A MATLAB table is a data type that provides a convenient way to store and manage heterogeneous data in a structured format, allowing easy access to both rows and columns.
Here’s a quick example of how to create a table in MATLAB:
% Create a sample table with two columns: Name and Age
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
T = table(Names, Ages);
disp(T);
Understanding MATLAB Tables
What are MATLAB Tables?
MATLAB tables are a powerful data structure designed to hold tabular data, allowing users to store and manipulate different types of data in a single variable. Unlike traditional arrays, where each element must be of the same type, MATLAB tables can contain heterogeneous data types. For instance, you can have a column of numeric values and another column of text, all within the same table. This makes tables particularly useful for statistical analysis and data exploration.
Key Features of MATLAB Tables
Tables come with a host of features that enhance data handling:
- Named Variables: Each column in a table can have its own name, making it easier to reference data effectively. This feature is particularly beneficial in large datasets where remembering variable indices can be cumbersome.
- Row Access: You can access data by referencing specific rows, which simplifies data filtering and selection.
- Flexible Data Manipulation: Tables allow for various operations, including sorting, filtering, joining, and merging, providing robust functionality for data analysis.
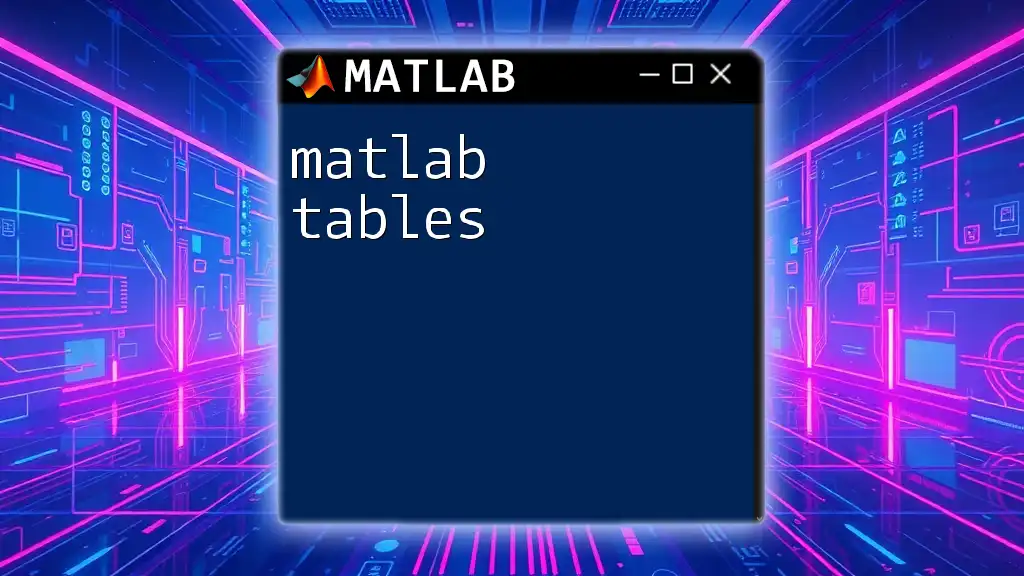
Creating Tables in MATLAB
Creating a Table from Scratch
Creating a table in MATLAB is straightforward. You can use the `table` function, which allows you to construct a table from existing variables.
The basic syntax is:
T = table(column1, column2, ..., 'VariableNames', {'name1', 'name2', ...});
Example:
Let's say you want to create a table with the age and height of several individuals:
Age = [23; 34; 45];
Height = [5.5; 6.2; 5.9];
T = table(Age, Height, 'VariableNames', {'Age', 'Height'});
In this example, we created a table `T` with two columns: Age and Height, clearly labeled for easy reference.
Loading Data into a Table
In real-world scenarios, you often need to work with existing datasets. MATLAB simplifies this with functions like `readtable`, which allows you to load data from sources like Excel or CSV files directly into a table.
For example, you can load data from a CSV file as follows:
T = readtable('data.csv');
This command reads the contents of 'data.csv' and creates a MATLAB table `T` using the column headers from the file as variable names.
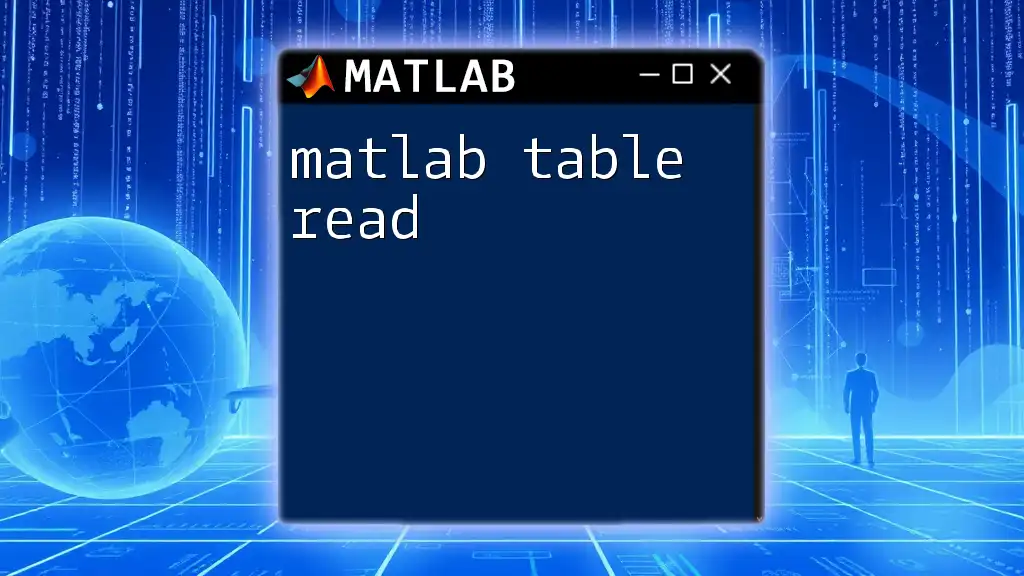
Accessing and Modifying Table Data
Accessing Data in Tables
Accessing data in MATLAB tables is efficient and intuitive.
Accessing Entire Variables
You can access entire variables using dot notation, which clearly identifies the variable name:
ageData = T.Age;
Accessing Rows and Columns
To access specific rows or subsets of the table, you can use indexing. For example, to retrieve the first row of the table:
firstRow = T(1, :);
If you want to access a subset of rows and specific columns:
subset = T(1:2, {'Age', 'Height'});
Modifying Values in Tables
Tables are flexible and allow you to modify their contents easily. You can add new rows and columns or remove existing ones.
Adding New Rows and Columns
For example, to add a new column that captures weight data:
T.Weight = [150; 180; 160];
You can also add new rows using the `end` keyword to append data:
newRow = {50, 5.7, 165}; % Assume columns are Age, Height, Weight
T(end + 1, :) = newRow;
Removing Rows and Columns
Removing data points is just as easy. To remove a column from your table, you can simply set it to an empty array:
T.Height = [];
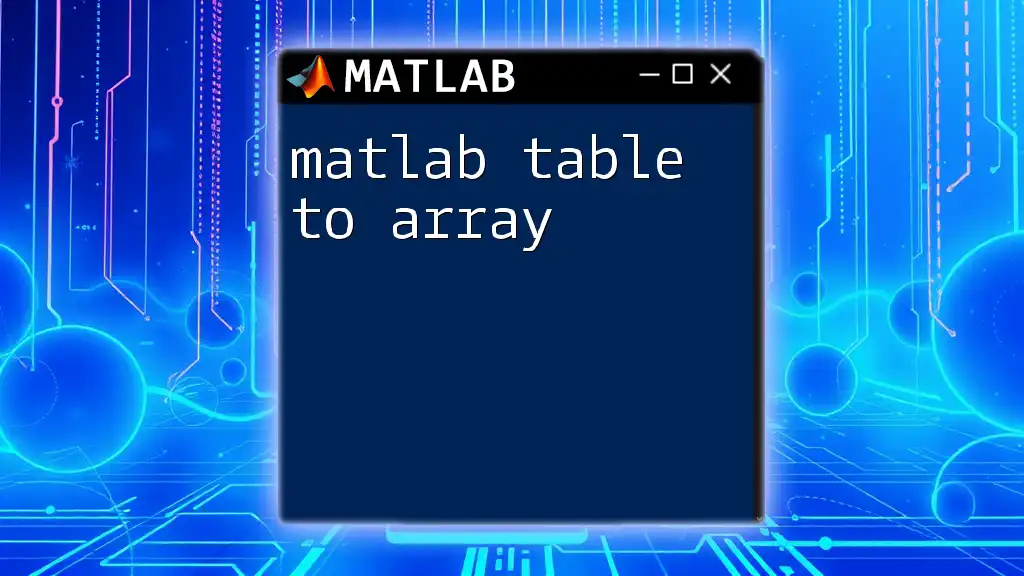
Sorting and Filtering Tables
Sorting Data
Sorting data is a common task in data analysis. The `sortrows` function allows you to order the rows of a table based on the values in specific columns.
T_sorted = sortrows(T, 'Age');
In this example, `T` is sorted by the Age column, producing a new table `T_sorted`.
Filtering Data
Filtering uses logical indexing to isolate specific rows based on conditions. For example, to find individuals older than 30:
filtered = T(T.Age > 30, :);
This command creates a new table with only those rows where the age exceeds 30.
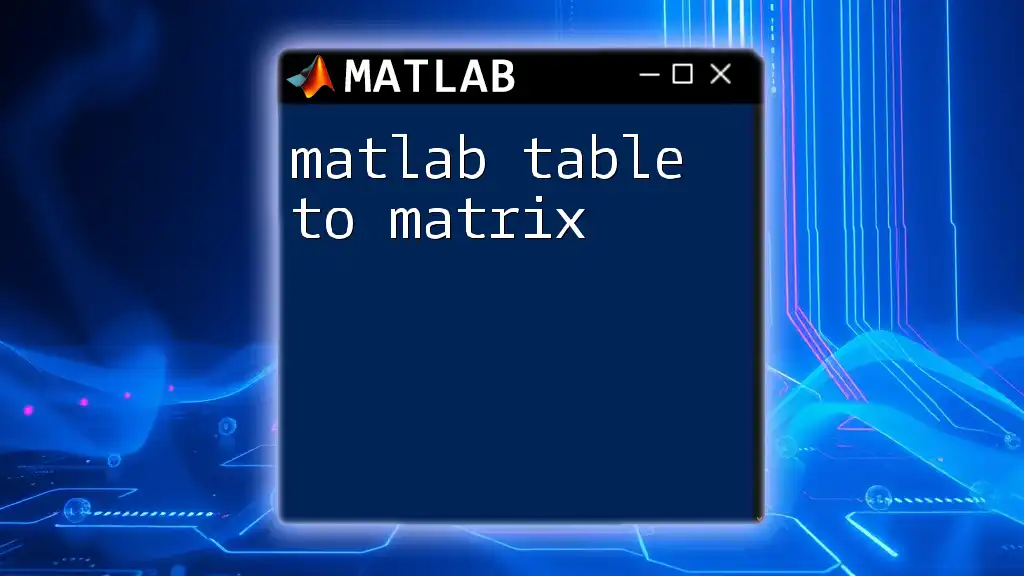
Useful Functions for Tables
Summary Statistics
To quickly obtain a summary of the contents of your table, you can use the `summary` function:
summary(T);
This function provides a quick overview of each variable, including data types and basic statistics.
Merging Tables
When working with multiple datasets, you may need to merge tables. Functions like `join` and `outerjoin` facilitate this process.
For instance, consider you have another table with group labels:
T2 = table([23; 34], ['A'; 'B'], 'VariableNames', {'Age', 'Group'});
T_merged = outerjoin(T, T2, 'MergeKeys', true);
This example merges `T` and `T2` while aligning the rows based on the Age variable.
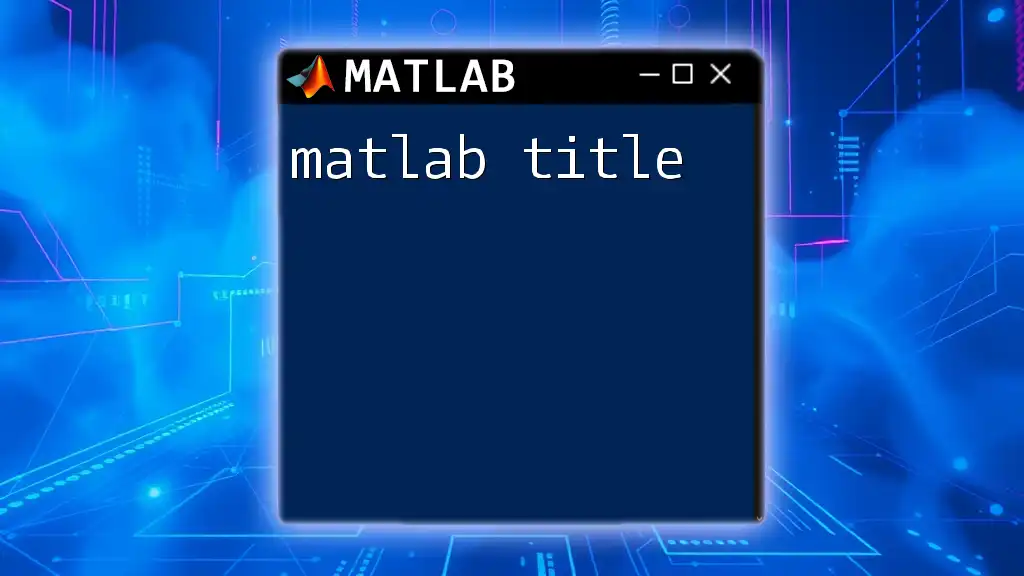
Visualization with Tables
Plotting Data from Tables
MATLAB's plotting capabilities work seamlessly with tables, making visual data exploration straightforward. For example, you can scatter plot Age vs. Height easily:
scatter(T.Age, T.Height);
xlabel('Age');
ylabel('Height');
title('Age vs Height');
This command generates a scatter plot, offering quick insights into the relationship between age and height.
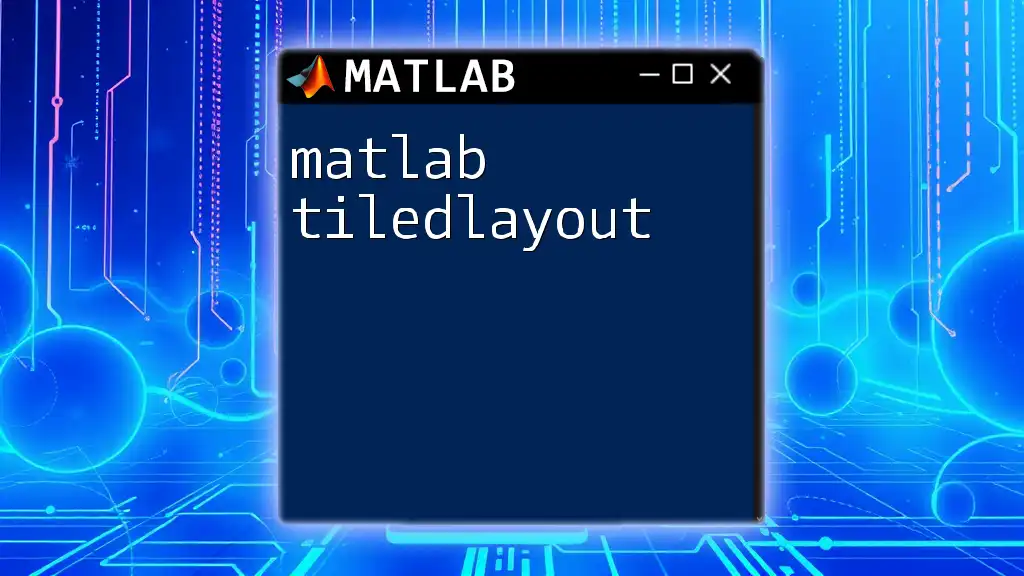
Conclusion
MATLAB tables are an invaluable resource for data analysis, facilitating the manipulation and visualization of complex datasets. From creating and modifying tables to filtering and merging data, tables provide an effective and flexible way to handle data. Experimenting with the capabilities of tables will broaden your skills and enhance your ability to analyze and visualize data. Be sure to explore advanced functionalities to leverage the full potential of MATLAB tables in your projects.
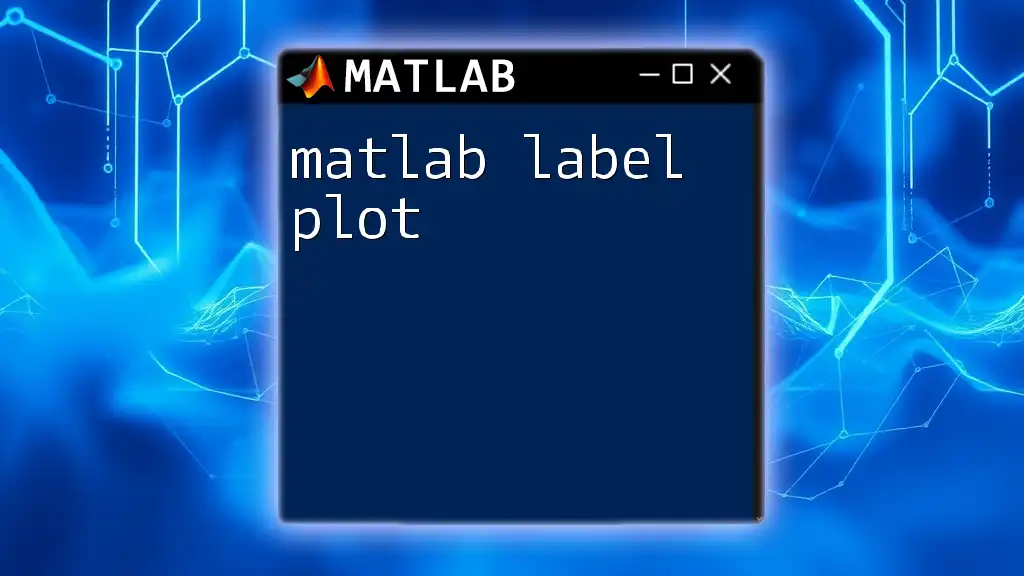
Additional Resources
If you’re looking for more information on MATLAB tables, check out the [MATLAB Table Documentation](https://www.mathworks.com/help/matlab/ref/table.html) and explore tutorials that further enhance your understanding of this powerful data structure.
FAQs
In this section, you'll find answers to common questions related to MATLAB tables. Feel free to reach out or explore further if you encounter challenges while working with tables.