In this post, we will demonstrate how to import data from an Excel file into MATLAB and create a simple plot of the imported data.
% Import data from Excel and plot it
data = readtable('data.xlsx'); % Replace 'data.xlsx' with your file name
plot(data.Time, data.Value); % Adjust 'Time' and 'Value' to your column names
xlabel('Time'); % Label for x-axis
ylabel('Value'); % Label for y-axis
title('Plot of Value vs Time'); % Title for the plot
grid on; % Optional: add grid
Understanding Excel Data Formats
Common Excel File Formats
When working with Excel data, it's crucial to understand the differences between file formats. The two most common formats you might encounter are .xls and .xlsx. The .xls format is an older version that supports a maximum of 65,536 rows and 256 columns. In contrast, .xlsx allows for a significantly larger dataset, accommodating up to 1,048,576 rows and 16,384 columns.
Additionally, in certain cases, you might come across CSV (Comma-Separated Values) files. These files are particularly useful for managing datasets with no complex formatting and are often utilized to transfer data between different software applications.
Structuring Your Excel Data
For successful data import into MATLAB, ensuring that your Excel data is well-structured is essential. A well-organized dataset typically consists of:
- Headers: First row containing descriptive column names.
- Data Types: Mixing numeric and text data should be avoided in the same column.
An example of a properly structured dataset might look like this:
Column1 | Column2 | Column3 |
---|---|---|
1 | 4.5 | Text1 |
2 | 8.2 | Text2 |
3 | 6.1 | Text3 |
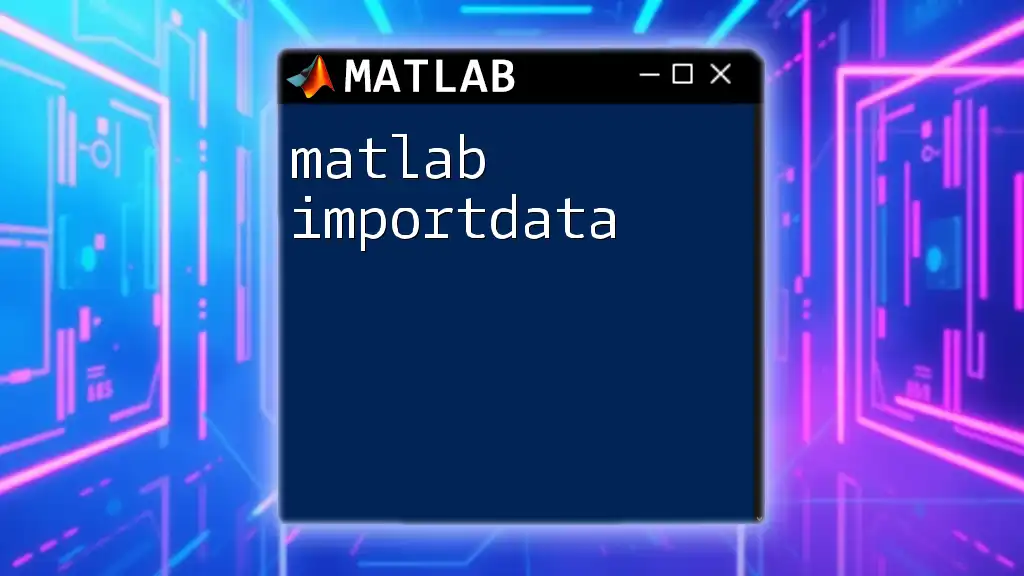
Importing Excel Files into MATLAB
Using `readtable`
The `readtable` function is the most efficient method for importing Excel data into MATLAB. It reads the data into a table format, making it easy to manipulate and analyze.
To import an Excel file, simply use the following command:
data = readtable('data.xlsx');
When this command executes, it retrieves all data, converting it directly into a MATLAB table. The headers from the first row of the Excel file become the variable names in the table.
Using `xlsread` (Prior to R2020a)
If you're using an older version of MATLAB, you might prefer the `xlsread` function. Here's how it can be used:
[num, txt, raw] = xlsread('data.xlsx');
- num: Contains numeric data.
- txt: Contains text data.
- raw: Contains all data in a cell array format.
This function is useful for users who need more control over data types but is less flexible than `readtable`.
Handling Excel Sheets
Both `readtable` and `xlsread` allow you to specify which sheet to import and the range of data. For example, using `readtable`, you can denote the specific sheet and range like this:
data = readtable('data.xlsx', 'Sheet', 'Sheet1', 'Range', 'A1:C10');
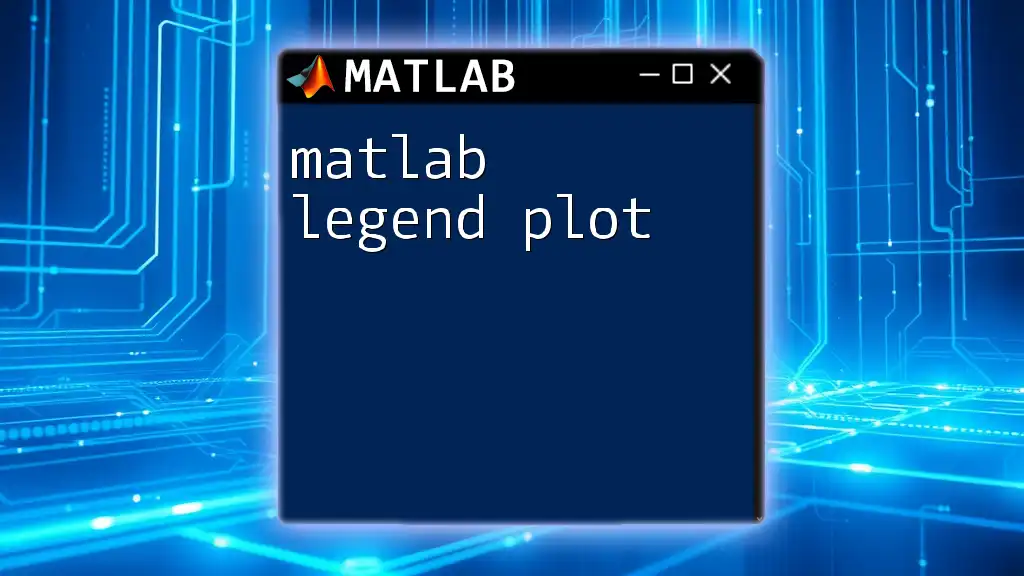
Processing Imported Data
Data Cleaning
After importing your data, it’s essential to clean it. MATLAB provides the `rmmissing` function to remove any rows containing NaN values. Here is how you can apply it:
cleanData = rmmissing(data);
By doing this, you ensure that your dataset is free from any missing values that could skew your analysis or plotting.
Accessing Specific Data
To access specific columns or rows from your imported dataset:
x = cleanData.Column1; % Accessing data from 'Column1'
y = cleanData.Column2; % Accessing data from 'Column2'
This snippet shows how to retrieve data from particular columns for further processing or plotting.
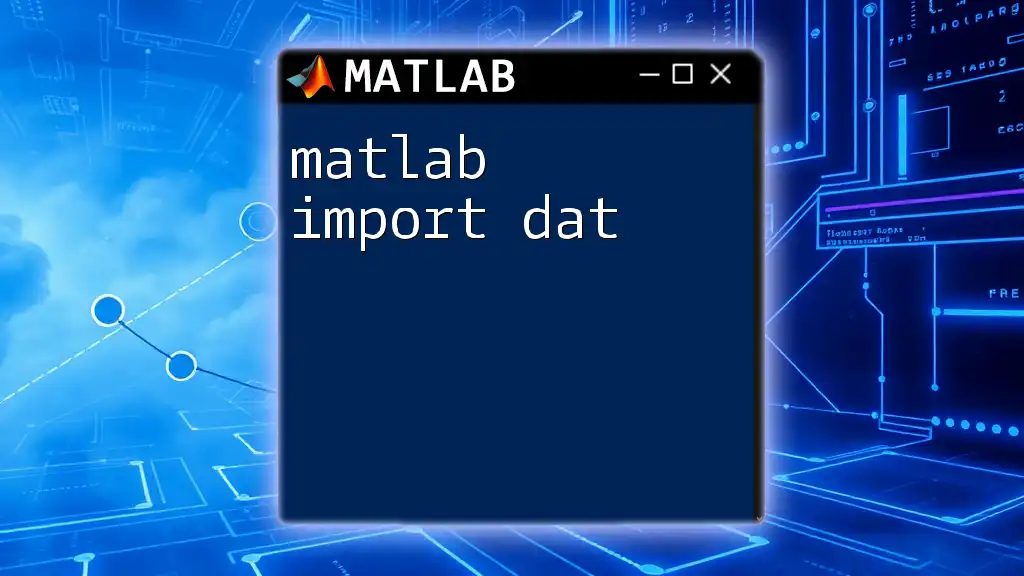
Plotting Data with MATLAB
Basic Plotting with `plot()`
Creating a simple 2D plot in MATLAB is straightforward. Here is a basic example:
plot(x, y);
title('My Data Plot');
xlabel('X-axis Label');
ylabel('Y-axis Label');
grid on;
This command generates a standard line plot with a title, x-label, and y-label for clarity.
Customizing Plots
Customization is key to enhancing the interpretability of your plots. You can add legends, titles, and labels easily:
legend('Data Series 1');
Incorporating legends becomes particularly useful when comparing multiple datasets.
Advanced Plotting Techniques
If you want to create scatter plots instead of line plots, you can use the `scatter` function:
scatter(x, y, 'filled');
Scatter plots are effective for displaying relationships between two variables, especially when looking for correlations or trends.
Saving Plots
After creating your plots, it’s often useful to save them for future reference. You can save a plot with the `saveas` function:
saveas(gcf, 'my_plot.png');
This command saves the current figure as a PNG file, making it easy to share or include in reports.
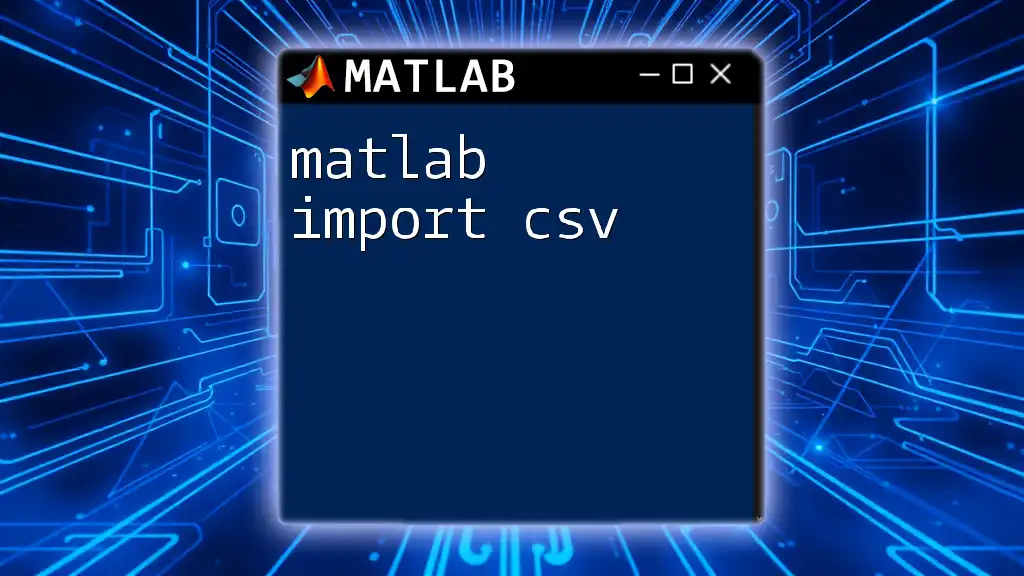
Common Pitfalls and Troubleshooting
Issues with Data Types
It's common to encounter issues related to data types when importing Excel files. If numeric values are stored as text in Excel, you may need to convert them using `str2double`. Here's a quick way to ensure your data is in the right format:
numericData = str2double(cleanData.Database_Column);
Import Errors
When dealing with large datasets, it's essential to handle potential errors during importation. You can use a try-catch block:
try
data = readtable('data.xlsx');
catch ME
disp(ME.message);
end
This approach helps catch and display error messages, allowing you to troubleshoot effectively.
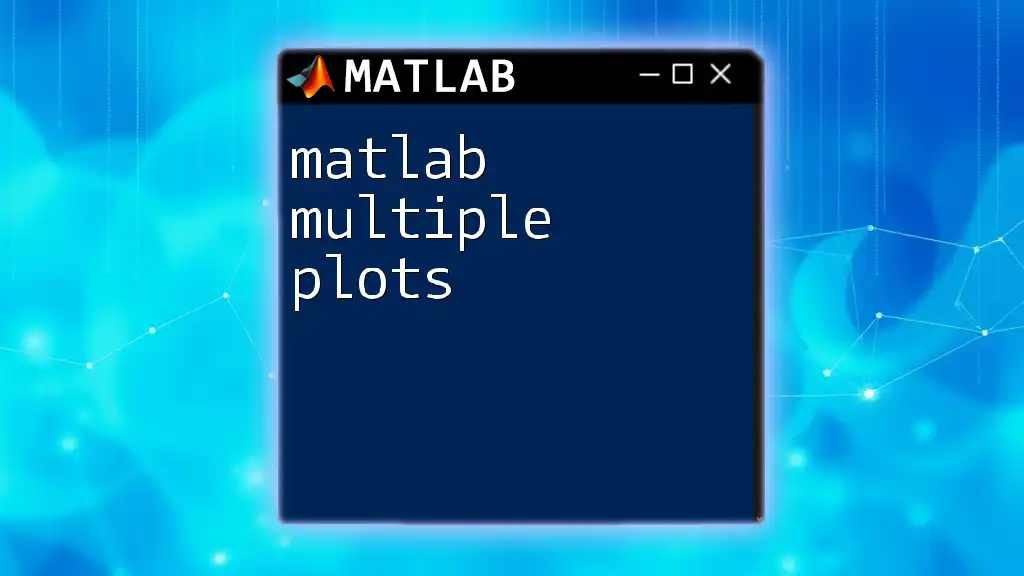
Conclusion
By mastering the process of MATLAB import Excel and plot, you empower yourself to handle data analysis tasks with ease. From importing and processing data to visualizing it through various types of plots, these skills are invaluable across scientific and engineering fields.
Embark on your journey to become proficient with MATLAB by practicing these techniques and exploring more advanced topics available in our future trainings.