The `isnan` function in MATLAB checks for NaN (Not-a-Number) values in an array, returning a logical array of the same size with true (1) where the elements are NaN and false (0) otherwise.
Here's a code snippet illustrating the use of `isnan`:
% Example array
A = [1, 2, NaN, 4, NaN];
% Check for NaN values
result = isnan(A);
% Display the result
disp(result);
Understanding the isnan Function
The isnan function in MATLAB is integral to managing NaN (Not a Number) values, which often appear in datasets due to undefined operations or missing data. NaNs can lead to inaccurate results if not dealt with properly. Thus, understanding how to identify and manage these values is crucial for effective data analysis.
What is isnan?
The isnan function is designed to detect NaN values within an array or matrix. When called, it returns a logical array of the same size as the input, where each element is set to true (1) if the corresponding element of the input is NaN and false (0) otherwise. This function is essential for any developer or analyst working with datasets where missing or invalid entries may skew results.
Significance of isnan in Data Processing
Handling NaN values is a common requirement in data preprocessing. When conducting analyses such as statistical modeling or machine learning, having clean data is paramount. The isnan function not only helps achieve this cleanliness but also maintains the integrity of the analyses by ensuring that computations do not yield misleading results due to the presence of NaN values.
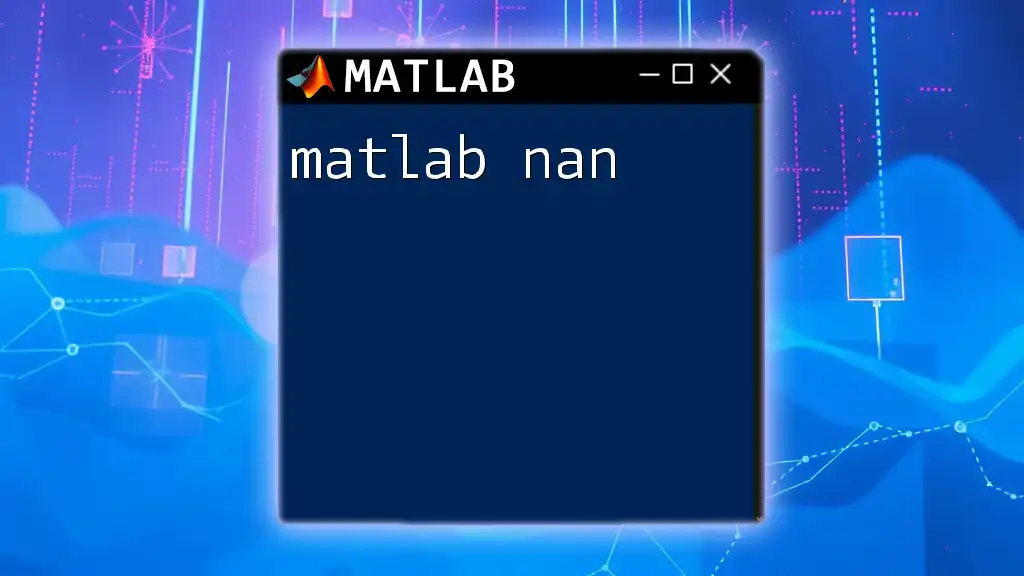
Syntax and Usage
Basic Syntax
The basic syntax for the isnan function is as follows:
tf = isnan(A)
Here, A is the input array or matrix that you wish to evaluate, and tf will be the output logical array indicating the presence of NaN values.
Return Value
The return value of the isnan function is a logical array of the same dimensions as A. Each element is either true (1) or false (0), offering an easy visual representation of where NaNs are located within the data.
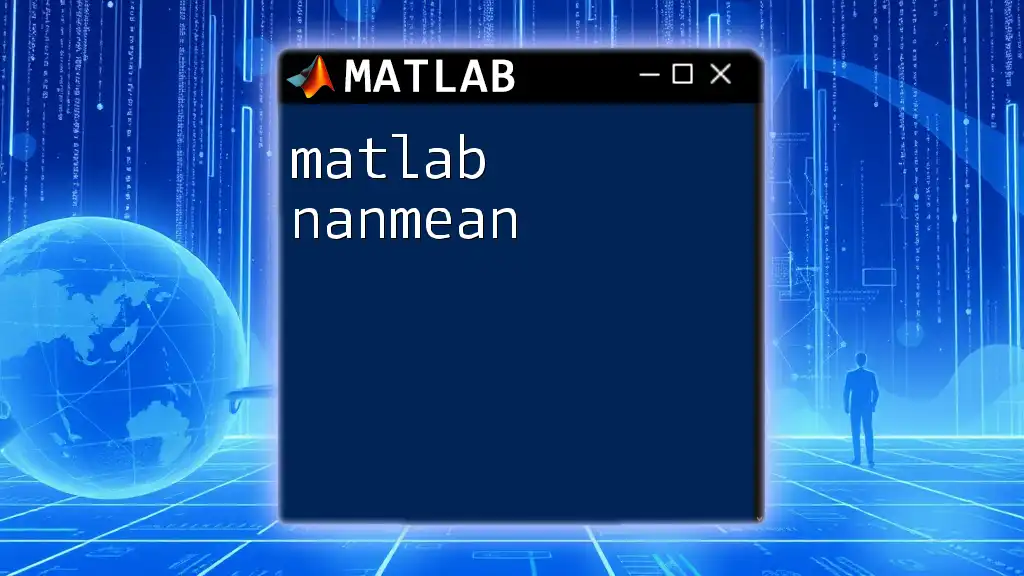
Examples of Using isnan
Example 1: Identifying NaN Values
To demonstrate the functionality of isnan, consider the following example:
A = [1, 2, NaN; 4, NaN, 6];
tf = isnan(A);
disp(tf); % Displays: [0 0 1; 0 1 0]
In this code snippet, we first define a matrix A. When we call isnan(A), the output tf indicates the positions of the NaN values in the matrix, providing clarity on which data needs addressing.
Example 2: Filtering Out NaN Values
Next, let's look at how we can use isnan to filter out NaN entries from a dataset:
A = [1, 2, NaN; 4, NaN, 6];
B = A(~isnan(A));
disp(B); % Displays: [1; 2; 4; 6]
In this example, we utilize logical indexing to select only those values in A that are not NaN. The resulting variable B contains a clean list of values, invaluable for subsequent calculations.
Example 3: Using isnan in Conditional Statements
The isnan function can also be used within loops or conditional structures, as shown below:
A = [1, 2, NaN; 4, 5, 6];
for i = 1:numel(A)
if isnan(A(i))
disp('Found NaN value');
end
end
This looping structure checks each element of the matrix A. When the condition isnan(A(i)) returns true, the code will output a message indicating that a NaN has been found. This is particularly useful for debugging or cleaning data before performing more complex analyses.
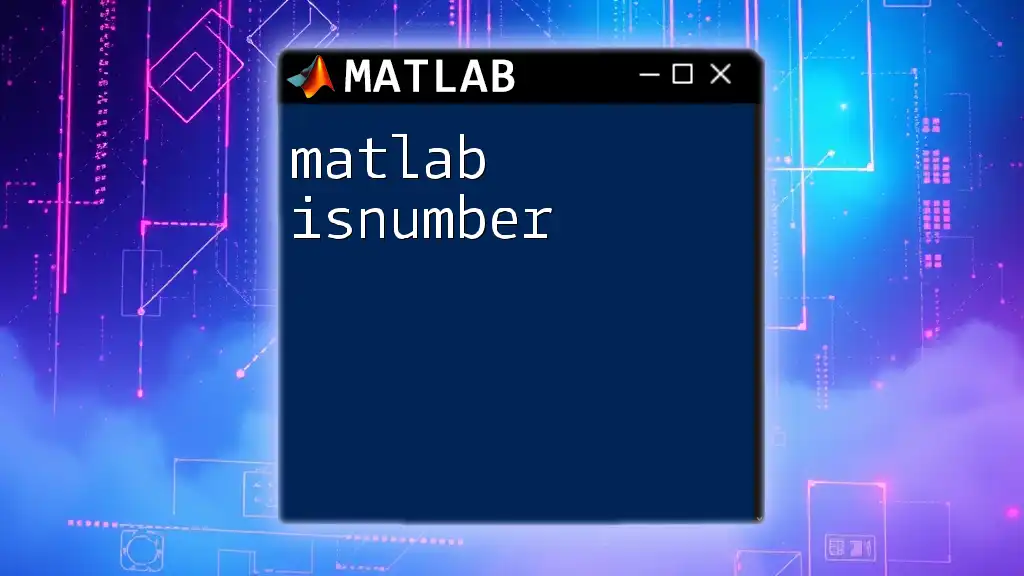
Handling NaN Values in Datasets
Importance of Dealing with NaN in Data Analysis
NaN values can significantly impact the results of statistical analyses. If a dataset contains NaN values and these are not accounted for—in any summary statistics or modeling—it can produce errors or misleading interpretations. Therefore, addressing these missing values is a pivotal step in data analysis.
Removing NaN Values
Methods for Removing NaN Entries
One effective method for removing NaN values is through the rmmissing() function. Here’s how to implement it:
A = [1, 2, NaN, 4, NaN, 6];
B = rmmissing(A);
disp(B); % Displays: [1 2 4 6]
In this example, the rmmissing function efficiently removes any entries that contain NaN values, resulting in a streamlined dataset B that can be used for further analysis.
Replacing NaN Values
Another strategy to handle NaN values is to replace them, as shown in this code snippet:
A = [1, 2, NaN; 4, NaN, 6];
A(isnan(A)) = 0; % Replacing NaN with 0
disp(A); % Displays: [1 2 0; 4 0 6]
Here, all NaN values in the array A are replaced with zeroes, which can be useful if zero is an acceptable substitute in your analysis. However, it's crucial to consider the implications of such replacements, as they can impact the resulting statistical outputs.
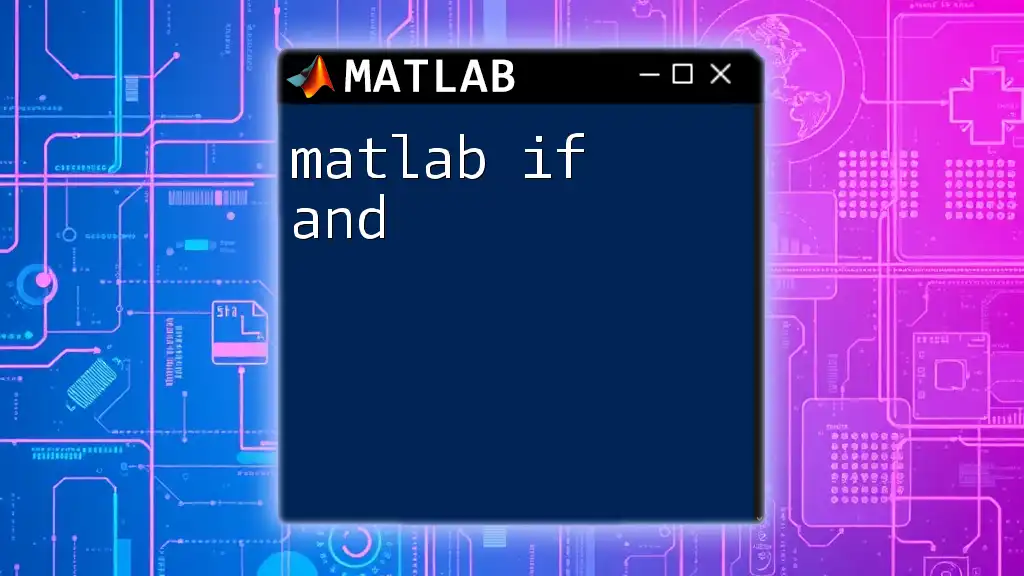
Performance Considerations
Efficiency of isnan in Large Datasets
The isnan function operates efficiently even with substantial datasets. However, it’s essential to be mindful of performance issues that could arise when processing extremely large matrices. The logical array resulting from isnan can consume significant memory, particularly if invoked repeatedly on large datasets.
Alternative Approaches
While isnan is a powerful tool, there are also other functions available in MATLAB, such as isfinite or isinf, which can be useful based on the context of what you're analyzing. Exploring these functions can enhance your data-cleaning strategies.
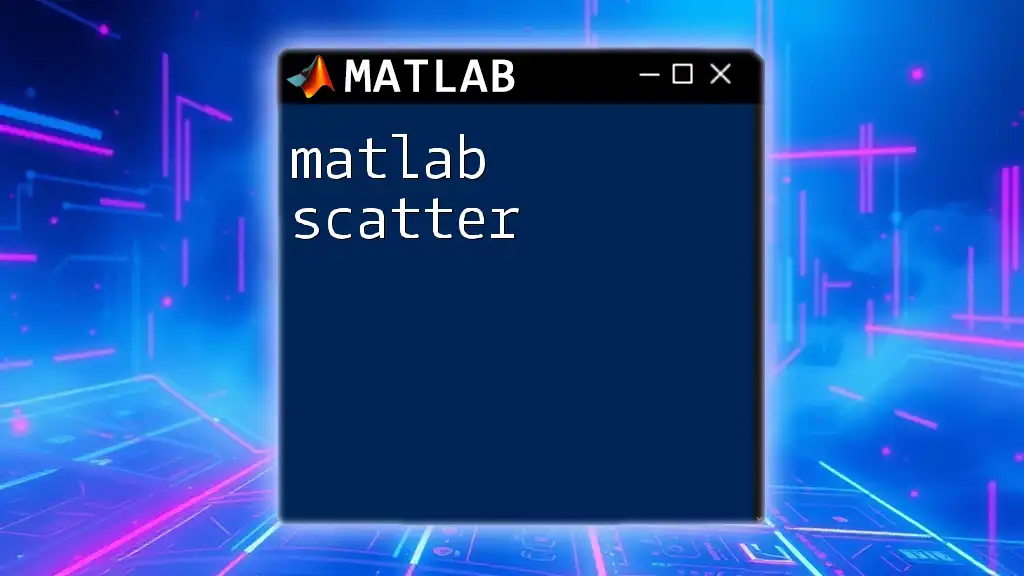
Best Practices for Using isnan
Tips for Effective NaN Management
- Regularly check for NaN values at the outset of your data analysis. This should include applying isnan as one of your initial preprocessing steps.
- Keep an organized record of all modifications made to your dataset, including where NaN values were found and how they were addressed.
Common Pitfalls
Be cautious about misunderstanding the output of isNaN, especially in cases where NaN values might not be the only form of missing data (like empty strings or infinities). Additionally, be careful when replacing NaN values; substituting them with inappropriate values can lead to misleading analyses.
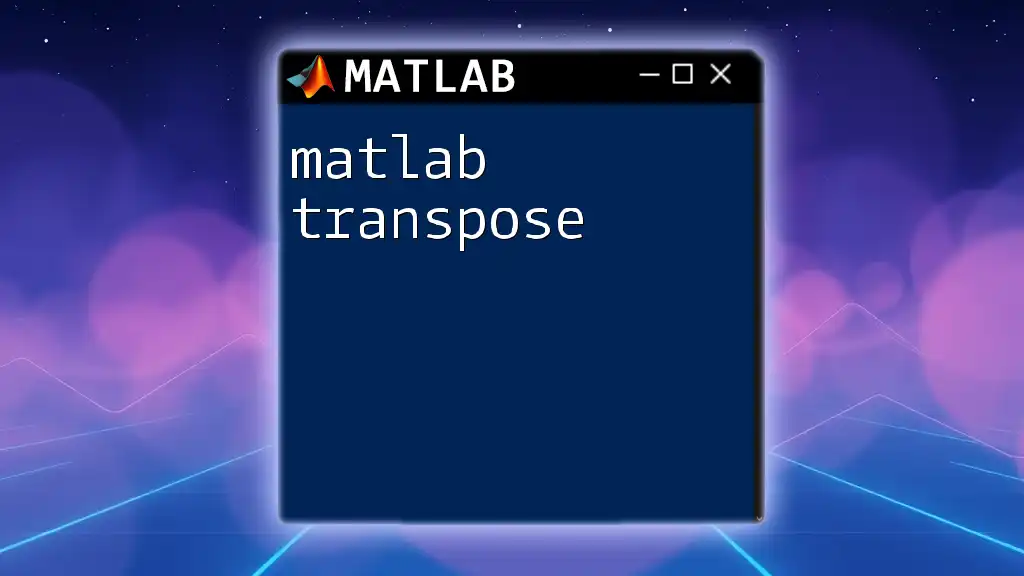
Conclusion
In summary, the matlab isnan function is an essential tool for managing NaN values in any dataset, allowing you to identify, remove, or replace these values effectively. A keen understanding of how to implement isnan appropriately can greatly enhance the quality and accuracy of your data analyses. As you continue your journey with MATLAB, consider diving deeper into its vast array of functions to further refine your data-handling techniques.
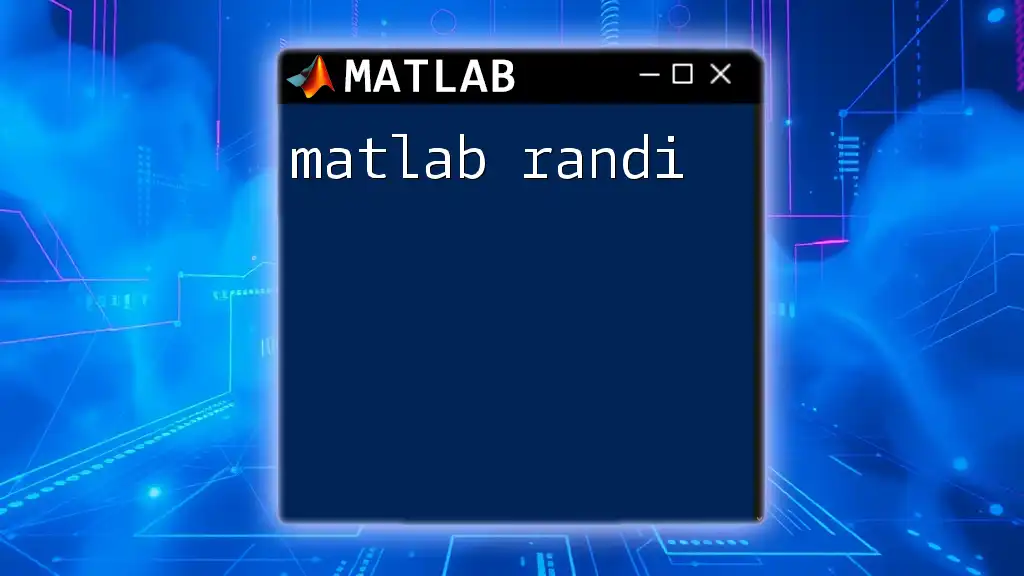
Further Resources
For additional information, review the official MATLAB documentation on isnan and other related functions. Consider participating in online courses or tutorials that focus on data analysis in MATLAB and don’t hesitate to explore recommended books and guides that cover broader programming strategies.