The `csvread` function in MATLAB is used to read comma-separated values (CSV) files and import the data into a matrix.
data = csvread('datafile.csv', row, col);
In this example, replace `'datafile.csv'` with the name of your CSV file, and specify `row` and `col` to indicate the starting position in the file from which to begin reading the data.
Understanding CSV Files
CSV, or Comma-Separated Values, is a widely used format for storing tabular data in plain text. Each row of data is separated by a newline, and each value within a row is separated by a comma. This format is often used for exporting and importing data between various applications, particularly in spreadsheet tools like Microsoft Excel and data analysis software.
CSV files are popular in data science and analytics due to their simplicity and compatibility across different platforms. They enable users to store large datasets efficiently and provide a straightforward method for exchanging data.
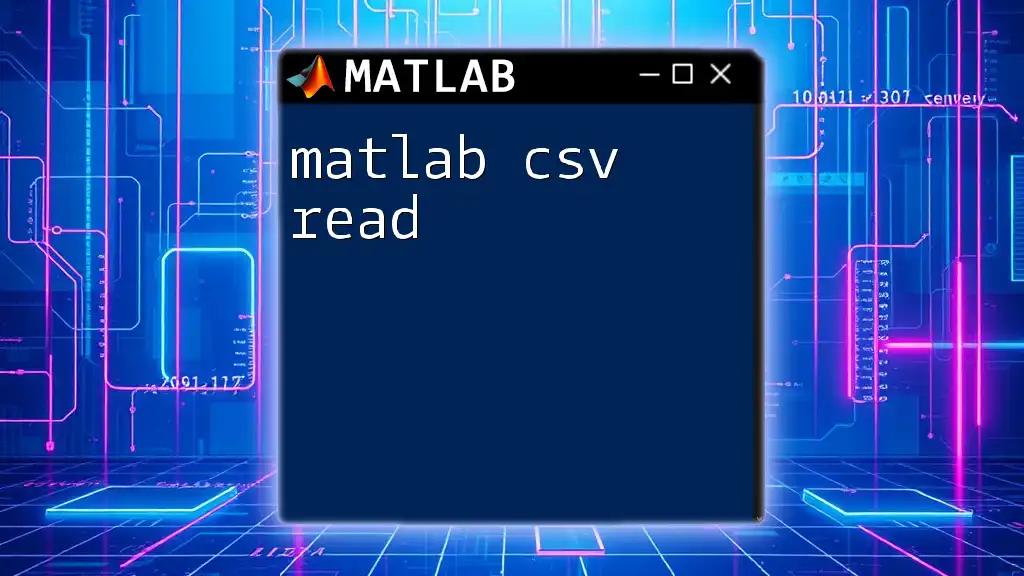
What is csvread?
The `csvread` function in MATLAB serves as an efficient way to read data from CSV files into a matrix format. The primary motivation for using `csvread` is its ability to quickly capture numerical data from .csv files while stripping away any text or extraneous formatting. This makes `csvread` particularly valuable for users who need to handle numeric datasets effectively.
Using `csvread` is suitable in various scenarios, including data analysis, visualization, and even machine learning. By enabling users to read data directly into MATLAB, `csvread` significantly streamlines the workflow of data processing.
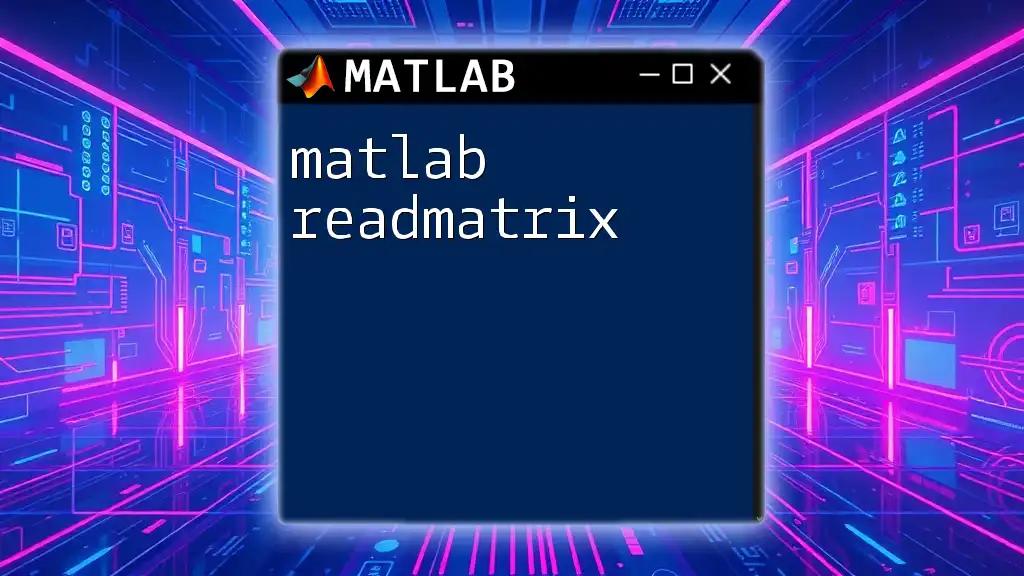
Syntax of csvread
The syntax of `csvread` is straightforward, and understanding its parameters is crucial for proper data import:
-
Basic Syntax:
M = csvread(filename)
-
Advanced Syntax:
M = csvread(filename, R, C)
Where:
- `filename` refers to the path of the CSV file you wish to read.
- `R` represents the starting row (the default is 0).
- `C` represents the starting column (the default is also 0).
These parameters allow for precise control over which part of the CSV file is read into MATLAB, enabling users to skip any rows or columns that are unnecessary for their analysis.
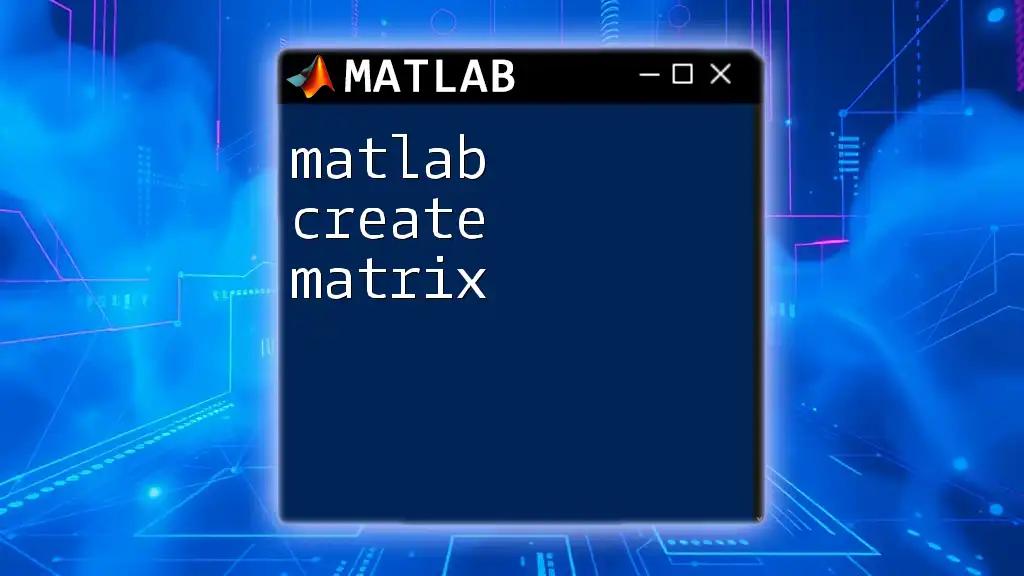
How to Use csvread
To effortlessly load data from a CSV file using `csvread`, you can follow these steps:
First, ensure that your CSV file is readily available in the specified path. Here’s a simple example of loading a basic CSV file:
M = csvread('datafile.csv');
Upon executing this command, MATLAB reads the entire CSV file starting from the top-left corner (0,0). The resulting matrix `M` contains all the numeric data from the CSV file.
Specifying Rows and Columns
A powerful feature of `csvread` is its ability to specify starting points by defining the row and column from which to begin reading. This is particularly helpful when your CSV file contains headers or metadata that you want to ignore.
For instance, if your data starts from the second row and the third column, you can use:
M = csvread('datafile.csv', 1, 2);
In this command:
- `1` indicates that MATLAB should start reading from the second row (counting starts from zero).
- `2` specifies that reading should begin from the third column.
This flexibility ensures users can import only the relevant portion of their data.
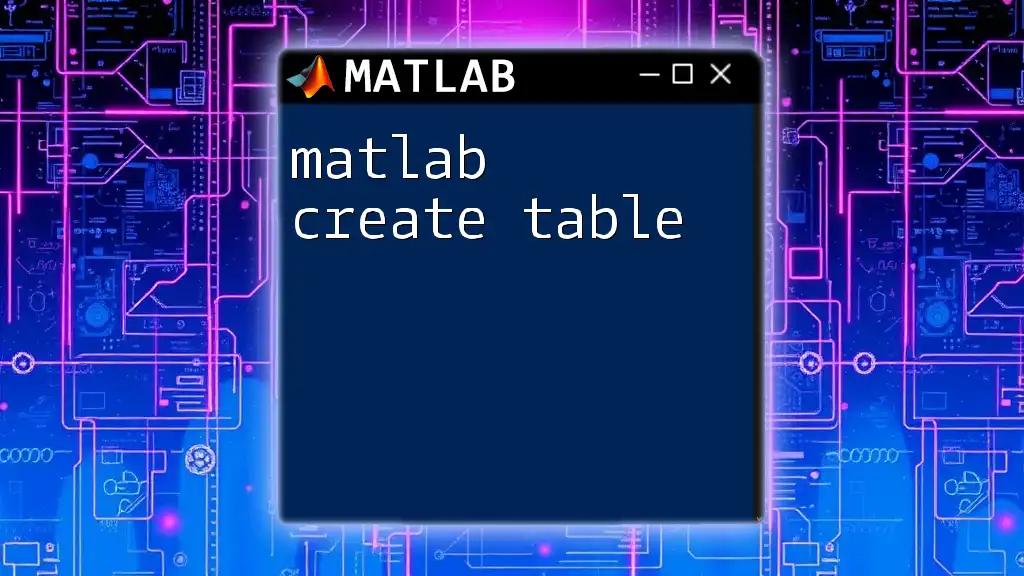
Error Handling in csvread
While using `csvread`, users may encounter a few common errors. It’s essential to understand these issues to troubleshoot effectively:
-
File Not Found: Ensure that the filepath specified in the `filename` parameter is correct. If MATLAB cannot locate the file, it will throw an error.
-
Incorrect Formatting: If the file contains non-numeric data or is improperly formatted, `csvread` may fail. To resolve this, check that your CSV file contains well-structured data.
To combat potential issues, it’s advisable to validate file integrity before running `csvread`. Use the `exist` function in MATLAB to check if the specified file exists.
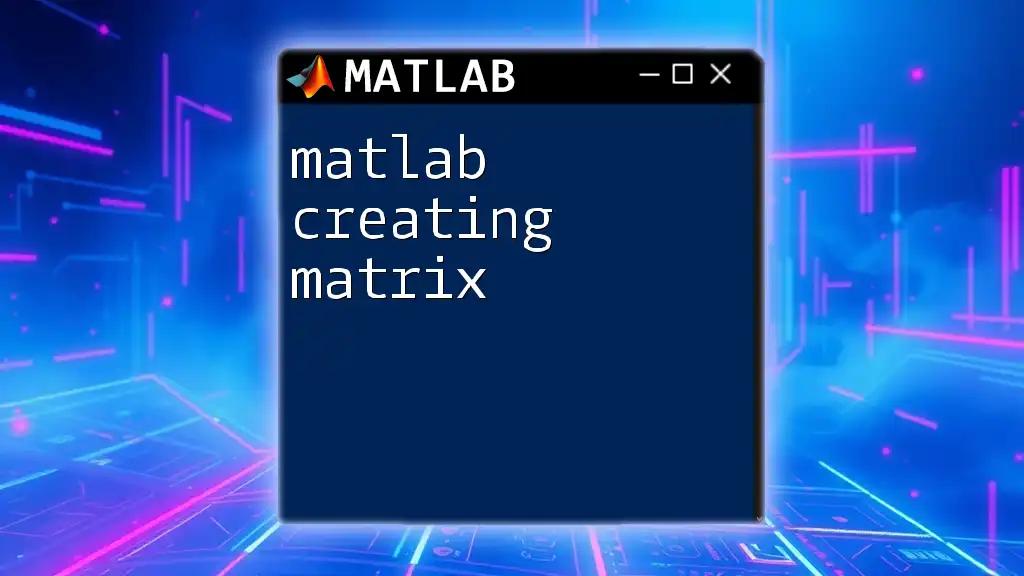
Alternatives to csvread
While `csvread` is a convenient function, MATLAB offers several alternatives for reading CSV files, especially with the introduction of newer functions:
-
`readmatrix`: This function provides more functionality, allowing for the reading of mixed data types and better error handling capabilities.
-
`readtable`: This function is useful when working with datasets that contain both numeric and text data. It returns a table rather than a matrix, which can be advantageous for data manipulation.
When choosing among these alternatives, consider the nature of your data and your specific requirements.
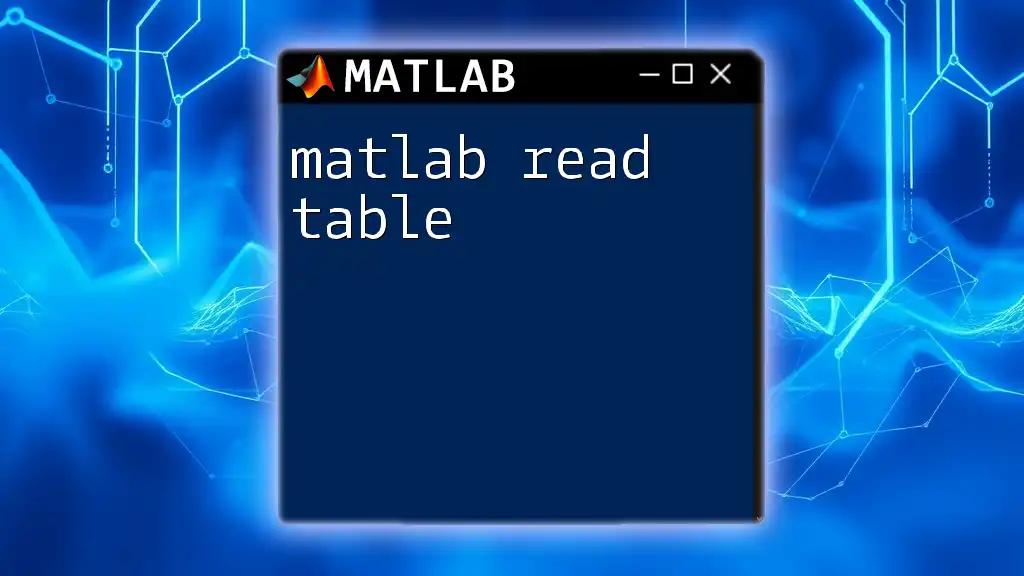
Best Practices for Working with CSV Files in MATLAB
To maximize the efficiency of your workflow when using `csvread` or any CSV reading function in MATLAB, adhere to these best practices:
-
Prepare Your CSV Data: Ensure your data is clean and well-structured. Remove unnecessary rows or columns before saving the file.
-
Choose Compatibility: When saving CSV files, use simple characters and avoid special formatting to ensure that MATLAB can interpret the data correctly.
-
Data Validation: Always validate the integrity of the dataset after loading it. Check for missing values or anomalies in your imported matrix.
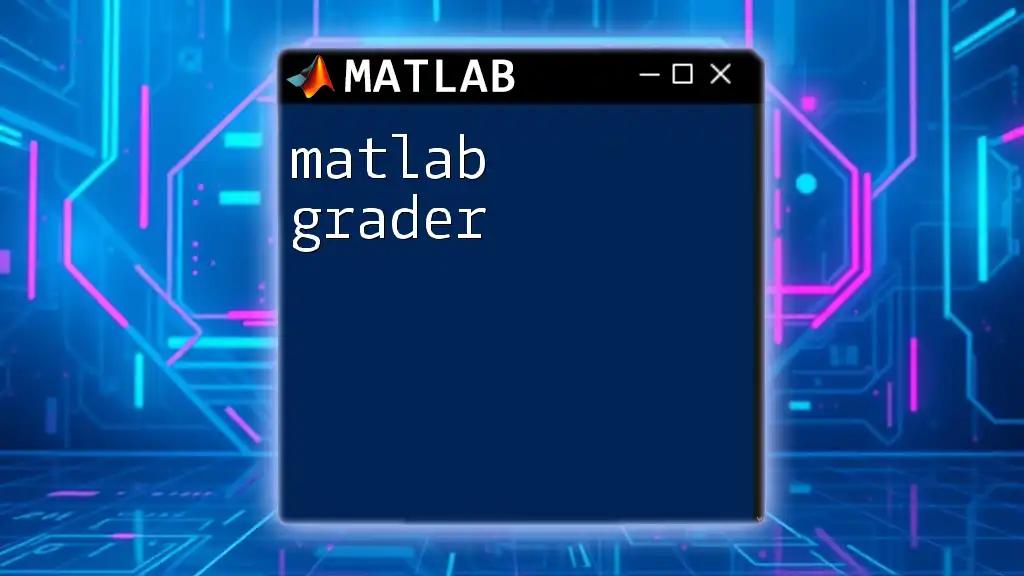
Practical Examples
Practical application of the `csvread` command can significantly enhance your data analysis capabilities. Here are two examples that demonstrate its utility:
Example 1: Analyzing Experimental Data
Assume you have a CSV file named `experiment_data.csv` that contains several rows of experimental results. You can load and analyze the data as follows:
M = csvread('experiment_data.csv');
mean_value = mean(M(:,2)); % Calculate mean of the second column
In this case, `M(:,2)` accesses the second column of the dataset, and `mean` calculates the average value, aiding in data insights.
Example 2: Visualizing Data
You can also visualize CSV data directly after importing it using `csvread`. For example, if your CSV contains time-series measurements, you might visualize it with:
M = csvread('timeseries_data.csv');
plot(M(:,1), M(:,2));
xlabel('Time (s)');
ylabel('Measurement');
title('Time Series Data Visualization');
This plot illustrates the relationship between time and measurements, making it easier to interpret results graphically.
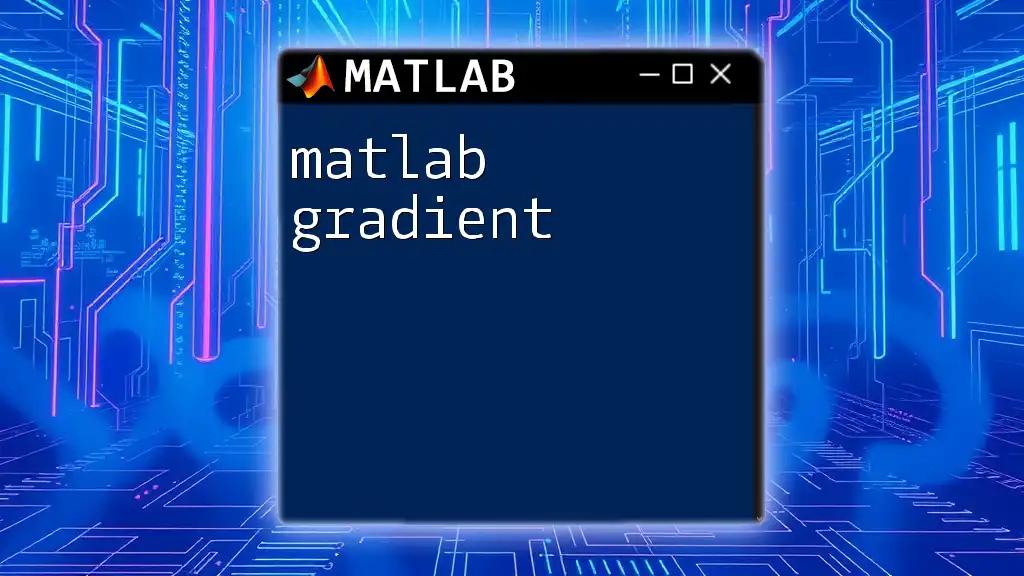
Conclusion
In summary, the `csvread` function is an indispensable tool for efficiently loading CSV data in MATLAB. Its ease of use, coupled with its ability to handle diverse datasets, makes it a go-to function for many data analysts. By mastering `csvread`, you can enhance your productivity and streamline data analysis workflows.
As you continue to practice with `csvread`, consider exploring more advanced features in MATLAB to further enrich your data handling skills. Engaging with various datasets will also solidify your understanding and build confidence as you work through challenges in data analysis.
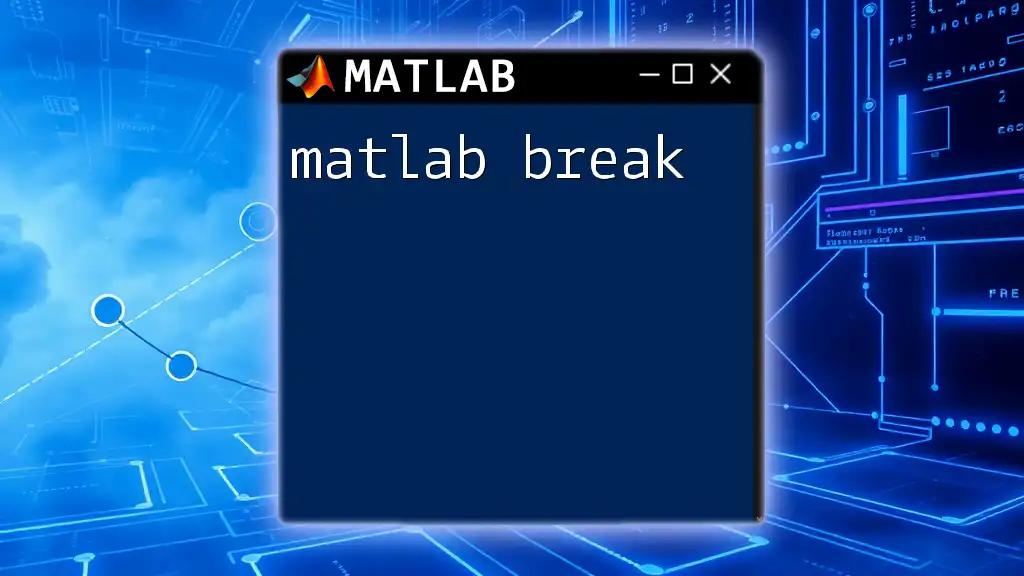
Additional Resources
For further learning, consult the official MATLAB documentation for `csvread`. Additionally, online courses and tutorials on MATLAB data handling will provide extensive insights and practical skills to apply your knowledge effectively.