In MATLAB, you can convert a table to a matrix by using the `table2array` function, which extracts the data from a specified table.
% Example: Convert a table 'T' to a matrix 'M'
M = table2array(T);
Understanding MATLAB Tables
What is a MATLAB Table?
In MATLAB, a table is a data type that allows for storing data in a structured manner, similar to a spreadsheet or a database table. Tables facilitate the storage of different data types together; each column can contain a different type of data (numeric, string, categorical, etc.) while rows represent observations. Compared to matrices, which can only contain data of the same type, tables are more versatile for handling real-world data sets.
One key scenario for using tables is when you're working with heterogeneous data where multiple characteristics need to be represented—like a dataset comprising names, ages, and heights for individuals. For example, while a matrix could only store numerical values, a table could store names as strings alongside these numbers, facilitating better organization and retrieval of data.
How to Create Tables in MATLAB
Creating a table in MATLAB is straightforward. You can utilize the `table` function to combine different data types easily. Here’s how you can create a simple table:
% Example code to create a table
Names = {'John'; 'Mary'; 'Alex'};
Ages = [28; 34; 42];
Heights = [5.9; 5.5; 6.0];
T = table(Names, Ages, Heights);
disp(T);
In this example, we constructed a table named `T` that includes three columns: `Names`, `Ages`, and `Heights`. Each row represents a different individual.
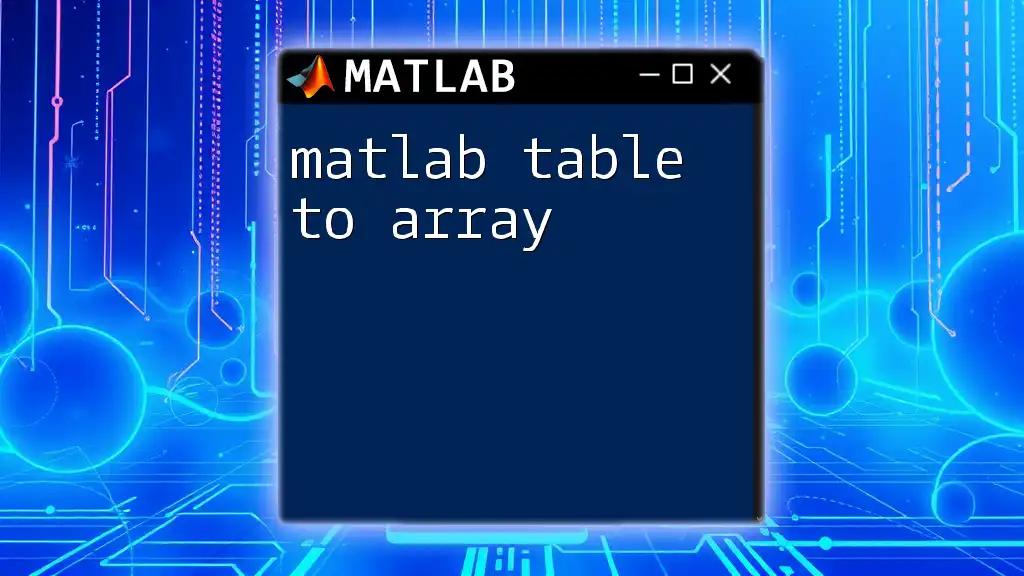
Converting MATLAB Table to Matrix
Why Convert Tables to Matrices?
While tables are useful for organization and data manipulation, there are scenarios where a matrix representation is more practical. For example, when performing mathematical operations such as matrix multiplication or statistical computations, you will often need your data in matrix form. Converting a MATLAB table to a matrix allows for seamless integration into algorithms or functions that expect a matrix input.
Step-by-Step Guide to Conversion
Basic Conversion Using `table2array`
One of the most common functions for converting a table to a matrix in MATLAB is `table2array`. This function transforms the data within the table into a standard numeric matrix.
Here’s a simple example using our previous table `T`:
% Convert table T to a matrix
M = table2array(T);
disp(M);
After running this code, the output will display the underlying numerical data of the table `T`, omitting the row names and column names.
Converting Specific Variables to Matrix
If you're interested in only certain columns from a table, you can specify the desired columns for conversion. For instance, if you only want the `Ages` and `Heights`, here's how you can do it:
% Select specific columns
M_subset = table2array(T(:, {'Ages', 'Heights'}));
disp(M_subset);
This will yield a matrix containing only the numerical values of the selected columns, which is particularly useful for focused analyses.
Handling Different Data Types
Numeric and Non-numeric Data
While converting a table to a matrix, one needs to be cautious about the data types. The `table2array` function expects numeric data; if the table contains non-numeric data (like strings or categorical variables), MATLAB will throw an error upon conversion.
To handle such scenarios, consider converting non-numeric columns to a suitable format before the conversion. You can choose to convert it to a categorical array or exclude those columns entirely if they are not essential for the analysis.
Preserving Table Variable Names
Retaining Column Names
It’s important to note that, unlike tables, matrices do not retain any variable names. If you need context about which data corresponds to which variable after conversion, consider storing the variable names in tandem.
Here’s an example of how to keep track of column names while also storing the matrix:
% Store matrix and names in a cell array
results = [{T.Properties.VariableNames}; num2cell(M)];
disp(results);
In this arrangement, we leverage a cell array to store both the variable names and data, enabling you to reference the original column identifiers post-conversion.
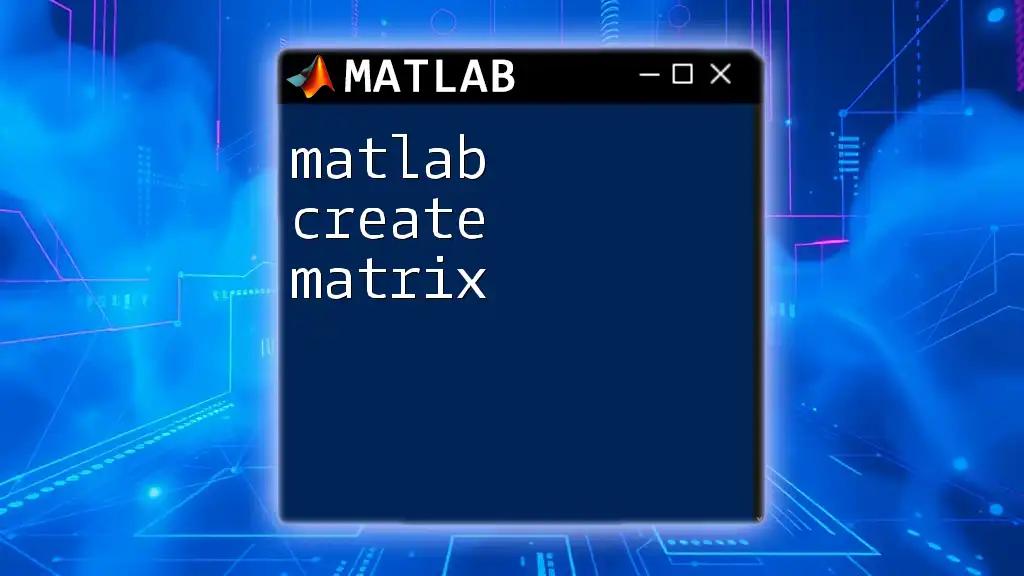
Practical Applications of Converting Tables to Matrices
Data Analysis and Machine Learning
In practical scenarios like data analysis and machine learning, converting tables to matrices is often necessary. Many algorithms, especially in machine learning, are designed to work exclusively with matrices, requiring you to preprocess your data accordingly. For example, before applying a regression model or clustering algorithm, the data typically must be in matrix format.
Mathematical Computations
Once a table has been converted to a matrix, it can be utilized for complex mathematical operations. For instance, consider performing matrix multiplication. Here’s a demonstration of how to do that:
% Example of matrix multiplication
B = [1; 1; 1]; % Another matrix for multiplication
result = M * B;
disp(result);
In this example, `M` represents the matrix derived from the table, and `B` is another matrix. The result will compile the matrix multiplication product, opening further avenues for analytical applications.
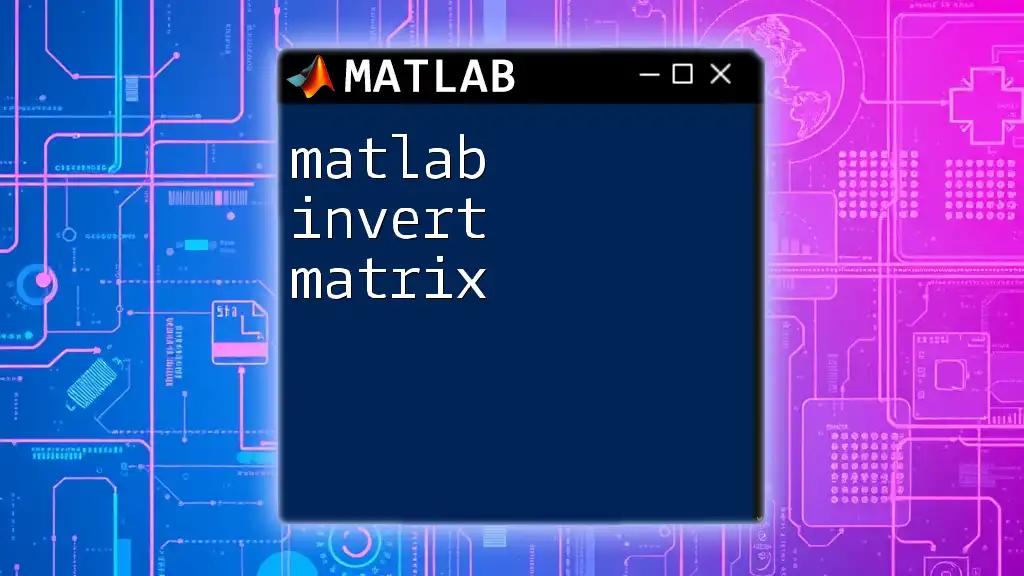
Troubleshooting Common Issues
Errors in Conversion
When working with data conversions, it’s common to encounter errors, particularly when the table contains mixed data types or incompatible structures. Ensure that all columns are numeric if using `table2array`, or pre-process your data to avoid conversion errors.
Performance Considerations
For larger datasets, keep performance in mind. Conversions can be resource-intensive for vast tables; thus, it’s advisable to optimize your code and, if possible, filter the data to only include the necessary variables before converting.
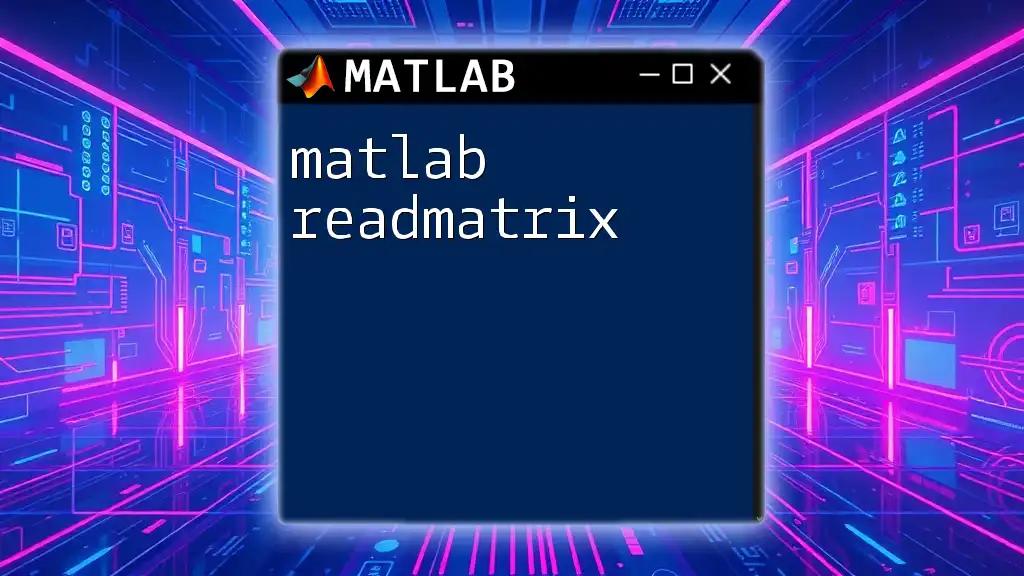
Conclusion
Efficiently converting a MATLAB table to a matrix can vastly improve your data manipulation and analysis capabilities. This guide has walked you through the processes from creation to conversion, ensuring you have the tools needed to integrate tables into your coding practice seamlessly. Get hands-on with MATLAB's tools, and embrace the versatility that table-to-matrix conversion offers in your data analysis journey.