MATLAB tables are a data type that allows you to store and manage heterogeneous data in a structured format, making it easier to work with variable names and access data efficiently.
% Creating a simple MATLAB table with sample data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [24; 30; 28];
Scores = [85.6; 90.2; 78.5];
T = table(Names, Ages, Scores);
disp(T);
What are MATLAB Tables?
MATLAB tables are a versatile data structure designed to manage heterogeneous data conveniently. Unlike traditional data types such as arrays or cell arrays, tables allow you to mix different data types in a single data set—such as numeric, text, and categorical data—while still providing meaningful labels for each column. This structure enables users to perform data analysis tasks more intuitively and efficiently.
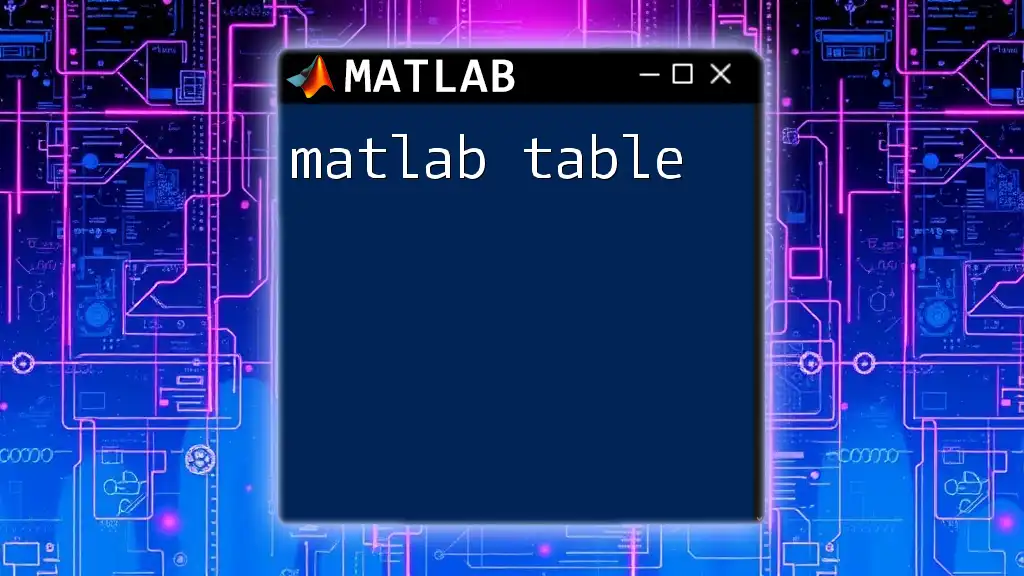
Why Use Tables in MATLAB?
Using tables in MATLAB has several advantages:
- Readability: Tables enhance data organization and readability. Each column can have a specific name, making it easier to understand the content at a glance.
- Integration: Tables allow for efficient integration with data visualization and analysis functions in MATLAB.
- Enhanced Data Management: Tables offer built-in support for metadata, such as row and column names, which simplifies data manipulation.
These features empower users to tackle complex data analysis tasks, from statistical analysis to machine learning.
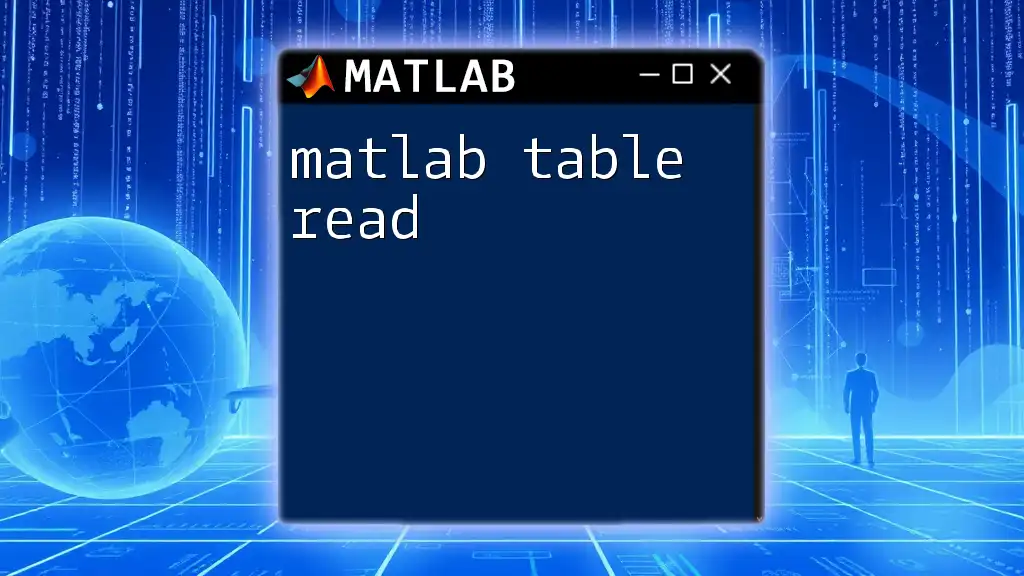
Creating MATLAB Tables
Creating a Table from Scratch
You can create a table from scratch by initializing it with data. MATLAB provides a straightforward syntax for defining tables.
For example, consider the following code snippet:
% Creating a simple table from arrays
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
tableData = table(Names, Ages);
In this example, we create a table named `tableData` that includes names and corresponding ages.
Creating a Table from Existing Data
Often, you may want to convert existing data into a table for further analysis. You can easily convert numerical arrays or cell arrays into tables.
Here's how to convert a matrix:
% Converting a matrix to a table
dataMatrix = [1:5; 6:10]';
dataTable = array2table(dataMatrix, 'VariableNames', {'Col1', 'Col2'});
In this snippet, a 2-column matrix is converted into a table `dataTable`, and we specify variable names for ease of access.
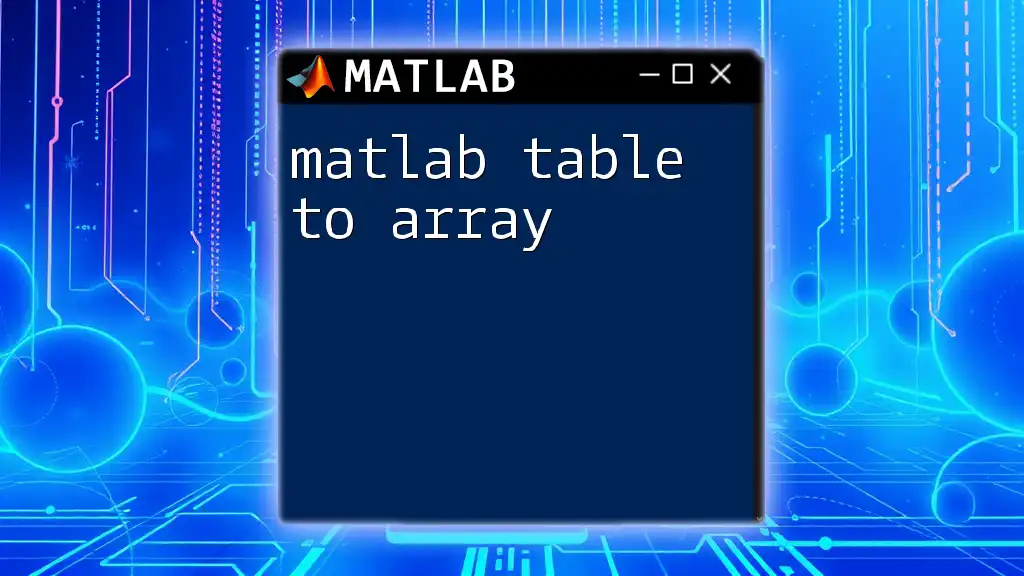
Accessing and Modifying Table Data
Accessing Table Variables
You can access specific variables (columns) in a table by using the dot notation. This feature allows for concise and intuitive data retrieval.
For example:
% Accessing a specific column
agesColumn = tableData.Ages;
Here, `agesColumn` will store all the ages from `tableData`.
Modifying Table Data
MATLAB allows you to update or change data within a table effortlessly. Let’s change Alice's age:
% Updating a specific entry
tableData.Ages(1) = 26; % Changes Alice's age
With this command, we set the first row in the `Ages` column to 26, reflecting Alice’s updated age.
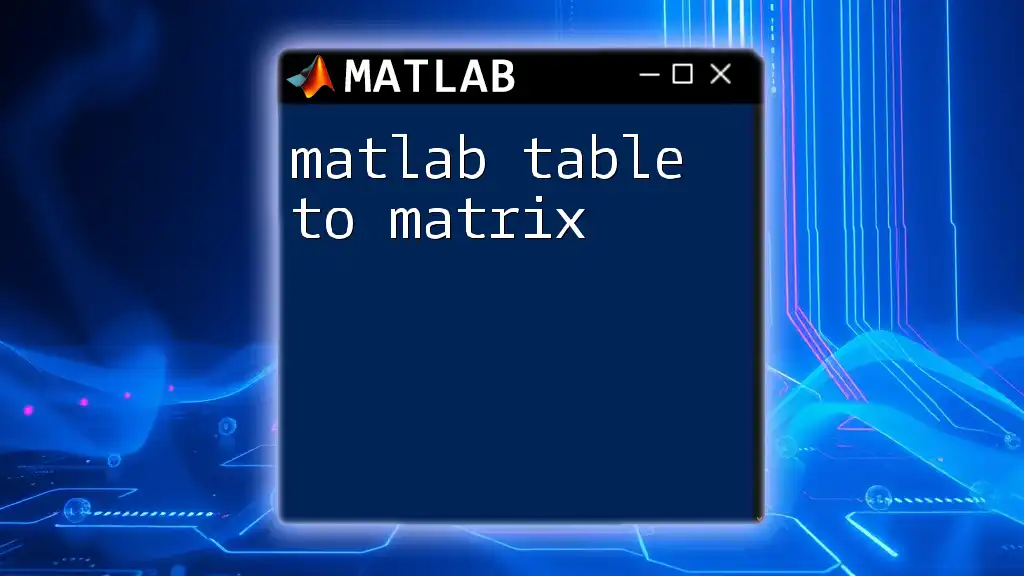
Table Properties
Understanding Table Properties
MATLAB tables come with many built-in properties that enhance their functionality. Properties like `VariableNames` and `RowNames` facilitate easier data management.
Retrieve the variable names from a table:
disp(tableData.Properties.VariableNames); % Display variable names
This will output the names of the columns in the table, improving your understanding of the contained data.
Assigning Row Names
You can also assign meaningful names to the rows of your table, further enhancing its readability:
tableData.Properties.RowNames = {'Row1', 'Row2', 'Row3'};
This command labels the rows of `tableData`, helping you keep track of individual entries.
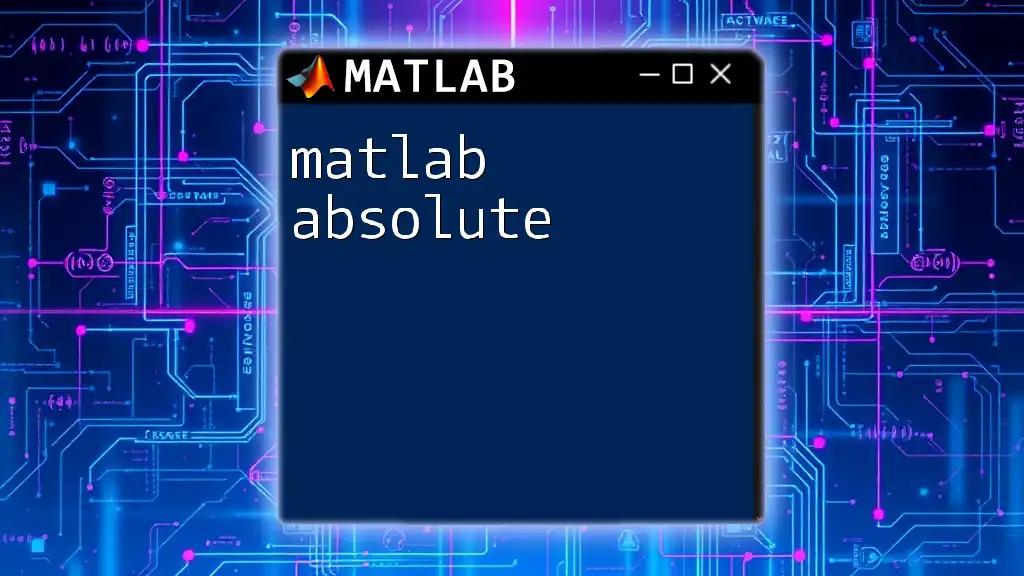
Performing Operations on Tables
Summarizing Data
MATLAB provides numerous built-in functions that allow you to perform statistical summaries directly on table data:
% Calculating the mean of ages
meanAge = mean(tableData.Ages);
In this example, we compute the mean age of all entries in the `tableData`.
Sorting Tables
Sorting enables you to organize tables based on the values within specific columns, enhancing data exploration:
% Sorting the table by age
sortedTable = sortrows(tableData, 'Ages');
This command sorts `tableData` by the `Ages` column in ascending order, putting the youngest individuals first.
Joining Tables
Joining tables is a common operation that allows for the integration of related datasets. Suppose we have another table with scores:
% Joining two tables
otherTable = table({'Alice'; 'Bob'}, [100; 200], 'VariableNames', {'Names', 'Scores'});
combinedTable = outerjoin(tableData, otherTable, 'Keys', 'Names', 'MergeKeys', true);
This example illustrates how to combine `tableData` with `otherTable` based on the `Names` column, helping you consolidate dispensed information.
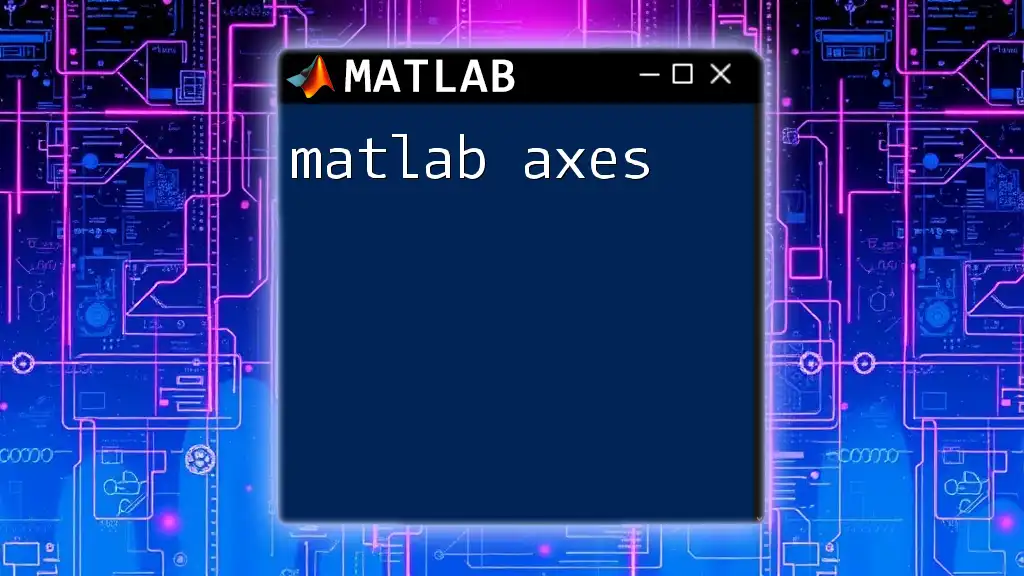
Advanced Operations with Tables
Table Indexing
You can use advanced indexing techniques to access or manipulate subsets of your table data. For example, logical indexing can filter rows based on specific criteria:
% Filtering rows based on condition
youngAdults = tableData(tableData.Ages < 30, :);
In this case, `youngAdults` stores all rows where the individuals are younger than 30.
Dealing with Missing Data
Handling missing data is critical in data analysis. MATLAB provides tools to manage NaN entries in your tables effectively. To remove rows with missing data, use the `rmmissing` function:
% Removing rows with missing data
cleanTable = rmmissing(tableData);
This command creates a new table, `cleanTable`, that contains only rows with complete data, omitting any that have NaN values.
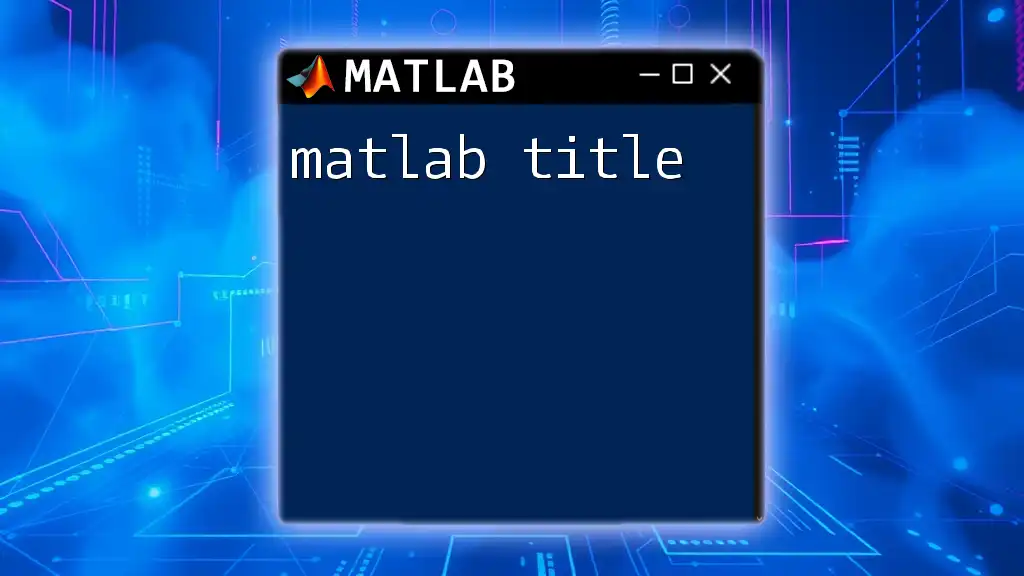
Visualizing Data in Tables
Visualization is essential for data interpretation. You can create plots directly from tables:
% Plotting ages
bar(tableData.Ages);
title('Ages Distribution');
This code generates a bar graph representing the age distributions within your `tableData`, making it easy to understand trends visually.
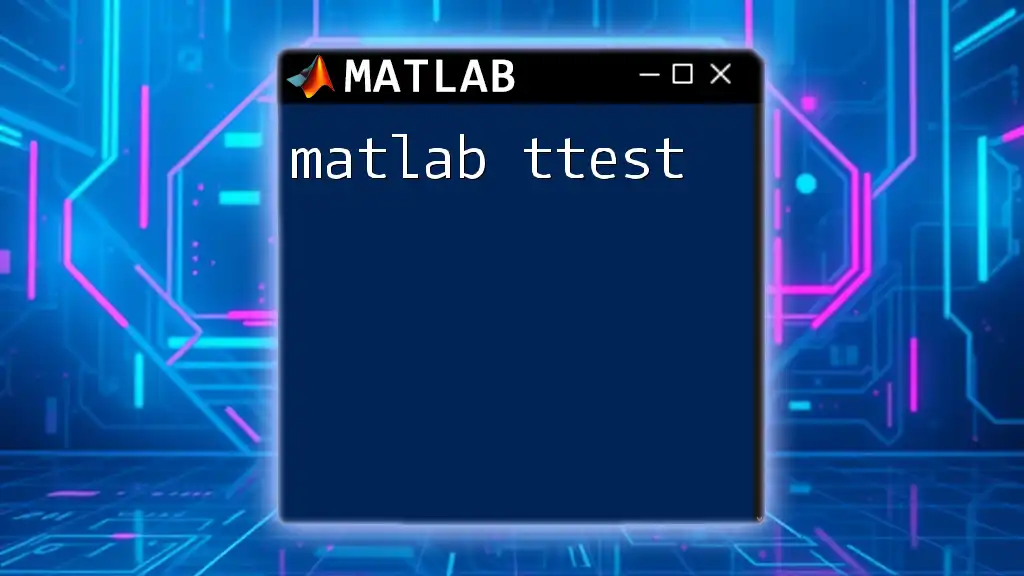
Exporting and Importing Tables
Saving Tables to Files
You can export tables to CSV or Excel files with a few simple commands. For instance, to save a table as a CSV:
% Exporting a table to CSV
writetable(tableData, 'myTable.csv');
By executing this command, `tableData` will be saved as a .csv file named "myTable.csv", which can be easily shared or analyzed in other applications.
Importing Tables from Files
Likewise, you can read table data from external files, allowing for easy integration of external datasets.
For example, to import table data from a CSV file:
% Importing table from CSV
importedTable = readtable('myTable.csv');
This command loads the content of "myTable.csv" into a new table named `importedTable`, ready for analysis.
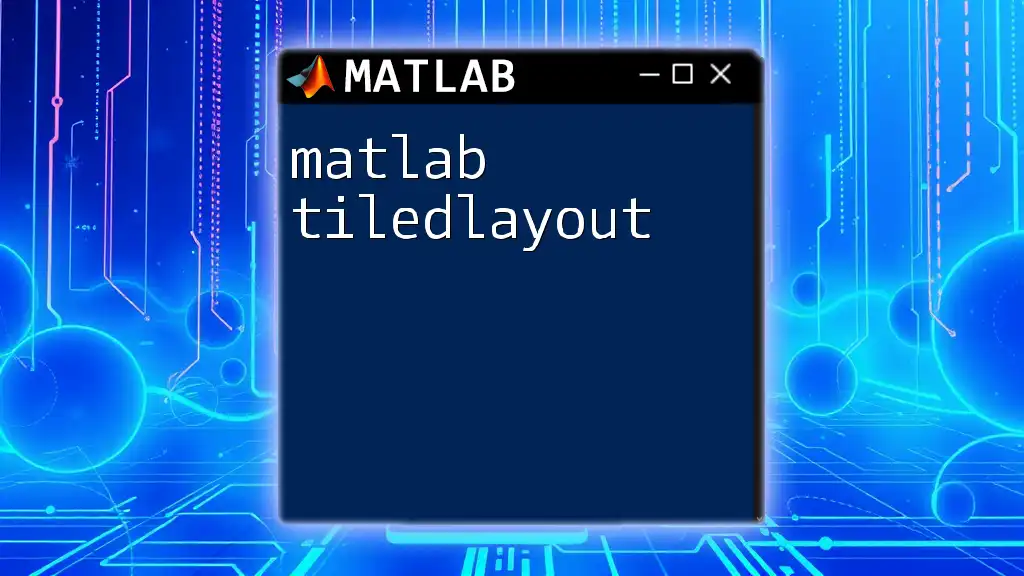
Recap of Key Points
Throughout this guide, we've explored the critical aspects of MATLAB tables, from creation and manipulation to advanced operations and data visualization. Understanding how to utilize tables effectively is essential for successful data analysis in MATLAB.
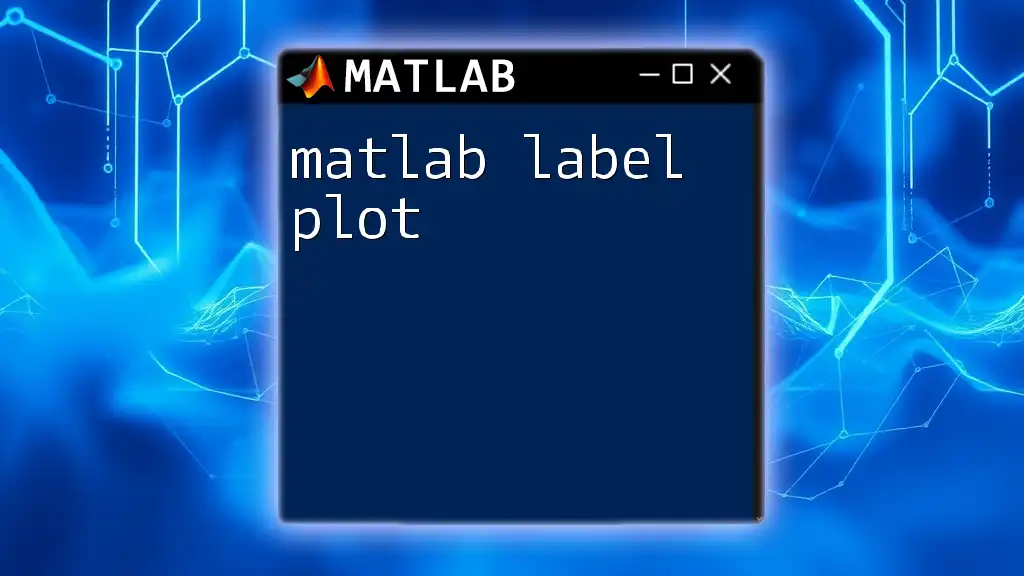
Encourage Experimentation
We encourage you to experiment with creating and manipulating tables in your projects. The flexibility and power of MATLAB tables can drastically improve your data analysis capabilities. Dive in, explore the functionalities, and take your data management skills to the next level!
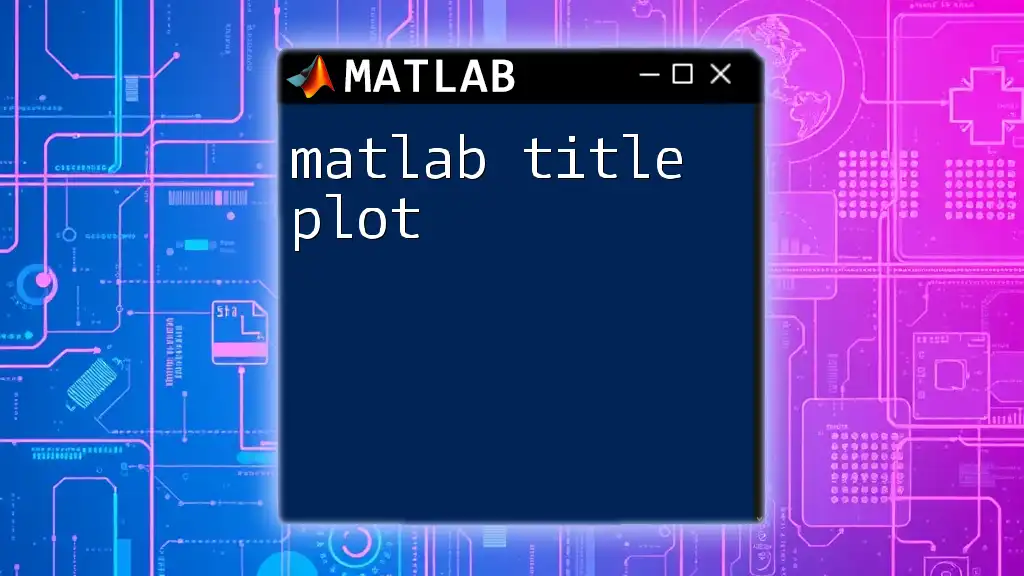
Additional Resources
To further enhance your MATLAB skills, consult the official [MATLAB Documentation](https://www.mathworks.com/help/matlab/table.html) on tables for comprehensive information and examples. You may also find numerous tutorials and books that dive deeper into the subject, offering new insights and advanced techniques.