The `disp` function in MATLAB is used to display text or numeric values in the Command Window without printing the variable name.
disp('Hello, World!');
Understanding the `disp` Function
What is the `disp` Function?
The `disp` function in MATLAB is a simple yet essential command used primarily for displaying information to the command window. Whether you're working with strings, numbers, or matrices, `disp` makes it straightforward to output your data without any complex formatting.
Unlike `fprintf`, which allows for formatted output and is typically used for more complex displays, `disp` focuses on ease of use. If you simply want to show variable values or arrays without fuss, `disp` is your go-to choice.
Syntax of `disp`
The basic syntax of the `disp` function is as follows:
disp(X)
In this syntax, `X` represents the variable, message, or data you wish to display. This versatility makes `disp` a vital part of programming in MATLAB.
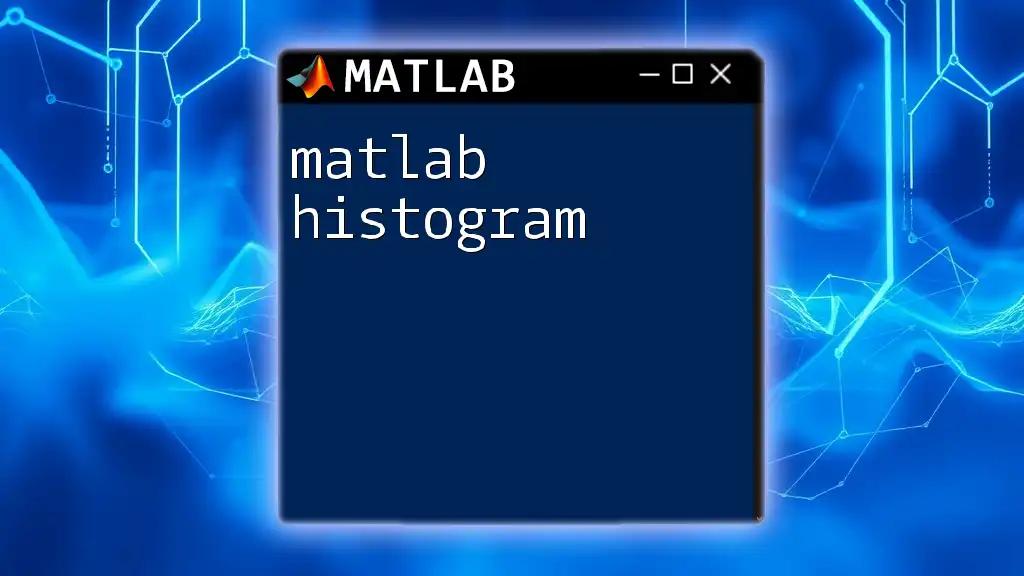
Basic Usage of `disp`
Displaying Text Strings
The simplest application of `disp` is to display text strings. For instance:
disp('Hello, World!')
When this command is executed, the output will be:
Hello, World!
This example demonstrates how `disp` can effectively convey messages. Importantly, MATLAB automatically handles the formatting of strings, so you don’t need to worry about complex syntax when your primary goal is just to display simple text.
Displaying Numeric Values
You can also use `disp` to display numeric values easily. Consider the following example:
num = 42;
disp(num)
When this code runs, you’ll see the output:
42
MATLAB converts the number into its string representation, making it friendly for general output purposes. This automatic conversion feature helps streamline coding, particularly when you’re working with numbers in your programs.
Displaying Arrays and Matrices
`disp` is equally competent when it comes to displaying arrays and matrices. For example:
A = [1, 2, 3; 4, 5, 6];
disp(A)
The output will appear as follows:
1 2 3
4 5 6
Here, `disp` formats the output in a readable manner. It automatically separates the elements of the matrix and presents them in a structured format, which is invaluable for viewing data arrays quickly.
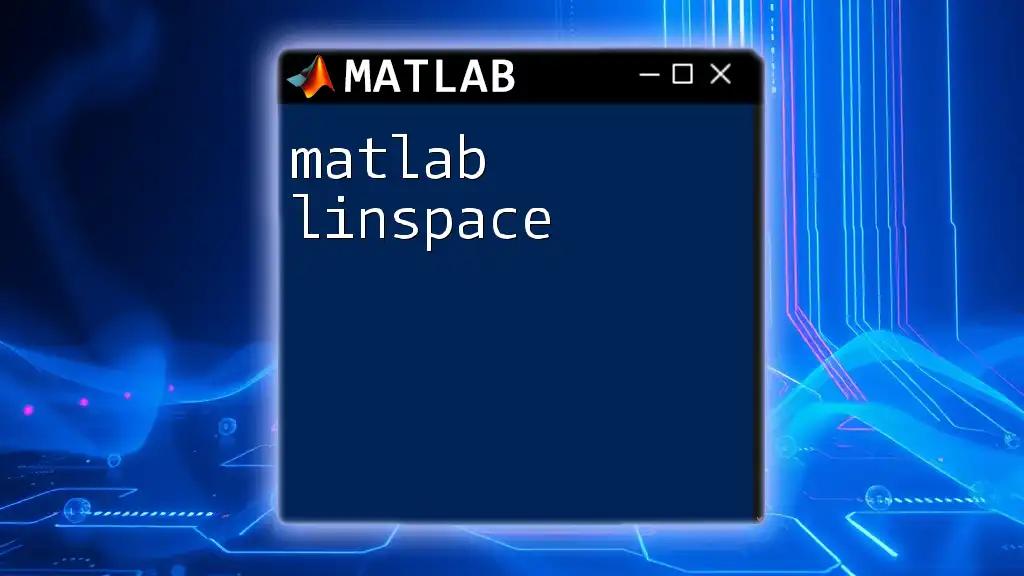
Advanced Usage of `disp`
Displaying Variables with Descriptive Labels
To enhance the clarity of your output, it’s often helpful to display variables alongside descriptive text. You can concatenate text strings and variable values using `disp`. For instance:
radius = 7;
disp(['The radius of the circle is: ', num2str(radius)])
This will yield the following output:
The radius of the circle is: 7
By utilizing the `num2str` function, you can seamlessly integrate numeric values into your display message. This approach is particularly useful for debugging or providing context to your outputs.
Using `disp` in Loops
`disp` is also handy when working within loops, facilitating the tracking of iterations or intermediate results. For example:
for i = 1:5
disp(['Iteration number: ', num2str(i)])
end
Executing this code will display:
Iteration number: 1
Iteration number: 2
Iteration number: 3
Iteration number: 4
Iteration number: 5
This demonstrates how `disp` can simplify output generation, making it easier to visualize the progression of loops and working states of your data in real time.
Formatting Output
While `disp` is limited to straightforward output, it can still accommodate some formatting. However, for precise formatting needs, you might want to use `fprintf`. Here's an example of how to use `disp` along with `num2str` for basic formatting:
value = 52.678;
disp(['Formatted value: ' num2str(value, '%.2f')])
The output would appear as:
Formatted value: 52.68
This technique incorporates numerical formatting through `num2str`, letting you control the number of decimal places for better presentation, although for more extensive formatting options, `fprintf` would be more suitable.
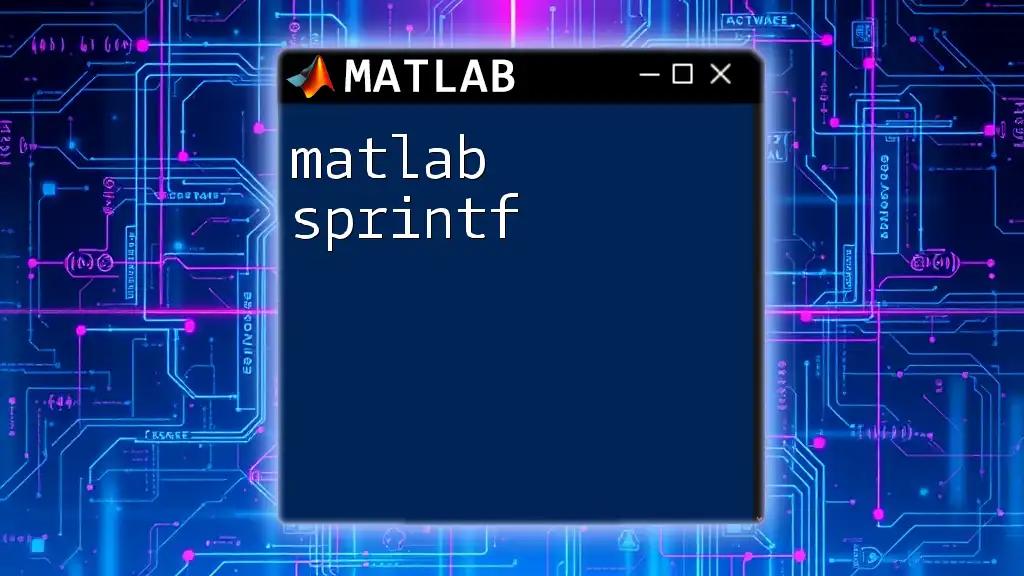
Common Pitfalls and Troubleshooting
Common Errors When Using `disp`
Even though `disp` is straightforward, users might encounter some common pitfalls. One typical error involves forgetting to provide an input variable:
disp() % This will result in an error
Another potential issue arises when trying to pass non-string or non-numeric inputs. Always ensure that your variable data types are compatible with `disp`.
Tips for Effective Display
To maximize the effectiveness of `disp`, consider these best practices:
- Check Variable Types: Always confirm the type of variable you're trying to display before using `disp`. It ensures that you're outputting meaningful information.
- Keep Output Concise: Strive for clarity in your outputs. Avoid overwhelming users with large amounts of data unless necessary.
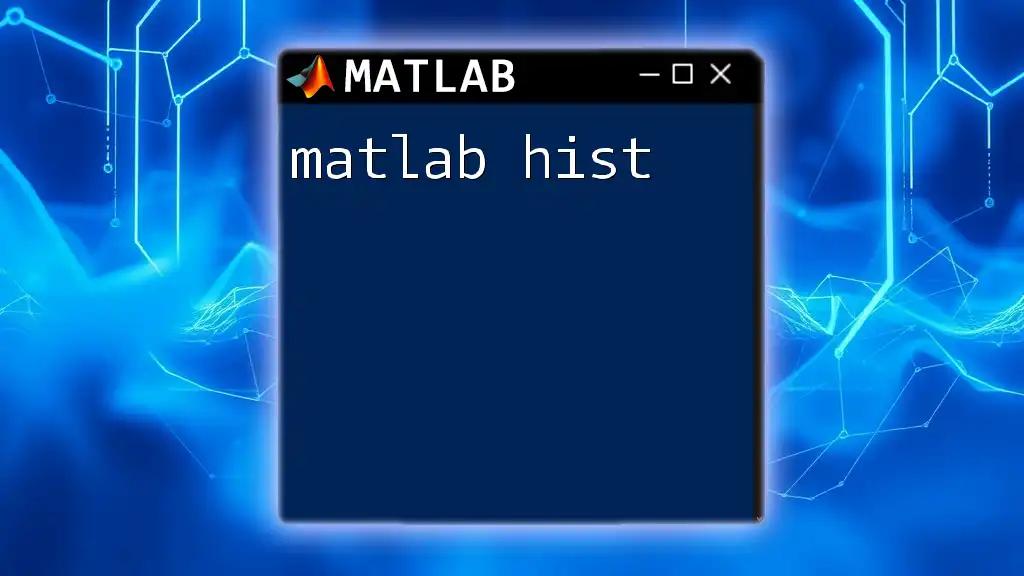
Conclusion
Summary of `disp` Function Utility
The `disp` function in MATLAB is a straightforward yet powerful tool for displaying information. Its simplicity offers an easy way to present results without complex syntax or formatting, making it ideal for quick output needs.
When to Use `disp` Over Other Functions
Choosing between `disp` and functions like `fprintf` or `display` depends on the context. Use `disp` when you want to present data quickly and simply, while relying on `fprintf` for more detailed, formatted outputs.
Encouragement for Practice
As you explore MATLAB further, utilize the `disp` function in your coding tasks. Experiment with various types of data and outputs to deepen your understanding of how this command can enhance your MATLAB programming experience.

Additional Resources
Further Reading
For more detailed information, visit the official MATLAB documentation on the [disp function](https://www.mathworks.com/help/matlab/ref/disp.html).
Community and Support
Engaging with the MATLAB community can also be beneficial. Join forums and platforms where you can ask questions, share ideas, and learn new techniques related to MATLAB programming.