MATLAB comments are used to provide explanations or annotations within code, allowing users to better understand the functionality without impacting the execution of the program.
Here’s a code snippet demonstrating how to add comments in MATLAB:
% This is a single-line comment in MATLAB
x = 5; % Assigns the value 5 to variable x
%{
This is a
multi-line comment
in MATLAB
%}
y = x^2; % Computes the square of x
What Are Comments?
Comments are snippets of text in your code that are not executed by MATLAB. Their primary purpose is to provide explanations or annotations for the surrounding code, allowing others—or your future self—to quickly understand what the code is intended to do.
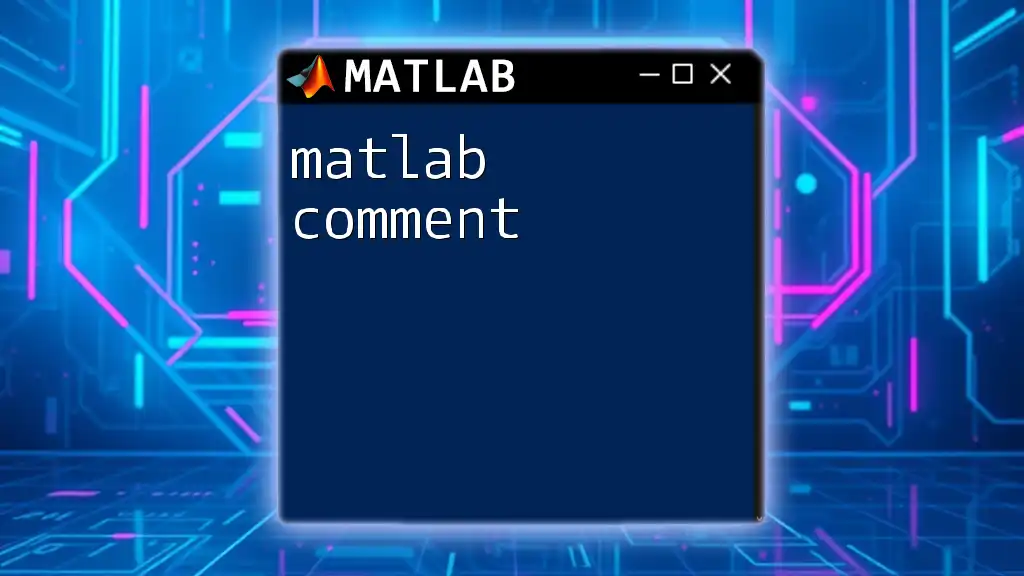
Why Use Comments in MATLAB?
Using comments in MATLAB enhances code readability and maintainability. They clarify the programmer's intentions, making it easier for others to follow the logic, especially in collaborative projects. Additionally, comments are invaluable for documenting your thought processes and justifications for particular coding choices, which can be vital when revisiting older code.
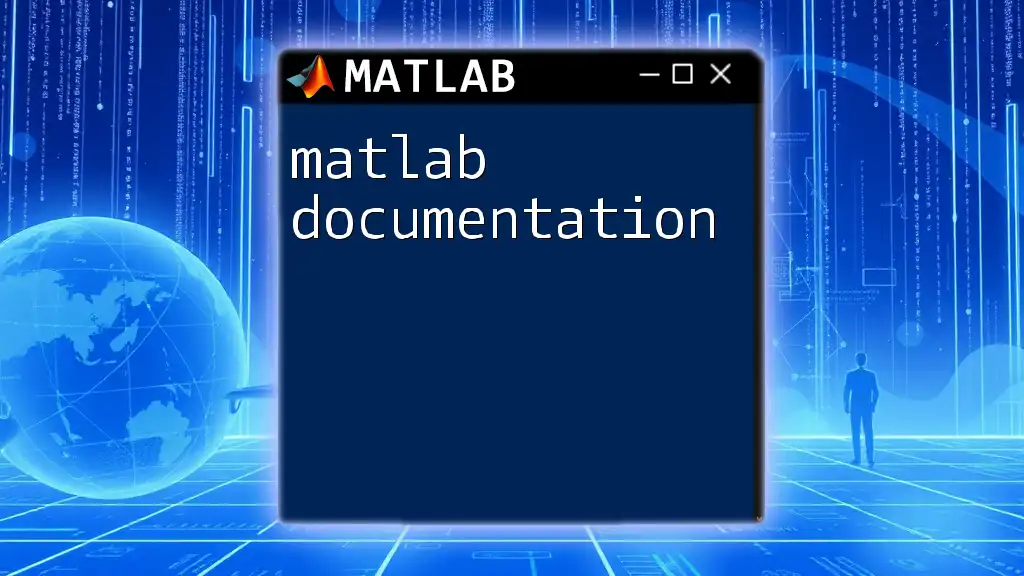
Types of Comments in MATLAB
Single-Line Comments
Single-line comments are created using the `%` symbol. Everything following this symbol on the same line is considered a comment and will be ignored by MATLAB during execution. This is ideal for brief notes or explanations.
Usage Example:
% This is a single-line comment in MATLAB
a = 5; % Assigning 5 to variable a
Best Practices
When you write comments using the `%` symbol, aim to keep them concise and relevant. For example, rather than stating the obvious:
x = 10; % Set x to 10
It is more helpful to clarify why that value is chosen:
x = 10; % Setting initial value for calculation
Multi-Line Comments
Multi-line comments allow for longer explanations and are defined using the `%{` and `%}` symbols. This is useful for providing detailed documentation, especially for complex algorithms or extended descriptions of functions.
Usage Example:
%{
This is a multi-line comment.
You can explain your code in detail here.
%}
Inline Comments
Inline comments are a great way to add context directly next to specific lines of code without interrupting the flow of the script.
Examples and Best Practices:
b = a + 3; % Adding 3 to variable a
Use inline comments judiciously to avoid clutter. Always strive for clarity—if a comment requires as much explanation as the code itself, consider restructuring your code or using a multi-line comment.
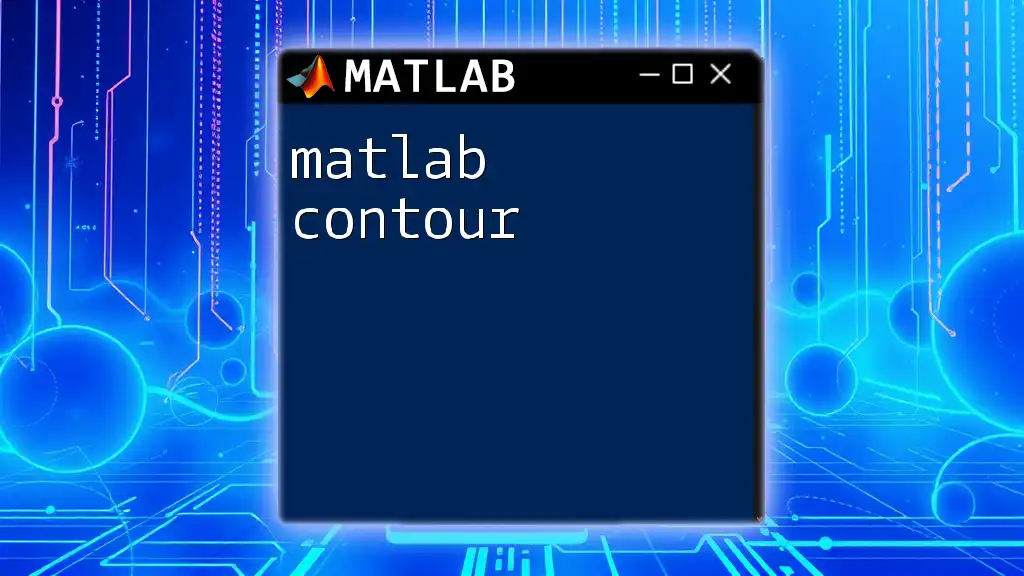
Effective Commenting Techniques
Writing Clear Comments
The key to effective commenting is clarity and precision. Your comments should communicate your intention without ambiguity. Instead of writing vague comments, focus on providing actionable information that can guide future use.
Example Commentary:
% Initialize the variable for total revenue
totalRevenue = 1000; % Revenue from sales
Structuring Your Comments
Organizing your comments can help to break down complex operations into understandable segments. This is especially useful in longer scripts where individual sections may serve different purposes.
Example of Structured Comments:
% Calculate the area of a circle
radius = 5; % Radius of the circle
area = pi * radius^2; % Area calculation using the formula A = πr²
Commenting Out Code
Sometimes, it is beneficial to temporarily disable sections of code for testing or debugging purposes. This can be achieved by commenting out the code.
Example Code:
% plot(x, y); % Commented out to disable plotting
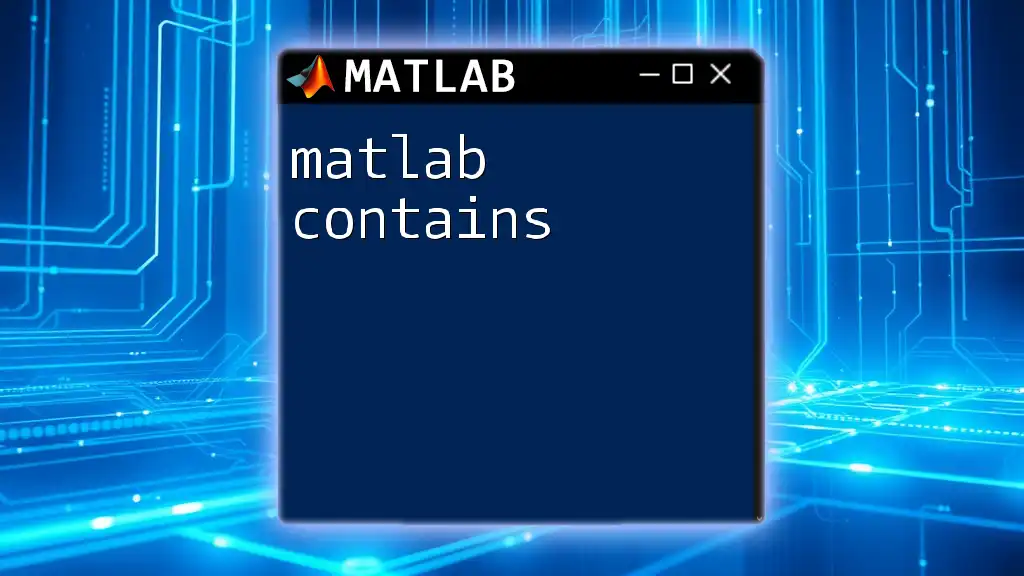
Using Comments for Documentation
Importance of Documentation
Comments can double as documentation, providing essential information to anyone who might use or modify the code in the future. Good commenting practices can save lots of time and confusion down the line, making it easier to maintain or refactor code.
Commenting for Future Reference
Comments can aid in future code maintenance by giving context as to why certain coding choices were made. This insight is crucial for revising or improving code.
Example of Documentation Comments:
% This function calculates the factorial of a number
% Input: positive integer n
% Output: factorial of n
function result = factorial(n)
Docstrings in MATLAB
In MATLAB, docstrings serve as a robust form of documentation. They allow you to describe what your function does, its inputs, and its outputs in a structured way.
Example of Using Docstrings:
function result = sumOfSquares(x)
% sumOfSquares calculates the sum of squares
% of the elements in vector x
result = sum(x.^2);
end
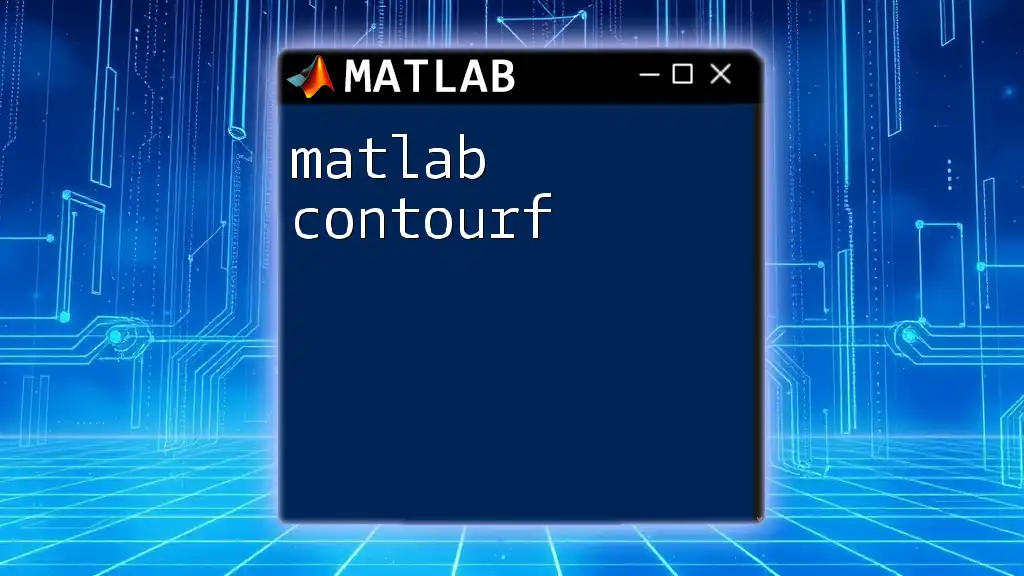
Common Mistakes to Avoid
Over-Commenting
Over-commenting is a common pitfall where comments are excessive and clutter the code. Aim for a balance that keeps your code both readable and clean.
Example of Over-Commenting:
a = 5; % Create a variable called a
Vague Comments
Vague comments can lead to confusion and misunderstandings. It's essential to be specific and informative in your commentary to avoid ambiguity.
Example of a Vague Comment:
% Do something with x
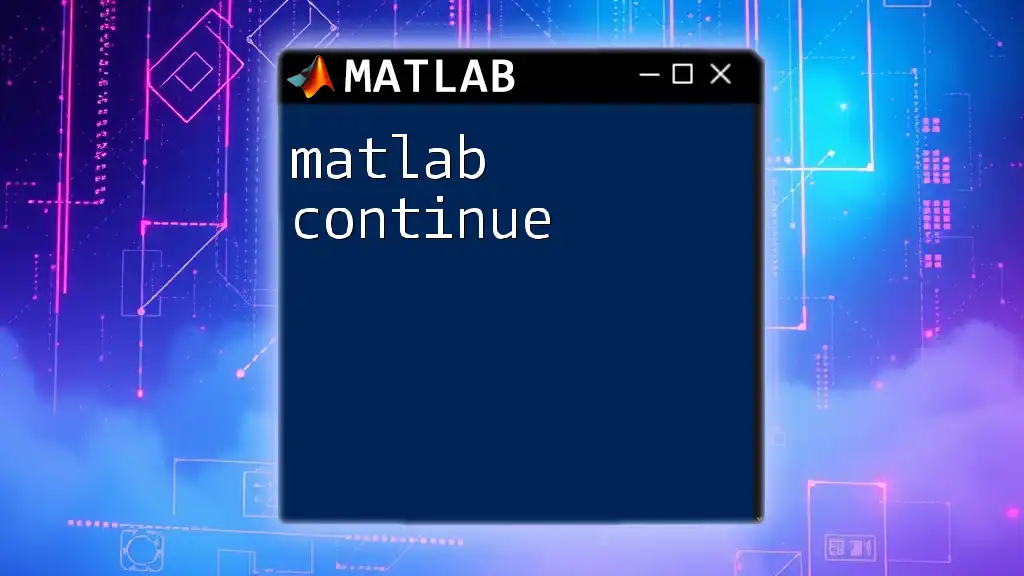
Best Practices for Commenting in MATLAB
Consistency
Maintaining a uniform commenting style across your scripts and functions is crucial. This consistency facilitates easier reading and comprehension.
Updating Comments
As your code evolves, it's vital to keep comments up to date. Comments must accurately reflect the current functionality of the code to remain useful.
Tools for Managing Comments
MATLAB’s editor provides several features for efficiently managing comments, such as toggling comments on selected lines, which helps streamline the process of commenting during coding sessions.
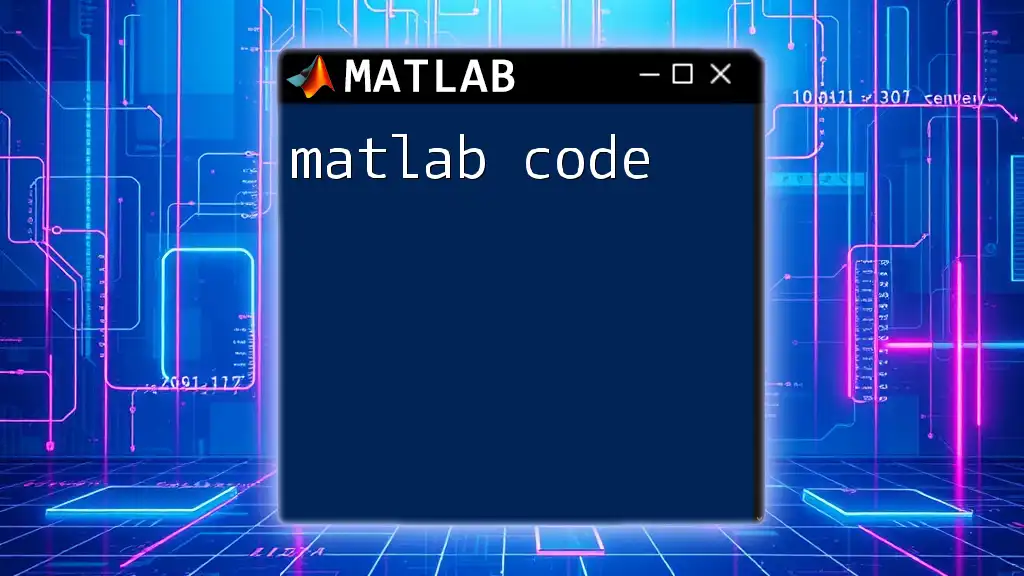
Conclusion
In conclusion, using MATLAB comments effectively can significantly enhance the quality, clarity, and maintainability of your code. By following the guidelines and best practices laid out in this guide, you can ensure that your comments serve their intended purpose—making your code easier to read, understand, and maintain. Whether you're working alone or as part of a larger team, investing time in thoughtful commenting will pay dividends in the long run.
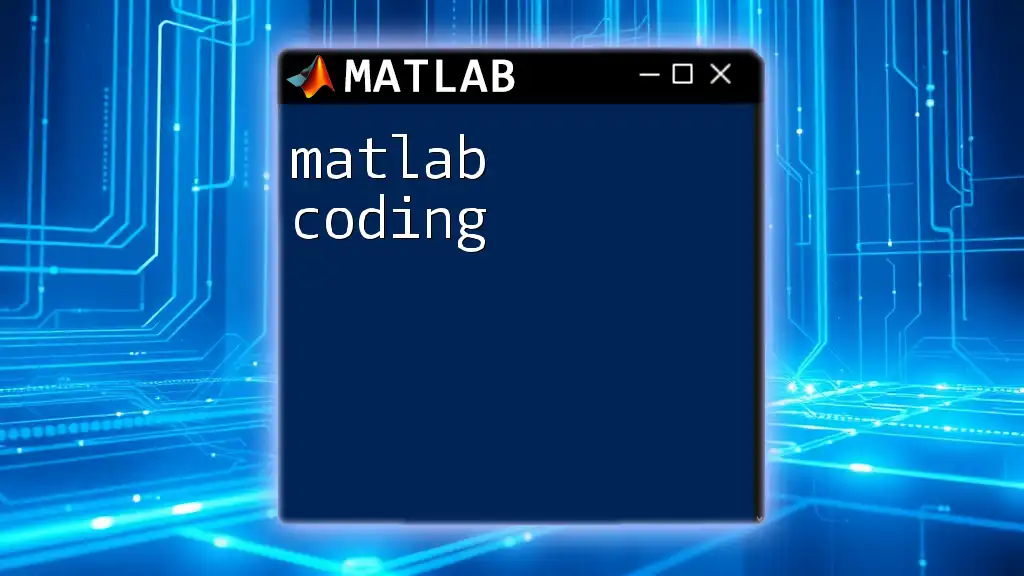
Call to Action
To learn more about MATLAB programming and efficient coding practices, consider exploring further resources, such as tutorials or courses designed to enhance your skills and support your journey in mastering MATLAB.