MATLAB supports various data types, including numeric arrays, cell arrays, structures, and tables, allowing flexible data manipulation and organization.
Here's a simple example of defining different data types in MATLAB:
% Numeric array
numArray = [1, 2, 3; 4, 5, 6];
% Cell array
cellArray = {1, 'text', [7, 8, 9]};
% Structure
structExample = struct('field1', 10, 'field2', 'example');
% Table
tableExample = table([1; 2], {'first'; 'second'}, 'VariableNames', {'Numbers', 'Text'});
Understanding MATLAB Data Types
MATLAB is built upon distinct data types that are crucial for how data is stored and manipulated. Understanding these MATLAB data types can dramatically enhance your programming efficiency, improve performance, and streamline your data analysis processes. Each data type serves a unique purpose, and choosing the right one can significantly affect the outcome of your calculations and data management.
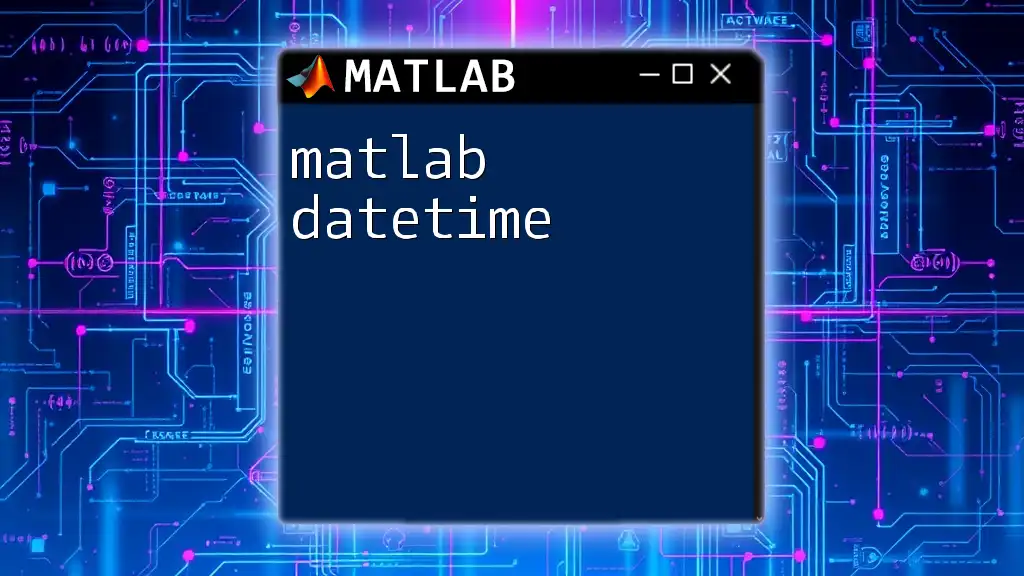
Fundamental Data Types in MATLAB
Numeric Types
Double-Precision Arrays
The most common type of numerical data in MATLAB is the double-precision array. MATLAB uses double-precision by default for representing numbers which allows for a good balance between range and accuracy.
Code Example:
x = 1.5; % double data type
Key uses include mathematical computations, matrix operations, and statistical analyses. Double arrays are characterized by their ability to represent a wide range of real numbers, making them suitable for scientific computations.
Single-Precision Arrays
A single-precision array uses less memory than a double-precision array but at the cost of some accuracy. It is specifically useful when memory efficiency is critical, such as when dealing with large datasets.
Code Example:
y = single(3.14); % single data type
Opt for single precision when working with large matrices or arrays that need to conserve memory and where slight inaccuracies are acceptable.
Integer Types
MATLAB supports various integer types such as `int8`, `int16`, `int32`, and `int64`. These types are particularly useful in situations requiring the representation of whole numbers without decimal points.
Code Example:
z = int32(100); % int32 data type
Choosing the appropriate integer type depends on the expected range of numbers. For example, `int8` can hold values from -128 to 127, while `int32` can accommodate a range from -2,147,483,648 to 2,147,483,647.
Character and String Arrays
Character Arrays
A character array in MATLAB is a matrix of characters. Each element of the array is an individual character, which can be used for string manipulations or to store textual information.
Code Example:
charArray = 'Hello, MATLAB!'; % character array
Character arrays are ideal for legacy code and certain situations, but they have largely been superseded by string arrays for textual operations.
String Arrays
With the introduction of string arrays in newer versions of MATLAB, working with text data has become more straightforward. String arrays are designed to simplify the manipulation of strings.
Code Example:
stringArray = "Welcome to MATLAB!"; % string array
The advantages of string arrays include better handling of element-wise operations and improved functionality for text data, such as searching and splitting.
Logical Data Type
Logical data types are binary in nature, representing only two possible values: true or false. These data types play a crucial role in control flow, logical indexing, and conditions.
Code Example:
logicalArray = [true, false, true]; % logical array
Logical arrays can be particularly useful in filtering data sets and performing operations based on specific conditions.
Complex Numbers
MATLAB has native support for complex numbers, making it an excellent choice for scientific computing. Complex numbers are represented in the form of `a + bi`, where `a` is the real part and `b` is the imaginary part.
Code Example:
complexNum = 3 + 4i; % complex number
These numbers are crucial in fields like electrical engineering, signal processing, and control systems, where complex mathematics are prevalent.
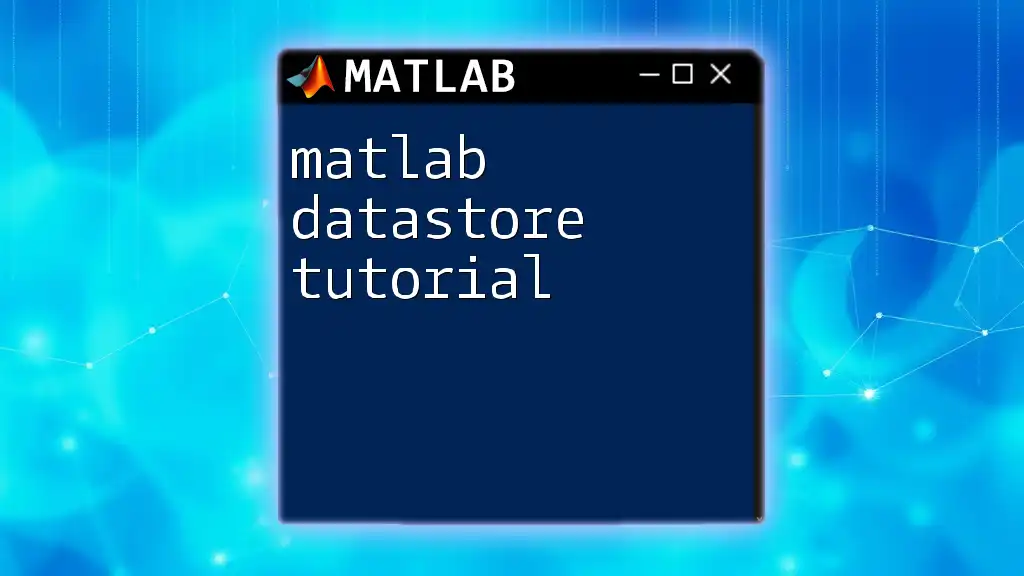
Special Data Types
Cell Arrays
Cell arrays are versatile data structures that allow for storage of mixed types of data. Unlike traditional arrays that require all elements to be of the same type, cell arrays can hold numbers, strings, or even other arrays.
Code Example:
cellArray = {1, 'text', [1, 2, 3]}; % cell array
Cell arrays are particularly beneficial when your data is heterogeneous and you need to preserve this variety without type restrictions.
Structures
Structures in MATLAB are another powerful way to organize data. They allow you to group different types of data under a common name, providing a meaningful organization for related variables.
Code Example:
student = struct('name', 'Alice', 'age', 21); % structure
Structures make it easier to manage complex data and can represent records similar to rows in a spreadsheet.
Tables
Tables are a high-level data structure ideal for storing mixed-type data and providing easy access to variable names, types, and descriptive statistics.
Code Example:
T = table(['Tom'; 'Jerry'], [5; 10]); % table
Tables are especially useful in data analysis and processing tasks, allowing you to handle data much like you would in a spreadsheet or database.
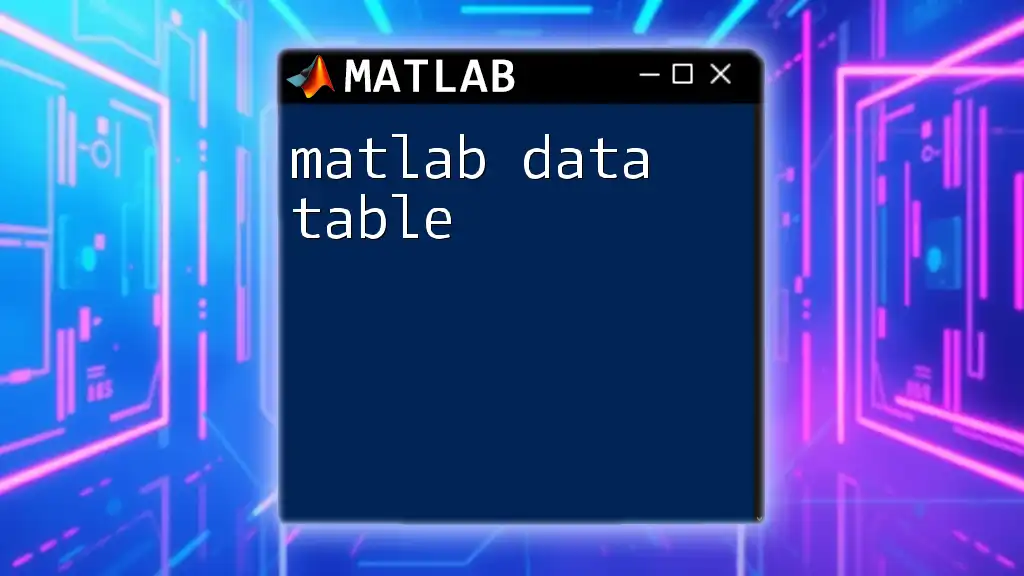
Type Conversion and Compatibility
Sometimes, you may need to convert data from one type to another. MATLAB provides various built-in functions to handle such conversions seamlessly.
For example, you can convert a number to a double using:
a = 5;
b = double(a); % Convert to double
Type conversion is essential for ensuring that your calculations remain accurate and that you avoid potential errors stemming from incompatible data types.
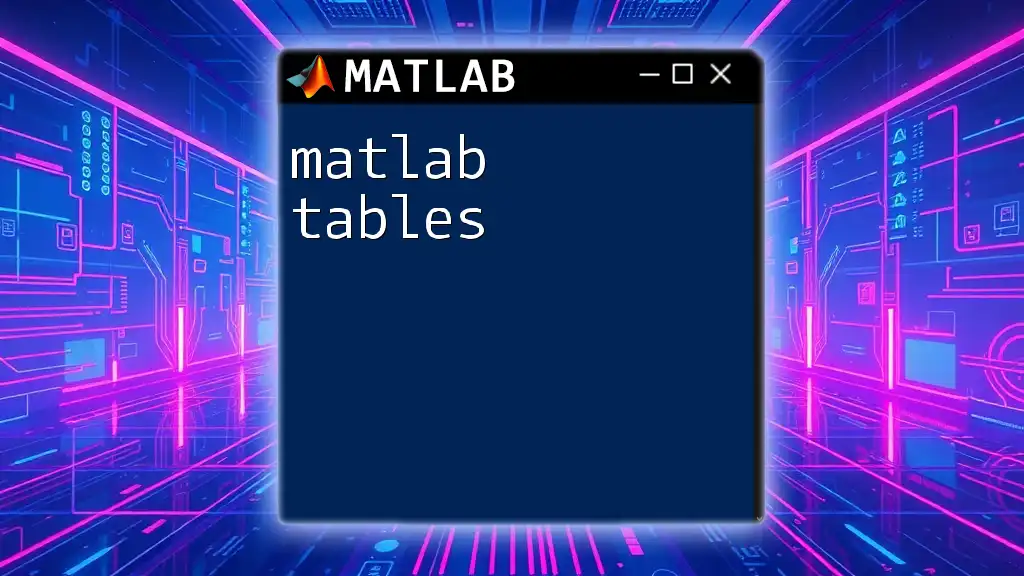
Summary
Understanding MATLAB data types is fundamental for any MATLAB user, as it directly influences the effectiveness and performance of your code. By being aware of the distinctions among numeric types, character and string arrays, logical data, complex numbers, and specialized structures, you can optimize your coding practices.
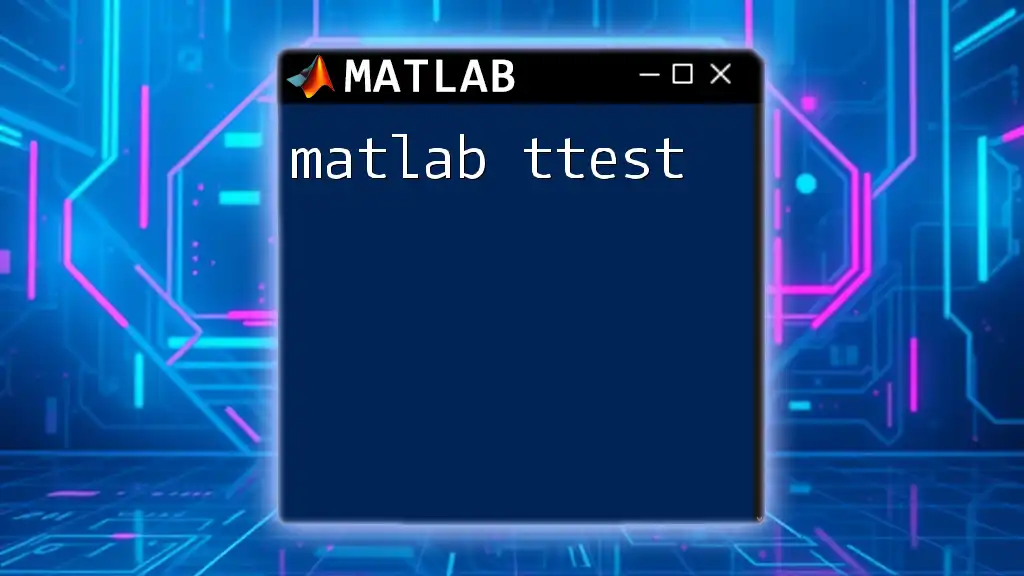
Additional Resources
For those eager to delve deeper, the official MATLAB documentation offers extensive resources and tutorials. Exploring various examples and engaging in practice exercises will enhance your command over these data types.
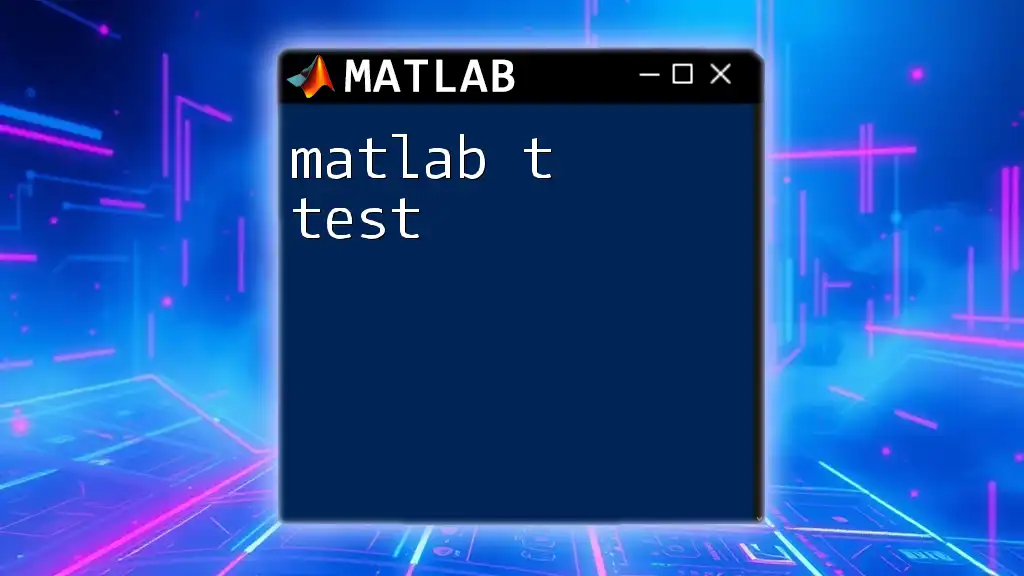
Conclusion
Mastering MATLAB data types is not merely about knowing the definitions; it's about effectively applying them in your projects to achieve the desired outcomes. As you familiarize yourself with these concepts, consider how they can facilitate your learning and enhance your programming capabilities in MATLAB.