The `atan2` function in MATLAB computes the four-quadrant inverse tangent of the quotient of its arguments, which is useful for determining the angle in radians between the positive x-axis and the point defined by the coordinates (y, x).
Here's an example of how to use it:
y = 3;
x = 4;
angle = atan2(y, x); % Returns the angle in radians
What is the atan2 Function?
The atan2 function in MATLAB calculates the four-quadrant inverse tangent of the quotient of its arguments. Unlike the traditional atan function that only returns values between -π/2 and π/2, atan2 considers the signs of both arguments to determine the correct quadrant for the angle. This is crucial when you're working with coordinates that can fall into any of the four quadrants of a Cartesian plane.
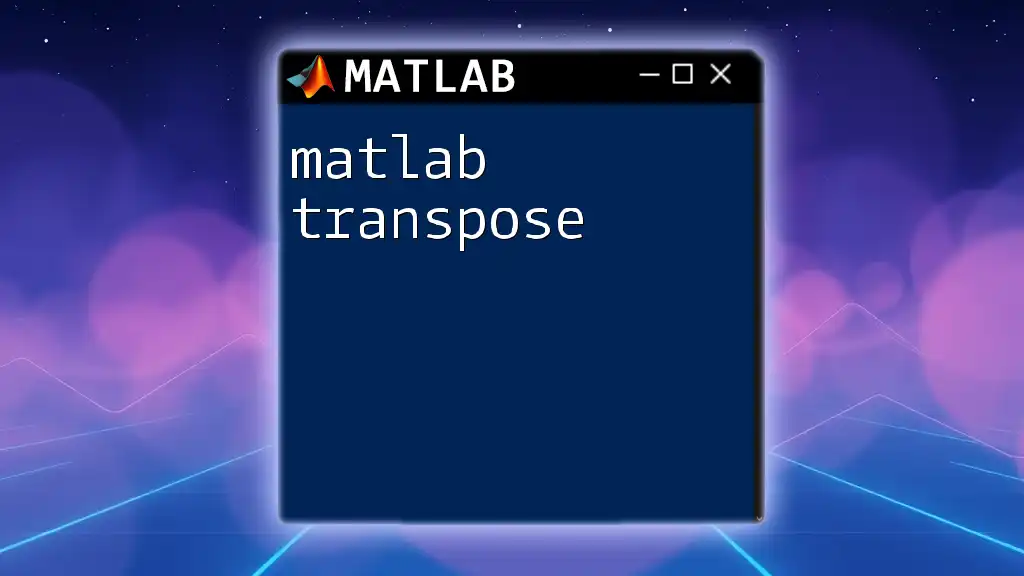
Why Use atan2?
Using atan2 eliminates ambiguity when determining an angle based on Cartesian coordinates. For instance, an angle with the same tangent can be represented in two different quadrants. By using atan2, which takes both the y (ordinate) and x (abscissa) coordinates, you obtain a unique angle that accurately reflects the position in the plane.
This function is particularly useful in various fields such as:
- Robotics – for determining the direction a robot should face relative to a target.
- Engineering – when analyzing forces and vectors acting in different dimensions.
- Computer Graphics – while calculating angles for rotations or direction vectors.
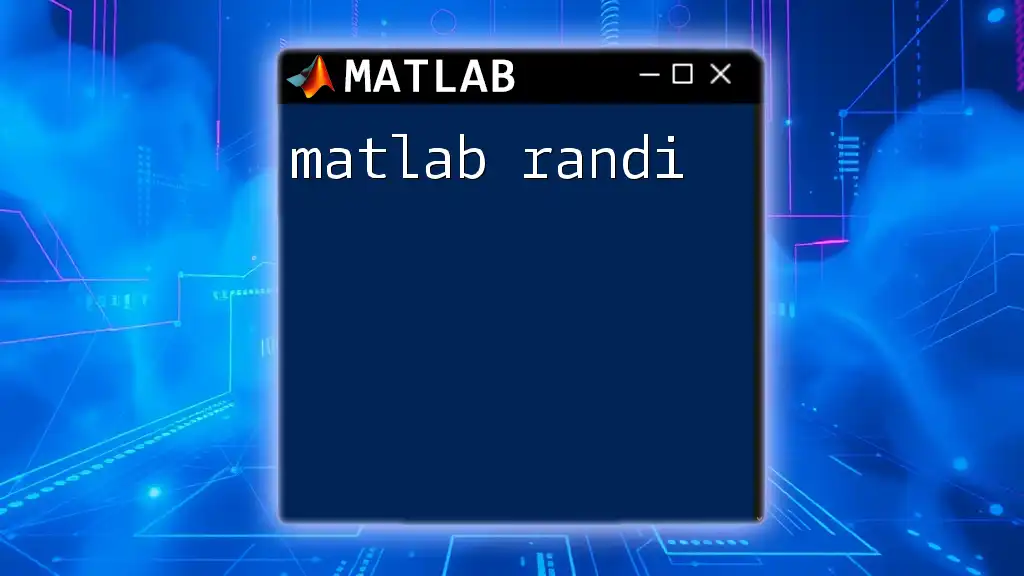
Syntax of atan2
Basic Syntax Structure
The syntax for using atan2 in MATLAB is quite straightforward:
angle = atan2(y, x);
Here, y is the value of the vertical coordinate, and x is the value of the horizontal coordinate. This function will then return the angle (in radians) between the positive x-axis of the Cartesian plane and the point (x,y).
Understanding Input Parameters
When utilizing atan2, you need to understand the role of the input parameters:
- y: This is the vertical coordinate of the point. It can be any real number (positive, negative, or zero).
- x: This is the horizontal coordinate. Just like y, it can also be any real number.
Selecting the appropriate parameters is essential. For instance:
- If given a point (3, 4), you would call `atan2(4, 3)` to determine the angle.
- If the point were (-3, -4), you would call `atan2(-4, -3)`.
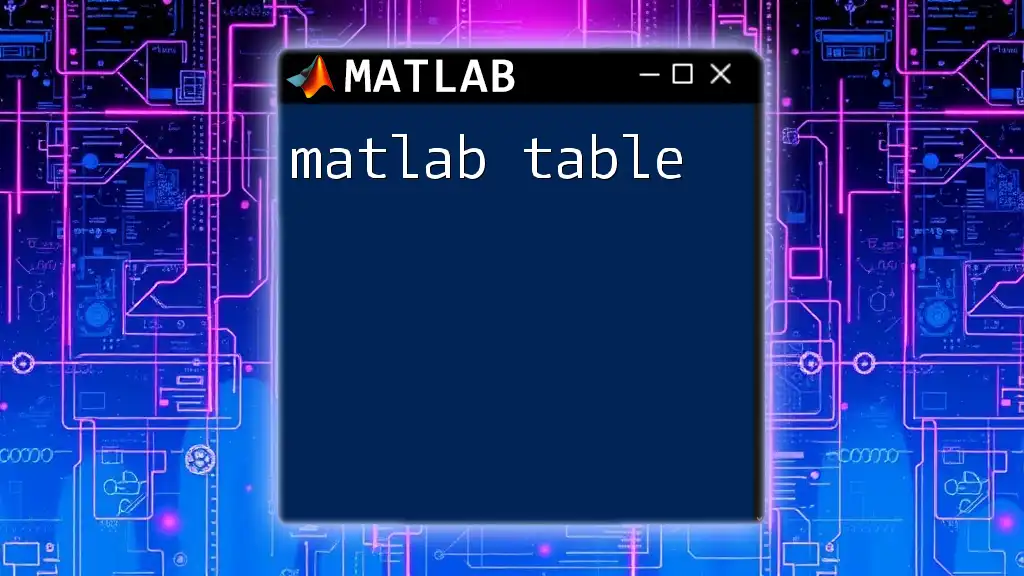
Understanding Output of atan2
Range of Output Angles
The output of atan2 is expressed in radians, with a range from -π to π. This means it can represent angles as follows:
- Quadrant I (0 to π/2): Both x and y are positive.
- Quadrant II (π/2 to π): x is negative, y is positive.
- Quadrant III (-π to -π/2): Both x and y are negative.
- Quadrant IV (-π/2 to 0): x is positive, y is negative.
Understanding this range is pivotal because it helps in visualizing the solution in a real-world context, such as directional angles for navigation.
Output Interpretation
Interpreting the output from atan2 is crucial to applying it practically. The produced angle indicates the direction you’d need to rotate from the positive x-axis to reach the point represented by (x,y). For instance, an output of 0 indicates that the point lies directly along the positive x-axis, whereas π indicates it's pointing directly left.
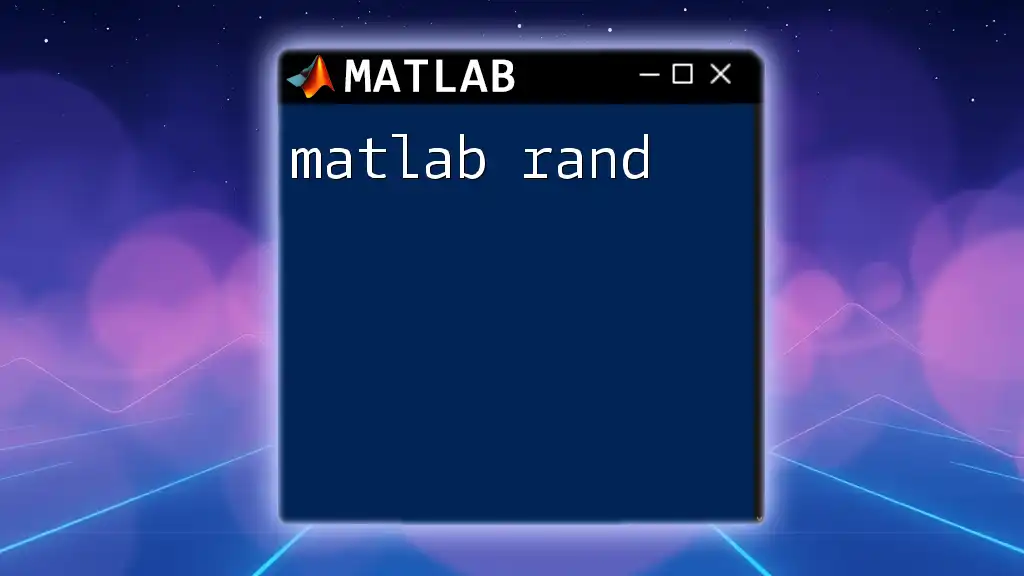
Practical Examples of Using atan2
Example 1: Basic Usage of atan2
Here's how you can use atan2 in a simple scenario:
y = 1;
x = 1;
angle = atan2(y, x);
disp(angle); % Output: 0.7854 (π/4 radians)
In this example, since both x and y are positive, the angle returned is in Quadrant I, representing 45 degrees or π/4 radians.
Example 2: Handling Negative Values
It's also essential to see how atan2 manages negative values:
y = -1;
x = 1;
angle = atan2(y, x);
disp(angle); % Output: -0.7854 (-π/4 radians)
Here, with a negative y-value and positive x, the function returns -π/4 radians, which is in Quadrant IV, confirming that the angle points downwards from the positive x-axis.
Example 3: Quadrant Analysis
Understanding how points in different quadrants influence the angle is essential. Consider the following:
points = [1, 1; -1, 1; -1, -1; 1, -1];
angles = atan2(points(:,2), points(:,1));
disp(angles); % Output corresponding to each point’s quadrant
Running this code calculates angles for points in all four quadrants, showcasing how the coordinates affect the angle outputs distinctly.
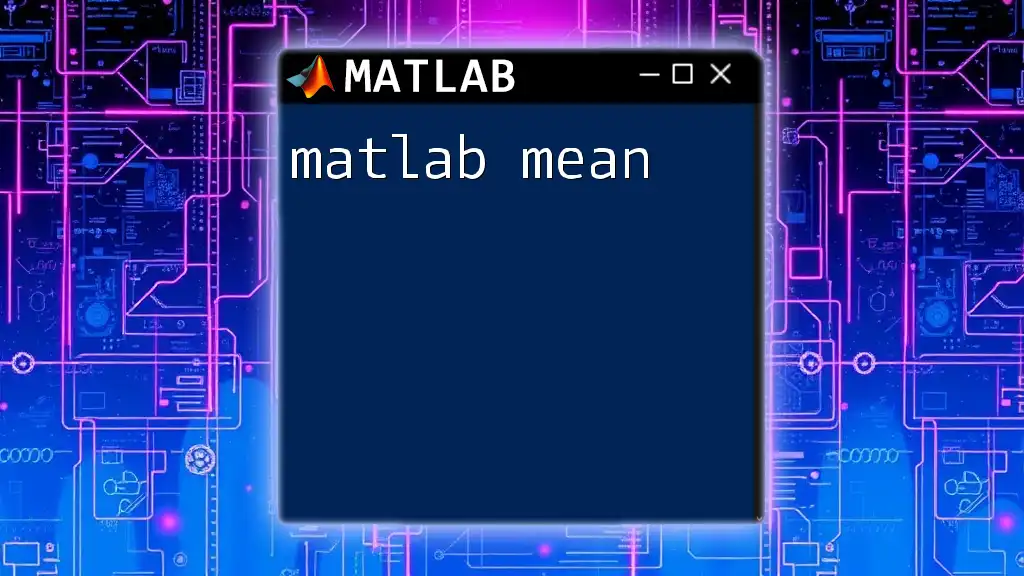
Applications of atan2 in Real-World Scenarios
Using atan2 in Robotics
In robotics, atan2 plays a critical role in navigation algorithms. For example, a robot that needs to turn towards a target based on its current position can effectively use atan2 to calculate the correct angle to turn:
robot_position = [2, 3];
target_position = [5, 7];
delta_y = target_position(2) - robot_position(2);
delta_x = target_position(1) - robot_position(1);
angle_to_target = atan2(delta_y, delta_x);
disp(angle_to_target); % Will give the angle to turn towards the target
Applications in Data Science and Machine Learning
In data science, using atan2 facilitates feature extraction from datasets that include spatial coordinates. By employing atan2, one can derive angular features to enhance predictive modeling.
For instance, when processing multi-dimensional data that includes locations, you can compute angles between various points to classify data accurately.
% Example of converting x and y coordinates to angles for clustering
x = rand(10,1); % Random x-coordinates
y = rand(10,1); % Random y-coordinates
angles = atan2(y, x);
disp(angles); % Angular representation of each point
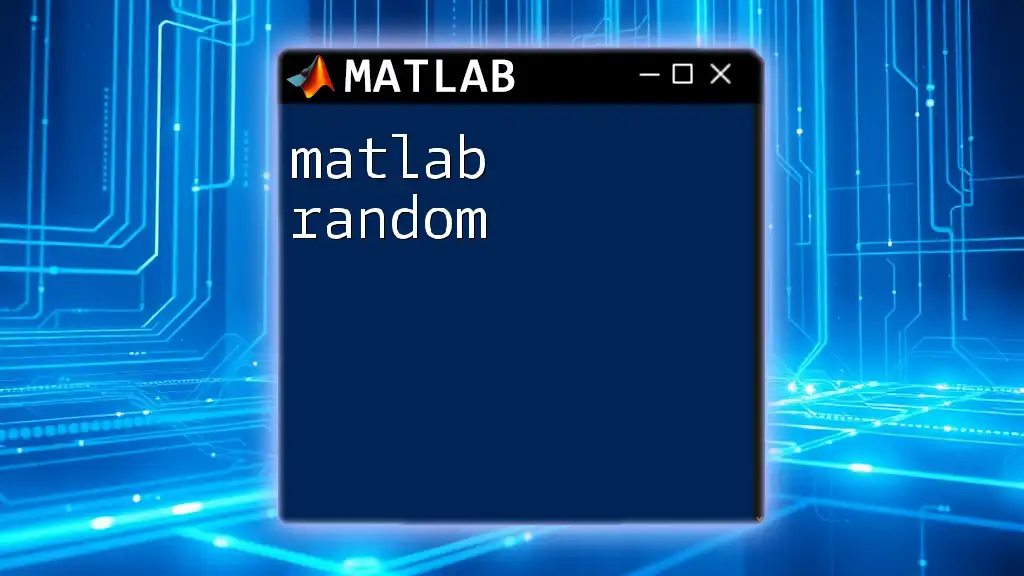
Best Practices for Using atan2
Selecting Coordinate Systems
When applying atan2, carefully choose your coordinate system based on the problem at hand. Cartesian coordinates are typically most straightforward, but polar coordinates can also be viable depending on the context of your application.
Handling Edge Cases
Be aware of potential edge cases when using atan2. For example, if both x and y are 0, atan2(0, 0) is undefined. Incorporating a check for these edge cases ensures your application remains robust:
if x == 0 && y == 0
error('Undefined angle for the point (0,0)');
end
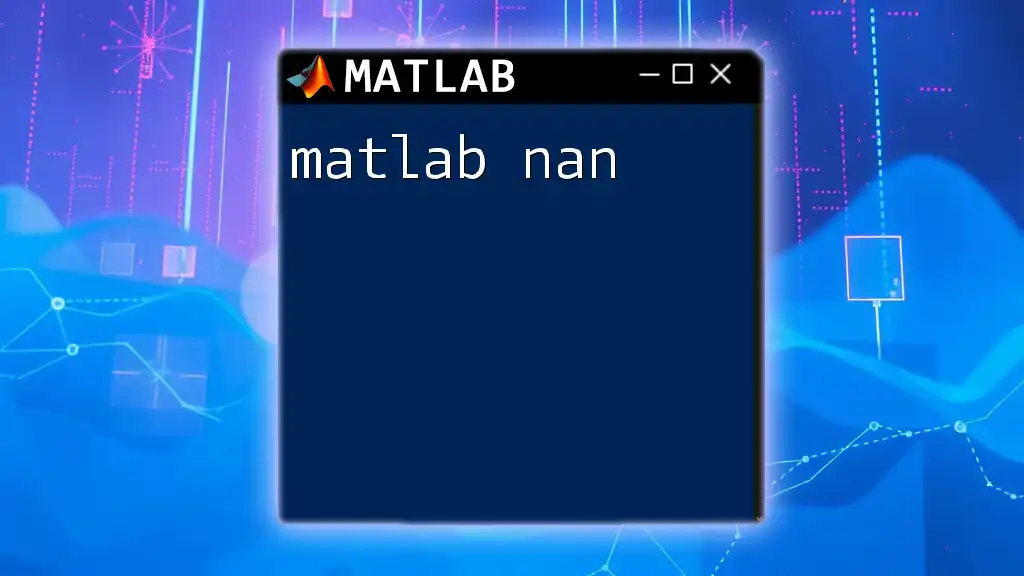
FAQs about atan2 in MATLAB
Common Questions and Answers
What happens when x = 0?
- When x is zero, atan2(y, 0) will return feedback based on the value of y. If y is positive, the result will be π/2. If y is negative, the result will be -π/2. If both are zero, it's undefined.
How to convert radians to degrees after using atan2?
- You can easily convert radians to degrees using the formula:
degrees = rad2deg(angle);
Can atan2 handle vectors?
- Yes, atan2 can process vectors. You can pass arrays of x and y coordinates, and it will return an array of angles.
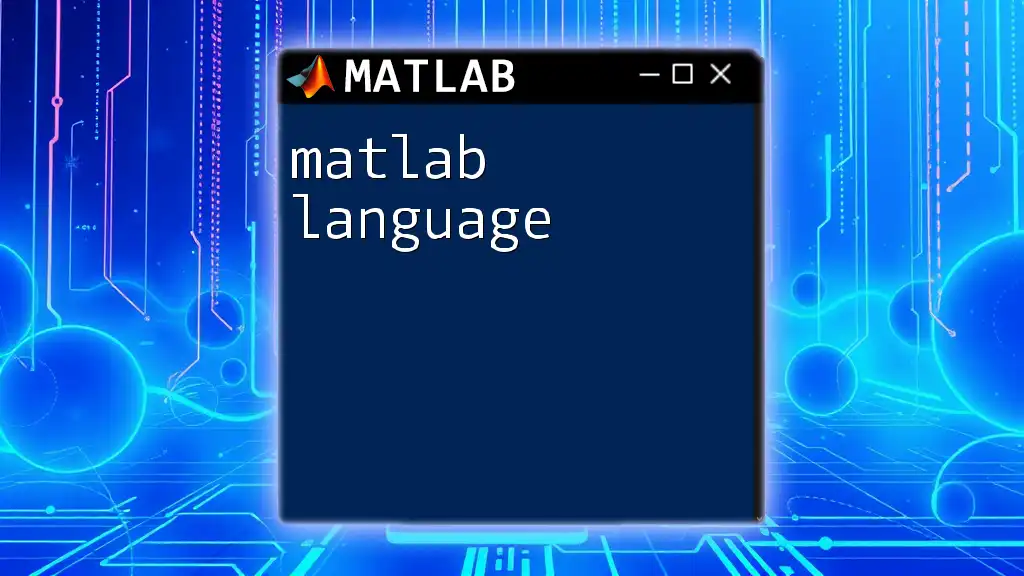
Recap of Key Points
In conclusion, the matlab atan2 function is a powerful tool for computing angles in a four-quadrant system. It eliminates ambiguity and allows for straightforward evaluations in various fields, from robotics to data analysis. Understanding its syntax, output, and practical applications can significantly enhance your effectiveness when using MATLAB for projects that require precision in angular calculations.
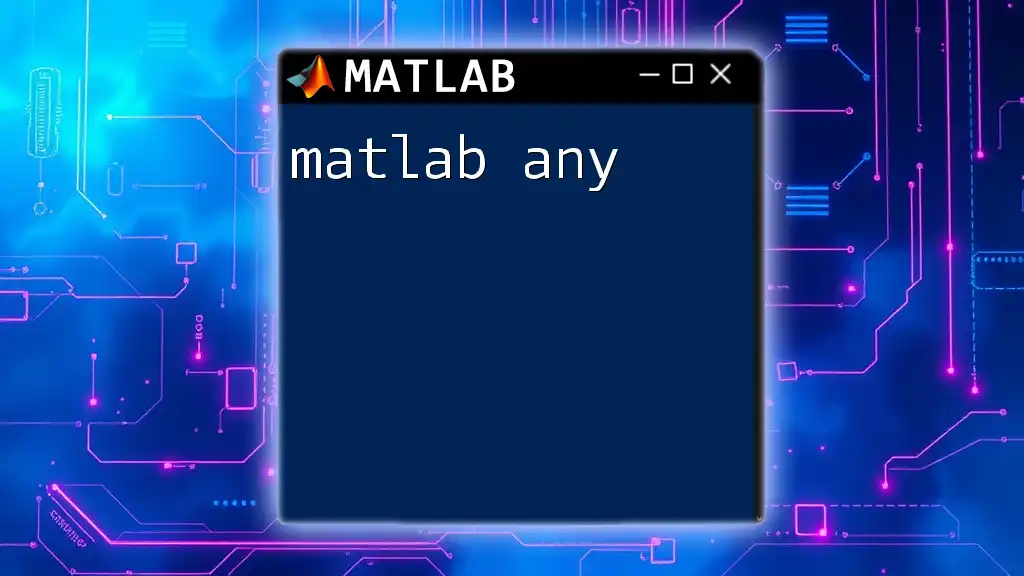
Encouragement to Experiment with Examples
Now that you know about the atan2 function, I encourage you to experiment with different inputs in MATLAB! Creating your scenarios will deepen your understanding and provide practical experience that is invaluable in applying this function in real-world projects.