A MATLAB dictionary (or "containers.Map") is a data structure that allows you to store key-value pairs, providing an efficient way to look up values by their corresponding keys.
% Create a dictionary with keys as strings and values as integers
dict = containers.Map({'apple', 'banana', 'cherry'}, [1, 2, 3]);
% Accessing a value using a key
value = dict('banana'); % Returns 2
Understanding MATLAB Dict
What is MATLAB Dict?
A MATLAB Dict (dictionary) is a powerful data structure in MATLAB that allows you to store data in key-value pairs. This organization differs from traditional arrays or cell arrays, as it offers a more flexible way to manage data. In a dictionary, each unique key is associated with a specific value, enabling efficient data retrieval and storage.
The dictionary is part of the `containers.Map` class in MATLAB, providing functions to create, store, and manipulate the associated data effectively.
Benefits of Using MATLAB Dict
Utilizing a MATLAB Dict has numerous advantages:
- Flexibility in Data Representation: You can hold different data types for values, including strings, numbers, arrays, and even other dictionaries.
- Efficient Data Retrieval and Storage: Key-value pairs streamline data access, allowing for faster lookups compared to traditional indexing.
- Organizing Complex Datasets: When handling large datasets or configurations, a dictionary organizes your data logically, making it easier to manage.
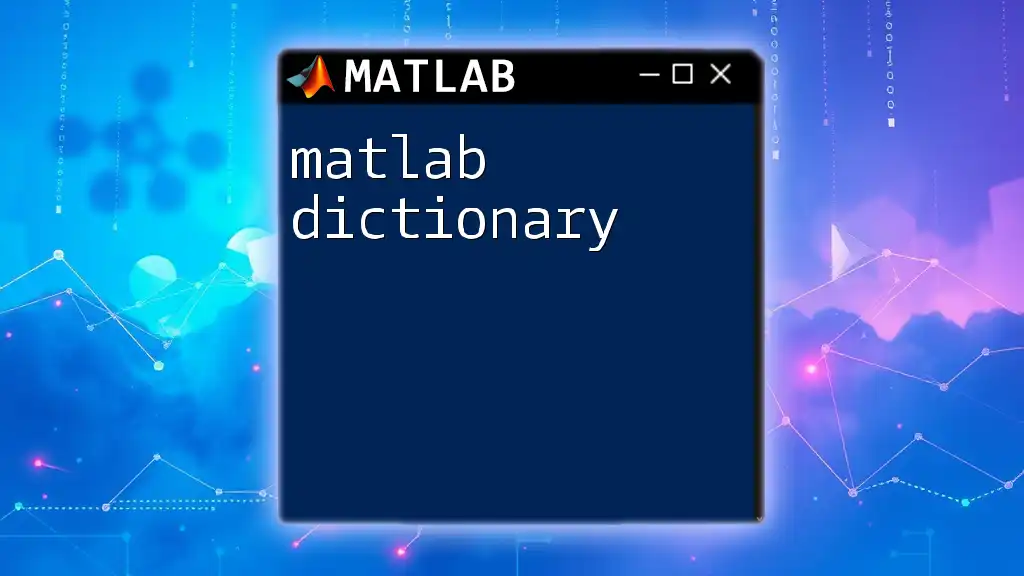
How to Create a MATLAB Dict
Basic Syntax for Creating a Dict
Creating a MATLAB Dict is straightforward. Use the `containers.Map` constructor to initiate a dictionary object:
myDict = containers.Map;
Populating the Dictionary
Adding Key-Value Pairs
You can populate your MATLAB Dict by adding key-value pairs. The syntax for adding pairs is quite intuitive:
myDict('key1') = 'value1';
myDict('key2') = 10;
In this example, we create two key-value pairs, where `'key1'` corresponds to the string `'value1'`, and `'key2'` corresponds to the number `10`.
Updating Existing Keys
If you need to modify a value associated with an existing key, you can simply reassign it:
myDict('key1') = 'updatedValue1';
This updates the value previously associated with `'key1'` to `'updatedValue1'`.
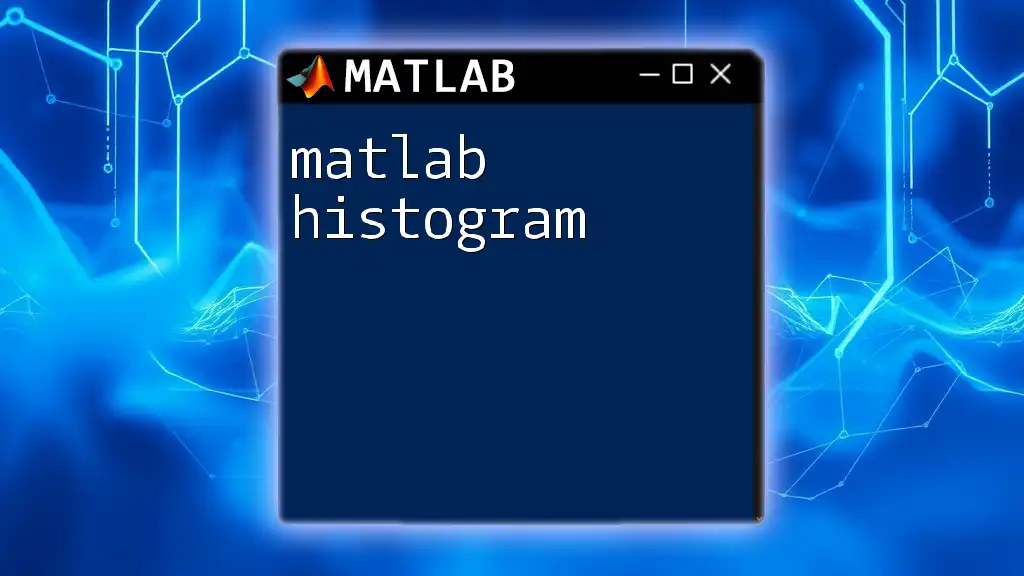
Accessing Dictionary Data
Retrieving Values Using Keys
Retrieving a value from a MATLAB Dict is fast and efficient. To fetch the value corresponding to a key, you use:
value = myDict('key1');
In this instance, `value` will be assigned the content associated with `'key1'`.
Handling Non-Existent Keys
When you attempt to access a value using a key that does not exist in the dictionary, MATLAB will throw an error. To prevent this, you can check if a key exists using the `isKey` function:
if isKey(myDict, 'key3')
disp(myDict('key3'));
else
disp('Key does not exist.');
end
This code snippet ensures that you only attempt to access keys that are present, thus avoiding runtime errors.
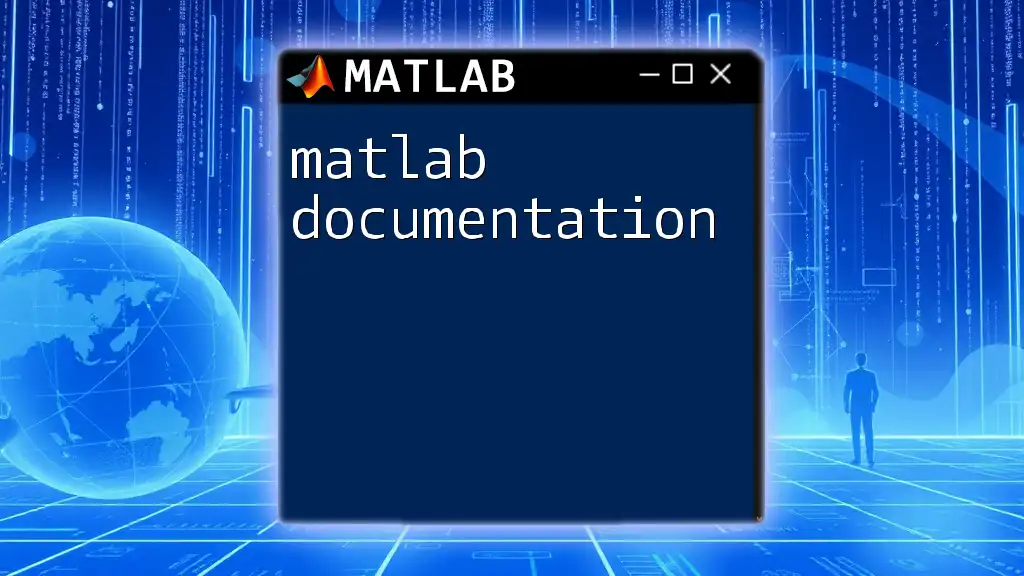
Advanced Features of MATLAB Dict
Iterating Over Keys and Values
Iterating through a MATLAB Dict is accomplished using the `keys` function to retrieve all keys. From there, you can access the values:
for k = keys(myDict)
disp([k{1}, ': ', myDict(k{1})]);
end
This loop displays all key-value pairs in the dictionary, helping you to explore its contents efficiently.
Importing Data into a Dictionary
Often, you may want to populate your MATLAB Dict from existing data structures. This can be done by directly assigning values from structures:
dataStruct = struct('name', 'Alice', 'age', 30);
myDict('name') = dataStruct.name;
myDict('age') = dataStruct.age;
Here, we create a structure with employee data and then store the values in the dictionary.
Exporting Data from a Dictionary
Conversely, if you need to convert the dictionary back into a more conventional format, you can extract keys and values as arrays:
keysList = keys(myDict);
valuesList = values(myDict);
Using these arrays allows for further manipulation or exporting to other formats as needed.
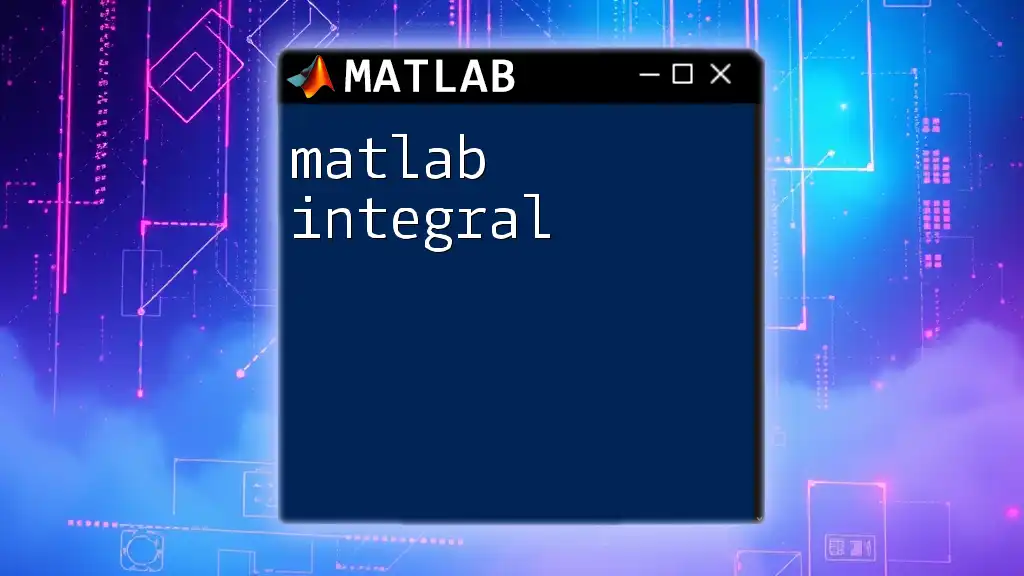
Practical Applications of MATLAB Dict
Case Study: Managing Employee Data
To illustrate the utility of a MATLAB Dict, consider a scenario where you want to manage employee data effectively. You can create a dictionary to hold names and corresponding IDs:
employeeDict = containers.Map({'ID1', 'ID2'}, {'Alice', 'Bob'});
With `employeeDict`, you can quickly search for an employee by ID and update their details as necessary.
Case Study: Configurations Storage
Another great application for MATLAB Dict is managing configuration settings in a project. For instance, storing application settings can be executed like this:
configDict = containers.Map({'SettingA', 'SettingB'}, {true, 'High'});
This methodology allows you to maintain configuration settings within an organized structure that’s easily accessible and modifiable.
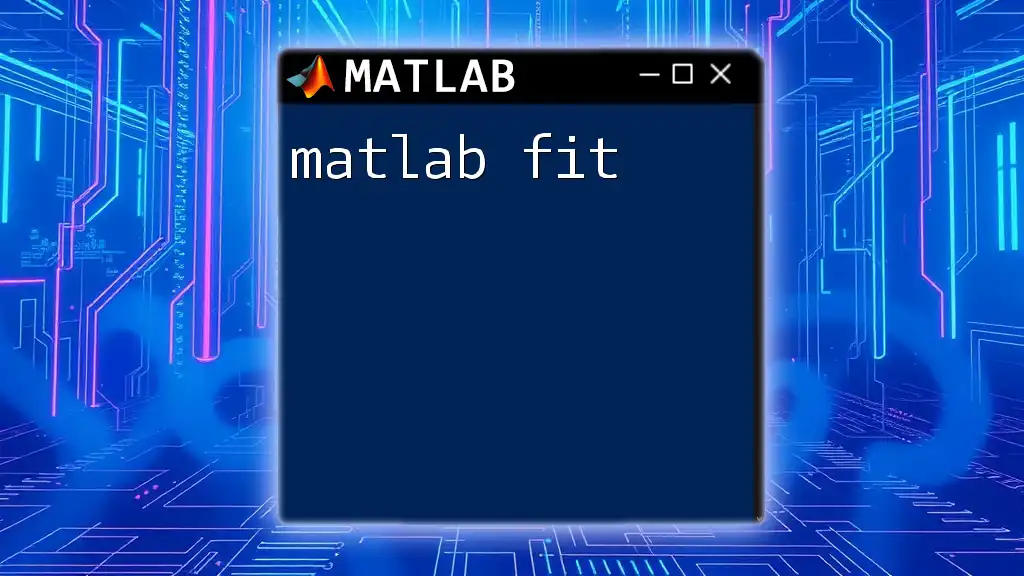
Common Pitfalls and Troubleshooting
Common Errors with MATLAB Dict
While working with MATLAB Dicts, certain errors may arise, such as attempting to access nonexistent keys. Ensure that you are checking key existence before retrieval using `isKey` to avoid these pitfalls.
Tips for Efficient Use of MATLAB Dict
To optimize the performance of your MATLAB Dict, follow these suggestions:
- Use meaningful key names that reflect the data they point to. This practice enhances readability.
- Keep data types consistent where possible to minimize the likelihood of errors during processing.
- Regularly clean up and check your dictionary to remove any obsolete keys.
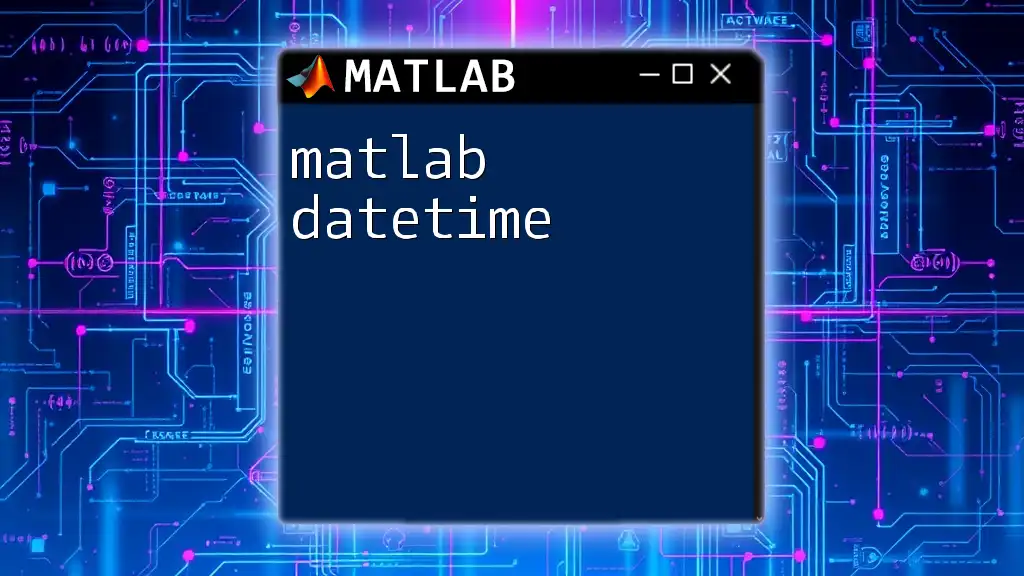
Conclusion
In summary, MATLAB Dict is an invaluable structure for anyone looking to manage data effectively in MATLAB. By storing data in key-value pairs, you can optimize how you handle complex datasets, improve data retrieval efficiency, and reduce the potential for errors. Dive into dictionaries and leverage their full potential in your MATLAB programming endeavors.