The `fit` function in MATLAB is used to create a mathematical model that fits a set of data points, allowing users to analyze and predict trends efficiently.
Here’s a simple example of using the `fit` function in MATLAB:
% Sample data
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Fit a linear model to the data
f = fit(x', y', 'poly1');
% Plot the data and the fitted curve
plot(f, x, y);
Understanding MATLAB Fit
What is MATLAB Fit?
MATLAB Fit is a powerful tool used for statistical analysis and model fitting in MATLAB. The primary goal of fitting is to create a mathematical model that describes the relationship between variables. This method becomes essential in many fields, such as engineering, finance, and biology, where data analysis is crucial. MATLAB offers various fitting techniques, from simple linear fits to complex nonlinear models, enabling users to discover patterns and make predictions from their data.
Applications of Curve Fitting
The applications of MATLAB fit span numerous disciplines. In engineering, it is often used to estimate the parameters of mechanical systems. In finance, analysts use curve fitting to model stock trends and forecast future prices. Similarly, in biology, researchers apply fitting techniques to analyze growth curves and other biological phenomena. These diverse applications highlight the significance of curve fitting in deriving insights from data.
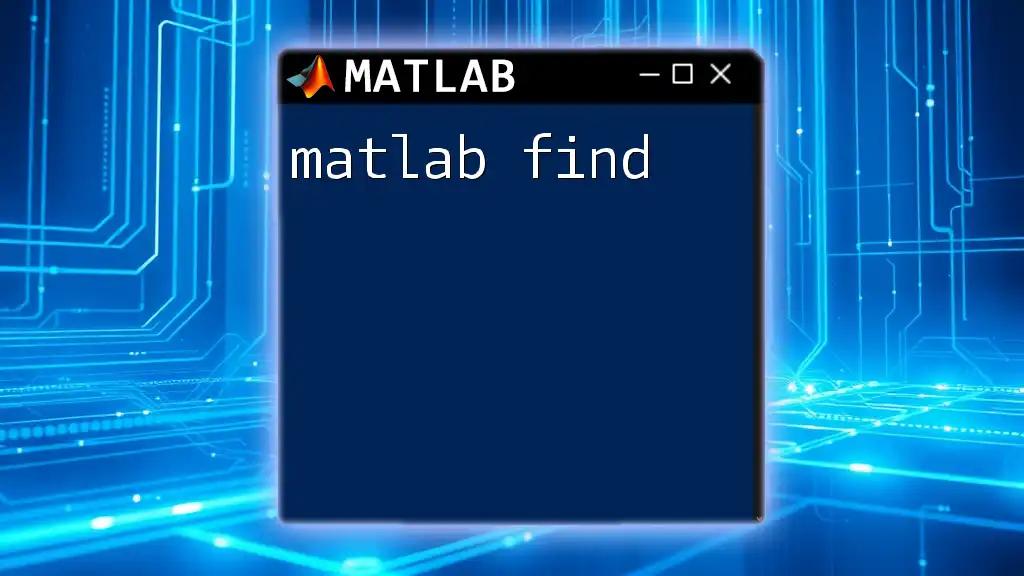
Getting Started with MATLAB Fit
Installing and Setting Up MATLAB
To begin your journey with MATLAB Fit, you need to ensure you have MATLAB installed on your machine. Follow the installation instructions provided on the MATLAB website. Additionally, make sure that the Statistics and Machine Learning Toolbox is installed, as it contains the essential functions required for fitting.
Basic Commands for Data Fitting
Before diving deeper into fitting techniques, familiarize yourself with the fundamental command syntax in MATLAB. Key commands include:
- `fit`: Main function for fitting models to data.
- `fittype`: Defines the type of fit to be used.
- `fitoptions`: Specifies options for fitting, such as tolerances and algorithm choices.
Understanding these commands will lay a solid foundation for more complex fitting tasks.
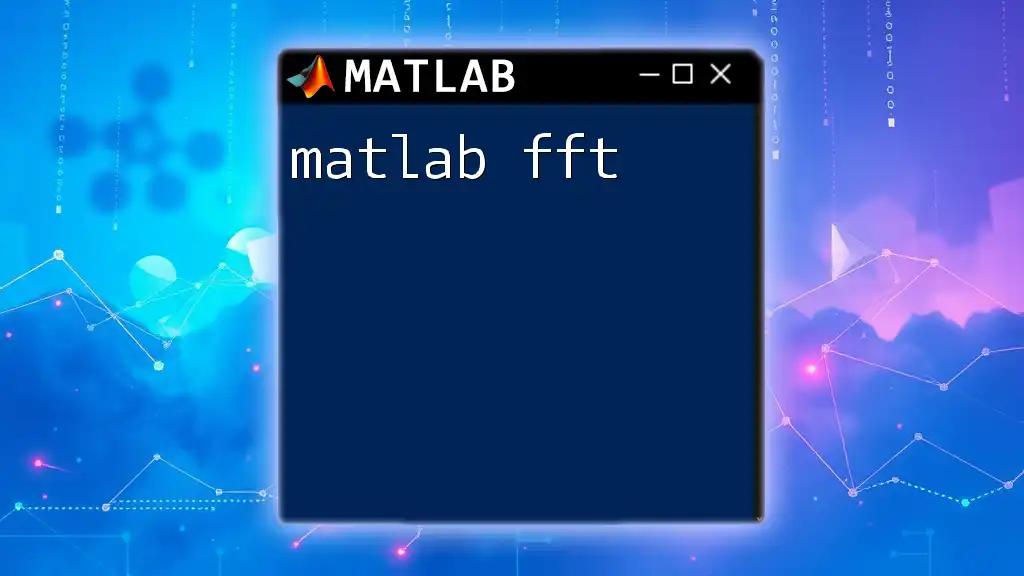
Types of Fitting in MATLAB
Linear vs. Nonlinear Fitting
Linear Fitting
Linear fitting is one of the simplest forms of curve fitting. It assumes a linear relationship between the dependent and independent variables. You can perform linear fitting in MATLAB using the `polyfit` function. Here's an example of linear fitting:
x = [1, 2, 3, 4, 5];
y = [2.1, 4.3, 5.9, 8.7, 10.2];
p = polyfit(x, y, 1); % Linear fit
In the above example, `p` will contain the coefficients of the best-fit line.
Nonlinear Fitting
In many cases, the relationship between variables is not linear. Nonlinear fitting allows you to model more complex relationships using nonlinear equations. You can apply the `fit` function for nonlinear models. Consider the following example:
x = [1, 2, 3, 4, 5];
y = [2.5, 1.6, 3.3, 5.1, 8.2];
ft = fittype('a*exp(b*x)');
fittedModel = fit(x', y', ft, 'StartPoint', [1, 1]);
In this code, we define an exponential model and provide an initial guess for the parameters via the `StartPoint` option.
Polynomial Fitting
Polynomial fitting is a specialized form of nonlinear fitting where we fit a polynomial function of a specified degree to the data. You can obtain polynomial coefficients using the `polyfit` function. Here’s a practical example:
x = linspace(-5, 5, 100);
y = x.^3 - 6*x.^2 + 4*x + 12 + randn(size(x)); % Sample data
[p, S] = polyfit(x, y, 3); % Cubic polynomial fit
This example will store the coefficients of a cubic polynomial in `p`, allowing for further analysis or plotting.
Custom Fitting with User-Defined Functions
Often, you may encounter scenarios where your data does not fit predefined models. In such cases, you can create and use custom fitting functions. Here’s an example of how to define and apply a custom fitting function:
customFun = @(b, x) b(1) * exp(b(2) * x);
beta0 = [1, 0.1]; % Initial guess
beta = nlinfit(x, y, customFun, beta0);
In this snippet, `customFun` specifies an exponential model with parameters to be estimated. The `nlinfit` function optimizes these parameters based on the provided data.
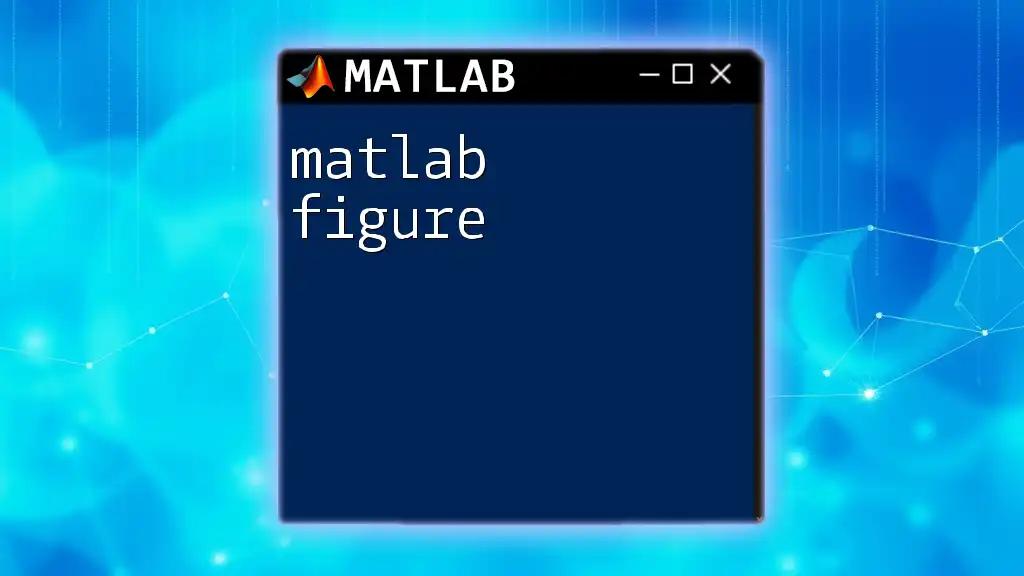
Advanced Techniques in Curve Fitting
Goodness of Fit
Assessing the goodness of fit is crucial for determining how well your model represents the data. Common metrics include R-squared, adjusted R-squared, and root mean squared error (RMSE). The R-squared value indicates the proportion of variance explained by the model. To calculate R-squared, use the formula:
R2 = 1 - (SSres / SStot); % SSres: Residual sum of squares, SStot: Total sum of squares
This metric helps compare how different models perform; a higher R-squared value generally indicates a better fit.
Handling Outliers
Outliers can significantly skew fitting results. Identifying and appropriately handling outliers is essential for achieving accurate fit. Techniques for handling outliers include robust fitting methods, which increase the model's resilience to extreme values. MATLAB provides functions designed to handle such issues effectively, allowing you to focus on meaningful patterns in the data.
Model Selection and Comparison
When working with multiple models, selecting the best one is vital. You can use Information Criteria (like Akaike Information Criterion (AIC) and Bayesian Information Criterion (BIC)) for model selection. These criteria penalize complex models, helping to avoid overfitting. A lower AIC or BIC value generally indicates a better model fit.

Visualization and Interpretation of Fit
Plotting Fitted Models
Visualizing the fitted model alongside the data helps you understand the fit's effectiveness. MATLAB provides extensive plotting functions for this purpose. Here’s how to visualize a fitted model:
figure;
plot(x, y, 'ro'); % Original data points
hold on;
plot(fittedModel, 'b-'); % Fitted curve
title('Data Points and Fitted Model');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data', 'Fitted Curve');
This code will produce a plot showing the original data and the fitted curve, enabling visual assessment of the fit.
Analyzing Fit Results
When you fit a model, MATLAB returns an output structure that contains valuable information. This structure typically includes regression coefficients, goodness-of-fit statistics, and diagnostic plots. Understanding how to interpret these outputs will enhance your fitting analysis and guide you toward making informed choices about your data.
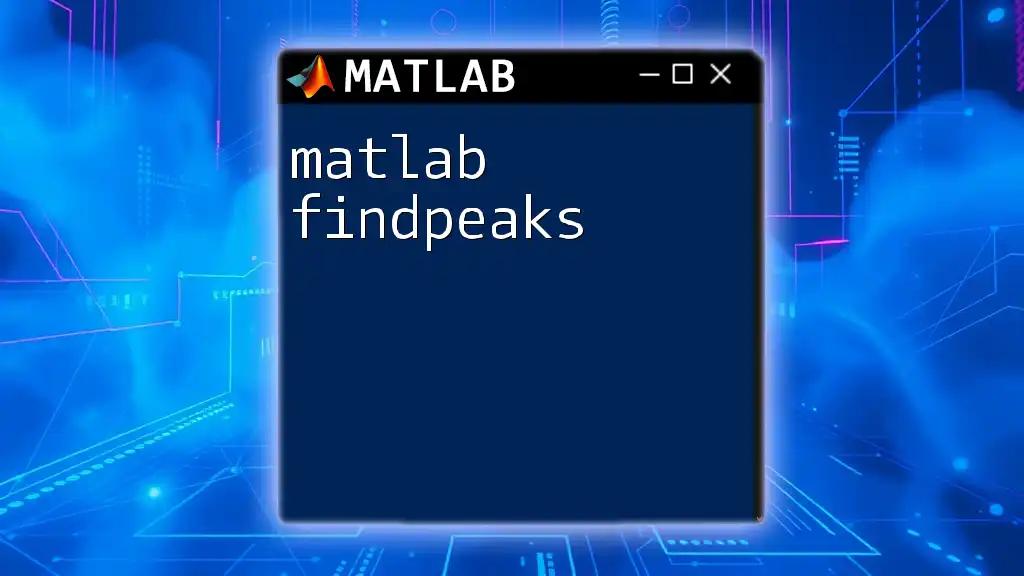
Troubleshooting Common Issues
Encountering challenges during fitting is common. Some typical pitfalls include poor fit due to inappropriate model choice or issues with data quality. To troubleshoot:
- Double-check your data for errors, such as missing or erroneous values.
- Choose an appropriate model that accurately represents the expected relationship.
- Experiment with different starting points when optimizing parameters.
By being aware of these common issues and applying best practices, you can significantly improve the fitting process.
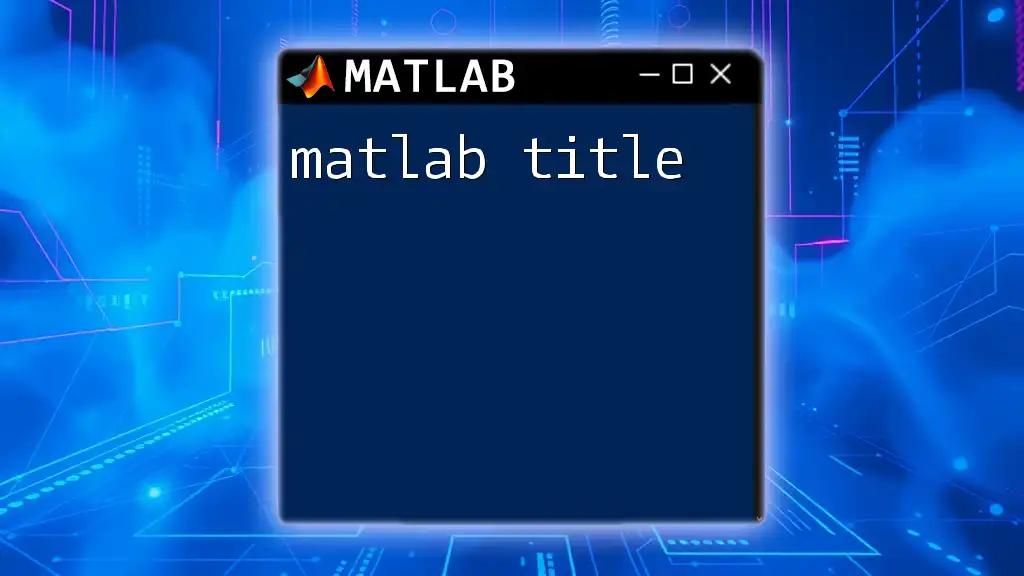
Conclusion
In conclusion, mastering MATLAB Fit opens up a wealth of opportunities for data analysis and modeling. From linear and nonlinear fitting techniques to assessing model performance and handling outliers, understanding these concepts is essential for deriving insights from your data. Continued exploration and hands-on practice with fitting commands will serve to deepen your understanding and proficiency in MATLAB Fit. To further enhance your skills, consider accessing additional resources, including documentation, tutorials, and courses that cover advanced fitting techniques and applications.