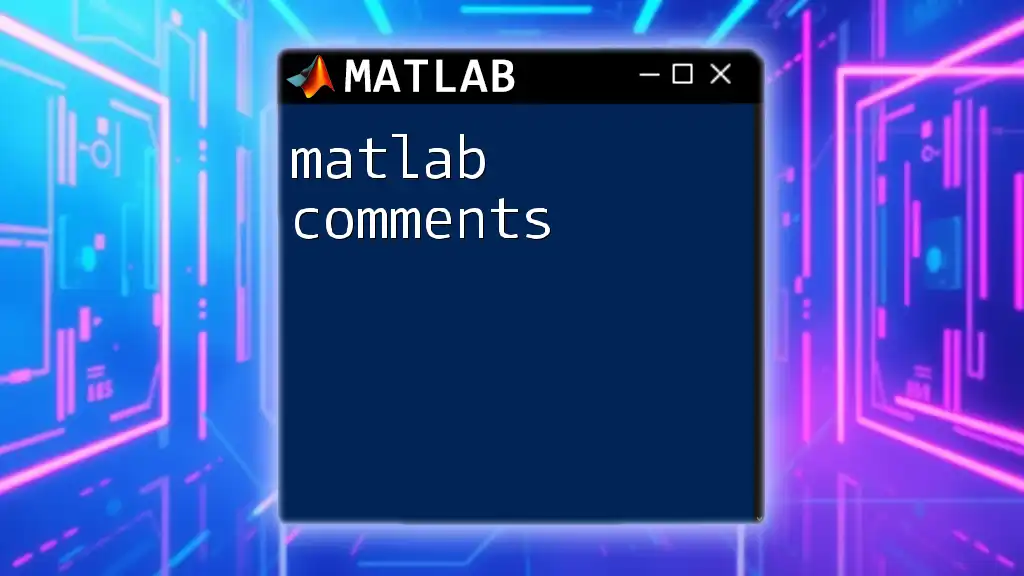
Why Use Comments in MATLAB?
Comments in MATLAB serve as an essential aspect of programming, primarily for enhancing the readability and understanding of the code. They allow developers to document their thought processes, making it easier for both the original programmer and others who might work on the code later to grasp its purpose and functionality. Proper commenting is particularly valuable in collaborative settings, where multiple individuals may need to understand and modify the same codebase.
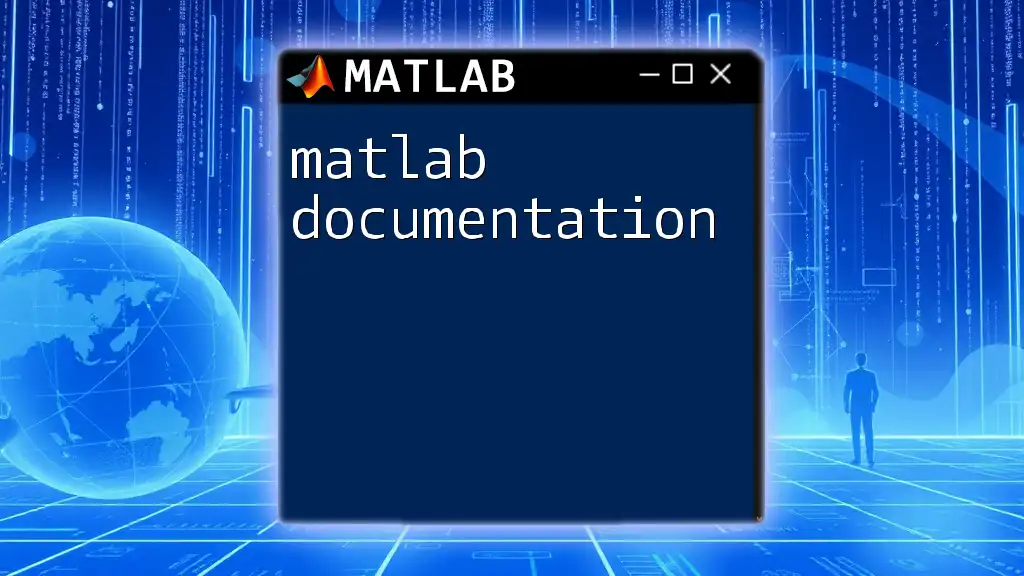
Types of Comments in MATLAB
Single-Line Comments
Single-line comments are the most straightforward way to add notes to your code. Using the percent symbol (%) at the beginning of a line tells MATLAB to ignore everything following it on that line. This simple mechanism allows you to provide quick notes or explanations.
Syntax and Simple Examples
Here's a quick look at how to use this type of comment:
% This is a single-line comment
x = 5; % Assigns the value 5 to x
In the example above, the comment `This is a single-line comment` clarifies what the subsequent line of code is doing. It’s quick and effective for small clarifications.
Block Comments
Sometimes, you may need to explain more complex sections of code. In such cases, block comments can be used. These are simply multiple single-line comments stacked together.
Creating Comments That Span Multiple Lines
% This is a block comment
% that spans multiple lines to provide
% more detailed information about the code below.
Block comments allow you to introduce more depth and context to your code, which is particularly useful when explaining intricate logic or assumptions.
Commenting Out Code
Commenting out code can be a helpful debugging technique. When you want to disable a part of your code temporarily, you can comment it out without deleting it.
Example of Commenting Out a Section of Code
% y = 3 * x; % Uncomment to execute this line for validation
This technique allows you to keep code for future reference while preventing it from executing, facilitating a smoother debugging process.
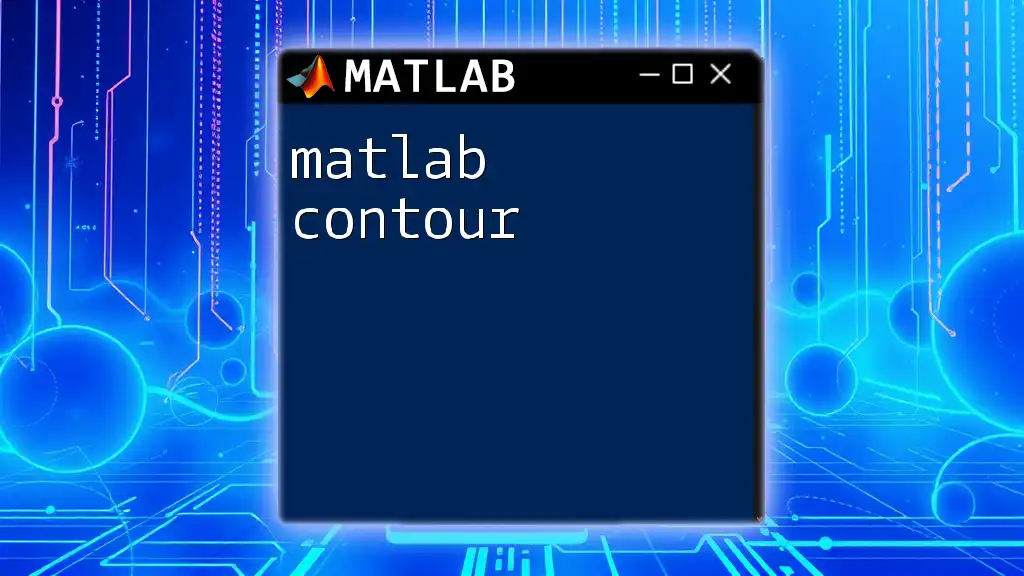
Best Practices for Writing Comments
Be Clear and Concise
Effective comments are clear and concise. Rather than cluttering your code with prolonged explanations, aim to provide simple and direct notes that get your point across.
Tips for Clarity
- Avoid jargon and overly complex language that might confuse readers.
- Aim to keep comments brief yet informative.
Here's an example showcasing good and bad commenting practices:
% Good: Calculate the area of a circle
area = pi * r^2;
% Bad: Doing a thing here
In the first case, the comment describes the functionality clearly, while the second lacks meaning.
Use Comments to Explain Why, Not Just What
While it’s important to indicate what your code does, explaining why the code is structured a certain way can be even more crucial.
Importance of Context in Comments
Providing context gives others insight into your decision-making process, especially if you took specific steps to optimize performance or avoid pitfalls.
For example:
% Why: Using a more efficient algorithm to reduce time complexity
result = optimizedFunction(data);
Here, the comment adds depth, clarifying the reason behind the choice of method.
Maintain Consistency
Consistency in your comments makes the code easier to read and understand. Establish a standard commenting format and stick to it throughout your code.
Example: Use of Bullet Points or Numbered Lists
You might consider using structured comments for complex processes. For instance:
% Steps to process data:
% 1. Load the data
% 2. Clean the data
% 3. Analyze the data
This structured approach improves readability and helps future users follow your thought process.
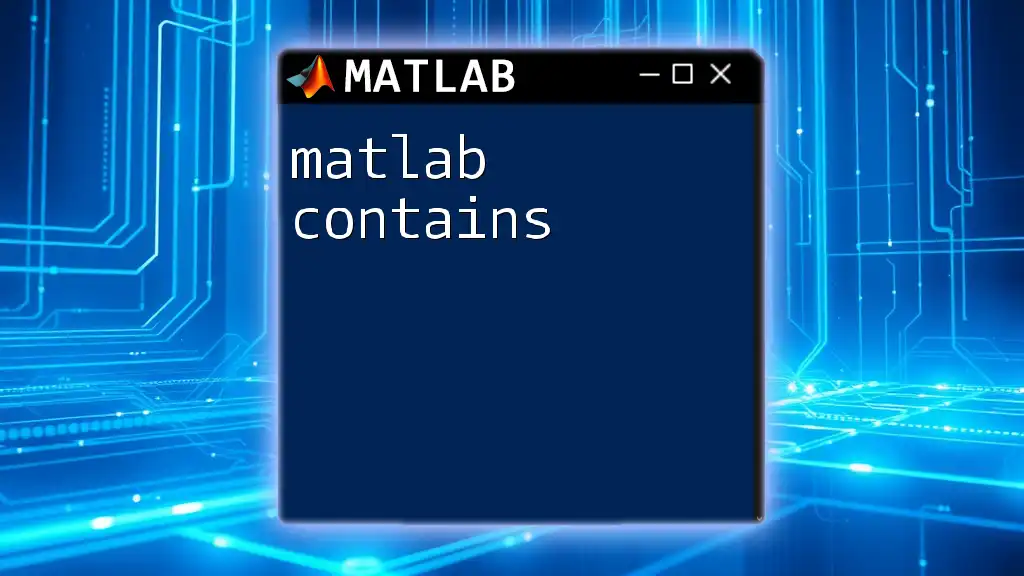
Utilizing the MATLAB Editor for Commenting
Shortcut Methods
The MATLAB editor offers various shortcuts that can enhance your efficiency when adding comments. For example, you can use Ctrl + R to comment out selected lines and Ctrl + T to uncomment them. These shortcuts streamline the process of managing comments, allowing you to focus more on coding.
Commenting Functionality in Live Scripts
MATLAB Live Scripts come with their own unique commenting features. In live scripts, comments can be embedded directly within sections of executable code, providing a more interactive way of documenting your analyses. This functionality offers both pros and cons; while it makes it easier to maintain context, it can sometimes clutter the visible code if not used carefully.
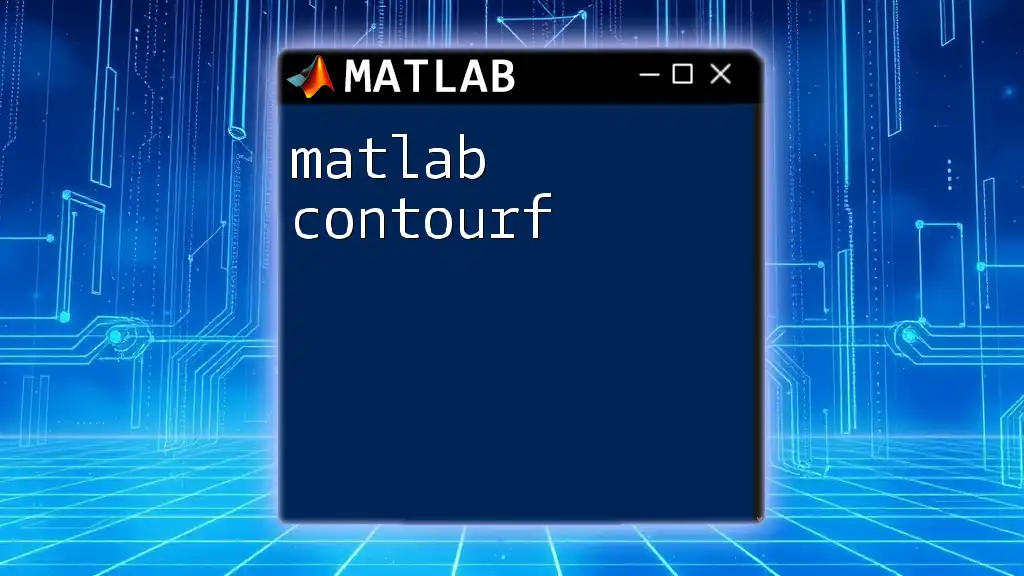
Conclusion
The significance of effective commenting in MATLAB cannot be overstated. Comments enhance readability, aid collaboration, and provide valuable context that extends the life of the code. When used properly, they transform a mere script into a well-documented and understandable piece of software development. Remember to practice good commenting habits regularly, and soon, writing clear and effective MATLAB comments will become second nature.
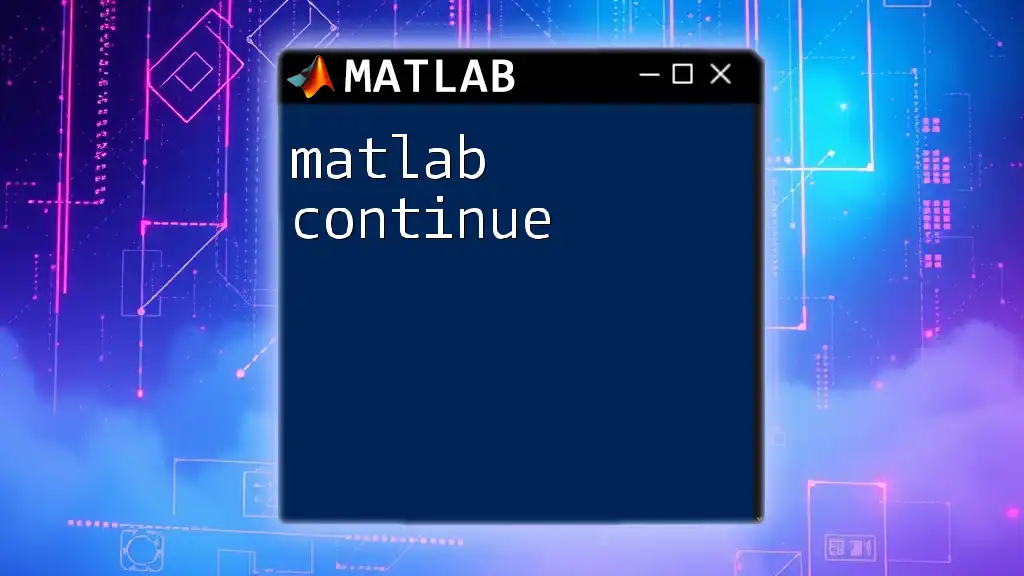
Additional Resources
For further reading, consider exploring the official MATLAB documentation on commenting and more advanced MATLAB courses to deepen your understanding of effective code documentation practices.