To calculate the distance between two points in MATLAB, you can use the `pdist2` function or the distance formula directly; here’s an example of using the distance formula for points \((x_1, y_1)\) and \((x_2, y_2)\).
% Define the points
point1 = [x1, y1];
point2 = [x2, y2];
% Calculate the distance
distance = sqrt(sum((point1 - point2).^2));
Understanding Euclidean Distance
What is Euclidean Distance?
Euclidean distance is the straight-line distance between two points in Euclidean space. It can be mathematically defined using the Pythagorean theorem. In a two-dimensional space, the distance \( d \) between two points \((x_1, y_1)\) and \((x_2, y_2)\) is calculated using the formula:
\[ d = \sqrt{(x_2 - x_1)^2 + (y_2 - y_1)^2} \]
For three-dimensional space, the formula extends to:
\[ d = \sqrt{(x_2 - x_1)^2 + (y_2 - y_1)^2 + (z_2 - z_1)^2} \]
Understanding this concept is crucial not only in mathematics but also in various real-world applications, including physics, computer graphics, and machine learning.
Visual Representation
Visualizing the Euclidean distance can significantly enhance comprehension. In a Cartesian coordinate system, the two points are represented as coordinates on a graph. Drawing a straight line between the two points illustrates the distance, helping to solidify the conceptual understanding of how distance works in a 2D or 3D space.
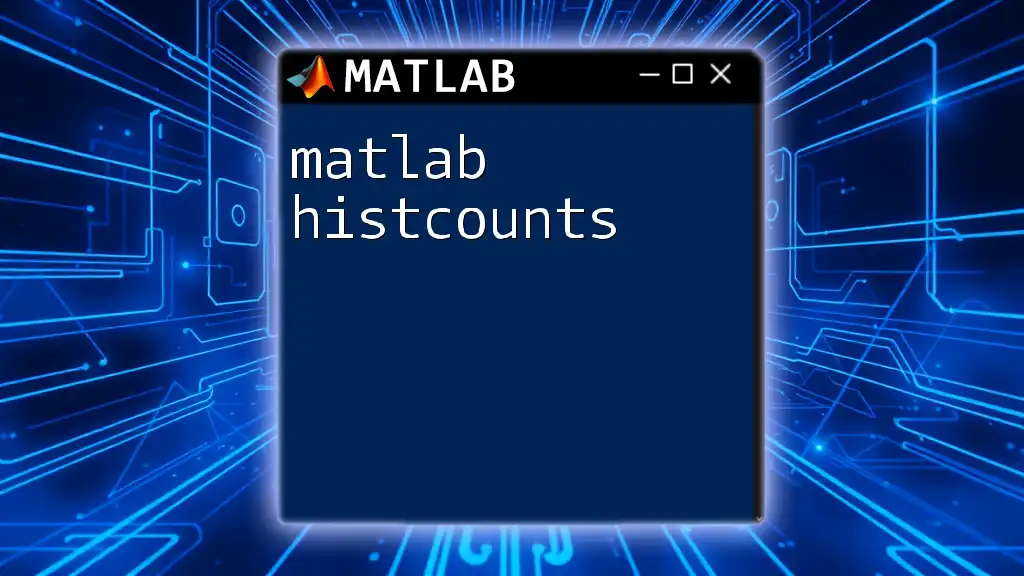
How to Compute Distance in MATLAB
Using Standard MATLAB Functions
MATLAB provides built-in functions that simplify the computation of distance between points. One such function is `pdist`, which can be utilized for a variety of distance calculations between a set of points.
Example of Using `pdist`
To compute the distance between two points, follow this example:
points = [1 2; 4 6]; % Define two points
distance = pdist(points); % Computes the distance
disp(distance);
In this example, the `pdist` function calculates the Euclidean distance between the two defined points. The output of the command will be a single number representing the distance, showcasing how straightforward the process can be.
Custom Distance Function
While built-in functions are efficient, creating custom functions can deepen your understanding and allow for flexibility. Here’s how to write your custom distance calculation function:
Writing `calcDistance.m`
Create a new file named `calcDistance.m`, which contains the following code:
function d = calcDistance(point1, point2)
d = sqrt(sum((point2 - point1) .^ 2));
end
This function takes two points as inputs and calculates the Euclidean distance by applying the formula we discussed earlier.
To use this function, call it as follows:
p1 = [1, 2];
p2 = [4, 6];
d = calcDistance(p1, p2);
disp(d);
When executed, this script will display the distance between points \( p1 \) and \( p2 \), demonstrating the utility and clarity of custom functions in MATLAB.
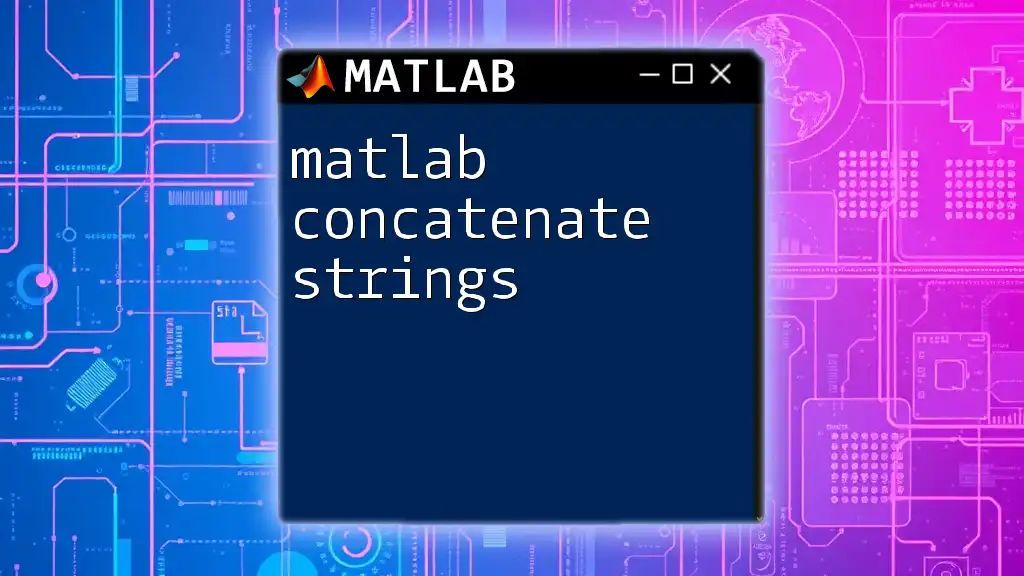
Applications of Distance Calculation
In Data Analysis
Distance calculations are central to numerous data analysis techniques, especially in clustering algorithms, such as K-means. These algorithms classify data points into groups based on their distances to centroids, enabling insights and patterns to emerge from the dataset.
In Robotics
Robotics heavily relies on distance calculations for navigation and obstacle avoidance. When robots assess their surroundings, they calculate distances to various objects to navigate effectively and make decisions to avoid collisions.
In Machine Learning
Distance metrics like Euclidean distance play a pivotal role in machine learning, particularly in classification tasks. They help algorithms determine how closely related different data points are, facilitating effective neighborhood-based methods, such as K-nearest neighbors (KNN).
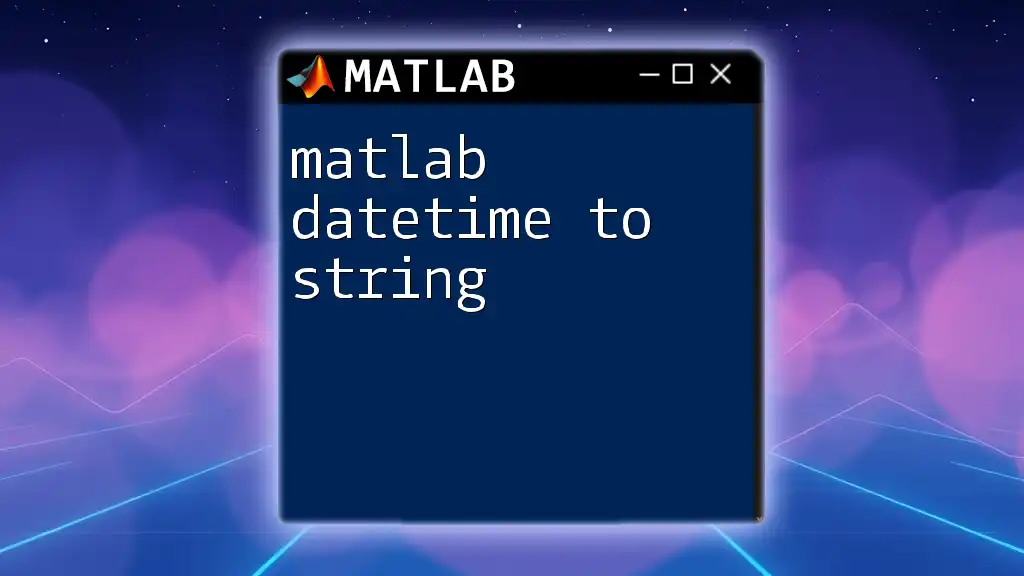
Comparing Different Distance Metrics
Manhattan Distance
Manhattan distance, also known as "taxicab" distance, measures distance by only allowing moves along grid-like paths. The formula is straightforward:
\[ d = |x_2 - x_1| + |y_2 - y_1| \]
Here’s how to implement this in MATLAB:
function d = manhattanDistance(point1, point2)
d = sum(abs(point2 - point1));
end
Chebyshev Distance
Chebyshev distance measures the greatest of the absolute differences along any coordinate dimension. It is useful in certain applications where movement can occur diagonally. The formula looks like this:
\[ d = \max(|x_2 - x_1|, |y_2 - y_1|) \]
To implement this metric in MATLAB, you might write:
function d = chebyshevDistance(point1, point2)
d = max(abs(point2 - point1));
end
Choosing the Right Distance Metric
Selecting the appropriate distance metric depends on the specific requirements of your application. Consider factors such as the data distribution, the nature of the problem (e.g., clustering vs classification), and the dimensionality of the data when choosing between Euclidean, Manhattan, Chebyshev, or other distance metrics.
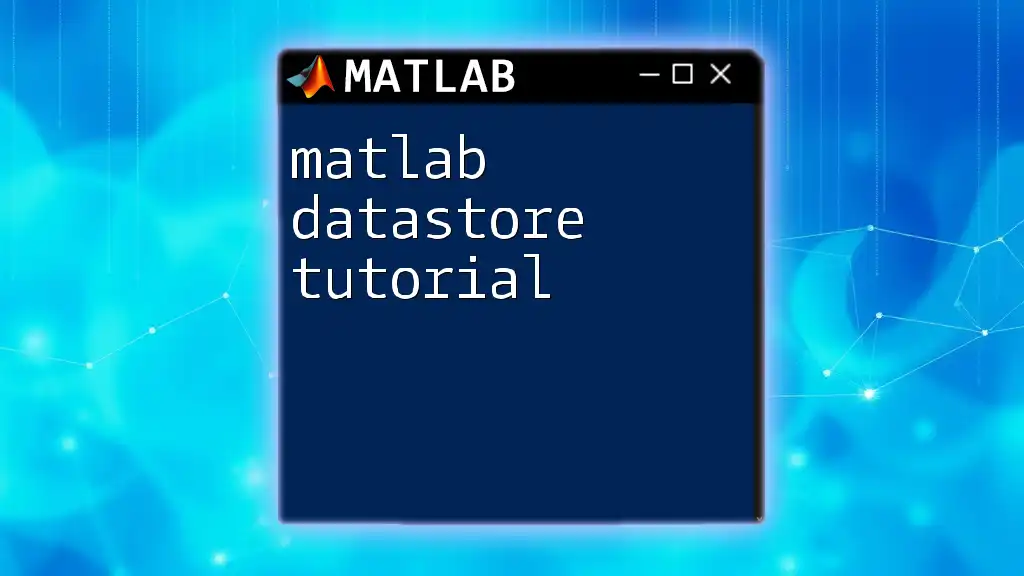
Conclusion
Understanding the MATLAB distance between two points is an essential skill that opens the door to a wide range of applications across fields like data analysis, robotics, and machine learning. By mastering both built-in functions and custom functions, you'll develop a solid grasp of distance calculations and their practical uses. Practice now with the examples and exercises provided, and you'll soon find yourself confident in applying these concepts proficiently in MATLAB.
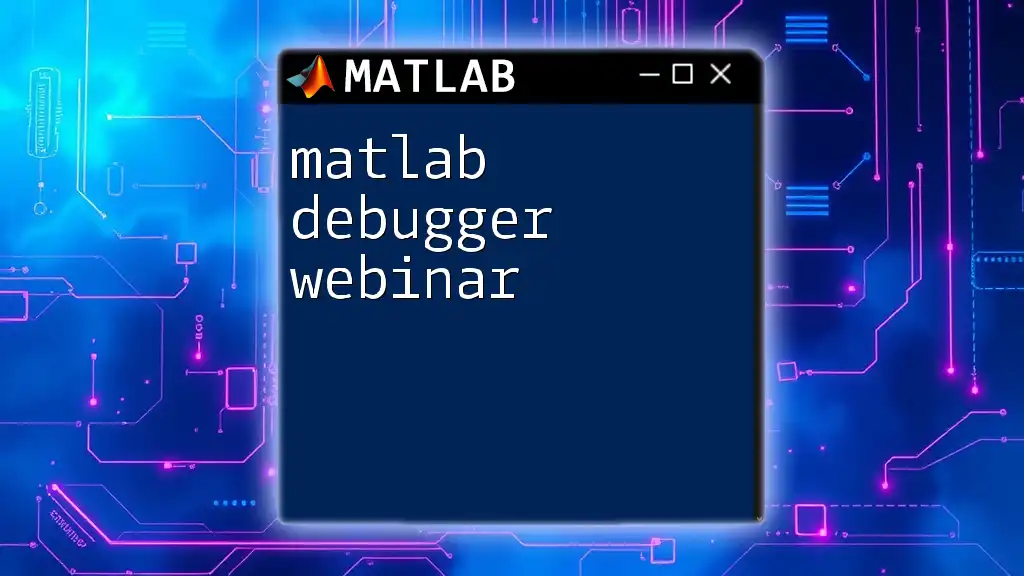
Additional Resources
Recommended Readings
Familiarize yourself with official MATLAB documentation and explore tutorials online to enhance your knowledge further.
Short Quizzes/Exercises
To solidify your understanding, try creating different distance functions for various applications or solving problems that involve calculating distances in diverse datasets.