A diagonal matrix in MATLAB is a square matrix where all the elements outside the main diagonal are zero, which can be easily created using the `diag` function.
Here's a code snippet demonstrating how to create a diagonal matrix:
D = diag([1, 2, 3]); % Creates a 3x3 diagonal matrix with 1, 2, and 3 on the diagonal
Understanding Diagonal Matrices
A diagonal matrix is a special type of square matrix where all non-diagonal elements are zero. This means that any matrix of the form:
\[ D = \begin{bmatrix} d_1 & 0 & 0 & \cdots & 0 \\ 0 & d_2 & 0 & \cdots & 0 \\ 0 & 0 & d_3 & \cdots & 0 \\ \vdots & \vdots & \vdots & \ddots & \vdots \\ 0 & 0 & 0 & \cdots & d_n \end{bmatrix} \]
is termed a diagonal matrix, where \(d_1, d_2, d_3,\) and \(d_n\) are the diagonal elements. These matrices possess specific properties that simplify many mathematical operations and computations, making them fundamental in various fields.
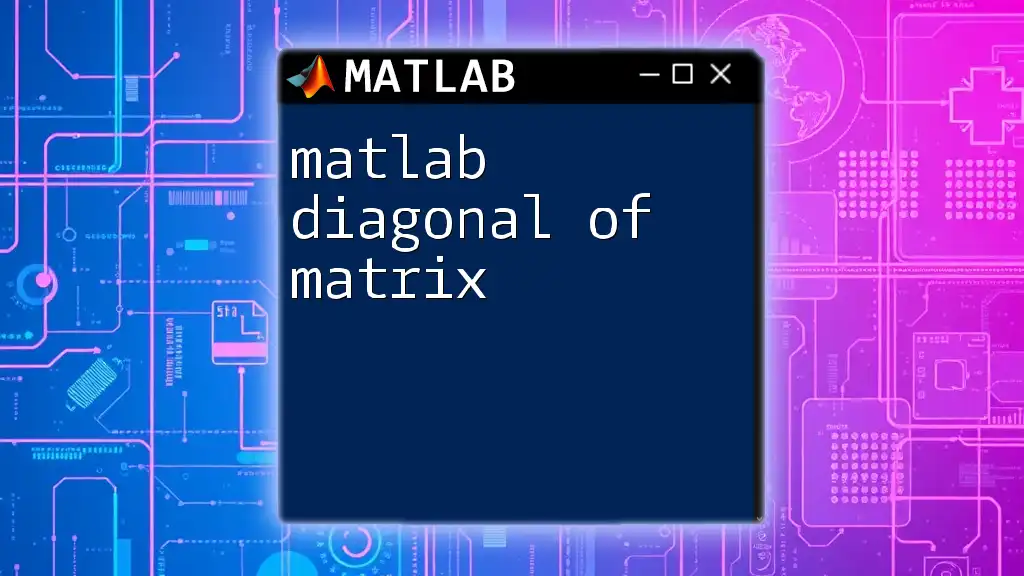
Creating Diagonal Matrices in MATLAB
Using the `diag` Function
MATLAB provides a simple and efficient way to create diagonal matrices using the `diag` function. This function can generate a diagonal matrix from a vector or extract the diagonal elements from a matrix.
Basic Syntax: The syntax for creating a diagonal matrix from a vector is as follows:
D = diag(v);
Here, `v` is a vector, and `D` will be the diagonal matrix formed from the elements of `v`.
Example of Creating a Diagonal Matrix
Let's say we want to create a \(3 \times 3\) diagonal matrix from a vector. Consider the following vector:
v = [1, 2, 3];
D = diag(v);
In this case, matrix `D` will look like:
\[ D = \begin{bmatrix} 1 & 0 & 0 \\ 0 & 2 & 0 \\ 0 & 0 & 3 \end{bmatrix} \]
Here, each element of the vector becomes a corresponding diagonal entry of the matrix, with all other entries set to zero.
Creating a Diagonal Matrix from Elements
Another method to create a diagonal matrix is to manually define its elements. For example, to manually create a diagonal matrix with predefined diagonal elements:
D = [1 0 0; 0 2 0; 0 0 3];
This creates the same diagonal matrix as shown previously. Each row and column configuration ensures that only diagonal elements retain their values while others are set to zero. This is useful when the diagonal values are known upfront.
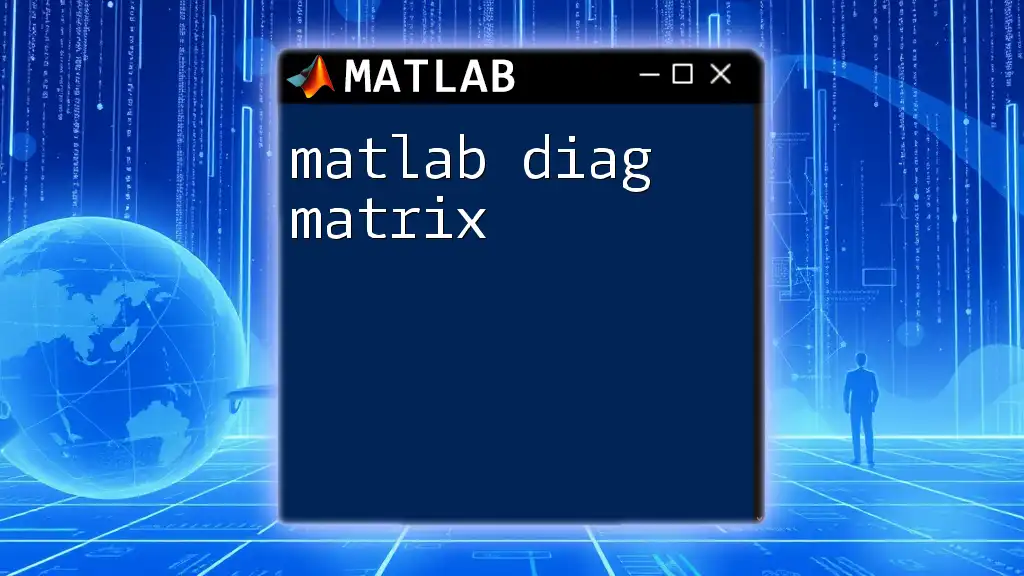
Properties of Diagonal Matrices
Diagonal matrices have numerous properties that can significantly ease computations in linear algebra.
Basic Characteristics
One of the most notable features of diagonal matrices is that their diagonal elements play a significant role in matrix operations. For a diagonal matrix:
- The diagonal elements \(d_i\) are the only non-zero entries.
- It is straightforward to calculate the trace of the matrix, which is simply the sum of its diagonal elements:
\[ \text{Trace}(D) = d_1 + d_2 + ... + d_n. \]
Rank and Determinant
The rank of a diagonal matrix is often equal to the number of non-zero diagonal entries. Thus, if a diagonal matrix has \(k\) non-zero elements, its rank is \(k\).
Calculating the determinant of a diagonal matrix is also simplified; it is equal to the product of its diagonal elements:
detD = det(D); % For D created earlier
This brings significant advantages when solving systems or performing matrix operations, as the computations reduce to multiplying (or checking) a few values.
Eigenvalues and Eigenvectors
In a diagonal matrix, the eigenvalues correspond to the values on the diagonal. The eigenvectors are often associated with standard basis vectors. For example, for the diagonal matrix \(D\), the eigenvalues are \(d_1, d_2, \ldots, d_n\), each corresponding to the eigenvector basis of \([1,0,0], [0,1,0], [0,0,1]\).
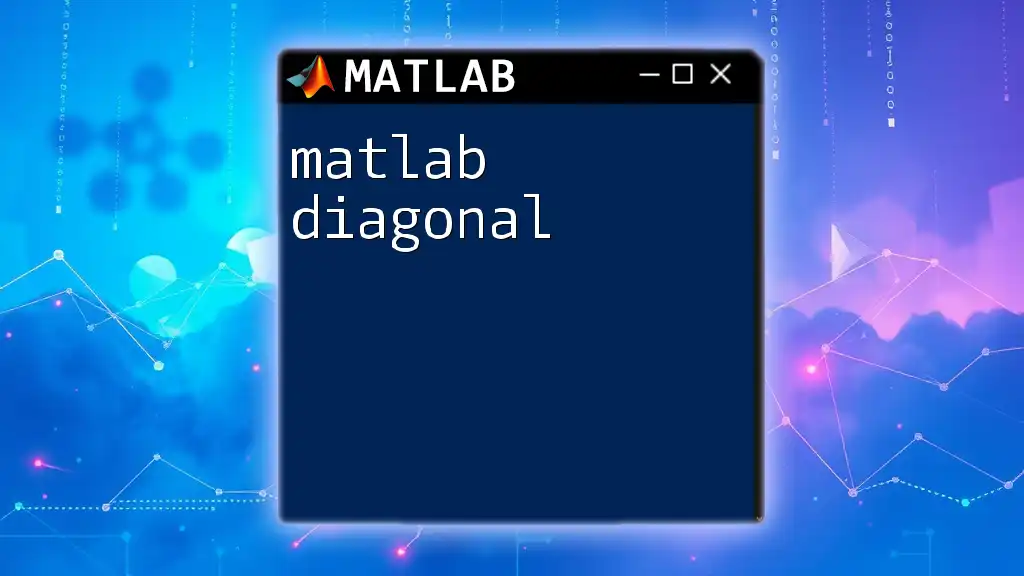
Operations on Diagonal Matrices
Diagonal matrices lend themselves to straightforward operations, which can be executed efficiently in MATLAB.
Addition and Subtraction
Adding or subtracting two diagonal matrices results in another diagonal matrix, where each diagonal entry is simply the sum or the difference of the corresponding entries:
A = diag([1, 3, 5]);
B = diag([2, 4, 6]);
C = A + B; % This results in diag([3, 7, 11])
Scalar Multiplication
Scalar multiplication can also be performed easily with diagonal matrices. When a diagonal matrix is multiplied by a scalar, each diagonal element is multiplied by that scalar:
k = 5;
D_scaled = k * D; % Scales each diagonal entry of D
Matrix Multiplication
The multiplication of two diagonal matrices is another straightforward operation. The product is a diagonal matrix where the entries are the products of the corresponding diagonal elements:
D1 = diag([1, 2]);
D2 = diag([3, 4]);
D_product = D1 * D2; % Results in diag([3, 8])
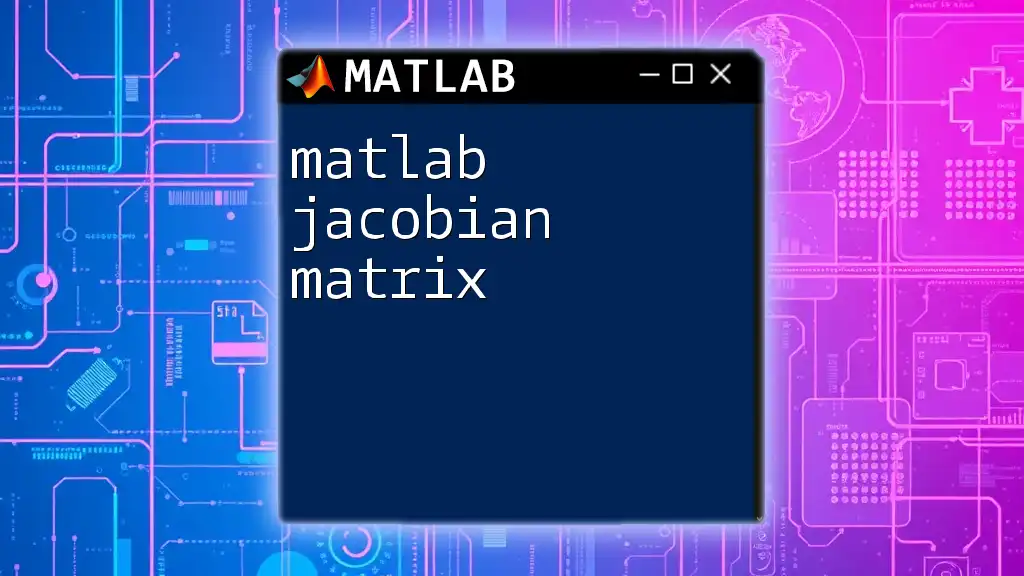
Applications of Diagonal Matrices
Diagonal matrices are widely used in various applications across different fields due to their simplicity and computational efficiency.
In Linear Algebra
In linear algebra, diagonal matrices are instrumental for simplifying complex calculations. Because multiplying by a diagonal matrix only affects the diagonal entries of the other matrix, they help to facilitate tasks such as diagonalization and solving linear systems.
In Image Processing
In image processing, diagonal matrices can be applied in transformation operations. For example, they can help scale images by affecting pixel values along specific dimensions without altering others.
In Data Science
In the field of data science and machine learning, diagonal matrices are utilized for dimensionality reduction techniques and covariance matrices in statistical analysis. They provide a way to simplify datasets by condensing multivariate distributions into manageable forms.
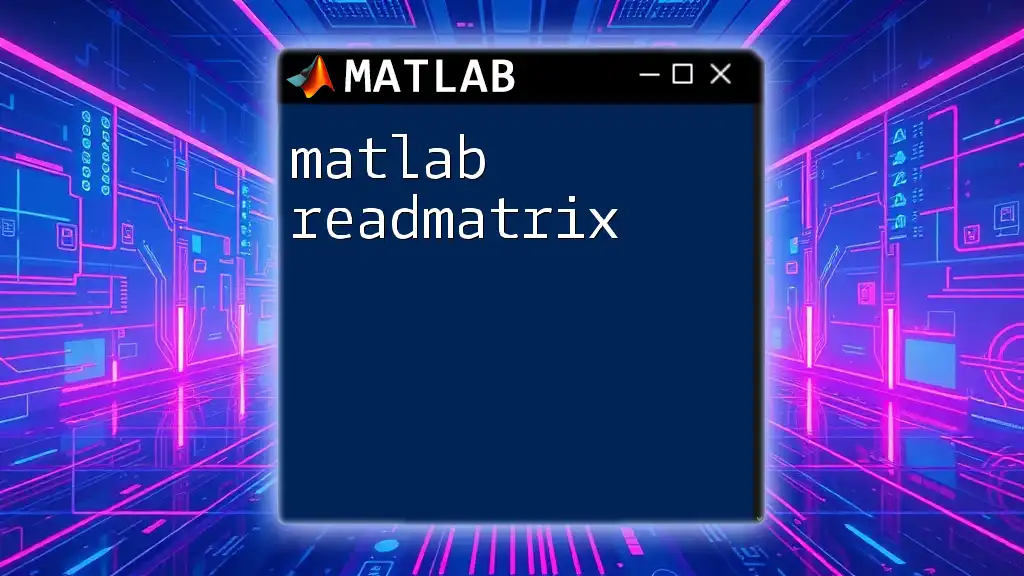
Conclusion
In summary, understanding the concept of MATLAB diagonal matrices is crucial for anyone dealing with linear algebra and its applications. Their simplicity allows for clear operations, ranging from creation to complex manipulations. With the knowledge of the methods and properties outlined in this comprehensive guide, you are better equipped to leverage diagonal matrices in your MATLAB projects and computational tasks.
Next Steps
To further solidify your understanding, practice with the provided code snippets and explore additional resources for advanced learning. Implementing diagonal matrices in your calculations will streamline the process and improve your proficiency in MATLAB.
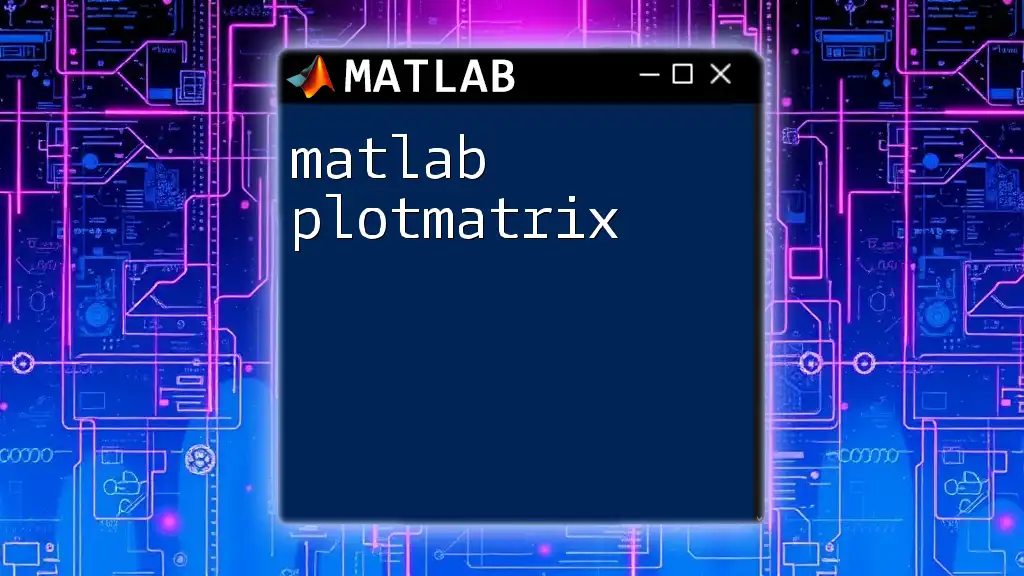
Additional Resources
For more detailed learning, explore the following topics:
- MATLAB documentation on the `diag` function.
- Books on linear algebra with a MATLAB focus.
- Online tutorials dedicated to matrix operations and applications.