In MATLAB, you can convert a `datetime` object to a string using the `datetime` function with the `Format` property to specify the desired date string format.
Here's a code snippet demonstrating this:
dt = datetime('2023-10-25'); % Create a datetime object
str = datestr(dt, 'yyyy-mm-dd'); % Convert datetime to string
Understanding the Datetime Data Type
What is Datetime?
The datetime data type in MATLAB is specifically designed to represent date and time in a precise and easy-to-use format. Unlike other representations such as datevec or datenum, which can be cumbersome, the datetime object allows for a more intuitive approach with added functionalities. It handles time zone information and can represent dates from different calendars seamlessly.
Creating a Datetime Object
Creating a datetime object is simple. You can use various constructors depending on your needs. Here is a basic example:
dt = datetime('2023-10-15 14:30:00');
This command creates a datetime object representing October 15, 2023, at 14:30. You can also create datetime objects using different formats or even from string arrays that follow specific patterns.
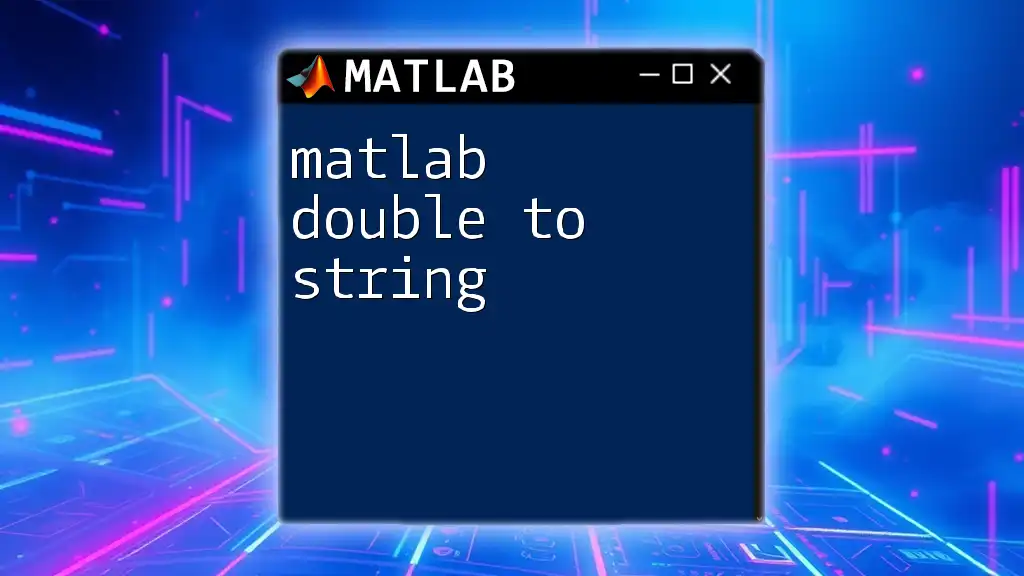
Converting Datetime to String
Basic Conversion Using `datestr`
To convert a datetime object into a string, the datestr function is often used. This function allows you to get a string representation of the datetime in a standard format.
For example, consider the following code:
dt_str = datestr(dt);
This conversion uses MATLAB's default formatting to return the string "15-Oct-2023 14:30:00". Understanding the default format is helpful, but often customization is necessary.
Customizing String Format
Format Specifiers
When you want control over the output format, the datestr function supports format specifiers. These specifiers allow you to specify exactly how you want various components of the date and time to appear.
- 'yyyy' for the full year
- 'MM' for the month
- 'dd' for the day
- 'HH' for hours in 24-hour format
- 'mm' for minutes
- 'ss' for seconds
Using these specifiers, you can create strings that suit your formatting needs.
Code Example: Custom Date Format
To illustrate custom formatting, take a look at the following example where we format the datetime string to show the date in a different structure:
dt_str_custom = datestr(dt, 'dd-mm-yyyy HH:MM:ss');
The output here will be "15-10-2023 14:30:00". This format is especially useful for data logging or when preparing logs for readability.
Using String Formatting Functions
`datetime` with Custom Format
MATLAB also offers functionality to transform datetime objects into strings using different approaches. You can create a string formatted exactly as you wish, utilizing the char function:
dt_str_format = char(dt, 'yyyy-MM-dd HH:mm:ss');
This will yield the result "2023-10-15 14:30:00". This is particularly useful when you want to output datetime directly in a specific format without additional functions.
Performance Considerations
When working with large datasets, performance can become crucial. Converting arrays of datetime objects might slow down your processes, especially if done repeatedly within loops. To enhance the efficiency of datetime string conversion, consider:
- Using vectorized operations when possible
- Minimizing repetitive calls to functions by storing intermediate results
- Evaluating your design: sometimes, maintaining datetime objects for calculations might be more advantageous than converting them multiple times.
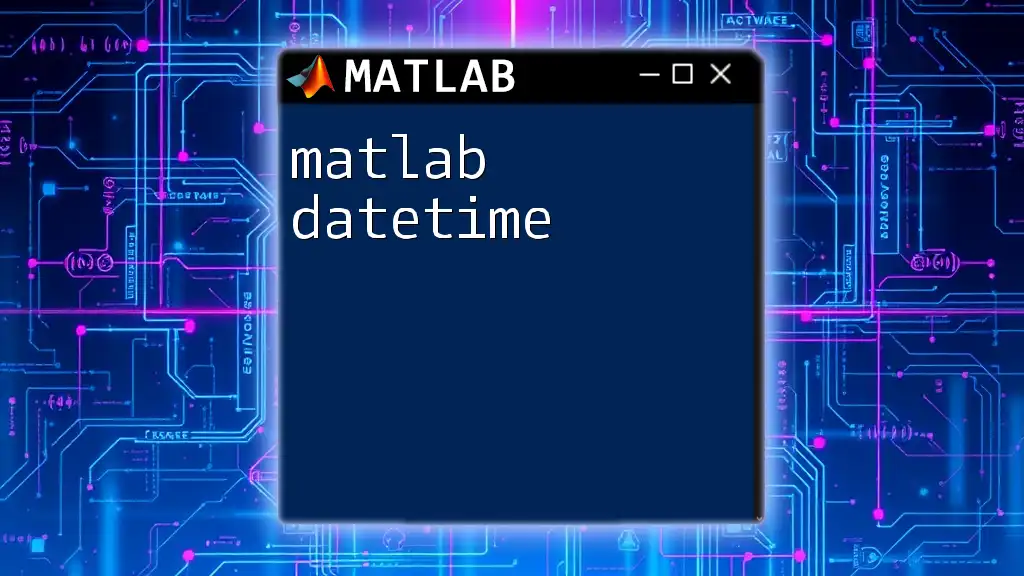
Practical Use Cases
Use Case: Preparing Data for Visualization
When visualizing data, you might find it necessary to convert datetime objects to strings for labeling axes. Here's how you can incorporate the datetime strings into a plot:
plot(dt, rand(1, 10)); % where dt is your datetime array
xlabel('Date and Time');
ylabel('Random Values');
This visualization becomes clearer when the x-axis has properly formatted datetime strings that are easily readable.
Use Case: Exporting Data to Files
Another common use case for converting datetime to strings is exporting data. For instance, when writing a table to a CSV file, having datetime in string format is essential for clarity:
writetable(table(dt_str), 'data.csv');
This approach ensures that anyone reading the CSV file can quickly understand the date and time information without parsing complex formats.
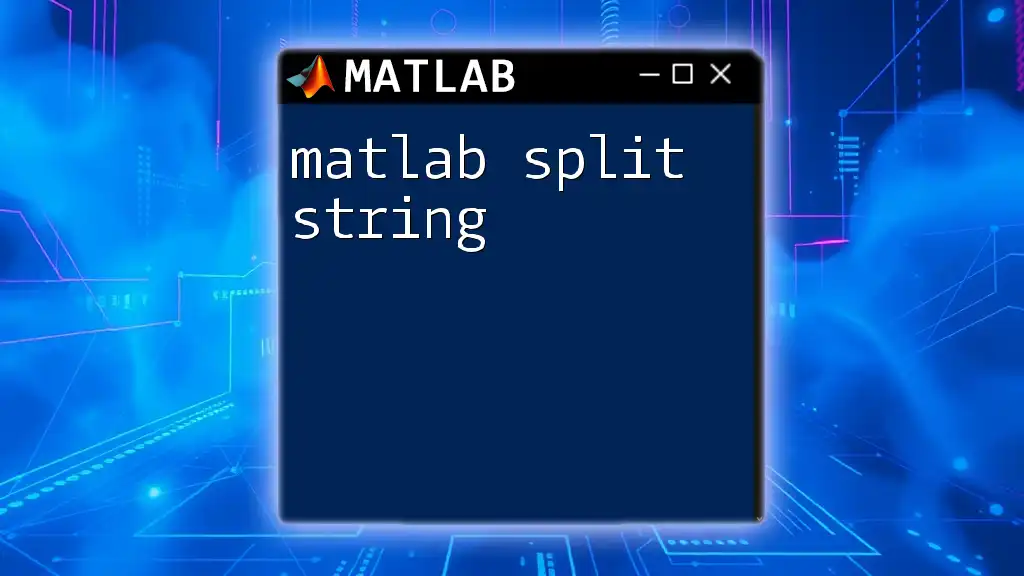
Troubleshooting Common Issues
Handling Time Zones
Time zones can complicate datetime conversion significantly. Depending on your application, you might need to account for UTC, or any specific local time zones. Ensure your datetime objects are properly initialized with timezone information to avoid discrepancies.
dt_tz = datetime('now', 'TimeZone', 'local');
This code creates a datetime object that reflects the local time zone appropriately, making any conversion to string much more reliable.
Format Errors and Exceptions
When converting datetime to strings, you may encounter format errors. Common warnings include incorrect format strings or ambiguous date representations. When debugging, ensure you carefully check:
- The provided format specifiers
- The datetime object's integrity (valid range, properties)
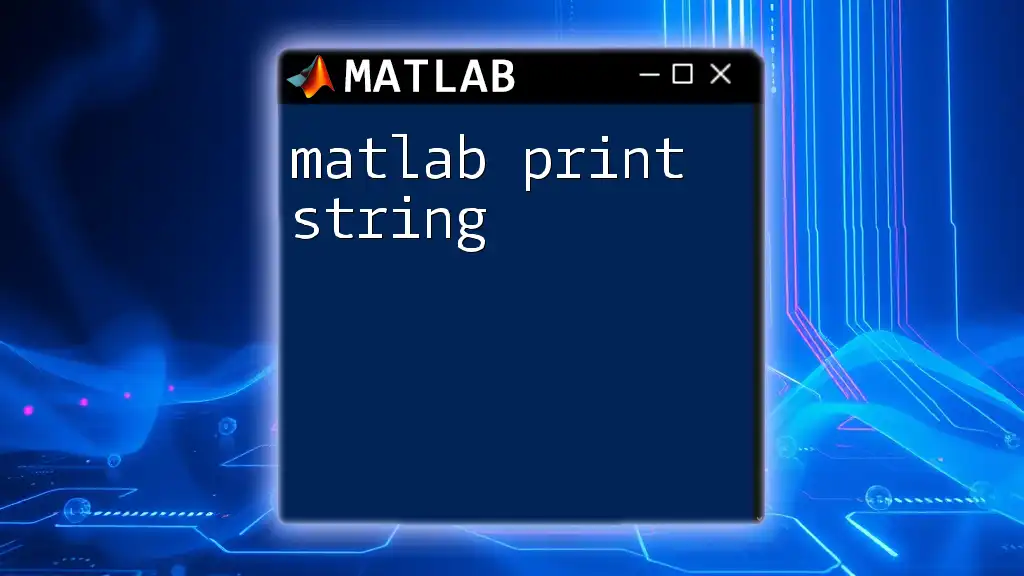
Best Practices
When dealing with matlab datetime to string operations, follow these guidelines for optimal performance:
- Prefer using the datetime format over simple strings when performing calculations or comparisons.
- Only convert to strings when necessary, especially when preparing outputs or logs.
- Familiarize yourself with the various format specifiers to enhance your control over string outputs.
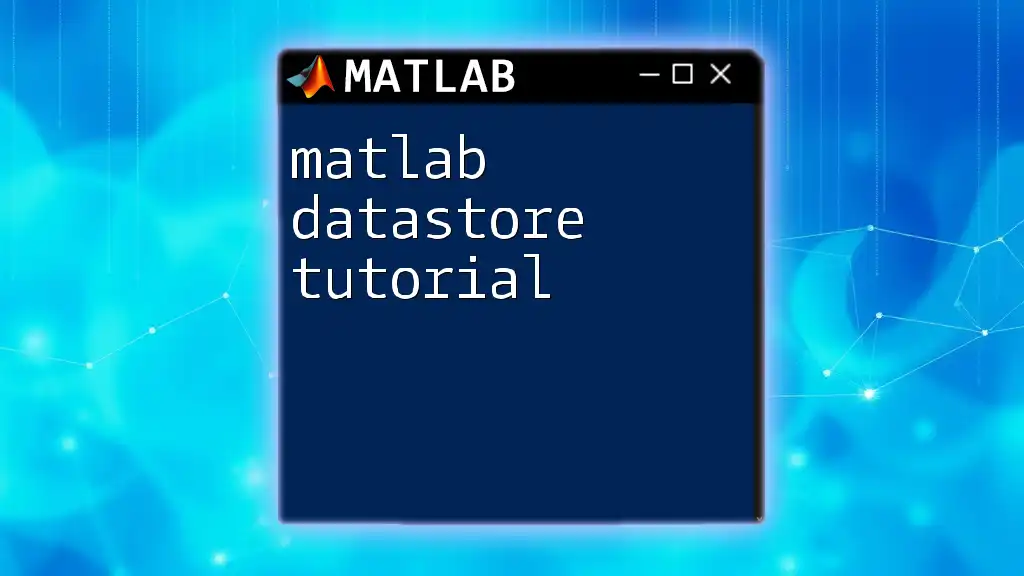
Conclusion
Understanding how to effectively convert matlab datetime to string is a powerful skill that enhances data handling and presentation. Mastering functions like datestr and leveraging format specifiers allows you to present your data clearly and efficiently. Be sure to practice these techniques in real-world scenarios to solidify your knowledge.
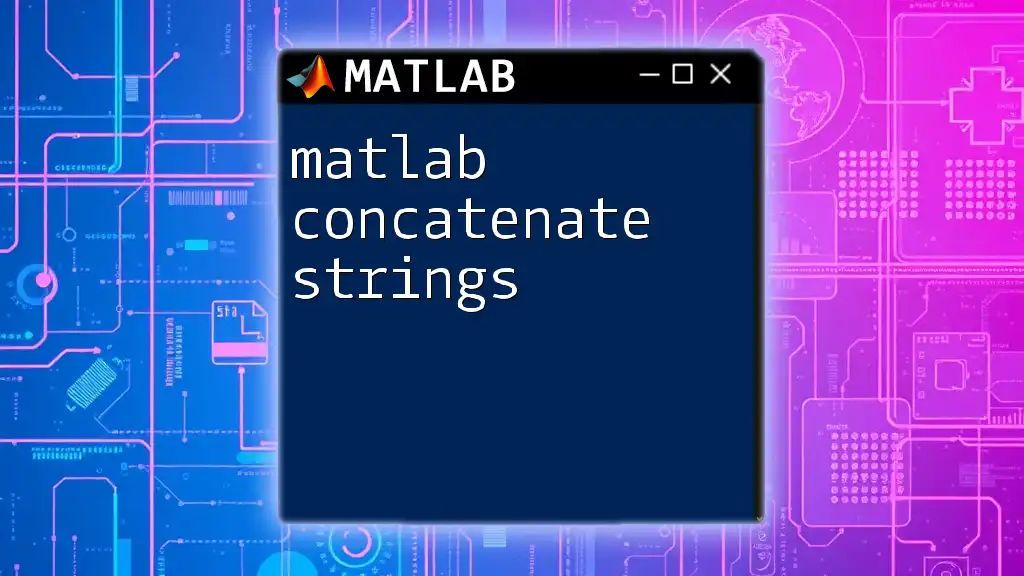
Additional Resources
For more detailed information, consider referring to the official MATLAB documentation on datetime and string functions. Exploring additional textbooks or online courses can also deepen your understanding of MATLAB functionalities.
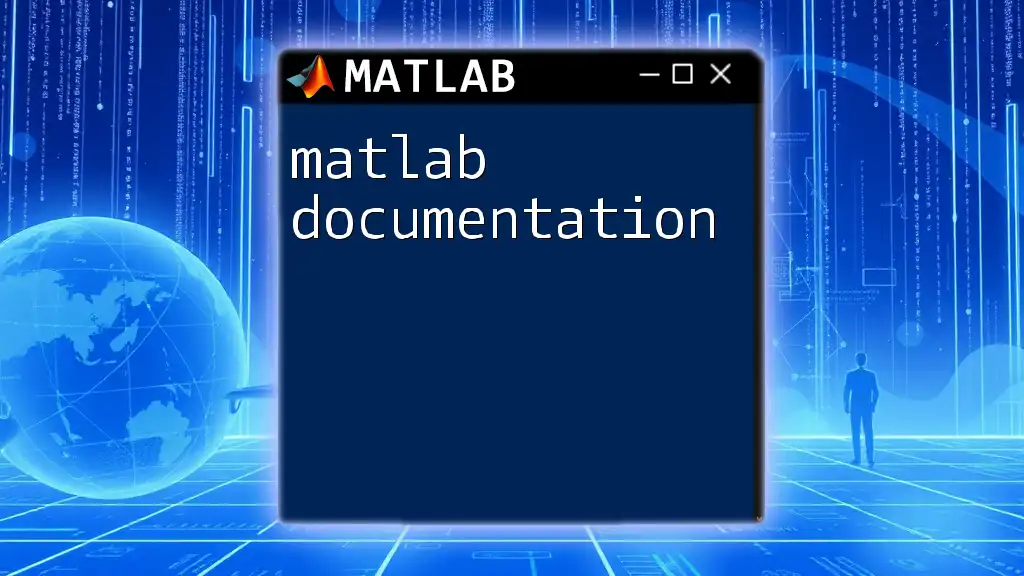
FAQs
What is the difference between `datestr` and `datetime`?
The `datestr` function is used to convert datetime objects to strings, while datetime functions deal with creating and manipulating datetime objects.
Can I convert an array of datetimes to strings at once?
Yes, you can pass an array of datetime objects to `datestr` to receive an array of strings in return.
What to do if my datetime object includes timezone info?
Ensure the conversion is aware of the time zone by utilizing the appropriate parameters in the conversion functions. Always verify that the resulting string accurately reflects the expected local or UTC time.