The MATLAB command `cos` computes the cosine of an angle given in radians, which can be easily implemented in your scripts to evaluate the cosine of a specific value.
Here’s a code snippet demonstrating its use:
angle = pi/4; % Angle in radians
cosine_value = cos(angle); % Calculate the cosine of the angle
disp(cosine_value); % Display the result
Understanding the `cos` Function
Definition of the Cosine Function
The cosine function, denoted as \( \cos(x) \), is a fundamental trigonometric function derived from the concept of the unit circle. For any angle \( x \), the cosine value represents the horizontal coordinate of the point where the terminal side of the angle intersects the unit circle (circle with a radius of 1). In many scientific and engineering applications, the cosine function plays a pivotal role in harmonic motion, signal processing, and various modeling tasks.
Purpose of the `cos` Function in MATLAB
In MATLAB, the `cos` function is used to compute the cosine of angles given in radians. This capability is integral for tasks that involve calculations in physics, engineering simulations, and a variety of mathematical computations. Knowing how to leverage the `cos` function effectively can enhance your problem-solving skills in scientific computing.
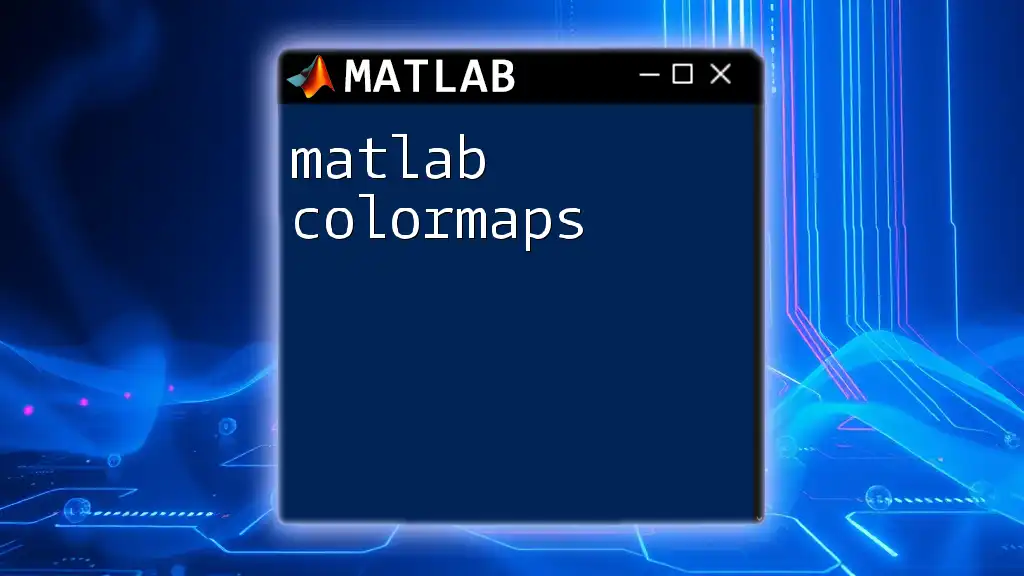
Syntax of the `cos` Function
The general syntax of the `cos` function in MATLAB is straightforward:
Y = cos(X)
Here, `X` can be a scalar, vector, or matrix containing angles specified in radians, and `Y` will be the corresponding cosine values for those angles. It’s essential to convert degrees to radians when using the `cos` function in MATLAB.
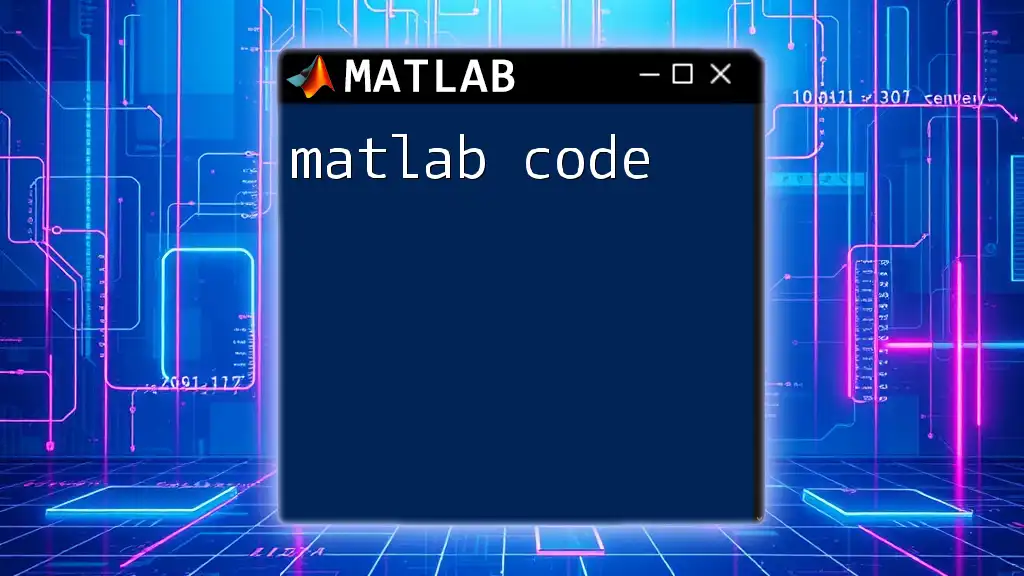
Using the `cos` Function in MATLAB
Basic Usage
To compute the cosine of a single angle, simply input the angle in radians:
angle = pi/3; % 60 degrees
result = cos(angle);
disp(result); % Display result
In this example, the `cos` function calculates the cosine of \( \frac{\pi}{3} \), which is 0.5. Remember, angles must be in radians; using degrees without conversion will lead to incorrect results.
Array and Matrix Support
One of the powerful features of MATLAB is its ability to handle arrays and matrices seamlessly. The `cos` function can be applied directly to matrices or vectors, returning the cosine result for each element.
For example, if you wish to evaluate the cosine of a set of angles, you can use:
angles = [0, pi/6, pi/4, pi/3, pi/2]; % Angles in radians
results = cos(angles);
disp(results); % Display cosine values
The output will provide the cosine values for each angle in the array, making computations efficient and concise.
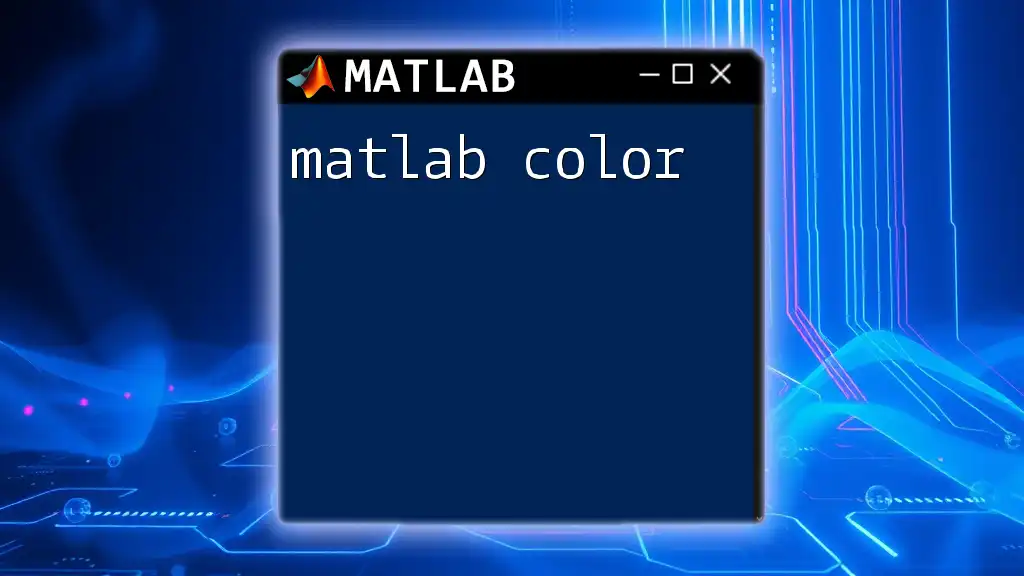
Advanced Applications of the `cos` Function
Plotting the Cosine Function
Visualizing functions is a crucial aspect of analysis in MATLAB. You can easily plot the cosine function over a range of angles to observe its periodic nature. The following code generates a plot of the cosine function for angles between 0 and \( 2\pi \):
x = 0:0.01:2*pi; % range of angles
y = cos(x);
plot(x, y);
title('Cosine Function Plot');
xlabel('Angle (radians)');
ylabel('cos(x)');
grid on;
In this plot, you’ll observe the oscillating behavior of the cosine function, confirming that it takes values between -1 and 1 with a periodicity of \( 2\pi \).
Using `cos` in Calculations
Real-world applications of the `cos` function often arise in physics and engineering. For instance, consider calculating the horizontal distance traveled by a projectile launched at an angle. If a projectile is launched at 45 degrees with an initial speed of 10 m/s, the horizontal distance (assuming no air resistance) can be calculated as follows:
Problem: Calculate the horizontal distance traveled for a projectile launched at 45 degrees with a speed of 10 m/s.
Solution:
launch_angle = 45; % Degrees
speed = 10; % m/s
horizontal_distance = speed * cosd(launch_angle);
disp(horizontal_distance);
Here, `cosd` is used to convert degrees to radians automatically, making the calculation straightforward. This application illustrates how the `cos` function assists in solving practical engineering problems.
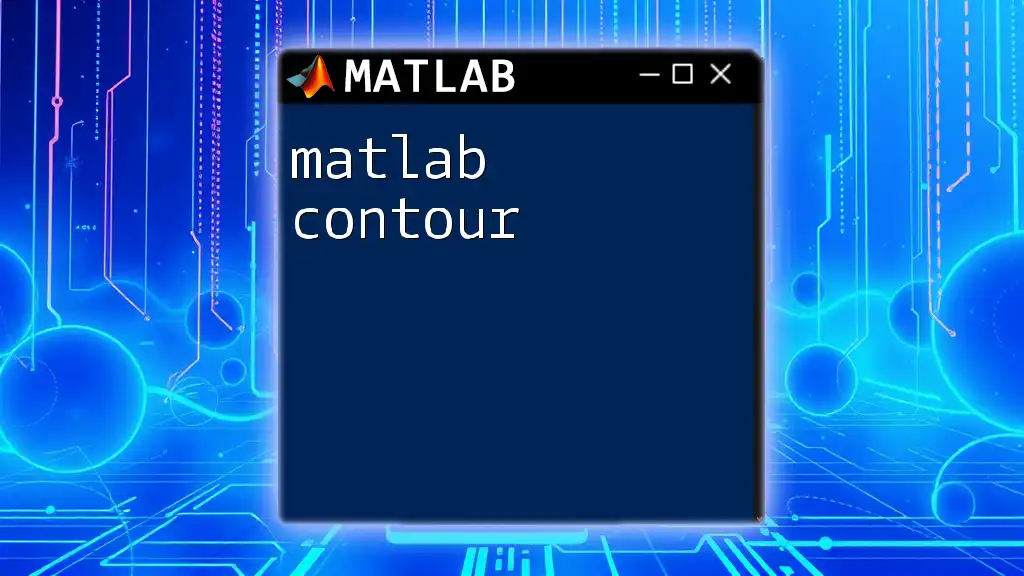
Common Errors and Troubleshooting
Common Mistakes When Using the `cos` Function
While using the `cos` function, a common mistake is assuming that angles are in degrees rather than radians. Always ensure the angles are properly converted before performing calculations.
How to Troubleshoot `cos` Function Errors
When encountering issues with your cosine calculations, consider the following tips:
- Check the units of input angles—confirm that they are in radians if using `cos`.
- Ensure no input values are NaN or inf, as these can lead to unexpected results.
- Use debugging techniques such as `disp()` to display intermediate values to trace the source of errors.
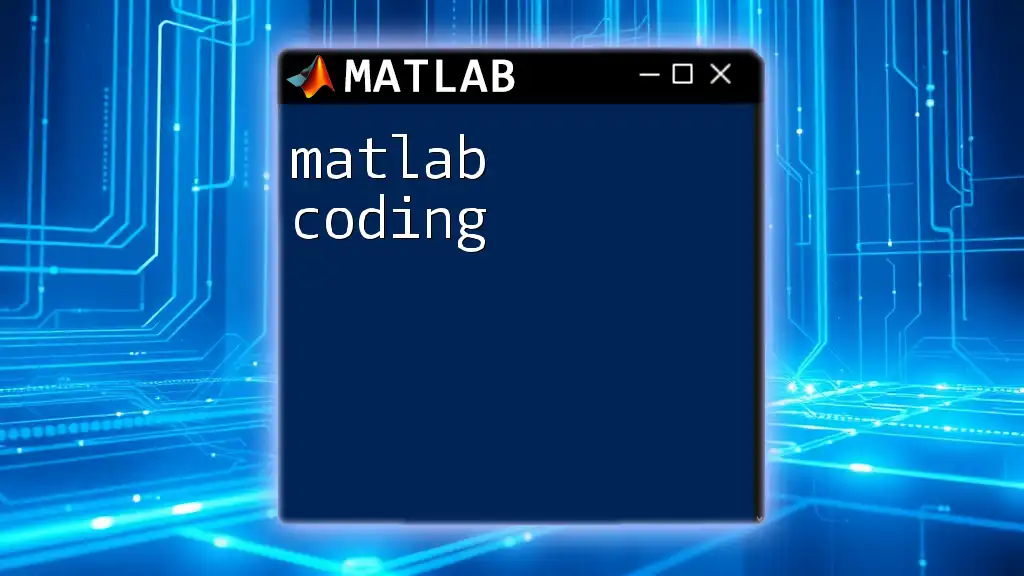
Conclusion
In summary, the `matlab cos` function is an essential tool for performing trigonometric computations effectively. With its simplicity and integration into MATLAB’s environment, you can tackle various mathematical problems and explore more advanced applications in engineering and scientific fields. By practicing the use of the `cos` function, you can gain proficiency and confidence in your MATLAB skills.