The MATLAB `filter` function applies a digital filter to a one-dimensional data sequence, allowing you to process signals or data arrays based on specified filter coefficients.
Here's an example of how to use the `filter` function in MATLAB:
% Example of a simple low-pass filter using the filter function
b = [1, 1]/2; % Numerator coefficients
a = 1; % Denominator coefficients
data = randn(1, 100); % Generate random data
filtered_data = filter(b, a, data); % Apply the filter
Understanding Filters
What is a Filter?
A filter is a mathematical function that processes a signal or dataset to remove unwanted components or enhance desired ones. They play a crucial role in various applications, such as audio processing, image processing, and data analysis. Filters are broadly classified into types based on their frequency response characteristics:
- Low-pass filters allow signals with frequencies lower than a certain threshold to pass through while attenuating higher frequencies.
- High-pass filters do the opposite, allowing high frequencies to pass while blocking lower frequencies.
- Band-pass filters permit a specific range of frequencies to pass and attenuate frequencies outside this range.
- Band-stop filters or notch filters reject frequencies within a specified range but allow others to pass.
In practical scenarios, filters can be found in signal detection, communications, and filtering noise from captured measurements.
Why Use Filters in MATLAB?
MATLAB provides a user-friendly environment with built-in functions that make implementing filters straightforward. The advantages of using filters in MATLAB include:
- Built-in Functions: MATLAB offers several predefined functions to design and apply both FIR (Finite Impulse Response) and IIR (Infinite Impulse Response) filters.
- Flexibility and Customization: Users can create customized filters to meet specific requirements, giving them full control over the filtering process.
- Real-World Applications: Filters are invaluable in various fields, including engineering (for signal processing), finance (for trend analysis), and medicine (for analyzing physiological data).
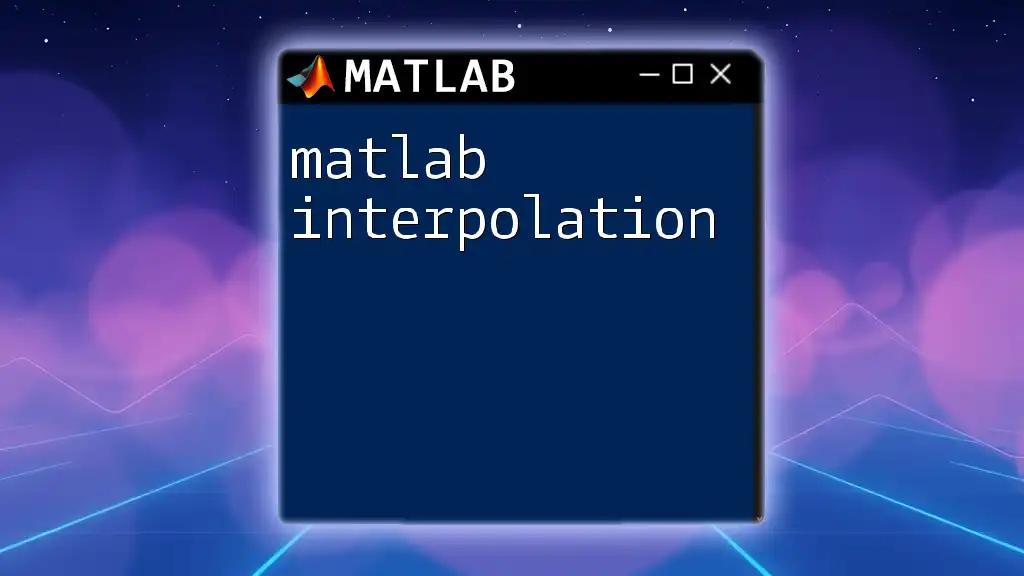
Getting Started with MATLAB Filters
Installing MATLAB
To start using the MATLAB filter commands, you first need to install MATLAB. Follow these steps for a seamless installation:
- Download MATLAB from the official MathWorks website.
- Follow the installation wizard, ensuring that all necessary toolboxes, particularly the Signal Processing Toolbox, are included.
- Activate your installation with your MathWorks account or license key.
First Steps in MATLAB
After installing MATLAB, it's essential to familiarize yourself with its basic commands and components:
-
Creating Arrays and Matrices: This is fundamental as filters operate on arrays. You can create simple arrays with commands like:
x = [1, 2, 3, 4, 5]; % A row vector y = [1; 2; 3; 4; 5]; % A column vector
-
Basic Commands: Knowing how to manipulate arrays, plot functions, and execute scripts is essential for filtering operations.
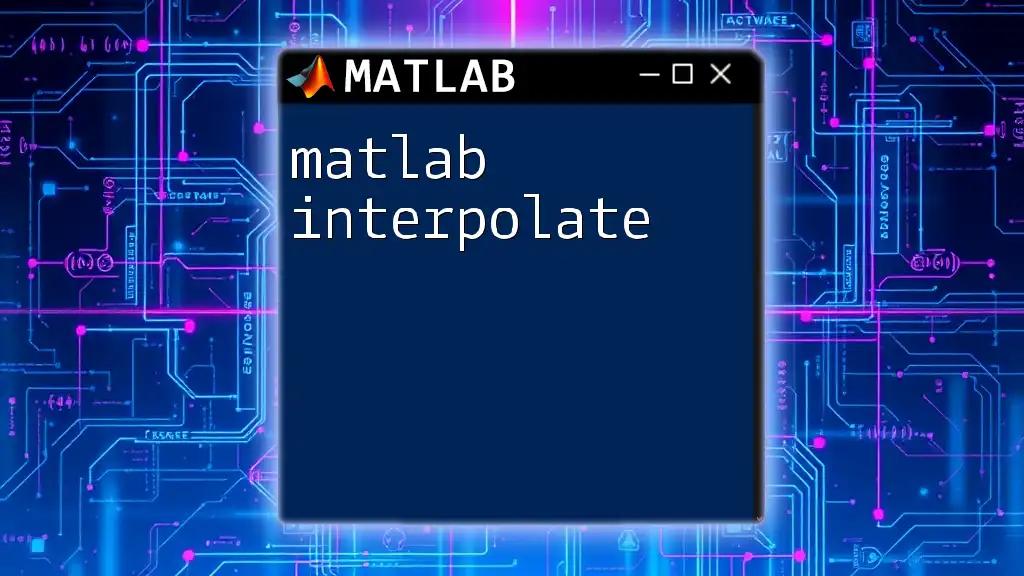
Types of Filters in MATLAB
FIR Filters
Introduction to FIR Filters
FIR filters are characterized by their finite impulse response. They are often easier to design and analyze, making them a popular choice for numerous applications. The key aspects of FIR filters include linear phase response and stability.
Code Snippet: Designing an FIR Filter
To create a simple low-pass FIR filter, use the following code:
b = fir1(20, 0.5); % Create a low-pass FIR filter with cutoff frequency of 0.5
fvtool(b, 1); % Visualize the filter's magnitude response
IIR Filters
Introduction to IIR Filters
IIR filters have an infinite impulse response and can achieve a desired filter characteristic with a lower order than FIR filters. They're commonly used for their efficiency in terms of computational resources.
Code Snippet: Designing an IIR Filter
Here is how to create a low-pass IIR filter using a Butterworth design:
[b, a] = butter(4, 0.5); % Create a low-pass IIR filter of order 4
fvtool(b, a); % Visualize the filter's frequency response
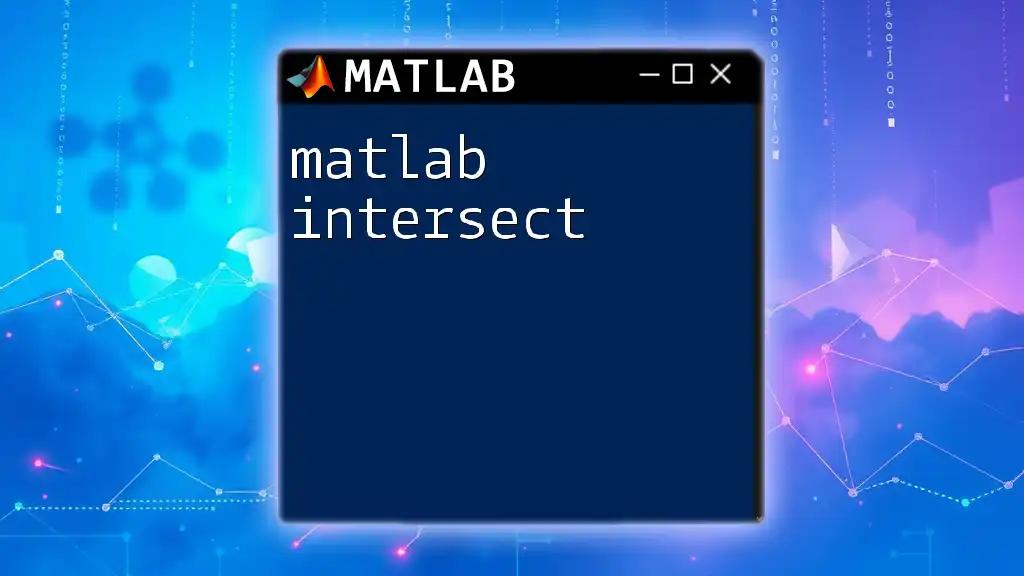
Applying Filters in MATLAB
Using the `filter` Function
The `filter` function is central to applying filters in MATLAB.
How the `filter` Function Works
This function uses the transfer function representation of the filter, where 'b' represents the numerator coefficients and 'a' represents the denominator coefficients.
Example Code:
To apply an FIR filter to a random signal, you can use:
x = randn(1000, 1); % Generate a random signal
y = filter(b, 1, x); % Apply the FIR filter to the signal
plot(y); % Visualize the filtered output
Using the `filtfilt` Function
The `filtfilt` function is essential for zero-phase filtering, processing the input signal in both forward and backward directions.
Understanding Zero-Phase Filtering
This technique helps eliminate phase distortion, which is particularly important in applications requiring accurate timing, like audio processing.
Example Code:
To use `filtfilt`, you can write:
y_filtfilt = filtfilt(b, 1, x); % Apply zero-phase filtering
plot(y_filtfilt); % Visualize the filtered output
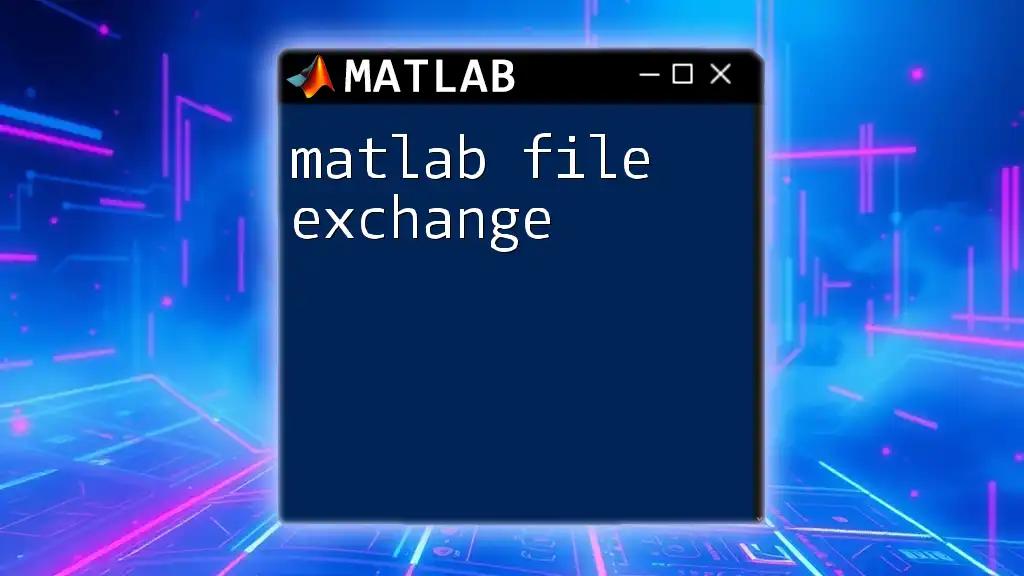
Visualizing Filter Responses
Frequency Response
Understanding the frequency response of a filter helps evaluate its performance.
Code Snippet: Visualizing Frequency Response
To analyze how the filter behaves across different frequencies, use:
freqz(b, a); % Display the frequency response of the filter
Impulse Response
The impulse response provides insight into how the filter responds over time.
Code Snippet: Visualizing Impulse Response
To visualize the impulse response for your filter, you can execute:
impz(b, a); % Display the impulse response of the filter
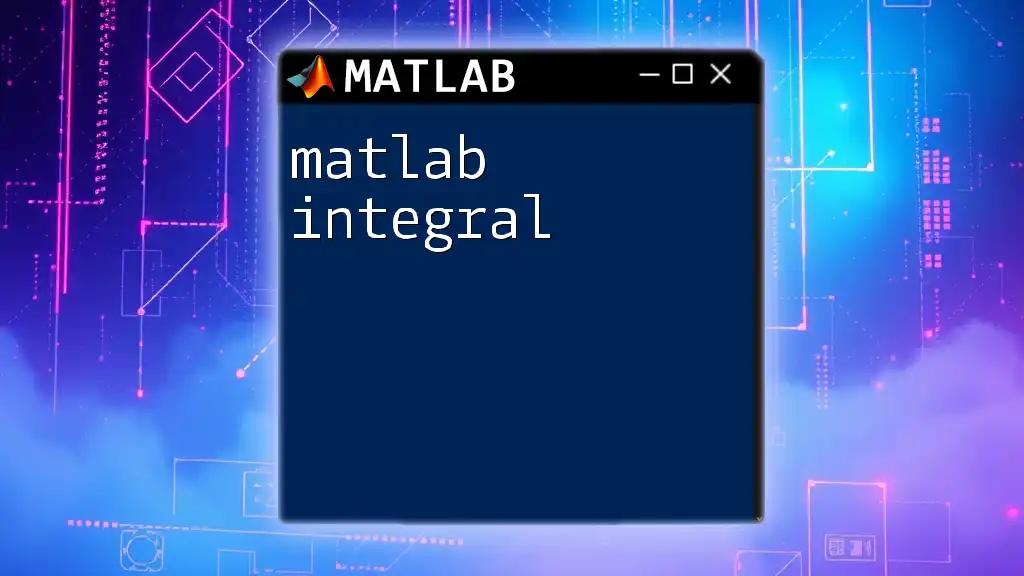
Advanced Topics
Designing Custom Filters
Using the `fdatool`
MATLAB's Filter Design and Analysis Tool (fdatool) is a graphical user interface that simplifies filter design. You can specify requirements such as passband, stopband, and ripple.
Using `fdatool`, you can create custom filters without deep programming knowledge and export the design to MATLAB code for further adjustments or integration into your applications.
Adaptive Filtering Techniques
Adaptive filters can dynamically adjust their parameters in response to changing input signals. These are particularly useful in noise cancellation and echo removal.
Introduction to Adaptive Filters
An example of an adaptive filter algorithm is the Least Mean Squares (LMS) algorithm, which is widely utilized for its simplicity and effectiveness.
Example Code for Adaptive Filtering
Here’s a basic example of how you would implement an adaptive filter:
mu = 0.01; % Step size
n = 512; % Number of iterations
x = randn(n, 1); % Generate input signal
d = sin(2*pi*(1:n)/100)'; % Desired signal
% Adaptive filter implementation can be placed here...
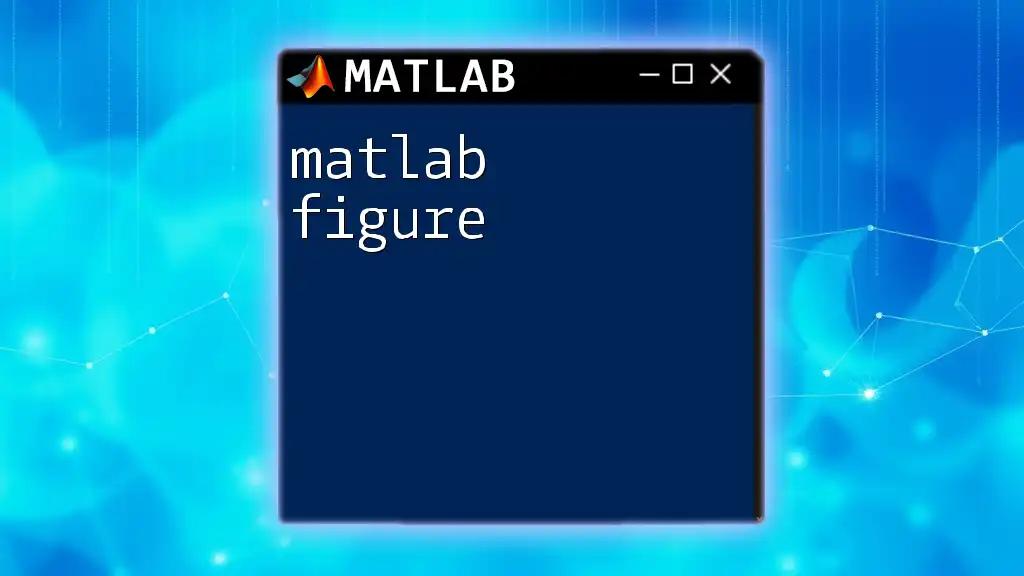
Troubleshooting Common Issues
When implementing filters, you might encounter several common issues, such as unexpected responses or unstable filter behavior.
Common Errors and Solutions
- Unexpected Filter Behavior: Double-check your filter coefficients and ensure proper filter design.
- Signal Length Issues: Ensure that your input and filter coefficients are compatible in terms of dimensions.
Developing a solid understanding of the tools and functions available in MATLAB can help identify and resolve these challenges effectively.
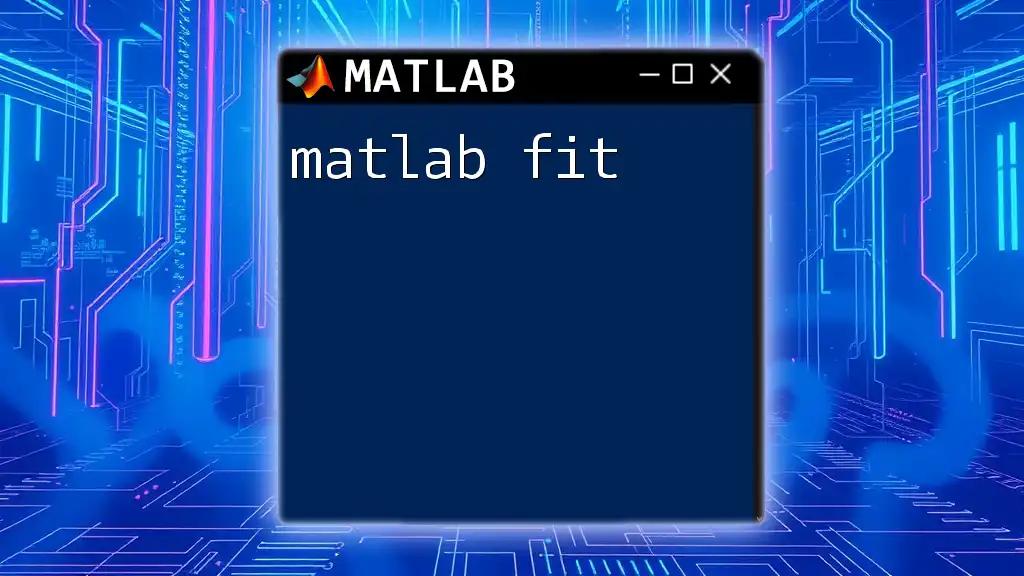
Conclusion
Working with MATLAB filters allows users to perform sophisticated signal processing operations effortlessly. From FIR and IIR filters to adaptive filtering techniques, MATLAB provides extensive functionalities for anyone looking to conduct serious data analysis and manipulation.
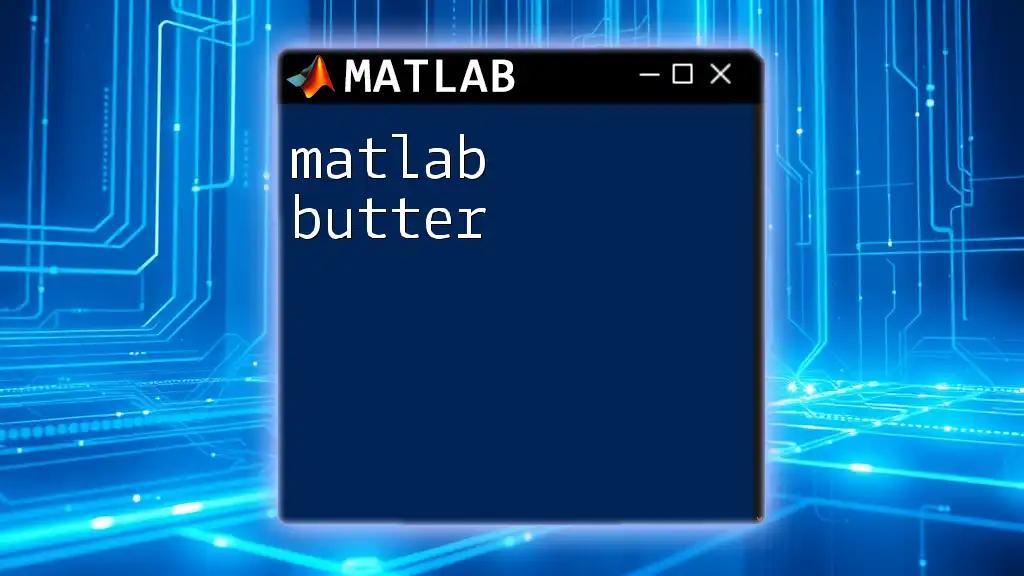
Call to Action
Explore our courses and tutorials designed to deepen your understanding of MATLAB commands and enhance your data processing skills. Join our community to gain access to valuable resources, tips, and state-of-the-art techniques.
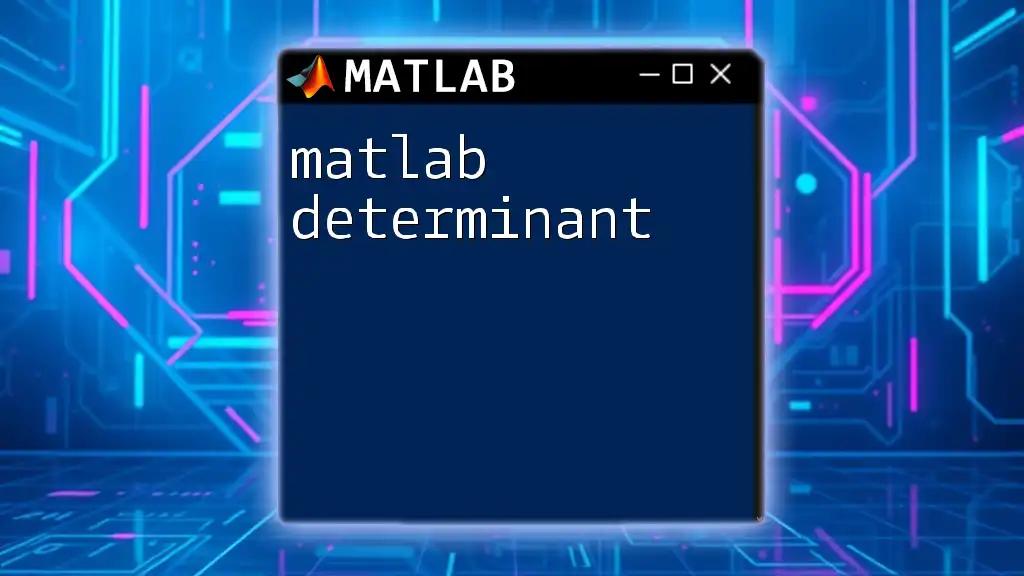
Additional Resources
For further learning, consider exploring MATLAB's official documentation, recommended books on signal processing, and community forums for additional support and collaboration with peers.