Principal components in MATLAB are used to reduce the dimensionality of datasets while retaining the most significant variations by transforming the original variables into a new set of uncorrelated variables called principal components.
Here’s a simple example to perform PCA (Principal Component Analysis) using MATLAB:
% Load example data
data = rand(100, 5); % Generating random data for demonstration
% Standardize the data
data_meaned = data - mean(data);
% Calculate the covariance matrix
cov_mat = cov(data_meaned);
% Compute the eigenvalues and eigenvectors
[eigenvectors, eigenvalues] = eig(cov_mat);
% Sort the eigenvalues and corresponding eigenvectors
[sorted_eigenvalues, index] = sort(diag(eigenvalues), 'descend');
sorted_eigenvectors = eigenvectors(:, index);
% Select the top k eigenvectors (eigenvector matrix)
k = 2; % Number of principal components
projected_data = data_meaned * sorted_eigenvectors(:, 1:k);
Understanding Principal Components
What are Principal Components?
Principal components are key variables derived from a larger set of variables in such a way that they capture the most significant information from the data while minimizing redundancy. In essence, principal components provide a way to reduce the complexity of data by transforming it into a new coordinate system, where the greatest variance lies along the first coordinate (the first principal component), the second greatest variance along the second coordinate, and so forth.
The importance of principal components is particularly evident in high-dimensional datasets, where visualizing and interpreting data can become challenging. By employing PCA, analysts can achieve a representation of the data that highlights its essential features without overwhelming complexity.
The Mathematical Foundation of PCA
At the heart of PCA lies its mathematical foundation. To comprehend principal components, one must understand:
-
Covariance Matrices: These matrices represent how much variables vary together. A covariance matrix reveals the relationships among your data's features, enabling the identification of patterns.
-
Eigenvalues and Eigenvectors: When performing PCA, we calculate the covariance matrix of the data and then derive the eigenvalues and eigenvectors. Each eigenvalue indicates the amount of variance captured by its corresponding eigenvector, which shows the direction of the data spread.
Understanding these concepts is crucial, as they dictate how PCA discerns the structure in the data. The eigenvector with the highest eigenvalue becomes the first principal component, representing the maximum variance in the data.
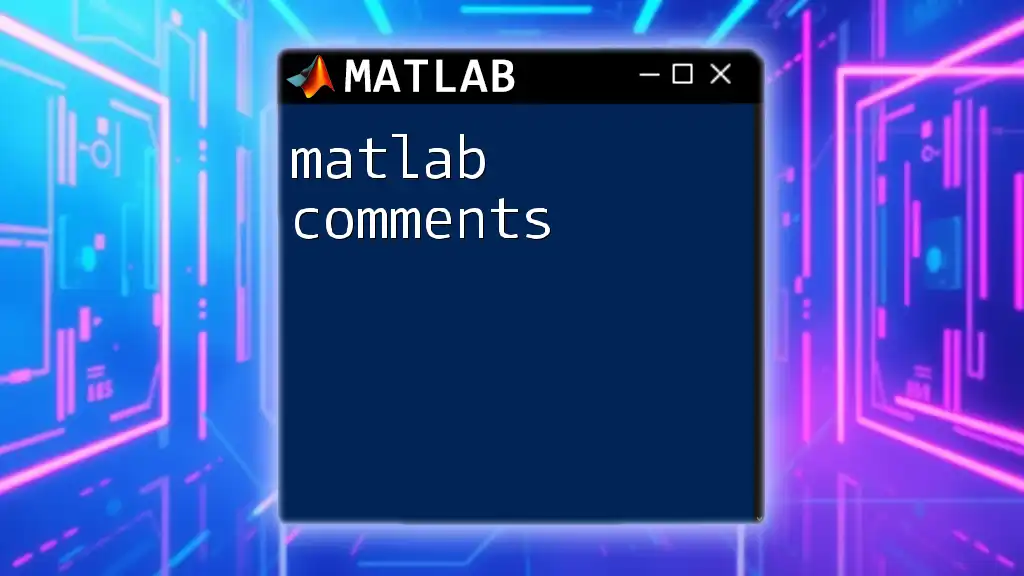
Setting Up Your Data in MATLAB
Preparing Your Dataset
Before you can begin applying PCA, it’s critical to prepare your dataset adequately. One essential step is data normalization. Raw data often has different units or scales across features, which can skew results. Normalization ensures that each feature contributes equally to the analysis.
In MATLAB, you can normalize your data using the following command:
normalized_data = zscore(data);
This command standardizes your dataset to have a mean of zero and a standard deviation of one. By achieving normalization, you set the stage for accurate PCA results.
Creating a Covariance Matrix
Once your data is normalized, the next step is calculating the covariance matrix. The covariance matrix reveals how each variable's variations relate to one another.
In MATLAB, you can generate a covariance matrix using:
covariance_matrix = cov(normalized_data);
This code snippet produces a matrix where each element represents the covariance between pairs of variables. Understanding the covariance structure of your data is foundational for the subsequent PCA process.
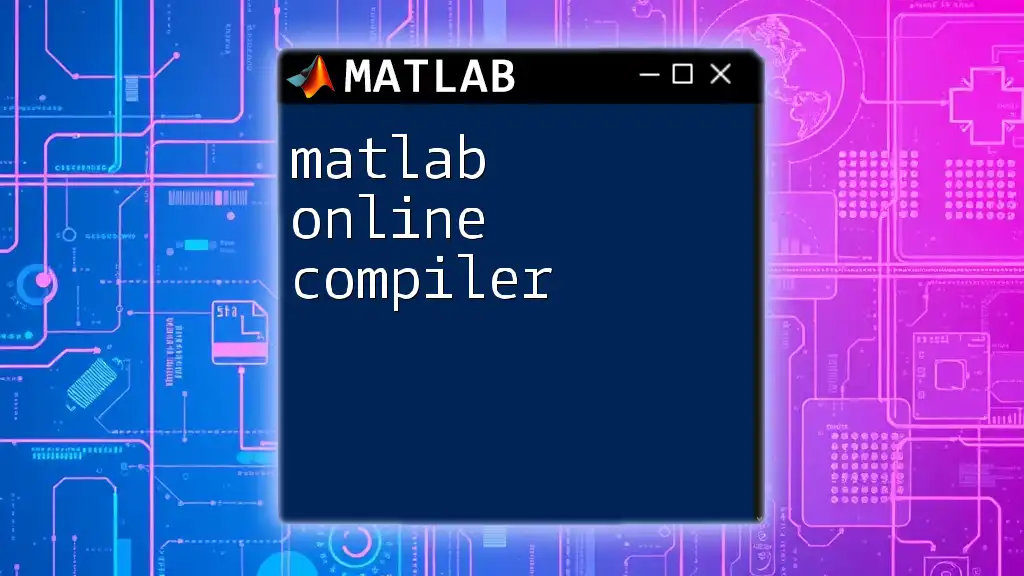
Performing PCA in MATLAB
Using the `pca` Function
MATLAB simplifies PCA with its built-in `pca` function, allowing you to efficiently carry out the analysis with less complexity. The function outputs three key components:
- Scores: the principal component scores for each observation in your dataset.
- Coefficients: the principal component coefficients, showing the contribution of each original variable.
- Latent: the eigenvalues associated with each principal component, indicating the amount of variance each captures.
An example of using the `pca` function is as follows:
[coeff, score, latent] = pca(normalized_data);
Using this single line of code, you can access all the essential outputs of PCA. Analyze the results by examining `latent` for the variance explanation by each component, and use `score` for visualization.
Custom PCA Implementation
While using the built-in function is efficient, understanding how to implement PCA manually enhances your grasp of the underlying algorithms. Performing PCA manually involves several steps:
- Calculate the covariance matrix (as shown above).
- Obtain eigenvalues and eigenvectors:
[eigenvectors, eigenvalues] = eig(covariance_matrix);
-
Sort the eigenvalues in decreasing order and select corresponding eigenvectors.
-
Form a feature vector using selected eigenvectors, which can then be used to reorient your dataset:
feature_vector = eigenvectors(:, end-k+1:end); % For k principal components
- Project the data onto the new space:
pca_result = normalized_data * feature_vector;
This step-by-step methodical approach to PCA empowers you to visualize and comprehend the process behind dimensionality reduction better.
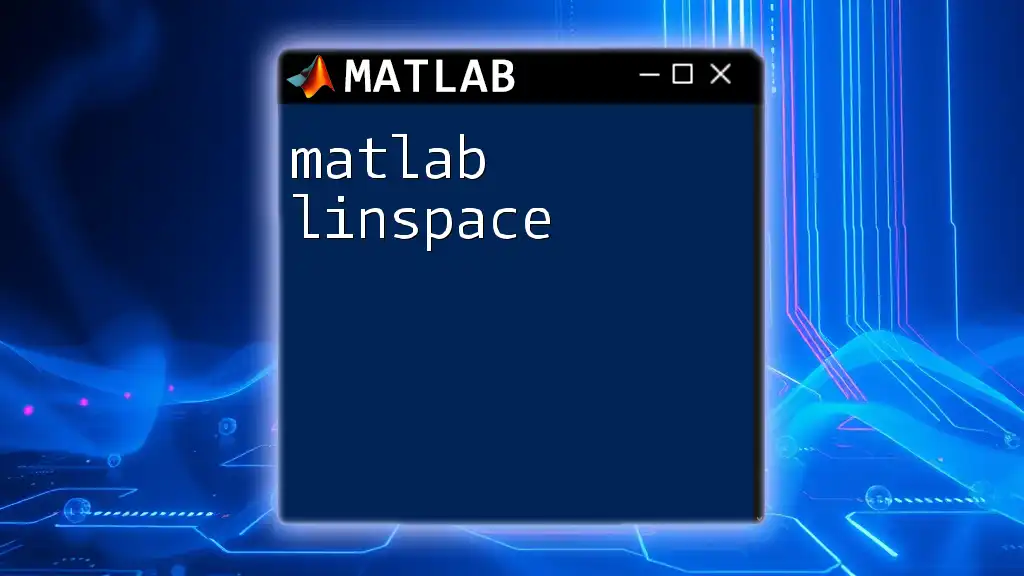
Visualizing Principal Components
Plotting PCA Results
Visual representation plays a crucial role in PCA. By plotting the principal components, you gain insights into underlying patterns, trends, and clusters within the data. In MATLAB, you can use the following code snippet to create a scatter plot of the first two principal components:
scatter(score(:,1), score(:,2));
title('PCA: First Two Principal Components');
xlabel('Principal Component 1');
ylabel('Principal Component 2');
This creates a visual understanding of how your data points relate to one another in the context of the reduced dimensions.
Interpretation of PCA Plots
When interpreting PCA scatter plots, look for clusters or patterns that suggest relationships among the data points. Concentrated clusters may indicate groups that share similarities, whereas outliers may reveal unique cases. Understanding these visual patterns can guide further analysis or decision-making.
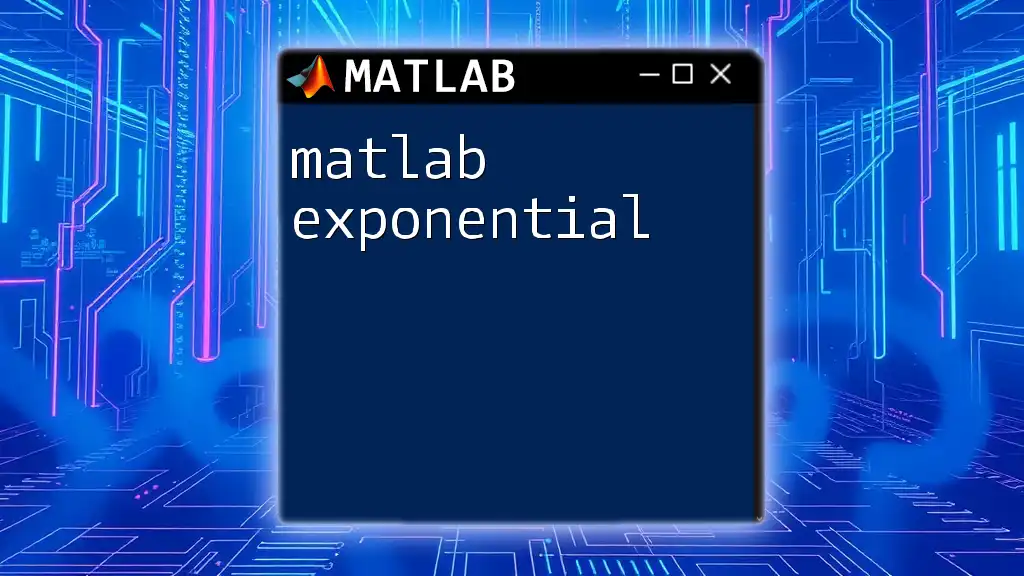
Applications of PCA
Use Cases of PCA in Real-world Scenarios
PCA has extensive applications across various fields. For example, in finance, PCA aids in risk management by summarizing asset returns into fewer dimensions. In image processing, it simplifies image databases by capturing variations in pixel intensity.
An illustrative example of PCA in facial recognition can involve compressing the dataset to improve the speed and efficiency of recognition algorithms. This operation can look like:
% Assuming 'face_images' is a matrix with pixel data
[coeff_faces, score_faces, latent_faces] = pca(face_images);
Integrating PCA with Other Techniques
Combining PCA with machine learning can yield powerful insights. For instance, after reducing dimensions with PCA, you can apply clustering algorithms like k-means to identify patterns.
% Implementing k-means on PCA-reduced data
k = 3; % Number of clusters
[idx, C] = kmeans(score(:,1:2), k);
This method enhances clarity and understanding of segmentations within your dataset, making subsequent analyses more manageable.
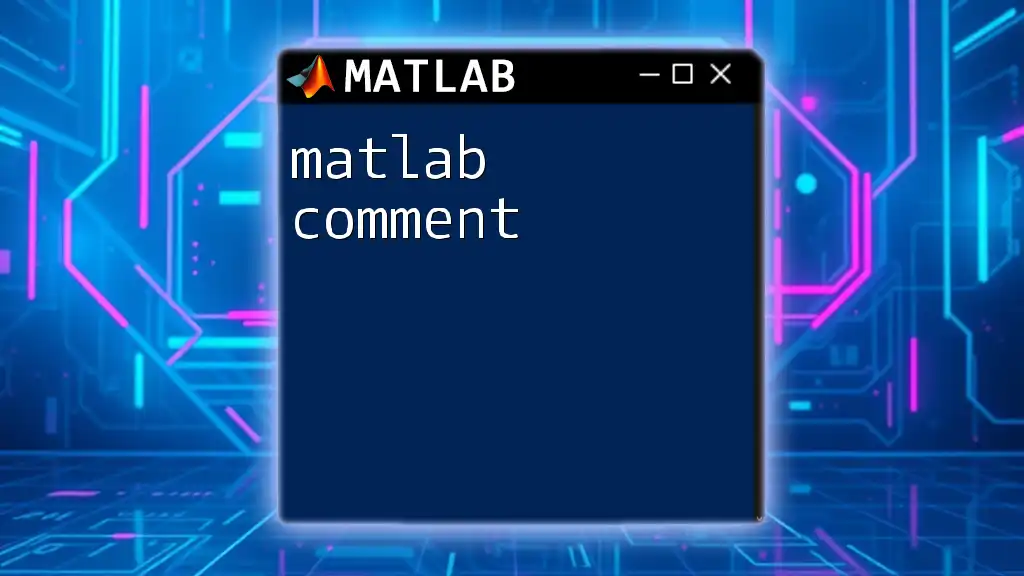
Best Practices for Using PCA in MATLAB
Choosing the Right Number of Components
Determining the appropriate number of principal components is crucial. A common method is to plot the Scree Plot, which visualizes the eigenvalues in descending order. The point where the plot levels off suggests the optimal number of components:
plot(latent);
title('Scree Plot');
xlabel('Principal Component');
ylabel('Eigenvalue');
Handling Limitations of PCA
Despite its strengths, PCA is not without limitations. It assumes linear relationships in data and may struggle with certain types of data distributions. To mitigate these concerns, consider applying Kernel PCA or exploring other dimensionality reduction methods like t-SNE for non-linear structures.
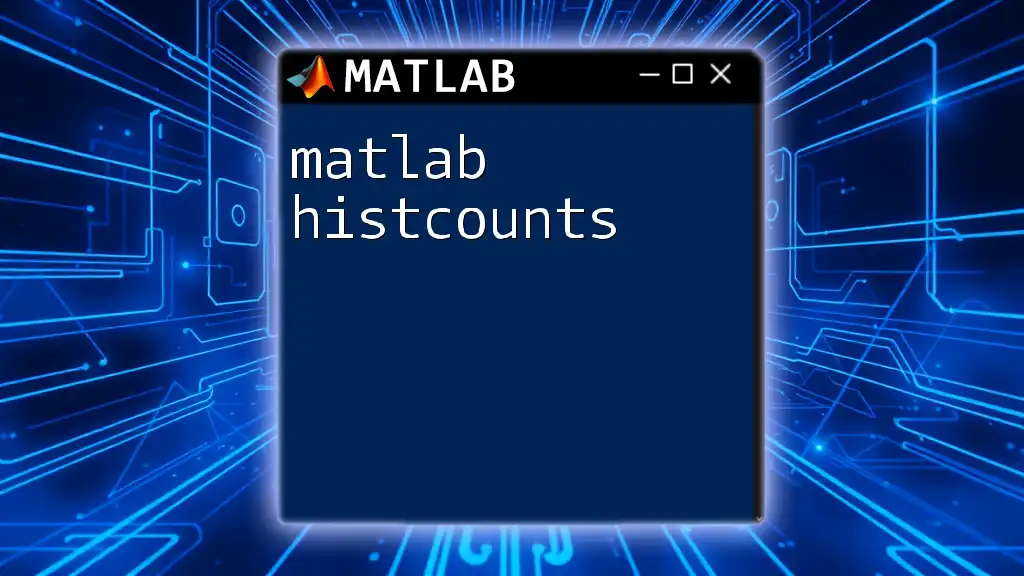
Conclusion
Mastering MATLAB principal components is foundational for anyone involved in data analysis. By understanding PCA—from its mathematical underpinnings to its practical applications—you can unlock the full potential of your datasets, simplifying complex data while maintaining its essence. Whether you're reducing dimensionality for further analysis or improving visualizations, mastering MATLAB principal components can significantly enhance your analytical capabilities.
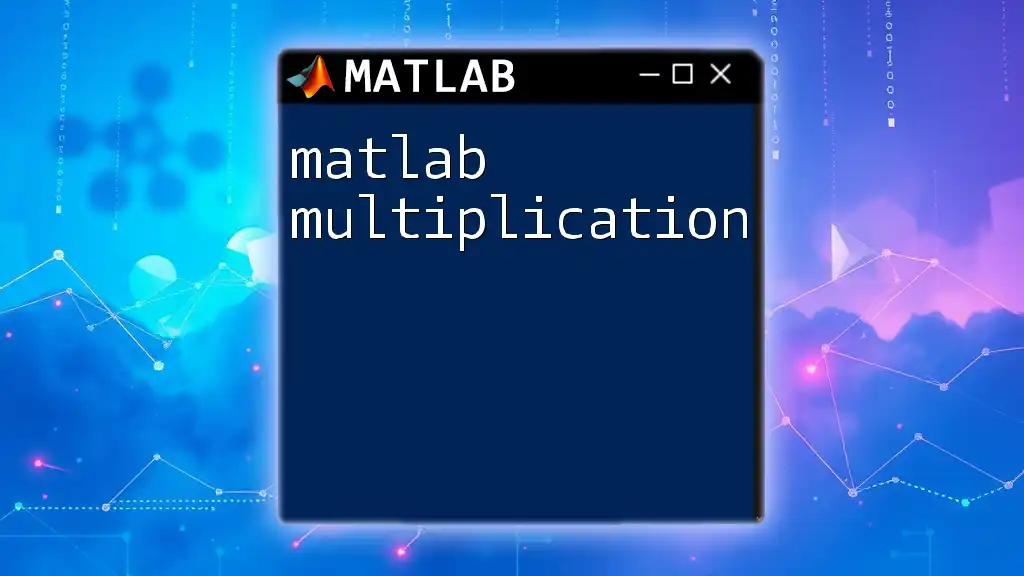
Further Reading and Resources
Recommended MATLAB Documentation and Tutorials
To deepen your understanding, refer to the official [MATLAB PCA documentation](https://www.mathworks.com/help/stats/pca.html) for detailed explanations and examples.
Suggested MATLAB Toolboxes and Add-Ons
Explore other MATLAB toolboxes that complement PCA, such as the Statistics and Machine Learning Toolbox for advanced features and functions related to PCA, feature selection, and further data analysis techniques.