In MATLAB, imaginary numbers are represented using the 'i' or 'j' suffix, allowing for complex number calculations, as shown in the following code snippet:
z = 3 + 4i; % Creates a complex number with a real part of 3 and an imaginary part of 4
Understanding Imaginary Numbers
Definition of Imaginary Numbers
Imaginary numbers are one of the fundamental concepts in mathematics, defined as multiples of the imaginary unit \( i \), where \( i = \sqrt{-1} \). This means that the square of \( i \) equals -1. In the context of mathematics, imaginary numbers allow us to extend the number system beyond real numbers, forming what we know as complex numbers. Each complex number is expressed in the form \( z = a + bi \), where \( a \) represents the real part and \( b \) represents the imaginary part.
The Role of Imaginary Numbers in Mathematics
Imaginary numbers are not just theoretical constructs. They play a critical role in various fields, including physics, engineering, and applied mathematics. For example, in electrical engineering, imaginary numbers are essential in analyzing alternating current (AC) circuits through the use of phasors. Moreover, in control theory, they help in understanding the stability of systems by allowing us to represent oscillations and dampings mathematically.
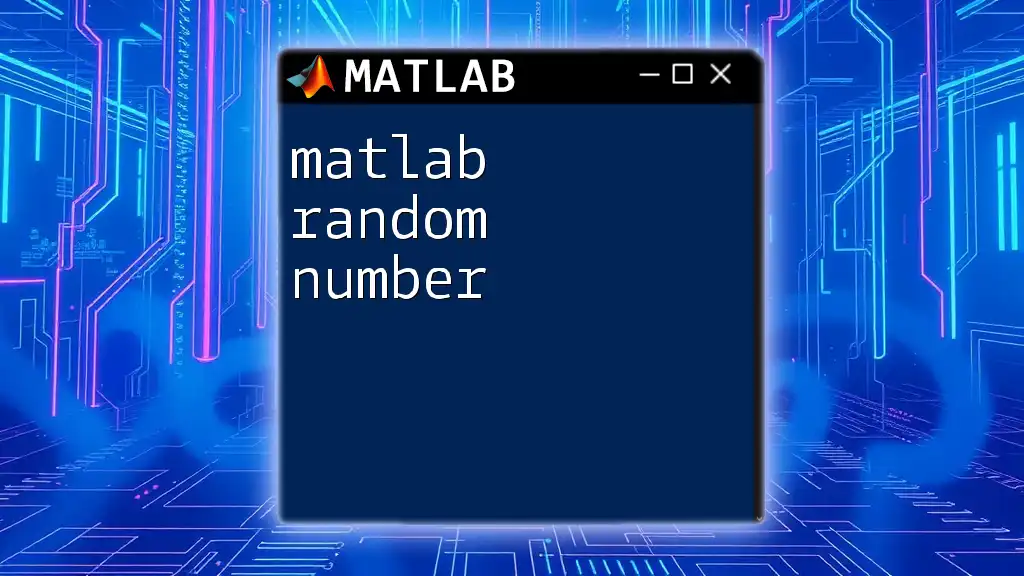
Working with Imaginary Numbers in MATLAB
Basic Syntax for Imaginary Numbers
In MATLAB, you can easily work with imaginary numbers using specific notations. You can define an imaginary number directly by appending `i` or `j` to your real number. For example:
z = 3 + 4i;
Alternatively, you can also use `1i` or `1j` to create imaginary numbers. Both `i` and `j` serve the same purpose in MATLAB, though some users prefer one over the other to avoid potential variable conflicts.
Creating Complex Numbers
Using the `complex` Function
MATLAB provides a dedicated function to create complex numbers called `complex()`. This function requires two parameters, the real part and the imaginary part. For example:
z = complex(3, 4);
In this command, `3` is the real part and `4` is the imaginary part. The resulting variable `z` now holds the complex number \( 3 + 4i \).
Direct Assignment
You can also create complex numbers through direct assignment. This is straightforward and user-friendly. For instance:
z = 3 + 4i; % or 3 + 4j
It's essential to recognize that both `i` and `j` can be used to indicate the imaginary component in MATLAB. However, if you accidentally redefine either `i` or `j` in your code, you may encounter unexpected results.
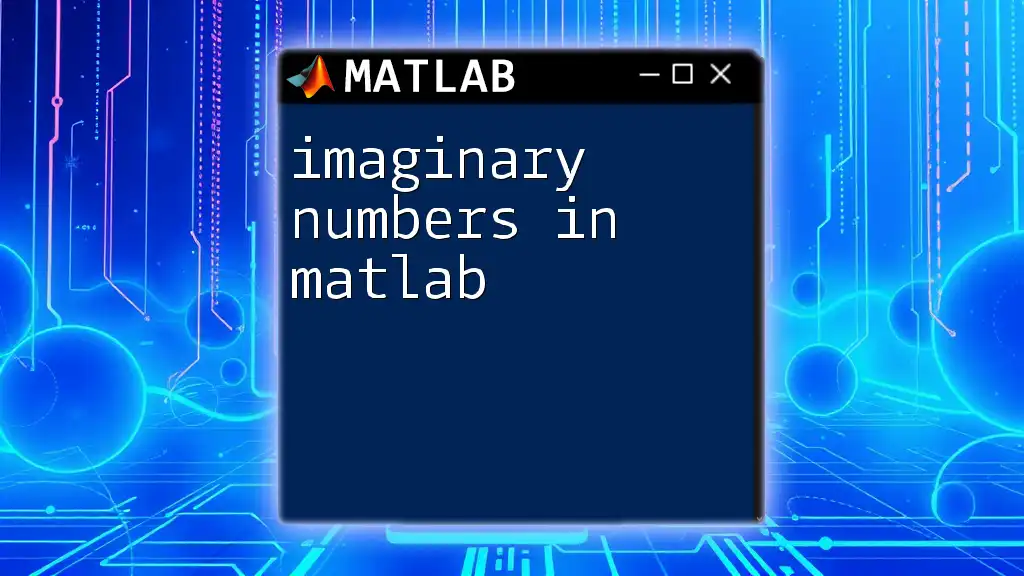
Performing Calculations with Imaginary Numbers
Basic Operations
Addition and Subtraction
Using MATLAB, addition and subtraction of complex numbers operates just like with real numbers. Here's an example to illustrate:
a = 2 + 3i;
b = 1 + 2i;
c = a + b; % (2+3i) + (1+2i) = 3 + 5i
In this snippet, when we add `a` and `b`, the real and imaginary parts are combined separately, resulting in a new complex number \( 3 + 5i \).
Multiplication and Division
Similarly, multiplication and division of complex numbers can be performed seamlessly. Here's how it works:
d = a * b; % Multiplication
e = a / b; % Division
In these operations, MATLAB uses the underlying principles of complex arithmetic automatically to return the expected results, which can include both real and imaginary components.
Visualization of Complex Numbers
Using MATLAB Plots
Visualizing complex numbers can lead to a better understanding of their properties and relationships. You can create a plot of complex numbers in the complex plane, where the x-axis represents the real part and the y-axis represents the imaginary part. Here’s a MATLAB code example:
z = [1+2i, 2+3i, -1+1i];
plot(real(z), imag(z), 'o');
xlabel('Real Part');
ylabel('Imaginary Part');
title('Plot of Complex Numbers');
grid on;
This example plots three complex numbers, helping to visually interpret their positions in the complex plane.
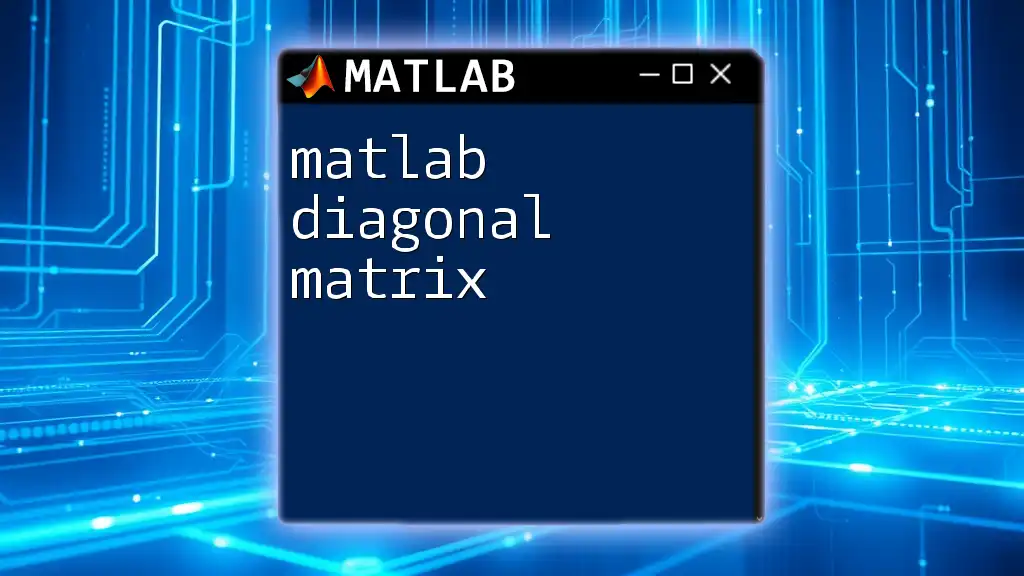
Functions Involving Imaginary Numbers
Built-in MATLAB Functions
Absolute Value and Angle
MATLAB comes equipped with built-in functions to compute various properties of complex numbers, such as their absolute value (magnitude) and angle (phase). You can use:
abs_z = abs(z); % Magnitude
angle_z = angle(z); % Phase
The `abs()` function returns the distance from the origin to the point representing the complex number in the complex plane, while the `angle()` function provides the angle (in radians) from the positive x-axis to the line connecting the origin to the point.
Conjugate of a Complex Number
Another important operation is finding the conjugate of a complex number, which is useful in various mathematical manipulations. You can obtain the conjugate using the following command:
conj_z = conj(z); % Conjugate
The conjugate of a complex number \( a + bi \) is \( a - bi \), and this transformation has significant implications in many calculations, such as simplifying division.
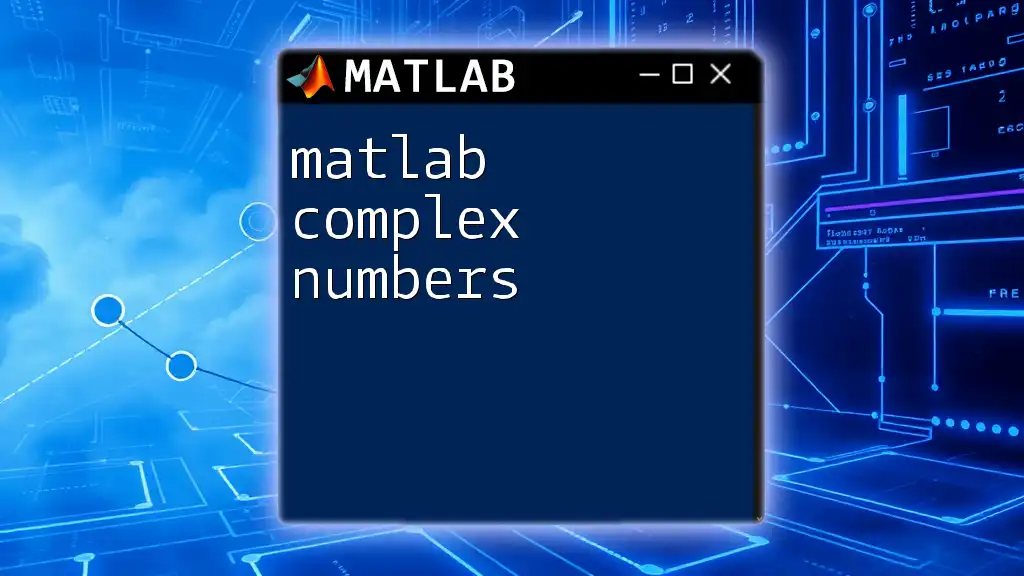
Applications of Imaginary Numbers in MATLAB
Electrical Engineering Applications
The incorporation of imaginary numbers is fundamental in electrical engineering, particularly in AC circuit analysis. Phasors allow for the representation of sinusoidal signals in a simpler form that incorporates both amplitude and phase using complex numbers. By using MATLAB, engineers can compute circuit behaviors with astonishing ease, leveraging the properties of imaginary numbers for efficiency.
Signal Processing
Imaginary numbers also have critical applications in signal processing, particularly in the context of Fourier transformations, where signals are decomposed into their frequency components. Utilizing the `fft` function in MATLAB, you can perform a Fast Fourier Transform as follows:
signal = [1, 2, 3, 4];
transformed_signal = fft(signal);
This transformation allows you to analyze frequency content efficiently, making complex computations more manageable.
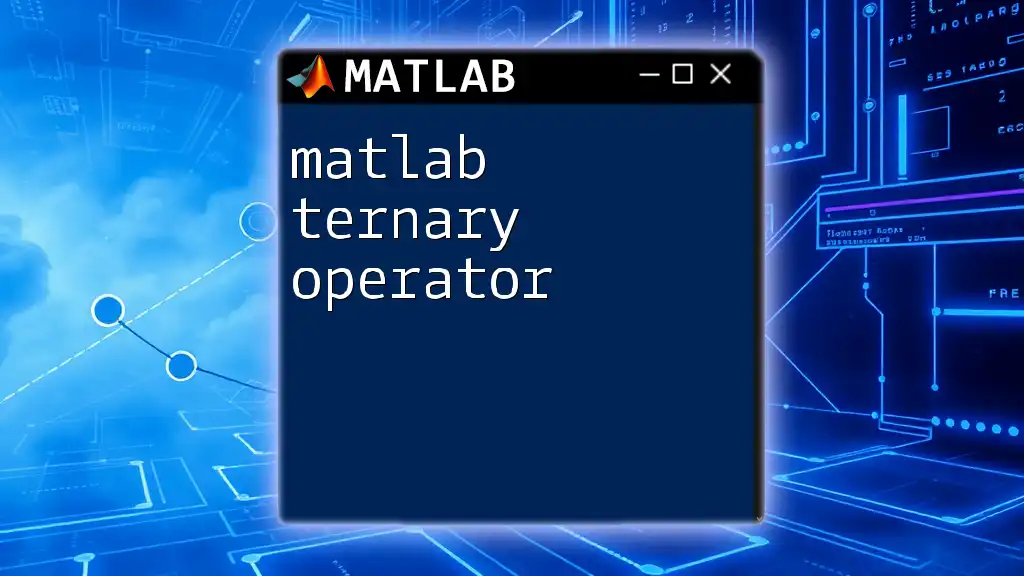
Troubleshooting Common Issues
Common Mistakes in Handling Imaginary Numbers
While working with MATLAB and imaginary numbers, it's essential to avoid common pitfalls. One typical mistake is redefining `i` or `j`, which can lead to unexpected behaviors in your calculations. Make sure to check your variable assignments to prevent these types of errors. Additionally, when plotting complex data, ensure you correctly interpret the plotted graphs, as confusing the axes can result in misinterpretation.
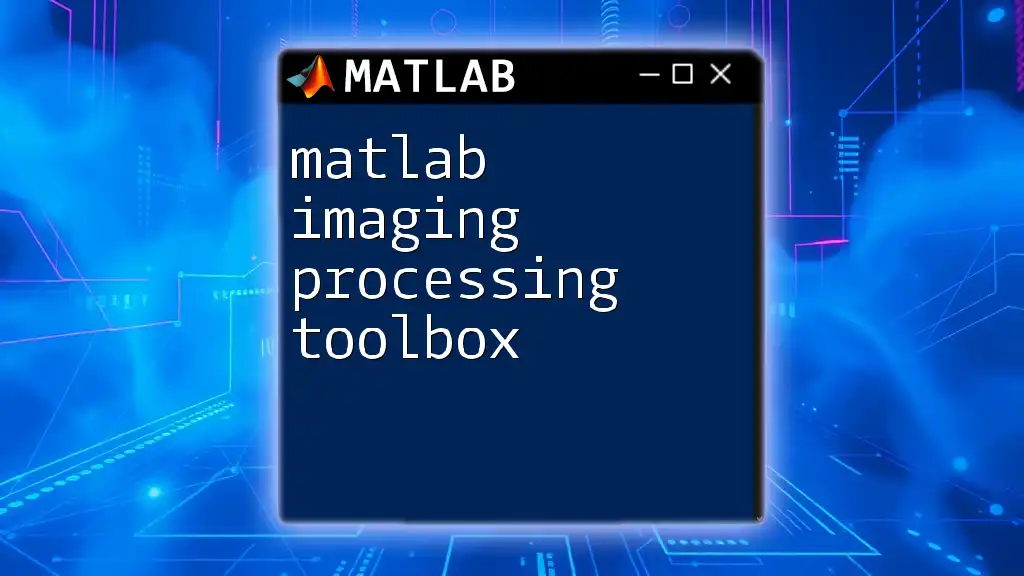
Conclusion
In summary, understanding and effectively utilizing imaginary numbers in MATLAB opens up a world of mathematical possibilities. By leveraging the native syntax, functions, and visualizations that MATLAB offers, users can grasp complex concepts and apply them to practical engineering problems. Armed with this knowledge, you're well-equipped to explore further and apply imaginary numbers with confidence in your own projects and problem-solving endeavors.
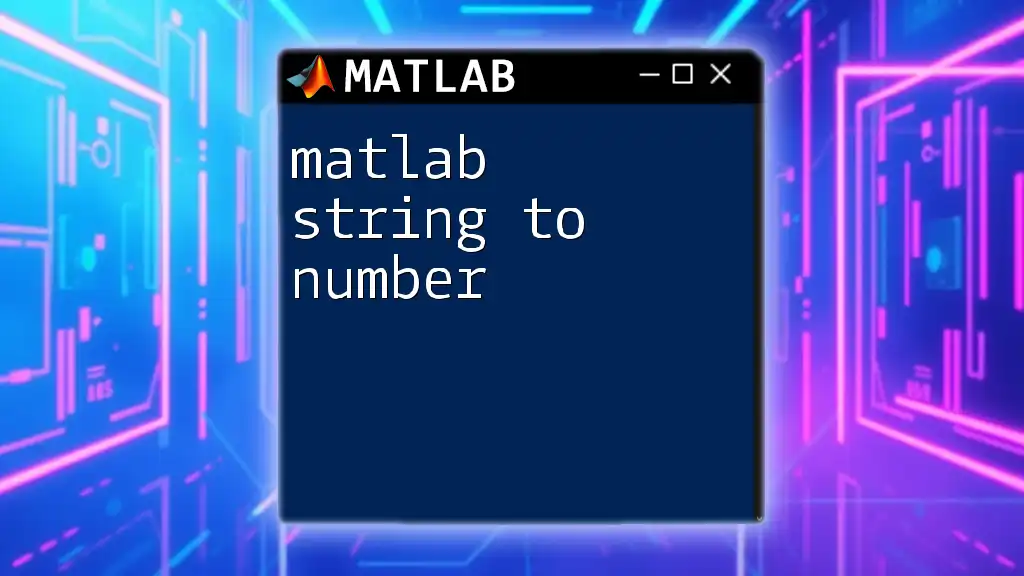
Further Reading and Resources
For more in-depth exploration of imaginary numbers and their applications in MATLAB, consulting the official MATLAB documentation or specialized textbooks focused on complex analysis and MATLAB programming will provide valuable insights and examples.